OpenStack Client Tools
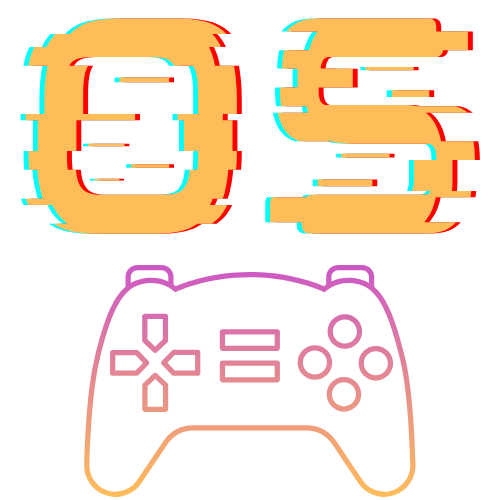
Welcome to the world of next generation OpenStack client tools written in Rust.
As a programming language Rust is getting more and more traction in the low level programming. It has very unique programming safety features what makes it a very good fit in a complex world of OpenStack. As a compiled language it is also a very good fit for the CLI tools allowing users to escape the python dependency issues. In the containerization era placing a small size binary is so much easier.
Current focus of the project is at introducing Rust as a programming language into the ecosystem of OpenStack user facing tooling and provides SDK as well as CLI and TUI.
Design principles
After long time maintaining OpenStack client facing tools it became clear that it cannot continue the same way as before. OpenStack services are very different and are not behaving similar to each other. There were multiple attempts to standardize APIs across services, but it didn't worked. Then there were attempts to try to standardize services on the SDK level. This is partially working, but require a very high implementation effort and permanent maintenance. Reverse-engineering service API by looking at the API-REF documentation is very time consuming. A huge issue is also that API-REF is being a human-written document that very often diverges from the code what leads to the issues when covering those resources in SDK/CLI. Tracking the API evolving is another aspect of the maintenance effort.
As a solution a completely different approach has been chosen to reduce maintenance effort while at the same time guaranteeing that API bindings match to what service is supporting in reality. Instead of human reading the API-REF written by another human who maybe was involved in the implementation of the feature OpenAPI specs is being chosen as a source of truth. Since such specs were also not existing and multiple attempts to introduce OpenAPI in OpenStack failed the process was restarted again. Currently there is a lot of work happening in OpenStack to produce specs for majority of the services. Main component responsible for that is codegenerator. Apart of inspecting source code of the selected OpenStack services it is also capable of generating tools in this repository. There is of course a set of the framework code, but the REST API wrapping and commands implementation is fully generated.
At the end of the day it means that there is no need to touch the generated code at all. Once resources available in the OpenAPI spec of the service are being initially integrated into the subprojects here they become maintained by the generator. New features added into the resource by the service would be automatically updated once OpenAPI spec is being updated.
Generating code from the OpenAPI has another logical consequence: generated code is providing the same features as the API itself. So if API is doing thing not very logical the SDK/CLI will do it in the same way. Previously it was always landing on the shoulders of SDK/CLI maintainers to try to cope with it. Now if API is bad - API author is to blame.
- Code being automatically generated from OpenAPI specs of the service APIs.
- Unix philosophy: "do one thing well". Every resource/command coverage tries to focus only on the exact API. Combination of API calls is not in scope of the generated code. "Simple is better then complex" (The Zen of Python).
- SDK/CLI bindings are wrapping the API with no additional guessing or normalization.
- User is in full control of input and output. Microversion X.Y has a concrete body schema and with no faulty merges between different versions.
Components
openstack_sdk
- SDKopenstack_cli
- The new and shiny CLI for OpenStackopenstack_tui
- Text (Terminal) User Interfacestructable_derive
- Helper crate for having Output in some way similar to old OpenStackClient
Trying out
Install binary
It is possible to install compiled version from the GitHub releases. It comes with a dedicated installer in every release and can be retrieved with the following command:
curl --proto '=https' --tlsv1.2 -LsSf https://github.com/gtema/openstack/releases/download/openstack_cli-v0.8.2/openstack_cli-installer.sh | sh
TUI can be installed similarly:
curl --proto '=https' --tlsv1.2 -LsSf https://github.com/gtema/openstack/releases/download/openstack_tui-v0.1.6/openstack_tui-installer.sh | sh
Build locally
Alternatively it is possible to compile project from sources. Since the project
is a pure Rust
it requires having a Rust compile suite.
cargo b
Run
Once the binary is available just start playing with it. If you already have
your clouds.yaml
config file from python-openstackclient
you are free to
go:
osc --help
osc --os-cloud devstack compute flavor list
Authentication and authorization
Understanding authentication in OpenStack is far away from being a trivial task. In general authentication and authorization are different things, but they are mixed into 1 API request. When trying to authenticate user is passing identification data (username and password or similar) together with the requested authorization (project scope, domain scope or similar). As a response to this API request a session Token is being returned to the user that needs to be always sent with any following request. When authorization scope need to be changed (i.e. perform API in a scope of a different project) a re-authorization need to be performed. That may be done with the same user identification data or using existing session token.
Existing python based OpenStack tools are keeping only one active session going through re-authorization whenever required. There is a support for the token caching which is bound to the used authorization request. When a new session is requested a search (when enabled) is being performed in the cache and a matching token is being returned which is then retried. When MFA or SSO are being used this process is introducing a very ugly user experience forcing user to re-entrer verification data every time a new scope is being requested with a new session.
In this project authentication and authorization in user facing applications is handled differently. Auth caching to the file system is enabled by default. User identification data (auth_url + user name + user domain name) is combined into the hash which is then used as a 1st level caching key and points to the hashmap of authorization data and corresponding successful authorization response data. A key in the 2nd level is a hash calculated from the scope information and a value is a returned token with catalog and expiration information. This way of handling information allows to immediately retrieve valid auth information for the requested scope if it already exists (which may be even shared between processes) or reuse valid authentication data to get new valid requested authorization saving user from need to re-process MFA or SSO requirements.
Configuration
Rust based tools support typical
clouds.yaml
/secure.yaml
files for configuration.
Authentication methods
Currently only a subset of all possible authentication methods is covered with the work on adding further method ongoing
v3Token
A most basic auth method is an API token (X-Auth-Token
). In the clouds.yaml
this requires setting auth_type
to one of the [v3token
, token
]. The token
itself should be specified in the token
attribute.
v3Password
A most common auth method is a username/password. In the clouds.yaml
this
requires setting auth_type
to one of the [v3password
, password
] or
leaving it empty.
Following attributes specify the authentication data:
username
- The user nameuser_id
- The user IDpassword
- The user passworduser_domain_name
- The name of the domain user belongs touser_domain_id
- The ID of the domain user belongs to
It is required to specify username
or user_id
as well as user_domain_name
or user_domain_id
.
v3Totp
Once user login is protected with the MFA a OTP token must be specified. It is
represented as passcode
, but it not intended to be used directly in the clouds.yaml
v3Multifactor
A better way to handle MFA is by using a v3multifactor
auth type. In this
case configuration looks a little bit different:
auth_type
=v3multifactor
auth_methods
is a list of individualauth_type
s combined in the authentication flow (i.e['v3password', 'v3totp']
)
When a cloud connection is being established in an interactive mode and server responds that it require additional authentication methods those would be processed based on the available data.
v3WebSso
An authentication method that is getting more a more popular is a Single Sign On using remote Identity Data Provider. This flow requires user to authenticate itself in the browser by the IDP directly. It is required to provide following data in the configuration in order for this mode to be used:
auth_type
=v3websso
identity_provider
- identity provider as configured in the Keystoneprotocol
- IDP protocol as configured in the Keystone
Note: This authentication type only works in the interactive mode. That
means in the case of the CLI that there must be a valid terminal (echo foo | osc identity user create
will not work)
v3ApplicationCredential
Application credentials provide a way to delegate a user’s authorization to an application without sharing the user’s password authentication. This is a useful security measure, especially for situations where the user’s identification is provided by an external source, such as LDAP or a single-sign-on service. Instead of storing user passwords in config files, a user creates an application credential for a specific project, with all or a subset of the role assignments they have on that project, and then stores the application credential identifier and secret in the config file.
Multiple application credentials may be active at once, so you can easily rotate application credentials by creating a second one, converting your applications to use it one by one, and finally deleting the first one.
Application credentials are limited by the lifespan of the user that created them. If the user is deleted, disabled, or loses a role assignment on a project, the application credential is deleted.
Required configuration:
auth_type
=v3applicationcredential
application_credential_secret
- a secret part of the application credentialapplication_credential_id
- application credential identityapplication_credential_name
- application credential name. Note: It is required to specify user data when using application credential nameuser_id
- user ID whenapplication_credential_name
is useduser_name
- user name whenapplication_credential_name
is useduser_domain_id
- User domain ID whenapplication_credential_name
is useduser_domain_name
- User domain ID whenapplication_credential_name
is used
Either application_credential_id
is required or application_credential_name
in which case additionally the user information is required.
Caching
As described above in difference to the Python OpenStack tooling authentication
caching is enabled by default. It can be disabled using cache.auth: false
in
the clouds.yaml
.
Data is cached locally in the ~/.osc
folder. It is represented by set of
files where file name is constructed as a hash of authentication information
(discarding sensitive data). Content of the file is a serialized map of
authorization data (scope) with the token information (catalog, expiration,
etc).
Every time a new connection need to be established first a search in the cache is performed to find an exact match using supplied authentication and authorization information. When there is no usable information (no information at all or cached token is already expired) a search is performed for any valid token ignoring the scope (authz). When a valid token is found in the cache it is used to obtain a new authorization with required scope. Otherwise a new authentication is being performed.
API operation mapping structure
Python based OpenStack API binding tools are structured on a resource base, where every API resource is a class/object having multiple methods for the resource CRUD and other operations. Moreover microversion differences are also being dealt inside this single object. This causes method typing being problematic and not definite (i.e. when create and get operations return different structures or microversions require modified types).
Since Rust is a strongly typed programming language the same approach is not going to work (neither this approach proved to be a good one). Every unique API call (url + method + payload type) is represented by a dedicated module (simple Enum are still mapped into same module). All RPC-like actions of OpenStack services are also represented by a dediated module. Also when the operation supports different request body schemas in different microversions it is also implemented by a dedicated module. This gives user a better control by using an object with definite constraints explicitly declaring support of a certain microversion.
OpenStack API bindings (SDK)
Every platform API requires SDK bindings to various programming languages.
OpenStack API bindings for Rust are not an exception. The OpenStack comes with
openstack_sdk
crate providing an SDK with both synchronous and asynchronous
interfaces.
API bindings are generated from the OpenAPI specs of corresponding services. That means that those are only wrapping the API and usually not providing additional convenience features. The major benefit is that no maintenance efforts are required for the code being generated. Once OpenStack service updates OpenAPI spec for the integrated resources the changes would be immediately available on the next regeneration.
Features
- Sync and Async interface
Query
,Find
andPagination
interfaces implementing basic functionalityRawQuery
interface providing more control over the API invocation with upload and download capabilities.- Every combination of URL + http method + body schema is represented by a dedicated module
- User is in charge of return data schema.
Structure
Every single API call is represented by a dedicated module with a structure
implementing REST Endpoint
interface. That means that a GET
operation is a
dedicated implementation compared to a POST
operation. Like described in the
Structure document every RPC-like action and every microversion
is implemented with a single module.
Using
The simplest example demonstrating how to list compute flavors:
#![allow(unused)] fn main() { use openstack_sdk::api::{paged, Pagination, QueryAsync}; use openstack_sdk::{AsyncOpenStack, config::ConfigFile, OpenStackError}; use openstack_sdk::types::ServiceType; use openstack_sdk::api::compute::v2::flavor::list; async fn list_flavors() -> Result<(), OpenStackError> { // Get the builder for the listing Flavors Endpoint let mut ep_builder = list::Request::builder(); // Set the `min_disk` query param ep_builder.min_disk("15"); let ep = ep_builder.build().unwrap(); let cfg = ConfigFile::new().unwrap(); // Get connection config from clouds.yaml/secure.yaml let profile = cfg.get_cloud_config("devstack").unwrap().unwrap(); // Establish connection let mut session = AsyncOpenStack::new(&profile).await?; // Invoke service discovery when desired. session.discover_service_endpoint(&ServiceType::Compute).await?; // Execute the call with pagination limiting maximum amount of entries to 1000 let data: Vec<serde_json::Value> = paged(ep, Pagination::Limit(1000)) .query_async(&session) .await.unwrap(); println!("Data = {:?}", data); Ok(()) } }
Documentation
Current crate documentation is known to be very poor. It will be addressed in future, but for now the best way to figure out how it works is to look at openstack_cli and openstack_tui using it.
Crate documentation is published here
Project documentation is available here
OpenStackClient
osc
is a CLI for the OpenStack written in Rust. It is relying on the
corresponding openstack_sdk
crate (library) and is generated using OpenAPI
specifications. That means that the maintenance effort for the tool is much
lower compared to the fully human written python-openstackclient
. Due to the
fact of being auto-generated there are certain differences to the python cli
but also an enforced UX consistency.
NOTE: As a new tool it tries to solve some issues with the original
python-openstackclient
. That means that it can not provide seamless migration
from one tool to another.
Commands implementation code is being produced by codegenerator what means only low maintenance is required for that code.
Features
-
Advanced authentication caching built-in and enabled by default
-
Status based resource coloring (resource list table rows are colored by the resource state)
-
Output configuration (using
$XDG_CONFIG_DIR/osc/config.yaml
it is possible to configure which fields should be returned when listing resources to enable customization). -
Strict microversion binding for resource modification requests (instead of
openstack server create ...
which will not work with all microversions you useosc compute server create290
which will only work if server supports it. It is similar toopenstack --os-compute-api-version X.Y
). It behaves the same on every cloud independent of which microversion this cloud supports (as long as it supports required microversion). -
Can be wonderfully combined with jq for ultimate control of the necessary data (
osc server list -o json | jq -r ".[].flavor.original_name"
) -
Output everything what cloud sent (
osc compute server list -o json
to return fields that we never even knew about, but the cloud sent us). -
osc
api as an API wrapper allowing user to perform any direct API call specifying service type, url, method and payload. This can be used for example when certain resource is not currently implemented natively. -
osc auth with subcommands for dealing explicitly with authentication (showing current auth info, renewing auth, MFA/SSO support)
Microversions
Initially python-openstackclient
was using lowest microversion unless
additional argument specifying microversion was passed. Later, during switching
commands towards using of the OpenStackSDK
a highest possible microversion
started being used (again unless user explicitly requested microversion with
--XXX-api-version Y.Z
). One common thing both approaches use is to give user
control over the version what is crucial to guarantee stability. The
disadvantage of both approaches is that they come with certain opinions that
does not necessarily match what user expects and make expectation on what will
happen hard. For the end user reading help page of the command is pretty
complex and error prone when certain parameters appear, disappear and re-appear
with different types between microversion. Implementing (and using) the command
is also both complex and error prone in this case.
osc
is trying to get the best of 2 approaches and providing dedicated
commands for microversions (i.e. create20
, create294
). Latest microversion
command is always having a general alias (create
in the above case) to let
user explicitly use latest microversion, what, however, does not guarantee it
can be invoked with requested parameters. This approach allows user to be very
explicit in the requirement and have a guarantee of the expected parameters.
When a newer microversion is required user should explicitly to do "migration"
step adapting the invocation to a newer set of parameters. Microversion (or
functionality) deprecation is also much simpler this way and is handled by
marking the whole command deprecated and/or drop it completely.
Request timing
osc
supports --timing
argument that enables capturing of all HTTP requests
and outputs timings grouped by URL (ignoring the query parameters) and method.
Command interface
osc
OpenStack command line interface.
Configuration
As all OpenStack tools it fully supports clouds.yaml
Certain aspects of the CLI itself (not the cloud connection) can be configured using $XDG_CONFIG_HOME/osc/config.yaml
file. With it it is possible, for example, to configure which resource fields are returned when no other output controlling parameters has be passed.
Example:
yaml views: compute.server: # Listing compute servers will only return ID, NAME and IMAGE columns unless `-o wide` or `-f XXX` parameters are being passed fields: [id, name, image] dns.zone/recordset: # DNS zone recordsets are listed in the wide mode by default. wide: true
Features
-
osc api
as an API wrapper allowing user to perform any direct API call specifying service type, url, method and payload. This can be used for example when certain resource is not currently implemented natively. -
osc auth
with subcommands for dealing explicitly with authentication (showing current auth info, renewing auth, MFA/SSO support) -
Every resource is having a service type in the command solving confusions like user groups vs volume groups
-
Every multi-word resource name is "-" separated (i.e. floating-ip, access-rule)
Output
osc ... -o json
as an explicit machine readable format output. It allows seeing raw resource json representation as send by the API without any processing on the client side.
Note: the result is not the raw json response, but the raw json resource information found underneath expected resource key. This mode can be used i.e. to see fields that are not expected by the osc
and allows further easy machine processing with tools like jq
osc ... -o wide
for list operations to return all known fields. By default list operation will only return a subset of known generic resource fields to prevent multiline tables. This mode (together with not specifying-o
parameter at all) is considered as an output for humans. Field names are not generally renamed and are names as the API returns them.
Shell autocompletion
osc
supports generation of the completion file for diverse shells. This can be enabled i.e. by executing
bash echo 'source <(osc completion bash)' >>~/.bashrc
Usage: osc [OPTIONS] <COMMAND>
Available subcommands:
osc api
— Perform direct REST API requests with authorizationosc auth
— Cloud Authentication operationsosc block-storage
— Block Storage (Volume) service (Cinder) commandsosc catalog
— Catalog commands argsosc compute
— Compute service (Nova) operationsosc container-infrastructure
— Container Infra service (Magnum) operationsosc dns
— DNS service (Designate) operationsosc identity
— Identity (Keystone) commandsosc image
— Image service operationsosc load-balancer
— Load Balancer service operationsosc network
— Network (Neutron) commandsosc object-store
— Object Store service (Swift) commandsosc placement
— The placement API service was introduced in the 14.0.0 Newton release within the nova repository and extracted to the placement repository in the 19.0.0 Stein release. This is a REST API stack and data model used to track resource provider inventories and usages, along with different classes of resources. For example, a resource provider can be a compute node, a shared storage pool, or an IP allocation pool. The placement service tracks the inventory and usage of each provider. For example, an instance created on a compute node may be a consumer of resources such as RAM and CPU from a compute node resource provider, disk from an external shared storage pool resource provider and IP addresses from an external IP pool resource providerosc completion
— Output shell completion code for the specified shell (bash, zsh, fish, or powershell). The shell code must be evaluated to provide interactive completion ofosc
commands. This can be done by sourcing it from the .bash_profile
Options:
-
--os-cloud <OS_CLOUD>
— Name reference to the clouds.yaml entry for the cloud configuration -
--os-project-id <OS_PROJECT_ID>
— Project ID to use instead of the one in connection profile -
--os-project-name <OS_PROJECT_NAME>
— Project Name to use instead of the one in the connection profile -
--os-client-config-file <OS_CLIENT_CONFIG_FILE>
— Custom path to theclouds.yaml
config file -
--os-client-secure-file <OS_CLIENT_SECURE_FILE>
— Custom path to thesecure.yaml
config file -
-o
,--output <OUTPUT>
— Output formatPossible values:
json
: Json outputwide
: Wide (Human readable table with extra attributes). Note: this has effect only in list operations
-
-f
,--fields <FIELDS>
— Fields to return in the output (only in normal and wide mode) -
-p
,--pretty
— Pretty print the output -
-v
,--verbose
— Verbosity level. Repeat to increase level -
--timing
— Record HTTP request timings
osc api
Perform direct REST API requests with authorization
This command enables direct REST API call with the authorization and version discovery handled transparently. This may be used when required operation is not implemented by the osc
or some of the parameters require special handling.
Example:
console osc --os-cloud devstack api compute flavors/detail | jq
Usage: osc api [OPTIONS] <SERVICE_TYPE> <URL>
Arguments:
<SERVICE_TYPE>
— Service type as used in the service catalog<URL>
— Rest URL (relative to the endpoint information from the service catalog). Do not start URL with the "/" to respect endpoint version information
Options:
-
-m
,--method <METHOD>
— HTTP MethodDefault value:
get
Possible values:
head
: HEADget
: GETpatch
: PATCHput
: PUTpost
: POSTdelete
: DELETE
-
-H
,--header <key=value>
— Additional headers -
--body <BODY>
— Request body to be used
osc auth
Cloud Authentication operations
This command provides various authorization operations (login, show, status, etc)
Usage: osc auth <COMMAND>
Available subcommands:
osc auth login
— Login to the cloud and get a valid authorization tokenosc auth show
— Show current authorization information for the cloud
osc auth login
Fetch a new valid authorization token for the cloud.
This command writes token to the stdout
Usage: osc auth login [OPTIONS]
Options:
--renew
— Require token renewal
osc auth show
Show current authorization information for the cloud
This command returns authentication and authorization information for the currently active connection. It includes issue and expiration information, user data, list of granted roles and project/domain information.
NOTE: The command does not support selecting individual fields in the output, but it supports -o json
command and returns full available information in json format what allows further processing with jq
Usage: osc auth show
osc block-storage
Block Storage (Volume) service (Cinder) commands
Usage: osc block-storage <COMMAND>
Available subcommands:
osc block-storage attachment
— Attachments (attachments)osc block-storage availability-zone
— Availability zonesosc block-storage backup
— Backupsosc block-storage cluster
— Clusters (clusters)osc block-storage default-type
— Default Volume Types (default-types)osc block-storage extension
— API extensions (extensions)osc block-storage group
— Generic volume groups (groups)osc block-storage group-snapshot
— GroupSnapshot snapshots (group_snapshots)osc block-storage group-type
— Group types (group_types)osc block-storage host
— Hosts extension (os-hosts)osc block-storage limit
— Limits (limits)osc block-storage message
— Messages (messages)osc block-storage os-volume-transfer
— Volume transfersosc block-storage qos-spec
— Quality of service (QoS) specifications (qos-specs)osc block-storage service
— Services (os-services)osc block-storage snapshot
— Volume snapshots (snapshots)osc block-storage snapshot-manage
— SnapshotManage manage extension (manageable_snapshots)osc block-storage resource-filter
— Resource filtersosc block-storage type
— Block Storage VolumeType type commandsosc block-storage volume
— Block Storage Volume commandsosc block-storage volume-manage
— Volume manage extension (manageable_volumes)osc block-storage volume-transfer
— Volume transfers (volume-transfers) (3.55 or later)
osc block-storage attachment
Attachments (attachments)
Lists all, lists all with details, shows details for, creates, and deletes attachment.
Note
Everything except for Complete attachment is new as of the 3.27 microversion. Complete attachment is new as of the 3.44 microversion.
When you create, list, update, or delete attachment, the possible status values are:
-
attached: A volume is attached for the attachment.
-
attaching: A volume is attaching for the attachment.
-
detached: A volume is detached for the attachment.
-
reserved: A volume is reserved for the attachment.
-
error_attaching: A volume is error attaching for the attachment.
-
error_detaching: A volume is error detaching for the attachment.
-
deleted: The attachment is deleted.
Usage: osc block-storage attachment <COMMAND>
Available subcommands:
osc block-storage attachment complete
— Empty body for os-complete actionosc block-storage attachment create354
— Create an attachmentosc block-storage attachment create327
— Create an attachmentosc block-storage attachment delete
— Delete an attachmentosc block-storage attachment list
— Return a detailed list of attachmentsosc block-storage attachment set327
— Update an attachment recordosc block-storage attachment show
— Return data about the given attachment
osc block-storage attachment complete
Empty body for os-complete action
Usage: osc block-storage attachment complete <ID>
Arguments:
<ID>
— id parameter for /v3/attachments/{id}/action API
osc block-storage attachment create354
Create an attachment.
This method can be used to create an empty attachment (reserve) or to create and initialize a volume attachment based on the provided input parameters.
If the caller does not yet have the connector information but needs to reserve an attachment for the volume (ie Nova BootFromVolume) the create can be called with just the volume-uuid and the server identifier. This will reserve an attachment, mark the volume as reserved and prevent any new attachment_create calls from being made until the attachment is updated (completed).
The alternative is that the connection can be reserved and initialized all at once with a single call if the caller has all of the required information (connector data) at the time of the call.
NOTE: In Nova terms server == instance, the server_id parameter referenced below is the UUID of the Instance, for non-nova consumers this can be a server UUID or some other arbitrary unique identifier.
Starting from microversion 3.54, we can pass the attach mode as argument in the request body.
Expected format of the input parameter 'body':
{ "attachment": { "volume_uuid": "volume-uuid", "instance_uuid": "null|nova-server-uuid", "connector": "null|<connector-object>", "mode": "null|rw|ro" } }
Example connector:
{ "connector": { "initiator": "iqn.1993-08.org.debian:01:cad181614cec", "ip": "192.168.1.20", "platform": "x86_64", "host": "tempest-1", "os_type": "linux2", "multipath": false, "mountpoint": "/dev/vdb", "mode": "null|rw|ro" } }
NOTE all that's required for a reserve is volume_uuid and an instance_uuid.
returns: A summary view of the attachment object
Usage: osc block-storage attachment create354 [OPTIONS] --volume-uuid <VOLUME_UUID>
Options:
-
--connector <key=value>
— Theconnector
object -
--instance-uuid <INSTANCE_UUID>
— The UUID of the volume which the attachment belongs to -
--mode <MODE>
— The attach mode of attachment, acceptable values are read-only (‘ro’) and read-and-write (‘rw’).New in version 3.54
Possible values:
ro
,rw
-
--volume-uuid <VOLUME_UUID>
— The UUID of the volume which the attachment belongs to
osc block-storage attachment create327
Create an attachment.
This method can be used to create an empty attachment (reserve) or to create and initialize a volume attachment based on the provided input parameters.
If the caller does not yet have the connector information but needs to reserve an attachment for the volume (ie Nova BootFromVolume) the create can be called with just the volume-uuid and the server identifier. This will reserve an attachment, mark the volume as reserved and prevent any new attachment_create calls from being made until the attachment is updated (completed).
The alternative is that the connection can be reserved and initialized all at once with a single call if the caller has all of the required information (connector data) at the time of the call.
NOTE: In Nova terms server == instance, the server_id parameter referenced below is the UUID of the Instance, for non-nova consumers this can be a server UUID or some other arbitrary unique identifier.
Starting from microversion 3.54, we can pass the attach mode as argument in the request body.
Expected format of the input parameter 'body':
{ "attachment": { "volume_uuid": "volume-uuid", "instance_uuid": "null|nova-server-uuid", "connector": "null|<connector-object>", "mode": "null|rw|ro" } }
Example connector:
{ "connector": { "initiator": "iqn.1993-08.org.debian:01:cad181614cec", "ip": "192.168.1.20", "platform": "x86_64", "host": "tempest-1", "os_type": "linux2", "multipath": false, "mountpoint": "/dev/vdb", "mode": "null|rw|ro" } }
NOTE all that's required for a reserve is volume_uuid and an instance_uuid.
returns: A summary view of the attachment object
Usage: osc block-storage attachment create327 [OPTIONS] --volume-uuid <VOLUME_UUID>
Options:
--connector <key=value>
— Theconnector
object--instance-uuid <INSTANCE_UUID>
— The UUID of the volume which the attachment belongs to--volume-uuid <VOLUME_UUID>
— The UUID of the volume which the attachment belongs to
osc block-storage attachment delete
Delete an attachment.
Disconnects/Deletes the specified attachment, returns a list of any known shared attachment-id's for the effected backend device.
returns: A summary list of any attachments sharing this connection
Usage: osc block-storage attachment delete <ID>
Arguments:
<ID>
— id parameter for /v3/attachments/{id} API
osc block-storage attachment list
Return a detailed list of attachments
Usage: osc block-storage attachment list [OPTIONS]
Options:
-
--all-tenants <ALL_TENANTS>
— Shows details for all project. Admin onlyPossible values:
true
,false
-
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--offset <OFFSET>
— Used in conjunction with limit to return a slice of items. offset is where to start in the list -
--sort <SORT>
— Comma-separated list of sort keys and optional sort directions in the form of < key > [: < direction > ]. A valid direction is asc (ascending) or desc (descending) -
--sort-dir <SORT_DIR>
— Sorts by one or more sets of attribute and sort direction combinations. If you omit the sort direction in a set, default is desc. Deprecated in favour of the combined sort parameterPossible values:
asc
,desc
-
--sort-key <SORT_KEY>
— Sorts by an attribute. A valid value is name, status, container_format, disk_format, size, id, created_at, or updated_at. Default is created_at. The API uses the natural sorting direction of the sort_key attribute value. Deprecated in favour of the combined sort parameter -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc block-storage attachment set327
Update an attachment record.
Update a reserved attachment record with connector information and set up the appropriate connection_info from the driver.
Expected format of the input parameter 'body':
{ "attachment": { "connector": { "initiator": "iqn.1993-08.org.debian:01:cad181614cec", "ip": "192.168.1.20", "platform": "x86_64", "host": "tempest-1", "os_type": "linux2", "multipath": false, "mountpoint": "/dev/vdb", "mode": "None|rw|ro" } } }
Usage: osc block-storage attachment set327 [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v3/attachments/{id} API
Options:
--connector <key=value>
osc block-storage attachment show
Return data about the given attachment
Usage: osc block-storage attachment show <ID>
Arguments:
<ID>
— id parameter for /v3/attachments/{id} API
osc block-storage availability-zone
Availability zones
Lists and gets detailed availability zone information.
Usage: osc block-storage availability-zone <COMMAND>
Available subcommands:
osc block-storage availability-zone list
— Describe all known availability zones
osc block-storage availability-zone list
Describe all known availability zones
Usage: osc block-storage availability-zone list
osc block-storage backup
Backups
A backup is a full copy of a volume stored in an external service. The service can be configured. The only supported service is Object Storage. A backup can subsequently be restored from the external service to either the same volume that the backup was originally taken from or to a new volume.
Usage: osc block-storage backup <COMMAND>
Available subcommands:
osc block-storage backup create351
— Create a new backuposc block-storage backup create343
— Create a new backuposc block-storage backup create30
— Create a new backuposc block-storage backup delete
— Delete a backuposc block-storage backup export
— Export a backuposc block-storage backup force-delete
— Empty body for os-force_delete actionosc block-storage backup import
— Import a backuposc block-storage backup list
— Returns a detailed list of backupsosc block-storage backup reset-status
— Command without description in OpenAPIosc block-storage backup set343
— Update a backuposc block-storage backup set39
— Update a backuposc block-storage backup show
— Return data about the given backup
osc block-storage backup create351
Create a new backup
Usage: osc block-storage backup create351 [OPTIONS] --volume-id <VOLUME_ID>
Options:
-
--availability-zone <AVAILABILITY_ZONE>
— The backup availability zone key value pair.New in version 3.51
-
--container <CONTAINER>
— The container name or null -
--description <DESCRIPTION>
— The backup description or null -
--force <FORCE>
— Indicates whether to backup, even if the volume is attached. Default isfalse
. See valid boolean valuesPossible values:
true
,false
-
--incremental <INCREMENTAL>
— Indicates whether to backup, even if the volume is attached. Default isfalse
. See valid boolean valuesPossible values:
true
,false
-
--metadata <key=value>
— The backup metadata key value pairs.New in version 3.43
-
--name <NAME>
— The name of the Volume Backup -
--snapshot-id <SNAPSHOT_ID>
— The UUID of the source snapshot that you want to back up -
--volume-id <VOLUME_ID>
— The UUID of the volume that you want to back up
osc block-storage backup create343
Create a new backup
Usage: osc block-storage backup create343 [OPTIONS] --volume-id <VOLUME_ID>
Options:
-
--container <CONTAINER>
— The container name or null -
--description <DESCRIPTION>
— The backup description or null -
--force <FORCE>
— Indicates whether to backup, even if the volume is attached. Default isfalse
. See valid boolean valuesPossible values:
true
,false
-
--incremental <INCREMENTAL>
— Indicates whether to backup, even if the volume is attached. Default isfalse
. See valid boolean valuesPossible values:
true
,false
-
--metadata <key=value>
— The backup metadata key value pairs.New in version 3.43
-
--name <NAME>
— The name of the Volume Backup -
--snapshot-id <SNAPSHOT_ID>
— The UUID of the source snapshot that you want to back up -
--volume-id <VOLUME_ID>
— The UUID of the volume that you want to back up
osc block-storage backup create30
Create a new backup
Usage: osc block-storage backup create30 [OPTIONS] --volume-id <VOLUME_ID>
Options:
-
--container <CONTAINER>
— The container name or null -
--description <DESCRIPTION>
— The backup description or null -
--force <FORCE>
— Indicates whether to backup, even if the volume is attached. Default isfalse
. See valid boolean valuesPossible values:
true
,false
-
--incremental <INCREMENTAL>
— Indicates whether to backup, even if the volume is attached. Default isfalse
. See valid boolean valuesPossible values:
true
,false
-
--name <NAME>
— The name of the Volume Backup -
--snapshot-id <SNAPSHOT_ID>
— The UUID of the source snapshot that you want to back up -
--volume-id <VOLUME_ID>
— The UUID of the volume that you want to back up
osc block-storage backup delete
Delete a backup
Usage: osc block-storage backup delete <ID>
Arguments:
<ID>
— id parameter for /v3/backups/{id} API
osc block-storage backup export
Export a backup
Usage: osc block-storage backup export <ID>
Arguments:
<ID>
— id parameter for /v3/backups/{id}/export_record API
osc block-storage backup force-delete
Empty body for os-force_delete action
Usage: osc block-storage backup force-delete <ID>
Arguments:
<ID>
— id parameter for /v3/backups/{id}/action API
osc block-storage backup import
Import a backup
Usage: osc block-storage backup import --backup-service <BACKUP_SERVICE> --backup-url <BACKUP_URL>
Options:
--backup-service <BACKUP_SERVICE>
— The service used to perform the backup--backup-url <BACKUP_URL>
— An identifier string to locate the backup
osc block-storage backup list
Returns a detailed list of backups
Usage: osc block-storage backup list [OPTIONS]
Options:
-
--all-tenants <ALL_TENANTS>
— Shows details for all project. Admin onlyPossible values:
true
,false
-
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--offset <OFFSET>
— Used in conjunction with limit to return a slice of items. offset is where to start in the list -
--sort <SORT>
— Comma-separated list of sort keys and optional sort directions in the form of < key > [: < direction > ]. A valid direction is asc (ascending) or desc (descending) -
--sort-dir <SORT_DIR>
— Sorts by one or more sets of attribute and sort direction combinations. If you omit the sort direction in a set, default is desc. Deprecated in favour of the combined sort parameter -
--sort-key <SORT_KEY>
— Sorts by an attribute. A valid value is name, status, container_format, disk_format, size, id, created_at, or updated_at. Default is created_at. The API uses the natural sorting direction of the sort_key attribute value. Deprecated in favour of the combined sort parameter -
--with-count <WITH_COUNT>
— Whether to show count in API response or not, default is FalsePossible values:
true
,false
-
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc block-storage backup reset-status
Command without description in OpenAPI
Usage: osc block-storage backup reset-status --status <STATUS> <ID>
Arguments:
<ID>
— id parameter for /v3/backups/{id}/action API
Options:
--status <STATUS>
osc block-storage backup set343
Update a backup
Usage: osc block-storage backup set343 [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v3/backups/{id} API
Options:
--description <DESCRIPTION>
--metadata <key=value>
--name <NAME>
osc block-storage backup set39
Update a backup
Usage: osc block-storage backup set39 [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v3/backups/{id} API
Options:
--description <DESCRIPTION>
--name <NAME>
osc block-storage backup show
Return data about the given backup
Usage: osc block-storage backup show <ID>
Arguments:
<ID>
— id parameter for /v3/backups/{id} API
osc block-storage cluster
Clusters (clusters)
Administrator only. Lists all Cinder clusters, show cluster detail, enable or disable a cluster.
Each cinder service runs on a host computer (possibly multiple services on the same host; it depends how you decide to deploy cinder). In order to support High Availability scenarios, services can be grouped into clusters where the same type of service (for example, cinder-volume) can run on different hosts so that if one host goes down the service is still available on a different host. Since there’s no point having these services sitting around doing nothing while waiting for some other host to go down (which is also known as Active/Passive mode), grouping services into clusters also allows cinder to support Active/Active mode in which all services in a cluster are doing work all the time.
Note: Currently the only service that can be grouped into clusters is cinder-volume.
Clusters are determined by the deployment configuration; that’s why there is no ‘create-cluster’ API call listed below. Once your services are up and running, however, you can use the following API requests to get information about your clusters and to update their status.
Usage: osc block-storage cluster <COMMAND>
Available subcommands:
osc block-storage cluster list
— Return a detailed list of all existing clustersosc block-storage cluster set
— Enable/Disable scheduling for a clusterosc block-storage cluster show
— Return data for a given cluster name with optional binary
osc block-storage cluster list
Return a detailed list of all existing clusters.
Filter by is_up, disabled, num_hosts, and num_down_hosts.
Usage: osc block-storage cluster list [OPTIONS]
Options:
-
--active-backend-id <ACTIVE_BACKEND_ID>
— The ID of active storage backend. Only in cinder-volume service -
--binary <BINARY>
— Filter the cluster list result by binary name of the clustered services. One of cinder-api, cinder-scheduler, cinder-volume or cinder-backupPossible values:
cinder-api
,cinder-backup
,cinder-scheduler
,cinder-volume
-
--disabled <DISABLED>
— Filter the cluster list result by statusPossible values:
true
,false
-
--frozen <FROZEN>
— Whether the cluster is frozen or notPossible values:
true
,false
-
--is-up <IS_UP>
— Filter the cluster list result by statePossible values:
true
,false
-
--name <NAME>
— Filter the cluster list result by cluster name -
--num-down-hosts <NUM_DOWN_HOSTS>
— Filter the cluster list result by number of down hosts -
--num-hosts <NUM_HOSTS>
— Filter the cluster list result by number of hosts -
--replication-stats <REPLICATION_STATS>
— Filter the cluster list result by replication statusPossible values:
disabled
,enabled
osc block-storage cluster set
Enable/Disable scheduling for a cluster
Usage: osc block-storage cluster set [OPTIONS] --name <NAME> <ID>
Arguments:
<ID>
— id parameter for /v3/clusters/{id} API
Options:
--binary <BINARY>
— The binary name of the services in the cluster--disabled-reason <DISABLED_REASON>
— The reason for disabling a resource--name <NAME>
— The name to identify the service cluster
osc block-storage cluster show
Return data for a given cluster name with optional binary
Usage: osc block-storage cluster show <ID>
Arguments:
<ID>
— id parameter for /v3/clusters/{id} API
osc block-storage default-type
Default Volume Types (default-types)
Manage a default volume type for individual projects.
By default, a volume-create request that does not specify a volume-type will assign the configured system default volume type to the volume. You can override this behavior on a per-project basis by setting a different default volume type for any project.
Available in microversion 3.62 or higher.
NOTE: The default policy for list API is system admin so you would require a system scoped token to access it.
Usage: osc block-storage default-type <COMMAND>
Available subcommands:
osc block-storage default-type delete
— Unset a default volume type for a projectosc block-storage default-type list
— Return a list of default typesosc block-storage default-type set362
— Set a default volume type for the specified projectosc block-storage default-type show
— Return detail of a default type
osc block-storage default-type delete
Unset a default volume type for a project
Usage: osc block-storage default-type delete <ID>
Arguments:
<ID>
— id parameter for /v3/default-types/{id} API
osc block-storage default-type list
Return a list of default types
Usage: osc block-storage default-type list
osc block-storage default-type set362
Set a default volume type for the specified project
Usage: osc block-storage default-type set362 --volume-type <VOLUME_TYPE> <ID>
Arguments:
<ID>
— id parameter for /v3/default-types/{id} API
Options:
--volume-type <VOLUME_TYPE>
osc block-storage default-type show
Return detail of a default type
Usage: osc block-storage default-type show <ID>
Arguments:
<ID>
— id parameter for /v3/default-types/{id} API
osc block-storage extension
API extensions (extensions)
Usage: osc block-storage extension <COMMAND>
Available subcommands:
osc block-storage extension list
— Command without description in OpenAPI
osc block-storage extension list
Command without description in OpenAPI
Usage: osc block-storage extension list
osc block-storage group
Generic volume groups (groups)
Generic volume groups enable you to create a group of volumes and manage them together.
How is generic volume groups different from consistency groups? Currently consistency groups in cinder only support consistent group snapshot. It cannot be extended easily to serve other purposes. A project may want to put volumes used in the same application together in a group so that it is easier to manage them together, and this group of volumes may or may not support consistent group snapshot. Generic volume group is introduced to solve this problem. By decoupling the tight relationship between the group construct and the consistency concept, generic volume groups can be extended to support other features in the future.
Usage: osc block-storage group <COMMAND>
Available subcommands:
osc block-storage group create313
— Create a new grouposc block-storage group create-from-src314
— Command without description in OpenAPIosc block-storage group delete313
— Command without description in OpenAPIosc block-storage group disable-replication338
— Command without description in OpenAPIosc block-storage group enable-replication338
— Command without description in OpenAPIosc block-storage group failover-replication338
— Command without description in OpenAPIosc block-storage group list
— Returns a detailed list of groupsosc block-storage group list-replication-targets338
— Command without description in OpenAPIosc block-storage group reset-status320
— Command without description in OpenAPIosc block-storage group set313
— Update the grouposc block-storage group show
— Return data about the given group
osc block-storage group create313
Create a new group
Usage: osc block-storage group create313 [OPTIONS] --group-type <GROUP_TYPE>
Options:
-
--availability-zone <AVAILABILITY_ZONE>
— The name of the availability zone -
--description <DESCRIPTION>
— The group description -
--group-type <GROUP_TYPE>
— The group type ID -
--name <NAME>
— The group name -
--volume-types <VOLUME_TYPES>
— The list of volume types. In an environment with multiple-storage back ends, the scheduler determines where to send the volume based on the volume type. For information about how to use volume types to create multiple- storage back ends, see Configure multiple-storage back ends.Parameter is an array, may be provided multiple times.
osc block-storage group create-from-src314
Command without description in OpenAPI
Usage: osc block-storage group create-from-src314 [OPTIONS]
Options:
--description <DESCRIPTION>
--group-snapshot-id <GROUP_SNAPSHOT_ID>
--name <NAME>
--source-group-id <SOURCE_GROUP_ID>
osc block-storage group delete313
Command without description in OpenAPI
Usage: osc block-storage group delete313 [OPTIONS]
Options:
-
--delete-volumes <DELETE_VOLUMES>
Possible values:
true
,false
osc block-storage group disable-replication338
Command without description in OpenAPI
Usage: osc block-storage group disable-replication338 [OPTIONS]
Options:
--disable-replication <key=value>
osc block-storage group enable-replication338
Command without description in OpenAPI
Usage: osc block-storage group enable-replication338 [OPTIONS]
Options:
--enable-replication <key=value>
osc block-storage group failover-replication338
Command without description in OpenAPI
Usage: osc block-storage group failover-replication338 [OPTIONS]
Options:
-
--allow-attached-volume <ALLOW_ATTACHED_VOLUME>
Possible values:
true
,false
-
--secondary-backend-id <SECONDARY_BACKEND_ID>
osc block-storage group list
Returns a detailed list of groups
Usage: osc block-storage group list [OPTIONS]
Options:
-
--all-tenants <ALL_TENANTS>
— Shows details for all project. Admin onlyPossible values:
true
,false
-
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--offset <OFFSET>
— Used in conjunction with limit to return a slice of items. offset is where to start in the list -
--sort <SORT>
— Comma-separated list of sort keys and optional sort directions in the form of < key > [: < direction > ]. A valid direction is asc (ascending) or desc (descending) -
--sort-dir <SORT_DIR>
— Sorts by one or more sets of attribute and sort direction combinations. If you omit the sort direction in a set, default is desc. Deprecated in favour of the combined sort parameter -
--sort-key <SORT_KEY>
— Sorts by an attribute. A valid value is name, status, container_format, disk_format, size, id, created_at, or updated_at. Default is created_at. The API uses the natural sorting direction of the sort_key attribute value. Deprecated in favour of the combined sort parameter -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc block-storage group list-replication-targets338
Command without description in OpenAPI
Usage: osc block-storage group list-replication-targets338 [OPTIONS]
Options:
--list-replication-targets <key=value>
osc block-storage group reset-status320
Command without description in OpenAPI
Usage: osc block-storage group reset-status320 --status <STATUS>
Options:
--status <STATUS>
osc block-storage group set313
Update the group.
Expected format of the input parameter 'body':
{ "group": { "name": "my_group", "description": "My group", "add_volumes": "volume-uuid-1,volume-uuid-2,...", "remove_volumes": "volume-uuid-8,volume-uuid-9,..." } }
Usage: osc block-storage group set313 [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v3/groups/{id} API
Options:
--add-volumes <ADD_VOLUMES>
--description <DESCRIPTION>
--name <NAME>
--remove-volumes <REMOVE_VOLUMES>
osc block-storage group show
Return data about the given group
Usage: osc block-storage group show <ID>
Arguments:
<ID>
— id parameter for /v3/groups/{id} API
osc block-storage group-snapshot
GroupSnapshot snapshots (group_snapshots)
Lists all, lists all with details, shows details for, creates, and deletes group snapshots.
Usage: osc block-storage group-snapshot <COMMAND>
Available subcommands:
osc block-storage group-snapshot create314
— Create a new group_snapshotosc block-storage group-snapshot delete
— Delete a group_snapshotosc block-storage group-snapshot list
— Returns a detailed list of group_snapshotsosc block-storage group-snapshot reset-status319
— Command without description in OpenAPIosc block-storage group-snapshot show
— Return data about the given group_snapshot
osc block-storage group-snapshot create314
Create a new group_snapshot
Usage: osc block-storage group-snapshot create314 [OPTIONS] --group-id <GROUP_ID>
Options:
--description <DESCRIPTION>
— The group snapshot description--group-id <GROUP_ID>
— The ID of the group--name <NAME>
— The group snapshot name
osc block-storage group-snapshot delete
Delete a group_snapshot
Usage: osc block-storage group-snapshot delete <ID>
Arguments:
<ID>
— id parameter for /v3/group_snapshots/{id} API
osc block-storage group-snapshot list
Returns a detailed list of group_snapshots
Usage: osc block-storage group-snapshot list [OPTIONS]
Options:
-
--all-tenants <ALL_TENANTS>
— Shows details for all project. Admin onlyPossible values:
true
,false
-
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--offset <OFFSET>
— Used in conjunction with limit to return a slice of items. offset is where to start in the list -
--sort <SORT>
— Comma-separated list of sort keys and optional sort directions in the form of < key > [: < direction > ]. A valid direction is asc (ascending) or desc (descending) -
--sort-dir <SORT_DIR>
— Sorts by one or more sets of attribute and sort direction combinations. If you omit the sort direction in a set, default is desc. Deprecated in favour of the combined sort parameter -
--sort-key <SORT_KEY>
— Sorts by an attribute. A valid value is name, status, container_format, disk_format, size, id, created_at, or updated_at. Default is created_at. The API uses the natural sorting direction of the sort_key attribute value. Deprecated in favour of the combined sort parameter -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc block-storage group-snapshot reset-status319
Command without description in OpenAPI
Usage: osc block-storage group-snapshot reset-status319 --status <STATUS> <ID>
Arguments:
<ID>
— id parameter for /v3/group_snapshots/{id}/action API
Options:
--status <STATUS>
osc block-storage group-snapshot show
Return data about the given group_snapshot
Usage: osc block-storage group-snapshot show <ID>
Arguments:
<ID>
— id parameter for /v3/group_snapshots/{id} API
osc block-storage group-type
Group types (group_types)
To create a generic volume group, you must specify a group type.
Usage: osc block-storage group-type <COMMAND>
Available subcommands:
osc block-storage group-type create311
— Creates a new group typeosc block-storage group-type delete
— Deletes an existing group typeosc block-storage group-type group-spec
— Server metadataosc block-storage group-type list
— Returns the list of group typesosc block-storage group-type set311
— Command without description in OpenAPIosc block-storage group-type show
— Return a single group type item
osc block-storage group-type create311
Creates a new group type
Usage: osc block-storage group-type create311 [OPTIONS] --name <NAME>
Options:
-
--description <DESCRIPTION>
— The group type description -
--group-specs <key=value>
— A set of key and value pairs that contains the specifications for a group type -
--is-public <IS_PUBLIC>
— Whether the group type is publicly visible. See valid boolean valuesPossible values:
true
,false
-
--name <NAME>
— The group type name
osc block-storage group-type delete
Deletes an existing group type
Usage: osc block-storage group-type delete <ID>
Arguments:
<ID>
— id parameter for /v3/group_types/{id} API
osc block-storage group-type group-spec
Server metadata
Usage: osc block-storage group-type group-spec <COMMAND>
Available subcommands:
osc block-storage group-type group-spec create311
— Command without description in OpenAPIosc block-storage group-type group-spec delete
— Deletes an existing group specosc block-storage group-type group-spec list
— Returns the list of group specs for a given group typeosc block-storage group-type group-spec set311
— Command without description in OpenAPIosc block-storage group-type group-spec show
— Return a single extra spec item
osc block-storage group-type group-spec create311
Command without description in OpenAPI
Usage: osc block-storage group-type group-spec create311 [OPTIONS] <GROUP_TYPE_ID>
Arguments:
<GROUP_TYPE_ID>
— group_type_id parameter for /v3/group_types/{group_type_id}/group_specs/{id} API
Options:
--group-specs <key=value>
— A set of key and value pairs that contains the specifications for a group type
osc block-storage group-type group-spec delete
Deletes an existing group spec
Usage: osc block-storage group-type group-spec delete <GROUP_TYPE_ID> <ID>
Arguments:
<GROUP_TYPE_ID>
— group_type_id parameter for /v3/group_types/{group_type_id}/group_specs/{id} API<ID>
— id parameter for /v3/group_types/{group_type_id}/group_specs/{id} API
osc block-storage group-type group-spec list
Returns the list of group specs for a given group type
Usage: osc block-storage group-type group-spec list <GROUP_TYPE_ID>
Arguments:
<GROUP_TYPE_ID>
— group_type_id parameter for /v3/group_types/{group_type_id}/group_specs/{id} API
osc block-storage group-type group-spec set311
Command without description in OpenAPI
Usage: osc block-storage group-type group-spec set311 [OPTIONS] <GROUP_TYPE_ID> <ID>
Arguments:
<GROUP_TYPE_ID>
— group_type_id parameter for /v3/group_types/{group_type_id}/group_specs/{id} API<ID>
— id parameter for /v3/group_types/{group_type_id}/group_specs/{id} API
Options:
--property <key=value>
osc block-storage group-type group-spec show
Return a single extra spec item
Usage: osc block-storage group-type group-spec show <GROUP_TYPE_ID> <ID>
Arguments:
<GROUP_TYPE_ID>
— group_type_id parameter for /v3/group_types/{group_type_id}/group_specs/{id} API<ID>
— id parameter for /v3/group_types/{group_type_id}/group_specs/{id} API
osc block-storage group-type list
Returns the list of group types
Usage: osc block-storage group-type list [OPTIONS]
Options:
-
--all-tenants <ALL_TENANTS>
— Shows details for all project. Admin onlyPossible values:
true
,false
-
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--offset <OFFSET>
— Used in conjunction with limit to return a slice of items. offset is where to start in the list -
--sort <SORT>
— Comma-separated list of sort keys and optional sort directions in the form of < key > [: < direction > ]. A valid direction is asc (ascending) or desc (descending) -
--sort-dir <SORT_DIR>
— Sorts by one or more sets of attribute and sort direction combinations. If you omit the sort direction in a set, default is desc. Deprecated in favour of the combined sort parameter -
--sort-key <SORT_KEY>
— Sorts by an attribute. A valid value is name, status, container_format, disk_format, size, id, created_at, or updated_at. Default is created_at. The API uses the natural sorting direction of the sort_key attribute value. Deprecated in favour of the combined sort parameter -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc block-storage group-type set311
Command without description in OpenAPI
Usage: osc block-storage group-type set311 [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v3/group_types/{id} API
Options:
-
--description <DESCRIPTION>
-
--is-public <IS_PUBLIC>
Possible values:
true
,false
-
--name <NAME>
osc block-storage group-type show
Return a single group type item
Usage: osc block-storage group-type show <ID>
Arguments:
<ID>
— id parameter for /v3/group_types/{id} API
osc block-storage host
Hosts extension (os-hosts)
Administrators only, depending on policy settings.
Lists, shows hosts.
Usage: osc block-storage host <COMMAND>
Available subcommands:
osc block-storage host list
— Command without description in OpenAPIosc block-storage host show
— Shows the volume usage info given by hosts
osc block-storage host list
Command without description in OpenAPI
Usage: osc block-storage host list
osc block-storage host show
Shows the volume usage info given by hosts.
| | | | --- | --- | | param req: | security context | | param id: | hostname | | returns: | dict -- the host resources dictionary. ex.: {'host': [{'resource': D},..]} D: {'host': 'hostname','project': 'admin', 'volume_count': 1, 'total_volume_gb': 2048}
|
Usage: osc block-storage host show <ID>
Arguments:
<ID>
— id parameter for /v3/os-hosts/{id} API
osc block-storage limit
Limits (limits)
An absolute limit value of -1 indicates that the absolute limit for the item is infinite.
Usage: osc block-storage limit <COMMAND>
Available subcommands:
osc block-storage limit list
— Return all global and rate limit information
osc block-storage limit list
Return all global and rate limit information
Usage: osc block-storage limit list
osc block-storage message
Messages (messages)
Lists all, shows, and deletes messages. These are error messages generated by failed operations as a way to find out what happened when an asynchronous operation failed.
Usage: osc block-storage message <COMMAND>
Available subcommands:
osc block-storage message delete
— Delete a messageosc block-storage message list
— Returns a list of messages, transformed through view builderosc block-storage message show
— Return the given message
osc block-storage message delete
Delete a message
Usage: osc block-storage message delete <ID>
Arguments:
<ID>
— id parameter for /v3/messages/{id} API
osc block-storage message list
Returns a list of messages, transformed through view builder
Usage: osc block-storage message list [OPTIONS]
Options:
-
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--offset <OFFSET>
— Used in conjunction with limit to return a slice of items. offset is where to start in the list -
--sort <SORT>
— Comma-separated list of sort keys and optional sort directions in the form of < key > [: < direction > ]. A valid direction is asc (ascending) or desc (descending) -
--sort-dir <SORT_DIR>
— Sorts by one or more sets of attribute and sort direction combinations. If you omit the sort direction in a set, default is desc. Deprecated in favour of the combined sort parameterPossible values:
asc
,desc
-
--sort-key <SORT_KEY>
— Sorts by an attribute. A valid value is name, status, container_format, disk_format, size, id, created_at, or updated_at. Default is created_at. The API uses the natural sorting direction of the sort_key attribute value. Deprecated in favour of the combined sort parameter -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc block-storage message show
Return the given message
Usage: osc block-storage message show <ID>
Arguments:
<ID>
— id parameter for /v3/messages/{id} API
osc block-storage os-volume-transfer
Volume transfers
Transfers a volume from one user to another user.
Usage: osc block-storage os-volume-transfer <COMMAND>
Available subcommands:
osc block-storage os-volume-transfer accept
— Accept a new volume transferosc block-storage os-volume-transfer create
— Create a new volume transferosc block-storage os-volume-transfer delete
— Delete a transferosc block-storage os-volume-transfer list
— Returns a detailed list of transfersosc block-storage os-volume-transfer show
— Return data about active transfers
osc block-storage os-volume-transfer accept
Accept a new volume transfer
Usage: osc block-storage os-volume-transfer accept --auth-key <AUTH_KEY> <ID>
Arguments:
<ID>
— id parameter for /v3/os-volume-transfer/{id}/accept API
Options:
--auth-key <AUTH_KEY>
osc block-storage os-volume-transfer create
Create a new volume transfer
Usage: osc block-storage os-volume-transfer create [OPTIONS] --volume-id <VOLUME_ID>
Options:
--name <NAME>
— The name of the object--volume-id <VOLUME_ID>
— The UUID of the volume
osc block-storage os-volume-transfer delete
Delete a transfer
Usage: osc block-storage os-volume-transfer delete <ID>
Arguments:
<ID>
— id parameter for /v3/os-volume-transfer/{id} API
osc block-storage os-volume-transfer list
Returns a detailed list of transfers
Usage: osc block-storage os-volume-transfer list
osc block-storage os-volume-transfer show
Return data about active transfers
Usage: osc block-storage os-volume-transfer show <ID>
Arguments:
<ID>
— id parameter for /v3/os-volume-transfer/{id} API
osc block-storage qos-spec
Quality of service (QoS) specifications (qos-specs)
Administrators only, depending on policy settings.
Creates, lists, shows details for, associates, disassociates, sets keys, unsets keys, and deletes quality of service (QoS) specifications.
Usage: osc block-storage qos-spec <COMMAND>
Available subcommands:
osc block-storage qos-spec association
— Qos Spec associationosc block-storage qos-spec associate
— Associate a qos specs with a volume typeosc block-storage qos-spec create
— Command without description in OpenAPIosc block-storage qos-spec delete
— Deletes an existing qos specsosc block-storage qos-spec delete-keys
— Deletes specified keys in qos specsosc block-storage qos-spec disassociate
— Disassociate a qos specs from a volume typeosc block-storage qos-spec disassociate-all
— Disassociate a qos specs from all volume typesosc block-storage qos-spec list
— Returns the list of qos_specsosc block-storage qos-spec set
— Command without description in OpenAPIosc block-storage qos-spec show
— Return a single qos spec item
osc block-storage qos-spec association
Qos Spec association
Usage: osc block-storage qos-spec association <COMMAND>
Available subcommands:
osc block-storage qos-spec association list
— List all associations of given qos specs
osc block-storage qos-spec association list
List all associations of given qos specs
Usage: osc block-storage qos-spec association list <ID>
Arguments:
<ID>
— id parameter for /v3/qos-specs/{id}/associations API
osc block-storage qos-spec associate
Associate a qos specs with a volume type
Usage: osc block-storage qos-spec associate <ID>
Arguments:
<ID>
— id parameter for /v3/qos-specs/{id}/associate API
osc block-storage qos-spec create
Command without description in OpenAPI
Usage: osc block-storage qos-spec create --name <NAME>
Options:
--name <NAME>
— The name of the QoS specification
osc block-storage qos-spec delete
Deletes an existing qos specs
Usage: osc block-storage qos-spec delete <ID>
Arguments:
<ID>
— id parameter for /v3/qos-specs/{id} API
osc block-storage qos-spec delete-keys
Deletes specified keys in qos specs
Usage: osc block-storage qos-spec delete-keys [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v3/qos-specs/{id}/delete_keys API
Options:
--keys <KEYS>
— Parameter is an array, may be provided multiple times
osc block-storage qos-spec disassociate
Disassociate a qos specs from a volume type
Usage: osc block-storage qos-spec disassociate <ID>
Arguments:
<ID>
— id parameter for /v3/qos-specs/{id}/disassociate API
osc block-storage qos-spec disassociate-all
Disassociate a qos specs from all volume types
Usage: osc block-storage qos-spec disassociate-all <ID>
Arguments:
<ID>
— id parameter for /v3/qos-specs/{id}/disassociate_all API
osc block-storage qos-spec list
Returns the list of qos_specs
Usage: osc block-storage qos-spec list [OPTIONS]
Options:
-
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--offset <OFFSET>
— Used in conjunction with limit to return a slice of items. offset is where to start in the list -
--sort <SORT>
— Comma-separated list of sort keys and optional sort directions in the form of < key > [: < direction > ]. A valid direction is asc (ascending) or desc (descending) -
--sort-dir <SORT_DIR>
— Sorts by one or more sets of attribute and sort direction combinations. If you omit the sort direction in a set, default is desc. Deprecated in favour of the combined sort parameter -
--sort-key <SORT_KEY>
— Sorts by an attribute. A valid value is name, status, container_format, disk_format, size, id, created_at, or updated_at. Default is created_at. The API uses the natural sorting direction of the sort_key attribute value. Deprecated in favour of the combined sort parameter -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc block-storage qos-spec set
Command without description in OpenAPI
Usage: osc block-storage qos-spec set [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v3/qos-specs/{id} API
Options:
--qos-specs <key=value>
osc block-storage qos-spec show
Return a single qos spec item
Usage: osc block-storage qos-spec show <ID>
Arguments:
<ID>
— id parameter for /v3/qos-specs/{id} API
osc block-storage service
Services (os-services)
Administrator only. Lists all Cinder services, enables or disables a Cinder service, freeze or thaw the specified cinder-volume host, failover a replicating cinder-volume host.
Usage: osc block-storage service <COMMAND>
Available subcommands:
osc block-storage service list
— Return a list of all running services
osc block-storage service list
Return a list of all running services.
Filter by host & service name.
Usage: osc block-storage service list
osc block-storage snapshot
Volume snapshots (snapshots)
A snapshot is a point-in-time copy of the data that a volume contains.
When you create, list, or delete snapshots, these status values are possible:
-
creating: The snapshot is being created.
-
available: The snapshot is ready to use.
-
backing-up: The snapshot is being backed up.
-
deleting: The snapshot is being deleted.
-
error: A snapshot creation error occurred.
-
deleted: The snapshot has been deleted.
-
unmanaging: The snapshot is being unmanaged.
-
restoring: The snapshot is being restored to a volume.
-
error_deleting: A snapshot deletion error occurred.
Usage: osc block-storage snapshot <COMMAND>
Available subcommands:
osc block-storage snapshot create
— Creates a new snapshotosc block-storage snapshot delete
— Delete a snapshotosc block-storage snapshot force-delete
— Empty body for os-force_delete actionosc block-storage snapshot list
— Returns a detailed list of snapshotsosc block-storage snapshot reset-status
— Empty body for os-reset_status actionosc block-storage snapshot set
— Update a snapshotosc block-storage snapshot show
— Return data about the given snapshotosc block-storage snapshot unmanage
— Empty body for os-unmanage actionosc block-storage snapshot update-status
— Command without description in OpenAPI
osc block-storage snapshot create
Creates a new snapshot
Usage: osc block-storage snapshot create [OPTIONS] --volume-id <VOLUME_ID>
Options:
-
--description <DESCRIPTION>
— A description for the snapshot. Default isNone
-
--display-name <DISPLAY_NAME>
— The name of the snapshot -
--force <FORCE>
— Indicates whether to snapshot, even if the volume is attached. Default isfalse
. See valid boolean valuesPossible values:
true
,false
-
--metadata <key=value>
— One or more metadata key and value pairs for the snapshot -
--name <NAME>
— The name of the snapshot -
--volume-id <VOLUME_ID>
— The UUID of the volume
osc block-storage snapshot delete
Delete a snapshot
Usage: osc block-storage snapshot delete <ID>
Arguments:
<ID>
— id parameter for /v3/snapshots/{id} API
osc block-storage snapshot force-delete
Empty body for os-force_delete action
Usage: osc block-storage snapshot force-delete <ID>
Arguments:
<ID>
— id parameter for /v3/snapshots/{id}/action API
osc block-storage snapshot list
Returns a detailed list of snapshots
Usage: osc block-storage snapshot list [OPTIONS]
Options:
-
--all-tenants <ALL_TENANTS>
— Shows details for all project. Admin onlyPossible values:
true
,false
-
--consumes-quota <CONSUMES_QUOTA>
— Filters results by consumes_quota field. Resources that don’t use quotas are usually temporary internal resources created to perform an operation. Default is to not filter by it. Filtering by this option may not be always possible in a cloud, see List Resource Filters to determine whether this filter is available in your cloudPossible values:
true
,false
-
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--offset <OFFSET>
— Used in conjunction with limit to return a slice of items. offset is where to start in the list -
--sort <SORT>
— Comma-separated list of sort keys and optional sort directions in the form of < key > [: < direction > ]. A valid direction is asc (ascending) or desc (descending) -
--sort-dir <SORT_DIR>
— Sorts by one or more sets of attribute and sort direction combinations. If you omit the sort direction in a set, default is desc. Deprecated in favour of the combined sort parameterPossible values:
asc
,desc
-
--sort-key <SORT_KEY>
— Sorts by an attribute. A valid value is name, status, container_format, disk_format, size, id, created_at, or updated_at. Default is created_at. The API uses the natural sorting direction of the sort_key attribute value. Deprecated in favour of the combined sort parameter -
--with-count <WITH_COUNT>
— Whether to show count in API response or not, default is FalsePossible values:
true
,false
-
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc block-storage snapshot reset-status
Empty body for os-reset_status action
Usage: osc block-storage snapshot reset-status <ID>
Arguments:
<ID>
— id parameter for /v3/snapshots/{id}/action API
osc block-storage snapshot set
Update a snapshot
Usage: osc block-storage snapshot set [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v3/snapshots/{id} API
Options:
--description <DESCRIPTION>
--display-description <DISPLAY_DESCRIPTION>
--display-name <DISPLAY_NAME>
--name <NAME>
osc block-storage snapshot show
Return data about the given snapshot
Usage: osc block-storage snapshot show <ID>
Arguments:
<ID>
— id parameter for /v3/snapshots/{id} API
osc block-storage snapshot unmanage
Empty body for os-unmanage action
Usage: osc block-storage snapshot unmanage <ID>
Arguments:
<ID>
— id parameter for /v3/snapshots/{id}/action API
osc block-storage snapshot update-status
Command without description in OpenAPI
Usage: osc block-storage snapshot update-status [OPTIONS] --status <STATUS> <ID>
Arguments:
<ID>
— id parameter for /v3/snapshots/{id}/action API
Options:
--progress <PROGRESS>
--status <STATUS>
osc block-storage snapshot-manage
SnapshotManage manage extension (manageable_snapshots)
Creates or lists snapshots by using existing storage instead of allocating new storage.
Usage: osc block-storage snapshot-manage <COMMAND>
Available subcommands:
osc block-storage snapshot-manage create
— Instruct Cinder to manage a storage snapshot objectosc block-storage snapshot-manage list
— Returns a detailed list of snapshots available to manage
osc block-storage snapshot-manage create
Instruct Cinder to manage a storage snapshot object.
Manages an existing backend storage snapshot object (e.g. a Linux logical volume or a SAN disk) by creating the Cinder objects required to manage it, and possibly renaming the backend storage snapshot object (driver dependent).
From an API perspective, this operation behaves very much like a snapshot creation operation.
Required HTTP Body:
{ "snapshot": { "volume_id": "<Cinder volume already exists in volume backend>", "ref": "<Driver-specific reference to the existing storage object>" } }
See the appropriate Cinder drivers' implementations of the manage_snapshot method to find out the accepted format of 'ref'. For example,in LVM driver, it will be the logic volume name of snapshot which you want to manage.
This API call will return with an error if any of the above elements are missing from the request, or if the 'volume_id' element refers to a cinder volume that could not be found.
The snapshot will later enter the error state if it is discovered that 'ref' is bad.
Optional elements to 'snapshot' are:
name A name for the new snapshot. description A description for the new snapshot. metadata Key/value pairs to be associated with the new snapshot.
Usage: osc block-storage snapshot-manage create [OPTIONS] --volume-id <VOLUME_ID>
Options:
--description <DESCRIPTION>
--metadata <key=value>
--name <NAME>
--ref <JSON>
--volume-id <VOLUME_ID>
osc block-storage snapshot-manage list
Returns a detailed list of snapshots available to manage
Usage: osc block-storage snapshot-manage list
osc block-storage resource-filter
Resource filters
Lists all resource filters, available since microversion 3.33.
Usage: osc block-storage resource-filter <COMMAND>
Available subcommands:
osc block-storage resource-filter list
— Return a list of resource filters
osc block-storage resource-filter list
Return a list of resource filters
Usage: osc block-storage resource-filter list
osc block-storage type
Block Storage VolumeType type commands
To create an environment with multiple-storage back ends, you must specify a volume type. The API spawns Block Storage volume back ends as children to cinder-volume, and keys them from a unique queue. The API names the back ends cinder-volume.HOST.BACKEND. For example, cinder-volume.ubuntu.lvmdriver. When you create a volume, the scheduler chooses an appropriate back end for the volume type to handle the request.
For information about how to use volume types to create multiple- storage back ends, see Configure multiple-storage back ends.
Usage: osc block-storage type <COMMAND>
Available subcommands:
osc block-storage type add-project-access
— Command without description in OpenAPIosc block-storage type create
— Command without description in OpenAPIosc block-storage type delete
— Deletes an existing volume typeosc block-storage type encryption
— Volume Type Encryption commandsosc block-storage type extraspecs
— Type extra specsosc block-storage type list
— Returns the list of volume typesosc block-storage type remove-project-access
— Command without description in OpenAPIosc block-storage type set
— Command without description in OpenAPIosc block-storage type show
— Return a single volume type item
osc block-storage type add-project-access
Command without description in OpenAPI
Usage: osc block-storage type add-project-access --project <PROJECT> <ID>
Arguments:
<ID>
— id parameter for /v3/types/{id}/action API
Options:
--project <PROJECT>
osc block-storage type create
Command without description in OpenAPI
Usage: osc block-storage type create [OPTIONS] --name <NAME> <ID>
Arguments:
<ID>
— id parameter for /v3/types/{id}/action API
Options:
-
--description <DESCRIPTION>
-
--extra-specs <key=value>
-
--name <NAME>
-
--os-volume-type-access-is-public <OS_VOLUME_TYPE_ACCESS_IS_PUBLIC>
Possible values:
true
,false
osc block-storage type delete
Deletes an existing volume type
Usage: osc block-storage type delete <ID>
Arguments:
<ID>
— id parameter for /v3/types/{id} API
osc block-storage type encryption
Volume Type Encryption commands
Block Storage volume type assignment provides scheduling to a specific back-end, and can be used to specify actionable information for a back-end storage device.
Usage: osc block-storage type encryption <COMMAND>
Available subcommands:
osc block-storage type encryption create
— Create encryption specs for an existing volume typeosc block-storage type encryption delete
— Delete encryption specs for a given volume typeosc block-storage type encryption list
— Returns the encryption specs for a given volume typeosc block-storage type encryption set
— Update encryption specs for a given volume typeosc block-storage type encryption show
— Return a single encryption item
osc block-storage type encryption create
Create encryption specs for an existing volume type
Usage: osc block-storage type encryption create [OPTIONS] --control-location <CONTROL_LOCATION> --provider <PROVIDER> <TYPE_ID>
Arguments:
<TYPE_ID>
— type_id parameter for /v3/types/{type_id}/encryption/{id} API
Options:
-
--cipher <CIPHER>
-
--control-location <CONTROL_LOCATION>
Possible values:
back-end
,front-end
-
--key-size <KEY_SIZE>
-
--provider <PROVIDER>
osc block-storage type encryption delete
Delete encryption specs for a given volume type
Usage: osc block-storage type encryption delete <TYPE_ID> <ID>
Arguments:
<TYPE_ID>
— type_id parameter for /v3/types/{type_id}/encryption/{id} API<ID>
— id parameter for /v3/types/{type_id}/encryption/{id} API
osc block-storage type encryption list
Returns the encryption specs for a given volume type
Usage: osc block-storage type encryption list <TYPE_ID>
Arguments:
<TYPE_ID>
— type_id parameter for /v3/types/{type_id}/encryption/{id} API
osc block-storage type encryption set
Update encryption specs for a given volume type
Usage: osc block-storage type encryption set [OPTIONS] <TYPE_ID> <ID>
Arguments:
<TYPE_ID>
— type_id parameter for /v3/types/{type_id}/encryption/{id} API<ID>
— id parameter for /v3/types/{type_id}/encryption/{id} API
Options:
-
--cipher <CIPHER>
-
--control-location <CONTROL_LOCATION>
Possible values:
back-end
,front-end
-
--key-size <KEY_SIZE>
-
--provider <PROVIDER>
osc block-storage type encryption show
Return a single encryption item
Usage: osc block-storage type encryption show <TYPE_ID> <ID>
Arguments:
<TYPE_ID>
— type_id parameter for /v3/types/{type_id}/encryption/{id} API<ID>
— id parameter for /v3/types/{type_id}/encryption/{id} API
osc block-storage type extraspecs
Type extra specs
Usage: osc block-storage type extraspecs <COMMAND>
Available subcommands:
osc block-storage type extraspecs create
— Command without description in OpenAPIosc block-storage type extraspecs delete
— Deletes an existing extra specosc block-storage type extraspecs list
— Returns the list of extra specs for a given volume typeosc block-storage type extraspecs show
— Return a single extra spec itemosc block-storage type extraspecs set
— Command without description in OpenAPI
osc block-storage type extraspecs create
Command without description in OpenAPI
Usage: osc block-storage type extraspecs create [OPTIONS] <TYPE_ID>
Arguments:
<TYPE_ID>
— type_id parameter for /v3/types/{type_id}/extra_specs/{id} API
Options:
--extra-specs <key=value>
osc block-storage type extraspecs delete
Deletes an existing extra spec
Usage: osc block-storage type extraspecs delete <TYPE_ID> <ID>
Arguments:
<TYPE_ID>
— type_id parameter for /v3/types/{type_id}/extra_specs/{id} API<ID>
— id parameter for /v3/types/{type_id}/extra_specs/{id} API
osc block-storage type extraspecs list
Returns the list of extra specs for a given volume type
Usage: osc block-storage type extraspecs list <TYPE_ID>
Arguments:
<TYPE_ID>
— type_id parameter for /v3/types/{type_id}/extra_specs/{id} API
osc block-storage type extraspecs show
Return a single extra spec item
Usage: osc block-storage type extraspecs show <TYPE_ID> <ID>
Arguments:
<TYPE_ID>
— type_id parameter for /v3/types/{type_id}/extra_specs/{id} API<ID>
— id parameter for /v3/types/{type_id}/extra_specs/{id} API
osc block-storage type extraspecs set
Command without description in OpenAPI
Usage: osc block-storage type extraspecs set [OPTIONS] <TYPE_ID> <ID>
Arguments:
<TYPE_ID>
— type_id parameter for /v3/types/{type_id}/extra_specs/{id} API<ID>
— id parameter for /v3/types/{type_id}/extra_specs/{id} API
Options:
--property <key=value>
osc block-storage type list
Returns the list of volume types
Usage: osc block-storage type list
osc block-storage type remove-project-access
Command without description in OpenAPI
Usage: osc block-storage type remove-project-access --project <PROJECT> <ID>
Arguments:
<ID>
— id parameter for /v3/types/{id}/action API
Options:
--project <PROJECT>
osc block-storage type set
Command without description in OpenAPI
Usage: osc block-storage type set [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v3/types/{id} API
Options:
-
--description <DESCRIPTION>
-
--is-public <IS_PUBLIC>
Possible values:
true
,false
-
--name <NAME>
osc block-storage type show
Return a single volume type item
Usage: osc block-storage type show <ID>
Arguments:
<ID>
— id parameter for /v3/types/{id} API
osc block-storage volume
Block Storage Volume commands
Usage: osc block-storage volume <COMMAND>
Available subcommands:
osc block-storage volume create353
— Creates a new volumeosc block-storage volume create347
— Creates a new volumeosc block-storage volume create313
— Creates a new volumeosc block-storage volume create30
— Creates a new volumeosc block-storage volume delete
— Delete a volumeosc block-storage volume extend
— Command without description in OpenAPIosc block-storage volume list
— Returns a detailed list of volumesosc block-storage volume metadata
— Volume metadataosc block-storage volume set353
— Update a volumeosc block-storage volume set30
— Update a volumeosc block-storage volume show
— Return data about the given volume
osc block-storage volume create353
Creates a new volume.
| | | | --- | --- | | param req: | the request | | param body: | the request body | | returns: | dict -- the new volume dictionary | | raises HTTPNotFound, HTTPBadRequest: | | | | |
Usage: osc block-storage volume create353 [OPTIONS]
Options:
-
--os-sch-hnt-scheduler-hints <key=value>
— The dictionary of data to send to the scheduler -
--availability-zone <AVAILABILITY_ZONE>
— The name of the availability zone -
--backup-id <BACKUP_ID>
— The UUID of the backup.New in version 3.47
-
--consistencygroup-id <CONSISTENCYGROUP_ID>
— The UUID of the consistency group -
--description <DESCRIPTION>
— The volume description -
--display-description <DISPLAY_DESCRIPTION>
-
--display-name <DISPLAY_NAME>
-
--group-id <GROUP_ID>
-
--image-id <IMAGE_ID>
-
--image-ref <IMAGE_REF>
— The UUID of the image from which you want to create the volume. Required to create a bootable volume.New in version 3.46: Instead of directly consuming a zero-byte image that has been created by the Compute service when an instance snapshot was requested, the Block Storage service will use the
snapshot_id
contained in theblock_device_mapping
image property to locate the volume snapshot, and will use that to create the volume instead. -
--metadata <key=value>
— One or more metadata key and value pairs to be associated with the new volume -
--multiattach <MULTIATTACH>
Possible values:
true
,false
-
--name <NAME>
— The volume name -
--size <SIZE>
— The size of the volume, in gibibytes (GiB) -
--snapshot-id <SNAPSHOT_ID>
— The UUID of the consistency group -
--source-volid <SOURCE_VOLID>
— The UUID of the consistency group -
--volume-type <VOLUME_TYPE>
— The volume type (either name or ID). To create an environment with multiple-storage back ends, you must specify a volume type. Block Storage volume back ends are spawned as children tocinder- volume
, and they are keyed from a unique queue. They are namedcinder- volume.HOST.BACKEND
. For example,cinder- volume.ubuntu.lvmdriver
. When a volume is created, the scheduler chooses an appropriate back end to handle the request based on the volume type. Default isNone
. For information about how to use volume types to create multiple- storage back ends, see Configure multiple-storage back ends
osc block-storage volume create347
Creates a new volume.
| | | | --- | --- | | param req: | the request | | param body: | the request body | | returns: | dict -- the new volume dictionary | | raises HTTPNotFound, HTTPBadRequest: | | | | |
Usage: osc block-storage volume create347 [OPTIONS]
Options:
-
--os-sch-hnt-scheduler-hints <key=value>
— The dictionary of data to send to the scheduler -
--availability-zone <AVAILABILITY_ZONE>
— The name of the availability zone -
--backup-id <BACKUP_ID>
— The UUID of the backup.New in version 3.47
-
--consistencygroup-id <CONSISTENCYGROUP_ID>
— The UUID of the consistency group -
--description <DESCRIPTION>
— The volume description -
--display-description <DISPLAY_DESCRIPTION>
-
--display-name <DISPLAY_NAME>
-
--group-id <GROUP_ID>
-
--image-id <IMAGE_ID>
-
--image-ref <IMAGE_REF>
— The UUID of the image from which you want to create the volume. Required to create a bootable volume.New in version 3.46: Instead of directly consuming a zero-byte image that has been created by the Compute service when an instance snapshot was requested, the Block Storage service will use the
snapshot_id
contained in theblock_device_mapping
image property to locate the volume snapshot, and will use that to create the volume instead. -
--metadata <key=value>
— One or more metadata key and value pairs to be associated with the new volume -
--multiattach <MULTIATTACH>
Possible values:
true
,false
-
--name <NAME>
— The volume name -
--size <SIZE>
— The size of the volume, in gibibytes (GiB) -
--snapshot-id <SNAPSHOT_ID>
— The UUID of the consistency group -
--source-volid <SOURCE_VOLID>
— The UUID of the consistency group -
--volume-type <VOLUME_TYPE>
— The volume type (either name or ID). To create an environment with multiple-storage back ends, you must specify a volume type. Block Storage volume back ends are spawned as children tocinder- volume
, and they are keyed from a unique queue. They are namedcinder- volume.HOST.BACKEND
. For example,cinder- volume.ubuntu.lvmdriver
. When a volume is created, the scheduler chooses an appropriate back end to handle the request based on the volume type. Default isNone
. For information about how to use volume types to create multiple- storage back ends, see Configure multiple-storage back ends
osc block-storage volume create313
Creates a new volume.
| | | | --- | --- | | param req: | the request | | param body: | the request body | | returns: | dict -- the new volume dictionary | | raises HTTPNotFound, HTTPBadRequest: | | | | |
Usage: osc block-storage volume create313 [OPTIONS]
Options:
-
--os-sch-hnt-scheduler-hints <key=value>
— The dictionary of data to send to the scheduler -
--availability-zone <AVAILABILITY_ZONE>
— The name of the availability zone -
--consistencygroup-id <CONSISTENCYGROUP_ID>
— The UUID of the consistency group -
--description <DESCRIPTION>
— The volume description -
--display-description <DISPLAY_DESCRIPTION>
-
--display-name <DISPLAY_NAME>
-
--group-id <GROUP_ID>
-
--image-id <IMAGE_ID>
-
--image-ref <IMAGE_REF>
— The UUID of the image from which you want to create the volume. Required to create a bootable volume.New in version 3.46: Instead of directly consuming a zero-byte image that has been created by the Compute service when an instance snapshot was requested, the Block Storage service will use the
snapshot_id
contained in theblock_device_mapping
image property to locate the volume snapshot, and will use that to create the volume instead. -
--metadata <key=value>
— One or more metadata key and value pairs to be associated with the new volume -
--multiattach <MULTIATTACH>
Possible values:
true
,false
-
--name <NAME>
— The volume name -
--size <SIZE>
— The size of the volume, in gibibytes (GiB) -
--snapshot-id <SNAPSHOT_ID>
— The UUID of the consistency group -
--source-volid <SOURCE_VOLID>
— The UUID of the consistency group -
--volume-type <VOLUME_TYPE>
— The volume type (either name or ID). To create an environment with multiple-storage back ends, you must specify a volume type. Block Storage volume back ends are spawned as children tocinder- volume
, and they are keyed from a unique queue. They are namedcinder- volume.HOST.BACKEND
. For example,cinder- volume.ubuntu.lvmdriver
. When a volume is created, the scheduler chooses an appropriate back end to handle the request based on the volume type. Default isNone
. For information about how to use volume types to create multiple- storage back ends, see Configure multiple-storage back ends
osc block-storage volume create30
Creates a new volume.
| | | | --- | --- | | param req: | the request | | param body: | the request body | | returns: | dict -- the new volume dictionary | | raises HTTPNotFound, HTTPBadRequest: | | | | |
Usage: osc block-storage volume create30 [OPTIONS]
Options:
-
--os-sch-hnt-scheduler-hints <key=value>
— The dictionary of data to send to the scheduler -
--availability-zone <AVAILABILITY_ZONE>
— The name of the availability zone -
--consistencygroup-id <CONSISTENCYGROUP_ID>
— The UUID of the consistency group -
--description <DESCRIPTION>
— The volume description -
--display-description <DISPLAY_DESCRIPTION>
-
--display-name <DISPLAY_NAME>
-
--image-id <IMAGE_ID>
-
--image-ref <IMAGE_REF>
— The UUID of the image from which you want to create the volume. Required to create a bootable volume.New in version 3.46: Instead of directly consuming a zero-byte image that has been created by the Compute service when an instance snapshot was requested, the Block Storage service will use the
snapshot_id
contained in theblock_device_mapping
image property to locate the volume snapshot, and will use that to create the volume instead. -
--metadata <key=value>
— One or more metadata key and value pairs to be associated with the new volume -
--multiattach <MULTIATTACH>
Possible values:
true
,false
-
--name <NAME>
— The volume name -
--size <SIZE>
— The size of the volume, in gibibytes (GiB) -
--snapshot-id <SNAPSHOT_ID>
— The UUID of the consistency group -
--source-volid <SOURCE_VOLID>
— The UUID of the consistency group -
--volume-type <VOLUME_TYPE>
— The volume type (either name or ID). To create an environment with multiple-storage back ends, you must specify a volume type. Block Storage volume back ends are spawned as children tocinder- volume
, and they are keyed from a unique queue. They are namedcinder- volume.HOST.BACKEND
. For example,cinder- volume.ubuntu.lvmdriver
. When a volume is created, the scheduler chooses an appropriate back end to handle the request based on the volume type. Default isNone
. For information about how to use volume types to create multiple- storage back ends, see Configure multiple-storage back ends
osc block-storage volume delete
Delete a volume
Usage: osc block-storage volume delete <ID>
Arguments:
<ID>
— id parameter for /v3/volumes/{id} API
osc block-storage volume extend
Command without description in OpenAPI
Usage: osc block-storage volume extend --new-size <NEW_SIZE> <ID>
Arguments:
<ID>
— id parameter for /v3/volumes/{id}/action API
Options:
--new-size <NEW_SIZE>
osc block-storage volume list
Returns a detailed list of volumes
Usage: osc block-storage volume list [OPTIONS]
Options:
-
--all-tenants <ALL_TENANTS>
— Shows details for all project. Admin onlyPossible values:
true
,false
-
--consumes-quota <CONSUMES_QUOTA>
— Filters results by consumes_quota field. Resources that don’t use quotas are usually temporary internal resources created to perform an operation. Default is to not filter by it. Filtering by this option may not be always possible in a cloud, see List Resource Filters to determine whether this filter is available in your cloudPossible values:
true
,false
-
--created-at <CREATED_AT>
— Filters reuslts by a time that resources are created at with time comparison operators: gt/gte/eq/neq/lt/lte -
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--offset <OFFSET>
— Used in conjunction with limit to return a slice of items. offset is where to start in the list -
--sort <SORT>
— Comma-separated list of sort keys and optional sort directions in the form of < key > [: < direction > ]. A valid direction is asc (ascending) or desc (descending) -
--sort-dir <SORT_DIR>
— Sorts by one or more sets of attribute and sort direction combinations. If you omit the sort direction in a set, default is desc. Deprecated in favour of the combined sort parameterPossible values:
asc
,desc
-
--sort-key <SORT_KEY>
— Sorts by an attribute. A valid value is name, status, container_format, disk_format, size, id, created_at, or updated_at. Default is created_at. The API uses the natural sorting direction of the sort_key attribute value. Deprecated in favour of the combined sort parameter -
--updated-at <UPDATED_AT>
— Filters reuslts by a time that resources are updated at with time comparison operators: gt/gte/eq/neq/lt/lte -
--with-count <WITH_COUNT>
— Whether to show count in API response or not, default is FalsePossible values:
true
,false
-
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc block-storage volume metadata
Volume metadata
Lists metadata, creates or replaces one or more metadata items, and updates one or more metadata items for a volume.
Usage: osc block-storage volume metadata <COMMAND>
Available subcommands:
osc block-storage volume metadata create
— Command without description in OpenAPIosc block-storage volume metadata delete
— Deletes an existing metadataosc block-storage volume metadata list
— Returns the list of metadata for a given volumeosc block-storage volume metadata replace
— Command without description in OpenAPIosc block-storage volume metadata set
— Command without description in OpenAPIosc block-storage volume metadata show
— Return a single metadata item
osc block-storage volume metadata create
Command without description in OpenAPI
Usage: osc block-storage volume metadata create [OPTIONS] <VOLUME_ID>
Arguments:
<VOLUME_ID>
— volume_id parameter for /v3/volumes/{volume_id}/metadata API
Options:
--metadata <key=value>
— One or more metadata key and value pairs that are associated with the volume
osc block-storage volume metadata delete
Deletes an existing metadata
Usage: osc block-storage volume metadata delete <VOLUME_ID> <ID>
Arguments:
<VOLUME_ID>
— volume_id parameter for /v3/volumes/{volume_id}/metadata API<ID>
— id parameter for /v3/volumes/{volume_id}/metadata/{id} API
osc block-storage volume metadata list
Returns the list of metadata for a given volume
Usage: osc block-storage volume metadata list <VOLUME_ID>
Arguments:
<VOLUME_ID>
— volume_id parameter for /v3/volumes/{volume_id}/metadata API
osc block-storage volume metadata replace
Command without description in OpenAPI
Usage: osc block-storage volume metadata replace [OPTIONS] <VOLUME_ID>
Arguments:
<VOLUME_ID>
— volume_id parameter for /v3/volumes/{volume_id}/metadata API
Options:
--metadata <key=value>
— One or more metadata key and value pairs that are associated with the volume
osc block-storage volume metadata set
Command without description in OpenAPI
Usage: osc block-storage volume metadata set [OPTIONS] <VOLUME_ID> <ID>
Arguments:
<VOLUME_ID>
— volume_id parameter for /v3/volumes/{volume_id}/metadata API<ID>
— id parameter for /v3/volumes/{volume_id}/metadata/{id} API
Options:
--meta <key=value>
osc block-storage volume metadata show
Return a single metadata item
Usage: osc block-storage volume metadata show <VOLUME_ID> <ID>
Arguments:
<VOLUME_ID>
— volume_id parameter for /v3/volumes/{volume_id}/metadata API<ID>
— id parameter for /v3/volumes/{volume_id}/metadata/{id} API
osc block-storage volume set353
Update a volume
Usage: osc block-storage volume set353 [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v3/volumes/{id} API
Options:
--description <DESCRIPTION>
--display-description <DISPLAY_DESCRIPTION>
--display-name <DISPLAY_NAME>
--metadata <key=value>
--name <NAME>
osc block-storage volume set30
Update a volume
Usage: osc block-storage volume set30 [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v3/volumes/{id} API
Options:
--description <DESCRIPTION>
--display-description <DISPLAY_DESCRIPTION>
--display-name <DISPLAY_NAME>
--metadata <key=value>
--name <NAME>
osc block-storage volume show
Return data about the given volume
Usage: osc block-storage volume show <ID>
Arguments:
<ID>
— id parameter for /v3/volumes/{id} API
osc block-storage volume-manage
Volume manage extension (manageable_volumes)
Creates or lists volumes by using existing storage instead of allocating new storage.
Usage: osc block-storage volume-manage <COMMAND>
Available subcommands:
osc block-storage volume-manage create316
— Instruct Cinder to manage a storage objectosc block-storage volume-manage create30
— Instruct Cinder to manage a storage objectosc block-storage volume-manage list
— Returns a detailed list of volumes available to manage
osc block-storage volume-manage create316
Instruct Cinder to manage a storage object.
Manages an existing backend storage object (e.g. a Linux logical volume or a SAN disk) by creating the Cinder objects required to manage it, and possibly renaming the backend storage object (driver dependent)
From an API perspective, this operation behaves very much like a volume creation operation, except that properties such as image, snapshot and volume references don't make sense, because we are taking an existing storage object into Cinder management.
Required HTTP Body:
{ "volume": { "host": "<Cinder host on which the existing storage resides>", "cluster": "<Cinder cluster on which the storage resides>", "ref": "<Driver-specific reference to existing storage object>" } }
See the appropriate Cinder drivers' implementations of the manage_volume method to find out the accepted format of 'ref'.
This API call will return with an error if any of the above elements are missing from the request, or if the 'host' element refers to a cinder host that is not registered.
The volume will later enter the error state if it is discovered that 'ref' is bad.
Optional elements to 'volume' are:
name A name for the new volume. description A description for the new volume. volume_type ID or name of a volume type to associate with the new Cinder volume. Does not necessarily guarantee that the managed volume will have the properties described in the volume_type. The driver may choose to fail if it identifies that the specified volume_type is not compatible with the backend storage object. metadata Key/value pairs to be associated with the new volume. availability_zone The availability zone to associate with the new volume. bootable If set to True, marks the volume as bootable.
Usage: osc block-storage volume-manage create316 [OPTIONS] --ref <JSON>
Options:
-
--availability-zone <AVAILABILITY_ZONE>
-
--bootable <BOOTABLE>
Possible values:
true
,false
-
--cluster <CLUSTER>
-
--description <DESCRIPTION>
-
--host <HOST>
-
--metadata <key=value>
-
--name <NAME>
-
--ref <JSON>
-
--volume-type <VOLUME_TYPE>
osc block-storage volume-manage create30
Instruct Cinder to manage a storage object.
Manages an existing backend storage object (e.g. a Linux logical volume or a SAN disk) by creating the Cinder objects required to manage it, and possibly renaming the backend storage object (driver dependent)
From an API perspective, this operation behaves very much like a volume creation operation, except that properties such as image, snapshot and volume references don't make sense, because we are taking an existing storage object into Cinder management.
Required HTTP Body:
{ "volume": { "host": "<Cinder host on which the existing storage resides>", "cluster": "<Cinder cluster on which the storage resides>", "ref": "<Driver-specific reference to existing storage object>" } }
See the appropriate Cinder drivers' implementations of the manage_volume method to find out the accepted format of 'ref'.
This API call will return with an error if any of the above elements are missing from the request, or if the 'host' element refers to a cinder host that is not registered.
The volume will later enter the error state if it is discovered that 'ref' is bad.
Optional elements to 'volume' are:
name A name for the new volume. description A description for the new volume. volume_type ID or name of a volume type to associate with the new Cinder volume. Does not necessarily guarantee that the managed volume will have the properties described in the volume_type. The driver may choose to fail if it identifies that the specified volume_type is not compatible with the backend storage object. metadata Key/value pairs to be associated with the new volume. availability_zone The availability zone to associate with the new volume. bootable If set to True, marks the volume as bootable.
Usage: osc block-storage volume-manage create30 [OPTIONS] --ref <JSON>
Options:
-
--availability-zone <AVAILABILITY_ZONE>
-
--bootable <BOOTABLE>
Possible values:
true
,false
-
--description <DESCRIPTION>
-
--host <HOST>
-
--metadata <key=value>
-
--name <NAME>
-
--ref <JSON>
-
--volume-type <VOLUME_TYPE>
osc block-storage volume-manage list
Returns a detailed list of volumes available to manage
Usage: osc block-storage volume-manage list
osc block-storage volume-transfer
Volume transfers (volume-transfers) (3.55 or later)
Transfers a volume from one user to another user. This is the new transfer APIs with microversion 3.55.
Usage: osc block-storage volume-transfer <COMMAND>
Available subcommands:
osc block-storage volume-transfer accept
— Accept a new volume transferosc block-storage volume-transfer create355
— Create a new volume transferosc block-storage volume-transfer delete
— Delete a transferosc block-storage volume-transfer list
— Returns a detailed list of transfersosc block-storage volume-transfer show
— Return data about active transfers
osc block-storage volume-transfer accept
Accept a new volume transfer
Usage: osc block-storage volume-transfer accept --auth-key <AUTH_KEY> <ID>
Arguments:
<ID>
— id parameter for /v3/volume-transfers/{id}/accept API
Options:
--auth-key <AUTH_KEY>
osc block-storage volume-transfer create355
Create a new volume transfer
Usage: osc block-storage volume-transfer create355 [OPTIONS] --volume-id <VOLUME_ID>
Options:
-
--name <NAME>
— The name of the object -
--no-snapshots <NO_SNAPSHOTS>
— Transfer volume without snapshots. Defaults to False if not specified.New in version 3.55
Possible values:
true
,false
-
--volume-id <VOLUME_ID>
— The UUID of the volume
osc block-storage volume-transfer delete
Delete a transfer
Usage: osc block-storage volume-transfer delete <ID>
Arguments:
<ID>
— id parameter for /v3/volume-transfers/{id} API
osc block-storage volume-transfer list
Returns a detailed list of transfers
Usage: osc block-storage volume-transfer list [OPTIONS]
Options:
-
--all-tenants <ALL_TENANTS>
— Shows details for all project. Admin onlyPossible values:
true
,false
-
--is-public <IS_PUBLIC>
— Filter the volume transfer by public visibilityPossible values:
true
,false
-
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--offset <OFFSET>
— Used in conjunction with limit to return a slice of items. offset is where to start in the list -
--sort <SORT>
— Comma-separated list of sort keys and optional sort directions in the form of < key > [: < direction > ]. A valid direction is asc (ascending) or desc (descending) -
--sort-dir <SORT_DIR>
— Sorts by one or more sets of attribute and sort direction combinations. If you omit the sort direction in a set, default is desc. Deprecated in favour of the combined sort parameter -
--sort-key <SORT_KEY>
— Sorts by an attribute. A valid value is name, status, container_format, disk_format, size, id, created_at, or updated_at. Default is created_at. The API uses the natural sorting direction of the sort_key attribute value. Deprecated in favour of the combined sort parameter -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc block-storage volume-transfer show
Return data about active transfers
Usage: osc block-storage volume-transfer show <ID>
Arguments:
<ID>
— id parameter for /v3/volume-transfers/{id} API
osc catalog
Catalog commands args
Usage: osc catalog <COMMAND>
Available subcommands:
osc catalog list
— Shows current catalog informationosc catalog show
— Shows current catalog information
osc catalog list
Shows current catalog information
Usage: osc catalog list
osc catalog show
Shows current catalog information
Usage: osc catalog show --service-type <SERVICE_TYPE>
Options:
--service-type <SERVICE_TYPE>
— Service type
osc compute
Compute service (Nova) operations
Usage: osc compute <COMMAND>
Available subcommands:
osc compute aggregate
— Host Aggregatesosc compute assisted-volume-snapshot
— Assisted volume snapshots (os-assisted-volume-snapshots)osc compute availability-zone
— Availability zonesosc compute extension
— Extension commandsosc compute flavor
— Flavor commandsosc compute hypervisor
— Hypervisorsosc compute instance-usage-audit-log
— Server usage audit log (os-instance-usage-audit-log)osc compute keypair
— Keypairs commandsosc compute limit
— Limits (limits)osc compute migration
— Migrations (os-migrations)osc compute quota-class-set
— Quota class sets (os-quota-class-sets)osc compute quota-set
— Quota sets (os-quota-sets)osc compute server
— Serversosc compute server-external-event
— Create external events (os-server-external-events)osc compute server-group
— Server groups (os-server-groups)osc compute service
— Server groups (os-server-groups)osc compute simple-tenant-usage
— Usage reports (os-simple-tenant-usage)
osc compute aggregate
Creates and manages host aggregates. An aggregate assigns metadata to groups of compute nodes.
Policy defaults enable only users with the administrative role to perform operations with aggregates. Cloud providers can change these permissions through policy file configuration.
Usage: osc compute aggregate <COMMAND>
Available subcommands:
osc compute aggregate add-host
— Add Hostosc compute aggregate create21
— Create Aggregate (microversion = 2.1)osc compute aggregate cache-image
— Request Image Pre-caching for Aggregate (microversion = 2.81)osc compute aggregate delete
— Delete Aggregateosc compute aggregate list
— List Aggregatesosc compute aggregate remove-host
— Remove Hostosc compute aggregate show
— Show Aggregate Detailsosc compute aggregate set21
— Update Aggregate (microversion = 2.1)osc compute aggregate set-metadata
— Create Or Update Aggregate Metadata
osc compute aggregate add-host
Add Host
Usage: osc compute aggregate add-host --host <HOST> <ID>
Arguments:
<ID>
— id parameter for /v2.1/os-aggregates/{id}/action API
Options:
--host <HOST>
osc compute aggregate create21
Creates an aggregate. If specifying an option availability_zone, the aggregate is created as an availability zone and the availability zone is visible to normal users.
Normal response codes: 200
Error response codes: badRequest(400), unauthorized(401), forbidden(403), conflict(409)
Usage: osc compute aggregate create21 [OPTIONS] --name <NAME>
Options:
--availability-zone <AVAILABILITY_ZONE>
— The availability zone of the host aggregate. You should use a custom availability zone rather than the default returned by the os-availability-zone API. The availability zone must not include ‘:’ in its name--name <NAME>
— The name of the host aggregate
osc compute aggregate cache-image
Requests that a set of images be pre-cached on compute nodes within the referenced aggregate.
This API is available starting with microversion 2.81.
Normal response codes: 202
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute aggregate cache-image [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.1/os-aggregates/{id}/images API
Options:
-
--cache <CACHE>
— A list of image objects to cache.Parameter is an array, may be provided multiple times.
osc compute aggregate delete
Deletes an aggregate.
Normal response codes: 200
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute aggregate delete <ID>
Arguments:
<ID>
— id parameter for /v2.1/os-aggregates/{id} API
osc compute aggregate list
Lists all aggregates. Includes the ID, name, and availability zone for each aggregate.
Normal response codes: 200
Error response codes: unauthorized(401), forbidden(403)
Usage: osc compute aggregate list
osc compute aggregate remove-host
Remove Host
Usage: osc compute aggregate remove-host --host <HOST> <ID>
Arguments:
<ID>
— id parameter for /v2.1/os-aggregates/{id}/action API
Options:
--host <HOST>
osc compute aggregate show
Shows details for an aggregate. Details include hosts and metadata.
Normal response codes: 200
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute aggregate show <ID>
Arguments:
<ID>
— id parameter for /v2.1/os-aggregates/{id} API
osc compute aggregate set21
Updates either or both the name and availability zone for an aggregate. If the aggregate to be updated has host that already in the given availability zone, the request will fail with 400 error.
Normal response codes: 200
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404), conflict(409)
Usage: osc compute aggregate set21 [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.1/os-aggregates/{id} API
Options:
-
--availability-zone <AVAILABILITY_ZONE>
— The availability zone of the host aggregate. You should use a custom availability zone rather than the default returned by the os-availability-zone API. The availability zone must not include ‘:’ in its name.Warning
You should not change or unset the availability zone of an aggregate when that aggregate has hosts which contain servers in it since that may impact the ability for those servers to move to another host.
-
--name <NAME>
— The name of the host aggregate
osc compute aggregate set-metadata
Create Or Update Aggregate Metadata
Usage: osc compute aggregate set-metadata [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.1/os-aggregates/{id}/action API
Options:
--metadata <key=value>
osc compute assisted-volume-snapshot
Assisted volume snapshots (os-assisted-volume-snapshots)
Creates and deletes snapshots through an emulator/hypervisor. Only qcow2 file format is supported.
This API is only implemented by the libvirt compute driver.
An internal snapshot that lacks storage such as NFS can use an emulator/hypervisor to add the snapshot feature. This is used to enable snapshot of volumes on backends such as NFS by storing data as qcow2 files on these volumes.
This API is only ever called by Cinder, where it is used to create a snapshot for drivers that extend the remotefs Cinder driver.
Usage: osc compute assisted-volume-snapshot <COMMAND>
Available subcommands:
osc compute assisted-volume-snapshot create
— Create Assisted Volume Snapshotsosc compute assisted-volume-snapshot delete
— Delete Assisted Volume Snapshot
osc compute assisted-volume-snapshot create
Creates an assisted volume snapshot.
Normal response codes: 200
Error response codes: badRequest(400),unauthorized(401), forbidden(403)
Usage: osc compute assisted-volume-snapshot create --volume-id <VOLUME_ID> <--id <ID>|--new-file <NEW_FILE>|--snapshot-id <SNAPSHOT_ID>|--type <TYPE>>
Options:
-
--id <ID>
— Its an arbitrary string that gets passed back to the user -
--new-file <NEW_FILE>
— The name of the qcow2 file that Block Storage creates, which becomes the active image for the VM -
--snapshot-id <SNAPSHOT_ID>
— The UUID for a snapshot -
--type <TYPE>
— The snapshot type. A valid value isqcow2
Possible values:
qcow2
-
--volume-id <VOLUME_ID>
— The source volume ID
osc compute assisted-volume-snapshot delete
Deletes an assisted volume snapshot.
To make this request, add the delete_info
query parameter to the URI, as follows:
DELETE /os-assisted-volume-snapshots/421752a6-acf6-4b2d-bc7a-119f9148cd8c?delete_info=’{“volume_id”: “521752a6-acf6-4b2d-bc7a-119f9148cd8c”}’
Normal response codes: 204
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute assisted-volume-snapshot delete [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.1/os-assisted-volume-snapshots/{id} API
Options:
--delete-info <DELETE_INFO>
osc compute availability-zone
Availability zones
Lists and gets detailed availability zone information.
An availability zone is created or updated by setting the availability_zone parameter in the create, update, or create or update methods of the Host Aggregates API. See Host Aggregates for more details.
Usage: osc compute availability-zone <COMMAND>
Available subcommands:
osc compute availability-zone list
— Get Availability Zone Informationosc compute availability-zone list-detail
— Get Detailed Availability Zone Information
osc compute availability-zone list
Lists availability zone information.
Normal response codes: 200
Error response codes: unauthorized(401), forbidden(403)
Usage: osc compute availability-zone list
osc compute availability-zone list-detail
Gets detailed availability zone information. Policy defaults enable only users with the administrative role to perform this operation. Cloud providers can change these permissions through the policy.json
file.
Normal response codes: 200
Error response codes: unauthorized(401), forbidden(403)
Usage: osc compute availability-zone list-detail
osc compute extension
Extension commands
Usage: osc compute extension <COMMAND>
Available subcommands:
osc compute extension list
— List Extensionsosc compute extension show
— Show Extension Details
osc compute extension list
Lists all extensions to the API.
Normal response codes: 200
Error response codes: unauthorized(401)
Usage: osc compute extension list
osc compute extension show
Shows details for an extension, by alias.
Normal response codes: 200
Error response codes: unauthorized(401), itemNotFound(404)
Usage: osc compute extension show <ID>
Arguments:
<ID>
— id parameter for /v2.1/extensions/{id} API
osc compute flavor
Flavor commands
Flavors are a way to describe the basic dimensions of a server to be created including how much cpu, ram, and disk space are allocated to a server built with this flavor.
Usage: osc compute flavor <COMMAND>
Available subcommands:
osc compute flavor access
— Flavor access commandosc compute flavor create255
— Create Flavor (microversion = 2.55)osc compute flavor create21
— Create Flavor (microversion = 2.1)osc compute flavor create20
— Create Flavor (microversion = 2.0)osc compute flavor delete
— Delete Flavorosc compute flavor extraspecs
— Flavor extra specsosc compute flavor list
— List Flavors With Detailsosc compute flavor set255
— Update Flavor Description (microversion = 2.55)osc compute flavor show
— Show Flavor Details
osc compute flavor access
Flavor access command
Usage: osc compute flavor access <COMMAND>
Available subcommands:
osc compute flavor access add
— Add Flavor Access To Tenant (addTenantAccess Action)osc compute flavor access list
— List Flavor Access Information For Given Flavorosc compute flavor access remove
— Remove Flavor Access From Tenant (removeTenantAccess Action)
osc compute flavor access add
Add Flavor Access To Tenant (addTenantAccess Action)
Usage: osc compute flavor access add --tenant <TENANT> <ID>
Arguments:
<ID>
— id parameter for /v2.1/flavors/{id}/action API
Options:
--tenant <TENANT>
— The UUID of the tenant in a multi-tenancy cloud
osc compute flavor access list
List Flavor Access Information For Given Flavor
Usage: osc compute flavor access list <FLAVOR_ID>
Arguments:
<FLAVOR_ID>
— flavor_id parameter for /v2.1/flavors/{flavor_id}/os-flavor-access API
osc compute flavor access remove
Remove Flavor Access From Tenant (removeTenantAccess Action)
Usage: osc compute flavor access remove --tenant <TENANT> <ID>
Arguments:
<ID>
— id parameter for /v2.1/flavors/{id}/action API
Options:
--tenant <TENANT>
— The UUID of the tenant in a multi-tenancy cloud
osc compute flavor create255
Creates a flavor.
Creating a flavor is typically only available to administrators of a cloud because this has implications for scheduling efficiently in the cloud.
Normal response codes: 200
Error response codes: badRequest(400), unauthorized(401), forbidden(403), conflict(409)
Usage: osc compute flavor create255 [OPTIONS] --disk <DISK> --name <NAME> --ram <RAM> --vcpus <VCPUS>
Options:
-
--description <DESCRIPTION>
— A free form description of the flavor. Limited to 65535 characters in length. Only printable characters are allowed.New in version 2.55
-
--disk <DISK>
— The size of a dedicated swap disk that will be allocated, in MiB. If 0 (the default), no dedicated swap disk will be created -
--id <ID>
— Only alphanumeric characters with hyphen ‘-’, underscore ‘_’, spaces and dots ‘.’ are permitted. If an ID is not provided, then a default UUID will be assigned -
--name <NAME>
— The display name of a flavor -
--os-flavor-access-is-public <OS_FLAVOR_ACCESS_IS_PUBLIC>
— Whether the flavor is public (available to all projects) or scoped to a set of projects. Default is True if not specifiedPossible values:
true
,false
-
--os-flv-ext-data-ephemeral <OS_FLV_EXT_DATA_EPHEMERAL>
— The size of a dedicated swap disk that will be allocated, in MiB. If 0 (the default), no dedicated swap disk will be created -
--ram <RAM>
— The number of virtual CPUs that will be allocated to the server -
--rxtx-factor <RXTX_FACTOR>
— The receive / transmit factor (as a float) that will be set on ports if the network backend supports the QOS extension. Otherwise it will be ignored. It defaults to 1.0 -
--swap <SWAP>
— The size of a dedicated swap disk that will be allocated, in MiB. If 0 (the default), no dedicated swap disk will be created -
--vcpus <VCPUS>
— The number of virtual CPUs that will be allocated to the server
osc compute flavor create21
Creates a flavor.
Creating a flavor is typically only available to administrators of a cloud because this has implications for scheduling efficiently in the cloud.
Normal response codes: 200
Error response codes: badRequest(400), unauthorized(401), forbidden(403), conflict(409)
Usage: osc compute flavor create21 [OPTIONS] --disk <DISK> --name <NAME> --ram <RAM> --vcpus <VCPUS>
Options:
-
--disk <DISK>
— The size of a dedicated swap disk that will be allocated, in MiB. If 0 (the default), no dedicated swap disk will be created -
--id <ID>
— Only alphanumeric characters with hyphen ‘-’, underscore ‘_’, spaces and dots ‘.’ are permitted. If an ID is not provided, then a default UUID will be assigned -
--name <NAME>
— The display name of a flavor -
--os-flavor-access-is-public <OS_FLAVOR_ACCESS_IS_PUBLIC>
— Whether the flavor is public (available to all projects) or scoped to a set of projects. Default is True if not specifiedPossible values:
true
,false
-
--os-flv-ext-data-ephemeral <OS_FLV_EXT_DATA_EPHEMERAL>
— The size of a dedicated swap disk that will be allocated, in MiB. If 0 (the default), no dedicated swap disk will be created -
--ram <RAM>
— The number of virtual CPUs that will be allocated to the server -
--rxtx-factor <RXTX_FACTOR>
— The receive / transmit factor (as a float) that will be set on ports if the network backend supports the QOS extension. Otherwise it will be ignored. It defaults to 1.0 -
--swap <SWAP>
— The size of a dedicated swap disk that will be allocated, in MiB. If 0 (the default), no dedicated swap disk will be created -
--vcpus <VCPUS>
— The number of virtual CPUs that will be allocated to the server
osc compute flavor create20
Creates a flavor.
Creating a flavor is typically only available to administrators of a cloud because this has implications for scheduling efficiently in the cloud.
Normal response codes: 200
Error response codes: badRequest(400), unauthorized(401), forbidden(403), conflict(409)
Usage: osc compute flavor create20 [OPTIONS] --disk <DISK> --name <NAME> --ram <RAM> --vcpus <VCPUS>
Options:
-
--disk <DISK>
— The size of a dedicated swap disk that will be allocated, in MiB. If 0 (the default), no dedicated swap disk will be created -
--id <ID>
— Only alphanumeric characters with hyphen ‘-’, underscore ‘_’, spaces and dots ‘.’ are permitted. If an ID is not provided, then a default UUID will be assigned -
--name <NAME>
— The display name of a flavor -
--os-flavor-access-is-public <OS_FLAVOR_ACCESS_IS_PUBLIC>
— Whether the flavor is public (available to all projects) or scoped to a set of projects. Default is True if not specifiedPossible values:
true
,false
-
--os-flv-ext-data-ephemeral <OS_FLV_EXT_DATA_EPHEMERAL>
— The size of a dedicated swap disk that will be allocated, in MiB. If 0 (the default), no dedicated swap disk will be created -
--ram <RAM>
— The number of virtual CPUs that will be allocated to the server -
--rxtx-factor <RXTX_FACTOR>
— The receive / transmit factor (as a float) that will be set on ports if the network backend supports the QOS extension. Otherwise it will be ignored. It defaults to 1.0 -
--swap <SWAP>
— The size of a dedicated swap disk that will be allocated, in MiB. If 0 (the default), no dedicated swap disk will be created -
--vcpus <VCPUS>
— The number of virtual CPUs that will be allocated to the server
osc compute flavor delete
Deletes a flavor.
This is typically an admin only action. Deleting a flavor that is in use by existing servers is not recommended as it can cause incorrect data to be returned to the user under some operations.
Normal response codes: 202
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute flavor delete <ID>
Arguments:
<ID>
— id parameter for /v2.1/flavors/{id} API
osc compute flavor extraspecs
Flavor extra specs
Usage: osc compute flavor extraspecs <COMMAND>
Available subcommands:
osc compute flavor extraspecs create
— Create Extra Specs For A Flavorosc compute flavor extraspecs delete
— Delete An Extra Spec For A Flavorosc compute flavor extraspecs list
— List Extra Specs For A Flavorosc compute flavor extraspecs show
— Show An Extra Spec For A Flavorosc compute flavor extraspecs set
— Update An Extra Spec For A Flavor
osc compute flavor extraspecs create
Create Extra Specs For A Flavor
Usage: osc compute flavor extraspecs create [OPTIONS] <FLAVOR_ID>
Arguments:
<FLAVOR_ID>
— flavor_id parameter for /v2.1/flavors/{flavor_id}/os-extra_specs/{id} API
Options:
--extra-specs <key=value>
— A dictionary of the flavor’s extra-specs key-and-value pairs. It appears in the os-extra-specs’ “create” REQUEST body, as well as the os-extra-specs’ “create” and “list” RESPONSE body
osc compute flavor extraspecs delete
Delete An Extra Spec For A Flavor
Usage: osc compute flavor extraspecs delete <FLAVOR_ID> <ID>
Arguments:
<FLAVOR_ID>
— flavor_id parameter for /v2.1/flavors/{flavor_id}/os-extra_specs/{id} API<ID>
— id parameter for /v2.1/flavors/{flavor_id}/os-extra_specs/{id} API
osc compute flavor extraspecs list
List Extra Specs For A Flavor
Usage: osc compute flavor extraspecs list <FLAVOR_ID>
Arguments:
<FLAVOR_ID>
— flavor_id parameter for /v2.1/flavors/{flavor_id}/os-extra_specs/{id} API
osc compute flavor extraspecs show
Show An Extra Spec For A Flavor
Usage: osc compute flavor extraspecs show <FLAVOR_ID> <ID>
Arguments:
<FLAVOR_ID>
— flavor_id parameter for /v2.1/flavors/{flavor_id}/os-extra_specs/{id} API<ID>
— id parameter for /v2.1/flavors/{flavor_id}/os-extra_specs/{id} API
osc compute flavor extraspecs set
Update An Extra Spec For A Flavor
Usage: osc compute flavor extraspecs set [OPTIONS] <FLAVOR_ID> <ID>
Arguments:
<FLAVOR_ID>
— flavor_id parameter for /v2.1/flavors/{flavor_id}/os-extra_specs/{id} API<ID>
— id parameter for /v2.1/flavors/{flavor_id}/os-extra_specs/{id} API
Options:
--property <key=value>
osc compute flavor list
Lists flavors with details.
Normal response codes: 200
Error response codes: badRequest(400), unauthorized(401), forbidden(403)
Usage: osc compute flavor list [OPTIONS]
Options:
-
--is-public <IS_PUBLIC>
-
--limit <LIMIT>
-
--marker <MARKER>
-
--min-disk <MIN_DISK>
-
--min-ram <MIN_RAM>
-
--sort-dir <SORT_DIR>
Possible values:
asc
,desc
-
--sort-key <SORT_KEY>
Possible values:
created_at
,description
,disabled
,ephemeral_gb
,flavorid
,id
,is_public
,memory_mb
,name
,root_gb
,rxtx_factor
,swap
,updated_at
,vcpu_weight
,vcpus
-
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc compute flavor set255
Updates a flavor description.
This API is available starting with microversion 2.55.
Policy defaults enable only users with the administrative role to perform this operation. Cloud providers can change these permissions through the policy.json
file.
Normal response codes: 200
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute flavor set255 --description <DESCRIPTION> <ID>
Arguments:
<ID>
— id parameter for /v2.1/flavors/{id} API
Options:
--description <DESCRIPTION>
— A free form description of the flavor. Limited to 65535 characters in length. Only printable characters are allowed
osc compute flavor show
Shows details for a flavor.
Normal response codes: 200
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute flavor show <ID>
Arguments:
<ID>
— id parameter for /v2.1/flavors/{id} API
osc compute hypervisor
Hypervisors
Usage: osc compute hypervisor <COMMAND>
Available subcommands:
osc compute hypervisor list
— List Hypervisors Detailsosc compute hypervisor show
— Show Hypervisor Details
osc compute hypervisor list
Lists hypervisors details.
Policy defaults enable only users with the administrative role to perform this operation. Cloud providers can change these permissions through the policy.json
file.
Normal response codes: 200
Error response codes: badRequest(400), unauthorized(401), forbidden(403)
Usage: osc compute hypervisor list [OPTIONS]
Options:
-
--hypervisor-hostname-pattern <HYPERVISOR_HOSTNAME_PATTERN>
-
--limit <LIMIT>
-
--marker <MARKER>
-
--with-servers <WITH_SERVERS>
Possible values:
true
,false
-
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc compute hypervisor show
Shows details for a given hypervisor.
Policy defaults enable only users with the administrative role to perform this operation. Cloud providers can change these permissions through the policy.json
file.
Normal response codes: 200
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute hypervisor show [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.1/os-hypervisors/{id} API
Options:
-
--with-servers <WITH_SERVERS>
Possible values:
true
,false
osc compute instance-usage-audit-log
Server usage audit log (os-instance-usage-audit-log)
Audit server usage of the cloud. This API is dependent on the instance_usage_audit configuration option being set on all compute hosts where usage auditing is required.
Policy defaults enable only users with the administrative role to perform all os-instance-usage-audit-log related operations. Cloud providers can change these permissions through the policy.json file.
Usage: osc compute instance-usage-audit-log <COMMAND>
Available subcommands:
osc compute instance-usage-audit-log list
— List Server Usage Auditsosc compute instance-usage-audit-log show
— List Usage Audits Before Specified Time
osc compute instance-usage-audit-log list
Lists usage audits for all servers on all compute hosts where usage auditing is configured.
Normal response codes: 200
Error response codes: unauthorized(401), forbidden(403)
Usage: osc compute instance-usage-audit-log list
osc compute instance-usage-audit-log show
Lists usage audits that occurred before a specified time.
Normal response codes: 200
Error response codes: badRequest(400), unauthorized(401), forbidden(403)
Usage: osc compute instance-usage-audit-log show <ID>
Arguments:
<ID>
— Filters the response by the date and time before which to list usage audits
osc compute keypair
Keypairs commands
Generates, imports, and deletes SSH keys.
Usage: osc compute keypair <COMMAND>
Available subcommands:
osc compute keypair create292
— Import (or create) Keypair (microversion = 2.92)osc compute keypair create210
— Import (or create) Keypair (microversion = 2.10)osc compute keypair create22
— Import (or create) Keypair (microversion = 2.2)osc compute keypair create21
— Import (or create) Keypair (microversion = 2.1)osc compute keypair create20
— Import (or create) Keypair (microversion = 2.0)osc compute keypair delete
— Delete Keypairosc compute keypair list
— List Keypairsosc compute keypair show
— Show Keypair Details
osc compute keypair create292
Imports (or generates) a keypair.
Normal response codes: 200, 201
Error response codes: badRequest(400), unauthorized(401), forbidden(403), conflict(409)
Usage: osc compute keypair create292 [OPTIONS] --name <NAME> --public-key <PUBLIC_KEY>
Options:
-
--name <NAME>
— A name for the keypair which will be used to reference it later.Note
Since microversion 2.92, allowed characters are ASCII letters
[a-zA-Z]
, digits[0-9]
and the following special characters:[@._- ]
. -
--public-key <PUBLIC_KEY>
— The public ssh key to import. Was optional before microversion 2.92 : if you were omitting this value, a keypair was generated for you -
--type <TYPE>
— The type of the keypair. Allowed values aressh
orx509
.New in version 2.2
Possible values:
ssh
,x509
-
--user-id <USER_ID>
— The user_id for a keypair. This allows administrative users to upload keys for other users than themselves.New in version 2.10
osc compute keypair create210
Imports (or generates) a keypair.
Normal response codes: 200, 201
Error response codes: badRequest(400), unauthorized(401), forbidden(403), conflict(409)
Usage: osc compute keypair create210 [OPTIONS] --name <NAME>
Options:
-
--name <NAME>
— A name for the keypair which will be used to reference it later.Note
Since microversion 2.92, allowed characters are ASCII letters
[a-zA-Z]
, digits[0-9]
and the following special characters:[@._- ]
. -
--public-key <PUBLIC_KEY>
— The public ssh key to import. Was optional before microversion 2.92 : if you were omitting this value, a keypair was generated for you -
--type <TYPE>
— The type of the keypair. Allowed values aressh
orx509
.New in version 2.2
Possible values:
ssh
,x509
-
--user-id <USER_ID>
— The user_id for a keypair. This allows administrative users to upload keys for other users than themselves.New in version 2.10
osc compute keypair create22
Imports (or generates) a keypair.
Normal response codes: 200, 201
Error response codes: badRequest(400), unauthorized(401), forbidden(403), conflict(409)
Usage: osc compute keypair create22 [OPTIONS] --name <NAME>
Options:
-
--name <NAME>
— A name for the keypair which will be used to reference it later.Note
Since microversion 2.92, allowed characters are ASCII letters
[a-zA-Z]
, digits[0-9]
and the following special characters:[@._- ]
. -
--public-key <PUBLIC_KEY>
— The public ssh key to import. Was optional before microversion 2.92 : if you were omitting this value, a keypair was generated for you -
--type <TYPE>
— The type of the keypair. Allowed values aressh
orx509
.New in version 2.2
Possible values:
ssh
,x509
osc compute keypair create21
Imports (or generates) a keypair.
Normal response codes: 200, 201
Error response codes: badRequest(400), unauthorized(401), forbidden(403), conflict(409)
Usage: osc compute keypair create21 [OPTIONS] --name <NAME>
Options:
-
--name <NAME>
— A name for the keypair which will be used to reference it later.Note
Since microversion 2.92, allowed characters are ASCII letters
[a-zA-Z]
, digits[0-9]
and the following special characters:[@._- ]
. -
--public-key <PUBLIC_KEY>
— The public ssh key to import. Was optional before microversion 2.92 : if you were omitting this value, a keypair was generated for you
osc compute keypair create20
Imports (or generates) a keypair.
Normal response codes: 200, 201
Error response codes: badRequest(400), unauthorized(401), forbidden(403), conflict(409)
Usage: osc compute keypair create20 [OPTIONS] --name <NAME>
Options:
-
--name <NAME>
— A name for the keypair which will be used to reference it later.Note
Since microversion 2.92, allowed characters are ASCII letters
[a-zA-Z]
, digits[0-9]
and the following special characters:[@._- ]
. -
--public-key <PUBLIC_KEY>
— The public ssh key to import. Was optional before microversion 2.92 : if you were omitting this value, a keypair was generated for you
osc compute keypair delete
Deletes a keypair.
Normal response codes: 202, 204
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute keypair delete [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.1/os-keypairs/{id} API
Options:
--user-name <USER_NAME>
— User Name--user-id <USER_ID>
— User ID--current-user
— Current authenticated user
osc compute keypair list
Lists keypairs that are associated with the account.
Normal response codes: 200
Error response codes: unauthorized(401), forbidden(403)
Usage: osc compute keypair list [OPTIONS]
Options:
-
--limit <LIMIT>
-
--marker <MARKER>
-
--user-name <USER_NAME>
— User Name -
--user-id <USER_ID>
— User ID -
--current-user
— Current authenticated user -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc compute keypair show
Shows details for a keypair that is associated with the account.
Normal response codes: 200
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute keypair show [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.1/os-keypairs/{id} API
Options:
--user-name <USER_NAME>
— User Name--user-id <USER_ID>
— User ID--current-user
— Current authenticated user
osc compute limit
Limits (limits)
Shows rate and absolute limits for the project.
Usage: osc compute limit <COMMAND>
Available subcommands:
osc compute limit list
— Show Rate And Absolute Limits
osc compute limit list
Shows rate and absolute limits for the project.
Normal response codes: 200
Error response codes: unauthorized(401), forbidden(403)
Usage: osc compute limit list [OPTIONS]
Options:
--tenant-id <TENANT_ID>
osc compute migration
Migrations (os-migrations)
Shows data on migrations.
Usage: osc compute migration <COMMAND>
Available subcommands:
osc compute migration get
— List Migrations
osc compute migration get
Lists migrations.
Policy defaults enable only users with the administrative role to perform this operation. Cloud providers can change these permissions through the policy.json
file.
Starting from microversion 2.59, the response is sorted by created_at
and id
in descending order.
Normal response codes: 200
Error response codes: badRequest(400), unauthorized(401), forbidden(403)
Usage: osc compute migration get [OPTIONS]
Options:
--changes-before <CHANGES_BEFORE>
--changes-since <CHANGES_SINCE>
--hidden <HIDDEN>
--host <HOST>
--instance-uuid <INSTANCE_UUID>
--limit <LIMIT>
--marker <MARKER>
--migration-type <MIGRATION_TYPE>
--project-name <PROJECT_NAME>
— Project Name--project-id <PROJECT_ID>
— Project ID--current-project
— Current project--source-compute <SOURCE_COMPUTE>
--status <STATUS>
--user-name <USER_NAME>
— User Name--user-id <USER_ID>
— User ID--current-user
— Current authenticated user
osc compute quota-class-set
Quota class sets (os-quota-class-sets)
Show, Create or Update the quotas for a Quota Class. Nova supports implicit ‘default’ Quota Class only.
Note
Once a default limit is set via the default quota class via the API, that takes precedence over any changes to that resource limit in the configuration options. In other words, once you’ve changed things via the API, you either have to keep those synchronized with the configuration values or remove the default limit from the database manually as there is no REST API for removing quota class values from the database.
For Example: If you updated default quotas for instances, to 20, but didn’t change quota_instances in your nova.conf, you’d now have default quota for instances as 20 for all projects. If you then change quota_instances=5 in nova.conf, but didn’t update the default quota class via the API, you’ll still have a default quota of 20 for instances regardless of nova.conf. Refer: Quotas for more details.
Warning
There is a bug in the v2.1 API until microversion 2.49 and the legacy v2 compatible API which does not return the server_groups and server_group_members quotas in GET and PUT os-quota-class-sets API response, whereas the v2 API used to return those keys in the API response. There is workaround to get the server_groups and server_group_members quotas using “List Default Quotas For Tenant” API in Quota sets (os-quota-sets) but that is per project quota. This issue is fixed in microversion 2.50, here onwards server_groups and server_group_members keys are returned in API response body.
Usage: osc compute quota-class-set <COMMAND>
Available subcommands:
osc compute quota-class-set set21
— Create or Update Quotas for Quota Class (microversion = 2.1)osc compute quota-class-set show
— Show the quota for Quota Class
osc compute quota-class-set set21
Update the quotas for the Quota Class.
If the requested Quota Class is not found in the DB, then the API will create the one. Only ‘default’ quota class is valid and used to set the default quotas, all other quota class would not be used anywhere.
Normal response codes: 200
Error response codes: badRequest(400), unauthorized(401), forbidden(403)
Usage: osc compute quota-class-set set21 [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.1/os-quota-class-sets/{id} API
Options:
-
--cores <CORES>
— The number of allowed injected files for the quota class.Available until version 2.56
-
--fixed-ips <FIXED_IPS>
— The number of allowed injected files for the quota class.Available until version 2.56
-
--floating-ips <FLOATING_IPS>
— The number of allowed injected files for the quota class.Available until version 2.56
-
--injected-file-content-bytes <INJECTED_FILE_CONTENT_BYTES>
— The number of allowed injected files for the quota class.Available until version 2.56
-
--injected-file-path-bytes <INJECTED_FILE_PATH_BYTES>
— The number of allowed injected files for the quota class.Available until version 2.56
-
--injected-files <INJECTED_FILES>
— The number of allowed injected files for the quota class.Available until version 2.56
-
--instances <INSTANCES>
— The number of allowed injected files for the quota class.Available until version 2.56
-
--key-pairs <KEY_PAIRS>
— The number of allowed injected files for the quota class.Available until version 2.56
-
--metadata-items <METADATA_ITEMS>
— The number of allowed injected files for the quota class.Available until version 2.56
-
--networks <NETWORKS>
— The number of allowed injected files for the quota class.Available until version 2.56
-
--ram <RAM>
— The number of allowed injected files for the quota class.Available until version 2.56
-
--security-group-rules <SECURITY_GROUP_RULES>
— The number of allowed injected files for the quota class.Available until version 2.56
-
--security-groups <SECURITY_GROUPS>
— The number of allowed injected files for the quota class.Available until version 2.56
-
--server-group-members <SERVER_GROUP_MEMBERS>
— The number of allowed injected files for the quota class.Available until version 2.56
-
--server-groups <SERVER_GROUPS>
— The number of allowed injected files for the quota class.Available until version 2.56
osc compute quota-class-set show
Show the quota for the Quota Class.
Normal response codes: 200
Error response codes: unauthorized(401), forbidden(403)
Usage: osc compute quota-class-set show <ID>
Arguments:
<ID>
— id parameter for /v2.1/os-quota-class-sets/{id} API
osc compute quota-set
Quota sets (os-quota-sets)
Permits administrators, depending on policy settings, to view default quotas, view details for quotas, revert quotas to defaults, and update the quotas for a project or a project and user.
Usage: osc compute quota-set <COMMAND>
Available subcommands:
osc compute quota-set defaults
— List Default Quotas For Tenantosc compute quota-set delete
— Revert Quotas To Defaultsosc compute quota-set details
— Show The Detail of Quotaosc compute quota-set set21
— Update Quotas (microversion = 2.1)osc compute quota-set show
— Show A Quota
osc compute quota-set defaults
Lists the default quotas for a project.
Normal response codes: 200
Error response codes: badrequest(400), unauthorized(401), forbidden(403)
Usage: osc compute quota-set defaults <ID>
Arguments:
<ID>
— id parameter for /v2.1/os-quota-sets/{id}/defaults API
osc compute quota-set delete
Reverts the quotas to default values for a project or a project and a user.
To revert quotas for a project and a user, specify the user_id
query parameter.
Normal response codes: 202
Error response codes: unauthorized(401), forbidden(403)
Usage: osc compute quota-set delete [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.1/os-quota-sets/{id} API
Options:
--user-name <USER_NAME>
— User Name--user-id <USER_ID>
— User ID--current-user
— Current authenticated user
osc compute quota-set details
Show the detail of quota for a project or a project and a user.
To show a quota for a project and a user, specify the user_id
query parameter.
Normal response codes: 200
Error response codes: badrequest(400), unauthorized(401), forbidden(403)
Usage: osc compute quota-set details [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.1/os-quota-sets/{id}/detail API
Options:
--user-name <USER_NAME>
— User Name--user-id <USER_ID>
— User ID--current-user
— Current authenticated user
osc compute quota-set set21
Update the quotas for a project or a project and a user.
Users can force the update even if the quota has already been used and the reserved quota exceeds the new quota. To force the update, specify the "force": True
attribute in the request body, the default value is false
.
Normal response codes: 200
Error response codes: badRequest(400), unauthorized(401), forbidden(403)
Usage: osc compute quota-set set21 [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.1/os-quota-sets/{id} API
Options:
-
--user-name <USER_NAME>
— User Name -
--user-id <USER_ID>
— User ID -
--current-user
— Current authenticated user -
--cores <CORES>
— The number of allowed injected files for each tenant.Available until version 2.56
-
--fixed-ips <FIXED_IPS>
— The number of allowed injected files for each tenant.Available until version 2.56
-
--floating-ips <FLOATING_IPS>
— The number of allowed injected files for each tenant.Available until version 2.56
-
--force <FORCE>
— You can force the update even if the quota has already been used and the reserved quota exceeds the new quota. To force the update, specify the"force": "True"
. Default isFalse
Possible values:
true
,false
-
--injected-file-content-bytes <INJECTED_FILE_CONTENT_BYTES>
— The number of allowed injected files for each tenant.Available until version 2.56
-
--injected-file-path-bytes <INJECTED_FILE_PATH_BYTES>
— The number of allowed injected files for each tenant.Available until version 2.56
-
--injected-files <INJECTED_FILES>
— The number of allowed injected files for each tenant.Available until version 2.56
-
--instances <INSTANCES>
— The number of allowed injected files for each tenant.Available until version 2.56
-
--key-pairs <KEY_PAIRS>
— The number of allowed injected files for each tenant.Available until version 2.56
-
--metadata-items <METADATA_ITEMS>
— The number of allowed injected files for each tenant.Available until version 2.56
-
--networks <NETWORKS>
— The number of allowed injected files for each tenant.Available until version 2.56
-
--ram <RAM>
— The number of allowed injected files for each tenant.Available until version 2.56
-
--security-group-rules <SECURITY_GROUP_RULES>
— The number of allowed injected files for each tenant.Available until version 2.56
-
--security-groups <SECURITY_GROUPS>
— The number of allowed injected files for each tenant.Available until version 2.56
-
--server-group-members <SERVER_GROUP_MEMBERS>
— The number of allowed injected files for each tenant.Available until version 2.56
-
--server-groups <SERVER_GROUPS>
— The number of allowed injected files for each tenant.Available until version 2.56
osc compute quota-set show
Show the quota for a project or a project and a user.
Normal response codes: 200
Error response codes: badRequest(400), unauthorized(401), forbidden(403)
Usage: osc compute quota-set show [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.1/os-quota-sets/{id} API
Options:
--user-name <USER_NAME>
— User Name--user-id <USER_ID>
— User ID--current-user
— Current authenticated user
osc compute server
Servers (servers)
Lists, creates, shows details for, updates, and deletes servers.
Passwords
When you create a server, you can specify a password through the optional adminPass attribute. The password must meet the complexity requirements set by your OpenStack Compute provider. The server might enter an ERROR state if the complexity requirements are not met. In this case, a client might issue a change password action to reset the server password.
If you do not specify a password, the API generates and assigns a random password that it returns in the response object. This password meets the security requirements set by the compute provider. For security reasons, subsequent GET calls do not require this password.
Server metadata
You can specify custom server metadata at server launch time. The maximum size for each metadata key-value pair is 255 bytes. The compute provider determines the maximum number of key-value pairs for each server. You can query this value through the maxServerMeta absolute limit.
Usage: osc compute server <COMMAND>
Available subcommands:
osc compute server add-fixed-ip21
— Add (Associate) Fixed Ip (addFixedIp Action) (DEPRECATED) (microversion = 2.1)osc compute server add-floating-ip21
— Add (Associate) Floating Ip (addFloatingIp Action) (DEPRECATED) (microversion = 2.1)osc compute server add-security-group
— Add Security Group To A Server (addSecurityGroup Action)osc compute server change-password
— Change Administrative Password (changePassword Action)osc compute server confirm-resize
— Confirm Resized Server (confirmResize Action)osc compute server create294
— Create Server (microversion = 2.94)osc compute server create290
— Create Server (microversion = 2.90)osc compute server create274
— Create Server (microversion = 2.74)osc compute server create267
— Create Server (microversion = 2.67)osc compute server create263
— Create Server (microversion = 2.63)osc compute server create257
— Create Server (microversion = 2.57)osc compute server create252
— Create Server (microversion = 2.52)osc compute server create242
— Create Server (microversion = 2.42)osc compute server create237
— Create Server (microversion = 2.37)osc compute server create233
— Create Server (microversion = 2.33)osc compute server create232
— Create Server (microversion = 2.32)osc compute server create219
— Create Server (microversion = 2.19)osc compute server create21
— Create Server (microversion = 2.1)osc compute server create-backup21
— Create Server Back Up (createBackup Action) (microversion = 2.1)osc compute server create-image21
— Create Image (createImage Action) (microversion = 2.1)osc compute server delete
— Delete Serverosc compute server diagnostic
— Show Server Diagnosticsosc compute server evacuate214
— Evacuate Server (evacuate Action) (microversion = 2.14)osc compute server evacuate229
— Evacuate Server (evacuate Action) (microversion = 2.29)osc compute server evacuate268
— Evacuate Server (evacuate Action) (microversion = 2.68)osc compute server evacuate295
— Evacuate Server (evacuate Action) (microversion = 2.95)osc compute server force-delete
— Force-Delete Server (forceDelete Action)osc compute server get-console-output
— Show Console Output (os-getConsoleOutput Action)osc compute server instance-action
— Servers actionsosc compute server interface
— Port interfaces (servers, os-interface)osc compute server inject-network-info
— Inject Network Information (injectNetworkInfo Action)osc compute server ip
— Servers IPs (servers, ips)osc compute server list
— List Servers Detailedosc compute server live-migrate20
— Live-Migrate Server (os-migrateLive Action) (microversion = 2.0)osc compute server live-migrate225
— Live-Migrate Server (os-migrateLive Action) (microversion = 2.25)osc compute server live-migrate230
— Live-Migrate Server (os-migrateLive Action) (microversion = 2.30)osc compute server live-migrate268
— Live-Migrate Server (os-migrateLive Action) (microversion = 2.68)osc compute server lock273
— Lock Server (lock Action) (microversion = 2.73)osc compute server metadata
— Server metadataosc compute server migrate256
— Migrate Server (migrate Action) (microversion = 2.56)osc compute server migration
— Server migrations (servers, migrations)osc compute server password
— Servers passwordosc compute server pause
— Pause Server (pause Action)osc compute server reset-state
— Reset Server State (os-resetState Action)osc compute server reboot
— Reboot Server (reboot Action)osc compute server rebuild21
— Rebuild Server (rebuild Action) (microversion = 2.1)osc compute server rebuild219
— Rebuild Server (rebuild Action) (microversion = 2.19)osc compute server rebuild254
— Rebuild Server (rebuild Action) (microversion = 2.54)osc compute server rebuild257
— Rebuild Server (rebuild Action) (microversion = 2.57)osc compute server rebuild263
— Rebuild Server (rebuild Action) (microversion = 2.63)osc compute server rebuild290
— Rebuild Server (rebuild Action) (microversion = 2.90)osc compute server rebuild294
— Rebuild Server (rebuild Action) (microversion = 2.94)osc compute server remote-console
— Server Consolesosc compute server remove-fixed-ip21
— Remove (Disassociate) Fixed Ip (removeFixedIp Action) (DEPRECATED) (microversion = 2.1)osc compute server remove-floating-ip21
— Remove (Disassociate) Floating Ip (removeFloatingIp Action) (DEPRECATED) (microversion = 2.1)osc compute server remove-security-group
— Remove Security Group From A Server (removeSecurityGroup Action)osc compute server rescue
— Rescue Server (rescue Action)osc compute server reset-network
— Reset Networking On A Server (resetNetwork Action) (DEPRECATED)osc compute server resize
— Resize Server (resize Action)osc compute server restore
— Restore Soft-Deleted Instance (restore Action)osc compute server resume
— Resume Suspended Server (resume Action)osc compute server revert-resize
— Revert Resized Server (revertResize Action)osc compute server security-groups
— List Security Groups By Serverosc compute server set21
— Update Server (microversion = 2.1)osc compute server set219
— Update Server (microversion = 2.19)osc compute server set290
— Update Server (microversion = 2.90)osc compute server set294
— Update Server (microversion = 2.94)osc compute server shelve
— Shelve Server (shelve Action)osc compute server shelve-offload
— Shelf-Offload (Remove) Server (shelveOffload Action)osc compute server show
— Show Server Detailsosc compute server start
— Start Server (os-start Action)osc compute server stop
— Stop Server (os-stop Action)osc compute server suspend
— Suspend Server (suspend Action)osc compute server tag
— Lists tags, creates, replaces or deletes one or more tags for a server, checks the existence of a tag for a serverosc compute server topology
— Show Server Topologyosc compute server trigger-crash-dump217
— Command without description in OpenAPIosc compute server unlock21
— Unlock Server (unlock Action) (microversion = 2.1)osc compute server unpause
— Unpause Server (unpause Action)osc compute server unrescue
— Unrescue Server (unrescue Action)osc compute server unshelve277
— Unshelve (Restore) Shelved Server (unshelve Action) (microversion = 2.77)osc compute server unshelve291
— Unshelve (Restore) Shelved Server (unshelve Action) (microversion = 2.91)osc compute server volume-attachment
— Servers with volume attachments
osc compute server add-fixed-ip21
Adds a fixed IP address to a server instance, which associates that address with the server. The fixed IP address is retrieved from the network that you specify in the request.
Specify the addFixedIp
action and the network ID in the request body.
Policy defaults enable only users with the administrative role or the owner of the server to perform this operation. Cloud providers can change these permissions through the policy.json
file.
Normal response codes: 202
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute server add-fixed-ip21 --network-id <NETWORK_ID> <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
--network-id <NETWORK_ID>
— The network ID
osc compute server add-floating-ip21
Adds a floating IP address to a server, which associates that address with the server.
A pool of floating IP addresses, configured by the cloud administrator, is available in OpenStack Compute. The project quota defines the maximum number of floating IP addresses that you can allocate to the project. After you create (allocate) a floating IPaddress for a project, you can associate that address with the server. Specify the addFloatingIp
action in the request body.
If an instance is connected to multiple networks, you can associate a floating IP address with a specific fixed IP address by using the optional fixed_address
parameter.
Preconditions
The server must exist.
You can only add a floating IP address to the server when its status is ACTIVE
or STOPPED
Normal response codes: 202
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute server add-floating-ip21 [OPTIONS] --address <ADDRESS> <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
--address <ADDRESS>
— The fixed IP address with which you want to associate the floating IP address--fixed-address <FIXED_ADDRESS>
— The fixed IP address with which you want to associate the floating IP address
osc compute server add-security-group
Adds a security group to a server.
Specify the addSecurityGroup
action in the request body.
Normal response codes: 202
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404), conflict(409)
Usage: osc compute server add-security-group [OPTIONS] --name <NAME> <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
--name <NAME>
— The security group name--property <key=value>
— Additional properties to be sent with the request
osc compute server change-password
Changes the administrative password for a server.
Specify the changePassword
action in the request body.
Policy defaults enable only users with the administrative role or the owner of the server to perform this operation. Cloud providers can change these permissions through the policy.json
file.
Normal response codes: 202
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404), conflict(409), notImplemented(501)
Usage: osc compute server change-password --admin-pass <ADMIN_PASS> <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
--admin-pass <ADMIN_PASS>
— The administrative password for the server
osc compute server confirm-resize
Confirms a pending resize action for a server.
Specify the confirmResize
action in the request body.
After you make this request, you typically must keep polling the server status to determine whether the request succeeded. A successfully confirming resize operation shows a status of ACTIVE
or SHUTOFF
and a migration status of confirmed
. You can also see the resized server in the compute node that OpenStack Compute manages.
Preconditions
You can only confirm the resized server where the status is VERIFY_RESIZE
.
If the server is locked, you must have administrator privileges to confirm the server.
Troubleshooting
If the server status remains VERIFY_RESIZE
, the request failed. Ensure you meet the preconditions and run the request again. If the request fails again, the server status should be ERROR
and a migration status of error
. Investigate the compute back end or ask your cloud provider. There are some options for trying to correct the server status:
Note that the cloud provider may still need to cleanup any orphaned resources on the source hypervisor.
Normal response codes: 204
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404), conflict(409)
Usage: osc compute server confirm-resize --confirm-resize <JSON> <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
--confirm-resize <JSON>
osc compute server create294
Creates a server.
The progress of this operation depends on the location of the requested image, network I/O, host load, selected flavor, and other factors.
To check the progress of the request, make a GET /servers/{id}
request. This call returns a progress attribute, which is a percentage value from 0 to 100.
The Location
header returns the full URL to the newly created server and is available as a self
and bookmark
link in the server representation.
When you create a server, the response shows only the server ID, its links, and the admin password. You can get additional attributes through subsequent GET
requests on the server.
Include the block_device_mapping_v2
parameter in the create request body to boot a server from a volume.
Include the key_name
parameter in the create request body to add a keypair to the server when you create it. To create a keypair, make a create keypair request.
Preconditions
Asynchronous postconditions
Troubleshooting
Normal response codes: 202
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404), conflict(409)
Usage: osc compute server create294 [OPTIONS] --flavor-ref <FLAVOR_REF> --name <NAME> <--auto-networks|--networks <JSON>|--none-networks>
Options:
-
--build-near-host-ip <BUILD_NEAR_HOST_IP>
— Schedule the server on a host in the network specified with this parameter and a cidr (os:scheduler_hints.cidr
). It is available whenSimpleCIDRAffinityFilter
is available on cloud side -
--cidr <CIDR>
— Schedule the server on a host in the network specified with an IP address (os:scheduler_hints:build_near_host_ip
) and this parameter. Ifos:scheduler_hints:build_near_host_ip
is specified and this parameter is omitted,/24
is used. It is available whenSimpleCIDRAffinityFilter
is available on cloud side -
--different-cell <DIFFERENT_CELL>
— A list of cell routes or a cell route (string). Schedule the server in a cell that is not specified. It is available whenDifferentCellFilter
is available on cloud side that is cell v1 environment.Parameter is an array, may be provided multiple times.
-
--different-host <DIFFERENT_HOST>
— A list of server UUIDs or a server UUID. Schedule the server on a different host from a set of servers. It is available whenDifferentHostFilter
is available on cloud side.Parameter is an array, may be provided multiple times.
-
--group <GROUP>
— The server group UUID. Schedule the server according to a policy of the server group (anti-affinity
,affinity
,soft-anti-affinity
orsoft-affinity
). It is available whenServerGroupAffinityFilter
,ServerGroupAntiAffinityFilter
,ServerGroupSoftAntiAffinityWeigher
,ServerGroupSoftAffinityWeigher
are available on cloud side -
--query <JSON>
— Schedule the server by using a custom filter in JSON format. For example:It is available when
JsonFilter
is available on cloud side. -
--same-host <SAME_HOST>
— A list of server UUIDs or a server UUID. Schedule the server on the same host as another server in a set of servers. It is available whenSameHostFilter
is available on cloud side.Parameter is an array, may be provided multiple times.
-
--target-cell <TARGET_CELL>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--access-ipv4 <ACCESS_IPV4>
— IPv4 address that should be used to access this server -
--access-ipv6 <ACCESS_IPV6>
— IPv6 address that should be used to access this server -
--admin-pass <ADMIN_PASS>
— The administrative password of the server. If you omit this parameter, the operation generates a new password -
--availability-zone <AVAILABILITY_ZONE>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--block-device-mapping <JSON>
— Parameter is an array, may be provided multiple times -
--block-device-mapping-v2 <JSON>
— Enables fine grained control of the block device mapping for an instance. This is typically used for booting servers from volumes. An example format would look as follows:text > "block_device_mapping_v2": [{ > "boot_index": "0", > "uuid": "ac408821-c95a-448f-9292-73986c790911", > "source_type": "image", > "volume_size": "25", > "destination_type": "volume", > "delete_on_termination": true, > "tag": "disk1", > "disk_bus": "scsi"}] > >
In microversion 2.32,
tag
is an optional string attribute that can be used to assign a tag to the block device. This tag is then exposed to the guest in the metadata API and the config drive and is associated to hardware metadata for that block device, such as bus (ex: SCSI), bus address (ex: 1:0:2:0), and serial.A bug has caused the
tag
attribute to no longer be accepted starting with version 2.33. It has been restored in version 2.42.Parameter is an array, may be provided multiple times.
-
--config-drive <CONFIG_DRIVE>
— Indicates whether a config drive enables metadata injection. The config_drive setting provides information about a drive that the instance can mount at boot time. The instance reads files from the drive to get information that is normally available through the metadata service. This metadata is different from the user data. Not all cloud providers enable theconfig_drive
. Read more in the OpenStack End User GuidePossible values:
true
,false
-
--description <DESCRIPTION>
— A free form description of the server. Limited to 255 characters in length. Before microversion 2.19 this was set to the server name.New in version 2.19
-
--flavor-ref <FLAVOR_REF>
— The flavor reference, as an ID (including a UUID) or full URL, for the flavor for your server instance -
--host <HOST>
— The hostname of the hypervisor on which the server is to be created. The API will return 400 if no hypervisors are found with the given hostname. By default, it can be specified by administrators only.New in version 2.74
-
--hostname <HOSTNAME>
— The hostname to configure for the instance in the metadata service.Starting with microversion 2.94, this can be a Fully Qualified Domain Name (FQDN) of up to 255 characters in length.
Note
This information is published via the metadata service and requires application such as
cloud-init
to propagate it through to the instance.New in version 2.90
-
--hypervisor-hostname <HYPERVISOR_HOSTNAME>
— The hostname of the hypervisor on which the server is to be created. The API will return 400 if no hypervisors are found with the given hostname. By default, it can be specified by administrators only.New in version 2.74
-
--image-ref <IMAGE_REF>
— The UUID of the image to use for your server instance. This is not required in case of boot from volume. In all other cases it is required and must be a valid UUID otherwise API will return 400 -
--key-name <KEY_NAME>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--max-count <MAX_COUNT>
-
--metadata <key=value>
— Metadata key and value pairs. The maximum size of the metadata key and value is 255 bytes each -
--min-count <MIN_COUNT>
-
--name <NAME>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--auto-networks
-
--networks <JSON>
— Parameter is an array, may be provided multiple times -
--none-networks
-
--os-dcf-disk-config <OS_DCF_DISK_CONFIG>
— Controls how the API partitions the disk when you create, rebuild, or resize servers. A server inherits theOS-DCF:diskConfig
value from the image from which it was created, and an image inherits theOS-DCF:diskConfig
value from the server from which it was created. To override the inherited setting, you can include this attribute in the request body of a server create, rebuild, or resize request. If theOS-DCF:diskConfig
value for an image isMANUAL
, you cannot create a server from that image and set itsOS-DCF:diskConfig
value toAUTO
. A valid value is:AUTO
. The API builds the server with a single partition the size of the target flavor disk. The API automatically adjusts the file system to fit the entire partition. -MANUAL
. The API builds the server by using whatever partition scheme and file system is in the source image. If the target flavor disk is larger, the API does not partition the remaining disk space.
Possible values:
auto
,manual
-
--return-reservation-id <RETURN_RESERVATION_ID>
— Indicates whether a config drive enables metadata injection. The config_drive setting provides information about a drive that the instance can mount at boot time. The instance reads files from the drive to get information that is normally available through the metadata service. This metadata is different from the user data. Not all cloud providers enable theconfig_drive
. Read more in the OpenStack End User GuidePossible values:
true
,false
-
--security-groups <SECURITY_GROUPS>
— One or more security groups. Specify the name of the security group in thename
attribute. If you omit this attribute, the API creates the server in thedefault
security group. Requested security groups are not applied to pre-existing ports.Parameter is an array, may be provided multiple times.
-
--tags <TAGS>
— A list of tags. Tags have the following restrictions:- Tag is a Unicode bytestring no longer than 60 characters. - Tag is a non-empty string. - ‘/’ is not allowed to be in a tag name - Comma is not allowed to be in a tag name in order to simplify requests that specify lists of tags - All other characters are allowed to be in a tag name - Each server can have up to 50 tags.
New in version 2.52
Parameter is an array, may be provided multiple times.
-
--trusted-image-certificates <TRUSTED_IMAGE_CERTIFICATES>
— A list of trusted certificate IDs, which are used during image signature verification to verify the signing certificate. The list is restricted to a maximum of 50 IDs. This parameter is optional in server create requests if allowed by policy, and is not supported for volume-backed instances.New in version 2.63
Parameter is an array, may be provided multiple times.
-
--user-data <USER_DATA>
— Configuration information or scripts to use upon launch. Must be Base64 encoded. Restricted to 65535 bytes.Note
The
null
value allowed in Nova legacy v2 API, but due to the strict input validation, it isn’t allowed in Nova v2.1 API.
osc compute server create290
Creates a server.
The progress of this operation depends on the location of the requested image, network I/O, host load, selected flavor, and other factors.
To check the progress of the request, make a GET /servers/{id}
request. This call returns a progress attribute, which is a percentage value from 0 to 100.
The Location
header returns the full URL to the newly created server and is available as a self
and bookmark
link in the server representation.
When you create a server, the response shows only the server ID, its links, and the admin password. You can get additional attributes through subsequent GET
requests on the server.
Include the block_device_mapping_v2
parameter in the create request body to boot a server from a volume.
Include the key_name
parameter in the create request body to add a keypair to the server when you create it. To create a keypair, make a create keypair request.
Preconditions
Asynchronous postconditions
Troubleshooting
Normal response codes: 202
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404), conflict(409)
Usage: osc compute server create290 [OPTIONS] --flavor-ref <FLAVOR_REF> --name <NAME> <--auto-networks|--networks <JSON>|--none-networks>
Options:
-
--build-near-host-ip <BUILD_NEAR_HOST_IP>
— Schedule the server on a host in the network specified with this parameter and a cidr (os:scheduler_hints.cidr
). It is available whenSimpleCIDRAffinityFilter
is available on cloud side -
--cidr <CIDR>
— Schedule the server on a host in the network specified with an IP address (os:scheduler_hints:build_near_host_ip
) and this parameter. Ifos:scheduler_hints:build_near_host_ip
is specified and this parameter is omitted,/24
is used. It is available whenSimpleCIDRAffinityFilter
is available on cloud side -
--different-cell <DIFFERENT_CELL>
— A list of cell routes or a cell route (string). Schedule the server in a cell that is not specified. It is available whenDifferentCellFilter
is available on cloud side that is cell v1 environment.Parameter is an array, may be provided multiple times.
-
--different-host <DIFFERENT_HOST>
— A list of server UUIDs or a server UUID. Schedule the server on a different host from a set of servers. It is available whenDifferentHostFilter
is available on cloud side.Parameter is an array, may be provided multiple times.
-
--group <GROUP>
— The server group UUID. Schedule the server according to a policy of the server group (anti-affinity
,affinity
,soft-anti-affinity
orsoft-affinity
). It is available whenServerGroupAffinityFilter
,ServerGroupAntiAffinityFilter
,ServerGroupSoftAntiAffinityWeigher
,ServerGroupSoftAffinityWeigher
are available on cloud side -
--query <JSON>
— Schedule the server by using a custom filter in JSON format. For example:It is available when
JsonFilter
is available on cloud side. -
--same-host <SAME_HOST>
— A list of server UUIDs or a server UUID. Schedule the server on the same host as another server in a set of servers. It is available whenSameHostFilter
is available on cloud side.Parameter is an array, may be provided multiple times.
-
--target-cell <TARGET_CELL>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--access-ipv4 <ACCESS_IPV4>
— IPv4 address that should be used to access this server -
--access-ipv6 <ACCESS_IPV6>
— IPv6 address that should be used to access this server -
--admin-pass <ADMIN_PASS>
— The administrative password of the server. If you omit this parameter, the operation generates a new password -
--availability-zone <AVAILABILITY_ZONE>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--block-device-mapping <JSON>
— Parameter is an array, may be provided multiple times -
--block-device-mapping-v2 <JSON>
— Enables fine grained control of the block device mapping for an instance. This is typically used for booting servers from volumes. An example format would look as follows:text > "block_device_mapping_v2": [{ > "boot_index": "0", > "uuid": "ac408821-c95a-448f-9292-73986c790911", > "source_type": "image", > "volume_size": "25", > "destination_type": "volume", > "delete_on_termination": true, > "tag": "disk1", > "disk_bus": "scsi"}] > >
In microversion 2.32,
tag
is an optional string attribute that can be used to assign a tag to the block device. This tag is then exposed to the guest in the metadata API and the config drive and is associated to hardware metadata for that block device, such as bus (ex: SCSI), bus address (ex: 1:0:2:0), and serial.A bug has caused the
tag
attribute to no longer be accepted starting with version 2.33. It has been restored in version 2.42.Parameter is an array, may be provided multiple times.
-
--config-drive <CONFIG_DRIVE>
— Indicates whether a config drive enables metadata injection. The config_drive setting provides information about a drive that the instance can mount at boot time. The instance reads files from the drive to get information that is normally available through the metadata service. This metadata is different from the user data. Not all cloud providers enable theconfig_drive
. Read more in the OpenStack End User GuidePossible values:
true
,false
-
--description <DESCRIPTION>
— A free form description of the server. Limited to 255 characters in length. Before microversion 2.19 this was set to the server name.New in version 2.19
-
--flavor-ref <FLAVOR_REF>
— The flavor reference, as an ID (including a UUID) or full URL, for the flavor for your server instance -
--host <HOST>
— The hostname of the hypervisor on which the server is to be created. The API will return 400 if no hypervisors are found with the given hostname. By default, it can be specified by administrators only.New in version 2.74
-
--hostname <HOSTNAME>
— The hostname to configure for the instance in the metadata service.Starting with microversion 2.94, this can be a Fully Qualified Domain Name (FQDN) of up to 255 characters in length.
Note
This information is published via the metadata service and requires application such as
cloud-init
to propagate it through to the instance.New in version 2.90
-
--hypervisor-hostname <HYPERVISOR_HOSTNAME>
— The hostname of the hypervisor on which the server is to be created. The API will return 400 if no hypervisors are found with the given hostname. By default, it can be specified by administrators only.New in version 2.74
-
--image-ref <IMAGE_REF>
— The UUID of the image to use for your server instance. This is not required in case of boot from volume. In all other cases it is required and must be a valid UUID otherwise API will return 400 -
--key-name <KEY_NAME>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--max-count <MAX_COUNT>
-
--metadata <key=value>
— Metadata key and value pairs. The maximum size of the metadata key and value is 255 bytes each -
--min-count <MIN_COUNT>
-
--name <NAME>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--auto-networks
-
--networks <JSON>
— Parameter is an array, may be provided multiple times -
--none-networks
-
--os-dcf-disk-config <OS_DCF_DISK_CONFIG>
— Controls how the API partitions the disk when you create, rebuild, or resize servers. A server inherits theOS-DCF:diskConfig
value from the image from which it was created, and an image inherits theOS-DCF:diskConfig
value from the server from which it was created. To override the inherited setting, you can include this attribute in the request body of a server create, rebuild, or resize request. If theOS-DCF:diskConfig
value for an image isMANUAL
, you cannot create a server from that image and set itsOS-DCF:diskConfig
value toAUTO
. A valid value is:AUTO
. The API builds the server with a single partition the size of the target flavor disk. The API automatically adjusts the file system to fit the entire partition. -MANUAL
. The API builds the server by using whatever partition scheme and file system is in the source image. If the target flavor disk is larger, the API does not partition the remaining disk space.
Possible values:
auto
,manual
-
--return-reservation-id <RETURN_RESERVATION_ID>
— Indicates whether a config drive enables metadata injection. The config_drive setting provides information about a drive that the instance can mount at boot time. The instance reads files from the drive to get information that is normally available through the metadata service. This metadata is different from the user data. Not all cloud providers enable theconfig_drive
. Read more in the OpenStack End User GuidePossible values:
true
,false
-
--security-groups <SECURITY_GROUPS>
— One or more security groups. Specify the name of the security group in thename
attribute. If you omit this attribute, the API creates the server in thedefault
security group. Requested security groups are not applied to pre-existing ports.Parameter is an array, may be provided multiple times.
-
--tags <TAGS>
— A list of tags. Tags have the following restrictions:- Tag is a Unicode bytestring no longer than 60 characters. - Tag is a non-empty string. - ‘/’ is not allowed to be in a tag name - Comma is not allowed to be in a tag name in order to simplify requests that specify lists of tags - All other characters are allowed to be in a tag name - Each server can have up to 50 tags.
New in version 2.52
Parameter is an array, may be provided multiple times.
-
--trusted-image-certificates <TRUSTED_IMAGE_CERTIFICATES>
— A list of trusted certificate IDs, which are used during image signature verification to verify the signing certificate. The list is restricted to a maximum of 50 IDs. This parameter is optional in server create requests if allowed by policy, and is not supported for volume-backed instances.New in version 2.63
Parameter is an array, may be provided multiple times.
-
--user-data <USER_DATA>
— Configuration information or scripts to use upon launch. Must be Base64 encoded. Restricted to 65535 bytes.Note
The
null
value allowed in Nova legacy v2 API, but due to the strict input validation, it isn’t allowed in Nova v2.1 API.
osc compute server create274
Creates a server.
The progress of this operation depends on the location of the requested image, network I/O, host load, selected flavor, and other factors.
To check the progress of the request, make a GET /servers/{id}
request. This call returns a progress attribute, which is a percentage value from 0 to 100.
The Location
header returns the full URL to the newly created server and is available as a self
and bookmark
link in the server representation.
When you create a server, the response shows only the server ID, its links, and the admin password. You can get additional attributes through subsequent GET
requests on the server.
Include the block_device_mapping_v2
parameter in the create request body to boot a server from a volume.
Include the key_name
parameter in the create request body to add a keypair to the server when you create it. To create a keypair, make a create keypair request.
Preconditions
Asynchronous postconditions
Troubleshooting
Normal response codes: 202
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404), conflict(409)
Usage: osc compute server create274 [OPTIONS] --flavor-ref <FLAVOR_REF> --name <NAME> <--auto-networks|--networks <JSON>|--none-networks>
Options:
-
--build-near-host-ip <BUILD_NEAR_HOST_IP>
— Schedule the server on a host in the network specified with this parameter and a cidr (os:scheduler_hints.cidr
). It is available whenSimpleCIDRAffinityFilter
is available on cloud side -
--cidr <CIDR>
— Schedule the server on a host in the network specified with an IP address (os:scheduler_hints:build_near_host_ip
) and this parameter. Ifos:scheduler_hints:build_near_host_ip
is specified and this parameter is omitted,/24
is used. It is available whenSimpleCIDRAffinityFilter
is available on cloud side -
--different-cell <DIFFERENT_CELL>
— A list of cell routes or a cell route (string). Schedule the server in a cell that is not specified. It is available whenDifferentCellFilter
is available on cloud side that is cell v1 environment.Parameter is an array, may be provided multiple times.
-
--different-host <DIFFERENT_HOST>
— A list of server UUIDs or a server UUID. Schedule the server on a different host from a set of servers. It is available whenDifferentHostFilter
is available on cloud side.Parameter is an array, may be provided multiple times.
-
--group <GROUP>
— The server group UUID. Schedule the server according to a policy of the server group (anti-affinity
,affinity
,soft-anti-affinity
orsoft-affinity
). It is available whenServerGroupAffinityFilter
,ServerGroupAntiAffinityFilter
,ServerGroupSoftAntiAffinityWeigher
,ServerGroupSoftAffinityWeigher
are available on cloud side -
--query <JSON>
— Schedule the server by using a custom filter in JSON format. For example:It is available when
JsonFilter
is available on cloud side. -
--same-host <SAME_HOST>
— A list of server UUIDs or a server UUID. Schedule the server on the same host as another server in a set of servers. It is available whenSameHostFilter
is available on cloud side.Parameter is an array, may be provided multiple times.
-
--target-cell <TARGET_CELL>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--access-ipv4 <ACCESS_IPV4>
— IPv4 address that should be used to access this server -
--access-ipv6 <ACCESS_IPV6>
— IPv6 address that should be used to access this server -
--admin-pass <ADMIN_PASS>
— The administrative password of the server. If you omit this parameter, the operation generates a new password -
--availability-zone <AVAILABILITY_ZONE>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--block-device-mapping <JSON>
— Parameter is an array, may be provided multiple times -
--block-device-mapping-v2 <JSON>
— Enables fine grained control of the block device mapping for an instance. This is typically used for booting servers from volumes. An example format would look as follows:text > "block_device_mapping_v2": [{ > "boot_index": "0", > "uuid": "ac408821-c95a-448f-9292-73986c790911", > "source_type": "image", > "volume_size": "25", > "destination_type": "volume", > "delete_on_termination": true, > "tag": "disk1", > "disk_bus": "scsi"}] > >
In microversion 2.32,
tag
is an optional string attribute that can be used to assign a tag to the block device. This tag is then exposed to the guest in the metadata API and the config drive and is associated to hardware metadata for that block device, such as bus (ex: SCSI), bus address (ex: 1:0:2:0), and serial.A bug has caused the
tag
attribute to no longer be accepted starting with version 2.33. It has been restored in version 2.42.Parameter is an array, may be provided multiple times.
-
--config-drive <CONFIG_DRIVE>
— Indicates whether a config drive enables metadata injection. The config_drive setting provides information about a drive that the instance can mount at boot time. The instance reads files from the drive to get information that is normally available through the metadata service. This metadata is different from the user data. Not all cloud providers enable theconfig_drive
. Read more in the OpenStack End User GuidePossible values:
true
,false
-
--description <DESCRIPTION>
— A free form description of the server. Limited to 255 characters in length. Before microversion 2.19 this was set to the server name.New in version 2.19
-
--flavor-ref <FLAVOR_REF>
— The flavor reference, as an ID (including a UUID) or full URL, for the flavor for your server instance -
--host <HOST>
— The hostname of the hypervisor on which the server is to be created. The API will return 400 if no hypervisors are found with the given hostname. By default, it can be specified by administrators only.New in version 2.74
-
--hypervisor-hostname <HYPERVISOR_HOSTNAME>
— The hostname of the hypervisor on which the server is to be created. The API will return 400 if no hypervisors are found with the given hostname. By default, it can be specified by administrators only.New in version 2.74
-
--image-ref <IMAGE_REF>
— The UUID of the image to use for your server instance. This is not required in case of boot from volume. In all other cases it is required and must be a valid UUID otherwise API will return 400 -
--key-name <KEY_NAME>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--max-count <MAX_COUNT>
-
--metadata <key=value>
— Metadata key and value pairs. The maximum size of the metadata key and value is 255 bytes each -
--min-count <MIN_COUNT>
-
--name <NAME>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--auto-networks
-
--networks <JSON>
— Parameter is an array, may be provided multiple times -
--none-networks
-
--os-dcf-disk-config <OS_DCF_DISK_CONFIG>
— Controls how the API partitions the disk when you create, rebuild, or resize servers. A server inherits theOS-DCF:diskConfig
value from the image from which it was created, and an image inherits theOS-DCF:diskConfig
value from the server from which it was created. To override the inherited setting, you can include this attribute in the request body of a server create, rebuild, or resize request. If theOS-DCF:diskConfig
value for an image isMANUAL
, you cannot create a server from that image and set itsOS-DCF:diskConfig
value toAUTO
. A valid value is:AUTO
. The API builds the server with a single partition the size of the target flavor disk. The API automatically adjusts the file system to fit the entire partition. -MANUAL
. The API builds the server by using whatever partition scheme and file system is in the source image. If the target flavor disk is larger, the API does not partition the remaining disk space.
Possible values:
auto
,manual
-
--return-reservation-id <RETURN_RESERVATION_ID>
— Indicates whether a config drive enables metadata injection. The config_drive setting provides information about a drive that the instance can mount at boot time. The instance reads files from the drive to get information that is normally available through the metadata service. This metadata is different from the user data. Not all cloud providers enable theconfig_drive
. Read more in the OpenStack End User GuidePossible values:
true
,false
-
--security-groups <SECURITY_GROUPS>
— One or more security groups. Specify the name of the security group in thename
attribute. If you omit this attribute, the API creates the server in thedefault
security group. Requested security groups are not applied to pre-existing ports.Parameter is an array, may be provided multiple times.
-
--tags <TAGS>
— A list of tags. Tags have the following restrictions:- Tag is a Unicode bytestring no longer than 60 characters. - Tag is a non-empty string. - ‘/’ is not allowed to be in a tag name - Comma is not allowed to be in a tag name in order to simplify requests that specify lists of tags - All other characters are allowed to be in a tag name - Each server can have up to 50 tags.
New in version 2.52
Parameter is an array, may be provided multiple times.
-
--trusted-image-certificates <TRUSTED_IMAGE_CERTIFICATES>
— A list of trusted certificate IDs, which are used during image signature verification to verify the signing certificate. The list is restricted to a maximum of 50 IDs. This parameter is optional in server create requests if allowed by policy, and is not supported for volume-backed instances.New in version 2.63
Parameter is an array, may be provided multiple times.
-
--user-data <USER_DATA>
— Configuration information or scripts to use upon launch. Must be Base64 encoded. Restricted to 65535 bytes.Note
The
null
value allowed in Nova legacy v2 API, but due to the strict input validation, it isn’t allowed in Nova v2.1 API.
osc compute server create267
Creates a server.
The progress of this operation depends on the location of the requested image, network I/O, host load, selected flavor, and other factors.
To check the progress of the request, make a GET /servers/{id}
request. This call returns a progress attribute, which is a percentage value from 0 to 100.
The Location
header returns the full URL to the newly created server and is available as a self
and bookmark
link in the server representation.
When you create a server, the response shows only the server ID, its links, and the admin password. You can get additional attributes through subsequent GET
requests on the server.
Include the block_device_mapping_v2
parameter in the create request body to boot a server from a volume.
Include the key_name
parameter in the create request body to add a keypair to the server when you create it. To create a keypair, make a create keypair request.
Preconditions
Asynchronous postconditions
Troubleshooting
Normal response codes: 202
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404), conflict(409)
Usage: osc compute server create267 [OPTIONS] --flavor-ref <FLAVOR_REF> --name <NAME> <--auto-networks|--networks <JSON>|--none-networks>
Options:
-
--build-near-host-ip <BUILD_NEAR_HOST_IP>
— Schedule the server on a host in the network specified with this parameter and a cidr (os:scheduler_hints.cidr
). It is available whenSimpleCIDRAffinityFilter
is available on cloud side -
--cidr <CIDR>
— Schedule the server on a host in the network specified with an IP address (os:scheduler_hints:build_near_host_ip
) and this parameter. Ifos:scheduler_hints:build_near_host_ip
is specified and this parameter is omitted,/24
is used. It is available whenSimpleCIDRAffinityFilter
is available on cloud side -
--different-cell <DIFFERENT_CELL>
— A list of cell routes or a cell route (string). Schedule the server in a cell that is not specified. It is available whenDifferentCellFilter
is available on cloud side that is cell v1 environment.Parameter is an array, may be provided multiple times.
-
--different-host <DIFFERENT_HOST>
— A list of server UUIDs or a server UUID. Schedule the server on a different host from a set of servers. It is available whenDifferentHostFilter
is available on cloud side.Parameter is an array, may be provided multiple times.
-
--group <GROUP>
— The server group UUID. Schedule the server according to a policy of the server group (anti-affinity
,affinity
,soft-anti-affinity
orsoft-affinity
). It is available whenServerGroupAffinityFilter
,ServerGroupAntiAffinityFilter
,ServerGroupSoftAntiAffinityWeigher
,ServerGroupSoftAffinityWeigher
are available on cloud side -
--query <JSON>
— Schedule the server by using a custom filter in JSON format. For example:It is available when
JsonFilter
is available on cloud side. -
--same-host <SAME_HOST>
— A list of server UUIDs or a server UUID. Schedule the server on the same host as another server in a set of servers. It is available whenSameHostFilter
is available on cloud side.Parameter is an array, may be provided multiple times.
-
--target-cell <TARGET_CELL>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--access-ipv4 <ACCESS_IPV4>
— IPv4 address that should be used to access this server -
--access-ipv6 <ACCESS_IPV6>
— IPv6 address that should be used to access this server -
--admin-pass <ADMIN_PASS>
— The administrative password of the server. If you omit this parameter, the operation generates a new password -
--availability-zone <AVAILABILITY_ZONE>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--block-device-mapping <JSON>
— Parameter is an array, may be provided multiple times -
--block-device-mapping-v2 <JSON>
— Enables fine grained control of the block device mapping for an instance. This is typically used for booting servers from volumes. An example format would look as follows:text > "block_device_mapping_v2": [{ > "boot_index": "0", > "uuid": "ac408821-c95a-448f-9292-73986c790911", > "source_type": "image", > "volume_size": "25", > "destination_type": "volume", > "delete_on_termination": true, > "tag": "disk1", > "disk_bus": "scsi"}] > >
In microversion 2.32,
tag
is an optional string attribute that can be used to assign a tag to the block device. This tag is then exposed to the guest in the metadata API and the config drive and is associated to hardware metadata for that block device, such as bus (ex: SCSI), bus address (ex: 1:0:2:0), and serial.A bug has caused the
tag
attribute to no longer be accepted starting with version 2.33. It has been restored in version 2.42.Parameter is an array, may be provided multiple times.
-
--config-drive <CONFIG_DRIVE>
— Indicates whether a config drive enables metadata injection. The config_drive setting provides information about a drive that the instance can mount at boot time. The instance reads files from the drive to get information that is normally available through the metadata service. This metadata is different from the user data. Not all cloud providers enable theconfig_drive
. Read more in the OpenStack End User GuidePossible values:
true
,false
-
--description <DESCRIPTION>
— A free form description of the server. Limited to 255 characters in length. Before microversion 2.19 this was set to the server name.New in version 2.19
-
--flavor-ref <FLAVOR_REF>
— The flavor reference, as an ID (including a UUID) or full URL, for the flavor for your server instance -
--image-ref <IMAGE_REF>
— The UUID of the image to use for your server instance. This is not required in case of boot from volume. In all other cases it is required and must be a valid UUID otherwise API will return 400 -
--key-name <KEY_NAME>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--max-count <MAX_COUNT>
-
--metadata <key=value>
— Metadata key and value pairs. The maximum size of the metadata key and value is 255 bytes each -
--min-count <MIN_COUNT>
-
--name <NAME>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--auto-networks
-
--networks <JSON>
— Parameter is an array, may be provided multiple times -
--none-networks
-
--os-dcf-disk-config <OS_DCF_DISK_CONFIG>
— Controls how the API partitions the disk when you create, rebuild, or resize servers. A server inherits theOS-DCF:diskConfig
value from the image from which it was created, and an image inherits theOS-DCF:diskConfig
value from the server from which it was created. To override the inherited setting, you can include this attribute in the request body of a server create, rebuild, or resize request. If theOS-DCF:diskConfig
value for an image isMANUAL
, you cannot create a server from that image and set itsOS-DCF:diskConfig
value toAUTO
. A valid value is:AUTO
. The API builds the server with a single partition the size of the target flavor disk. The API automatically adjusts the file system to fit the entire partition. -MANUAL
. The API builds the server by using whatever partition scheme and file system is in the source image. If the target flavor disk is larger, the API does not partition the remaining disk space.
Possible values:
auto
,manual
-
--return-reservation-id <RETURN_RESERVATION_ID>
— Indicates whether a config drive enables metadata injection. The config_drive setting provides information about a drive that the instance can mount at boot time. The instance reads files from the drive to get information that is normally available through the metadata service. This metadata is different from the user data. Not all cloud providers enable theconfig_drive
. Read more in the OpenStack End User GuidePossible values:
true
,false
-
--security-groups <SECURITY_GROUPS>
— One or more security groups. Specify the name of the security group in thename
attribute. If you omit this attribute, the API creates the server in thedefault
security group. Requested security groups are not applied to pre-existing ports.Parameter is an array, may be provided multiple times.
-
--tags <TAGS>
— A list of tags. Tags have the following restrictions:- Tag is a Unicode bytestring no longer than 60 characters. - Tag is a non-empty string. - ‘/’ is not allowed to be in a tag name - Comma is not allowed to be in a tag name in order to simplify requests that specify lists of tags - All other characters are allowed to be in a tag name - Each server can have up to 50 tags.
New in version 2.52
Parameter is an array, may be provided multiple times.
-
--trusted-image-certificates <TRUSTED_IMAGE_CERTIFICATES>
— A list of trusted certificate IDs, which are used during image signature verification to verify the signing certificate. The list is restricted to a maximum of 50 IDs. This parameter is optional in server create requests if allowed by policy, and is not supported for volume-backed instances.New in version 2.63
Parameter is an array, may be provided multiple times.
-
--user-data <USER_DATA>
— Configuration information or scripts to use upon launch. Must be Base64 encoded. Restricted to 65535 bytes.Note
The
null
value allowed in Nova legacy v2 API, but due to the strict input validation, it isn’t allowed in Nova v2.1 API.
osc compute server create263
Creates a server.
The progress of this operation depends on the location of the requested image, network I/O, host load, selected flavor, and other factors.
To check the progress of the request, make a GET /servers/{id}
request. This call returns a progress attribute, which is a percentage value from 0 to 100.
The Location
header returns the full URL to the newly created server and is available as a self
and bookmark
link in the server representation.
When you create a server, the response shows only the server ID, its links, and the admin password. You can get additional attributes through subsequent GET
requests on the server.
Include the block_device_mapping_v2
parameter in the create request body to boot a server from a volume.
Include the key_name
parameter in the create request body to add a keypair to the server when you create it. To create a keypair, make a create keypair request.
Preconditions
Asynchronous postconditions
Troubleshooting
Normal response codes: 202
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404), conflict(409)
Usage: osc compute server create263 [OPTIONS] --flavor-ref <FLAVOR_REF> --name <NAME> <--auto-networks|--networks <JSON>|--none-networks>
Options:
-
--build-near-host-ip <BUILD_NEAR_HOST_IP>
— Schedule the server on a host in the network specified with this parameter and a cidr (os:scheduler_hints.cidr
). It is available whenSimpleCIDRAffinityFilter
is available on cloud side -
--cidr <CIDR>
— Schedule the server on a host in the network specified with an IP address (os:scheduler_hints:build_near_host_ip
) and this parameter. Ifos:scheduler_hints:build_near_host_ip
is specified and this parameter is omitted,/24
is used. It is available whenSimpleCIDRAffinityFilter
is available on cloud side -
--different-cell <DIFFERENT_CELL>
— A list of cell routes or a cell route (string). Schedule the server in a cell that is not specified. It is available whenDifferentCellFilter
is available on cloud side that is cell v1 environment.Parameter is an array, may be provided multiple times.
-
--different-host <DIFFERENT_HOST>
— A list of server UUIDs or a server UUID. Schedule the server on a different host from a set of servers. It is available whenDifferentHostFilter
is available on cloud side.Parameter is an array, may be provided multiple times.
-
--group <GROUP>
— The server group UUID. Schedule the server according to a policy of the server group (anti-affinity
,affinity
,soft-anti-affinity
orsoft-affinity
). It is available whenServerGroupAffinityFilter
,ServerGroupAntiAffinityFilter
,ServerGroupSoftAntiAffinityWeigher
,ServerGroupSoftAffinityWeigher
are available on cloud side -
--query <JSON>
— Schedule the server by using a custom filter in JSON format. For example:It is available when
JsonFilter
is available on cloud side. -
--same-host <SAME_HOST>
— A list of server UUIDs or a server UUID. Schedule the server on the same host as another server in a set of servers. It is available whenSameHostFilter
is available on cloud side.Parameter is an array, may be provided multiple times.
-
--target-cell <TARGET_CELL>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--access-ipv4 <ACCESS_IPV4>
— IPv4 address that should be used to access this server -
--access-ipv6 <ACCESS_IPV6>
— IPv6 address that should be used to access this server -
--admin-pass <ADMIN_PASS>
— The administrative password of the server. If you omit this parameter, the operation generates a new password -
--availability-zone <AVAILABILITY_ZONE>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--block-device-mapping <JSON>
— Parameter is an array, may be provided multiple times -
--block-device-mapping-v2 <JSON>
— Enables fine grained control of the block device mapping for an instance. This is typically used for booting servers from volumes. An example format would look as follows:text > "block_device_mapping_v2": [{ > "boot_index": "0", > "uuid": "ac408821-c95a-448f-9292-73986c790911", > "source_type": "image", > "volume_size": "25", > "destination_type": "volume", > "delete_on_termination": true, > "tag": "disk1", > "disk_bus": "scsi"}] > >
In microversion 2.32,
tag
is an optional string attribute that can be used to assign a tag to the block device. This tag is then exposed to the guest in the metadata API and the config drive and is associated to hardware metadata for that block device, such as bus (ex: SCSI), bus address (ex: 1:0:2:0), and serial.A bug has caused the
tag
attribute to no longer be accepted starting with version 2.33. It has been restored in version 2.42.Parameter is an array, may be provided multiple times.
-
--config-drive <CONFIG_DRIVE>
— Indicates whether a config drive enables metadata injection. The config_drive setting provides information about a drive that the instance can mount at boot time. The instance reads files from the drive to get information that is normally available through the metadata service. This metadata is different from the user data. Not all cloud providers enable theconfig_drive
. Read more in the OpenStack End User GuidePossible values:
true
,false
-
--description <DESCRIPTION>
— A free form description of the server. Limited to 255 characters in length. Before microversion 2.19 this was set to the server name.New in version 2.19
-
--flavor-ref <FLAVOR_REF>
— The flavor reference, as an ID (including a UUID) or full URL, for the flavor for your server instance -
--image-ref <IMAGE_REF>
— The UUID of the image to use for your server instance. This is not required in case of boot from volume. In all other cases it is required and must be a valid UUID otherwise API will return 400 -
--key-name <KEY_NAME>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--max-count <MAX_COUNT>
-
--metadata <key=value>
— Metadata key and value pairs. The maximum size of the metadata key and value is 255 bytes each -
--min-count <MIN_COUNT>
-
--name <NAME>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--auto-networks
-
--networks <JSON>
— Parameter is an array, may be provided multiple times -
--none-networks
-
--os-dcf-disk-config <OS_DCF_DISK_CONFIG>
— Controls how the API partitions the disk when you create, rebuild, or resize servers. A server inherits theOS-DCF:diskConfig
value from the image from which it was created, and an image inherits theOS-DCF:diskConfig
value from the server from which it was created. To override the inherited setting, you can include this attribute in the request body of a server create, rebuild, or resize request. If theOS-DCF:diskConfig
value for an image isMANUAL
, you cannot create a server from that image and set itsOS-DCF:diskConfig
value toAUTO
. A valid value is:AUTO
. The API builds the server with a single partition the size of the target flavor disk. The API automatically adjusts the file system to fit the entire partition. -MANUAL
. The API builds the server by using whatever partition scheme and file system is in the source image. If the target flavor disk is larger, the API does not partition the remaining disk space.
Possible values:
auto
,manual
-
--return-reservation-id <RETURN_RESERVATION_ID>
— Indicates whether a config drive enables metadata injection. The config_drive setting provides information about a drive that the instance can mount at boot time. The instance reads files from the drive to get information that is normally available through the metadata service. This metadata is different from the user data. Not all cloud providers enable theconfig_drive
. Read more in the OpenStack End User GuidePossible values:
true
,false
-
--security-groups <SECURITY_GROUPS>
— One or more security groups. Specify the name of the security group in thename
attribute. If you omit this attribute, the API creates the server in thedefault
security group. Requested security groups are not applied to pre-existing ports.Parameter is an array, may be provided multiple times.
-
--tags <TAGS>
— A list of tags. Tags have the following restrictions:- Tag is a Unicode bytestring no longer than 60 characters. - Tag is a non-empty string. - ‘/’ is not allowed to be in a tag name - Comma is not allowed to be in a tag name in order to simplify requests that specify lists of tags - All other characters are allowed to be in a tag name - Each server can have up to 50 tags.
New in version 2.52
Parameter is an array, may be provided multiple times.
-
--trusted-image-certificates <TRUSTED_IMAGE_CERTIFICATES>
— A list of trusted certificate IDs, which are used during image signature verification to verify the signing certificate. The list is restricted to a maximum of 50 IDs. This parameter is optional in server create requests if allowed by policy, and is not supported for volume-backed instances.New in version 2.63
Parameter is an array, may be provided multiple times.
-
--user-data <USER_DATA>
— Configuration information or scripts to use upon launch. Must be Base64 encoded. Restricted to 65535 bytes.Note
The
null
value allowed in Nova legacy v2 API, but due to the strict input validation, it isn’t allowed in Nova v2.1 API.
osc compute server create257
Creates a server.
The progress of this operation depends on the location of the requested image, network I/O, host load, selected flavor, and other factors.
To check the progress of the request, make a GET /servers/{id}
request. This call returns a progress attribute, which is a percentage value from 0 to 100.
The Location
header returns the full URL to the newly created server and is available as a self
and bookmark
link in the server representation.
When you create a server, the response shows only the server ID, its links, and the admin password. You can get additional attributes through subsequent GET
requests on the server.
Include the block_device_mapping_v2
parameter in the create request body to boot a server from a volume.
Include the key_name
parameter in the create request body to add a keypair to the server when you create it. To create a keypair, make a create keypair request.
Preconditions
Asynchronous postconditions
Troubleshooting
Normal response codes: 202
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404), conflict(409)
Usage: osc compute server create257 [OPTIONS] --flavor-ref <FLAVOR_REF> --name <NAME> <--auto-networks|--networks <JSON>|--none-networks>
Options:
-
--build-near-host-ip <BUILD_NEAR_HOST_IP>
— Schedule the server on a host in the network specified with this parameter and a cidr (os:scheduler_hints.cidr
). It is available whenSimpleCIDRAffinityFilter
is available on cloud side -
--cidr <CIDR>
— Schedule the server on a host in the network specified with an IP address (os:scheduler_hints:build_near_host_ip
) and this parameter. Ifos:scheduler_hints:build_near_host_ip
is specified and this parameter is omitted,/24
is used. It is available whenSimpleCIDRAffinityFilter
is available on cloud side -
--different-cell <DIFFERENT_CELL>
— A list of cell routes or a cell route (string). Schedule the server in a cell that is not specified. It is available whenDifferentCellFilter
is available on cloud side that is cell v1 environment.Parameter is an array, may be provided multiple times.
-
--different-host <DIFFERENT_HOST>
— A list of server UUIDs or a server UUID. Schedule the server on a different host from a set of servers. It is available whenDifferentHostFilter
is available on cloud side.Parameter is an array, may be provided multiple times.
-
--group <GROUP>
— The server group UUID. Schedule the server according to a policy of the server group (anti-affinity
,affinity
,soft-anti-affinity
orsoft-affinity
). It is available whenServerGroupAffinityFilter
,ServerGroupAntiAffinityFilter
,ServerGroupSoftAntiAffinityWeigher
,ServerGroupSoftAffinityWeigher
are available on cloud side -
--query <JSON>
— Schedule the server by using a custom filter in JSON format. For example:It is available when
JsonFilter
is available on cloud side. -
--same-host <SAME_HOST>
— A list of server UUIDs or a server UUID. Schedule the server on the same host as another server in a set of servers. It is available whenSameHostFilter
is available on cloud side.Parameter is an array, may be provided multiple times.
-
--target-cell <TARGET_CELL>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--access-ipv4 <ACCESS_IPV4>
— IPv4 address that should be used to access this server -
--access-ipv6 <ACCESS_IPV6>
— IPv6 address that should be used to access this server -
--admin-pass <ADMIN_PASS>
— The administrative password of the server. If you omit this parameter, the operation generates a new password -
--availability-zone <AVAILABILITY_ZONE>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--block-device-mapping <JSON>
— Parameter is an array, may be provided multiple times -
--block-device-mapping-v2 <JSON>
— Enables fine grained control of the block device mapping for an instance. This is typically used for booting servers from volumes. An example format would look as follows:text > "block_device_mapping_v2": [{ > "boot_index": "0", > "uuid": "ac408821-c95a-448f-9292-73986c790911", > "source_type": "image", > "volume_size": "25", > "destination_type": "volume", > "delete_on_termination": true, > "tag": "disk1", > "disk_bus": "scsi"}] > >
In microversion 2.32,
tag
is an optional string attribute that can be used to assign a tag to the block device. This tag is then exposed to the guest in the metadata API and the config drive and is associated to hardware metadata for that block device, such as bus (ex: SCSI), bus address (ex: 1:0:2:0), and serial.A bug has caused the
tag
attribute to no longer be accepted starting with version 2.33. It has been restored in version 2.42.Parameter is an array, may be provided multiple times.
-
--config-drive <CONFIG_DRIVE>
— Indicates whether a config drive enables metadata injection. The config_drive setting provides information about a drive that the instance can mount at boot time. The instance reads files from the drive to get information that is normally available through the metadata service. This metadata is different from the user data. Not all cloud providers enable theconfig_drive
. Read more in the OpenStack End User GuidePossible values:
true
,false
-
--description <DESCRIPTION>
— A free form description of the server. Limited to 255 characters in length. Before microversion 2.19 this was set to the server name.New in version 2.19
-
--flavor-ref <FLAVOR_REF>
— The flavor reference, as an ID (including a UUID) or full URL, for the flavor for your server instance -
--image-ref <IMAGE_REF>
— The UUID of the image to use for your server instance. This is not required in case of boot from volume. In all other cases it is required and must be a valid UUID otherwise API will return 400 -
--key-name <KEY_NAME>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--max-count <MAX_COUNT>
-
--metadata <key=value>
— Metadata key and value pairs. The maximum size of the metadata key and value is 255 bytes each -
--min-count <MIN_COUNT>
-
--name <NAME>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--auto-networks
-
--networks <JSON>
— Parameter is an array, may be provided multiple times -
--none-networks
-
--os-dcf-disk-config <OS_DCF_DISK_CONFIG>
— Controls how the API partitions the disk when you create, rebuild, or resize servers. A server inherits theOS-DCF:diskConfig
value from the image from which it was created, and an image inherits theOS-DCF:diskConfig
value from the server from which it was created. To override the inherited setting, you can include this attribute in the request body of a server create, rebuild, or resize request. If theOS-DCF:diskConfig
value for an image isMANUAL
, you cannot create a server from that image and set itsOS-DCF:diskConfig
value toAUTO
. A valid value is:AUTO
. The API builds the server with a single partition the size of the target flavor disk. The API automatically adjusts the file system to fit the entire partition. -MANUAL
. The API builds the server by using whatever partition scheme and file system is in the source image. If the target flavor disk is larger, the API does not partition the remaining disk space.
Possible values:
auto
,manual
-
--return-reservation-id <RETURN_RESERVATION_ID>
— Indicates whether a config drive enables metadata injection. The config_drive setting provides information about a drive that the instance can mount at boot time. The instance reads files from the drive to get information that is normally available through the metadata service. This metadata is different from the user data. Not all cloud providers enable theconfig_drive
. Read more in the OpenStack End User GuidePossible values:
true
,false
-
--security-groups <SECURITY_GROUPS>
— One or more security groups. Specify the name of the security group in thename
attribute. If you omit this attribute, the API creates the server in thedefault
security group. Requested security groups are not applied to pre-existing ports.Parameter is an array, may be provided multiple times.
-
--tags <TAGS>
— A list of tags. Tags have the following restrictions:- Tag is a Unicode bytestring no longer than 60 characters. - Tag is a non-empty string. - ‘/’ is not allowed to be in a tag name - Comma is not allowed to be in a tag name in order to simplify requests that specify lists of tags - All other characters are allowed to be in a tag name - Each server can have up to 50 tags.
New in version 2.52
Parameter is an array, may be provided multiple times.
-
--user-data <USER_DATA>
— Configuration information or scripts to use upon launch. Must be Base64 encoded. Restricted to 65535 bytes.Note
The
null
value allowed in Nova legacy v2 API, but due to the strict input validation, it isn’t allowed in Nova v2.1 API.
osc compute server create252
Creates a server.
The progress of this operation depends on the location of the requested image, network I/O, host load, selected flavor, and other factors.
To check the progress of the request, make a GET /servers/{id}
request. This call returns a progress attribute, which is a percentage value from 0 to 100.
The Location
header returns the full URL to the newly created server and is available as a self
and bookmark
link in the server representation.
When you create a server, the response shows only the server ID, its links, and the admin password. You can get additional attributes through subsequent GET
requests on the server.
Include the block_device_mapping_v2
parameter in the create request body to boot a server from a volume.
Include the key_name
parameter in the create request body to add a keypair to the server when you create it. To create a keypair, make a create keypair request.
Preconditions
Asynchronous postconditions
Troubleshooting
Normal response codes: 202
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404), conflict(409)
Usage: osc compute server create252 [OPTIONS] --flavor-ref <FLAVOR_REF> --name <NAME> <--auto-networks|--networks <JSON>|--none-networks>
Options:
-
--build-near-host-ip <BUILD_NEAR_HOST_IP>
— Schedule the server on a host in the network specified with this parameter and a cidr (os:scheduler_hints.cidr
). It is available whenSimpleCIDRAffinityFilter
is available on cloud side -
--cidr <CIDR>
— Schedule the server on a host in the network specified with an IP address (os:scheduler_hints:build_near_host_ip
) and this parameter. Ifos:scheduler_hints:build_near_host_ip
is specified and this parameter is omitted,/24
is used. It is available whenSimpleCIDRAffinityFilter
is available on cloud side -
--different-cell <DIFFERENT_CELL>
— A list of cell routes or a cell route (string). Schedule the server in a cell that is not specified. It is available whenDifferentCellFilter
is available on cloud side that is cell v1 environment.Parameter is an array, may be provided multiple times.
-
--different-host <DIFFERENT_HOST>
— A list of server UUIDs or a server UUID. Schedule the server on a different host from a set of servers. It is available whenDifferentHostFilter
is available on cloud side.Parameter is an array, may be provided multiple times.
-
--group <GROUP>
— The server group UUID. Schedule the server according to a policy of the server group (anti-affinity
,affinity
,soft-anti-affinity
orsoft-affinity
). It is available whenServerGroupAffinityFilter
,ServerGroupAntiAffinityFilter
,ServerGroupSoftAntiAffinityWeigher
,ServerGroupSoftAffinityWeigher
are available on cloud side -
--query <JSON>
— Schedule the server by using a custom filter in JSON format. For example:It is available when
JsonFilter
is available on cloud side. -
--same-host <SAME_HOST>
— A list of server UUIDs or a server UUID. Schedule the server on the same host as another server in a set of servers. It is available whenSameHostFilter
is available on cloud side.Parameter is an array, may be provided multiple times.
-
--target-cell <TARGET_CELL>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--access-ipv4 <ACCESS_IPV4>
— IPv4 address that should be used to access this server -
--access-ipv6 <ACCESS_IPV6>
— IPv6 address that should be used to access this server -
--admin-pass <ADMIN_PASS>
— The administrative password of the server. If you omit this parameter, the operation generates a new password -
--availability-zone <AVAILABILITY_ZONE>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--block-device-mapping <JSON>
— Parameter is an array, may be provided multiple times -
--block-device-mapping-v2 <JSON>
— Enables fine grained control of the block device mapping for an instance. This is typically used for booting servers from volumes. An example format would look as follows:text > "block_device_mapping_v2": [{ > "boot_index": "0", > "uuid": "ac408821-c95a-448f-9292-73986c790911", > "source_type": "image", > "volume_size": "25", > "destination_type": "volume", > "delete_on_termination": true, > "tag": "disk1", > "disk_bus": "scsi"}] > >
In microversion 2.32,
tag
is an optional string attribute that can be used to assign a tag to the block device. This tag is then exposed to the guest in the metadata API and the config drive and is associated to hardware metadata for that block device, such as bus (ex: SCSI), bus address (ex: 1:0:2:0), and serial.A bug has caused the
tag
attribute to no longer be accepted starting with version 2.33. It has been restored in version 2.42.Parameter is an array, may be provided multiple times.
-
--config-drive <CONFIG_DRIVE>
— Indicates whether a config drive enables metadata injection. The config_drive setting provides information about a drive that the instance can mount at boot time. The instance reads files from the drive to get information that is normally available through the metadata service. This metadata is different from the user data. Not all cloud providers enable theconfig_drive
. Read more in the OpenStack End User GuidePossible values:
true
,false
-
--description <DESCRIPTION>
— A free form description of the server. Limited to 255 characters in length. Before microversion 2.19 this was set to the server name.New in version 2.19
-
--flavor-ref <FLAVOR_REF>
— The flavor reference, as an ID (including a UUID) or full URL, for the flavor for your server instance -
--image-ref <IMAGE_REF>
— The UUID of the image to use for your server instance. This is not required in case of boot from volume. In all other cases it is required and must be a valid UUID otherwise API will return 400 -
--key-name <KEY_NAME>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--max-count <MAX_COUNT>
-
--metadata <key=value>
— Metadata key and value pairs. The maximum size of the metadata key and value is 255 bytes each -
--min-count <MIN_COUNT>
-
--name <NAME>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--auto-networks
-
--networks <JSON>
— Parameter is an array, may be provided multiple times -
--none-networks
-
--os-dcf-disk-config <OS_DCF_DISK_CONFIG>
— Controls how the API partitions the disk when you create, rebuild, or resize servers. A server inherits theOS-DCF:diskConfig
value from the image from which it was created, and an image inherits theOS-DCF:diskConfig
value from the server from which it was created. To override the inherited setting, you can include this attribute in the request body of a server create, rebuild, or resize request. If theOS-DCF:diskConfig
value for an image isMANUAL
, you cannot create a server from that image and set itsOS-DCF:diskConfig
value toAUTO
. A valid value is:AUTO
. The API builds the server with a single partition the size of the target flavor disk. The API automatically adjusts the file system to fit the entire partition. -MANUAL
. The API builds the server by using whatever partition scheme and file system is in the source image. If the target flavor disk is larger, the API does not partition the remaining disk space.
Possible values:
auto
,manual
-
--personality <JSON>
— The file path and contents, text only, to inject into the server at launch. The maximum size of the file path data is 255 bytes. The maximum limit is the number of allowed bytes in the decoded, rather than encoded, data.Available until version 2.56
Parameter is an array, may be provided multiple times.
-
--return-reservation-id <RETURN_RESERVATION_ID>
— Indicates whether a config drive enables metadata injection. The config_drive setting provides information about a drive that the instance can mount at boot time. The instance reads files from the drive to get information that is normally available through the metadata service. This metadata is different from the user data. Not all cloud providers enable theconfig_drive
. Read more in the OpenStack End User GuidePossible values:
true
,false
-
--security-groups <SECURITY_GROUPS>
— One or more security groups. Specify the name of the security group in thename
attribute. If you omit this attribute, the API creates the server in thedefault
security group. Requested security groups are not applied to pre-existing ports.Parameter is an array, may be provided multiple times.
-
--tags <TAGS>
— A list of tags. Tags have the following restrictions:- Tag is a Unicode bytestring no longer than 60 characters. - Tag is a non-empty string. - ‘/’ is not allowed to be in a tag name - Comma is not allowed to be in a tag name in order to simplify requests that specify lists of tags - All other characters are allowed to be in a tag name - Each server can have up to 50 tags.
New in version 2.52
Parameter is an array, may be provided multiple times.
-
--user-data <USER_DATA>
— Configuration information or scripts to use upon launch. Must be Base64 encoded. Restricted to 65535 bytes.Note
The
null
value allowed in Nova legacy v2 API, but due to the strict input validation, it isn’t allowed in Nova v2.1 API.
osc compute server create242
Creates a server.
The progress of this operation depends on the location of the requested image, network I/O, host load, selected flavor, and other factors.
To check the progress of the request, make a GET /servers/{id}
request. This call returns a progress attribute, which is a percentage value from 0 to 100.
The Location
header returns the full URL to the newly created server and is available as a self
and bookmark
link in the server representation.
When you create a server, the response shows only the server ID, its links, and the admin password. You can get additional attributes through subsequent GET
requests on the server.
Include the block_device_mapping_v2
parameter in the create request body to boot a server from a volume.
Include the key_name
parameter in the create request body to add a keypair to the server when you create it. To create a keypair, make a create keypair request.
Preconditions
Asynchronous postconditions
Troubleshooting
Normal response codes: 202
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404), conflict(409)
Usage: osc compute server create242 [OPTIONS] --flavor-ref <FLAVOR_REF> --name <NAME> <--auto-networks|--networks <JSON>|--none-networks>
Options:
-
--build-near-host-ip <BUILD_NEAR_HOST_IP>
— Schedule the server on a host in the network specified with this parameter and a cidr (os:scheduler_hints.cidr
). It is available whenSimpleCIDRAffinityFilter
is available on cloud side -
--cidr <CIDR>
— Schedule the server on a host in the network specified with an IP address (os:scheduler_hints:build_near_host_ip
) and this parameter. Ifos:scheduler_hints:build_near_host_ip
is specified and this parameter is omitted,/24
is used. It is available whenSimpleCIDRAffinityFilter
is available on cloud side -
--different-cell <DIFFERENT_CELL>
— A list of cell routes or a cell route (string). Schedule the server in a cell that is not specified. It is available whenDifferentCellFilter
is available on cloud side that is cell v1 environment.Parameter is an array, may be provided multiple times.
-
--different-host <DIFFERENT_HOST>
— A list of server UUIDs or a server UUID. Schedule the server on a different host from a set of servers. It is available whenDifferentHostFilter
is available on cloud side.Parameter is an array, may be provided multiple times.
-
--group <GROUP>
— The server group UUID. Schedule the server according to a policy of the server group (anti-affinity
,affinity
,soft-anti-affinity
orsoft-affinity
). It is available whenServerGroupAffinityFilter
,ServerGroupAntiAffinityFilter
,ServerGroupSoftAntiAffinityWeigher
,ServerGroupSoftAffinityWeigher
are available on cloud side -
--query <JSON>
— Schedule the server by using a custom filter in JSON format. For example:It is available when
JsonFilter
is available on cloud side. -
--same-host <SAME_HOST>
— A list of server UUIDs or a server UUID. Schedule the server on the same host as another server in a set of servers. It is available whenSameHostFilter
is available on cloud side.Parameter is an array, may be provided multiple times.
-
--target-cell <TARGET_CELL>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--access-ipv4 <ACCESS_IPV4>
— IPv4 address that should be used to access this server -
--access-ipv6 <ACCESS_IPV6>
— IPv6 address that should be used to access this server -
--admin-pass <ADMIN_PASS>
— The administrative password of the server. If you omit this parameter, the operation generates a new password -
--availability-zone <AVAILABILITY_ZONE>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--block-device-mapping <JSON>
— Parameter is an array, may be provided multiple times -
--block-device-mapping-v2 <JSON>
— Enables fine grained control of the block device mapping for an instance. This is typically used for booting servers from volumes. An example format would look as follows:text > "block_device_mapping_v2": [{ > "boot_index": "0", > "uuid": "ac408821-c95a-448f-9292-73986c790911", > "source_type": "image", > "volume_size": "25", > "destination_type": "volume", > "delete_on_termination": true, > "tag": "disk1", > "disk_bus": "scsi"}] > >
In microversion 2.32,
tag
is an optional string attribute that can be used to assign a tag to the block device. This tag is then exposed to the guest in the metadata API and the config drive and is associated to hardware metadata for that block device, such as bus (ex: SCSI), bus address (ex: 1:0:2:0), and serial.A bug has caused the
tag
attribute to no longer be accepted starting with version 2.33. It has been restored in version 2.42.Parameter is an array, may be provided multiple times.
-
--config-drive <CONFIG_DRIVE>
— Indicates whether a config drive enables metadata injection. The config_drive setting provides information about a drive that the instance can mount at boot time. The instance reads files from the drive to get information that is normally available through the metadata service. This metadata is different from the user data. Not all cloud providers enable theconfig_drive
. Read more in the OpenStack End User GuidePossible values:
true
,false
-
--description <DESCRIPTION>
— A free form description of the server. Limited to 255 characters in length. Before microversion 2.19 this was set to the server name.New in version 2.19
-
--flavor-ref <FLAVOR_REF>
— The flavor reference, as an ID (including a UUID) or full URL, for the flavor for your server instance -
--image-ref <IMAGE_REF>
— The UUID of the image to use for your server instance. This is not required in case of boot from volume. In all other cases it is required and must be a valid UUID otherwise API will return 400 -
--key-name <KEY_NAME>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--max-count <MAX_COUNT>
-
--metadata <key=value>
— Metadata key and value pairs. The maximum size of the metadata key and value is 255 bytes each -
--min-count <MIN_COUNT>
-
--name <NAME>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--auto-networks
-
--networks <JSON>
— Parameter is an array, may be provided multiple times -
--none-networks
-
--os-dcf-disk-config <OS_DCF_DISK_CONFIG>
— Controls how the API partitions the disk when you create, rebuild, or resize servers. A server inherits theOS-DCF:diskConfig
value from the image from which it was created, and an image inherits theOS-DCF:diskConfig
value from the server from which it was created. To override the inherited setting, you can include this attribute in the request body of a server create, rebuild, or resize request. If theOS-DCF:diskConfig
value for an image isMANUAL
, you cannot create a server from that image and set itsOS-DCF:diskConfig
value toAUTO
. A valid value is:AUTO
. The API builds the server with a single partition the size of the target flavor disk. The API automatically adjusts the file system to fit the entire partition. -MANUAL
. The API builds the server by using whatever partition scheme and file system is in the source image. If the target flavor disk is larger, the API does not partition the remaining disk space.
Possible values:
auto
,manual
-
--personality <JSON>
— The file path and contents, text only, to inject into the server at launch. The maximum size of the file path data is 255 bytes. The maximum limit is the number of allowed bytes in the decoded, rather than encoded, data.Available until version 2.56
Parameter is an array, may be provided multiple times.
-
--return-reservation-id <RETURN_RESERVATION_ID>
— Indicates whether a config drive enables metadata injection. The config_drive setting provides information about a drive that the instance can mount at boot time. The instance reads files from the drive to get information that is normally available through the metadata service. This metadata is different from the user data. Not all cloud providers enable theconfig_drive
. Read more in the OpenStack End User GuidePossible values:
true
,false
-
--security-groups <SECURITY_GROUPS>
— One or more security groups. Specify the name of the security group in thename
attribute. If you omit this attribute, the API creates the server in thedefault
security group. Requested security groups are not applied to pre-existing ports.Parameter is an array, may be provided multiple times.
-
--user-data <USER_DATA>
— Configuration information or scripts to use upon launch. Must be Base64 encoded. Restricted to 65535 bytes.Note
The
null
value allowed in Nova legacy v2 API, but due to the strict input validation, it isn’t allowed in Nova v2.1 API.
osc compute server create237
Creates a server.
The progress of this operation depends on the location of the requested image, network I/O, host load, selected flavor, and other factors.
To check the progress of the request, make a GET /servers/{id}
request. This call returns a progress attribute, which is a percentage value from 0 to 100.
The Location
header returns the full URL to the newly created server and is available as a self
and bookmark
link in the server representation.
When you create a server, the response shows only the server ID, its links, and the admin password. You can get additional attributes through subsequent GET
requests on the server.
Include the block_device_mapping_v2
parameter in the create request body to boot a server from a volume.
Include the key_name
parameter in the create request body to add a keypair to the server when you create it. To create a keypair, make a create keypair request.
Preconditions
Asynchronous postconditions
Troubleshooting
Normal response codes: 202
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404), conflict(409)
Usage: osc compute server create237 [OPTIONS] --flavor-ref <FLAVOR_REF> --name <NAME> <--auto-networks|--networks <JSON>|--none-networks>
Options:
-
--build-near-host-ip <BUILD_NEAR_HOST_IP>
— Schedule the server on a host in the network specified with this parameter and a cidr (os:scheduler_hints.cidr
). It is available whenSimpleCIDRAffinityFilter
is available on cloud side -
--cidr <CIDR>
— Schedule the server on a host in the network specified with an IP address (os:scheduler_hints:build_near_host_ip
) and this parameter. Ifos:scheduler_hints:build_near_host_ip
is specified and this parameter is omitted,/24
is used. It is available whenSimpleCIDRAffinityFilter
is available on cloud side -
--different-cell <DIFFERENT_CELL>
— A list of cell routes or a cell route (string). Schedule the server in a cell that is not specified. It is available whenDifferentCellFilter
is available on cloud side that is cell v1 environment.Parameter is an array, may be provided multiple times.
-
--different-host <DIFFERENT_HOST>
— A list of server UUIDs or a server UUID. Schedule the server on a different host from a set of servers. It is available whenDifferentHostFilter
is available on cloud side.Parameter is an array, may be provided multiple times.
-
--group <GROUP>
— The server group UUID. Schedule the server according to a policy of the server group (anti-affinity
,affinity
,soft-anti-affinity
orsoft-affinity
). It is available whenServerGroupAffinityFilter
,ServerGroupAntiAffinityFilter
,ServerGroupSoftAntiAffinityWeigher
,ServerGroupSoftAffinityWeigher
are available on cloud side -
--query <JSON>
— Schedule the server by using a custom filter in JSON format. For example:It is available when
JsonFilter
is available on cloud side. -
--same-host <SAME_HOST>
— A list of server UUIDs or a server UUID. Schedule the server on the same host as another server in a set of servers. It is available whenSameHostFilter
is available on cloud side.Parameter is an array, may be provided multiple times.
-
--target-cell <TARGET_CELL>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--access-ipv4 <ACCESS_IPV4>
— IPv4 address that should be used to access this server -
--access-ipv6 <ACCESS_IPV6>
— IPv6 address that should be used to access this server -
--admin-pass <ADMIN_PASS>
— The administrative password of the server. If you omit this parameter, the operation generates a new password -
--availability-zone <AVAILABILITY_ZONE>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--block-device-mapping <JSON>
— Parameter is an array, may be provided multiple times -
--block-device-mapping-v2 <JSON>
— Enables fine grained control of the block device mapping for an instance. This is typically used for booting servers from volumes. An example format would look as follows:text > "block_device_mapping_v2": [{ > "boot_index": "0", > "uuid": "ac408821-c95a-448f-9292-73986c790911", > "source_type": "image", > "volume_size": "25", > "destination_type": "volume", > "delete_on_termination": true, > "tag": "disk1", > "disk_bus": "scsi"}] > >
In microversion 2.32,
tag
is an optional string attribute that can be used to assign a tag to the block device. This tag is then exposed to the guest in the metadata API and the config drive and is associated to hardware metadata for that block device, such as bus (ex: SCSI), bus address (ex: 1:0:2:0), and serial.A bug has caused the
tag
attribute to no longer be accepted starting with version 2.33. It has been restored in version 2.42.Parameter is an array, may be provided multiple times.
-
--config-drive <CONFIG_DRIVE>
— Indicates whether a config drive enables metadata injection. The config_drive setting provides information about a drive that the instance can mount at boot time. The instance reads files from the drive to get information that is normally available through the metadata service. This metadata is different from the user data. Not all cloud providers enable theconfig_drive
. Read more in the OpenStack End User GuidePossible values:
true
,false
-
--description <DESCRIPTION>
— A free form description of the server. Limited to 255 characters in length. Before microversion 2.19 this was set to the server name.New in version 2.19
-
--flavor-ref <FLAVOR_REF>
— The flavor reference, as an ID (including a UUID) or full URL, for the flavor for your server instance -
--image-ref <IMAGE_REF>
— The UUID of the image to use for your server instance. This is not required in case of boot from volume. In all other cases it is required and must be a valid UUID otherwise API will return 400 -
--key-name <KEY_NAME>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--max-count <MAX_COUNT>
-
--metadata <key=value>
— Metadata key and value pairs. The maximum size of the metadata key and value is 255 bytes each -
--min-count <MIN_COUNT>
-
--name <NAME>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--auto-networks
-
--networks <JSON>
— Parameter is an array, may be provided multiple times -
--none-networks
-
--os-dcf-disk-config <OS_DCF_DISK_CONFIG>
— Controls how the API partitions the disk when you create, rebuild, or resize servers. A server inherits theOS-DCF:diskConfig
value from the image from which it was created, and an image inherits theOS-DCF:diskConfig
value from the server from which it was created. To override the inherited setting, you can include this attribute in the request body of a server create, rebuild, or resize request. If theOS-DCF:diskConfig
value for an image isMANUAL
, you cannot create a server from that image and set itsOS-DCF:diskConfig
value toAUTO
. A valid value is:AUTO
. The API builds the server with a single partition the size of the target flavor disk. The API automatically adjusts the file system to fit the entire partition. -MANUAL
. The API builds the server by using whatever partition scheme and file system is in the source image. If the target flavor disk is larger, the API does not partition the remaining disk space.
Possible values:
auto
,manual
-
--personality <JSON>
— The file path and contents, text only, to inject into the server at launch. The maximum size of the file path data is 255 bytes. The maximum limit is the number of allowed bytes in the decoded, rather than encoded, data.Available until version 2.56
Parameter is an array, may be provided multiple times.
-
--return-reservation-id <RETURN_RESERVATION_ID>
— Indicates whether a config drive enables metadata injection. The config_drive setting provides information about a drive that the instance can mount at boot time. The instance reads files from the drive to get information that is normally available through the metadata service. This metadata is different from the user data. Not all cloud providers enable theconfig_drive
. Read more in the OpenStack End User GuidePossible values:
true
,false
-
--security-groups <SECURITY_GROUPS>
— One or more security groups. Specify the name of the security group in thename
attribute. If you omit this attribute, the API creates the server in thedefault
security group. Requested security groups are not applied to pre-existing ports.Parameter is an array, may be provided multiple times.
-
--user-data <USER_DATA>
— Configuration information or scripts to use upon launch. Must be Base64 encoded. Restricted to 65535 bytes.Note
The
null
value allowed in Nova legacy v2 API, but due to the strict input validation, it isn’t allowed in Nova v2.1 API.
osc compute server create233
Creates a server.
The progress of this operation depends on the location of the requested image, network I/O, host load, selected flavor, and other factors.
To check the progress of the request, make a GET /servers/{id}
request. This call returns a progress attribute, which is a percentage value from 0 to 100.
The Location
header returns the full URL to the newly created server and is available as a self
and bookmark
link in the server representation.
When you create a server, the response shows only the server ID, its links, and the admin password. You can get additional attributes through subsequent GET
requests on the server.
Include the block_device_mapping_v2
parameter in the create request body to boot a server from a volume.
Include the key_name
parameter in the create request body to add a keypair to the server when you create it. To create a keypair, make a create keypair request.
Preconditions
Asynchronous postconditions
Troubleshooting
Normal response codes: 202
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404), conflict(409)
Usage: osc compute server create233 [OPTIONS] --flavor-ref <FLAVOR_REF> --name <NAME>
Options:
-
--build-near-host-ip <BUILD_NEAR_HOST_IP>
— Schedule the server on a host in the network specified with this parameter and a cidr (os:scheduler_hints.cidr
). It is available whenSimpleCIDRAffinityFilter
is available on cloud side -
--cidr <CIDR>
— Schedule the server on a host in the network specified with an IP address (os:scheduler_hints:build_near_host_ip
) and this parameter. Ifos:scheduler_hints:build_near_host_ip
is specified and this parameter is omitted,/24
is used. It is available whenSimpleCIDRAffinityFilter
is available on cloud side -
--different-cell <DIFFERENT_CELL>
— A list of cell routes or a cell route (string). Schedule the server in a cell that is not specified. It is available whenDifferentCellFilter
is available on cloud side that is cell v1 environment.Parameter is an array, may be provided multiple times.
-
--different-host <DIFFERENT_HOST>
— A list of server UUIDs or a server UUID. Schedule the server on a different host from a set of servers. It is available whenDifferentHostFilter
is available on cloud side.Parameter is an array, may be provided multiple times.
-
--group <GROUP>
— The server group UUID. Schedule the server according to a policy of the server group (anti-affinity
,affinity
,soft-anti-affinity
orsoft-affinity
). It is available whenServerGroupAffinityFilter
,ServerGroupAntiAffinityFilter
,ServerGroupSoftAntiAffinityWeigher
,ServerGroupSoftAffinityWeigher
are available on cloud side -
--query <JSON>
— Schedule the server by using a custom filter in JSON format. For example:It is available when
JsonFilter
is available on cloud side. -
--same-host <SAME_HOST>
— A list of server UUIDs or a server UUID. Schedule the server on the same host as another server in a set of servers. It is available whenSameHostFilter
is available on cloud side.Parameter is an array, may be provided multiple times.
-
--target-cell <TARGET_CELL>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--access-ipv4 <ACCESS_IPV4>
— IPv4 address that should be used to access this server -
--access-ipv6 <ACCESS_IPV6>
— IPv6 address that should be used to access this server -
--admin-pass <ADMIN_PASS>
— The administrative password of the server. If you omit this parameter, the operation generates a new password -
--availability-zone <AVAILABILITY_ZONE>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--block-device-mapping <JSON>
— Parameter is an array, may be provided multiple times -
--block-device-mapping-v2 <JSON>
— Enables fine grained control of the block device mapping for an instance. This is typically used for booting servers from volumes. An example format would look as follows:text > "block_device_mapping_v2": [{ > "boot_index": "0", > "uuid": "ac408821-c95a-448f-9292-73986c790911", > "source_type": "image", > "volume_size": "25", > "destination_type": "volume", > "delete_on_termination": true, > "tag": "disk1", > "disk_bus": "scsi"}] > >
In microversion 2.32,
tag
is an optional string attribute that can be used to assign a tag to the block device. This tag is then exposed to the guest in the metadata API and the config drive and is associated to hardware metadata for that block device, such as bus (ex: SCSI), bus address (ex: 1:0:2:0), and serial.A bug has caused the
tag
attribute to no longer be accepted starting with version 2.33. It has been restored in version 2.42.Parameter is an array, may be provided multiple times.
-
--config-drive <CONFIG_DRIVE>
— Indicates whether a config drive enables metadata injection. The config_drive setting provides information about a drive that the instance can mount at boot time. The instance reads files from the drive to get information that is normally available through the metadata service. This metadata is different from the user data. Not all cloud providers enable theconfig_drive
. Read more in the OpenStack End User GuidePossible values:
true
,false
-
--description <DESCRIPTION>
— A free form description of the server. Limited to 255 characters in length. Before microversion 2.19 this was set to the server name.New in version 2.19
-
--flavor-ref <FLAVOR_REF>
— The flavor reference, as an ID (including a UUID) or full URL, for the flavor for your server instance -
--image-ref <IMAGE_REF>
— The UUID of the image to use for your server instance. This is not required in case of boot from volume. In all other cases it is required and must be a valid UUID otherwise API will return 400 -
--key-name <KEY_NAME>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--max-count <MAX_COUNT>
-
--metadata <key=value>
— Metadata key and value pairs. The maximum size of the metadata key and value is 255 bytes each -
--min-count <MIN_COUNT>
-
--name <NAME>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--networks <JSON>
— A list ofnetwork
object. Required parameter when there are multiple networks defined for the tenant. When you do not specify the networks parameter, the server attaches to the only network created for the current tenant. Optionally, you can create one or more NICs on the server. To provision the server instance with a NIC for a network, specify the UUID of the network in theuuid
attribute in anetworks
object. To provision the server instance with a NIC for an already existing port, specify the port-id in theport
attribute in anetworks
object.If multiple networks are defined, the order in which they appear in the guest operating system will not necessarily reflect the order in which they are given in the server boot request. Guests should therefore not depend on device order to deduce any information about their network devices. Instead, device role tags should be used: introduced in 2.32, broken in 2.37, and re-introduced and fixed in 2.42, the
tag
is an optional, string attribute that can be used to assign a tag to a virtual network interface. This tag is then exposed to the guest in the metadata API and the config drive and is associated to hardware metadata for that network interface, such as bus (ex: PCI), bus address (ex: 0000:00:02.0), and MAC address.A bug has caused the
tag
attribute to no longer be accepted starting with version 2.37. Therefore, network interfaces could only be tagged in versions 2.32 to 2.36 inclusively. Version 2.42 has restored thetag
attribute.Starting with microversion 2.37, this field is required and the special string values auto and none can be specified for networks. auto tells the Compute service to use a network that is available to the project, if one exists. If one does not exist, the Compute service will attempt to automatically allocate a network for the project (if possible). none tells the Compute service to not allocate a network for the instance. The auto and none values cannot be used with any other network values, including other network uuids, ports, fixed IPs or device tags. These are requested as strings for the networks value, not in a list. See the associated example.
Parameter is an array, may be provided multiple times.
-
--os-dcf-disk-config <OS_DCF_DISK_CONFIG>
— Controls how the API partitions the disk when you create, rebuild, or resize servers. A server inherits theOS-DCF:diskConfig
value from the image from which it was created, and an image inherits theOS-DCF:diskConfig
value from the server from which it was created. To override the inherited setting, you can include this attribute in the request body of a server create, rebuild, or resize request. If theOS-DCF:diskConfig
value for an image isMANUAL
, you cannot create a server from that image and set itsOS-DCF:diskConfig
value toAUTO
. A valid value is:AUTO
. The API builds the server with a single partition the size of the target flavor disk. The API automatically adjusts the file system to fit the entire partition. -MANUAL
. The API builds the server by using whatever partition scheme and file system is in the source image. If the target flavor disk is larger, the API does not partition the remaining disk space.
Possible values:
auto
,manual
-
--personality <JSON>
— The file path and contents, text only, to inject into the server at launch. The maximum size of the file path data is 255 bytes. The maximum limit is the number of allowed bytes in the decoded, rather than encoded, data.Available until version 2.56
Parameter is an array, may be provided multiple times.
-
--return-reservation-id <RETURN_RESERVATION_ID>
— Indicates whether a config drive enables metadata injection. The config_drive setting provides information about a drive that the instance can mount at boot time. The instance reads files from the drive to get information that is normally available through the metadata service. This metadata is different from the user data. Not all cloud providers enable theconfig_drive
. Read more in the OpenStack End User GuidePossible values:
true
,false
-
--security-groups <SECURITY_GROUPS>
— One or more security groups. Specify the name of the security group in thename
attribute. If you omit this attribute, the API creates the server in thedefault
security group. Requested security groups are not applied to pre-existing ports.Parameter is an array, may be provided multiple times.
-
--user-data <USER_DATA>
— Configuration information or scripts to use upon launch. Must be Base64 encoded. Restricted to 65535 bytes.Note
The
null
value allowed in Nova legacy v2 API, but due to the strict input validation, it isn’t allowed in Nova v2.1 API.
osc compute server create232
Creates a server.
The progress of this operation depends on the location of the requested image, network I/O, host load, selected flavor, and other factors.
To check the progress of the request, make a GET /servers/{id}
request. This call returns a progress attribute, which is a percentage value from 0 to 100.
The Location
header returns the full URL to the newly created server and is available as a self
and bookmark
link in the server representation.
When you create a server, the response shows only the server ID, its links, and the admin password. You can get additional attributes through subsequent GET
requests on the server.
Include the block_device_mapping_v2
parameter in the create request body to boot a server from a volume.
Include the key_name
parameter in the create request body to add a keypair to the server when you create it. To create a keypair, make a create keypair request.
Preconditions
Asynchronous postconditions
Troubleshooting
Normal response codes: 202
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404), conflict(409)
Usage: osc compute server create232 [OPTIONS] --flavor-ref <FLAVOR_REF> --name <NAME>
Options:
-
--build-near-host-ip <BUILD_NEAR_HOST_IP>
— Schedule the server on a host in the network specified with this parameter and a cidr (os:scheduler_hints.cidr
). It is available whenSimpleCIDRAffinityFilter
is available on cloud side -
--cidr <CIDR>
— Schedule the server on a host in the network specified with an IP address (os:scheduler_hints:build_near_host_ip
) and this parameter. Ifos:scheduler_hints:build_near_host_ip
is specified and this parameter is omitted,/24
is used. It is available whenSimpleCIDRAffinityFilter
is available on cloud side -
--different-cell <DIFFERENT_CELL>
— A list of cell routes or a cell route (string). Schedule the server in a cell that is not specified. It is available whenDifferentCellFilter
is available on cloud side that is cell v1 environment.Parameter is an array, may be provided multiple times.
-
--different-host <DIFFERENT_HOST>
— A list of server UUIDs or a server UUID. Schedule the server on a different host from a set of servers. It is available whenDifferentHostFilter
is available on cloud side.Parameter is an array, may be provided multiple times.
-
--group <GROUP>
— The server group UUID. Schedule the server according to a policy of the server group (anti-affinity
,affinity
,soft-anti-affinity
orsoft-affinity
). It is available whenServerGroupAffinityFilter
,ServerGroupAntiAffinityFilter
,ServerGroupSoftAntiAffinityWeigher
,ServerGroupSoftAffinityWeigher
are available on cloud side -
--query <JSON>
— Schedule the server by using a custom filter in JSON format. For example:It is available when
JsonFilter
is available on cloud side. -
--same-host <SAME_HOST>
— A list of server UUIDs or a server UUID. Schedule the server on the same host as another server in a set of servers. It is available whenSameHostFilter
is available on cloud side.Parameter is an array, may be provided multiple times.
-
--target-cell <TARGET_CELL>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--access-ipv4 <ACCESS_IPV4>
— IPv4 address that should be used to access this server -
--access-ipv6 <ACCESS_IPV6>
— IPv6 address that should be used to access this server -
--admin-pass <ADMIN_PASS>
— The administrative password of the server. If you omit this parameter, the operation generates a new password -
--availability-zone <AVAILABILITY_ZONE>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--block-device-mapping <JSON>
— Parameter is an array, may be provided multiple times -
--block-device-mapping-v2 <JSON>
— Enables fine grained control of the block device mapping for an instance. This is typically used for booting servers from volumes. An example format would look as follows:text > "block_device_mapping_v2": [{ > "boot_index": "0", > "uuid": "ac408821-c95a-448f-9292-73986c790911", > "source_type": "image", > "volume_size": "25", > "destination_type": "volume", > "delete_on_termination": true, > "tag": "disk1", > "disk_bus": "scsi"}] > >
In microversion 2.32,
tag
is an optional string attribute that can be used to assign a tag to the block device. This tag is then exposed to the guest in the metadata API and the config drive and is associated to hardware metadata for that block device, such as bus (ex: SCSI), bus address (ex: 1:0:2:0), and serial.A bug has caused the
tag
attribute to no longer be accepted starting with version 2.33. It has been restored in version 2.42.Parameter is an array, may be provided multiple times.
-
--config-drive <CONFIG_DRIVE>
— Indicates whether a config drive enables metadata injection. The config_drive setting provides information about a drive that the instance can mount at boot time. The instance reads files from the drive to get information that is normally available through the metadata service. This metadata is different from the user data. Not all cloud providers enable theconfig_drive
. Read more in the OpenStack End User GuidePossible values:
true
,false
-
--description <DESCRIPTION>
— A free form description of the server. Limited to 255 characters in length. Before microversion 2.19 this was set to the server name.New in version 2.19
-
--flavor-ref <FLAVOR_REF>
— The flavor reference, as an ID (including a UUID) or full URL, for the flavor for your server instance -
--image-ref <IMAGE_REF>
— The UUID of the image to use for your server instance. This is not required in case of boot from volume. In all other cases it is required and must be a valid UUID otherwise API will return 400 -
--key-name <KEY_NAME>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--max-count <MAX_COUNT>
-
--metadata <key=value>
— Metadata key and value pairs. The maximum size of the metadata key and value is 255 bytes each -
--min-count <MIN_COUNT>
-
--name <NAME>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--networks <JSON>
— A list ofnetwork
object. Required parameter when there are multiple networks defined for the tenant. When you do not specify the networks parameter, the server attaches to the only network created for the current tenant. Optionally, you can create one or more NICs on the server. To provision the server instance with a NIC for a network, specify the UUID of the network in theuuid
attribute in anetworks
object. To provision the server instance with a NIC for an already existing port, specify the port-id in theport
attribute in anetworks
object.If multiple networks are defined, the order in which they appear in the guest operating system will not necessarily reflect the order in which they are given in the server boot request. Guests should therefore not depend on device order to deduce any information about their network devices. Instead, device role tags should be used: introduced in 2.32, broken in 2.37, and re-introduced and fixed in 2.42, the
tag
is an optional, string attribute that can be used to assign a tag to a virtual network interface. This tag is then exposed to the guest in the metadata API and the config drive and is associated to hardware metadata for that network interface, such as bus (ex: PCI), bus address (ex: 0000:00:02.0), and MAC address.A bug has caused the
tag
attribute to no longer be accepted starting with version 2.37. Therefore, network interfaces could only be tagged in versions 2.32 to 2.36 inclusively. Version 2.42 has restored thetag
attribute.Starting with microversion 2.37, this field is required and the special string values auto and none can be specified for networks. auto tells the Compute service to use a network that is available to the project, if one exists. If one does not exist, the Compute service will attempt to automatically allocate a network for the project (if possible). none tells the Compute service to not allocate a network for the instance. The auto and none values cannot be used with any other network values, including other network uuids, ports, fixed IPs or device tags. These are requested as strings for the networks value, not in a list. See the associated example.
Parameter is an array, may be provided multiple times.
-
--os-dcf-disk-config <OS_DCF_DISK_CONFIG>
— Controls how the API partitions the disk when you create, rebuild, or resize servers. A server inherits theOS-DCF:diskConfig
value from the image from which it was created, and an image inherits theOS-DCF:diskConfig
value from the server from which it was created. To override the inherited setting, you can include this attribute in the request body of a server create, rebuild, or resize request. If theOS-DCF:diskConfig
value for an image isMANUAL
, you cannot create a server from that image and set itsOS-DCF:diskConfig
value toAUTO
. A valid value is:AUTO
. The API builds the server with a single partition the size of the target flavor disk. The API automatically adjusts the file system to fit the entire partition. -MANUAL
. The API builds the server by using whatever partition scheme and file system is in the source image. If the target flavor disk is larger, the API does not partition the remaining disk space.
Possible values:
auto
,manual
-
--personality <JSON>
— The file path and contents, text only, to inject into the server at launch. The maximum size of the file path data is 255 bytes. The maximum limit is the number of allowed bytes in the decoded, rather than encoded, data.Available until version 2.56
Parameter is an array, may be provided multiple times.
-
--return-reservation-id <RETURN_RESERVATION_ID>
— Indicates whether a config drive enables metadata injection. The config_drive setting provides information about a drive that the instance can mount at boot time. The instance reads files from the drive to get information that is normally available through the metadata service. This metadata is different from the user data. Not all cloud providers enable theconfig_drive
. Read more in the OpenStack End User GuidePossible values:
true
,false
-
--security-groups <SECURITY_GROUPS>
— One or more security groups. Specify the name of the security group in thename
attribute. If you omit this attribute, the API creates the server in thedefault
security group. Requested security groups are not applied to pre-existing ports.Parameter is an array, may be provided multiple times.
-
--user-data <USER_DATA>
— Configuration information or scripts to use upon launch. Must be Base64 encoded. Restricted to 65535 bytes.Note
The
null
value allowed in Nova legacy v2 API, but due to the strict input validation, it isn’t allowed in Nova v2.1 API.
osc compute server create219
Creates a server.
The progress of this operation depends on the location of the requested image, network I/O, host load, selected flavor, and other factors.
To check the progress of the request, make a GET /servers/{id}
request. This call returns a progress attribute, which is a percentage value from 0 to 100.
The Location
header returns the full URL to the newly created server and is available as a self
and bookmark
link in the server representation.
When you create a server, the response shows only the server ID, its links, and the admin password. You can get additional attributes through subsequent GET
requests on the server.
Include the block_device_mapping_v2
parameter in the create request body to boot a server from a volume.
Include the key_name
parameter in the create request body to add a keypair to the server when you create it. To create a keypair, make a create keypair request.
Preconditions
Asynchronous postconditions
Troubleshooting
Normal response codes: 202
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404), conflict(409)
Usage: osc compute server create219 [OPTIONS] --flavor-ref <FLAVOR_REF> --name <NAME>
Options:
-
--build-near-host-ip <BUILD_NEAR_HOST_IP>
— Schedule the server on a host in the network specified with this parameter and a cidr (os:scheduler_hints.cidr
). It is available whenSimpleCIDRAffinityFilter
is available on cloud side -
--cidr <CIDR>
— Schedule the server on a host in the network specified with an IP address (os:scheduler_hints:build_near_host_ip
) and this parameter. Ifos:scheduler_hints:build_near_host_ip
is specified and this parameter is omitted,/24
is used. It is available whenSimpleCIDRAffinityFilter
is available on cloud side -
--different-cell <DIFFERENT_CELL>
— A list of cell routes or a cell route (string). Schedule the server in a cell that is not specified. It is available whenDifferentCellFilter
is available on cloud side that is cell v1 environment.Parameter is an array, may be provided multiple times.
-
--different-host <DIFFERENT_HOST>
— A list of server UUIDs or a server UUID. Schedule the server on a different host from a set of servers. It is available whenDifferentHostFilter
is available on cloud side.Parameter is an array, may be provided multiple times.
-
--group <GROUP>
— The server group UUID. Schedule the server according to a policy of the server group (anti-affinity
,affinity
,soft-anti-affinity
orsoft-affinity
). It is available whenServerGroupAffinityFilter
,ServerGroupAntiAffinityFilter
,ServerGroupSoftAntiAffinityWeigher
,ServerGroupSoftAffinityWeigher
are available on cloud side -
--query <JSON>
— Schedule the server by using a custom filter in JSON format. For example:It is available when
JsonFilter
is available on cloud side. -
--same-host <SAME_HOST>
— A list of server UUIDs or a server UUID. Schedule the server on the same host as another server in a set of servers. It is available whenSameHostFilter
is available on cloud side.Parameter is an array, may be provided multiple times.
-
--target-cell <TARGET_CELL>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--access-ipv4 <ACCESS_IPV4>
— IPv4 address that should be used to access this server -
--access-ipv6 <ACCESS_IPV6>
— IPv6 address that should be used to access this server -
--admin-pass <ADMIN_PASS>
— The administrative password of the server. If you omit this parameter, the operation generates a new password -
--availability-zone <AVAILABILITY_ZONE>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--block-device-mapping <JSON>
— Parameter is an array, may be provided multiple times -
--block-device-mapping-v2 <JSON>
— Enables fine grained control of the block device mapping for an instance. This is typically used for booting servers from volumes. An example format would look as follows:text > "block_device_mapping_v2": [{ > "boot_index": "0", > "uuid": "ac408821-c95a-448f-9292-73986c790911", > "source_type": "image", > "volume_size": "25", > "destination_type": "volume", > "delete_on_termination": true, > "tag": "disk1", > "disk_bus": "scsi"}] > >
In microversion 2.32,
tag
is an optional string attribute that can be used to assign a tag to the block device. This tag is then exposed to the guest in the metadata API and the config drive and is associated to hardware metadata for that block device, such as bus (ex: SCSI), bus address (ex: 1:0:2:0), and serial.A bug has caused the
tag
attribute to no longer be accepted starting with version 2.33. It has been restored in version 2.42.Parameter is an array, may be provided multiple times.
-
--config-drive <CONFIG_DRIVE>
— Indicates whether a config drive enables metadata injection. The config_drive setting provides information about a drive that the instance can mount at boot time. The instance reads files from the drive to get information that is normally available through the metadata service. This metadata is different from the user data. Not all cloud providers enable theconfig_drive
. Read more in the OpenStack End User GuidePossible values:
true
,false
-
--description <DESCRIPTION>
— A free form description of the server. Limited to 255 characters in length. Before microversion 2.19 this was set to the server name.New in version 2.19
-
--flavor-ref <FLAVOR_REF>
— The flavor reference, as an ID (including a UUID) or full URL, for the flavor for your server instance -
--image-ref <IMAGE_REF>
— The UUID of the image to use for your server instance. This is not required in case of boot from volume. In all other cases it is required and must be a valid UUID otherwise API will return 400 -
--key-name <KEY_NAME>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--max-count <MAX_COUNT>
-
--metadata <key=value>
— Metadata key and value pairs. The maximum size of the metadata key and value is 255 bytes each -
--min-count <MIN_COUNT>
-
--name <NAME>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--networks <JSON>
— A list ofnetwork
object. Required parameter when there are multiple networks defined for the tenant. When you do not specify the networks parameter, the server attaches to the only network created for the current tenant. Optionally, you can create one or more NICs on the server. To provision the server instance with a NIC for a network, specify the UUID of the network in theuuid
attribute in anetworks
object. To provision the server instance with a NIC for an already existing port, specify the port-id in theport
attribute in anetworks
object.If multiple networks are defined, the order in which they appear in the guest operating system will not necessarily reflect the order in which they are given in the server boot request. Guests should therefore not depend on device order to deduce any information about their network devices. Instead, device role tags should be used: introduced in 2.32, broken in 2.37, and re-introduced and fixed in 2.42, the
tag
is an optional, string attribute that can be used to assign a tag to a virtual network interface. This tag is then exposed to the guest in the metadata API and the config drive and is associated to hardware metadata for that network interface, such as bus (ex: PCI), bus address (ex: 0000:00:02.0), and MAC address.A bug has caused the
tag
attribute to no longer be accepted starting with version 2.37. Therefore, network interfaces could only be tagged in versions 2.32 to 2.36 inclusively. Version 2.42 has restored thetag
attribute.Starting with microversion 2.37, this field is required and the special string values auto and none can be specified for networks. auto tells the Compute service to use a network that is available to the project, if one exists. If one does not exist, the Compute service will attempt to automatically allocate a network for the project (if possible). none tells the Compute service to not allocate a network for the instance. The auto and none values cannot be used with any other network values, including other network uuids, ports, fixed IPs or device tags. These are requested as strings for the networks value, not in a list. See the associated example.
Parameter is an array, may be provided multiple times.
-
--os-dcf-disk-config <OS_DCF_DISK_CONFIG>
— Controls how the API partitions the disk when you create, rebuild, or resize servers. A server inherits theOS-DCF:diskConfig
value from the image from which it was created, and an image inherits theOS-DCF:diskConfig
value from the server from which it was created. To override the inherited setting, you can include this attribute in the request body of a server create, rebuild, or resize request. If theOS-DCF:diskConfig
value for an image isMANUAL
, you cannot create a server from that image and set itsOS-DCF:diskConfig
value toAUTO
. A valid value is:AUTO
. The API builds the server with a single partition the size of the target flavor disk. The API automatically adjusts the file system to fit the entire partition. -MANUAL
. The API builds the server by using whatever partition scheme and file system is in the source image. If the target flavor disk is larger, the API does not partition the remaining disk space.
Possible values:
auto
,manual
-
--personality <JSON>
— The file path and contents, text only, to inject into the server at launch. The maximum size of the file path data is 255 bytes. The maximum limit is the number of allowed bytes in the decoded, rather than encoded, data.Available until version 2.56
Parameter is an array, may be provided multiple times.
-
--return-reservation-id <RETURN_RESERVATION_ID>
— Indicates whether a config drive enables metadata injection. The config_drive setting provides information about a drive that the instance can mount at boot time. The instance reads files from the drive to get information that is normally available through the metadata service. This metadata is different from the user data. Not all cloud providers enable theconfig_drive
. Read more in the OpenStack End User GuidePossible values:
true
,false
-
--security-groups <SECURITY_GROUPS>
— One or more security groups. Specify the name of the security group in thename
attribute. If you omit this attribute, the API creates the server in thedefault
security group. Requested security groups are not applied to pre-existing ports.Parameter is an array, may be provided multiple times.
-
--user-data <USER_DATA>
— Configuration information or scripts to use upon launch. Must be Base64 encoded. Restricted to 65535 bytes.Note
The
null
value allowed in Nova legacy v2 API, but due to the strict input validation, it isn’t allowed in Nova v2.1 API.
osc compute server create21
Creates a server.
The progress of this operation depends on the location of the requested image, network I/O, host load, selected flavor, and other factors.
To check the progress of the request, make a GET /servers/{id}
request. This call returns a progress attribute, which is a percentage value from 0 to 100.
The Location
header returns the full URL to the newly created server and is available as a self
and bookmark
link in the server representation.
When you create a server, the response shows only the server ID, its links, and the admin password. You can get additional attributes through subsequent GET
requests on the server.
Include the block_device_mapping_v2
parameter in the create request body to boot a server from a volume.
Include the key_name
parameter in the create request body to add a keypair to the server when you create it. To create a keypair, make a create keypair request.
Preconditions
Asynchronous postconditions
Troubleshooting
Normal response codes: 202
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404), conflict(409)
Usage: osc compute server create21 [OPTIONS] --flavor-ref <FLAVOR_REF> --name <NAME>
Options:
-
--build-near-host-ip <BUILD_NEAR_HOST_IP>
— Schedule the server on a host in the network specified with this parameter and a cidr (os:scheduler_hints.cidr
). It is available whenSimpleCIDRAffinityFilter
is available on cloud side -
--cidr <CIDR>
— Schedule the server on a host in the network specified with an IP address (os:scheduler_hints:build_near_host_ip
) and this parameter. Ifos:scheduler_hints:build_near_host_ip
is specified and this parameter is omitted,/24
is used. It is available whenSimpleCIDRAffinityFilter
is available on cloud side -
--different-cell <DIFFERENT_CELL>
— A list of cell routes or a cell route (string). Schedule the server in a cell that is not specified. It is available whenDifferentCellFilter
is available on cloud side that is cell v1 environment.Parameter is an array, may be provided multiple times.
-
--different-host <DIFFERENT_HOST>
— A list of server UUIDs or a server UUID. Schedule the server on a different host from a set of servers. It is available whenDifferentHostFilter
is available on cloud side.Parameter is an array, may be provided multiple times.
-
--group <GROUP>
— The server group UUID. Schedule the server according to a policy of the server group (anti-affinity
,affinity
,soft-anti-affinity
orsoft-affinity
). It is available whenServerGroupAffinityFilter
,ServerGroupAntiAffinityFilter
,ServerGroupSoftAntiAffinityWeigher
,ServerGroupSoftAffinityWeigher
are available on cloud side -
--query <JSON>
— Schedule the server by using a custom filter in JSON format. For example:It is available when
JsonFilter
is available on cloud side. -
--same-host <SAME_HOST>
— A list of server UUIDs or a server UUID. Schedule the server on the same host as another server in a set of servers. It is available whenSameHostFilter
is available on cloud side.Parameter is an array, may be provided multiple times.
-
--target-cell <TARGET_CELL>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--access-ipv4 <ACCESS_IPV4>
— IPv4 address that should be used to access this server -
--access-ipv6 <ACCESS_IPV6>
— IPv6 address that should be used to access this server -
--admin-pass <ADMIN_PASS>
— The administrative password of the server. If you omit this parameter, the operation generates a new password -
--availability-zone <AVAILABILITY_ZONE>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--block-device-mapping <JSON>
— Parameter is an array, may be provided multiple times -
--block-device-mapping-v2 <JSON>
— Enables fine grained control of the block device mapping for an instance. This is typically used for booting servers from volumes. An example format would look as follows:text > "block_device_mapping_v2": [{ > "boot_index": "0", > "uuid": "ac408821-c95a-448f-9292-73986c790911", > "source_type": "image", > "volume_size": "25", > "destination_type": "volume", > "delete_on_termination": true, > "tag": "disk1", > "disk_bus": "scsi"}] > >
In microversion 2.32,
tag
is an optional string attribute that can be used to assign a tag to the block device. This tag is then exposed to the guest in the metadata API and the config drive and is associated to hardware metadata for that block device, such as bus (ex: SCSI), bus address (ex: 1:0:2:0), and serial.A bug has caused the
tag
attribute to no longer be accepted starting with version 2.33. It has been restored in version 2.42.Parameter is an array, may be provided multiple times.
-
--config-drive <CONFIG_DRIVE>
— Indicates whether a config drive enables metadata injection. The config_drive setting provides information about a drive that the instance can mount at boot time. The instance reads files from the drive to get information that is normally available through the metadata service. This metadata is different from the user data. Not all cloud providers enable theconfig_drive
. Read more in the OpenStack End User GuidePossible values:
true
,false
-
--flavor-ref <FLAVOR_REF>
— The flavor reference, as an ID (including a UUID) or full URL, for the flavor for your server instance -
--image-ref <IMAGE_REF>
— The UUID of the image to use for your server instance. This is not required in case of boot from volume. In all other cases it is required and must be a valid UUID otherwise API will return 400 -
--key-name <KEY_NAME>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--max-count <MAX_COUNT>
-
--metadata <key=value>
— Metadata key and value pairs. The maximum size of the metadata key and value is 255 bytes each -
--min-count <MIN_COUNT>
-
--name <NAME>
— A target cell name. Schedule the server in a host in the cell specified. It is available whenTargetCellFilter
is available on cloud side that is cell v1 environment -
--networks <JSON>
— A list ofnetwork
object. Required parameter when there are multiple networks defined for the tenant. When you do not specify the networks parameter, the server attaches to the only network created for the current tenant. Optionally, you can create one or more NICs on the server. To provision the server instance with a NIC for a network, specify the UUID of the network in theuuid
attribute in anetworks
object. To provision the server instance with a NIC for an already existing port, specify the port-id in theport
attribute in anetworks
object.If multiple networks are defined, the order in which they appear in the guest operating system will not necessarily reflect the order in which they are given in the server boot request. Guests should therefore not depend on device order to deduce any information about their network devices. Instead, device role tags should be used: introduced in 2.32, broken in 2.37, and re-introduced and fixed in 2.42, the
tag
is an optional, string attribute that can be used to assign a tag to a virtual network interface. This tag is then exposed to the guest in the metadata API and the config drive and is associated to hardware metadata for that network interface, such as bus (ex: PCI), bus address (ex: 0000:00:02.0), and MAC address.A bug has caused the
tag
attribute to no longer be accepted starting with version 2.37. Therefore, network interfaces could only be tagged in versions 2.32 to 2.36 inclusively. Version 2.42 has restored thetag
attribute.Starting with microversion 2.37, this field is required and the special string values auto and none can be specified for networks. auto tells the Compute service to use a network that is available to the project, if one exists. If one does not exist, the Compute service will attempt to automatically allocate a network for the project (if possible). none tells the Compute service to not allocate a network for the instance. The auto and none values cannot be used with any other network values, including other network uuids, ports, fixed IPs or device tags. These are requested as strings for the networks value, not in a list. See the associated example.
Parameter is an array, may be provided multiple times.
-
--os-dcf-disk-config <OS_DCF_DISK_CONFIG>
— Controls how the API partitions the disk when you create, rebuild, or resize servers. A server inherits theOS-DCF:diskConfig
value from the image from which it was created, and an image inherits theOS-DCF:diskConfig
value from the server from which it was created. To override the inherited setting, you can include this attribute in the request body of a server create, rebuild, or resize request. If theOS-DCF:diskConfig
value for an image isMANUAL
, you cannot create a server from that image and set itsOS-DCF:diskConfig
value toAUTO
. A valid value is:AUTO
. The API builds the server with a single partition the size of the target flavor disk. The API automatically adjusts the file system to fit the entire partition. -MANUAL
. The API builds the server by using whatever partition scheme and file system is in the source image. If the target flavor disk is larger, the API does not partition the remaining disk space.
Possible values:
auto
,manual
-
--personality <JSON>
— The file path and contents, text only, to inject into the server at launch. The maximum size of the file path data is 255 bytes. The maximum limit is the number of allowed bytes in the decoded, rather than encoded, data.Available until version 2.56
Parameter is an array, may be provided multiple times.
-
--return-reservation-id <RETURN_RESERVATION_ID>
— Indicates whether a config drive enables metadata injection. The config_drive setting provides information about a drive that the instance can mount at boot time. The instance reads files from the drive to get information that is normally available through the metadata service. This metadata is different from the user data. Not all cloud providers enable theconfig_drive
. Read more in the OpenStack End User GuidePossible values:
true
,false
-
--security-groups <SECURITY_GROUPS>
— One or more security groups. Specify the name of the security group in thename
attribute. If you omit this attribute, the API creates the server in thedefault
security group. Requested security groups are not applied to pre-existing ports.Parameter is an array, may be provided multiple times.
-
--user-data <USER_DATA>
— Configuration information or scripts to use upon launch. Must be Base64 encoded. Restricted to 65535 bytes.Note
The
null
value allowed in Nova legacy v2 API, but due to the strict input validation, it isn’t allowed in Nova v2.1 API.
osc compute server create-backup21
Create Server Back Up (createBackup Action) (microversion = 2.1)
Usage: osc compute server create-backup21 [OPTIONS] --backup-type <BACKUP_TYPE> --name <NAME> --rotation <ROTATION> <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
--backup-type <BACKUP_TYPE>
— The type of the backup, for example,daily
--metadata <key=value>
— Metadata key and value pairs. The maximum size of the metadata key and value is 255 bytes each--name <NAME>
— The name of the image to be backed up--rotation <ROTATION>
— The rotation of the back up image, the oldest image will be removed when image count exceed the rotation count
osc compute server create-image21
Create Image (createImage Action) (microversion = 2.1)
Usage: osc compute server create-image21 [OPTIONS] --name <NAME> <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
--metadata <key=value>
— Metadata key and value pairs for the image. The maximum size for each metadata key and value pair is 255 bytes--name <NAME>
— The display name of an Image
osc compute server delete
Deletes a server.
By default, the instance is going to be (hard) deleted immediately from the system, but you can set reclaim_instance_interval
> 0 to make the API soft delete the instance, so that the instance won’t be deleted until the reclaim_instance_interval
has expired since the instance was soft deleted. The instance marked as SOFT_DELETED
can be recovered via restore
action before it’s really deleted from the system.
Preconditions
Asynchronous postconditions
Troubleshooting
Normal response codes: 204
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404), conflict(409)
Usage: osc compute server delete <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id} API
osc compute server diagnostic
Shows basic usage data for a server.
Policy defaults enable only users with the administrative role. Cloud providers can change these permissions through the policy.json
file.
Normal response codes: 200
Error response codes: unauthorized(401), forbidden(403), notfound(404), conflict(409), notimplemented(501)
Usage: osc compute server diagnostic <SERVER_ID>
Arguments:
<SERVER_ID>
— server_id parameter for /v2.1/servers/{server_id}/diagnostics API
osc compute server evacuate214
Evacuate Server (evacuate Action) (microversion = 2.14)
Usage: osc compute server evacuate214 [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
-
--admin-pass <ADMIN_PASS>
— An administrative password to access the evacuated server. If you omit this parameter, the operation generates a new password. Up to API version 2.13, ifonSharedStorage
is set toTrue
and this parameter is specified, an error is raised -
--host <HOST>
— The name or ID of the host to which the server is evacuated. If you omit this parameter, the scheduler chooses a host.Warning
Prior to microversion 2.29, specifying a host will bypass validation by the scheduler, which could result in failures to actually evacuate the instance to the specified host, or over-subscription of the host. It is recommended to either not specify a host so that the scheduler will pick one, or specify a host with microversion >= 2.29 and without
force=True
set.
osc compute server evacuate229
Evacuate Server (evacuate Action) (microversion = 2.29)
Usage: osc compute server evacuate229 [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
-
--admin-pass <ADMIN_PASS>
— An administrative password to access the evacuated server. If you omit this parameter, the operation generates a new password. Up to API version 2.13, ifonSharedStorage
is set toTrue
and this parameter is specified, an error is raised -
--force <FORCE>
— Force an evacuation by not verifying the provided destination host by the scheduler.Warning
This could result in failures to actually evacuate the instance to the specified host. It is recommended to either not specify a host so that the scheduler will pick one, or specify a host without
force=True
set.Furthermore, this should not be specified when evacuating instances managed by a clustered hypervisor driver like ironic since you cannot specify a node, so the compute service will pick a node randomly which may not be able to accommodate the instance.
New in version 2.29
Available until version 2.67
Possible values:
true
,false
-
--host <HOST>
— The name or ID of the host to which the server is evacuated. If you omit this parameter, the scheduler chooses a host.Warning
Prior to microversion 2.29, specifying a host will bypass validation by the scheduler, which could result in failures to actually evacuate the instance to the specified host, or over-subscription of the host. It is recommended to either not specify a host so that the scheduler will pick one, or specify a host with microversion >= 2.29 and without
force=True
set.
osc compute server evacuate268
Evacuate Server (evacuate Action) (microversion = 2.68)
Usage: osc compute server evacuate268 [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
-
--admin-pass <ADMIN_PASS>
— An administrative password to access the evacuated server. If you omit this parameter, the operation generates a new password. Up to API version 2.13, ifonSharedStorage
is set toTrue
and this parameter is specified, an error is raised -
--host <HOST>
— The name or ID of the host to which the server is evacuated. If you omit this parameter, the scheduler chooses a host.Warning
Prior to microversion 2.29, specifying a host will bypass validation by the scheduler, which could result in failures to actually evacuate the instance to the specified host, or over-subscription of the host. It is recommended to either not specify a host so that the scheduler will pick one, or specify a host with microversion >= 2.29 and without
force=True
set.
osc compute server evacuate295
Evacuate Server (evacuate Action) (microversion = 2.95)
Usage: osc compute server evacuate295 [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
-
--admin-pass <ADMIN_PASS>
— An administrative password to access the evacuated server. If you omit this parameter, the operation generates a new password. Up to API version 2.13, ifonSharedStorage
is set toTrue
and this parameter is specified, an error is raised -
--host <HOST>
— The name or ID of the host to which the server is evacuated. If you omit this parameter, the scheduler chooses a host.Warning
Prior to microversion 2.29, specifying a host will bypass validation by the scheduler, which could result in failures to actually evacuate the instance to the specified host, or over-subscription of the host. It is recommended to either not specify a host so that the scheduler will pick one, or specify a host with microversion >= 2.29 and without
force=True
set.
osc compute server force-delete
Force-deletes a server before deferred cleanup.
Specify the forceDelete
action in the request body.
Policy defaults enable only users with the administrative role or the owner of the server to perform this operation. Cloud providers can change these permissions through the policy.json
file.
Normal response codes: 202
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404), conflict(409)
Usage: osc compute server force-delete --force-delete <JSON> <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
--force-delete <JSON>
osc compute server get-console-output
Shows console output for a server.
This API returns the text of the console since boot. The content returned may be large. Limit the lines of console text, beginning at the tail of the content, by setting the optional length
parameter in the request body.
The server to get console log from should set export LC_ALL=en_US.UTF-8
in order to avoid incorrect unicode error.
Normal response codes: 200
Error response codes: unauthorized(401), forbidden(403), notFound(404), conflict(409), methodNotImplemented(501)
Usage: osc compute server get-console-output [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
-
--length <LENGTH>
— The number of lines to fetch from the end of console log. All lines will be returned if this is not specified.Note
This parameter can be specified as not only ‘integer’ but also ‘string’.
osc compute server instance-action
Servers actions
List actions and action details for a server.
Usage: osc compute server instance-action <COMMAND>
Available subcommands:
osc compute server instance-action list
— List Actions For Serverosc compute server instance-action show
— Show Server Action Details
osc compute server instance-action list
Lists actions for a server.
Action information of deleted instances can be returned for requests starting with microversion 2.21.
Policy defaults enable only users with the administrative role or the owner of the server to perform this operation. Cloud providers can change these permissions through the policy.json
file.
Normal response codes: 200
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute server instance-action list [OPTIONS] <SERVER_ID>
Arguments:
<SERVER_ID>
— server_id parameter for /v2.1/servers/{server_id}/os-instance-actions/{id} API
Options:
-
--changes-before <CHANGES_BEFORE>
-
--changes-since <CHANGES_SINCE>
-
--limit <LIMIT>
-
--marker <MARKER>
-
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc compute server instance-action show
Shows details for a server action.
Action details of deleted instances can be returned for requests later than microversion 2.21.
Policy defaults enable only users with the administrative role or the owner of the server to perform this operation. Cloud providers can change these permissions through the policy.json
file.
Normal response codes: 200
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute server instance-action show <SERVER_ID> <ID>
Arguments:
<SERVER_ID>
— server_id parameter for /v2.1/servers/{server_id}/os-instance-actions/{id} API<ID>
— id parameter for /v2.1/servers/{server_id}/os-instance-actions/{id} API
osc compute server interface
Port interfaces (servers, os-interface)
List port interfaces, show port interface details of the given server. Create a port interface and uses it to attach a port to the given server, detach a port interface from the given server.
Usage: osc compute server interface <COMMAND>
Available subcommands:
osc compute server interface create249
— Create Interface (microversion = 2.49)osc compute server interface delete
— Detach Interfaceosc compute server interface list
— List Port Interfacesosc compute server interface show
— Show Port Interface Details
osc compute server interface create249
Creates a port interface and uses it to attach a port to a server.
Normal response codes: 200
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404), conflict(409), computeFault(500), NotImplemented(501)
Usage: osc compute server interface create249 [OPTIONS] <SERVER_ID>
Arguments:
<SERVER_ID>
— server_id parameter for /v2.1/servers/{server_id}/os-interface/{id} API
Options:
-
--fixed-ips <FIXED_IPS>
— Fixed IP addresses. If you request a specific fixed IP address without anet_id
, the request returns aBad Request (400)
response code.Parameter is an array, may be provided multiple times.
-
--net-id <NET_ID>
— The ID of the network for which you want to create a port interface. Thenet_id
andport_id
parameters are mutually exclusive. If you do not specify thenet_id
parameter, the OpenStack Networking API v2.0 uses the network information cache that is associated with the instance -
--port-id <PORT_ID>
— The ID of the port for which you want to create an interface. Thenet_id
andport_id
parameters are mutually exclusive. If you do not specify theport_id
parameter, the OpenStack Networking API v2.0 allocates a port and creates an interface for it on the network -
--tag <TAG>
— A device role tag that can be applied to a network interface when attaching it to the VM. The guest OS of a server that has devices tagged in this manner can access hardware metadata about the tagged devices from the metadata API and on the config drive, if enabled.New in version 2.49
osc compute server interface delete
Detaches a port interface from a server.
Normal response codes: 202
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404), conflict(409), NotImplemented(501)
Usage: osc compute server interface delete <SERVER_ID> <ID>
Arguments:
<SERVER_ID>
— server_id parameter for /v2.1/servers/{server_id}/os-interface/{id} API<ID>
— id parameter for /v2.1/servers/{server_id}/os-interface/{id} API
osc compute server interface list
Lists port interfaces that are attached to a server.
Normal response codes: 200
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404), NotImplemented(501)
Usage: osc compute server interface list <SERVER_ID>
Arguments:
<SERVER_ID>
— server_id parameter for /v2.1/servers/{server_id}/os-interface/{id} API
osc compute server interface show
Shows details for a port interface that is attached to a server.
Normal response codes: 200
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute server interface show <SERVER_ID> <ID>
Arguments:
<SERVER_ID>
— server_id parameter for /v2.1/servers/{server_id}/os-interface/{id} API<ID>
— id parameter for /v2.1/servers/{server_id}/os-interface/{id} API
osc compute server inject-network-info
Injects network information into a server.
Specify the injectNetworkInfo
action in the request body.
Policy defaults enable only users with the administrative role to perform this operation. Cloud providers can change these permissions through the policy.json
file.
Normal response codes: 202
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404), conflict(409)
Usage: osc compute server inject-network-info --inject-network-info <JSON> <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
--inject-network-info <JSON>
osc compute server ip
Servers IPs (servers, ips)
Lists the IP addresses for an instance and shows details for an IP address.
Usage: osc compute server ip <COMMAND>
Available subcommands:
osc compute server ip list
— List Ipsosc compute server ip show
— Show Ip Details
osc compute server ip list
Lists IP addresses that are assigned to an instance.
Policy defaults enable only users with the administrative role or the owner of the server to perform this operation. Cloud providers can change these permissions through the policy.json
file.
Normal response codes: 200
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute server ip list <SERVER_ID>
Arguments:
<SERVER_ID>
— server_id parameter for /v2.1/servers/{server_id}/ips/{id} API
osc compute server ip show
Shows IP addresses details for a network label of a server instance.
Policy defaults enable only users with the administrative role or the owner of the server to perform this operation. Cloud providers can change these permissions through the policy.json
file.
Normal response codes: 200
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute server ip show <SERVER_ID> <ID>
Arguments:
<SERVER_ID>
— server_id parameter for /v2.1/servers/{server_id}/ips/{id} API<ID>
— id parameter for /v2.1/servers/{server_id}/ips/{id} API
osc compute server list
For each server, shows server details including config drive, extended status, and server usage information.
The extended status information appears in the OS-EXT-STS:vm_state, OS-EXT-STS:power_state, and OS-EXT-STS:task_state attributes.
The server usage information appears in the OS-SRV-USG:launched_at and OS-SRV-USG:terminated_at attributes.
HostId is unique per account and is not globally unique.
Normal response codes: 200
Error response codes: badRequest(400), unauthorized(401), forbidden(403)
Usage: osc compute server list [OPTIONS]
Options:
-
--access-ip-v4 <ACCESS_IP_V4>
-
--access-ip-v6 <ACCESS_IP_V6>
-
--all-tenants <ALL_TENANTS>
-
--auto-disk-config <AUTO_DISK_CONFIG>
-
--availability-zone <AVAILABILITY_ZONE>
-
--block-device-mapping <BLOCK_DEVICE_MAPPING>
-
--changes-before <CHANGES_BEFORE>
-
--changes-since <CHANGES_SINCE>
-
--config-drive <CONFIG_DRIVE>
-
--created-at <CREATED_AT>
-
--deleted <DELETED>
-
--description <DESCRIPTION>
-
--display-description <DISPLAY_DESCRIPTION>
-
--display-name <DISPLAY_NAME>
-
--flavor <FLAVOR>
-
--host <HOST>
-
--hostname <HOSTNAME>
-
--image <IMAGE>
-
--image-ref <IMAGE_REF>
-
--info-cache <INFO_CACHE>
-
--ip <IP>
-
--ip6 <IP6>
-
--kernel-id <KERNEL_ID>
-
--key-name <KEY_NAME>
-
--launch-index <LAUNCH_INDEX>
-
--launched-at <LAUNCHED_AT>
-
--limit <LIMIT>
-
--locked <LOCKED>
-
--locked-by <LOCKED_BY>
-
--marker <MARKER>
-
--metadata <METADATA>
-
--name <NAME>
-
--node <NODE>
-
--not-tags <NOT_TAGS>
-
--not-tags-any <NOT_TAGS_ANY>
-
--pci-devices <PCI_DEVICES>
-
--power-state <POWER_STATE>
-
--progress <PROGRESS>
-
--project-name <PROJECT_NAME>
— Project Name -
--project-id <PROJECT_ID>
— Project ID -
--current-project
— Current project -
--ramdisk-id <RAMDISK_ID>
-
--reservation-id <RESERVATION_ID>
-
--root-device-name <ROOT_DEVICE_NAME>
-
--security-groups <SECURITY_GROUPS>
-
--services <SERVICES>
-
--soft-deleted <SOFT_DELETED>
-
--sort-dir <SORT_DIR>
-
--sort-key <SORT_KEY>
Possible values:
access_ip_v4
,access_ip_v6
,auto_disk_config
,availability_zone
,config_drive
,created_at
,display_description
,display_name
,host
,hostname
,image_ref
,instance_type_id
,kernel_id
,key_name
,launch_index
,launched_at
,locked
,locked_by
,node
,power_state
,progress
,project_id
,ramdisk_id
,root_device_name
,task_state
,terminated_at
,updated_at
,user_id
,uuid
,vm_state
-
--status <STATUS>
-
--system-metadata <SYSTEM_METADATA>
-
--tags <TAGS>
-
--tags-any <TAGS_ANY>
-
--task-state <TASK_STATE>
-
--tenant-id <TENANT_ID>
-
--terminated-at <TERMINATED_AT>
-
--user-name <USER_NAME>
— User Name -
--user-id <USER_ID>
— User ID -
--current-user
— Current authenticated user -
--uuid <UUID>
-
--vm-state <VM_STATE>
-
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc compute server live-migrate20
Live-Migrate Server (os-migrateLive Action) (microversion = 2.0)
Usage: osc compute server live-migrate20 --block-migration <BLOCK_MIGRATION> --disk-over-commit <DISK_OVER_COMMIT> --host <HOST> <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
-
--block-migration <BLOCK_MIGRATION>
— Set toTrue
to enable over commit when the destination host is checked for available disk space. Set toFalse
to disable over commit. This setting affects only the libvirt virt driver.Available until version 2.25
Possible values:
true
,false
-
--disk-over-commit <DISK_OVER_COMMIT>
— Set toTrue
to enable over commit when the destination host is checked for available disk space. Set toFalse
to disable over commit. This setting affects only the libvirt virt driver.Available until version 2.25
Possible values:
true
,false
-
--host <HOST>
— The host to which to migrate the server. If this parameter isNone
, the scheduler chooses a host.Warning
Prior to microversion 2.30, specifying a host will bypass validation by the scheduler, which could result in failures to actually migrate the instance to the specified host, or over-subscription of the host. It is recommended to either not specify a host so that the scheduler will pick one, or specify a host with microversion >= 2.30 and without
force=True
set.
osc compute server live-migrate225
Live-Migrate Server (os-migrateLive Action) (microversion = 2.25)
Usage: osc compute server live-migrate225 --block-migration <BLOCK_MIGRATION> --host <HOST> <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
-
--block-migration <BLOCK_MIGRATION>
— Migrates local disks by using block migration. Set toauto
which means nova will detect whether source and destination hosts on shared storage. if they are on shared storage, the live-migration won’t be block migration. Otherwise the block migration will be executed. Set toTrue
, means the request will fail when the source or destination host uses shared storage. Set toFalse
means the request will fail when the source and destination hosts are not on the shared storage.New in version 2.25
Possible values:
true
,false
-
--host <HOST>
— The host to which to migrate the server. If this parameter isNone
, the scheduler chooses a host.Warning
Prior to microversion 2.30, specifying a host will bypass validation by the scheduler, which could result in failures to actually migrate the instance to the specified host, or over-subscription of the host. It is recommended to either not specify a host so that the scheduler will pick one, or specify a host with microversion >= 2.30 and without
force=True
set.
osc compute server live-migrate230
Live-Migrate Server (os-migrateLive Action) (microversion = 2.30)
Usage: osc compute server live-migrate230 [OPTIONS] --block-migration <BLOCK_MIGRATION> --host <HOST> <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
-
--block-migration <BLOCK_MIGRATION>
— Migrates local disks by using block migration. Set toauto
which means nova will detect whether source and destination hosts on shared storage. if they are on shared storage, the live-migration won’t be block migration. Otherwise the block migration will be executed. Set toTrue
, means the request will fail when the source or destination host uses shared storage. Set toFalse
means the request will fail when the source and destination hosts are not on the shared storage.New in version 2.25
Possible values:
true
,false
-
--force <FORCE>
— Force a live-migration by not verifying the provided destination host by the scheduler.Warning
This could result in failures to actually live migrate the instance to the specified host. It is recommended to either not specify a host so that the scheduler will pick one, or specify a host without
force=True
set.New in version 2.30
Available until version 2.67
Possible values:
true
,false
-
--host <HOST>
— The host to which to migrate the server. If this parameter isNone
, the scheduler chooses a host.Warning
Prior to microversion 2.30, specifying a host will bypass validation by the scheduler, which could result in failures to actually migrate the instance to the specified host, or over-subscription of the host. It is recommended to either not specify a host so that the scheduler will pick one, or specify a host with microversion >= 2.30 and without
force=True
set.
osc compute server live-migrate268
Live-Migrate Server (os-migrateLive Action) (microversion = 2.68)
Usage: osc compute server live-migrate268 --block-migration <BLOCK_MIGRATION> --host <HOST> <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
-
--block-migration <BLOCK_MIGRATION>
— Migrates local disks by using block migration. Set toauto
which means nova will detect whether source and destination hosts on shared storage. if they are on shared storage, the live-migration won’t be block migration. Otherwise the block migration will be executed. Set toTrue
, means the request will fail when the source or destination host uses shared storage. Set toFalse
means the request will fail when the source and destination hosts are not on the shared storage.New in version 2.25
Possible values:
true
,false
-
--host <HOST>
— The host to which to migrate the server. If this parameter isNone
, the scheduler chooses a host.Warning
Prior to microversion 2.30, specifying a host will bypass validation by the scheduler, which could result in failures to actually migrate the instance to the specified host, or over-subscription of the host. It is recommended to either not specify a host so that the scheduler will pick one, or specify a host with microversion >= 2.30 and without
force=True
set.
osc compute server lock273
Lock Server (lock Action) (microversion = 2.73)
Usage: osc compute server lock273 [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
--locked-reason <LOCKED_REASON>
osc compute server metadata
Lists metadata, creates or replaces one or more metadata items, and updates one or more metadata items for a server.
Shows details for, creates or replaces, and updates a metadata item, by key, for a server.
Usage: osc compute server metadata <COMMAND>
Available subcommands:
osc compute server metadata create
— Create or Update Metadata Itemsosc compute server metadata delete
— Delete Metadata Itemosc compute server metadata list
— List All Metadataosc compute server metadata replace
— Replace Metadata Itemsosc compute server metadata set
— Create Or Update Metadata Itemosc compute server metadata show
— Show Metadata Item Details
osc compute server metadata create
Create or update one or more metadata items for a server.
Creates any metadata items that do not already exist in the server, replaces exists metadata items that match keys. Does not modify items that are not in the request.
Policy defaults enable only users with the administrative role or the owner of the server to perform this operation. Cloud providers can change these permissions through the policy.json
file.
Normal response codes: 200
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404), conflict(409)
Usage: osc compute server metadata create [OPTIONS] <SERVER_ID>
Arguments:
<SERVER_ID>
— server_id parameter for /v2.1/servers/{server_id}/metadata/{id} API
Options:
--metadata <key=value>
— Metadata key and value pairs. The maximum size for each metadata key and value pair is 255 bytes
osc compute server metadata delete
Deletes a metadata item, by key, from a server.
Policy defaults enable only users with the administrative role or the owner of the server to perform this operation. Cloud providers can change these permissions through the policy.json
file.
Normal response codes: 204
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404), conflict(409)
Usage: osc compute server metadata delete <SERVER_ID> <ID>
Arguments:
<SERVER_ID>
— server_id parameter for /v2.1/servers/{server_id}/metadata/{id} API<ID>
— id parameter for /v2.1/servers/{server_id}/metadata/{id} API
osc compute server metadata list
Lists all metadata for a server.
Policy defaults enable only users with the administrative role or the owner of the server to perform this operation. Cloud providers can change these permissions through the policy.json
file.
Normal response codes: 200
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute server metadata list <SERVER_ID>
Arguments:
<SERVER_ID>
— server_id parameter for /v2.1/servers/{server_id}/metadata/{id} API
osc compute server metadata replace
Replaces one or more metadata items for a server.
Creates any metadata items that do not already exist in the server. Removes and completely replaces any metadata items that already exist in the server with the metadata items in the request.
Policy defaults enable only users with the administrative role or the owner of the server to perform this operation. Cloud providers can change these permissions through the policy.json
file.
Normal response codes: 200
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404), conflict(409)
Usage: osc compute server metadata replace [OPTIONS] <SERVER_ID>
Arguments:
<SERVER_ID>
— server_id parameter for /v2.1/servers/{server_id}/metadata/{id} API
Options:
--metadata <key=value>
— Metadata key and value pairs. The maximum size for each metadata key and value pair is 255 bytes
osc compute server metadata set
Creates or replaces a metadata item, by key, for a server.
Creates a metadata item that does not already exist in the server. Replaces existing metadata items that match keys with the metadata item in the request.
Policy defaults enable only users with the administrative role or the owner of the server to perform this operation. Cloud providers can change these permissions through the policy.json
file.
Normal response codes: 200
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404), conflict(409)
Usage: osc compute server metadata set [OPTIONS] <SERVER_ID> <ID>
Arguments:
<SERVER_ID>
— server_id parameter for /v2.1/servers/{server_id}/metadata/{id} API<ID>
— id parameter for /v2.1/servers/{server_id}/metadata/{id} API
Options:
--meta <key=value>
osc compute server metadata show
Shows details for a metadata item, by key, for a server.
Policy defaults enable only users with the administrative role or the owner of the server to perform this operation. Cloud providers can change these permissions through the policy.json
file.
Normal response codes: 200
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute server metadata show <SERVER_ID> <ID>
Arguments:
<SERVER_ID>
— server_id parameter for /v2.1/servers/{server_id}/metadata/{id} API<ID>
— id parameter for /v2.1/servers/{server_id}/metadata/{id} API
osc compute server migrate256
Migrates a server to a host.
Specify the migrate
action in the request body.
Up to microversion 2.55, the scheduler chooses the host. Starting from microversion 2.56, the host
parameter is available to specify the destination host. If you specify null
or don’t specify this parameter, the scheduler chooses a host.
Asynchronous Postconditions
A successfully migrated server shows a VERIFY_RESIZE
status and finished
migration status. If the cloud has configured the resize_confirm_window option of the Compute service to a positive value, the Compute service automatically confirms the migrate operation after the configured interval.
There are two different policies for this action, depending on whether the host parameter is set. Both defaults enable only users with the administrative role to perform this operation. Cloud providers can change these permissions through the policy.json
file.
Normal response codes: 202
Error response codes: badRequest(400), unauthorized(401), forbidden(403) itemNotFound(404), conflict(409)
Usage: osc compute server migrate256 [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
--host <HOST>
osc compute server migration
Server migrations (servers, migrations)
List, show, perform actions on and delete server migrations.
Usage: osc compute server migration <COMMAND>
Available subcommands:
osc compute server migration delete
— Delete (Abort) Migrationosc compute server migration force-complete222
— Force Migration Complete Action (force_complete Action) (microversion = 2.22)osc compute server migration list
— List Migrationsosc compute server migration show
— Show Migration Details
osc compute server migration delete
Abort an in-progress live migration.
Policy defaults enable only users with the administrative role to perform this operation. Cloud providers can change these permissions through the policy.json
file.
Preconditions
The server OS-EXT-STS:task_state value must be migrating
.
If the server is locked, you must have administrator privileges to force the completion of the server migration.
For microversions from 2.24 to 2.64 the migration status must be running
, for microversion 2.65 and greater, the migration status can also be queued
and preparing
.
Asynchronous Postconditions
After you make this request, you typically must keep polling the server status to determine whether the request succeeded. You may also monitor the migration using:
Troubleshooting
If the server status remains MIGRATING
for an inordinate amount of time, the request may have failed. Ensure you meet the preconditions and run the request again. If the request fails again, investigate the compute back end.
Normal response codes: 202
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404), conflict(409)
Usage: osc compute server migration delete <SERVER_ID> <ID>
Arguments:
<SERVER_ID>
— server_id parameter for /v2.1/servers/{server_id}/migrations/{id} API<ID>
— id parameter for /v2.1/servers/{server_id}/migrations/{id} API
osc compute server migration force-complete222
Force an in-progress live migration for a given server to complete.
Specify the force_complete
action in the request body.
Policy defaults enable only users with the administrative role to perform this operation. Cloud providers can change these permissions through the policy.json
file.
Preconditions
The server OS-EXT-STS:vm_state value must be active
and the server OS-EXT-STS:task_state value must be migrating
.
If the server is locked, you must have administrator privileges to force the completion of the server migration.
The migration status must be running
.
Asynchronous Postconditions
After you make this request, you typically must keep polling the server status to determine whether the request succeeded.
Troubleshooting
If the server status remains MIGRATING
for an inordinate amount of time, the request may have failed. Ensure you meet the preconditions and run the request again. If the request fails again, investigate the compute back end. More details can be found in the admin guide.
Normal response codes: 202
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404), conflict(409)
Usage: osc compute server migration force-complete222 <SERVER_ID> <ID>
Arguments:
<SERVER_ID>
— server_id parameter for /v2.1/servers/{server_id}/migrations/{id}/action API<ID>
— id parameter for /v2.1/servers/{server_id}/migrations/{id}/action API
osc compute server migration list
Lists in-progress live migrations for a given server.
Policy defaults enable only users with the administrative role to perform this operation. Cloud providers can change these permissions through the policy.json
file.
Normal response codes: 200
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute server migration list <SERVER_ID>
Arguments:
<SERVER_ID>
— server_id parameter for /v2.1/servers/{server_id}/migrations/{id} API
osc compute server migration show
Show details for an in-progress live migration for a given server.
Policy defaults enable only users with the administrative role to perform this operation. Cloud providers can change these permissions through the policy.json
file.
Normal response codes: 200
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute server migration show <SERVER_ID> <ID>
Arguments:
<SERVER_ID>
— server_id parameter for /v2.1/servers/{server_id}/migrations/{id} API<ID>
— id parameter for /v2.1/servers/{server_id}/migrations/{id} API
osc compute server password
Servers password
Shows the encrypted administrative password. Also, clears the encrypted administrative password for a server, which removes it from the metadata server.
Usage: osc compute server password <COMMAND>
Available subcommands:
osc compute server password delete
— Clear Admin Passwordosc compute server password show
— Show Server Password
osc compute server password delete
Clears the encrypted administrative password for a server, which removes it from the database.
This action does not actually change the instance server password.
Policy defaults enable only users with the administrative role or the owner of the server to perform this operation. Cloud providers can change these permissions through the policy.json
file.
Normal response codes: 204
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute server password delete <SERVER_ID>
Arguments:
<SERVER_ID>
— server_id parameter for /v2.1/servers/{server_id}/os-server-password API
osc compute server password show
Shows the administrative password for a server.
This operation calls the metadata service to query metadata information and does not read password information from the server itself.
The password saved in the metadata service is typically encrypted using the public SSH key injected into this server, so the SSH private key is needed to read the password.
Policy defaults enable only users with the administrative role or the owner of the server to perform this operation. Cloud providers can change these permissions through the policy.json
file.
Normal response codes: 200
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute server password show <SERVER_ID>
Arguments:
<SERVER_ID>
— server_id parameter for /v2.1/servers/{server_id}/os-server-password API
osc compute server pause
Pauses a server. Changes its status to PAUSED
.
Specify the pause
action in the request body.
Policy defaults enable only users with the administrative role or the owner of the server to perform this operation. Cloud providers can change these permissions through the policy.json
file.
Normal response codes: 202
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404), conflict(409), notImplemented(501)
Usage: osc compute server pause --pause <JSON> <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
--pause <JSON>
osc compute server reset-state
Resets the state of a server.
Specify the os-resetState
action and the state
in the request body.
Policy defaults enable only users with the administrative role to perform this operation. Cloud providers can change these permissions through the policy.json
file.
Normal response codes: 202
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute server reset-state --state <STATE> <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
-
--state <STATE>
— The state of the server to be set,active
orerror
are validPossible values:
active
,error
osc compute server reboot
Reboots a server.
Specify the reboot
action in the request body.
Preconditions
The preconditions for rebooting a server depend on the type of reboot.
You can only SOFT reboot a server when its status is ACTIVE
.
You can only HARD reboot a server when its status is one of:
If the server is locked, you must have administrator privileges to reboot the server.
Asynchronous Postconditions
After you successfully reboot a server, its status changes to ACTIVE
.
Normal response codes: 202
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404), conflict(409)
Usage: osc compute server reboot --type <TYPE> <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
-
--type <TYPE>
— The type of the reboot action. The valid values areHARD
andSOFT
. ASOFT
reboot attempts a graceful shutdown and restart of the server. AHARD
reboot attempts a forced shutdown and restart of the server. TheHARD
reboot corresponds to the power cycles of the serverPossible values:
hard
,soft
osc compute server rebuild21
Rebuild Server (rebuild Action) (microversion = 2.1)
Usage: osc compute server rebuild21 [OPTIONS] --image-ref <IMAGE_REF> <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
-
--access-ipv4 <ACCESS_IPV4>
— IPv4 address that should be used to access this server -
--access-ipv6 <ACCESS_IPV6>
— IPv6 address that should be used to access this server -
--admin-pass <ADMIN_PASS>
— The administrative password of the server. If you omit this parameter, the operation generates a new password -
--image-ref <IMAGE_REF>
— The UUID of the image to rebuild for your server instance. It must be a valid UUID otherwise API will return 400. To rebuild a volume-backed server with a new image, at least microversion 2.93 needs to be provided in the request else the request will fall back to old behaviour i.e. the API will return 400 (for an image different from the image used when creating the volume). For non-volume-backed servers, specifying a new image will result in validating that the image is acceptable for the current compute host on which the server exists. If the new image is not valid, the server will go intoERROR
status -
--metadata <key=value>
— Metadata key and value pairs. The maximum size of the metadata key and value is 255 bytes each -
--name <NAME>
— The server name -
--os-dcf-disk-config <OS_DCF_DISK_CONFIG>
— Controls how the API partitions the disk when you create, rebuild, or resize servers. A server inherits theOS-DCF:diskConfig
value from the image from which it was created, and an image inherits theOS-DCF:diskConfig
value from the server from which it was created. To override the inherited setting, you can include this attribute in the request body of a server create, rebuild, or resize request. If theOS-DCF:diskConfig
value for an image isMANUAL
, you cannot create a server from that image and set itsOS-DCF:diskConfig
value toAUTO
. A valid value is:AUTO
. The API builds the server with a single partition the size of the target flavor disk. The API automatically adjusts the file system to fit the entire partition. -MANUAL
. The API builds the server by using whatever partition scheme and file system is in the source image. If the target flavor disk is larger, the API does not partition the remaining disk space.
Possible values:
auto
,manual
-
--personality <JSON>
— The file path and contents, text only, to inject into the server at launch. The maximum size of the file path data is 255 bytes. The maximum limit is the number of allowed bytes in the decoded, rather than encoded, data.Available until version 2.56
Parameter is an array, may be provided multiple times.
-
--preserve-ephemeral <PRESERVE_EPHEMERAL>
— Indicates whether the server is rebuilt with the preservation of the ephemeral partition (true
).Note
This only works with baremetal servers provided by Ironic. Passing it to any other server instance results in a fault and will prevent the rebuild from happening.
Possible values:
true
,false
osc compute server rebuild219
Rebuild Server (rebuild Action) (microversion = 2.19)
Usage: osc compute server rebuild219 [OPTIONS] --image-ref <IMAGE_REF> <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
-
--access-ipv4 <ACCESS_IPV4>
— IPv4 address that should be used to access this server -
--access-ipv6 <ACCESS_IPV6>
— IPv6 address that should be used to access this server -
--admin-pass <ADMIN_PASS>
— The administrative password of the server. If you omit this parameter, the operation generates a new password -
--description <DESCRIPTION>
— A free form description of the server. Limited to 255 characters in length. Before microversion 2.19 this was set to the server name.New in version 2.19
-
--image-ref <IMAGE_REF>
— The UUID of the image to rebuild for your server instance. It must be a valid UUID otherwise API will return 400. To rebuild a volume-backed server with a new image, at least microversion 2.93 needs to be provided in the request else the request will fall back to old behaviour i.e. the API will return 400 (for an image different from the image used when creating the volume). For non-volume-backed servers, specifying a new image will result in validating that the image is acceptable for the current compute host on which the server exists. If the new image is not valid, the server will go intoERROR
status -
--metadata <key=value>
— Metadata key and value pairs. The maximum size of the metadata key and value is 255 bytes each -
--name <NAME>
— The server name -
--os-dcf-disk-config <OS_DCF_DISK_CONFIG>
— Controls how the API partitions the disk when you create, rebuild, or resize servers. A server inherits theOS-DCF:diskConfig
value from the image from which it was created, and an image inherits theOS-DCF:diskConfig
value from the server from which it was created. To override the inherited setting, you can include this attribute in the request body of a server create, rebuild, or resize request. If theOS-DCF:diskConfig
value for an image isMANUAL
, you cannot create a server from that image and set itsOS-DCF:diskConfig
value toAUTO
. A valid value is:AUTO
. The API builds the server with a single partition the size of the target flavor disk. The API automatically adjusts the file system to fit the entire partition. -MANUAL
. The API builds the server by using whatever partition scheme and file system is in the source image. If the target flavor disk is larger, the API does not partition the remaining disk space.
Possible values:
auto
,manual
-
--personality <JSON>
— The file path and contents, text only, to inject into the server at launch. The maximum size of the file path data is 255 bytes. The maximum limit is the number of allowed bytes in the decoded, rather than encoded, data.Available until version 2.56
Parameter is an array, may be provided multiple times.
-
--preserve-ephemeral <PRESERVE_EPHEMERAL>
— Indicates whether the server is rebuilt with the preservation of the ephemeral partition (true
).Note
This only works with baremetal servers provided by Ironic. Passing it to any other server instance results in a fault and will prevent the rebuild from happening.
Possible values:
true
,false
osc compute server rebuild254
Rebuild Server (rebuild Action) (microversion = 2.54)
Usage: osc compute server rebuild254 [OPTIONS] --image-ref <IMAGE_REF> <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
-
--access-ipv4 <ACCESS_IPV4>
— IPv4 address that should be used to access this server -
--access-ipv6 <ACCESS_IPV6>
— IPv6 address that should be used to access this server -
--admin-pass <ADMIN_PASS>
— The administrative password of the server. If you omit this parameter, the operation generates a new password -
--description <DESCRIPTION>
— A free form description of the server. Limited to 255 characters in length. Before microversion 2.19 this was set to the server name.New in version 2.19
-
--image-ref <IMAGE_REF>
— The UUID of the image to rebuild for your server instance. It must be a valid UUID otherwise API will return 400. To rebuild a volume-backed server with a new image, at least microversion 2.93 needs to be provided in the request else the request will fall back to old behaviour i.e. the API will return 400 (for an image different from the image used when creating the volume). For non-volume-backed servers, specifying a new image will result in validating that the image is acceptable for the current compute host on which the server exists. If the new image is not valid, the server will go intoERROR
status -
--key-name <KEY_NAME>
— Key pair name for rebuild API. Ifnull
is specified, the existing keypair is unset.Note
Users within the same project are able to rebuild other user’s instances in that project with a new keypair. Keys are owned by users (which is the only resource that’s true of). Servers are owned by projects. Because of this a rebuild with a key_name is looking up the keypair by the user calling rebuild.
New in version 2.54
-
--metadata <key=value>
— Metadata key and value pairs. The maximum size of the metadata key and value is 255 bytes each -
--name <NAME>
— The server name -
--os-dcf-disk-config <OS_DCF_DISK_CONFIG>
— Controls how the API partitions the disk when you create, rebuild, or resize servers. A server inherits theOS-DCF:diskConfig
value from the image from which it was created, and an image inherits theOS-DCF:diskConfig
value from the server from which it was created. To override the inherited setting, you can include this attribute in the request body of a server create, rebuild, or resize request. If theOS-DCF:diskConfig
value for an image isMANUAL
, you cannot create a server from that image and set itsOS-DCF:diskConfig
value toAUTO
. A valid value is:AUTO
. The API builds the server with a single partition the size of the target flavor disk. The API automatically adjusts the file system to fit the entire partition. -MANUAL
. The API builds the server by using whatever partition scheme and file system is in the source image. If the target flavor disk is larger, the API does not partition the remaining disk space.
Possible values:
auto
,manual
-
--personality <JSON>
— The file path and contents, text only, to inject into the server at launch. The maximum size of the file path data is 255 bytes. The maximum limit is the number of allowed bytes in the decoded, rather than encoded, data.Available until version 2.56
Parameter is an array, may be provided multiple times.
-
--preserve-ephemeral <PRESERVE_EPHEMERAL>
— Indicates whether the server is rebuilt with the preservation of the ephemeral partition (true
).Note
This only works with baremetal servers provided by Ironic. Passing it to any other server instance results in a fault and will prevent the rebuild from happening.
Possible values:
true
,false
osc compute server rebuild257
Rebuild Server (rebuild Action) (microversion = 2.57)
Usage: osc compute server rebuild257 [OPTIONS] --image-ref <IMAGE_REF> <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
-
--access-ipv4 <ACCESS_IPV4>
— IPv4 address that should be used to access this server -
--access-ipv6 <ACCESS_IPV6>
— IPv6 address that should be used to access this server -
--admin-pass <ADMIN_PASS>
— The administrative password of the server. If you omit this parameter, the operation generates a new password -
--description <DESCRIPTION>
— A free form description of the server. Limited to 255 characters in length. Before microversion 2.19 this was set to the server name.New in version 2.19
-
--image-ref <IMAGE_REF>
— The UUID of the image to rebuild for your server instance. It must be a valid UUID otherwise API will return 400. To rebuild a volume-backed server with a new image, at least microversion 2.93 needs to be provided in the request else the request will fall back to old behaviour i.e. the API will return 400 (for an image different from the image used when creating the volume). For non-volume-backed servers, specifying a new image will result in validating that the image is acceptable for the current compute host on which the server exists. If the new image is not valid, the server will go intoERROR
status -
--key-name <KEY_NAME>
— Key pair name for rebuild API. Ifnull
is specified, the existing keypair is unset.Note
Users within the same project are able to rebuild other user’s instances in that project with a new keypair. Keys are owned by users (which is the only resource that’s true of). Servers are owned by projects. Because of this a rebuild with a key_name is looking up the keypair by the user calling rebuild.
New in version 2.54
-
--metadata <key=value>
— Metadata key and value pairs. The maximum size of the metadata key and value is 255 bytes each -
--name <NAME>
— The server name -
--os-dcf-disk-config <OS_DCF_DISK_CONFIG>
— Controls how the API partitions the disk when you create, rebuild, or resize servers. A server inherits theOS-DCF:diskConfig
value from the image from which it was created, and an image inherits theOS-DCF:diskConfig
value from the server from which it was created. To override the inherited setting, you can include this attribute in the request body of a server create, rebuild, or resize request. If theOS-DCF:diskConfig
value for an image isMANUAL
, you cannot create a server from that image and set itsOS-DCF:diskConfig
value toAUTO
. A valid value is:AUTO
. The API builds the server with a single partition the size of the target flavor disk. The API automatically adjusts the file system to fit the entire partition. -MANUAL
. The API builds the server by using whatever partition scheme and file system is in the source image. If the target flavor disk is larger, the API does not partition the remaining disk space.
Possible values:
auto
,manual
-
--preserve-ephemeral <PRESERVE_EPHEMERAL>
— Indicates whether the server is rebuilt with the preservation of the ephemeral partition (true
).Note
This only works with baremetal servers provided by Ironic. Passing it to any other server instance results in a fault and will prevent the rebuild from happening.
Possible values:
true
,false
-
--user-data <USER_DATA>
— Configuration information or scripts to use upon rebuild. Must be Base64 encoded. Restricted to 65535 bytes. Ifnull
is specified, the existing user_data is unset.New in version 2.57
osc compute server rebuild263
Rebuild Server (rebuild Action) (microversion = 2.63)
Usage: osc compute server rebuild263 [OPTIONS] --image-ref <IMAGE_REF> <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
-
--access-ipv4 <ACCESS_IPV4>
— IPv4 address that should be used to access this server -
--access-ipv6 <ACCESS_IPV6>
— IPv6 address that should be used to access this server -
--admin-pass <ADMIN_PASS>
— The administrative password of the server. If you omit this parameter, the operation generates a new password -
--description <DESCRIPTION>
— A free form description of the server. Limited to 255 characters in length. Before microversion 2.19 this was set to the server name.New in version 2.19
-
--image-ref <IMAGE_REF>
— The UUID of the image to rebuild for your server instance. It must be a valid UUID otherwise API will return 400. To rebuild a volume-backed server with a new image, at least microversion 2.93 needs to be provided in the request else the request will fall back to old behaviour i.e. the API will return 400 (for an image different from the image used when creating the volume). For non-volume-backed servers, specifying a new image will result in validating that the image is acceptable for the current compute host on which the server exists. If the new image is not valid, the server will go intoERROR
status -
--key-name <KEY_NAME>
— Key pair name for rebuild API. Ifnull
is specified, the existing keypair is unset.Note
Users within the same project are able to rebuild other user’s instances in that project with a new keypair. Keys are owned by users (which is the only resource that’s true of). Servers are owned by projects. Because of this a rebuild with a key_name is looking up the keypair by the user calling rebuild.
New in version 2.54
-
--metadata <key=value>
— Metadata key and value pairs. The maximum size of the metadata key and value is 255 bytes each -
--name <NAME>
— The server name -
--os-dcf-disk-config <OS_DCF_DISK_CONFIG>
— Controls how the API partitions the disk when you create, rebuild, or resize servers. A server inherits theOS-DCF:diskConfig
value from the image from which it was created, and an image inherits theOS-DCF:diskConfig
value from the server from which it was created. To override the inherited setting, you can include this attribute in the request body of a server create, rebuild, or resize request. If theOS-DCF:diskConfig
value for an image isMANUAL
, you cannot create a server from that image and set itsOS-DCF:diskConfig
value toAUTO
. A valid value is:AUTO
. The API builds the server with a single partition the size of the target flavor disk. The API automatically adjusts the file system to fit the entire partition. -MANUAL
. The API builds the server by using whatever partition scheme and file system is in the source image. If the target flavor disk is larger, the API does not partition the remaining disk space.
Possible values:
auto
,manual
-
--preserve-ephemeral <PRESERVE_EPHEMERAL>
— Indicates whether the server is rebuilt with the preservation of the ephemeral partition (true
).Note
This only works with baremetal servers provided by Ironic. Passing it to any other server instance results in a fault and will prevent the rebuild from happening.
Possible values:
true
,false
-
--trusted-image-certificates <TRUSTED_IMAGE_CERTIFICATES>
— A list of trusted certificate IDs, which are used during image signature verification to verify the signing certificate. The list is restricted to a maximum of 50 IDs. This parameter is optional in server rebuild requests if allowed by policy, and is not supported for volume-backed instances.If
null
is specified, the existing trusted certificate IDs are either unset or reset to the configured defaults.New in version 2.63
Parameter is an array, may be provided multiple times.
-
--user-data <USER_DATA>
— Configuration information or scripts to use upon rebuild. Must be Base64 encoded. Restricted to 65535 bytes. Ifnull
is specified, the existing user_data is unset.New in version 2.57
osc compute server rebuild290
Rebuild Server (rebuild Action) (microversion = 2.90)
Usage: osc compute server rebuild290 [OPTIONS] --image-ref <IMAGE_REF> <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
-
--access-ipv4 <ACCESS_IPV4>
— IPv4 address that should be used to access this server -
--access-ipv6 <ACCESS_IPV6>
— IPv6 address that should be used to access this server -
--admin-pass <ADMIN_PASS>
— The administrative password of the server. If you omit this parameter, the operation generates a new password -
--description <DESCRIPTION>
— A free form description of the server. Limited to 255 characters in length. Before microversion 2.19 this was set to the server name.New in version 2.19
-
--hostname <HOSTNAME>
— The hostname to configure for the instance in the metadata service.Starting with microversion 2.94, this can be a Fully Qualified Domain Name (FQDN) of up to 255 characters in length.
Note
This information is published via the metadata service and requires application such as
cloud-init
to propagate it through to the instance.New in version 2.90
-
--image-ref <IMAGE_REF>
— The UUID of the image to rebuild for your server instance. It must be a valid UUID otherwise API will return 400. To rebuild a volume-backed server with a new image, at least microversion 2.93 needs to be provided in the request else the request will fall back to old behaviour i.e. the API will return 400 (for an image different from the image used when creating the volume). For non-volume-backed servers, specifying a new image will result in validating that the image is acceptable for the current compute host on which the server exists. If the new image is not valid, the server will go intoERROR
status -
--key-name <KEY_NAME>
— Key pair name for rebuild API. Ifnull
is specified, the existing keypair is unset.Note
Users within the same project are able to rebuild other user’s instances in that project with a new keypair. Keys are owned by users (which is the only resource that’s true of). Servers are owned by projects. Because of this a rebuild with a key_name is looking up the keypair by the user calling rebuild.
New in version 2.54
-
--metadata <key=value>
— Metadata key and value pairs. The maximum size of the metadata key and value is 255 bytes each -
--name <NAME>
— The server name -
--os-dcf-disk-config <OS_DCF_DISK_CONFIG>
— Controls how the API partitions the disk when you create, rebuild, or resize servers. A server inherits theOS-DCF:diskConfig
value from the image from which it was created, and an image inherits theOS-DCF:diskConfig
value from the server from which it was created. To override the inherited setting, you can include this attribute in the request body of a server create, rebuild, or resize request. If theOS-DCF:diskConfig
value for an image isMANUAL
, you cannot create a server from that image and set itsOS-DCF:diskConfig
value toAUTO
. A valid value is:AUTO
. The API builds the server with a single partition the size of the target flavor disk. The API automatically adjusts the file system to fit the entire partition. -MANUAL
. The API builds the server by using whatever partition scheme and file system is in the source image. If the target flavor disk is larger, the API does not partition the remaining disk space.
Possible values:
auto
,manual
-
--preserve-ephemeral <PRESERVE_EPHEMERAL>
— Indicates whether the server is rebuilt with the preservation of the ephemeral partition (true
).Note
This only works with baremetal servers provided by Ironic. Passing it to any other server instance results in a fault and will prevent the rebuild from happening.
Possible values:
true
,false
-
--trusted-image-certificates <TRUSTED_IMAGE_CERTIFICATES>
— A list of trusted certificate IDs, which are used during image signature verification to verify the signing certificate. The list is restricted to a maximum of 50 IDs. This parameter is optional in server rebuild requests if allowed by policy, and is not supported for volume-backed instances.If
null
is specified, the existing trusted certificate IDs are either unset or reset to the configured defaults.New in version 2.63
Parameter is an array, may be provided multiple times.
-
--user-data <USER_DATA>
— Configuration information or scripts to use upon rebuild. Must be Base64 encoded. Restricted to 65535 bytes. Ifnull
is specified, the existing user_data is unset.New in version 2.57
osc compute server rebuild294
Rebuild Server (rebuild Action) (microversion = 2.94)
Usage: osc compute server rebuild294 [OPTIONS] --image-ref <IMAGE_REF> <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
-
--access-ipv4 <ACCESS_IPV4>
— IPv4 address that should be used to access this server -
--access-ipv6 <ACCESS_IPV6>
— IPv6 address that should be used to access this server -
--admin-pass <ADMIN_PASS>
— The administrative password of the server. If you omit this parameter, the operation generates a new password -
--description <DESCRIPTION>
— A free form description of the server. Limited to 255 characters in length. Before microversion 2.19 this was set to the server name.New in version 2.19
-
--hostname <HOSTNAME>
— The hostname to configure for the instance in the metadata service.Starting with microversion 2.94, this can be a Fully Qualified Domain Name (FQDN) of up to 255 characters in length.
Note
This information is published via the metadata service and requires application such as
cloud-init
to propagate it through to the instance.New in version 2.90
-
--image-ref <IMAGE_REF>
— The UUID of the image to rebuild for your server instance. It must be a valid UUID otherwise API will return 400. To rebuild a volume-backed server with a new image, at least microversion 2.93 needs to be provided in the request else the request will fall back to old behaviour i.e. the API will return 400 (for an image different from the image used when creating the volume). For non-volume-backed servers, specifying a new image will result in validating that the image is acceptable for the current compute host on which the server exists. If the new image is not valid, the server will go intoERROR
status -
--key-name <KEY_NAME>
— Key pair name for rebuild API. Ifnull
is specified, the existing keypair is unset.Note
Users within the same project are able to rebuild other user’s instances in that project with a new keypair. Keys are owned by users (which is the only resource that’s true of). Servers are owned by projects. Because of this a rebuild with a key_name is looking up the keypair by the user calling rebuild.
New in version 2.54
-
--metadata <key=value>
— Metadata key and value pairs. The maximum size of the metadata key and value is 255 bytes each -
--name <NAME>
— The server name -
--os-dcf-disk-config <OS_DCF_DISK_CONFIG>
— Controls how the API partitions the disk when you create, rebuild, or resize servers. A server inherits theOS-DCF:diskConfig
value from the image from which it was created, and an image inherits theOS-DCF:diskConfig
value from the server from which it was created. To override the inherited setting, you can include this attribute in the request body of a server create, rebuild, or resize request. If theOS-DCF:diskConfig
value for an image isMANUAL
, you cannot create a server from that image and set itsOS-DCF:diskConfig
value toAUTO
. A valid value is:AUTO
. The API builds the server with a single partition the size of the target flavor disk. The API automatically adjusts the file system to fit the entire partition. -MANUAL
. The API builds the server by using whatever partition scheme and file system is in the source image. If the target flavor disk is larger, the API does not partition the remaining disk space.
Possible values:
auto
,manual
-
--preserve-ephemeral <PRESERVE_EPHEMERAL>
— Indicates whether the server is rebuilt with the preservation of the ephemeral partition (true
).Note
This only works with baremetal servers provided by Ironic. Passing it to any other server instance results in a fault and will prevent the rebuild from happening.
Possible values:
true
,false
-
--trusted-image-certificates <TRUSTED_IMAGE_CERTIFICATES>
— A list of trusted certificate IDs, which are used during image signature verification to verify the signing certificate. The list is restricted to a maximum of 50 IDs. This parameter is optional in server rebuild requests if allowed by policy, and is not supported for volume-backed instances.If
null
is specified, the existing trusted certificate IDs are either unset or reset to the configured defaults.New in version 2.63
Parameter is an array, may be provided multiple times.
-
--user-data <USER_DATA>
— Configuration information or scripts to use upon rebuild. Must be Base64 encoded. Restricted to 65535 bytes. Ifnull
is specified, the existing user_data is unset.New in version 2.57
osc compute server remote-console
Server Consoles
Manage server consoles.
Usage: osc compute server remote-console <COMMAND>
Available subcommands:
osc compute server remote-console create26
— Create Console (microversion = 2.6)osc compute server remote-console create28
— Create Console (microversion = 2.8)
osc compute server remote-console create26
The API provides a unified request for creating a remote console. The user can get a URL to connect the console from this API. The URL includes the token which is used to get permission to access the console. Servers may support different console protocols. To return a remote console using a specific protocol, such as VNC, set the protocol
parameter to vnc
.
Normal response codes: 200
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404), conflict(409), notImplemented(501)
Usage: osc compute server remote-console create26 --protocol <PROTOCOL> --type <TYPE> <SERVER_ID>
Arguments:
<SERVER_ID>
— server_id parameter for /v2.1/servers/{server_id}/remote-consoles API
Options:
-
--protocol <PROTOCOL>
— The protocol of remote console. The valid values arevnc
,spice
,serial
andmks
. The protocolmks
is added since Microversion2.8
Possible values:
serial
,spice
,vnc
-
--type <TYPE>
— The type of remote console. The valid values arenovnc
,spice-html5
,spice-direct
,serial
, andwebmks
. The typewebmks
was added in Microversion2.8
, and the typespice-direct
was added in Microversion2.99
Possible values:
novnc
,serial
,spice-html5
,xvpvnc
osc compute server remote-console create28
The API provides a unified request for creating a remote console. The user can get a URL to connect the console from this API. The URL includes the token which is used to get permission to access the console. Servers may support different console protocols. To return a remote console using a specific protocol, such as VNC, set the protocol
parameter to vnc
.
Normal response codes: 200
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404), conflict(409), notImplemented(501)
Usage: osc compute server remote-console create28 --protocol <PROTOCOL> --type <TYPE> <SERVER_ID>
Arguments:
<SERVER_ID>
— server_id parameter for /v2.1/servers/{server_id}/remote-consoles API
Options:
-
--protocol <PROTOCOL>
— The protocol of remote console. The valid values arevnc
,spice
,serial
andmks
. The protocolmks
is added since Microversion2.8
Possible values:
mks
,serial
,spice
,vnc
-
--type <TYPE>
— The type of remote console. The valid values arenovnc
,spice-html5
,spice-direct
,serial
, andwebmks
. The typewebmks
was added in Microversion2.8
, and the typespice-direct
was added in Microversion2.99
Possible values:
novnc
,serial
,spice-html5
,webmks
,xvpvnc
osc compute server remove-fixed-ip21
Removes, or disassociates, a fixed IP address from a server.
Specify the removeFixedIp
action in the request body.
Policy defaults enable only users with the administrative role or the owner of the server to perform this operation. Cloud providers can change these permissions through the policy.json
file.
Normal response codes: 202
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute server remove-fixed-ip21 --address <ADDRESS> <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
--address <ADDRESS>
— The IP address
osc compute server remove-floating-ip21
Removes, or disassociates, a floating IP address from a server.
The IP address is returned to the pool of IP addresses that is available for all projects. When you remove a floating IP address and that IP address is still associated with a running instance, it is automatically disassociated from that instance.
Specify the removeFloatingIp
action in the request body.
Normal response codes: 202
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404), conflict(409)
Usage: osc compute server remove-floating-ip21 --address <ADDRESS> <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
--address <ADDRESS>
— The floating IP address
osc compute server remove-security-group
Removes a security group from a server.
Specify the removeSecurityGroup
action in the request body.
Normal response codes: 202
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404), conflict(409)
Usage: osc compute server remove-security-group [OPTIONS] --name <NAME> <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
--name <NAME>
— The security group name--property <key=value>
— Additional properties to be sent with the request
osc compute server rescue
Puts a server in rescue mode and changes its status to RESCUE
.
Specify the rescue
action in the request body.
If you specify the rescue_image_ref
extended attribute, the image is used to rescue the instance. If you omit an image reference, the base image reference is used by default.
Asynchronous Postconditions
After you successfully rescue a server and make a GET /servers/{server_id}
request, its status changes to RESCUE
.
Normal response codes: 200
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404), conflict(409), notImplemented(501)
Usage: osc compute server rescue [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
--admin-pass <ADMIN_PASS>
--rescue-image-ref <RESCUE_IMAGE_REF>
osc compute server reset-network
Resets networking on a server.
Specify the resetNetwork
action in the request body.
Policy defaults enable only users with the administrative role to perform this operation. Cloud providers can change these permissions through the policy.json
file.
Normal response codes: 202
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404), conflict(409), gone(410)
Usage: osc compute server reset-network <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
osc compute server resize
Resizes a server.
Specify the resize
action in the request body.
Preconditions
You can only resize a server when its status is ACTIVE
or SHUTOFF
.
If the server is locked, you must have administrator privileges to resize the server.
Asynchronous Postconditions
A successfully resized server shows a VERIFY_RESIZE
status and finished
migration status. If the cloud has configured the resize_confirm_window option of the Compute service to a positive value, the Compute service automatically confirms the resize operation after the configured interval.
Normal response codes: 202
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404), conflict(409)
Usage: osc compute server resize [OPTIONS] --flavor-ref <FLAVOR_REF> <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
-
--flavor-ref <FLAVOR_REF>
— The flavor ID for resizing the server. The size of the disk in the flavor being resized to must be greater than or equal to the size of the disk in the current flavor.If a specified flavor ID is the same as the current one of the server, the request returns a
Bad Request (400)
response code. -
--os-dcf-disk-config <OS_DCF_DISK_CONFIG>
— Controls how the API partitions the disk when you create, rebuild, or resize servers. A server inherits theOS-DCF:diskConfig
value from the image from which it was created, and an image inherits theOS-DCF:diskConfig
value from the server from which it was created. To override the inherited setting, you can include this attribute in the request body of a server create, rebuild, or resize request. If theOS-DCF:diskConfig
value for an image isMANUAL
, you cannot create a server from that image and set itsOS-DCF:diskConfig
value toAUTO
. A valid value is:AUTO
. The API builds the server with a single partition the size of the target flavor disk. The API automatically adjusts the file system to fit the entire partition. -MANUAL
. The API builds the server by using whatever partition scheme and file system is in the source image. If the target flavor disk is larger, the API does not partition the remaining disk space.
Possible values:
auto
,manual
osc compute server restore
Restores a previously soft-deleted server instance. You cannot use this method to restore deleted instances.
Specify the restore
action in the request body.
Policy defaults enable only users with the administrative role or the owner of the server to perform this operation. Cloud providers can change these permissions through the policy.json
file.
Normal response codes: 202
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404), conflict(409)
Usage: osc compute server restore --restore <JSON> <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
--restore <JSON>
osc compute server resume
Resumes a suspended server and changes its status to ACTIVE
.
Specify the resume
action in the request body.
Policy defaults enable only users with the administrative role or the owner of the server to perform this operation. Cloud providers can change these permissions through the policy.json
file.
Normal response codes: 202
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404), conflict(409)
Usage: osc compute server resume --resume <JSON> <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
--resume <JSON>
osc compute server revert-resize
Cancels and reverts a pending resize action for a server.
Specify the revertResize
action in the request body.
Preconditions
You can only revert the resized server where the status is VERIFY_RESIZE
and the OS-EXT-STS:vm_state is resized
.
If the server is locked, you must have administrator privileges to revert the resizing.
Asynchronous Postconditions
After you make this request, you typically must keep polling the server status to determine whether the request succeeded. A reverting resize operation shows a status of REVERT_RESIZE
and a task_state of resize_reverting
. If successful, the status will return to ACTIVE
or SHUTOFF
. You can also see the reverted server in the compute node that OpenStack Compute manages.
Troubleshooting
If the server status remains VERIFY_RESIZE
, the request failed. Ensure you meet the preconditions and run the request again. If the request fails again, investigate the compute back end.
The server is not reverted in the compute node that OpenStack Compute manages.
Normal response codes: 202
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404), conflict(409)
Usage: osc compute server revert-resize --revert-resize <JSON> <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
--revert-resize <JSON>
osc compute server security-groups
Lists security groups for a server.
Normal response codes: 200
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute server security-groups <SERVER_ID>
Arguments:
<SERVER_ID>
— server_id parameter for /v2.1/servers/{server_id}/os-security-groups API
osc compute server set21
Updates the editable attributes of an existing server.
Normal response codes: 200
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute server set21 [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id} API
Options:
-
--access-ipv4 <ACCESS_IPV4>
— IPv4 address that should be used to access this server -
--access-ipv6 <ACCESS_IPV6>
— IPv6 address that should be used to access this server -
--name <NAME>
— The server name -
--os-dcf-disk-config <OS_DCF_DISK_CONFIG>
— Controls how the API partitions the disk when you create, rebuild, or resize servers. A server inherits theOS-DCF:diskConfig
value from the image from which it was created, and an image inherits theOS-DCF:diskConfig
value from the server from which it was created. To override the inherited setting, you can include this attribute in the request body of a server create, rebuild, or resize request. If theOS-DCF:diskConfig
value for an image isMANUAL
, you cannot create a server from that image and set itsOS-DCF:diskConfig
value toAUTO
. A valid value is:AUTO
. The API builds the server with a single partition the size of the target flavor disk. The API automatically adjusts the file system to fit the entire partition. -MANUAL
. The API builds the server by using whatever partition scheme and file system is in the source image. If the target flavor disk is larger, the API does not partition the remaining disk space.
Possible values:
auto
,manual
osc compute server set219
Updates the editable attributes of an existing server.
Normal response codes: 200
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute server set219 [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id} API
Options:
-
--access-ipv4 <ACCESS_IPV4>
— IPv4 address that should be used to access this server -
--access-ipv6 <ACCESS_IPV6>
— IPv6 address that should be used to access this server -
--description <DESCRIPTION>
— A free form description of the server. Limited to 255 characters in length. Before microversion 2.19 this was set to the server name.New in version 2.19
-
--name <NAME>
— The server name -
--os-dcf-disk-config <OS_DCF_DISK_CONFIG>
— Controls how the API partitions the disk when you create, rebuild, or resize servers. A server inherits theOS-DCF:diskConfig
value from the image from which it was created, and an image inherits theOS-DCF:diskConfig
value from the server from which it was created. To override the inherited setting, you can include this attribute in the request body of a server create, rebuild, or resize request. If theOS-DCF:diskConfig
value for an image isMANUAL
, you cannot create a server from that image and set itsOS-DCF:diskConfig
value toAUTO
. A valid value is:AUTO
. The API builds the server with a single partition the size of the target flavor disk. The API automatically adjusts the file system to fit the entire partition. -MANUAL
. The API builds the server by using whatever partition scheme and file system is in the source image. If the target flavor disk is larger, the API does not partition the remaining disk space.
Possible values:
auto
,manual
osc compute server set290
Updates the editable attributes of an existing server.
Normal response codes: 200
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute server set290 [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id} API
Options:
-
--access-ipv4 <ACCESS_IPV4>
— IPv4 address that should be used to access this server -
--access-ipv6 <ACCESS_IPV6>
— IPv6 address that should be used to access this server -
--description <DESCRIPTION>
— A free form description of the server. Limited to 255 characters in length. Before microversion 2.19 this was set to the server name.New in version 2.19
-
--hostname <HOSTNAME>
— The hostname to configure for the instance in the metadata service.Starting with microversion 2.94, this can be a Fully Qualified Domain Name (FQDN) of up to 255 characters in length.
Note
This information is published via the metadata service and requires application such as
cloud-init
to propagate it through to the instance.New in version 2.90
-
--name <NAME>
— The server name -
--os-dcf-disk-config <OS_DCF_DISK_CONFIG>
— Controls how the API partitions the disk when you create, rebuild, or resize servers. A server inherits theOS-DCF:diskConfig
value from the image from which it was created, and an image inherits theOS-DCF:diskConfig
value from the server from which it was created. To override the inherited setting, you can include this attribute in the request body of a server create, rebuild, or resize request. If theOS-DCF:diskConfig
value for an image isMANUAL
, you cannot create a server from that image and set itsOS-DCF:diskConfig
value toAUTO
. A valid value is:AUTO
. The API builds the server with a single partition the size of the target flavor disk. The API automatically adjusts the file system to fit the entire partition. -MANUAL
. The API builds the server by using whatever partition scheme and file system is in the source image. If the target flavor disk is larger, the API does not partition the remaining disk space.
Possible values:
auto
,manual
osc compute server set294
Updates the editable attributes of an existing server.
Normal response codes: 200
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute server set294 [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id} API
Options:
-
--access-ipv4 <ACCESS_IPV4>
— IPv4 address that should be used to access this server -
--access-ipv6 <ACCESS_IPV6>
— IPv6 address that should be used to access this server -
--description <DESCRIPTION>
— A free form description of the server. Limited to 255 characters in length. Before microversion 2.19 this was set to the server name.New in version 2.19
-
--hostname <HOSTNAME>
— The hostname to configure for the instance in the metadata service.Starting with microversion 2.94, this can be a Fully Qualified Domain Name (FQDN) of up to 255 characters in length.
Note
This information is published via the metadata service and requires application such as
cloud-init
to propagate it through to the instance.New in version 2.90
-
--name <NAME>
— The server name -
--os-dcf-disk-config <OS_DCF_DISK_CONFIG>
— Controls how the API partitions the disk when you create, rebuild, or resize servers. A server inherits theOS-DCF:diskConfig
value from the image from which it was created, and an image inherits theOS-DCF:diskConfig
value from the server from which it was created. To override the inherited setting, you can include this attribute in the request body of a server create, rebuild, or resize request. If theOS-DCF:diskConfig
value for an image isMANUAL
, you cannot create a server from that image and set itsOS-DCF:diskConfig
value toAUTO
. A valid value is:AUTO
. The API builds the server with a single partition the size of the target flavor disk. The API automatically adjusts the file system to fit the entire partition. -MANUAL
. The API builds the server by using whatever partition scheme and file system is in the source image. If the target flavor disk is larger, the API does not partition the remaining disk space.
Possible values:
auto
,manual
osc compute server shelve
Shelves a server.
Specify the shelve
action in the request body.
All associated data and resources are kept but anything still in memory is not retained. To restore a shelved instance, use the unshelve
action. To remove a shelved instance, use the shelveOffload
action.
Policy defaults enable only users with the administrative role or the owner of the server to perform this operation. Cloud providers can change these permissions through the policy.json
file.
Preconditions
The server status must be ACTIVE
, SHUTOFF
, PAUSED
, or SUSPENDED
.
If the server is locked, you must have administrator privileges to shelve the server.
Asynchronous Postconditions
After you successfully shelve a server, its status changes to SHELVED
and the image status is ACTIVE
. The server instance data appears on the compute node that the Compute service manages.
If you boot the server from volumes or set the shelved_offload_time
option to 0, the Compute service automatically deletes the instance on compute nodes and changes the server status to SHELVED_OFFLOADED
.
Troubleshooting
If the server status does not change to SHELVED
or SHELVED_OFFLOADED
, the shelve operation failed. Ensure that you meet the preconditions and run the request again. If the request fails again, investigate whether another operation is running that causes a race condition.
Normal response codes: 202
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404), conflict(409)
Usage: osc compute server shelve --shelve <JSON> <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
--shelve <JSON>
osc compute server shelve-offload
Shelf-offloads, or removes, a shelved server.
Specify the shelveOffload
action in the request body.
Data and resource associations are deleted. If an instance is no longer needed, you can remove that instance from the hypervisor to minimize resource usage.
Policy defaults enable only users with the administrative role or the owner of the server to perform this operation. Cloud providers can change these permissions through the policy.json
file.
Preconditions
The server status must be SHELVED
.
If the server is locked, you must have administrator privileges to shelve-offload the server.
Asynchronous Postconditions
After you successfully shelve-offload a server, its status changes to SHELVED_OFFLOADED
. The server instance data appears on the compute node.
Troubleshooting
If the server status does not change to SHELVED_OFFLOADED
, the shelve-offload operation failed. Ensure that you meet the preconditions and run the request again. If the request fails again, investigate whether another operation is running that causes a race condition.
Normal response codes: 202
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404), conflict(409)
Usage: osc compute server shelve-offload --shelve-offload <JSON> <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
--shelve-offload <JSON>
osc compute server show
Shows details for a server.
Includes server details including configuration drive, extended status, and server usage information.
The extended status information appears in the OS-EXT-STS:vm_state
, OS-EXT-STS:power_state
, and OS-EXT-STS:task_state
attributes.
The server usage information appears in the OS-SRV-USG:launched_at
and OS-SRV-USG:terminated_at
attributes.
HostId is unique per account and is not globally unique.
Preconditions
The server must exist.
Normal response codes: 200
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute server show <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id} API
osc compute server start
Starts a stopped server and changes its status to ACTIVE
.
Specify the os-start
action in the request body.
Preconditions
The server status must be SHUTOFF
.
If the server is locked, you must have administrator privileges to start the server.
Asynchronous Postconditions
After you successfully start a server, its status changes to ACTIVE
.
Troubleshooting
If the server status does not change to ACTIVE
, the start operation failed. Ensure that you meet the preconditions and run the request again. If the request fails again, investigate whether another operation is running that causes a race condition.
Normal response codes: 202
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404), conflict(409)
Usage: osc compute server start --os-start <JSON> <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
--os-start <JSON>
osc compute server stop
Stops a running server and changes its status to SHUTOFF
.
Specify the os-stop
action in the request body.
Preconditions
The server status must be ACTIVE
or ERROR
.
If the server is locked, you must have administrator privileges to stop the server.
Asynchronous Postconditions
After you successfully stop a server, its status changes to SHUTOFF
. This API operation does not delete the server instance data and the data will be available again after os-start
action.
Normal response codes: 202
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404), conflict(409)
Usage: osc compute server stop --os-stop <JSON> <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
--os-stop <JSON>
osc compute server suspend
Suspends a server and changes its status to SUSPENDED
.
Specify the suspend
action in the request body.
Policy defaults enable only users with the administrative role or the owner of the server to perform this operation. Cloud providers can change these permissions through the policy.json
file.
Normal response codes: 202
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404), conflict(409)
Usage: osc compute server suspend --suspend <JSON> <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
--suspend <JSON>
osc compute server tag
Lists tags, creates, replaces or deletes one or more tags for a server, checks the existence of a tag for a server.
Available since version 2.26
Tags have the following restrictions:
-
Tag is a Unicode bytestring no longer than 60 characters.
-
Tag is a non-empty string.
-
‘/’ is not allowed to be in a tag name
-
Comma is not allowed to be in a tag name in order to simplify requests that specify lists of tags
-
All other characters are allowed to be in a tag name
-
Each server can have up to 50 tags.
Usage: osc compute server tag <COMMAND>
Available subcommands:
osc compute server tag add
— Add a Single Tagosc compute server tag check
— Check Tag Existenceosc compute server tag delete
— Delete a Single Tagosc compute server tag list
— List Tagsosc compute server tag purge
— Delete All Tagsosc compute server tag replace226
— Replace Tags (microversion = 2.26)
osc compute server tag add
Adds a single tag to the server if server has no specified tag. Response code in this case is 201.
If the server has specified tag just returns 204.
Normal response codes: 201, 204
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute server tag add <SERVER_ID> <ID>
Arguments:
<SERVER_ID>
— server_id parameter for /v2.1/servers/{server_id}/tags/{id} API<ID>
— id parameter for /v2.1/servers/{server_id}/tags/{id} API
osc compute server tag check
Checks tag existence on the server. If tag exists response with 204 status code will be returned. Otherwise returns 404.
Normal response codes: 204
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute server tag check <SERVER_ID> <ID>
Arguments:
<SERVER_ID>
— server_id parameter for /v2.1/servers/{server_id}/tags/{id} API<ID>
— id parameter for /v2.1/servers/{server_id}/tags/{id} API
osc compute server tag delete
Deletes a single tag from the specified server.
Normal response codes: 204
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute server tag delete <SERVER_ID> <ID>
Arguments:
<SERVER_ID>
— server_id parameter for /v2.1/servers/{server_id}/tags/{id} API<ID>
— id parameter for /v2.1/servers/{server_id}/tags/{id} API
osc compute server tag list
Lists all tags for a server.
Normal response codes: 200
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute server tag list <SERVER_ID>
Arguments:
<SERVER_ID>
— server_id parameter for /v2.1/servers/{server_id}/tags/{id} API
osc compute server tag purge
Deletes all tags from the specified server.
Normal response codes: 204
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute server tag purge <SERVER_ID>
Arguments:
<SERVER_ID>
— server_id parameter for /v2.1/servers/{server_id}/tags/{id} API
osc compute server tag replace226
Replaces all tags on specified server with the new set of tags.
Normal response codes: 200
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute server tag replace226 [OPTIONS] <SERVER_ID>
Arguments:
<SERVER_ID>
— server_id parameter for /v2.1/servers/{server_id}/tags/{id} API
Options:
-
--tags <TAGS>
— A list of tags. The maximum count of tags in this list is 50.Parameter is an array, may be provided multiple times.
osc compute server topology
Shows NUMA topology information for a server.
Policy defaults enable only users with the administrative role or the owners of the server to perform this operation. Cloud providers can change these permissions through the policy.json
file.
Normal response codes: 200
Error response codes: unauthorized(401), notfound(404), forbidden(403)
Usage: osc compute server topology <SERVER_ID>
Arguments:
<SERVER_ID>
— server_id parameter for /v2.1/servers/{server_id}/topology API
osc compute server trigger-crash-dump217
Command without description in OpenAPI
Usage: osc compute server trigger-crash-dump217 <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
osc compute server unlock21
Unlocks a locked server.
Specify the unlock
action in the request body.
Policy defaults enable only users with the administrative role or the owner of the server to perform this operation. Cloud providers can change these permissions through the policy.json
file.
Normal response codes: 202
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute server unlock21 --unlock <JSON> <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
--unlock <JSON>
osc compute server unpause
Unpauses a paused server and changes its status to ACTIVE
.
Specify the unpause
action in the request body.
Policy defaults enable only users with the administrative role or the owner of the server to perform this operation. Cloud providers can change these permissions through the policy.json
file.
Normal response codes: 202
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404), conflict(409), notImplemented(501)
Usage: osc compute server unpause --unpause <JSON> <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
--unpause <JSON>
osc compute server unrescue
Unrescues a server. Changes status to ACTIVE
.
Specify the unrescue
action in the request body.
Preconditions
The server must exist.
You can only unrescue a server when its status is RESCUE
.
Asynchronous Postconditions
After you successfully unrescue a server and make a GET /servers/{server_id}
request, its status changes to ACTIVE
.
Normal response codes: 202
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404), conflict(409), notImplemented(501)
Usage: osc compute server unrescue --unrescue <JSON> <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
--unrescue <JSON>
osc compute server unshelve277
Unshelve (Restore) Shelved Server (unshelve Action) (microversion = 2.77)
Usage: osc compute server unshelve277 --availability-zone <AVAILABILITY_ZONE> <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
--availability-zone <AVAILABILITY_ZONE>
osc compute server unshelve291
Unshelve (Restore) Shelved Server (unshelve Action) (microversion = 2.91)
Usage: osc compute server unshelve291 [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.1/servers/{id}/action API
Options:
--availability-zone <AVAILABILITY_ZONE>
--host <HOST>
osc compute server volume-attachment
Servers with volume attachments
Attaches volumes that are created through the volume API to server instances. Also, lists volume attachments for a server, shows details for a volume attachment, and detaches a volume.
Usage: osc compute server volume-attachment <COMMAND>
Available subcommands:
osc compute server volume-attachment create20
— Attach a volume to an instance (microversion = 2.0)osc compute server volume-attachment create249
— Attach a volume to an instance (microversion = 2.49)osc compute server volume-attachment create279
— Attach a volume to an instance (microversion = 2.79)osc compute server volume-attachment delete
— Detach a volume from an instanceosc compute server volume-attachment list
— List volume attachments for an instanceosc compute server volume-attachment set20
— Update a volume attachment (microversion = 2.0)osc compute server volume-attachment set285
— Update a volume attachment (microversion = 2.85)osc compute server volume-attachment show
— Show a detail of a volume attachment
osc compute server volume-attachment create20
Attach a volume to an instance.
Normal response codes: 200
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404), conflict(409)
Usage: osc compute server volume-attachment create20 [OPTIONS] --volume-id <VOLUME_ID> <SERVER_ID>
Arguments:
<SERVER_ID>
— server_id parameter for /v2.1/servers/{server_id}/os-volume_attachments/{id} API
Options:
--device <DEVICE>
— Name of the device such as,/dev/vdb
. Omit or set this parameter to null for auto-assignment, if supported. If you specify this parameter, the device must not exist in the guest operating system. Note that as of the 12.0.0 Liberty release, the Nova libvirt driver no longer honors a user-supplied device name. This is the same behavior as if the device name parameter is not supplied on the request--volume-id <VOLUME_ID>
— The UUID of the volume to attach
osc compute server volume-attachment create249
Attach a volume to an instance.
Normal response codes: 200
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404), conflict(409)
Usage: osc compute server volume-attachment create249 [OPTIONS] --volume-id <VOLUME_ID> <SERVER_ID>
Arguments:
<SERVER_ID>
— server_id parameter for /v2.1/servers/{server_id}/os-volume_attachments/{id} API
Options:
-
--device <DEVICE>
— Name of the device such as,/dev/vdb
. Omit or set this parameter to null for auto-assignment, if supported. If you specify this parameter, the device must not exist in the guest operating system. Note that as of the 12.0.0 Liberty release, the Nova libvirt driver no longer honors a user-supplied device name. This is the same behavior as if the device name parameter is not supplied on the request -
--tag <TAG>
— A device role tag that can be applied to a volume when attaching it to the VM. The guest OS of a server that has devices tagged in this manner can access hardware metadata about the tagged devices from the metadata API and on the config drive, if enabled.Note
Tagged volume attachment is not supported for shelved-offloaded instances.
New in version 2.49
-
--volume-id <VOLUME_ID>
— The UUID of the volume to attach
osc compute server volume-attachment create279
Attach a volume to an instance.
Normal response codes: 200
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404), conflict(409)
Usage: osc compute server volume-attachment create279 [OPTIONS] --volume-id <VOLUME_ID> <SERVER_ID>
Arguments:
<SERVER_ID>
— server_id parameter for /v2.1/servers/{server_id}/os-volume_attachments/{id} API
Options:
-
--delete-on-termination <DELETE_ON_TERMINATION>
— To delete the attached volume when the server is destroyed, specifytrue
. Otherwise, specifyfalse
. Default:false
New in version 2.79
Possible values:
true
,false
-
--device <DEVICE>
— Name of the device such as,/dev/vdb
. Omit or set this parameter to null for auto-assignment, if supported. If you specify this parameter, the device must not exist in the guest operating system. Note that as of the 12.0.0 Liberty release, the Nova libvirt driver no longer honors a user-supplied device name. This is the same behavior as if the device name parameter is not supplied on the request -
--tag <TAG>
— A device role tag that can be applied to a volume when attaching it to the VM. The guest OS of a server that has devices tagged in this manner can access hardware metadata about the tagged devices from the metadata API and on the config drive, if enabled.Note
Tagged volume attachment is not supported for shelved-offloaded instances.
New in version 2.49
-
--volume-id <VOLUME_ID>
— The UUID of the volume to attach
osc compute server volume-attachment delete
Detach a volume from an instance.
Normal response codes: 202
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404), conflict(409)
Usage: osc compute server volume-attachment delete <SERVER_ID> <ID>
Arguments:
<SERVER_ID>
— server_id parameter for /v2.1/servers/{server_id}/os-volume_attachments/{id} API<ID>
— id parameter for /v2.1/servers/{server_id}/os-volume_attachments/{id} API
osc compute server volume-attachment list
List volume attachments for an instance.
Normal response codes: 200
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute server volume-attachment list [OPTIONS] <SERVER_ID>
Arguments:
<SERVER_ID>
— server_id parameter for /v2.1/servers/{server_id}/os-volume_attachments/{id} API
Options:
-
--limit <LIMIT>
-
--offset <OFFSET>
-
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc compute server volume-attachment set20
Update a volume attachment.
Policy default role is ‘rule:system_admin_or_owner’, its scope is [system, project], which allow project members or system admins to change the fields of an attached volume of a server. Policy defaults enable only users with the administrative role to change volumeId
via this operation. Cloud providers can change these permissions through the policy.json
file.
Updating, or what is commonly referred to as “swapping”, volume attachments with volumes that have more than one read/write attachment, is not supported.
Normal response codes: 202
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404), conflict(409)
Usage: osc compute server volume-attachment set20 --volume-id <VOLUME_ID> <SERVER_ID> <ID>
Arguments:
<SERVER_ID>
— server_id parameter for /v2.1/servers/{server_id}/os-volume_attachments/{id} API<ID>
— id parameter for /v2.1/servers/{server_id}/os-volume_attachments/{id} API
Options:
--volume-id <VOLUME_ID>
— The UUID of the volume to attach instead of the attached volume
osc compute server volume-attachment set285
Update a volume attachment.
Policy default role is ‘rule:system_admin_or_owner’, its scope is [system, project], which allow project members or system admins to change the fields of an attached volume of a server. Policy defaults enable only users with the administrative role to change volumeId
via this operation. Cloud providers can change these permissions through the policy.json
file.
Updating, or what is commonly referred to as “swapping”, volume attachments with volumes that have more than one read/write attachment, is not supported.
Normal response codes: 202
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404), conflict(409)
Usage: osc compute server volume-attachment set285 [OPTIONS] --volume-id <VOLUME_ID> <SERVER_ID> <ID>
Arguments:
<SERVER_ID>
— server_id parameter for /v2.1/servers/{server_id}/os-volume_attachments/{id} API<ID>
— id parameter for /v2.1/servers/{server_id}/os-volume_attachments/{id} API
Options:
-
--delete-on-termination <DELETE_ON_TERMINATION>
— A flag indicating if the attached volume will be deleted when the server is deleted.New in version 2.85
Possible values:
true
,false
-
--device <DEVICE>
— Name of the device in the attachment object, such as,/dev/vdb
.New in version 2.85
-
--id <ID>
— The UUID of the attachment.New in version 2.85
-
--server-id <SERVER_ID>
— The UUID of the server.New in version 2.85
-
--tag <TAG>
— The device tag applied to the volume block device ornull
.New in version 2.85
-
--volume-id <VOLUME_ID>
— The UUID of the volume to attach instead of the attached volume
osc compute server volume-attachment show
Show a detail of a volume attachment.
Normal response codes: 200
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute server volume-attachment show <SERVER_ID> <ID>
Arguments:
<SERVER_ID>
— server_id parameter for /v2.1/servers/{server_id}/os-volume_attachments/{id} API<ID>
— id parameter for /v2.1/servers/{server_id}/os-volume_attachments/{id} API
osc compute server-external-event
Create external events (os-server-external-events)
Creates one or more external events. The API dispatches each event to a server instance.
Warning
This is an admin level service API only designed to be used by other OpenStack services. The point of this API is to coordinate between Nova and Neutron, Nova and Cinder, Nova and Ironic (and potentially future services) on activities they both need to be involved in, such as network hotplugging.
Unless you are writing Neutron, Cinder or Ironic code you should not be using this API.
Usage: osc compute server-external-event <COMMAND>
Available subcommands:
osc compute server-external-event create20
— Run Events (microversion = 2.0)osc compute server-external-event create251
— Run Events (microversion = 2.51)osc compute server-external-event create276
— Run Events (microversion = 2.76)osc compute server-external-event create282
— Run Events (microversion = 2.82)osc compute server-external-event create293
— Run Events (microversion = 2.93)
osc compute server-external-event create20
Creates one or more external events, which the API dispatches to the host a server is assigned to. If the server is not currently assigned to a host the event will not be delivered.
You will receive back the list of events that you submitted, with an updated code
and status
indicating their level of success.
Normal response codes: 200, 207
A 200 will be returned if all events succeeded, 207 will be returned if any events could not be processed. The code
attribute for the event will explain further what went wrong.
Error response codes: badRequest(400), unauthorized(401), forbidden(403)
Usage: osc compute server-external-event create20 [OPTIONS]
Options:
-
--events <JSON>
— List of external events to process.Parameter is an array, may be provided multiple times.
osc compute server-external-event create251
Creates one or more external events, which the API dispatches to the host a server is assigned to. If the server is not currently assigned to a host the event will not be delivered.
You will receive back the list of events that you submitted, with an updated code
and status
indicating their level of success.
Normal response codes: 200, 207
A 200 will be returned if all events succeeded, 207 will be returned if any events could not be processed. The code
attribute for the event will explain further what went wrong.
Error response codes: badRequest(400), unauthorized(401), forbidden(403)
Usage: osc compute server-external-event create251 [OPTIONS]
Options:
-
--events <JSON>
— List of external events to process.Parameter is an array, may be provided multiple times.
osc compute server-external-event create276
Creates one or more external events, which the API dispatches to the host a server is assigned to. If the server is not currently assigned to a host the event will not be delivered.
You will receive back the list of events that you submitted, with an updated code
and status
indicating their level of success.
Normal response codes: 200, 207
A 200 will be returned if all events succeeded, 207 will be returned if any events could not be processed. The code
attribute for the event will explain further what went wrong.
Error response codes: badRequest(400), unauthorized(401), forbidden(403)
Usage: osc compute server-external-event create276 [OPTIONS]
Options:
-
--events <JSON>
— List of external events to process.Parameter is an array, may be provided multiple times.
osc compute server-external-event create282
Creates one or more external events, which the API dispatches to the host a server is assigned to. If the server is not currently assigned to a host the event will not be delivered.
You will receive back the list of events that you submitted, with an updated code
and status
indicating their level of success.
Normal response codes: 200, 207
A 200 will be returned if all events succeeded, 207 will be returned if any events could not be processed. The code
attribute for the event will explain further what went wrong.
Error response codes: badRequest(400), unauthorized(401), forbidden(403)
Usage: osc compute server-external-event create282 [OPTIONS]
Options:
-
--events <JSON>
— List of external events to process.Parameter is an array, may be provided multiple times.
osc compute server-external-event create293
Creates one or more external events, which the API dispatches to the host a server is assigned to. If the server is not currently assigned to a host the event will not be delivered.
You will receive back the list of events that you submitted, with an updated code
and status
indicating their level of success.
Normal response codes: 200, 207
A 200 will be returned if all events succeeded, 207 will be returned if any events could not be processed. The code
attribute for the event will explain further what went wrong.
Error response codes: badRequest(400), unauthorized(401), forbidden(403)
Usage: osc compute server-external-event create293 [OPTIONS]
Options:
-
--events <JSON>
— List of external events to process.Parameter is an array, may be provided multiple times.
osc compute server-group
Server groups (os-server-groups)
Lists, shows information for, creates, and deletes server groups.
Usage: osc compute server-group <COMMAND>
Available subcommands:
osc compute server-group create264
— Create Server Group (microversion = 2.64)osc compute server-group create215
— Create Server Group (microversion = 2.15)osc compute server-group create20
— Create Server Group (microversion = 2.0)osc compute server-group delete
— Delete Server Grouposc compute server-group list
— List Server Groupsosc compute server-group show
— Show Server Group Details
osc compute server-group create264
Creates a server group.
Normal response codes: 200
Error response codes: badRequest(400), unauthorized(401), forbidden(403), conflict(409)
Usage: osc compute server-group create264 [OPTIONS] --name <NAME> --policy <POLICY>
Options:
-
--name <NAME>
— The name of the server group -
--policy <POLICY>
— Thepolicy
field represents the name of the policy. The current valid policy names are:anti-affinity
- servers in this group must be scheduled to different hosts. -affinity
- servers in this group must be scheduled to the same host. -soft-anti-affinity
- servers in this group should be scheduled to different hosts if possible, but if not possible then they should still be scheduled instead of resulting in a build failure. -soft-affinity
- servers in this group should be scheduled to the same host if possible, but if not possible then they should still be scheduled instead of resulting in a build failure.
New in version 2.64
Possible values:
affinity
,anti-affinity
,soft-affinity
,soft-anti-affinity
-
--max-server-per-host <MAX_SERVER_PER_HOST>
osc compute server-group create215
Creates a server group.
Normal response codes: 200
Error response codes: badRequest(400), unauthorized(401), forbidden(403), conflict(409)
Usage: osc compute server-group create215 [OPTIONS] --name <NAME>
Options:
-
--name <NAME>
— The name of the server group -
--policies <POLICIES>
— A list of exactly one policy name to associate with the server group. The current valid policy names are:anti-affinity
- servers in this group must be scheduled to different hosts. -affinity
- servers in this group must be scheduled to the same host. -soft-anti-affinity
- servers in this group should be scheduled to different hosts if possible, but if not possible then they should still be scheduled instead of resulting in a build failure. This policy was added in microversion 2.15. -soft-affinity
- servers in this group should be scheduled to the same host if possible, but if not possible then they should still be scheduled instead of resulting in a build failure. This policy was added in microversion 2.15.
Available until version 2.63
Parameter is an array, may be provided multiple times.
osc compute server-group create20
Creates a server group.
Normal response codes: 200
Error response codes: badRequest(400), unauthorized(401), forbidden(403), conflict(409)
Usage: osc compute server-group create20 [OPTIONS] --name <NAME>
Options:
-
--name <NAME>
— The name of the server group -
--policies <POLICIES>
— A list of exactly one policy name to associate with the server group. The current valid policy names are:anti-affinity
- servers in this group must be scheduled to different hosts. -affinity
- servers in this group must be scheduled to the same host. -soft-anti-affinity
- servers in this group should be scheduled to different hosts if possible, but if not possible then they should still be scheduled instead of resulting in a build failure. This policy was added in microversion 2.15. -soft-affinity
- servers in this group should be scheduled to the same host if possible, but if not possible then they should still be scheduled instead of resulting in a build failure. This policy was added in microversion 2.15.
Available until version 2.63
Parameter is an array, may be provided multiple times.
osc compute server-group delete
Deletes a server group.
Normal response codes: 204
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute server-group delete <ID>
Arguments:
<ID>
— id parameter for /v2.1/os-server-groups/{id} API
osc compute server-group list
Lists all server groups for the tenant.
Administrative users can use the all_projects
query parameter to list all server groups for all projects.
Normal response codes: 200
Error response codes: unauthorized(401), forbidden(403)
Usage: osc compute server-group list [OPTIONS]
Options:
-
--all-projects <ALL_PROJECTS>
-
--limit <LIMIT>
-
--offset <OFFSET>
-
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc compute server-group show
Shows details for a server group.
Normal response codes: 200
Error response codes: unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute server-group show <ID>
Arguments:
<ID>
— id parameter for /v2.1/os-server-groups/{id} API
osc compute service
Server groups (os-server-groups)
Lists, shows information for, creates, and deletes server groups.
Usage: osc compute service <COMMAND>
Available subcommands:
osc compute service delete
— Delete Compute Serviceosc compute service list
— List Compute Servicesosc compute service set253
— Update Compute Service (microversion = 2.53)osc compute service set211
— Update Compute Service (microversion = 2.11)osc compute service set20
— Update Compute Service (microversion = 2.0)
osc compute service delete
Deletes a service. If it’s a nova-compute
service, then the corresponding host will be removed from all the host aggregates as well.
Attempts to delete a nova-compute
service which is still hosting instances will result in a 409 HTTPConflict response. The instances will need to be migrated or deleted before a compute service can be deleted.
Similarly, attempts to delete a nova-compute
service which is involved in in-progress migrations will result in a 409 HTTPConflict response. The migrations will need to be completed, for example confirming or reverting a resize, or the instances will need to be deleted before the compute service can be deleted.
Normal response codes: 204
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404), conflict(409)
Usage: osc compute service delete <ID>
Arguments:
<ID>
— id parameter for /v2.1/os-services/{id} API
osc compute service list
Lists all running Compute services.
Provides details why any services were disabled.
Normal response codes: 200
Error response codes: unauthorized(401), forbidden(403)
Usage: osc compute service list [OPTIONS]
Options:
--binary <BINARY>
--host <HOST>
osc compute service set253
Update a compute service to enable or disable scheduling, including recording a reason why a compute service was disabled from scheduling. Set or unset the forced_down
flag for the service. This operation is only allowed on services whose binary
is nova-compute
.
This API is available starting with microversion 2.53.
Normal response codes: 200
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute service set253 [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.1/os-services/{id} API
Options:
-
--disabled-reason <DISABLED_REASON>
— The reason for disabling a service. The minimum length is 1 and the maximum length is 255. This may only be requested withstatus=disabled
-
--forced-down <FORCED_DOWN>
—forced_down
is a manual override to tell nova that the service in question has been fenced manually by the operations team (either hard powered off, or network unplugged). That signals that it is safe to proceed withevacuate
or other operations that nova has safety checks to prevent for hosts that are up.Warning
Setting a service forced down without completely fencing it will likely result in the corruption of VMs on that host.
Possible values:
true
,false
-
--status <STATUS>
— The status of the service. One ofenabled
ordisabled
Possible values:
disabled
,enabled
osc compute service set211
Update a compute service to enable or disable scheduling, including recording a reason why a compute service was disabled from scheduling. Set or unset the forced_down
flag for the service. This operation is only allowed on services whose binary
is nova-compute
.
This API is available starting with microversion 2.53.
Normal response codes: 200
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute service set211 [OPTIONS] --binary <BINARY> --host <HOST> <ID>
Arguments:
<ID>
— id parameter for /v2.1/os-services/{id} API
Options:
-
--binary <BINARY>
-
--disabled-reason <DISABLED_REASON>
— The reason for disabling a service. The minimum length is 1 and the maximum length is 255. This may only be requested withstatus=disabled
-
--forced-down <FORCED_DOWN>
—forced_down
is a manual override to tell nova that the service in question has been fenced manually by the operations team (either hard powered off, or network unplugged). That signals that it is safe to proceed withevacuate
or other operations that nova has safety checks to prevent for hosts that are up.Warning
Setting a service forced down without completely fencing it will likely result in the corruption of VMs on that host.
Possible values:
true
,false
-
--host <HOST>
osc compute service set20
Update a compute service to enable or disable scheduling, including recording a reason why a compute service was disabled from scheduling. Set or unset the forced_down
flag for the service. This operation is only allowed on services whose binary
is nova-compute
.
This API is available starting with microversion 2.53.
Normal response codes: 200
Error response codes: badRequest(400), unauthorized(401), forbidden(403), itemNotFound(404)
Usage: osc compute service set20 [OPTIONS] --binary <BINARY> --host <HOST> <ID>
Arguments:
<ID>
— id parameter for /v2.1/os-services/{id} API
Options:
--binary <BINARY>
--disabled-reason <DISABLED_REASON>
— The reason for disabling a service. The minimum length is 1 and the maximum length is 255. This may only be requested withstatus=disabled
--host <HOST>
osc compute simple-tenant-usage
Usage reports (os-simple-tenant-usage)
Reports usage statistics of compute and storage resources periodically for an individual tenant or all tenants. The usage statistics will include all instances’ CPU, memory and local disk during a specific period.
Warning
The os-simple-tenant-usage will report usage statistics based on the latest flavor that is configured in the virtual machine (VM), and ignoring stop, pause, and other events that might have happened with the VM. Therefore, it uses the time the VM existed in the cloud environment to execute the usage accounting.
More information can be found at http://eavesdrop.openstack.org/meetings/nova/2020/nova.2020-12-03-16.00.log.txt, and https://review.opendev.org/c/openstack/nova/+/711113
Microversion 2.40 added pagination (and next links) to the usage statistics via optional limit and marker query parameters. If limit isn’t provided, the configurable max_limit will be used which currently defaults to 1000. Older microversions will not accept these new paging query parameters, but they will start to silently limit by max_limit.
text /os-simple-tenant-usage?limit={limit}&marker={instance_uuid} /os-simple-tenant-usage/{tenant_id}?limit={limit}&marker={instance_uuid}
Note
A tenant’s usage statistics may span multiple pages when the number of instances exceeds limit, and API consumers will need to stitch together the aggregate results if they still want totals for all instances in a specific time window, grouped by tenant.
Usage: osc compute simple-tenant-usage <COMMAND>
Available subcommands:
osc compute simple-tenant-usage list
— List Tenant Usage Statistics For All Tenantsosc compute simple-tenant-usage show
— Show Usage Statistics For Tenant
osc compute simple-tenant-usage list
Lists usage statistics for all tenants.
Normal response codes: 200
Error response codes: badRequest(400), unauthorized(401), forbidden(403)
Usage: osc compute simple-tenant-usage list [OPTIONS]
Options:
-
--detailed <DETAILED>
-
--end <END>
-
--limit <LIMIT>
-
--marker <MARKER>
-
--start <START>
-
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc compute simple-tenant-usage show
Shows usage statistics for a tenant.
Normal response codes: 200
Error response codes: badRequest(400), unauthorized(401), forbidden(403)
Usage: osc compute simple-tenant-usage show [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.1/os-simple-tenant-usage/{id} API
Options:
--end <END>
--limit <LIMIT>
--marker <MARKER>
--start <START>
osc container-infrastructure
Container Infra service (Magnum) operations
Usage: osc container-infrastructure <COMMAND>
Available subcommands:
osc container-infrastructure certificate
— Manage certificates for clusterosc container-infrastructure cluster
— Manage Clusterosc container-infrastructure clustertemplate
— Manage Cluster Templatesosc container-infrastructure federation
— Manage Federationosc container-infrastructure service
— Magnum Stats APIosc container-infrastructure quota
— Magnum Quota APIosc container-infrastructure stat
— Magnum Stats APIosc container-infrastructure version
— Manage Version
osc container-infrastructure certificate
Manage certificates for cluster
Generates and show CA certificates for cluster.
Usage: osc container-infrastructure certificate <COMMAND>
Available subcommands:
osc container-infrastructure certificate create
— Sign a new certificate by the CAosc container-infrastructure certificate show
— Show details about the CA certificate for a cluster
osc container-infrastructure certificate create
Sign a new certificate by the CA.
| param certificate: | | | --- | --- | | | a certificate within the request body. |
Usage: osc container-infrastructure certificate create [OPTIONS]
Options:
--ca-cert-type <CA_CERT_TYPE>
--cluster-uuid <CLUSTER_UUID>
--created-at <CREATED_AT>
--csr <CSR>
--links <JSON>
— Parameter is an array, may be provided multiple times--pem <PEM>
--updated-at <UPDATED_AT>
osc container-infrastructure certificate show
Show details about the CA certificate for a cluster
Usage: osc container-infrastructure certificate show <ID>
Arguments:
<ID>
— certificate_id parameter for /v1/certificates/{certificate_id} API
osc container-infrastructure cluster
Manage Cluster
Lists, creates, shows details for, updates, and deletes Cluster.
Usage: osc container-infrastructure cluster <COMMAND>
Available subcommands:
osc container-infrastructure cluster create
— Create new clusterosc container-infrastructure cluster delete
— Delete a clusterosc container-infrastructure cluster list
— List all clustersosc container-infrastructure cluster nodegroup
— Manage Nodegrouposc container-infrastructure cluster show
— Show details of a cluster
osc container-infrastructure cluster create
Create new cluster
Usage: osc container-infrastructure cluster create [OPTIONS] --cluster-template-id <CLUSTER_TEMPLATE_ID>
Options:
-
--api-address <API_ADDRESS>
-
--cluster-template-id <CLUSTER_TEMPLATE_ID>
— The UUID of the cluster template -
--coe-version <COE_VERSION>
-
--container-version <CONTAINER_VERSION>
-
--create-timeout <CREATE_TIMEOUT>
— The timeout for cluster creation in minutes. The value expected is a positive integer and the default is 60 minutes. If the timeout is reached during cluster creation process, the operation will be aborted and the cluster status will be set toCREATE_FAILED
-
--created-at <CREATED_AT>
-
--discovery-url <DISCOVERY_URL>
— The custom discovery url for node discovery. This is used by the COE to discover the servers that have been created to host the containers. The actual discovery mechanism varies with the COE. In some cases, Magnum fills in the server info in the discovery service. In other cases, if thediscovery_url
is not specified, Magnum will use the public discovery service at:In this case, Magnum will generate a unique url here for each uster and store the info for the servers.
-
--docker-volume-size <DOCKER_VOLUME_SIZE>
-
--faults <key=value>
-
--fixed-network <FIXED_NETWORK>
— The name or network ID of a Neutron network to provide connectivity to the internal network for the cluster -
--fixed-subnet <FIXED_SUBNET>
— Fixed subnet that are using to allocate network address for nodes in cluster -
--flavor-id <FLAVOR_ID>
— The nova flavor ID or name for booting the node servers. The default ism1.small
-
--floating-ip-enabled <FLOATING_IP_ENABLED>
— Whether enable or not using the floating IP of cloud provider. Some cloud providers used floating IP, some used public IP, thus Magnum provide this option for specifying the choice of using floating IP. If it’s not set, the value of floating_ip_enabled in template will be used -
--health-status <HEALTH_STATUS>
Possible values:
healthy
,unhealthy
,unknown
-
--health-status-reason <key=value>
-
--keypair <KEYPAIR>
— The name of the SSH keypair to configure in the cluster servers for ssh access. Users will need the key to be able to ssh to the servers in the cluster. The login name is specific to the cluster driver, for example with fedora-atomic image, default login name isfedora
-
--labels <key=value>
— Arbitrary labels in the form ofkey=value
pairs. The accepted keys and valid values are defined in the cluster drivers. They are used as a way to pass additional parameters that are specific to a cluster driver -
--labels-added <key=value>
-
--labels-overridden <key=value>
-
--labels-skipped <key=value>
-
--links <JSON>
— Parameter is an array, may be provided multiple times -
--master-addresses <MASTER_ADDRESSES>
— Parameter is an array, may be provided multiple times -
--master-count <MASTER_COUNT>
— The number of servers that will serve as master for the cluster. The default is 1. Set to more than 1 master to enable High Availability. If the optionmaster-lb-enabled
is specified in the cluster template, the master servers will be placed in a load balancer pool -
--master-flavor-id <MASTER_FLAVOR_ID>
— The flavor of the master node for this cluster template -
--master-lb-enabled <MASTER_LB_ENABLED>
— Since multiple masters may exist in a cluster, a Neutron load balancer is created to provide the API endpoint for the cluster and to direct requests to the masters. In some cases, such as when the LBaaS service is not available, this option can be set tofalse
to create a cluster without the load balancer. In this case, one of the masters will serve as the API endpoint. The default istrue
, i.e. to create the load balancer for the cluster -
--merge-labels <MERGE_LABELS>
-
--name <NAME>
— Name of the resource -
--node-addresses <NODE_ADDRESSES>
— Parameter is an array, may be provided multiple times -
--node-count <NODE_COUNT>
— The number of servers that will serve as node in the cluster. The default is 1 -
--project-id <PROJECT_ID>
-
--stack-id <STACK_ID>
-
--status <STATUS>
Possible values:
adopt-complete
,check-complete
,create-complete
,create-failed
,create-in-progress
,delete-complete
,delete-failed
,delete-in-progress
,restore-complete
,resume-complete
,resume-failed
,rollback-complete
,rollback-failed
,rollback-in-progress
,snapshot-complete
,update-complete
,update-failed
,update-in-progress
-
--status-reason <STATUS_REASON>
-
--updated-at <UPDATED_AT>
-
--user-id <USER_ID>
-
--uuid <UUID>
osc container-infrastructure cluster delete
Delete a cluster
Usage: osc container-infrastructure cluster delete <ID>
Arguments:
<ID>
— cluster_id parameter for /v1/clusters/{cluster_id} API
osc container-infrastructure cluster list
List all clusters
Usage: osc container-infrastructure cluster list
osc container-infrastructure cluster nodegroup
Manage Nodegroup
Lists, creates, shows details for, updates, and deletes Nodegroup.
Usage: osc container-infrastructure cluster nodegroup <COMMAND>
Available subcommands:
osc container-infrastructure cluster nodegroup delete
— Delete NodeGroup for a given project_id and resourceosc container-infrastructure cluster nodegroup list
— Retrieve a list of nodegroupsosc container-infrastructure cluster nodegroup purge
— Delete NodeGroup for a given project_id and resourceosc container-infrastructure cluster nodegroup show
— Retrieve information for the given nodegroup in a cluster
osc container-infrastructure cluster nodegroup delete
Delete NodeGroup for a given project_id and resource.
| param cluster_id: | | | --- | --- | | | cluster id. | | param nodegroup_id: | | | | resource name. |
Usage: osc container-infrastructure cluster nodegroup delete <ID>
Arguments:
<ID>
— nodegroup_id parameter for /v1/clusters/nodegroups/{nodegroup_id} API
osc container-infrastructure cluster nodegroup list
Retrieve a list of nodegroups.
| param cluster_id: | | | --- | --- | | | the cluster id or name | | param marker: | pagination marker for large data sets. | | param limit: | maximum number of resources to return in a single result. | | param sort_key: | column to sort results by. Default: id. | | param sort_dir: | direction to sort. "asc" or "desc". Default: asc. | | param role: | list all nodegroups with the specified role. |
Usage: osc container-infrastructure cluster nodegroup list
osc container-infrastructure cluster nodegroup purge
Delete NodeGroup for a given project_id and resource.
| param cluster_id: | | | --- | --- | | | cluster id. | | param nodegroup_id: | | | | resource name. |
Usage: osc container-infrastructure cluster nodegroup purge
osc container-infrastructure cluster nodegroup show
Retrieve information for the given nodegroup in a cluster.
| | | | --- | --- | | param id: | cluster id. | | param resource: | nodegroup id. |
Usage: osc container-infrastructure cluster nodegroup show <ID>
Arguments:
<ID>
— nodegroup_id parameter for /v1/clusters/nodegroups/{nodegroup_id} API
osc container-infrastructure cluster show
Show details of a cluster
Usage: osc container-infrastructure cluster show <ID>
Arguments:
<ID>
— cluster_id parameter for /v1/clusters/{cluster_id} API
osc container-infrastructure clustertemplate
Manage Cluster Templates
Lists, creates, shows details for, updates, and deletes Cluster Templates.
Usage: osc container-infrastructure clustertemplate <COMMAND>
Available subcommands:
osc container-infrastructure clustertemplate create
— Create new cluster templateosc container-infrastructure clustertemplate delete
— Delete a cluster templateosc container-infrastructure clustertemplate list
— List all cluster templatesosc container-infrastructure clustertemplate show
— Show details of a cluster template
osc container-infrastructure clustertemplate create
Create new cluster template
Usage: osc container-infrastructure clustertemplate create [OPTIONS] --image-id <IMAGE_ID>
Options:
-
--apiserver-port <APISERVER_PORT>
-
--cluster-distro <CLUSTER_DISTRO>
-
--coe <COE>
— Specify the Container Orchestration Engine to use. Supported COEs includekubernetes
. If your environment has additional cluster drivers installed, refer to the cluster driver documentation for the new COE namesPossible values:
kubernetes
-
--created-at <CREATED_AT>
-
--dns-nameserver <DNS_NAMESERVER>
— The DNS nameserver for the servers and containers in the cluster to use. This is configured in the private Neutron network for the cluster. The default is8.8.8.8
-
--docker-storage-driver <DOCKER_STORAGE_DRIVER>
— The name of a driver to manage the storage for the images and the container’s writable layer. The default isdevicemapper
-
--docker-volume-size <DOCKER_VOLUME_SIZE>
— The size in GB for the local storage on each server for the Docker daemon to cache the images and host the containers. Cinder volumes provide the storage. The default is 25 GB. For thedevicemapper
storage driver, the minimum value is 3GB. For theoverlay
storage driver, the minimum value is 1GB -
--driver <DRIVER>
-
--external-network-id <EXTERNAL_NETWORK_ID>
— The name or network ID of a Neutron network to provide connectivity to the external internet for the cluster. This network must be an external network, i.e. its attributerouter:external
must beTrue
. The servers in the cluster will be connected to a private network and Magnum will create a router between this private network and the external network. This will allow the servers to download images, access discovery service, etc, and the containers to install packages, etc. In the opposite direction, floating IPs will be allocated from the external network to provide access from the external internet to servers and the container services hosted in the cluster -
--fixed-network <FIXED_NETWORK>
— The name or network ID of a Neutron network to provide connectivity to the internal network for the cluster -
--fixed-subnet <FIXED_SUBNET>
— Fixed subnet that are using to allocate network address for nodes in cluster -
--flavor-id <FLAVOR_ID>
— The nova flavor ID or name for booting the node servers. The default ism1.small
-
--floating-ip-enabled <FLOATING_IP_ENABLED>
— Whether enable or not using the floating IP of cloud provider. Some cloud providers used floating IP, some used public IP, thus Magnum provide this option for specifying the choice of using floating IP -
--hidden <HIDDEN>
— Indicates whether the ClusterTemplate is hidden or not, the default value is false -
--http-proxy <HTTP_PROXY>
— The IP address for a proxy to use when direct http access from the servers to sites on the external internet is blocked. This may happen in certain countries or enterprises, and the proxy allows the servers and containers to access these sites. The format is a URL including a port number. The default isNone
-
--https-proxy <HTTPS_PROXY>
— The IP address for a proxy to use when direct https access from the servers to sites on the external internet is blocked. This may happen in certain countries or enterprises, and the proxy allows the servers and containers to access these sites. The format is a URL including a port number. The default isNone
-
--image-id <IMAGE_ID>
— The name or UUID of the base image in Glance to boot the servers for the cluster. The image must have the attributeos_distro
defined as appropriate for the cluster driver -
--insecure-registry <INSECURE_REGISTRY>
-
--keypair-id <KEYPAIR_ID>
— The name of the SSH keypair to configure in the cluster servers for ssh access. Users will need the key to be able to ssh to the servers in the cluster. The login name is specific to the cluster driver, for example with fedora-atomic image, default login name isfedora
-
--labels <key=value>
— Arbitrary labels in the form ofkey=value
pairs. The accepted keys and valid values are defined in the cluster drivers. They are used as a way to pass additional parameters that are specific to a cluster driver -
--links <JSON>
— Parameter is an array, may be provided multiple times -
--master-flavor-id <MASTER_FLAVOR_ID>
— The flavor of the master node for this cluster template -
--master-lb-enabled <MASTER_LB_ENABLED>
— Since multiple masters may exist in a cluster, a Neutron load balancer is created to provide the API endpoint for the cluster and to direct requests to the masters. In some cases, such as when the LBaaS service is not available, this option can be set tofalse
to create a cluster without the load balancer. In this case, one of the masters will serve as the API endpoint. The default istrue
, i.e. to create the load balancer for the cluster -
--name <NAME>
— Name of the resource -
--network-driver <NETWORK_DRIVER>
— The name of a network driver for providing the networks for the containers. Note that this is different and separate from the Neutron network for the cluster. The operation and networking model are specific to the particular driver -
--no-proxy <NO_PROXY>
— When a proxy server is used, some sites should not go through the proxy and should be accessed normally. In this case, users can specify these sites as a comma separated list of IPs. The default isNone
-
--project-id <PROJECT_ID>
-
--public <PUBLIC>
— Access to a cluster template is normally limited to the admin, owner or users within the same tenant as the owners. Setting this flag makes the cluster template public and accessible by other users. The default is not public -
--registry-enabled <REGISTRY_ENABLED>
— Docker images by default are pulled from the public Docker registry, but in some cases, users may want to use a private registry. This option provides an alternative registry based on the Registry V2: Magnum will create a local registry in the cluster backed by swift to host the images. The default is to use the public registry -
--server-type <SERVER_TYPE>
— The servers in the cluster can bevm
orbaremetal
. This parameter selects the type of server to create for the cluster. The default isvm
Possible values:
bm
,vm
-
--tags <TAGS>
— Administrator tags for the cluster template -
--tls-disabled <TLS_DISABLED>
— Transport Layer Security (TLS) is normally enabled to secure the cluster. In some cases, users may want to disable TLS in the cluster, for instance during development or to troubleshoot certain problems. Specifying this parameter will disable TLS so that users can access the COE endpoints without a certificate. The default is TLS enabled -
--updated-at <UPDATED_AT>
-
--user-id <USER_ID>
-
--uuid <UUID>
-
--volume-driver <VOLUME_DRIVER>
— The name of a volume driver for managing the persistent storage for the containers. The functionality supported are specific to the driver
osc container-infrastructure clustertemplate delete
Delete a cluster template
Usage: osc container-infrastructure clustertemplate delete <ID>
Arguments:
<ID>
— clustertemplate_id parameter for /v1/clustertemplates/{clustertemplate_id} API
osc container-infrastructure clustertemplate list
List all cluster templates
Usage: osc container-infrastructure clustertemplate list
osc container-infrastructure clustertemplate show
Show details of a cluster template
Usage: osc container-infrastructure clustertemplate show <ID>
Arguments:
<ID>
— clustertemplate_id parameter for /v1/clustertemplates/{clustertemplate_id} API
osc container-infrastructure federation
Manage Federation
Usage: osc container-infrastructure federation <COMMAND>
Available subcommands:
osc container-infrastructure federation create
— Create a new federationosc container-infrastructure federation delete
— Delete a federationosc container-infrastructure federation list
— Retrieve a list of federationsosc container-infrastructure federation show
— Retrieve information about a given Federation
osc container-infrastructure federation create
Create a new federation.
| param federation: | | | --- | --- | | | a federation within the request body. |
Usage: osc container-infrastructure federation create [OPTIONS]
Options:
-
--created-at <CREATED_AT>
-
--hostcluster-id <HOSTCLUSTER_ID>
-
--links <JSON>
— Parameter is an array, may be provided multiple times -
--member-ids <MEMBER_IDS>
— Parameter is an array, may be provided multiple times -
--name <NAME>
-
--properties <key=value>
-
--status <STATUS>
Possible values:
create-complete
,create-failed
,create-in-progress
,delete-complete
,delete-failed
,delete-in-progress
,update-complete
,update-failed
,update-in-progress
-
--status-reason <STATUS_REASON>
-
--updated-at <UPDATED_AT>
-
--uuid <UUID>
osc container-infrastructure federation delete
Delete a federation.
| param federation_ident: | | | --- | --- | | | UUID of federation or logical name of the federation. |
Usage: osc container-infrastructure federation delete <ID>
Arguments:
<ID>
— federation_id parameter for /v1/federations/{federation_id} API
osc container-infrastructure federation list
Retrieve a list of federations.
| | | | --- | --- | | param marker: | pagination marker for large data sets. | | param limit: | maximum number of resources to return in a single result. | | param sort_key: | column to sort results by. Default: id. | | param sort_dir: | direction to sort. "asc" or "desc". Default: asc. |
Usage: osc container-infrastructure federation list
osc container-infrastructure federation show
Retrieve information about a given Federation.
| param federation_ident: | | | --- | --- | | | UUID or logical name of the Federation. |
Usage: osc container-infrastructure federation show <ID>
Arguments:
<ID>
— federation_id parameter for /v1/federations/{federation_id} API
osc container-infrastructure service
Magnum Stats API
An admin user can get stats for the given tenant and also overall system stats. A non-admin user can get self stats.
Usage: osc container-infrastructure service <COMMAND>
Available subcommands:
osc container-infrastructure service list
— List container infrastructure management services
osc container-infrastructure service list
Enables administrative users to list all Magnum services.
Container infrastructure service information include service id, binary, host, report count, creation time, last updated time, health status, and the reason for disabling service.
Usage: osc container-infrastructure service list
osc container-infrastructure quota
Magnum Quota API
Lists, creates, shows details, and updates Quotas.
Usage: osc container-infrastructure quota <COMMAND>
Available subcommands:
osc container-infrastructure quota create
— Set new quotaosc container-infrastructure quota delete
— Delete Quota for a given project_id and resourceosc container-infrastructure quota list
— List all quotasosc container-infrastructure quota show
— Retrieve Quota information for the given project_id
osc container-infrastructure quota create
Set new quota
Usage: osc container-infrastructure quota create [OPTIONS]
Options:
-
--created-at <CREATED_AT>
-
--hard-limit <HARD_LIMIT>
-
--id <ID>
-
--project-id <PROJECT_ID>
-
--resource <RESOURCE>
Possible values:
cluster
-
--updated-at <UPDATED_AT>
osc container-infrastructure quota delete
Delete Quota for a given project_id and resource.
| param project_id: | | | --- | --- | | | project id. | | param resource: | resource name. |
Usage: osc container-infrastructure quota delete <ID>
Arguments:
<ID>
— quota_id parameter for /v1/quotas/{quota_id} API
osc container-infrastructure quota list
List all quotas
Usage: osc container-infrastructure quota list
osc container-infrastructure quota show
Retrieve Quota information for the given project_id.
| | | | --- | --- | | param id: | project id. | | param resource: | resource name. |
Usage: osc container-infrastructure quota show <ID>
Arguments:
<ID>
— quota_id parameter for /v1/quotas/{quota_id} API
osc container-infrastructure stat
Magnum Stats API
An admin user can get stats for the given tenant and also overall system stats. A non-admin user can get self stats.
Usage: osc container-infrastructure stat <COMMAND>
Available subcommands:
osc container-infrastructure stat show
— Show overall stats
osc container-infrastructure stat show
Show overall stats
Usage: osc container-infrastructure stat show
osc container-infrastructure version
Manage Version
Usage: osc container-infrastructure version <COMMAND>
Available subcommands:
osc container-infrastructure version show
— Command without description in OpenAPI
osc container-infrastructure version show
Command without description in OpenAPI
Usage: osc container-infrastructure version show
osc dns
DNS service (Designate) operations
Usage: osc dns <COMMAND>
Available subcommands:
osc dns limit
— DNS Project limits operationsosc dns quota
— DNS Quota operationsosc dns recordset
— DNS Project recordsets operationsosc dns reverse
— DNS Reverse recordsets (PTR) operationsosc dns zone
— DNS Zone operations
osc dns limit
DNS Project limits operations
Usage: osc dns limit <COMMAND>
Available subcommands:
osc dns limit get
— Get Project Limits
osc dns limit get
Get Project Limits
Usage: osc dns limit get
osc dns quota
DNS Quota operations
Usage: osc dns quota <COMMAND>
Available subcommands:
osc dns quota delete
— Reset Quotasosc dns quota show
— View Quotasosc dns quota set
— Set Quotas
osc dns quota delete
Reset Quotas
Usage: osc dns quota delete <--project-name <PROJECT_NAME>|--project-id <PROJECT_ID>|--current-project>
Options:
--project-name <PROJECT_NAME>
— Project Name--project-id <PROJECT_ID>
— Project ID--current-project
— Current project
osc dns quota show
View a projects quotas
This returns a key:value set of quotas on the system.
Usage: osc dns quota show <--project-name <PROJECT_NAME>|--project-id <PROJECT_ID>|--current-project>
Options:
--project-name <PROJECT_NAME>
— Project Name--project-id <PROJECT_ID>
— Project ID--current-project
— Current project
osc dns quota set
Set a projects quotas
The request should be a key:value set of quotas to be set
This returns a key:value set of quotas on the system.
Usage: osc dns quota set [OPTIONS] <--project-name <PROJECT_NAME>|--project-id <PROJECT_ID>|--current-project>
Options:
--project-name <PROJECT_NAME>
— Project Name--project-id <PROJECT_ID>
— Project ID--current-project
— Current project--api-export-size <API_EXPORT_SIZE>
--recordset-records <RECORDSET_RECORDS>
--zone-records <ZONE_RECORDS>
--zone-recorsets <ZONE_RECORSETS>
--zones <ZONES>
osc dns recordset
DNS Project recordsets operations
This command manages all Recordsets owned by a project.
Usage: osc dns recordset <COMMAND>
Available subcommands:
osc dns recordset list
— List all Recordsets owned by project
osc dns recordset list
List all Recordsets owned by project
Usage: osc dns recordset list [OPTIONS]
Options:
-
--type <TYPE>
— Filter results to only show zones that have a type matching the filterPossible values:
CATALOG
,PRIMARY
,SECONDARY
-
--data <DATA>
— Filter results to only show recordsets that have a record with data matching the filter -
--description <DESCRIPTION>
— Filter results to only show zones that have a description matching the filter -
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--market <MARKET>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--name <NAME>
— Filter results to only show zones that have a name matching the filter -
--sort-dir <SORT_DIR>
— Sorts the response by the requested sort direction. A valid value is asc (ascending) or desc (descending). Default is asc. You can specify multiple pairs of sort key and sort direction query parameters. If you omit the sort direction in a pair, the API uses the natural sorting direction of the server attribute that is provided as the sort_keyPossible values:
asc
,desc
-
--sort-key <SORT_KEY>
— Sorts the response by the this attribute value. Default is id. You can specify multiple pairs of sort key and sort direction query parameters. If you omit the sort direction in a pair, the API uses the natural sorting direction of the server attribute that is provided as the sort_keyPossible values:
created_at
,id
,name
,serial
,status
,tenant_id
,ttl
,updated_at
-
--status <STATUS>
— Filter results to only show zones that have a status matching the filterPossible values:
ACTIVE
,DELETED
,ERROR
,PENDING
,SUCCESS
,ZONE
-
--ttl <TTL>
— Filter results to only show zones that have a ttl matching the filter -
--zone-id <ZONE_ID>
— ID for the zone -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc dns reverse
DNS Reverse recordsets (PTR) operations
Usage: osc dns reverse <COMMAND>
Available subcommands:
osc dns reverse floatingip
— DNS PTR records operations
osc dns reverse floatingip
DNS PTR records operations
Usage: osc dns reverse floatingip <COMMAND>
Available subcommands:
osc dns reverse floatingip list
— List FloatingIP’s PTR recordosc dns reverse floatingip show
— Show FloatingIP’s PTR recordosc dns reverse floatingip set
— Set FloatingIP’s PTR record
osc dns reverse floatingip list
List FloatingIP’s PTR record
Usage: osc dns reverse floatingip list
osc dns reverse floatingip show
Show FloatingIP’s PTR record
Usage: osc dns reverse floatingip show <FIP_KEY>
Arguments:
<FIP_KEY>
— fip_key parameter for /v2/reverse/floatingips/{fip_key} API
osc dns reverse floatingip set
Set FloatingIP’s PTR record
Usage: osc dns reverse floatingip set [OPTIONS] <FIP_KEY>
Arguments:
<FIP_KEY>
— fip_key parameter for /v2/reverse/floatingips/{fip_key} API
Options:
--address <ADDRESS>
— The floatingip address for this PTR record--description <DESCRIPTION>
— Description for this PTR record--ptrdname <PTRDNAME>
— Domain name for this PTR record--ttl <TTL>
— Time to live for this PTR record
osc dns zone
DNS Zone operations
Usage: osc dns zone <COMMAND>
Available subcommands:
osc dns zone create
— Create Zoneosc dns zone delete
— Delete a Zoneosc dns zone list
— List Zonesosc dns zone nameserver
— DNS recordsets operationsosc dns zone recordset
— DNS recordsets operationsosc dns zone show
— Show a Zoneosc dns zone set
— Update a Zone
osc dns zone create
Create Zone
Usage: osc dns zone create [OPTIONS]
Options:
-
--attributes <key=value>
— Key:Value pairs of information about this zone, and the pool the user would like to place the zone in. This information can be used by the scheduler to place zones on the correct pool -
--description <DESCRIPTION>
— Description for this zone -
--email <EMAIL>
— e-mail for the zone. Used in SOA records for the zone -
--masters <MASTERS>
— Mandatory for secondary zones. The servers to slave from to get DNS informationParameter is an array, may be provided multiple times.
-
--name <NAME>
— DNS Name for the zone -
--ttl <TTL>
— TTL (Time to Live) for the zone -
--type <TYPE>
— Type of zone. PRIMARY is controlled by Designate, SECONDARY zones are slaved from another DNS Server. Defaults to PRIMARYPossible values:
catalog
,primary
,secondary
osc dns zone delete
Delete a Zone
Usage: osc dns zone delete <ID>
Arguments:
<ID>
— zone_id parameter for /v2/zones/{zone_id} API
osc dns zone list
List Zones
Usage: osc dns zone list [OPTIONS]
Options:
-
--type <TYPE>
— Filter results to only show zones that have a type matching the filterPossible values:
CATALOG
,PRIMARY
,SECONDARY
-
--description <DESCRIPTION>
— Filter results to only show zones that have a description matching the filter -
--email <EMAIL>
— Filter results to only show zones that have an email matching the filter -
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--market <MARKET>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--name <NAME>
— Filter results to only show zones that have a name matching the filter -
--sort-dir <SORT_DIR>
— Sorts the response by the requested sort direction. A valid value is asc (ascending) or desc (descending). Default is asc. You can specify multiple pairs of sort key and sort direction query parameters. If you omit the sort direction in a pair, the API uses the natural sorting direction of the server attribute that is provided as the sort_keyPossible values:
asc
,desc
-
--sort-key <SORT_KEY>
— Sorts the response by the this attribute value. Default is id. You can specify multiple pairs of sort key and sort direction query parameters. If you omit the sort direction in a pair, the API uses the natural sorting direction of the server attribute that is provided as the sort_keyPossible values:
created_at
,id
,name
,serial
,status
,tenant_id
,ttl
,updated_at
-
--status <STATUS>
— Filter results to only show zones that have a status matching the filterPossible values:
ACTIVE
,DELETED
,ERROR
,PENDING
,SUCCESS
,ZONE
-
--ttl <TTL>
— Filter results to only show zones that have a ttl matching the filter -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc dns zone nameserver
DNS recordsets operations
Usage: osc dns zone nameserver <COMMAND>
Available subcommands:
osc dns zone nameserver list
— Get the Name Servers for a Zone
osc dns zone nameserver list
Get the Name Servers for a Zone
Usage: osc dns zone nameserver list <--zone-name <ZONE_NAME>|--zone-id <ZONE_ID>>
Options:
--zone-name <ZONE_NAME>
— Zone Name--zone-id <ZONE_ID>
— Zone ID
osc dns zone recordset
DNS recordsets operations
Usage: osc dns zone recordset <COMMAND>
Available subcommands:
osc dns zone recordset create
— Create Recordsetosc dns zone recordset delete
— Delete a Recordsetosc dns zone recordset list
— List Recordsets in a Zoneosc dns zone recordset show
— Show a Recordsetosc dns zone recordset set
— Update a Recordset
osc dns zone recordset create
Create Recordset
Usage: osc dns zone recordset create [OPTIONS] <--zone-name <ZONE_NAME>|--zone-id <ZONE_ID>>
Options:
-
--zone-name <ZONE_NAME>
— Zone Name -
--zone-id <ZONE_ID>
— Zone ID -
--description <DESCRIPTION>
— Description for this recordset -
--name <NAME>
— DNS Name for the recordset -
--records <RECORDS>
— A list of data for this recordset. Each item will be a separate record in Designate These items should conform to the DNS spec for the record type - e.g. A records must be IPv4 addresses, CNAME records must be a hostname.Parameter is an array, may be provided multiple times.
-
--ttl <TTL>
— TTL (Time to Live) for the recordset -
--type <TYPE>
— They RRTYPE of the recordsetPossible values:
a
,aaaa
,caa
,cert
,cname
,mx
,naptr
,ns
,ptr
,soa
,spf
,srv
,sshfp
,txt
osc dns zone recordset delete
Delete a Recordset
Usage: osc dns zone recordset delete <--zone-name <ZONE_NAME>|--zone-id <ZONE_ID>> <ID>
Arguments:
<ID>
— recordset_id parameter for /v2/zones/{zone_id}/recordsets/{recordset_id} API
Options:
--zone-name <ZONE_NAME>
— Zone Name--zone-id <ZONE_ID>
— Zone ID
osc dns zone recordset list
List Recordsets in a Zone
Usage: osc dns zone recordset list [OPTIONS] <--zone-name <ZONE_NAME>|--zone-id <ZONE_ID>>
Options:
-
--type <TYPE>
— Filter results to only show zones that have a type matching the filterPossible values:
CATALOG
,PRIMARY
,SECONDARY
-
--data <DATA>
— Filter results to only show recordsets that have a record with data matching the filter -
--description <DESCRIPTION>
— Filter results to only show zones that have a description matching the filter -
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--market <MARKET>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--name <NAME>
— Filter results to only show zones that have a name matching the filter -
--sort-dir <SORT_DIR>
— Sorts the response by the requested sort direction. A valid value is asc (ascending) or desc (descending). Default is asc. You can specify multiple pairs of sort key and sort direction query parameters. If you omit the sort direction in a pair, the API uses the natural sorting direction of the server attribute that is provided as the sort_keyPossible values:
asc
,desc
-
--sort-key <SORT_KEY>
— Sorts the response by the this attribute value. Default is id. You can specify multiple pairs of sort key and sort direction query parameters. If you omit the sort direction in a pair, the API uses the natural sorting direction of the server attribute that is provided as the sort_keyPossible values:
created_at
,id
,name
,serial
,status
,tenant_id
,ttl
,updated_at
-
--status <STATUS>
— Filter results to only show zones that have a status matching the filterPossible values:
ACTIVE
,DELETED
,ERROR
,PENDING
,SUCCESS
,ZONE
-
--ttl <TTL>
— Filter results to only show zones that have a ttl matching the filter -
--zone-name <ZONE_NAME>
— Zone Name -
--zone-id <ZONE_ID>
— Zone ID -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc dns zone recordset show
Show a Recordset
Usage: osc dns zone recordset show <--zone-name <ZONE_NAME>|--zone-id <ZONE_ID>> <ID>
Arguments:
<ID>
— recordset_id parameter for /v2/zones/{zone_id}/recordsets/{recordset_id} API
Options:
--zone-name <ZONE_NAME>
— Zone Name--zone-id <ZONE_ID>
— Zone ID
osc dns zone recordset set
Update a Recordset
Usage: osc dns zone recordset set [OPTIONS] <--zone-name <ZONE_NAME>|--zone-id <ZONE_ID>> <ID>
Arguments:
<ID>
— recordset_id parameter for /v2/zones/{zone_id}/recordsets/{recordset_id} API
Options:
-
--zone-name <ZONE_NAME>
— Zone Name -
--zone-id <ZONE_ID>
— Zone ID -
--description <DESCRIPTION>
— Description for this recordset -
--records <RECORDS>
— A list of data for this recordset. Each item will be a separate record in Designate These items should conform to the DNS spec for the record type - e.g. A records must be IPv4 addresses, CNAME records must be a hostname.Parameter is an array, may be provided multiple times.
-
--ttl <TTL>
— TTL (Time to Live) for the recordset
osc dns zone show
Show a Zone
Usage: osc dns zone show <ID>
Arguments:
<ID>
— zone_id parameter for /v2/zones/{zone_id} API
osc dns zone set
Update a Zone
Usage: osc dns zone set [OPTIONS] <ID>
Arguments:
<ID>
— zone_id parameter for /v2/zones/{zone_id} API
Options:
--description <DESCRIPTION>
— Description for this zone--email <EMAIL>
— e-mail for the zone. Used in SOA records for the zone--ttl <TTL>
— TTL (Time to Live) for the zone
osc identity
Identity (Keystone) commands
The Identity service generates authentication tokens that permit access to the OpenStack services REST APIs. Clients obtain this token and the URL endpoints for other service APIs by supplying their valid credentials to the authentication service.
Each time you make a REST API request to an OpenStack service, you supply your authentication token in the X-Auth-Token request header.
Like most OpenStack projects, OpenStack Identity protects its APIs by defining policy rules based on a role-based access control (RBAC) approach.
The Identity service configuration file sets the name and location of a JSON policy file that stores these rules.
Usage: osc identity <COMMAND>
Available subcommands:
osc identity auth
— Identity Auth commandsosc identity access-rule
— Application Credentials - Access Rulesosc identity application-credential
— Application Credentialsosc identity credential
— Identity Credential commandsosc identity domain
— Identity Domain commandsosc identity endpoint
— Endpoint commandsosc identity endpoint-filter
— OS-EP-FILTER APIosc identity federation
— OS-Federationosc identity group
— Identity Group commandsosc identity limit
— Unified Limitsosc identity project
— Projectsosc identity region
— Region commandsosc identity registered-limit
— Unified Limits - Registered limitsosc identity role
— Identity Role commandsosc identity role-assignment
— Role Assignments commandsosc identity role-inference
— Role Inferences commandsosc identity service
— Service commandsosc identity user
— User commands
osc identity auth
Identity Auth commands
The Identity service generates tokens in exchange for authentication credentials. A token represents the authenticated identity of a user and, optionally, grants authorization on a specific project, domain, or the deployment system.
The body of an authentication request must include a payload that specifies the authentication methods, which are normally just password or token, the credentials, and, optionally, the authorization scope. You can scope a token to a project, domain, the deployment system, or the token can be unscoped. You cannot scope a token to multiple scope targets.
Tokens have IDs, which the Identity API returns in the X-Subject-Token response header.
In the case of multi-factor authentication (MFA) more than one authentication method needs to be supplied to authenticate. As of v3.12 a failure due to MFA rules only partially being met will result in an auth receipt ID being returned in the response header Openstack-Auth-Receipt, and a response body that details the receipt itself and the missing authentication methods. Supplying the auth receipt ID in the Openstack-Auth-Receipt header in a follow-up authentication request, with the missing authentication methods, will result in a valid token by reusing the successful methods from the first request. This allows MFA authentication to be a multi-step process.
After you obtain an authentication token, you can:
-
Make REST API requests to other OpenStack services. You supply the ID of your authentication token in the X-Auth-Token request header.
-
Validate your authentication token and list the domains, projects, roles, and endpoints that your token gives you access to.
-
Use your token to request another token scoped for a different domain and project.
-
Force the immediate revocation of a token.
-
List revoked public key infrastructure (PKI) tokens.
Usage: osc identity auth <COMMAND>
Available subcommands:
osc identity auth catalog
— Identity Catalog commandsosc identity auth domain
— Identity Domain scope commandsosc identity auth federation
— Identity Federated auch commandsosc identity auth project
— Identity project scope commandsosc identity auth system
— Identity system scope commandsosc identity auth token
— Identity token commands
osc identity auth catalog
Identity Catalog commands
A service is an OpenStack web service that you can access through a URL, i.e. an endpoint.
A service catalog lists the services that are available to the caller based upon the current authorization.
Usage: osc identity auth catalog <COMMAND>
Available subcommands:
osc identity auth catalog list
— Get service catalog
osc identity auth catalog list
New in version 3.3
This call returns a service catalog for the X-Auth-Token provided in the request, even if the token does not contain a catalog itself (for example, if it was generated using ?nocatalog).
The structure of the catalog object is identical to that contained in a token.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/auth_catalog
Usage: osc identity auth catalog list
osc identity auth domain
Identity Domain scope commands
authorization.
Usage: osc identity auth domain <COMMAND>
Available subcommands:
osc identity auth domain list
— Get available domain scopes
osc identity auth domain list
New in version 3.3
This call returns the list of domains that are available to be scoped to based on the X-Auth-Token provided in the request.
The structure is the same as listing domains.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/auth_domains
Usage: osc identity auth domain list
osc identity auth federation
Identity Federated auch commands
authorization.
Usage: osc identity auth federation <COMMAND>
Available subcommands:
osc identity auth federation identity-provider
— Identity WebSSO auth commandsosc identity auth federation websso
— Identity WebSSO auth commands
osc identity auth federation identity-provider
Identity WebSSO auth commands
Usage: osc identity auth federation identity-provider <COMMAND>
Available subcommands:
osc identity auth federation identity-provider protocol
— Identity WebSSO auth commands
osc identity auth federation identity-provider protocol
Identity WebSSO auth commands
Usage: osc identity auth federation identity-provider protocol <COMMAND>
Available subcommands:
osc identity auth federation identity-provider protocol websso
— Identity WebSSO auth commands
osc identity auth federation identity-provider protocol websso
Identity WebSSO auth commands
Usage: osc identity auth federation identity-provider protocol websso <COMMAND>
Available subcommands:
osc identity auth federation identity-provider protocol websso create
— POST operation on /v3/auth/OS-FEDERATION/identity_providers/{idp_id}/protocols/{protocol_id}/webssoosc identity auth federation identity-provider protocol websso get
— GET operation on /v3/auth/OS-FEDERATION/identity_providers/{idp_id}/protocols/{protocol_id}/websso
osc identity auth federation identity-provider protocol websso create
POST operation on /v3/auth/OS-FEDERATION/identity_providers/{idp_id}/protocols/{protocol_id}/websso
Usage: osc identity auth federation identity-provider protocol websso create <IDP_ID> <PROTOCOL_ID>
Arguments:
<IDP_ID>
— idp_id parameter for /v3/auth/OS-FEDERATION/identity_providers/{idp_id}/protocols/{protocol_id}/websso API<PROTOCOL_ID>
— protocol_id parameter for /v3/auth/OS-FEDERATION/identity_providers/{idp_id}/protocols/{protocol_id}/websso API
osc identity auth federation identity-provider protocol websso get
GET operation on /v3/auth/OS-FEDERATION/identity_providers/{idp_id}/protocols/{protocol_id}/websso
Usage: osc identity auth federation identity-provider protocol websso get <IDP_ID> <PROTOCOL_ID>
Arguments:
<IDP_ID>
— idp_id parameter for /v3/auth/OS-FEDERATION/identity_providers/{idp_id}/protocols/{protocol_id}/websso API<PROTOCOL_ID>
— protocol_id parameter for /v3/auth/OS-FEDERATION/identity_providers/{idp_id}/protocols/{protocol_id}/websso API
osc identity auth federation websso
Identity WebSSO auth commands
Usage: osc identity auth federation websso <COMMAND>
Available subcommands:
osc identity auth federation websso create
— POST operation on /v3/auth/OS-FEDERATION/websso/{protocol_id}osc identity auth federation websso show
— GET operation on /v3/auth/OS-FEDERATION/websso/{protocol_id}
osc identity auth federation websso create
POST operation on /v3/auth/OS-FEDERATION/websso/{protocol_id}
Usage: osc identity auth federation websso create <PROTOCOL_ID>
Arguments:
<PROTOCOL_ID>
— protocol_id parameter for /v3/auth/OS-FEDERATION/websso/{protocol_id} API
osc identity auth federation websso show
GET operation on /v3/auth/OS-FEDERATION/websso/{protocol_id}
Usage: osc identity auth federation websso show <PROTOCOL_ID>
Arguments:
<PROTOCOL_ID>
— protocol_id parameter for /v3/auth/OS-FEDERATION/websso/{protocol_id} API
osc identity auth project
Identity project scope commands
authorization.
Usage: osc identity auth project <COMMAND>
Available subcommands:
osc identity auth project list
— Get available project scopes
osc identity auth project list
New in version 3.3
This call returns the list of projects that are available to be scoped to based on the X-Auth-Token provided in the request.
The structure of the response is exactly the same as listing projects for a user.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/auth_projects
Usage: osc identity auth project list
osc identity auth system
Identity system scope commands
authorization.
Usage: osc identity auth system <COMMAND>
Available subcommands:
osc identity auth system list
— Get available system scopes
osc identity auth system list
New in version 3.10
This call returns the list of systems that are available to be scoped to based on the X-Auth-Token provided in the request.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/auth_system
Usage: osc identity auth system list
osc identity auth token
Identity token commands
authorization.
Usage: osc identity auth token <COMMAND>
Available subcommands:
osc identity auth token delete
— Revoke tokenosc identity auth token get
— Validate and show information for token
osc identity auth token delete
Revokes a token.
This call is similar to the HEAD /auth/tokens
call except that the X-Subject-Token
token is immediately not valid, regardless of the expires_at
attribute value. An additional X-Auth-Token
is not required.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/auth_tokens
Usage: osc identity auth token delete
osc identity auth token get
Validates and shows information for a token, including its expiration date and authorization scope.
Pass your own token in the X-Auth-Token
request header.
Pass the token that you want to validate in the X-Subject-Token
request header.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/auth_tokens
Usage: osc identity auth token get
osc identity access-rule
Application Credentials - Access Rules
Users have the option of delegating more fine-grained access control to their application credentials by using access rules. For example, to create an application credential that is constricted to creating servers in nova, the user can add the following access rules:
{ "access_rules": [{ "path": "/v2.1/servers", "method": "POST", "service": "compute" }] }
The "path" attribute of application credential access rules uses a wildcard syntax to make it more flexible. For example, to create an application credential that is constricted to listing server IP addresses, you could use either of the following access rules:
{ "access_rules": [ { "path": "/v2.1/servers/*/ips", "method": "GET", "service": "compute" } ] }
or equivalently:
{ "access_rules": [ { "path": "/v2.1/servers/{server_id}/ips", "method": "GET", "service": "compute" } ] }
In both cases, a request path containing any server ID will match the access rule. For even more flexibility, the recursive wildcard ** indicates that request paths containing any number of / will be matched. For example:
{ "access_rules": [ { "path": "/v2.1/**", "method": "GET", "service": "compute" } ] }
will match any nova API for version 2.1.
An access rule created for one application credential can be re-used by providing its ID to another application credential, for example:
{ "access_rules": [ { "id": "abcdef" } ] }
Usage: osc identity access-rule <COMMAND>
Available subcommands:
osc identity access-rule delete
— Delete access ruleosc identity access-rule list
— List access rulesosc identity access-rule show
— Show access rule details
osc identity access-rule delete
Delete an access rule. An access rule that is still in use by an application credential cannot be deleted.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/access_rules
Usage: osc identity access-rule delete <--user-name <USER_NAME>|--user-id <USER_ID>|--current-user> <ID>
Arguments:
<ID>
— access_rule_id parameter for /v3/users/{user_id}/access_rules/{access_rule_id} API
Options:
--user-name <USER_NAME>
— User Name--user-id <USER_ID>
— User ID--current-user
— Current authenticated user
osc identity access-rule list
List all access rules for a user.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/access_rules
Usage: osc identity access-rule list [OPTIONS] <--user-name <USER_NAME>|--user-id <USER_ID>|--current-user>
Options:
--method <METHOD>
— The request method that the application credential is permitted to use for a given API endpoint--path <PATH>
— The API path that the application credential is permitted to access--service <SERVICE>
— The service type identifier for the service that the application is permitted to access--user-name <USER_NAME>
— User Name--user-id <USER_ID>
— User ID--current-user
— Current authenticated user
osc identity access-rule show
Show details of an access rule.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/access_rules
Usage: osc identity access-rule show <--user-name <USER_NAME>|--user-id <USER_ID>|--current-user> <ID>
Arguments:
<ID>
— access_rule_id parameter for /v3/users/{user_id}/access_rules/{access_rule_id} API
Options:
--user-name <USER_NAME>
— User Name--user-id <USER_ID>
— User ID--current-user
— Current authenticated user
osc identity application-credential
Application Credentials
Application credentials provide a way to delegate a user’s authorization to an application without sharing the user’s password authentication. This is a useful security measure, especially for situations where the user’s identification is provided by an external source, such as LDAP or a single-sign-on service. Instead of storing user passwords in config files, a user creates an application credential for a specific project, with all or a subset of the role assignments they have on that project, and then stores the application credential identifier and secret in the config file.
Multiple application credentials may be active at once, so you can easily rotate application credentials by creating a second one, converting your applications to use it one by one, and finally deleting the first one.
Application credentials are limited by the lifespan of the user that created them. If the user is deleted, disabled, or loses a role assignment on a project, the application credential is deleted.
Application credentials can have their privileges limited in two ways. First, the owner may specify a subset of their own roles that the application credential may assume when getting a token for a project. For example, if a user has the member role on a project, they also have the implied role reader and can grant the application credential only the reader role for the project:
"roles": [ {"name": "reader"} ]
Users also have the option of delegating more fine-grained access control to their application credentials by using access rules. For example, to create an application credential that is constricted to creating servers in nova, the user can add the following access rules:
"access_rules": [ { "path": "/v2.1/servers", "method": "POST", "service": "compute" } ]
The "path" attribute of application credential access rules uses a wildcard syntax to make it more flexible. For example, to create an application credential that is constricted to listing server IP addresses, you could use either of the following access rules:
"access_rules": [ { "path": "/v2.1/servers/*/ips", "method": "GET", "service": "compute" } ]
or equivalently:
"access_rules": [ { "path": "/v2.1/servers/{server_id}/ips", "method": "GET", "service": "compute" } ]
In both cases, a request path containing any server ID will match the access rule. For even more flexibility, the recursive wildcard ** indicates that request paths containing any number of / will be matched. For example:
"access_rules": [ { "path": "/v2.1/**", "method": "GET", "service": "compute" } ]
will match any nova API for version 2.1.
An access rule created for one application credential can be re-used by providing its ID to another application credential, for example:
"access_rules": [ { "id": "abcdef" } ]
Usage: osc identity application-credential <COMMAND>
Available subcommands:
osc identity application-credential create
— Create application credentialosc identity application-credential delete
— Delete application credentialosc identity application-credential list
— List application credentialsosc identity application-credential show
— Show application credential details
osc identity application-credential create
Creates an application credential for a user on the project to which the current token is scoped.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/application_credentials
Usage: osc identity application-credential create [OPTIONS] --name <NAME> <--user-name <USER_NAME>|--user-id <USER_ID>|--current-user>
Options:
-
--user-name <USER_NAME>
— User Name -
--user-id <USER_ID>
— User ID -
--current-user
— Current authenticated user -
--access-rules <JSON>
— A list ofaccess_rules
objectsParameter is an array, may be provided multiple times.
-
--description <DESCRIPTION>
— A description of the application credential’s purpose -
--expires-at <EXPIRES_AT>
— An optional expiry time for the application credential. If unset, the application credential does not expire -
--id <ID>
— The UUID for the credential -
--name <NAME>
— The name of the application credential. Must be unique to a user -
--project-id <PROJECT_ID>
— The ID of the project the application credential was created for and that authentication requests using this application credential will be scoped to -
--roles <JSON>
— An optional list of role objects, identified by ID or name. The list may only contain roles that the user has assigned on the project. If not provided, the roles assigned to the application credential will be the same as the roles in the current token.Parameter is an array, may be provided multiple times.
-
--secret <SECRET>
— The secret that the application credential will be created with. If not provided, one will be generated -
--system <SYSTEM>
-
--unrestricted <UNRESTRICTED>
— An optional flag to restrict whether the application credential may be used for the creation or destruction of other application credentials or trusts. Defaults to falsePossible values:
true
,false
osc identity application-credential delete
Delete an application credential.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/application_credentials
Usage: osc identity application-credential delete <--user-name <USER_NAME>|--user-id <USER_ID>|--current-user> <ID>
Arguments:
<ID>
— application_credential_id parameter for /v3/users/{user_id}/application_credentials/{application_credential_id} API
Options:
--user-name <USER_NAME>
— User Name--user-id <USER_ID>
— User ID--current-user
— Current authenticated user
osc identity application-credential list
List all application credentials for a user.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/application_credentials
Usage: osc identity application-credential list [OPTIONS] <--user-name <USER_NAME>|--user-id <USER_ID>|--current-user>
Options:
--name <NAME>
— The name of the application credential. Must be unique to a user--user-name <USER_NAME>
— User Name--user-id <USER_ID>
— User ID--current-user
— Current authenticated user
osc identity application-credential show
Show details of an application credential.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/application_credentials
Usage: osc identity application-credential show <--user-name <USER_NAME>|--user-id <USER_ID>|--current-user> <ID>
Arguments:
<ID>
— application_credential_id parameter for /v3/users/{user_id}/application_credentials/{application_credential_id} API
Options:
--user-name <USER_NAME>
— User Name--user-id <USER_ID>
— User ID--current-user
— Current authenticated user
osc identity credential
Identity Credential commands
In exchange for a set of authentication credentials that the user submits, the Identity service generates and returns a token. A token represents the authenticated identity of a user and, optionally, grants authorization on a specific project or domain.
You can list all credentials, and create, show details for, update, and delete a credential.
Usage: osc identity credential <COMMAND>
Available subcommands:
osc identity credential create
— Create credentialosc identity credential delete
— Delete credentialosc identity credential list
— List credentialsosc identity credential set
— Update credentialosc identity credential show
— Show credential details
osc identity credential create
Creates a credential.
The following example shows how to create an EC2-style credential. The credential blob is a string that contains a JSON-serialized dictionary with the access
and secret
keys. This format is required when you specify the ec2
type. To specify other credentials, such as access_key
, change the type and contents of the data blob.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/credentials
Usage: osc identity credential create [OPTIONS] --blob <BLOB> --type <TYPE> --user-id <USER_ID>
Options:
--blob <BLOB>
— The credential itself, as a serialized blob--id <ID>
— The UUID for the credential--project-id <PROJECT_ID>
— The ID for the project--type <TYPE>
— The credential type, such asec2
orcert
. The implementation determines the list of supported types--user-id <USER_ID>
— The ID of the user who owns the credential
osc identity credential delete
Deletes a credential.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/credential
Usage: osc identity credential delete <ID>
Arguments:
<ID>
— credential_id parameter for /v3/credentials/{credential_id} API
osc identity credential list
Lists all credentials.
Optionally, you can include the user_id
or type
query parameter in the URI to filter the response by a user or credential type.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/credentials
Usage: osc identity credential list [OPTIONS]
Options:
--type <TYPE>
— The credential type, such as ec2 or cert. The implementation determines the list of supported types--user-name <USER_NAME>
— User Name--user-id <USER_ID>
— User ID--current-user
— Current authenticated user
osc identity credential set
Updates a credential.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/credential
Usage: osc identity credential set [OPTIONS] <ID>
Arguments:
<ID>
— credential_id parameter for /v3/credentials/{credential_id} API
Options:
--blob <BLOB>
— The credential itself, as a serialized blob--project-id <PROJECT_ID>
— The ID for the project--type <TYPE>
— The credential type, such asec2
orcert
. The implementation determines the list of supported types--user-id <USER_ID>
— The ID of the user who owns the credential
osc identity credential show
Shows details for a credential.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/credential
Usage: osc identity credential show <ID>
Arguments:
<ID>
— credential_id parameter for /v3/credentials/{credential_id} API
osc identity domain
Identity Domain commands
A domain is a collection of users, groups, and projects. Each group and project is owned by exactly one domain.
Each domain defines a namespace where certain API-visible name attributes exist, which affects whether those names must be globally unique or unique within that domain. In the Identity API, the uniqueness of these attributes is as follows:
-
Domain name. Globally unique across all domains.
-
Role name. Unique within the owning domain.
-
User name. Unique within the owning domain.
-
Project name. Unique within the owning domain.
-
Group name. Unique within the owning domain.
Usage: osc identity domain <COMMAND>
Available subcommands:
osc identity domain create
— Create domainosc identity domain config
— Config configurationosc identity domain delete
— Delete domainosc identity domain group
— Domain groupsosc identity domain list
— List domainsosc identity domain set
— Update domainosc identity domain show
— Show domain detailsosc identity domain user
— Domain groups
osc identity domain create
Creates a domain.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/domains
Usage: osc identity domain create [OPTIONS] --name <NAME>
Options:
-
--description <DESCRIPTION>
— The description of the domain -
--enabled <ENABLED>
— If set totrue
, domain is created enabled. If set tofalse
, domain is created disabled. The default istrue
.Users can only authorize against an enabled domain (and any of its projects). In addition, users can only authenticate if the domain that owns them is also enabled. Disabling a domain prevents both of these things.
Possible values:
true
,false
-
--explicit-domain-id <EXPLICIT_DOMAIN_ID>
— The ID of the domain. A domain created this way will not use an auto-generated ID, but will use the ID passed in instead. Identifiers passed in this way must conform to the existing ID generation scheme: UUID4 without dashes -
--name <NAME>
— The name of the domain -
--immutable <IMMUTABLE>
Possible values:
true
,false
-
--tags <TAGS>
— Parameter is an array, may be provided multiple times
osc identity domain config
Config configuration
You can manage domain-specific configuration options.
Config-specific configuration options are structured within their group objects. The API supports only the identity and ldap groups. These groups override the default configuration settings for the storage of users and groups by the Identity server.
You can create, update, and delete domain-specific configuration options by using the HTTP PUT , PATCH , and DELETE methods. When updating, it is only necessary to include those options that are being updated.
To create an option, use the PUT method. The Identity API does not return options that are considered sensitive, although you can create and update these options. The only option currently considered sensitive is the password option within the ldap group.
The API enables you to include sensitive options as part of non- sensitive options. For example, you can include the password as part of the url option.
If you try to create or update configuration options for groups other than whitelisted on the server side, the Forbidden (403) response code is returned.
For information about how to integrate the Identity service with LDAP, see Integrate Identity with LDAP.
A domain is a collection of users, groups, and projects. Each group and project is owned by exactly one domain.
Usage: osc identity domain config <COMMAND>
Available subcommands:
osc identity domain config default
— Show default configuration settingsosc identity domain config group
— Domain Group grouposc identity domain config purge
— Delete domain configurationosc identity domain config list
— Show domain configurationosc identity domain config replace
— Create domain configurationosc identity domain config set
— Update domain configuration
osc identity domain config default
The default configuration settings for the options that can be overridden can be retrieved.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/domain_config_default
Usage: osc identity domain config default
osc identity domain config group
Domain Group group
Group group is a driver specific configuration for the domain. It contains individual configuration options.
Usage: osc identity domain config group <COMMAND>
Available subcommands:
osc identity domain config group default
— Show default configuration for a grouposc identity domain config group delete
— Delete domain group configurationosc identity domain config group option
— Domain Group Optionosc identity domain config group set
— Update domain group configurationosc identity domain config group show
— Show domain group configuration
osc identity domain config group default
Reads the default configuration settings for a specific group.
The API supports only the identity
and ldap
groups.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/domain_config_default
Usage: osc identity domain config group default <GROUP>
Arguments:
<GROUP>
— group parameter for /v3/domains/config/{group}/{option}/default API
osc identity domain config group delete
Deletes a domain group configuration.
The API supports only the identity
and ldap
groups.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/domain_config_default
Usage: osc identity domain config group delete <--domain-name <DOMAIN_NAME>|--domain-id <DOMAIN_ID>|--current-domain> <GROUP>
Arguments:
<GROUP>
— group parameter for /v3/domains/{domain_id}/config/{group}/{option} API
Options:
--domain-name <DOMAIN_NAME>
— Domain Name--domain-id <DOMAIN_ID>
— Domain ID--current-domain
— Current domain
osc identity domain config group option
Domain Group Option
A domain group option is a configuration option of the domain specific driver.
Usage: osc identity domain config group option <COMMAND>
Available subcommands:
osc identity domain config group option default
— Show default option for a grouposc identity domain config group option delete
— Delete domain group option configurationosc identity domain config group option set
— Update domain group option configurationosc identity domain config group option show
— Show domain group option configuration
osc identity domain config group option default
Reads the default configuration setting for an option within a group.
The API supports only the identity
and ldap
groups. For the ldap
group, a valid value is url
or user_tree_dn
. For the identity
group, a valid value is driver
.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/domain_config_default
Usage: osc identity domain config group option default <GROUP> <OPTION>
Arguments:
<GROUP>
— group parameter for /v3/domains/config/{group}/{option}/default API<OPTION>
— option parameter for /v3/domains/config/{group}/{option}/default API
osc identity domain config group option delete
Deletes a domain group option configuration.
The API supports only the identity
and ldap
groups. For the ldap
group, a valid value is url
or user_tree_dn
. For the identity
group, a valid value is driver
.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/domain_config_default
Usage: osc identity domain config group option delete <--domain-name <DOMAIN_NAME>|--domain-id <DOMAIN_ID>|--current-domain> <GROUP> <OPTION>
Arguments:
<GROUP>
— group parameter for /v3/domains/{domain_id}/config/{group}/{option} API<OPTION>
— option parameter for /v3/domains/{domain_id}/config/{group}/{option} API
Options:
--domain-name <DOMAIN_NAME>
— Domain Name--domain-id <DOMAIN_ID>
— Domain ID--current-domain
— Current domain
osc identity domain config group option set
Updates a domain group option configuration.
The API supports only the identity
and ldap
groups. For the ldap
group, a valid value is url
or user_tree_dn
. For the identity
group, a valid value is driver
.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/domain_config_default
Usage: osc identity domain config group option set [OPTIONS] <--domain-name <DOMAIN_NAME>|--domain-id <DOMAIN_ID>|--current-domain> <GROUP> <OPTION>
Arguments:
<GROUP>
— group parameter for /v3/domains/{domain_id}/config/{group}/{option} API<OPTION>
— option parameter for /v3/domains/{domain_id}/config/{group}/{option} API
Options:
--domain-name <DOMAIN_NAME>
— Domain Name--domain-id <DOMAIN_ID>
— Domain ID--current-domain
— Current domain--config <key=value>
— Aconfig
object
osc identity domain config group option show
Shows details for a domain group option configuration.
The API supports only the identity
and ldap
groups. For the ldap
group, a valid value is url
or user_tree_dn
. For the identity
group, a valid value is driver
.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/domain_config_default
Usage: osc identity domain config group option show <--domain-name <DOMAIN_NAME>|--domain-id <DOMAIN_ID>|--current-domain> <GROUP> <OPTION>
Arguments:
<GROUP>
— group parameter for /v3/domains/{domain_id}/config/{group}/{option} API<OPTION>
— option parameter for /v3/domains/{domain_id}/config/{group}/{option} API
Options:
--domain-name <DOMAIN_NAME>
— Domain Name--domain-id <DOMAIN_ID>
— Domain ID--current-domain
— Current domain
osc identity domain config group set
Updates a domain group configuration.
The API supports only the identity
and ldap
groups. If you try to set configuration options for other groups, this call fails with the Forbidden (403)
response code.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/domain_config_default
Usage: osc identity domain config group set --config <JSON> <--domain-name <DOMAIN_NAME>|--domain-id <DOMAIN_ID>|--current-domain> <GROUP>
Arguments:
<GROUP>
— group parameter for /v3/domains/{domain_id}/config/{group}/{option} API
Options:
--domain-name <DOMAIN_NAME>
— Domain Name--domain-id <DOMAIN_ID>
— Domain ID--current-domain
— Current domain--config <JSON>
— Aconfig
object
osc identity domain config group show
Shows details for a domain group configuration.
The API supports only the identity
and ldap
groups.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/domain_config_default
Usage: osc identity domain config group show <--domain-name <DOMAIN_NAME>|--domain-id <DOMAIN_ID>|--current-domain> <GROUP>
Arguments:
<GROUP>
— group parameter for /v3/domains/{domain_id}/config/{group}/{option} API
Options:
--domain-name <DOMAIN_NAME>
— Domain Name--domain-id <DOMAIN_ID>
— Domain ID--current-domain
— Current domain
osc identity domain config purge
Deletes a domain configuration.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/domain_config
Usage: osc identity domain config purge <--domain-name <DOMAIN_NAME>|--domain-id <DOMAIN_ID>|--current-domain>
Options:
--domain-name <DOMAIN_NAME>
— Domain Name--domain-id <DOMAIN_ID>
— Domain ID--current-domain
— Current domain
osc identity domain config list
Shows details for a domain configuration.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/domain_config
Usage: osc identity domain config list <--domain-name <DOMAIN_NAME>|--domain-id <DOMAIN_ID>|--current-domain>
Options:
--domain-name <DOMAIN_NAME>
— Domain Name--domain-id <DOMAIN_ID>
— Domain ID--current-domain
— Current domain
osc identity domain config replace
Creates a domain configuration.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/domain_config
Usage: osc identity domain config replace --config <JSON> <--domain-name <DOMAIN_NAME>|--domain-id <DOMAIN_ID>|--current-domain>
Options:
--domain-name <DOMAIN_NAME>
— Domain Name--domain-id <DOMAIN_ID>
— Domain ID--current-domain
— Current domain--config <JSON>
— Aconfig
object
osc identity domain config set
Updates a domain configuration.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/domain_config
Usage: osc identity domain config set --config <JSON> <--domain-name <DOMAIN_NAME>|--domain-id <DOMAIN_ID>|--current-domain>
Options:
--domain-name <DOMAIN_NAME>
— Domain Name--domain-id <DOMAIN_ID>
— Domain ID--current-domain
— Current domain--config <JSON>
— Aconfig
object
osc identity domain delete
Deletes a domain. To minimize the risk of accidentally deleting a domain, you must first disable the domain by using the update domain method.
When you delete a domain, this call also deletes all entities owned by it, such as users, groups, and projects, and any credentials and granted roles that relate to those entities.
If you try to delete an enabled domain, this call returns the Forbidden (403)
response code.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/domain
Usage: osc identity domain delete <ID>
Arguments:
<ID>
— domain_id parameter for /v3/domains/{domain_id} API
osc identity domain group
Domain groups
Usage: osc identity domain group <COMMAND>
Available subcommands:
osc identity domain group role
— Domain group roles
osc identity domain group role
Domain group roles
OpenStack services typically determine whether a user’s API request should be allowed using Role Based Access Control (RBAC). For OpenStack this means the service compares the roles that user has on the project (as indicated by the roles in the token), against the roles required for the API in question (as defined in the service’s policy file). A user obtains roles on a project by having these assigned to them via the Identity service API.
Roles must initially be created as entities via the Identity services API and, once created, can then be assigned. You can assign roles to a user or group on a project, including projects owned by other domains. You can also assign roles to a user or group on a domain, although this is only currently relevant for using a domain scoped token to execute domain-level Identity service API requests.
Usage: osc identity domain group role <COMMAND>
Available subcommands:
osc identity domain group role delete
— Unassign role from group on domainosc identity domain group role list
— List role assignments for group on domainosc identity domain group role set
— Assign role to group on domainosc identity domain group role show
— Check if a group has a specific role on a domain
osc identity domain group role delete
Unassigns a role from a group on a domain.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/domain_group_role
Usage: osc identity domain group role delete <--domain-name <DOMAIN_NAME>|--domain-id <DOMAIN_ID>|--current-domain> <GROUP_ID> <ID>
Arguments:
<GROUP_ID>
— group_id parameter for /v3/domains/{domain_id}/groups/{group_id}/roles/{role_id} API<ID>
— role_id parameter for /v3/domains/{domain_id}/groups/{group_id}/roles/{role_id} API
Options:
--domain-name <DOMAIN_NAME>
— Domain Name--domain-id <DOMAIN_ID>
— Domain ID--current-domain
— Current domain
osc identity domain group role list
Lists role assignments for a group on a domain.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/domain_group_roles
Usage: osc identity domain group role list <--domain-name <DOMAIN_NAME>|--domain-id <DOMAIN_ID>|--current-domain> <GROUP_ID>
Arguments:
<GROUP_ID>
— group_id parameter for /v3/domains/{domain_id}/groups/{group_id}/roles/{role_id} API
Options:
--domain-name <DOMAIN_NAME>
— Domain Name--domain-id <DOMAIN_ID>
— Domain ID--current-domain
— Current domain
osc identity domain group role set
Assigns a role to a group on a domain.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/domain_group_role
Usage: osc identity domain group role set <--domain-name <DOMAIN_NAME>|--domain-id <DOMAIN_ID>|--current-domain> <GROUP_ID> <ID>
Arguments:
<GROUP_ID>
— group_id parameter for /v3/domains/{domain_id}/groups/{group_id}/roles/{role_id} API<ID>
— role_id parameter for /v3/domains/{domain_id}/groups/{group_id}/roles/{role_id} API
Options:
--domain-name <DOMAIN_NAME>
— Domain Name--domain-id <DOMAIN_ID>
— Domain ID--current-domain
— Current domain
osc identity domain group role show
Check if a group has a specific role on a domain.
GET/HEAD /v3/domains/{domain_id}/groups/{group_id}/roles/{role_id}
Usage: osc identity domain group role show <--domain-name <DOMAIN_NAME>|--domain-id <DOMAIN_ID>|--current-domain> <GROUP_ID> <ID>
Arguments:
<GROUP_ID>
— group_id parameter for /v3/domains/{domain_id}/groups/{group_id}/roles/{role_id} API<ID>
— role_id parameter for /v3/domains/{domain_id}/groups/{group_id}/roles/{role_id} API
Options:
--domain-name <DOMAIN_NAME>
— Domain Name--domain-id <DOMAIN_ID>
— Domain ID--current-domain
— Current domain
osc identity domain list
Lists all domains.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/domains
Usage: osc identity domain list [OPTIONS]
Options:
-
--enabled <ENABLED>
— If set to true, then only domains that are enabled will be returned, if set to false only that are disabled will be returned. Any value other than 0, including no value, will be interpreted as truePossible values:
true
,false
-
--limit <LIMIT>
-
--marker <MARKER>
— ID of the last fetched entry -
--name <NAME>
— The resource name -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc identity domain set
Updates a domain.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/domain
Usage: osc identity domain set [OPTIONS] <ID>
Arguments:
<ID>
— domain_id parameter for /v3/domains/{domain_id} API
Options:
-
--description <DESCRIPTION>
— The new description of the domain -
--enabled <ENABLED>
— If set totrue
, domain is enabled. If set tofalse
, domain is disabled. The default istrue
.Users can only authorize against an enabled domain (and any of its projects). In addition, users can only authenticate if the domain that owns them is also enabled. Disabling a domain prevents both of these things. When you disable a domain, all tokens that are authorized for that domain become invalid. However, if you reenable the domain, these tokens become valid again, providing that they haven’t expired.
Possible values:
true
,false
-
--name <NAME>
— The new name of the domain -
--immutable <IMMUTABLE>
Possible values:
true
,false
-
--tags <TAGS>
— Parameter is an array, may be provided multiple times
osc identity domain show
Shows details for a domain.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/domains
Usage: osc identity domain show <ID>
Arguments:
<ID>
— domain_id parameter for /v3/domains/{domain_id} API
osc identity domain user
Domain groups
Usage: osc identity domain user <COMMAND>
Available subcommands:
osc identity domain user role
— Domain user roles
osc identity domain user role
Domain user roles
OpenStack services typically determine whether a user’s API request should be allowed using Role Based Access Control (RBAC). For OpenStack this means the service compares the roles that user has on the project (as indicated by the roles in the token), against the roles required for the API in question (as defined in the service’s policy file). A user obtains roles on a project by having these assigned to them via the Identity service API.
Roles must initially be created as entities via the Identity services API and, once created, can then be assigned. You can assign roles to a user or group on a project, including projects owned by other domains. You can also assign roles to a user or group on a domain, although this is only currently relevant for using a domain scoped token to execute domain-level Identity service API requests.
Usage: osc identity domain user role <COMMAND>
Available subcommands:
osc identity domain user role delete
— Unassigns role from user on domainosc identity domain user role list
— List role assignments for user on domainosc identity domain user role set
— Assign role to user on domainosc identity domain user role show
— Check if a user has a specific role on the domain
osc identity domain user role delete
Unassigns a role from a user on a domain.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/domain_user_role
Usage: osc identity domain user role delete <--domain-name <DOMAIN_NAME>|--domain-id <DOMAIN_ID>|--current-domain> <--user-name <USER_NAME>|--user-id <USER_ID>|--current-user> <ID>
Arguments:
<ID>
— role_id parameter for /v3/domains/{domain_id}/users/{user_id}/roles/{role_id} API
Options:
--domain-name <DOMAIN_NAME>
— Domain Name--domain-id <DOMAIN_ID>
— Domain ID--current-domain
— Current domain--user-name <USER_NAME>
— User Name--user-id <USER_ID>
— User ID--current-user
— Current authenticated user
osc identity domain user role list
Lists role assignments for a user on a domain.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/domain_user_roles
Usage: osc identity domain user role list <--domain-name <DOMAIN_NAME>|--domain-id <DOMAIN_ID>|--current-domain> <--user-name <USER_NAME>|--user-id <USER_ID>|--current-user>
Options:
--domain-name <DOMAIN_NAME>
— Domain Name--domain-id <DOMAIN_ID>
— Domain ID--current-domain
— Current domain--user-name <USER_NAME>
— User Name--user-id <USER_ID>
— User ID--current-user
— Current authenticated user
osc identity domain user role set
Assigns a role to a user on a domain.
Relationship: https://developer.openstack.org/api-ref/identity/v3/index.html#assign-role-to-user-on-domain
Usage: osc identity domain user role set <--domain-name <DOMAIN_NAME>|--domain-id <DOMAIN_ID>|--current-domain> <--user-name <USER_NAME>|--user-id <USER_ID>|--current-user> <ID>
Arguments:
<ID>
— role_id parameter for /v3/domains/{domain_id}/users/{user_id}/roles/{role_id} API
Options:
--domain-name <DOMAIN_NAME>
— Domain Name--domain-id <DOMAIN_ID>
— Domain ID--current-domain
— Current domain--user-name <USER_NAME>
— User Name--user-id <USER_ID>
— User ID--current-user
— Current authenticated user
osc identity domain user role show
Check if a user has a specific role on the domain.
GET/HEAD /v3/domains/{domain_id}/users/{user_id}/roles/{role_id}
Usage: osc identity domain user role show <--domain-name <DOMAIN_NAME>|--domain-id <DOMAIN_ID>|--current-domain> <--user-name <USER_NAME>|--user-id <USER_ID>|--current-user> <ID>
Arguments:
<ID>
— role_id parameter for /v3/domains/{domain_id}/users/{user_id}/roles/{role_id} API
Options:
--domain-name <DOMAIN_NAME>
— Domain Name--domain-id <DOMAIN_ID>
— Domain ID--current-domain
— Current domain--user-name <USER_NAME>
— User Name--user-id <USER_ID>
— User ID--current-user
— Current authenticated user
osc identity endpoint
Endpoint commands
Usage: osc identity endpoint <COMMAND>
Available subcommands:
osc identity endpoint create
— Create endpointosc identity endpoint delete
— Delete endpointosc identity endpoint list
— List endpointsosc identity endpoint set
— Update endpointosc identity endpoint show
— Show endpoint details
osc identity endpoint create
Creates an endpoint.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/endpoints
Usage: osc identity endpoint create [OPTIONS] --interface <INTERFACE> --service-id <SERVICE_ID> --url <URL>
Options:
-
--description <DESCRIPTION>
— A description of the endpoint -
--enabled <ENABLED>
— Defines whether the endpoint appears in the service catalog: -false
. The endpoint does not appear in the service catalog. -true
. The endpoint appears in the service catalog. Default istrue
Possible values:
true
,false
-
--id <ID>
— The endpoint ID -
--interface <INTERFACE>
— The interface type, which describes the visibility of the endpoint. Value is: -public
. Visible by end users on a publicly available network interface. -internal
. Visible by end users on an unmetered internal network interface. -admin
. Visible by administrative users on a secure network interfacePossible values:
admin
,internal
,public
-
--name <NAME>
— The name of the endpoint -
--region <REGION>
— (Deprecated in v3.2) The geographic location of the service endpoint -
--region-id <REGION_ID>
— (Since v3.2) The ID of the region that contains the service endpoint -
--service-id <SERVICE_ID>
— The UUID of the service to which the endpoint belongs -
--url <URL>
— The endpoint URL
osc identity endpoint delete
Deletes an endpoint.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/endpoint
Usage: osc identity endpoint delete <ID>
Arguments:
<ID>
— endpoint_id parameter for /v3/endpoints/{endpoint_id} API
osc identity endpoint list
Lists all available endpoints.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/endpoints
Usage: osc identity endpoint list [OPTIONS]
Options:
-
--interface <INTERFACE>
— The interface type, which describes the visibility of the endpoint. Value is: -public. Visible by end users on a publicly available network interface. -internal. Visible by end users on an unmetered internal network interface.-admin. Visible by administrative users on a secure network interfacePossible values:
admin
,internal
,public
-
--region-id <REGION_ID>
— (Since v3.2) The ID of the region that contains the service endpoint -
--service-id <SERVICE_ID>
— The UUID of the service to which the endpoint belongs
osc identity endpoint set
Updates an endpoint.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/endpoint
Usage: osc identity endpoint set [OPTIONS] <ID>
Arguments:
<ID>
— endpoint_id parameter for /v3/endpoints/{endpoint_id} API
Options:
-
--description <DESCRIPTION>
— A description of the endpoint -
--enabled <ENABLED>
— Indicates whether the endpoint appears in the service catalog -false. The endpoint does not appear in the service catalog. -true. The endpoint appears in the service catalogPossible values:
true
,false
-
--interface <INTERFACE>
— The interface type, which describes the visibility of the endpoint. Value is: -public
. Visible by end users on a publicly available network interface. -internal
. Visible by end users on an unmetered internal network interface. -admin
. Visible by administrative users on a secure network interfacePossible values:
admin
,internal
,public
-
--name <NAME>
— The name of the endpoint -
--region <REGION>
— (Deprecated in v3.2) The geographic location of the service endpoint -
--region-id <REGION_ID>
— (Since v3.2) The ID of the region that contains the service endpoint -
--service-id <SERVICE_ID>
— The UUID of the service to which the endpoint belongs -
--url <URL>
— The endpoint URL
osc identity endpoint show
Shows details for an endpoint.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/endpoints
Usage: osc identity endpoint show <ID>
Arguments:
<ID>
— endpoint_id parameter for /v3/endpoints/{endpoint_id} API
osc identity endpoint-filter
OS-EP-FILTER API
This API enables the creation of custom catalogs using project scope. The result is the ability to advertise specific endpoints based on the project in use. The association can be done two different ways. The first is by building a direct association between the project and the endpoint, which implies that all tokens scoped to that particular project will receive a specific endpoint, or set of endpoints, in the service catalog. The second is by creating an endpoint group. An endpoint group is a filter that consists of at least one endpoint attribute. By associating a project to an endpoint group, all service catalogs scoped to that project will contain endpoints that match the attributes defined in the endpoint group. Using endpoint groups is a way to dynamically associate an endpoint, or a group of endpoints, to a specific project.
Usage: osc identity endpoint-filter <COMMAND>
Available subcommands:
osc identity endpoint-filter endpoint
— Endpoint project APIosc identity endpoint-filter endpoint-group
— EndpointGroup project APIosc identity endpoint-filter project
— Endpoint project API
osc identity endpoint-filter endpoint
Endpoint project API
Usage: osc identity endpoint-filter endpoint <COMMAND>
Available subcommands:
osc identity endpoint-filter endpoint project
— Endpoint project API
osc identity endpoint-filter endpoint project
Endpoint project API
Usage: osc identity endpoint-filter endpoint project <COMMAND>
Available subcommands:
osc identity endpoint-filter endpoint project list
— Return a list of projects associated with the endpoint
osc identity endpoint-filter endpoint project list
Return a list of projects associated with the endpoint
Usage: osc identity endpoint-filter endpoint project list <ENDPOINT_ID>
Arguments:
<ENDPOINT_ID>
— endpoint_id parameter for /v3/OS-EP-FILTER/endpoints/{endpoint_id}/projects API
osc identity endpoint-filter endpoint-group
EndpointGroup project API
Usage: osc identity endpoint-filter endpoint-group <COMMAND>
Available subcommands:
osc identity endpoint-filter endpoint-group create
— Create new endpoint groupsosc identity endpoint-filter endpoint-group delete
— DELETE operation on /v3/OS-EP-FILTER/endpoint_groups/{endpoint_group_id}osc identity endpoint-filter endpoint-group endpoint
— Endpoint project APIosc identity endpoint-filter endpoint-group list
— List all endpoint groupsosc identity endpoint-filter endpoint-group project
— Project project APIosc identity endpoint-filter endpoint-group set
— Update existing endpoint groupsosc identity endpoint-filter endpoint-group show
— Get Endpoint Group
osc identity endpoint-filter endpoint-group create
Create new endpoint groups.
POST /v3/OS-EP-FILTER/endpoint_groups
Usage: osc identity endpoint-filter endpoint-group create [OPTIONS] --name <NAME> <--enabled <ENABLED>|--interface <INTERFACE>|--region-id <REGION_ID>|--service-id <SERVICE_ID>>
Options:
-
--description <DESCRIPTION>
— The endpoint group description -
--enabled <ENABLED>
— Indicates whether the endpoint appears in the service catalog -false. The endpoint does not appear in the service catalog. -true. The endpoint appears in the service catalogPossible values:
true
,false
-
--interface <INTERFACE>
— The interface type, which describes the visibility of the endpoint. Value is: -public. Visible by end users on a publicly available network interface. -internal. Visible by end users on an unmetered internal network interface. -admin. Visible by administrative users on a secure network interfacePossible values:
admin
,internal
,public
-
--region-id <REGION_ID>
— (Since v3.2) The ID of the region that contains the service endpoint -
--service-id <SERVICE_ID>
— The UUID of the service to which the endpoint belongs -
--name <NAME>
— The name of the endpoint group
osc identity endpoint-filter endpoint-group delete
DELETE operation on /v3/OS-EP-FILTER/endpoint_groups/{endpoint_group_id}
Usage: osc identity endpoint-filter endpoint-group delete <ID>
Arguments:
<ID>
— endpoint_group_id parameter for /v3/OS-EP-FILTER/endpoint_groups/{endpoint_group_id} API
osc identity endpoint-filter endpoint-group endpoint
Endpoint project API
Usage: osc identity endpoint-filter endpoint-group endpoint <COMMAND>
Available subcommands:
osc identity endpoint-filter endpoint-group endpoint list
— GET operation on /v3/OS-EP-FILTER/endpoint_groups/{endpoint_group_id}/endpoints
osc identity endpoint-filter endpoint-group endpoint list
GET operation on /v3/OS-EP-FILTER/endpoint_groups/{endpoint_group_id}/endpoints
Usage: osc identity endpoint-filter endpoint-group endpoint list <ENDPOINT_GROUP_ID>
Arguments:
<ENDPOINT_GROUP_ID>
— endpoint_group_id parameter for /v3/OS-EP-FILTER/endpoint_groups/{endpoint_group_id}/endpoints API
osc identity endpoint-filter endpoint-group list
List all endpoint groups.
GET /v3/OS-EP-FILTER/endpoint_groups
Usage: osc identity endpoint-filter endpoint-group list [OPTIONS]
Options:
--name <NAME>
— The name of the endpoint group
osc identity endpoint-filter endpoint-group project
Project project API
Usage: osc identity endpoint-filter endpoint-group project <COMMAND>
Available subcommands:
osc identity endpoint-filter endpoint-group project delete
— DELETE operation on /v3/OS-EP-FILTER/endpoint_groups/{endpoint_group_id}/projects/{project_id}osc identity endpoint-filter endpoint-group project list
— GET operation on /v3/OS-EP-FILTER/endpoint_groups/{endpoint_group_id}/projectsosc identity endpoint-filter endpoint-group project set
— PUT operation on /v3/OS-EP-FILTER/endpoint_groups/{endpoint_group_id}/projects/{project_id}osc identity endpoint-filter endpoint-group project show
— GET operation on /v3/OS-EP-FILTER/endpoint_groups/{endpoint_group_id}/projects/{project_id}
osc identity endpoint-filter endpoint-group project delete
DELETE operation on /v3/OS-EP-FILTER/endpoint_groups/{endpoint_group_id}/projects/{project_id}
Usage: osc identity endpoint-filter endpoint-group project delete <--project-name <PROJECT_NAME>|--project-id <PROJECT_ID>|--current-project> <ENDPOINT_GROUP_ID>
Arguments:
<ENDPOINT_GROUP_ID>
— endpoint_group_id parameter for /v3/OS-EP-FILTER/endpoint_groups/{endpoint_group_id}/projects/{project_id} API
Options:
--project-name <PROJECT_NAME>
— Project Name--project-id <PROJECT_ID>
— Project ID--current-project
— Current project
osc identity endpoint-filter endpoint-group project list
GET operation on /v3/OS-EP-FILTER/endpoint_groups/{endpoint_group_id}/projects
Usage: osc identity endpoint-filter endpoint-group project list <ENDPOINT_GROUP_ID>
Arguments:
<ENDPOINT_GROUP_ID>
— endpoint_group_id parameter for /v3/OS-EP-FILTER/endpoint_groups/{endpoint_group_id}/projects/{project_id} API
osc identity endpoint-filter endpoint-group project set
PUT operation on /v3/OS-EP-FILTER/endpoint_groups/{endpoint_group_id}/projects/{project_id}
Usage: osc identity endpoint-filter endpoint-group project set [OPTIONS] <--project-name <PROJECT_NAME>|--project-id <PROJECT_ID>|--current-project> <ENDPOINT_GROUP_ID>
Arguments:
<ENDPOINT_GROUP_ID>
— endpoint_group_id parameter for /v3/OS-EP-FILTER/endpoint_groups/{endpoint_group_id}/projects/{project_id} API
Options:
--project-name <PROJECT_NAME>
— Project Name--project-id <PROJECT_ID>
— Project ID--current-project
— Current project--property <key=value>
osc identity endpoint-filter endpoint-group project show
GET operation on /v3/OS-EP-FILTER/endpoint_groups/{endpoint_group_id}/projects/{project_id}
Usage: osc identity endpoint-filter endpoint-group project show <--project-name <PROJECT_NAME>|--project-id <PROJECT_ID>|--current-project> <ENDPOINT_GROUP_ID>
Arguments:
<ENDPOINT_GROUP_ID>
— endpoint_group_id parameter for /v3/OS-EP-FILTER/endpoint_groups/{endpoint_group_id}/projects/{project_id} API
Options:
--project-name <PROJECT_NAME>
— Project Name--project-id <PROJECT_ID>
— Project ID--current-project
— Current project
osc identity endpoint-filter endpoint-group set
Update existing endpoint groups
PATCH /v3/OS-EP-FILTER/endpoint_groups/{endpoint_group_id}
Usage: osc identity endpoint-filter endpoint-group set [OPTIONS] <ID>
Arguments:
<ID>
— endpoint_group_id parameter for /v3/OS-EP-FILTER/endpoint_groups/{endpoint_group_id} API
Options:
-
--description <DESCRIPTION>
— The endpoint group description -
--enabled <ENABLED>
— Indicates whether the endpoint appears in the service catalog -false. The endpoint does not appear in the service catalog. -true. The endpoint appears in the service catalogPossible values:
true
,false
-
--interface <INTERFACE>
— The interface type, which describes the visibility of the endpoint. Value is: -public. Visible by end users on a publicly available network interface. -internal. Visible by end users on an unmetered internal network interface. -admin. Visible by administrative users on a secure network interfacePossible values:
admin
,internal
,public
-
--region-id <REGION_ID>
— (Since v3.2) The ID of the region that contains the service endpoint -
--service-id <SERVICE_ID>
— The UUID of the service to which the endpoint belongs -
--name <NAME>
— The name of the endpoint group
osc identity endpoint-filter endpoint-group show
Get Endpoint Group
GET /v3/OS-EP-FILTER/endpoint_groups/{endpoint_group_id}
Usage: osc identity endpoint-filter endpoint-group show <ID>
Arguments:
<ID>
— endpoint_group_id parameter for /v3/OS-EP-FILTER/endpoint_groups/{endpoint_group_id} API
osc identity endpoint-filter project
Endpoint project API
Usage: osc identity endpoint-filter project <COMMAND>
Available subcommands:
osc identity endpoint-filter project endpoint
— Endpoint project APIosc identity endpoint-filter project endpoint-group
— EndpointGroup project API
osc identity endpoint-filter project endpoint
Endpoint project API
Usage: osc identity endpoint-filter project endpoint <COMMAND>
Available subcommands:
osc identity endpoint-filter project endpoint delete
— DELETE operation on /v3/OS-EP-FILTER/projects/{project_id}/endpoints/{endpoint_id}osc identity endpoint-filter project endpoint list
— GET operation on /v3/OS-EP-FILTER/projects/{project_id}/endpointsosc identity endpoint-filter project endpoint set
— PUT operation on /v3/OS-EP-FILTER/projects/{project_id}/endpoints/{endpoint_id}osc identity endpoint-filter project endpoint show
— GET operation on /v3/OS-EP-FILTER/projects/{project_id}/endpoints/{endpoint_id}
osc identity endpoint-filter project endpoint delete
DELETE operation on /v3/OS-EP-FILTER/projects/{project_id}/endpoints/{endpoint_id}
Usage: osc identity endpoint-filter project endpoint delete <--project-name <PROJECT_NAME>|--project-id <PROJECT_ID>|--current-project> <ID>
Arguments:
<ID>
— endpoint_id parameter for /v3/OS-EP-FILTER/projects/{project_id}/endpoints/{endpoint_id} API
Options:
--project-name <PROJECT_NAME>
— Project Name--project-id <PROJECT_ID>
— Project ID--current-project
— Current project
osc identity endpoint-filter project endpoint list
GET operation on /v3/OS-EP-FILTER/projects/{project_id}/endpoints
Usage: osc identity endpoint-filter project endpoint list <--project-name <PROJECT_NAME>|--project-id <PROJECT_ID>|--current-project>
Options:
--project-name <PROJECT_NAME>
— Project Name--project-id <PROJECT_ID>
— Project ID--current-project
— Current project
osc identity endpoint-filter project endpoint set
PUT operation on /v3/OS-EP-FILTER/projects/{project_id}/endpoints/{endpoint_id}
Usage: osc identity endpoint-filter project endpoint set [OPTIONS] <--project-name <PROJECT_NAME>|--project-id <PROJECT_ID>|--current-project> <ID>
Arguments:
<ID>
— endpoint_id parameter for /v3/OS-EP-FILTER/projects/{project_id}/endpoints/{endpoint_id} API
Options:
--project-name <PROJECT_NAME>
— Project Name--project-id <PROJECT_ID>
— Project ID--current-project
— Current project--property <key=value>
osc identity endpoint-filter project endpoint show
GET operation on /v3/OS-EP-FILTER/projects/{project_id}/endpoints/{endpoint_id}
Usage: osc identity endpoint-filter project endpoint show <--project-name <PROJECT_NAME>|--project-id <PROJECT_ID>|--current-project> <ID>
Arguments:
<ID>
— endpoint_id parameter for /v3/OS-EP-FILTER/projects/{project_id}/endpoints/{endpoint_id} API
Options:
--project-name <PROJECT_NAME>
— Project Name--project-id <PROJECT_ID>
— Project ID--current-project
— Current project
osc identity endpoint-filter project endpoint-group
EndpointGroup project API
Usage: osc identity endpoint-filter project endpoint-group <COMMAND>
Available subcommands:
osc identity endpoint-filter project endpoint-group list
— GET operation on /v3/OS-EP-FILTER/projects/{project_id}/endpoint_groups
osc identity endpoint-filter project endpoint-group list
GET operation on /v3/OS-EP-FILTER/projects/{project_id}/endpoint_groups
Usage: osc identity endpoint-filter project endpoint-group list <--project-name <PROJECT_NAME>|--project-id <PROJECT_ID>|--current-project>
Options:
--project-name <PROJECT_NAME>
— Project Name--project-id <PROJECT_ID>
— Project ID--current-project
— Current project
osc identity federation
OS-Federation
Provide the ability for users to manage Identity Providers (IdPs) and establish a set of rules to map federation protocol attributes to Identity API attributes.
Usage: osc identity federation <COMMAND>
Available subcommands:
osc identity federation identity-provider
— Identity Providersosc identity federation mapping
— Mappingsosc identity federation service-provider
— Service Providersosc identity federation saml2-metadata
— A user may retrieve Metadata about an Identity Service acting as an Identity Provider
osc identity federation identity-provider
Identity Providers
An Identity Provider (IdP) is a third party service that is trusted by the Identity API to authenticate identities.
Usage: osc identity federation identity-provider <COMMAND>
Available subcommands:
osc identity federation identity-provider create
— Create an idp resource for federated authenticationosc identity federation identity-provider delete
— DELETE operation on /v3/OS-FEDERATION/identity_providers/{idp_id}osc identity federation identity-provider list
— List all identity providersosc identity federation identity-provider protocol
— Identity provider protocolsosc identity federation identity-provider set
— PATCH operation on /v3/OS-FEDERATION/identity_providers/{idp_id}osc identity federation identity-provider show
— Get an IDP resource
osc identity federation identity-provider create
Create an idp resource for federated authentication.
PUT /OS-FEDERATION/identity_providers/{idp_id}
Usage: osc identity federation identity-provider create [OPTIONS] <IDP_ID>
Arguments:
<IDP_ID>
— idp_id parameter for /v3/OS-FEDERATION/identity_providers/{idp_id} API
Options:
-
--authorization-ttl <AUTHORIZATION_TTL>
— The length of validity in minutes for group memberships carried over through mapping and persisted in the database. If left unset, the default value configured in keystone will be used, if enabled -
--description <DESCRIPTION>
— The identity provider description -
--domain-id <DOMAIN_ID>
— The ID of a domain that is associated with the identity provider. Federated users that authenticate with the identity provider will be created under the domain specified -
--enabled <ENABLED>
— Whether the identity provider is enabled or notPossible values:
true
,false
-
--remote-ids <REMOTE_IDS>
— List of the unique identity provider's remote IDsParameter is an array, may be provided multiple times.
osc identity federation identity-provider delete
DELETE operation on /v3/OS-FEDERATION/identity_providers/{idp_id}
Usage: osc identity federation identity-provider delete <IDP_ID>
Arguments:
<IDP_ID>
— idp_id parameter for /v3/OS-FEDERATION/identity_providers/{idp_id} API
osc identity federation identity-provider list
List all identity providers.
GET/HEAD /OS-FEDERATION/identity_providers
Usage: osc identity federation identity-provider list [OPTIONS]
Options:
-
--enabled <ENABLED>
— Whether the identity provider is enabled or notPossible values:
true
,false
-
--id <ID>
— The identity provider ID
osc identity federation identity-provider protocol
Identity provider protocols
A protocol entry contains information that dictates which mapping rules to use for a given incoming request. An IdP may have multiple supported protocols.
Required attributes:
- mapping_id (string): Indicates which mapping should be used to process federated authentication requests.
Optional attributes:
- remote_id_attribute (string): Key to obtain the entity ID of the Identity Provider from the HTTPD environment. For mod_shib, this would be Shib-Identity-Provider. For mod_auth_openidc, this could be HTTP_OIDC_ISS. For mod_auth_mellon, this could be MELLON_IDP. This overrides the default value provided in keystone.conf.
Usage: osc identity federation identity-provider protocol <COMMAND>
Available subcommands:
osc identity federation identity-provider protocol create
— Create protocol for an IDPosc identity federation identity-provider protocol delete
— Delete a protocol from an IDPosc identity federation identity-provider protocol list
— List protocols for an IDPosc identity federation identity-provider protocol set
— Update protocol for an IDPosc identity federation identity-provider protocol show
— Get protocols for an IDP
osc identity federation identity-provider protocol create
Create protocol for an IDP.
PUT /OS-Federation/identity_providers/{idp_id}/protocols/{protocol_id}
Usage: osc identity federation identity-provider protocol create [OPTIONS] --mapping-id <MAPPING_ID> <IDP_ID> <ID>
Arguments:
<IDP_ID>
— idp_id parameter for /v3/OS-FEDERATION/identity_providers/{idp_id}/protocols API<ID>
— protocol_id parameter for /v3/OS-FEDERATION/identity_providers/{idp_id}/protocols/{protocol_id} API
Options:
--mapping-id <MAPPING_ID>
--remote-id-attribute <REMOTE_ID_ATTRIBUTE>
osc identity federation identity-provider protocol delete
Delete a protocol from an IDP.
DELETE /OS-FEDERATION/identity_providers/ {idp_id}/protocols/{protocol_id}
Usage: osc identity federation identity-provider protocol delete <IDP_ID> <ID>
Arguments:
<IDP_ID>
— idp_id parameter for /v3/OS-FEDERATION/identity_providers/{idp_id}/protocols API<ID>
— protocol_id parameter for /v3/OS-FEDERATION/identity_providers/{idp_id}/protocols/{protocol_id} API
osc identity federation identity-provider protocol list
List protocols for an IDP.
HEAD/GET /OS-FEDERATION/identity_providers/{idp_id}/protocols
Usage: osc identity federation identity-provider protocol list <IDP_ID>
Arguments:
<IDP_ID>
— idp_id parameter for /v3/OS-FEDERATION/identity_providers/{idp_id}/protocols API
osc identity federation identity-provider protocol set
Update protocol for an IDP.
PATCH /OS-FEDERATION/identity_providers/ {idp_id}/protocols/{protocol_id}
Usage: osc identity federation identity-provider protocol set [OPTIONS] --mapping-id <MAPPING_ID> <IDP_ID> <ID>
Arguments:
<IDP_ID>
— idp_id parameter for /v3/OS-FEDERATION/identity_providers/{idp_id}/protocols API<ID>
— protocol_id parameter for /v3/OS-FEDERATION/identity_providers/{idp_id}/protocols/{protocol_id} API
Options:
--mapping-id <MAPPING_ID>
--remote-id-attribute <REMOTE_ID_ATTRIBUTE>
osc identity federation identity-provider protocol show
Get protocols for an IDP.
HEAD/GET /OS-FEDERATION/identity_providers/ {idp_id}/protocols/{protocol_id}
Usage: osc identity federation identity-provider protocol show <IDP_ID> <ID>
Arguments:
<IDP_ID>
— idp_id parameter for /v3/OS-FEDERATION/identity_providers/{idp_id}/protocols API<ID>
— protocol_id parameter for /v3/OS-FEDERATION/identity_providers/{idp_id}/protocols/{protocol_id} API
osc identity federation identity-provider set
PATCH operation on /v3/OS-FEDERATION/identity_providers/{idp_id}
Usage: osc identity federation identity-provider set [OPTIONS] <IDP_ID>
Arguments:
<IDP_ID>
— idp_id parameter for /v3/OS-FEDERATION/identity_providers/{idp_id} API
Options:
-
--authorization-ttl <AUTHORIZATION_TTL>
— The length of validity in minutes for group memberships carried over through mapping and persisted in the database. If left unset, the default value configured in keystone will be used, if enabled -
--description <DESCRIPTION>
— The identity provider description -
--enabled <ENABLED>
— Whether the identity provider is enabled or notPossible values:
true
,false
-
--remote-ids <REMOTE_IDS>
— List of the unique identity provider's remote IDsParameter is an array, may be provided multiple times.
osc identity federation identity-provider show
Get an IDP resource.
GET/HEAD /OS-FEDERATION/identity_providers/{idp_id}
Usage: osc identity federation identity-provider show <IDP_ID>
Arguments:
<IDP_ID>
— idp_id parameter for /v3/OS-FEDERATION/identity_providers/{idp_id} API
osc identity federation mapping
Mappings
A mapping is a set of rules to map federation protocol attributes to Identity API objects. An Identity Provider can have a single mapping specified per protocol. A mapping is simply a list of rules.
Usage: osc identity federation mapping <COMMAND>
Available subcommands:
osc identity federation mapping create
— Create a mappingosc identity federation mapping delete
— Delete a mappingosc identity federation mapping list
— GET operation on /v3/OS-FEDERATION/mappingsosc identity federation mapping set
— Update an attribute mapping for identity federationosc identity federation mapping show
— GET operation on /v3/OS-FEDERATION/mappings/{mapping_id}
osc identity federation mapping create
Create a mapping.
PUT /OS-FEDERATION/mappings/{mapping_id}
Usage: osc identity federation mapping create [OPTIONS] <ID>
Arguments:
<ID>
— mapping_id parameter for /v3/OS-FEDERATION/mappings/{mapping_id} API
Options:
--rules <JSON>
— Parameter is an array, may be provided multiple times--schema-version <SCHEMA_VERSION>
— Mapping schema version
osc identity federation mapping delete
Delete a mapping.
DELETE /OS-FEDERATION/mappings/{mapping_id}
Usage: osc identity federation mapping delete <ID>
Arguments:
<ID>
— mapping_id parameter for /v3/OS-FEDERATION/mappings/{mapping_id} API
osc identity federation mapping list
GET operation on /v3/OS-FEDERATION/mappings
Usage: osc identity federation mapping list
osc identity federation mapping set
Update an attribute mapping for identity federation.
PATCH /OS-FEDERATION/mappings/{mapping_id}
Usage: osc identity federation mapping set [OPTIONS] <ID>
Arguments:
<ID>
— mapping_id parameter for /v3/OS-FEDERATION/mappings/{mapping_id} API
Options:
--rules <JSON>
— Parameter is an array, may be provided multiple times--schema-version <SCHEMA_VERSION>
— Mapping schema version
osc identity federation mapping show
GET operation on /v3/OS-FEDERATION/mappings/{mapping_id}
Usage: osc identity federation mapping show <ID>
Arguments:
<ID>
— mapping_id parameter for /v3/OS-FEDERATION/mappings/{mapping_id} API
osc identity federation service-provider
Service Providers
A service provider is a third party service that is trusted by the Identity Service.
Usage: osc identity federation service-provider <COMMAND>
Available subcommands:
osc identity federation service-provider create
— Create a service providerosc identity federation service-provider delete
— Delete a service providerosc identity federation service-provider list
— List service providersosc identity federation service-provider set
— Update a service providerosc identity federation service-provider show
— Get a service provider
osc identity federation service-provider create
Create a service provider.
PUT /OS-FEDERATION/service_providers/{service_provider_id}
Usage: osc identity federation service-provider create [OPTIONS] --auth-url <AUTH_URL> --sp-url <SP_URL> <ID>
Arguments:
<ID>
— service_provider_id parameter for /v3/OS-FEDERATION/service_providers/{service_provider_id} API
Options:
-
--auth-url <AUTH_URL>
— The URL to authenticate against -
--description <DESCRIPTION>
— The description of the service provider -
--enabled <ENABLED>
— Whether the service provider is enabled or notPossible values:
true
,false
-
--relay-state-prefix <RELAY_STATE_PREFIX>
— The prefix of the RelayState SAML attribute -
--sp-url <SP_URL>
— The service provider's URL
osc identity federation service-provider delete
Delete a service provider.
DELETE /OS-FEDERATION/service_providers/{service_provider_id}
Usage: osc identity federation service-provider delete <ID>
Arguments:
<ID>
— service_provider_id parameter for /v3/OS-FEDERATION/service_providers/{service_provider_id} API
osc identity federation service-provider list
List service providers.
GET/HEAD /OS-FEDERATION/service_providers
Usage: osc identity federation service-provider list [OPTIONS]
Options:
-
--enabled <ENABLED>
— Whether the service provider is enabled or notPossible values:
true
,false
-
--id <ID>
— The service provider ID
osc identity federation service-provider set
Update a service provider.
PATCH /OS-FEDERATION/service_providers/{service_provider_id}
Usage: osc identity federation service-provider set [OPTIONS] <ID>
Arguments:
<ID>
— service_provider_id parameter for /v3/OS-FEDERATION/service_providers/{service_provider_id} API
Options:
-
--auth-url <AUTH_URL>
— The URL to authenticate against -
--description <DESCRIPTION>
— The description of the service provider -
--enabled <ENABLED>
— Whether the service provider is enabled or notPossible values:
true
,false
-
--relay-state-prefix <RELAY_STATE_PREFIX>
— The prefix of the RelayState SAML attribute -
--sp-url <SP_URL>
— The service provider's URL
osc identity federation service-provider show
Get a service provider.
GET/HEAD /OS-FEDERATION/service_providers/{service_provider_id}
Usage: osc identity federation service-provider show <ID>
Arguments:
<ID>
— service_provider_id parameter for /v3/OS-FEDERATION/service_providers/{service_provider_id} API
osc identity federation saml2-metadata
A user may retrieve Metadata about an Identity Service acting as an Identity Provider.
The response will be a full document with Metadata properties. Note that for readability, this example certificate has been truncated.
Usage: osc identity federation saml2-metadata <COMMAND>
Available subcommands:
osc identity federation saml2-metadata show
— Get SAML2 metadata
osc identity federation saml2-metadata show
Get SAML2 metadata.
GET/HEAD /OS-FEDERATION/saml2/metadata
Usage: osc identity federation saml2-metadata show
osc identity group
Identity Group commands
A group is a collection of users. Each group is owned by a domain.
You can use groups to ease the task of managing role assignments for users. Assigning a role to a group on a project or domain is equivalent to assigning the role to each group member on that project or domain.
When you unassign a role from a group, that role is automatically unassigned from any user that is a member of the group. Any tokens that authenticates those users to the relevant project or domain are revoked.
As with users, a group without any role assignments is useless from the perspective of an OpenStack service and has no access to resources. However, a group without role assignments is permitted as a way of acquiring or loading users and groups from external sources before mapping them to projects and domains.
Usage: osc identity group <COMMAND>
Available subcommands:
osc identity group create
— Create grouposc identity group delete
— Delete grouposc identity group list
— List groupsosc identity group set
— Update grouposc identity group show
— Show group detailsosc identity group user
— Identity User User membership commands
osc identity group create
Creates a group.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/groups
Usage: osc identity group create [OPTIONS] --name <NAME>
Options:
--description <DESCRIPTION>
— The description of the group--domain-id <DOMAIN_ID>
— The ID of the domain of the group. If the domain ID is not provided in the request, the Identity service will attempt to pull the domain ID from the token used in the request. Note that this requires the use of a domain-scoped token--name <NAME>
— The name of the group
osc identity group delete
Deletes a group.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/group
Usage: osc identity group delete <ID>
Arguments:
<ID>
— group_id parameter for /v3/groups/{group_id} API
osc identity group list
Lists groups.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/groups
Usage: osc identity group list [OPTIONS]
Options:
-
--domain-name <DOMAIN_NAME>
— Domain Name -
--domain-id <DOMAIN_ID>
— Domain ID -
--current-domain
— Current domain -
--limit <LIMIT>
-
--marker <MARKER>
— ID of the last fetched entry -
--name <NAME>
— The resource name -
--sort-dir <SORT_DIR>
— Sort direction. A valid value is asc (ascending) or desc (descending)Possible values:
asc
,desc
-
--sort-key <SORT_KEY>
— Sorts resources by attribute -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc identity group set
Updates a group.
If the back-end driver does not support this functionality, the call returns the Not Implemented (501)
response code.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/group
Usage: osc identity group set [OPTIONS] <ID>
Arguments:
<ID>
— group_id parameter for /v3/groups/{group_id} API
Options:
--description <DESCRIPTION>
— The new description of the group--name <NAME>
— The new name of the group
osc identity group show
Shows details for a group.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/group
Usage: osc identity group show <ID>
Arguments:
<ID>
— group_id parameter for /v3/groups/{group_id} API
osc identity group user
Identity User User membership commands
Usage: osc identity group user <COMMAND>
Available subcommands:
osc identity group user delete
— Remove user from grouposc identity group user list
— List users in grouposc identity group user set
— Add user to grouposc identity group user show
— Check if a user is in a group
osc identity group user delete
Removes a user from a group.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/group_user
Usage: osc identity group user delete <--user-name <USER_NAME>|--user-id <USER_ID>|--current-user> <GROUP_ID>
Arguments:
<GROUP_ID>
— group_id parameter for /v3/groups/{group_id}/users/{user_id} API
Options:
--user-name <USER_NAME>
— User Name--user-id <USER_ID>
— User ID--current-user
— Current authenticated user
osc identity group user list
Lists the users that belong to a group.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/group_users
Usage: osc identity group user list [OPTIONS] <GROUP_ID>
Arguments:
<GROUP_ID>
— group_id parameter for /v3/groups/{group_id}/users/{user_id} API
Options:
--password-expires-at <PASSWORD_EXPIRES_AT>
— Filter results based on which user passwords have expired. The query should include an operator and a timestamp with a colon (:) separating the two, for example:password_expires_at={operator}:{timestamp}
. Valid operators are:lt
,lte
,gt
,gte
,eq
, andneq
. Valid timestamps are of the form: YYYY-MM-DDTHH:mm:ssZ
osc identity group user set
Adds a user to a group.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/group_user
Usage: osc identity group user set <--user-name <USER_NAME>|--user-id <USER_ID>|--current-user> <GROUP_ID>
Arguments:
<GROUP_ID>
— group_id parameter for /v3/groups/{group_id}/users/{user_id} API
Options:
--user-name <USER_NAME>
— User Name--user-id <USER_ID>
— User ID--current-user
— Current authenticated user
osc identity group user show
Check if a user is in a group.
GET/HEAD /groups/{group_id}/users/{user_id}
Usage: osc identity group user show <--user-name <USER_NAME>|--user-id <USER_ID>|--current-user> <GROUP_ID>
Arguments:
<GROUP_ID>
— group_id parameter for /v3/groups/{group_id}/users/{user_id} API
Options:
--user-name <USER_NAME>
— User Name--user-id <USER_ID>
— User ID--current-user
— Current authenticated user
osc identity limit
Unified Limits
In OpenStack, a quota system mainly contains two parts: limit and usage. The Unified limits in Keystone is a replacement of the limit part. It contains two kinds of resources: Registered Limit and Limit. A registered limit is a default limit. It is usually created by the services which are registered in Keystone. A limit is the limit that override the registered limit for each project.
Usage: osc identity limit <COMMAND>
Available subcommands:
osc identity limit create
— Create Limitsosc identity limit delete
— Delete Limitosc identity limit list
— List Limitsosc identity limit model
— Get Enforcement Modelosc identity limit set
— Update Limitosc identity limit show
— Show Limit Details
osc identity limit create
Creates limits. It supports to create more than one limit in one request.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/limits
Usage: osc identity limit create [OPTIONS]
Options:
-
--limits <JSON>
— A list oflimits
objectsParameter is an array, may be provided multiple times.
osc identity limit delete
Deletes a limit.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/limit
Usage: osc identity limit delete <ID>
Arguments:
<ID>
— limit_id parameter for /v3/limits/{limit_id} API
osc identity limit list
Lists Limits.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/limits
Usage: osc identity limit list [OPTIONS]
Options:
--domain-name <DOMAIN_NAME>
— Domain Name--domain-id <DOMAIN_ID>
— Domain ID--current-domain
— Current domain--project-name <PROJECT_NAME>
— Project Name--project-id <PROJECT_ID>
— Project ID--current-project
— Current project--region-id <REGION_ID>
— The ID of the region--resource-name <RESOURCE_NAME>
— The resource name--service-id <SERVICE_ID>
— Filters the response by a service ID
osc identity limit model
Return the configured limit enforcement model.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/limit_model
Usage: osc identity limit model
osc identity limit set
Updates the specified limit. It only supports to update resource_limit
or description
for the limit.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/limit
Usage: osc identity limit set [OPTIONS] <ID>
Arguments:
<ID>
— limit_id parameter for /v3/limits/{limit_id} API
Options:
--description <DESCRIPTION>
— The limit description--resource-limit <RESOURCE_LIMIT>
— The override limit
osc identity limit show
Shows details for a limit.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/limit
Usage: osc identity limit show <ID>
Arguments:
<ID>
— limit_id parameter for /v3/limits/{limit_id} API
osc identity project
Projects
A project is the base unit of resource ownership. Resources are owned by a specific project. A project is owned by a specific domain.
(Since Identity API v3.4) You can create a hierarchy of projects by setting a parent_id when you create a project. All projects in a hierarchy must be owned by the same domain.
(Since Identity API v3.6) Projects may, in addition to acting as containers for OpenStack resources, act as a domain (by setting the attribute is_domain to true), in which case it provides a namespace in which users, groups and other projects can be created. In fact, a domain created using the POST /domains API will actually be represented as a project with is_domain set to true with no parent (parent_id is null).
Given this, all projects are considered part of a project hierarchy. Projects created in a domain prior to v3.6 are represented as a two-level hierarchy, with a project that has is_domain set to true as the root and all other projects referencing the root as their parent.
A project acting as a domain can potentially also act as a container for OpenStack resources, although this depends on whether the policy rule for the relevant resource creation allows this.
Note
A project’s name must be unique within a domain and no more than 64 characters. A project’s name must be able to be sent within valid JSON, which could be any UTF-8 character. However, this is constrained to the given backend where project names are stored. For instance, MySQL’s restrictions states that UTF-8 support is constrained to the characters in the Basic Multilingual Plane (BMP). Supplementary characters are not permitted. Note that this last restriction is generally true for all names within resources of the Identity API. Creating a project without using a domain scoped token, i.e. using a project scoped token or a system scoped token, and also without specifying a domain or domain_id, the project will automatically be created on the default domain.
Usage: osc identity project <COMMAND>
Available subcommands:
osc identity project create
— Create projectosc identity project delete
— Delete projectosc identity project group
— Project Group commandsosc identity project list
— List projectsosc identity project set
— Update projectosc identity project show
— Show project detailsosc identity project user
— Project User commands
osc identity project create
Creates a project, where the project may act as a domain.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/projects
Usage: osc identity project create [OPTIONS] --name <NAME>
Options:
-
--description <DESCRIPTION>
— The description of the project -
--domain-id <DOMAIN_ID>
— The ID of the domain for the project.For projects acting as a domain, the
domain_id
must not be specified, it will be generated by the Identity service implementation.For regular projects (i.e. those not acing as a domain), if
domain_id
is not specified, butparent_id
is specified, then the domain ID of the parent will be used. If neitherdomain_id
orparent_id
is specified, the Identity service implementation will default to the domain to which the client’s token is scoped. If bothdomain_id
andparent_id
are specified, and they do not indicate the same domain, anBad Request (400)
will be returned. -
--enabled <ENABLED>
— If set totrue
, project is enabled. If set tofalse
, project is disabled. The default istrue
Possible values:
true
,false
-
--is-domain <IS_DOMAIN>
— If set totrue
, project is enabled. If set tofalse
, project is disabled. The default istrue
Possible values:
true
,false
-
--name <NAME>
— The name of the project, which must be unique within the owning domain. A project can have the same name as its domain -
--immutable <IMMUTABLE>
Possible values:
true
,false
-
--parent-id <PARENT_ID>
— The ID of the parent of the project.If specified on project creation, this places the project within a hierarchy and implicitly defines the owning domain, which will be the same domain as the parent specified. If
parent_id
is not specified andis_domain
isfalse
, then the project will use its owning domain as its parent. Ifis_domain
istrue
(i.e. the project is acting as a domain), thenparent_id
must not specified (or if it is, it must benull
) since domains have no parents.parent_id
is immutable, and can’t be updated after the project is created - hence a project cannot be moved within the hierarchy.New in version 3.4
-
--tags <TAGS>
— A list of simple strings assigned to a project. Tags can be used to classify projects into groups.Parameter is an array, may be provided multiple times.
osc identity project delete
Deletes a project.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/project
Usage: osc identity project delete <ID>
Arguments:
<ID>
— project_id parameter for /v3/projects/{project_id} API
osc identity project group
Project Group commands
This command allows managing of the user group roles on the project
Usage: osc identity project group <COMMAND>
Available subcommands:
osc identity project group role
— Identity Project User Group Role commands
osc identity project group role
Identity Project User Group Role commands
This command allows managing of the user roles on the project
Usage: osc identity project group role <COMMAND>
Available subcommands:
osc identity project group role delete
— Unassign role from group on projectosc identity project group role list
— List role assignments for group on projectosc identity project group role set
— Assign role to group on projectosc identity project group role show
— Check grant for project, group, role
osc identity project group role delete
Unassigns a role from a group on a project.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/project_group_role
Usage: osc identity project group role delete <--project-name <PROJECT_NAME>|--project-id <PROJECT_ID>|--current-project> <GROUP_ID> <ID>
Arguments:
<GROUP_ID>
— group_id parameter for /v3/projects/{project_id}/groups/{group_id}/roles API<ID>
— role_id parameter for /v3/projects/{project_id}/groups/{group_id}/roles/{role_id} API
Options:
--project-name <PROJECT_NAME>
— Project Name--project-id <PROJECT_ID>
— Project ID--current-project
— Current project
osc identity project group role list
Lists role assignments for a group on a project.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/project_user_role
Usage: osc identity project group role list <--project-name <PROJECT_NAME>|--project-id <PROJECT_ID>|--current-project> <GROUP_ID>
Arguments:
<GROUP_ID>
— group_id parameter for /v3/projects/{project_id}/groups/{group_id}/roles API
Options:
--project-name <PROJECT_NAME>
— Project Name--project-id <PROJECT_ID>
— Project ID--current-project
— Current project
osc identity project group role set
Assigns a role to a group on a project.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/project_group_role
Usage: osc identity project group role set <--project-name <PROJECT_NAME>|--project-id <PROJECT_ID>|--current-project> <GROUP_ID> <ID>
Arguments:
<GROUP_ID>
— group_id parameter for /v3/projects/{project_id}/groups/{group_id}/roles API<ID>
— role_id parameter for /v3/projects/{project_id}/groups/{group_id}/roles/{role_id} API
Options:
--project-name <PROJECT_NAME>
— Project Name--project-id <PROJECT_ID>
— Project ID--current-project
— Current project
osc identity project group role show
Check grant for project, group, role.
GET/HEAD /v3/projects/{project_id/groups/{group_id}/roles/{role_id}
Usage: osc identity project group role show <--project-name <PROJECT_NAME>|--project-id <PROJECT_ID>|--current-project> <GROUP_ID> <ID>
Arguments:
<GROUP_ID>
— group_id parameter for /v3/projects/{project_id}/groups/{group_id}/roles API<ID>
— role_id parameter for /v3/projects/{project_id}/groups/{group_id}/roles/{role_id} API
Options:
--project-name <PROJECT_NAME>
— Project Name--project-id <PROJECT_ID>
— Project ID--current-project
— Current project
osc identity project list
Lists projects.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/projects
Usage: osc identity project list [OPTIONS]
Options:
-
--domain-name <DOMAIN_NAME>
— Domain Name -
--domain-id <DOMAIN_ID>
— Domain ID -
--current-domain
— Current domain -
--enabled <ENABLED>
Possible values:
true
,false
-
--is-domain <IS_DOMAIN>
Possible values:
true
,false
-
--limit <LIMIT>
-
--marker <MARKER>
— ID of the last fetched entry -
--name <NAME>
— The resource name -
--not-tags <NOT_TAGS>
-
--not-tags-any <NOT_TAGS_ANY>
-
--parent-id <PARENT_ID>
-
--tags <TAGS>
-
--tags-any <TAGS_ANY>
-
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc identity project set
Updates a project.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/project
Usage: osc identity project set [OPTIONS] <ID>
Arguments:
<ID>
— project_id parameter for /v3/projects/{project_id} API
Options:
-
--description <DESCRIPTION>
— The description of the project -
--enabled <ENABLED>
— If set totrue
, project is enabled. If set tofalse
, project is disabledPossible values:
true
,false
-
--name <NAME>
— The name of the project, which must be unique within the owning domain. A project can have the same name as its domain -
--immutable <IMMUTABLE>
Possible values:
true
,false
-
--tags <TAGS>
— A list of simple strings assigned to a project. Tags can be used to classify projects into groups.Parameter is an array, may be provided multiple times.
osc identity project show
Shows details for a project.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/project
Usage: osc identity project show <ID>
Arguments:
<ID>
— project_id parameter for /v3/projects/{project_id} API
osc identity project user
Project User commands
This command allows managing of the user roles on the project
Usage: osc identity project user <COMMAND>
Available subcommands:
osc identity project user role
— Identity Project User Role commands
osc identity project user role
Identity Project User Role commands
This command allows managing of the user roles on the project
Usage: osc identity project user role <COMMAND>
Available subcommands:
osc identity project user role delete
— Unassign role from user on projectosc identity project user role list
— List role assignments for user on projectosc identity project user role set
— Assign role to user on projectosc identity project user role show
— Check grant for project, user, role
osc identity project user role delete
Unassigns a role from a user on a project.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/project_user_role
Usage: osc identity project user role delete <--project-name <PROJECT_NAME>|--project-id <PROJECT_ID>|--current-project> <--user-name <USER_NAME>|--user-id <USER_ID>|--current-user> <ID>
Arguments:
<ID>
— role_id parameter for /v3/projects/{project_id}/users/{user_id}/roles/{role_id} API
Options:
--project-name <PROJECT_NAME>
— Project Name--project-id <PROJECT_ID>
— Project ID--current-project
— Current project--user-name <USER_NAME>
— User Name--user-id <USER_ID>
— User ID--current-user
— Current authenticated user
osc identity project user role list
Lists role assignments for a user on a project.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/project_user_role
Usage: osc identity project user role list <--project-name <PROJECT_NAME>|--project-id <PROJECT_ID>|--current-project> <--user-name <USER_NAME>|--user-id <USER_ID>|--current-user>
Options:
--project-name <PROJECT_NAME>
— Project Name--project-id <PROJECT_ID>
— Project ID--current-project
— Current project--user-name <USER_NAME>
— User Name--user-id <USER_ID>
— User ID--current-user
— Current authenticated user
osc identity project user role set
Assigns a role to a user on a project.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/project_user_role
Usage: osc identity project user role set <--project-name <PROJECT_NAME>|--project-id <PROJECT_ID>|--current-project> <--user-name <USER_NAME>|--user-id <USER_ID>|--current-user> <ID>
Arguments:
<ID>
— role_id parameter for /v3/projects/{project_id}/users/{user_id}/roles/{role_id} API
Options:
--project-name <PROJECT_NAME>
— Project Name--project-id <PROJECT_ID>
— Project ID--current-project
— Current project--user-name <USER_NAME>
— User Name--user-id <USER_ID>
— User ID--current-user
— Current authenticated user
osc identity project user role show
Check grant for project, user, role.
GET/HEAD /v3/projects/{project_id/users/{user_id}/roles/{role_id}
Usage: osc identity project user role show <--project-name <PROJECT_NAME>|--project-id <PROJECT_ID>|--current-project> <--user-name <USER_NAME>|--user-id <USER_ID>|--current-user> <ID>
Arguments:
<ID>
— role_id parameter for /v3/projects/{project_id}/users/{user_id}/roles/{role_id} API
Options:
--project-name <PROJECT_NAME>
— Project Name--project-id <PROJECT_ID>
— Project ID--current-project
— Current project--user-name <USER_NAME>
— User Name--user-id <USER_ID>
— User ID--current-user
— Current authenticated user
osc identity region
Region commands
A region is a general division of an OpenStack deployment. You can associate zero or more sub-regions with a region to create a tree- like structured hierarchy.
Although a region does not have a geographical connotation, a deployment can use a geographical name for a region ID, such as us- east.
You can list, create, update, show details for, and delete regions.
Usage: osc identity region <COMMAND>
Available subcommands:
osc identity region create
— Create regionosc identity region delete
— Delete regionosc identity region list
— List regionsosc identity region set
— Update regionosc identity region show
— Show region details
osc identity region create
Creates a region.
When you create the region, you can optionally specify a region ID. If you include characters in the region ID that are not allowed in a URI, you must URL-encode the ID. If you omit an ID, the API assigns an ID to the region.
The following errors might occur:
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/regions
Usage: osc identity region create [OPTIONS]
Options:
--description <DESCRIPTION>
— The region description--parent-id <PARENT_ID>
— To make this region a child of another region, set this parameter to the ID of the parent region
osc identity region delete
Deletes a region.
The following error might occur:
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/region
Usage: osc identity region delete <ID>
Arguments:
<ID>
— region_id parameter for /v3/regions/{region_id} API
osc identity region list
Lists regions.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/regions
Usage: osc identity region list [OPTIONS]
Options:
--parent-region-id <PARENT_REGION_ID>
— Filters the response by a parent region, by ID
osc identity region set
Updates a region.
You can update the description or parent region ID for a region. You cannot update the region ID.
The following error might occur:
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/region
Usage: osc identity region set [OPTIONS] <ID>
Arguments:
<ID>
— region_id parameter for /v3/regions/{region_id} API
Options:
--description <DESCRIPTION>
— The region description--parent-id <PARENT_ID>
— To make this region a child of another region, set this parameter to the ID of the parent region
osc identity region show
Shows details for a region, by ID.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/regions
Usage: osc identity region show <ID>
Arguments:
<ID>
— region_id parameter for /v3/regions/{region_id} API
osc identity registered-limit
Unified Limits - Registered limits
In OpenStack, a quota system mainly contains two parts: limit and usage. The Unified limits in Keystone is a replacement of the limit part. It contains two kinds of resources: Registered RegisteredLimit and RegisteredLimit. A registered limit is a default limit. It is usually created by the services which are registered in Keystone. A limit is the limit that override the registered limit for each project.
Usage: osc identity registered-limit <COMMAND>
Available subcommands:
osc identity registered-limit create
— Create Registered Limitsosc identity registered-limit delete
— Delete Registered Limitosc identity registered-limit list
— List Registered Limitsosc identity registered-limit set
— Update Registered Limitosc identity registered-limit show
— Show Registered Limit Details
osc identity registered-limit create
Creates registered limits. It supports to create more than one registered limit in one request.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/registered_limits
Usage: osc identity registered-limit create [OPTIONS]
Options:
-
--registered-limits <JSON>
— A list ofregistered_limits
objectsParameter is an array, may be provided multiple times.
osc identity registered-limit delete
Deletes a registered limit.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/registered_limit
Usage: osc identity registered-limit delete <ID>
Arguments:
<ID>
— registered_limit_id parameter for /v3/registered_limits/{registered_limit_id} API
osc identity registered-limit list
Lists Registered Limits.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/registered_limits
Usage: osc identity registered-limit list [OPTIONS]
Options:
--region-id <REGION_ID>
— The ID of the region--resource-name <RESOURCE_NAME>
— The resource name--service-id <SERVICE_ID>
— Filters the response by a service ID
osc identity registered-limit set
Updates the specified registered limit.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/registered_limit
Usage: osc identity registered-limit set [OPTIONS] <ID>
Arguments:
<ID>
— registered_limit_id parameter for /v3/registered_limits/{registered_limit_id} API
Options:
--default-limit <DEFAULT_LIMIT>
— The default limit for the registered limit--description <DESCRIPTION>
— The registered limit description--region-id <REGION_ID>
— The ID of the region that contains the service endpoint. Either service_id, resource_name, or region_id must be different than existing value otherwise it will raise 409--resource-name <RESOURCE_NAME>
— The resource name. Either service_id, resource_name or region_id must be different than existing value otherwise it will raise 409--service-id <SERVICE_ID>
— The UUID of the service to update to which the registered limit belongs. Either service_id, resource_name, or region_id must be different than existing value otherwise it will raise 409
osc identity registered-limit show
Shows details for a registered limit.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/registered_limit
Usage: osc identity registered-limit show <ID>
Arguments:
<ID>
— registered_limit_id parameter for /v3/registered_limits/{registered_limit_id} API
osc identity role
Identity Role commands
OpenStack services typically determine whether a user’s API request should be allowed using Role Based Access Control (RBAC). For OpenStack this means the service compares the roles that user has on the project (as indicated by the roles in the token), against the roles required for the API in question (as defined in the service’s policy file). A user obtains roles on a project by having these assigned to them via the Identity service API.
Roles must initially be created as entities via the Identity services API and, once created, can then be assigned. You can assign roles to a user or group on a project, including projects owned by other domains. You can also assign roles to a user or group on a domain, although this is only currently relevant for using a domain scoped token to execute domain-level Identity service API requests.
Usage: osc identity role <COMMAND>
Available subcommands:
osc identity role assignment
— Role Assignments commandsosc identity role create
— Create roleosc identity role delete
— Delete roleosc identity role imply
— Identity Implied Imply commandsosc identity role inference
— Role Inferences commandsosc identity role list
— List rolesosc identity role set
— Update roleosc identity role show
— Show role details
osc identity role assignment
Role Assignments commands
Usage: osc identity role assignment <COMMAND>
Available subcommands:
osc identity role assignment list
— List role assignments
osc identity role assignment list
Get a list of role assignments.
If no query parameters are specified, then this API will return a list of all role assignments.
Since this list is likely to be very long, this API would typically always be used with one of more of the filter queries. Some typical examples are:
GET /v3/role_assignments?user.id={user_id}
would list all role assignments involving the specified user.
GET /v3/role_assignments?scope.project.id={project_id}
would list all role assignments involving the specified project.
It is also possible to list all role assignments within a tree of projects: GET /v3/role_assignments?scope.project.id={project_id}&include_subtree=true
would list all role assignments involving the specified project and all sub-projects. include_subtree=true
can only be specified in conjunction with scope.project.id
, specifying it without this will result in an HTTP 400 Bad Request being returned.
Each role assignment entity in the collection contains a link to the assignment that gave rise to this entity.
The scope section in the list response is extended to allow the representation of role assignments that are inherited to projects.
The query filter scope.OS-INHERIT:inherited_to
can be used to filter based on role assignments that are inherited. The only value of scope.OS-INHERIT:inherited_to
that is currently supported is projects
, indicating that this role is inherited to all projects of the owning domain or parent project.
If the query parameter effective
is specified, rather than simply returning a list of role assignments that have been made, the API returns a list of effective assignments at the user, project and domain level, having allowed for the effects of group membership, role inference rules as well as inheritance from the parent domain or project. Since the effects of group membership have already been allowed for, the group role assignment entities themselves will not be returned in the collection. Likewise, since the effects of inheritance have already been allowed for, the role assignment entities themselves that specify the inheritance will also not be returned in the collection. This represents the effective role assignments that would be included in a scoped token. The same set of query parameters can also be used in combination with the effective
parameter.
For example:
GET /v3/role_assignments?user.id={user_id}&effective
would, in other words, answer the question “what can this user actually do?”.
GET /v3/role_assignments?user.id={user_id}&scope.project.id={project_id}&effective
would return the equivalent set of role assignments that would be included in the token response of a project scoped token.
An example response for an API call with the query parameter effective
specified is given below:
The entity links
section of a response using the effective
query parameter also contains, for entities that are included by virtue of group membership, a url that can be used to access the membership of the group.
If the query parameter include_names
is specified, rather than simply returning the entity IDs in the role assignments, the collection will additionally include the names of the entities. For example:
GET /v3/role_assignments?user.id={user_id}&effective&include_names=true
would return:
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/role_assignments
Usage: osc identity role assignment list [OPTIONS]
Options:
--effective <EFFECTIVE>
--group-id <GROUP_ID>
--include-names <INCLUDE_NAMES>
--include-subtree <INCLUDE_SUBTREE>
--role-id <ROLE_ID>
--scope-domain-id <SCOPE_DOMAIN_ID>
— The ID of the domain--scope-os-inherit-inherited-to <SCOPE_OS_INHERIT_INHERITED_TO>
--scope-project-id <SCOPE_PROJECT_ID>
— The ID of the project--scope-system <SCOPE_SYSTEM>
--user-id <USER_ID>
osc identity role create
Creates a role.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/roles
Usage: osc identity role create [OPTIONS] --name <NAME>
Options:
-
--description <DESCRIPTION>
— Add description about the role -
--domain-id <DOMAIN_ID>
— The ID of the domain of the role -
--name <NAME>
— The role name -
--immutable <IMMUTABLE>
Possible values:
true
,false
osc identity role delete
Deletes a role.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/role
Usage: osc identity role delete <ID>
Arguments:
<ID>
— role_id parameter for /v3/roles/{role_id} API
osc identity role imply
Identity Implied Imply commands
Usage: osc identity role imply <COMMAND>
Available subcommands:
osc identity role imply delete
— Delete role inference ruleosc identity role imply list
— List implied (inference) roles for roleosc identity role imply set
— Create role inference ruleosc identity role imply show
— Get role inference rule
osc identity role imply delete
Deletes a role inference rule.
Relationship: https://developer.openstack.org/api-ref/identity/v3/#delete-role-inference-rule
Usage: osc identity role imply delete <PRIOR_ROLE_ID> <IMPLIED_ROLE_ID>
Arguments:
<PRIOR_ROLE_ID>
— prior_role_id parameter for /v3/roles/{prior_role_id}/implies/{implied_role_id} API<IMPLIED_ROLE_ID>
— implied_role_id parameter for /v3/roles/{prior_role_id}/implies/{implied_role_id} API
osc identity role imply list
Lists implied (inference) roles for a role.
Relationship: https://developer.openstack.org/api-ref/identity/v3/#list-implied-roles-for-role
Usage: osc identity role imply list <PRIOR_ROLE_ID>
Arguments:
<PRIOR_ROLE_ID>
— prior_role_id parameter for /v3/roles/{prior_role_id}/implies/{implied_role_id} API
osc identity role imply set
Creates a role inference rule.
Relationship: https://developer.openstack.org/api-ref/identity/v3/#create-role-inference-rule
Usage: osc identity role imply set <PRIOR_ROLE_ID> <IMPLIED_ROLE_ID>
Arguments:
<PRIOR_ROLE_ID>
— prior_role_id parameter for /v3/roles/{prior_role_id}/implies/{implied_role_id} API<IMPLIED_ROLE_ID>
— implied_role_id parameter for /v3/roles/{prior_role_id}/implies/{implied_role_id} API
osc identity role imply show
Gets a role inference rule.
Relationship: https://developer.openstack.org/api-ref/identity/v3/#get-role-inference-rule
Usage: osc identity role imply show <PRIOR_ROLE_ID> <IMPLIED_ROLE_ID>
Arguments:
<PRIOR_ROLE_ID>
— prior_role_id parameter for /v3/roles/{prior_role_id}/implies/{implied_role_id} API<IMPLIED_ROLE_ID>
— implied_role_id parameter for /v3/roles/{prior_role_id}/implies/{implied_role_id} API
osc identity role inference
Role Inferences commands
Operating the role inferences (implied roles)
Usage: osc identity role inference <COMMAND>
Available subcommands:
osc identity role inference list
— List all role inference rules
osc identity role inference list
Lists all role inference rules.
Relationship: https://developer.openstack.org/api-ref/identity/v3/#list-all-role-inference-rules
Usage: osc identity role inference list
osc identity role list
Lists roles.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/roles
Usage: osc identity role list [OPTIONS]
Options:
--domain-name <DOMAIN_NAME>
— Domain Name--domain-id <DOMAIN_ID>
— Domain ID--current-domain
— Current domain--name <NAME>
— The resource name
osc identity role set
Updates a role.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/role
Usage: osc identity role set [OPTIONS] <ID>
Arguments:
<ID>
— role_id parameter for /v3/roles/{role_id} API
Options:
-
--description <DESCRIPTION>
— The new role description -
--domain-id <DOMAIN_ID>
— The ID of the domain -
--name <NAME>
— The new role name -
--immutable <IMMUTABLE>
Possible values:
true
,false
osc identity role show
Shows details for a role.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/role
Usage: osc identity role show <ID>
Arguments:
<ID>
— role_id parameter for /v3/roles/{role_id} API
osc identity role-assignment
Role Assignments commands
Usage: osc identity role-assignment <COMMAND>
Available subcommands:
osc identity role-assignment list
— List role assignments
osc identity role-assignment list
Get a list of role assignments.
If no query parameters are specified, then this API will return a list of all role assignments.
Since this list is likely to be very long, this API would typically always be used with one of more of the filter queries. Some typical examples are:
GET /v3/role_assignments?user.id={user_id}
would list all role assignments involving the specified user.
GET /v3/role_assignments?scope.project.id={project_id}
would list all role assignments involving the specified project.
It is also possible to list all role assignments within a tree of projects: GET /v3/role_assignments?scope.project.id={project_id}&include_subtree=true
would list all role assignments involving the specified project and all sub-projects. include_subtree=true
can only be specified in conjunction with scope.project.id
, specifying it without this will result in an HTTP 400 Bad Request being returned.
Each role assignment entity in the collection contains a link to the assignment that gave rise to this entity.
The scope section in the list response is extended to allow the representation of role assignments that are inherited to projects.
The query filter scope.OS-INHERIT:inherited_to
can be used to filter based on role assignments that are inherited. The only value of scope.OS-INHERIT:inherited_to
that is currently supported is projects
, indicating that this role is inherited to all projects of the owning domain or parent project.
If the query parameter effective
is specified, rather than simply returning a list of role assignments that have been made, the API returns a list of effective assignments at the user, project and domain level, having allowed for the effects of group membership, role inference rules as well as inheritance from the parent domain or project. Since the effects of group membership have already been allowed for, the group role assignment entities themselves will not be returned in the collection. Likewise, since the effects of inheritance have already been allowed for, the role assignment entities themselves that specify the inheritance will also not be returned in the collection. This represents the effective role assignments that would be included in a scoped token. The same set of query parameters can also be used in combination with the effective
parameter.
For example:
GET /v3/role_assignments?user.id={user_id}&effective
would, in other words, answer the question “what can this user actually do?”.
GET /v3/role_assignments?user.id={user_id}&scope.project.id={project_id}&effective
would return the equivalent set of role assignments that would be included in the token response of a project scoped token.
An example response for an API call with the query parameter effective
specified is given below:
The entity links
section of a response using the effective
query parameter also contains, for entities that are included by virtue of group membership, a url that can be used to access the membership of the group.
If the query parameter include_names
is specified, rather than simply returning the entity IDs in the role assignments, the collection will additionally include the names of the entities. For example:
GET /v3/role_assignments?user.id={user_id}&effective&include_names=true
would return:
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/role_assignments
Usage: osc identity role-assignment list [OPTIONS]
Options:
--effective <EFFECTIVE>
--group-id <GROUP_ID>
--include-names <INCLUDE_NAMES>
--include-subtree <INCLUDE_SUBTREE>
--role-id <ROLE_ID>
--scope-domain-id <SCOPE_DOMAIN_ID>
— The ID of the domain--scope-os-inherit-inherited-to <SCOPE_OS_INHERIT_INHERITED_TO>
--scope-project-id <SCOPE_PROJECT_ID>
— The ID of the project--scope-system <SCOPE_SYSTEM>
--user-id <USER_ID>
osc identity role-inference
Role Inferences commands
Operating the role inferences (implied roles)
Usage: osc identity role-inference <COMMAND>
Available subcommands:
osc identity role-inference list
— List all role inference rules
osc identity role-inference list
Lists all role inference rules.
Relationship: https://developer.openstack.org/api-ref/identity/v3/#list-all-role-inference-rules
Usage: osc identity role-inference list
osc identity service
Service commands
A service is an OpenStack web service that you can access through a URL, i.e. an endpoint.
You can create, list, show details for, update, and delete services. When you create or update a service, you can enable the service, which causes it and its endpoints to appear in the service catalog.
Usage: osc identity service <COMMAND>
Available subcommands:
osc identity service create
— Create serviceosc identity service delete
— Delete serviceosc identity service list
— List servicesosc identity service set
— Update serviceosc identity service show
— Show service details
osc identity service create
Creates a service.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/services
Usage: osc identity service create [OPTIONS] --type <TYPE>
Options:
-
--enabled <ENABLED>
— Defines whether the service and its endpoints appear in the service catalog: -false
. The service and its endpoints do not appear in the service catalog. -true
. The service and its endpoints appear in the service catalogPossible values:
true
,false
-
--name <NAME>
— The service name -
--type <TYPE>
— The service type, which describes the API implemented by the service. Value iscompute
,ec2
,identity
,image
,network
, orvolume
osc identity service delete
Deletes a service.
If you try to delete a service that still has associated endpoints, this call either deletes all associated endpoints or fails until all endpoints are deleted.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/service
Usage: osc identity service delete <ID>
Arguments:
<ID>
— service_id parameter for /v3/services/{service_id} API
osc identity service list
Lists all services.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/services
Usage: osc identity service list [OPTIONS]
Options:
--service <SERVICE>
— Filters the response by a domain ID
osc identity service set
Updates a service.
The request body is the same as the create service request body, except that you include only those attributes that you want to update.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/services
Usage: osc identity service set [OPTIONS] <ID>
Arguments:
<ID>
— service_id parameter for /v3/services/{service_id} API
Options:
-
--enabled <ENABLED>
— Defines whether the service and its endpoints appear in the service catalog: -false
. The service and its endpoints do not appear in the service catalog. -true
. The service and its endpoints appear in the service catalog. Default istrue
Possible values:
true
,false
-
--name <NAME>
— The service name -
--type <TYPE>
— The service type, which describes the API implemented by the service. Value iscompute
,ec2
,identity
,image
,network
, orvolume
osc identity service show
Shows details for a service.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/service
Usage: osc identity service show <ID>
Arguments:
<ID>
— service_id parameter for /v3/services/{service_id} API
osc identity user
User commands
A user is an individual API consumer that is owned by a domain. A role explicitly associates a user with projects or domains. A user with no assigned roles has no access to OpenStack resources.
You can list, create, show details for, update, delete, and change the password for users.
You can also list groups, projects, and role assignments for a specified user.
Usage: osc identity user <COMMAND>
Available subcommands:
osc identity user create
— Create userosc identity user delete
— Delete userosc identity user groups
— List groups to which a user belongsosc identity user list
— List usersosc identity user password
— User password commandsosc identity user projects
— List projects for userosc identity user set
— Update userosc identity user show
— Show user details
osc identity user create
Creates a user.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/users
Usage: osc identity user create [OPTIONS] --name <NAME>
Options:
-
--default-project-id <DEFAULT_PROJECT_ID>
— The ID of the default project for the user. A user’s default project must not be a domain. Setting this attribute does not grant any actual authorization on the project, and is merely provided for convenience. Therefore, the referenced project does not need to exist within the user domain. (Since v3.1) If the user does not have authorization to their default project, the default project is ignored at token creation. (Since v3.1) Additionally, if your default project is not valid, a token is issued without an explicit scope of authorization -
--description <DESCRIPTION>
— The resource description -
--domain-id <DOMAIN_ID>
— The ID of the domain of the user. If the domain ID is not provided in the request, the Identity service will attempt to pull the domain ID from the token used in the request. Note that this requires the use of a domain-scoped token -
--enabled <ENABLED>
— If the user is enabled, this value istrue
. If the user is disabled, this value isfalse
Possible values:
true
,false
-
--federated <JSON>
— List of federated objects associated with a user. Each object in the list contains theidp_id
andprotocols
.protocols
is a list of objects, each of which containsprotocol_id
andunique_id
of the protocol and user respectively. For example:Parameter is an array, may be provided multiple times.
-
--name <NAME>
— The user name. Must be unique within the owning domain -
--ignore-change-password-upon-first-use <IGNORE_CHANGE_PASSWORD_UPON_FIRST_USE>
Possible values:
true
,false
-
--ignore-lockout-failure-attempts <IGNORE_LOCKOUT_FAILURE_ATTEMPTS>
Possible values:
true
,false
-
--ignore-password-expiry <IGNORE_PASSWORD_EXPIRY>
Possible values:
true
,false
-
--ignore-user-inactivity <IGNORE_USER_INACTIVITY>
Possible values:
true
,false
-
--lock-password <LOCK_PASSWORD>
Possible values:
true
,false
-
--multi-factor-auth-enabled <MULTI_FACTOR_AUTH_ENABLED>
Possible values:
true
,false
-
--multi-factor-auth-rules <[String] as JSON>
— Parameter is an array, may be provided multiple times -
--password <PASSWORD>
— The password for the user
osc identity user delete
Deletes a user.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/user
Usage: osc identity user delete <ID>
Arguments:
<ID>
— user_id parameter for /v3/users/{user_id} API
osc identity user groups
Lists groups to which a user belongs.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/user_groups
Usage: osc identity user groups <--user-name <USER_NAME>|--user-id <USER_ID>|--current-user>
Options:
--user-name <USER_NAME>
— User Name--user-id <USER_ID>
— User ID--current-user
— Current authenticated user
osc identity user list
Lists users.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/users
Usage: osc identity user list [OPTIONS]
Options:
-
--domain-name <DOMAIN_NAME>
— Domain Name -
--domain-id <DOMAIN_ID>
— Domain ID -
--current-domain
— Current domain -
--enabled <ENABLED>
— Whether the identity provider is enabled or notPossible values:
true
,false
-
--idp-id <IDP_ID>
— Filters the response by an identity provider ID -
--limit <LIMIT>
-
--marker <MARKER>
— ID of the last fetched entry -
--name <NAME>
— The resource name -
--password-expires-at <PASSWORD_EXPIRES_AT>
— Filter results based on which user passwords have expired. The query should include an operator and a timestamp with a colon (:) separating the two, for example:password_expires_at={operator}:{timestamp}
Valid operators are: lt, lte, gt, gte, eq, and neq- lt: expiration time lower than the timestamp - lte: expiration time lower than or equal to the timestamp - gt: expiration time higher than the timestamp - gte: expiration time higher than or equal to the timestamp - eq: expiration time equal to the timestamp - neq: expiration time not equal to the timestamp
Valid timestamps are of the form:
YYYY-MM-DDTHH:mm:ssZ
.For example:/v3/users?password_expires_at=lt:2016-12-08T22:02:00Z
The example would return a list of users whose password expired before the timestamp(2016-12-08T22:02:00Z).
-
--protocol-id <PROTOCOL_ID>
— Filters the response by a protocol ID -
--sort-dir <SORT_DIR>
— Sort direction. A valid value is asc (ascending) or desc (descending)Possible values:
asc
,desc
-
--sort-key <SORT_KEY>
— Sorts resources by attribute -
--unique-id <UNIQUE_ID>
— Filters the response by a unique ID -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc identity user password
User password commands
This subcommand allows user to change the password
Usage: osc identity user password <COMMAND>
Available subcommands:
osc identity user password set
— Change password for user
osc identity user password set
Changes the password for a user.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/user_change_password
Usage: osc identity user password set [OPTIONS] <--user-name <USER_NAME>|--user-id <USER_ID>|--current-user>
Options:
--user-name <USER_NAME>
— User Name--user-id <USER_ID>
— User ID--current-user
— Current authenticated user--original-password <ORIGINAL_PASSWORD>
— The original password for the user--password <PASSWORD>
— The new password for the user
osc identity user projects
List projects to which the user has authorization to access.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/user_projects
Usage: osc identity user projects <--user-name <USER_NAME>|--user-id <USER_ID>|--current-user>
Options:
--user-name <USER_NAME>
— User Name--user-id <USER_ID>
— User ID--current-user
— Current authenticated user
osc identity user set
Updates a user.
If the back-end driver does not support this functionality, this call might return the HTTP Not Implemented (501)
response code.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/user
Usage: osc identity user set [OPTIONS] <ID>
Arguments:
<ID>
— user_id parameter for /v3/users/{user_id} API
Options:
-
--default-project-id <DEFAULT_PROJECT_ID>
— The new ID of the default project for the user -
--description <DESCRIPTION>
— The resource description -
--enabled <ENABLED>
— Enables or disables the user. An enabled user can authenticate and receive authorization. A disabled user cannot authenticate or receive authorization. Additionally, all tokens that the user holds become no longer valid. If you reenable this user, pre-existing tokens do not become valid. To enable the user, set totrue
. To disable the user, set tofalse
. Default istrue
Possible values:
true
,false
-
--federated <JSON>
— List of federated objects associated with a user. Each object in the list contains theidp_id
andprotocols
.protocols
is a list of objects, each of which containsprotocol_id
andunique_id
of the protocol and user respectively. For example:Parameter is an array, may be provided multiple times.
-
--name <NAME>
— The new name for the user. Must be unique within the owning domain -
--ignore-change-password-upon-first-use <IGNORE_CHANGE_PASSWORD_UPON_FIRST_USE>
Possible values:
true
,false
-
--ignore-lockout-failure-attempts <IGNORE_LOCKOUT_FAILURE_ATTEMPTS>
Possible values:
true
,false
-
--ignore-password-expiry <IGNORE_PASSWORD_EXPIRY>
Possible values:
true
,false
-
--ignore-user-inactivity <IGNORE_USER_INACTIVITY>
Possible values:
true
,false
-
--lock-password <LOCK_PASSWORD>
Possible values:
true
,false
-
--multi-factor-auth-enabled <MULTI_FACTOR_AUTH_ENABLED>
Possible values:
true
,false
-
--multi-factor-auth-rules <[String] as JSON>
— Parameter is an array, may be provided multiple times -
--password <PASSWORD>
— The new password for the user
osc identity user show
Shows details for a user.
Relationship: https://docs.openstack.org/api/openstack-identity/3/rel/user
Usage: osc identity user show <ID>
Arguments:
<ID>
— user_id parameter for /v3/users/{user_id} API
osc image
Image service operations
Usage: osc image <COMMAND>
Available subcommands:
osc image image
— Image commandsosc image metadef
— Metadef commandsosc image schema
— Schema commands
osc image image
Image commands
Usage: osc image image <COMMAND>
Available subcommands:
osc image image create
— Create imageosc image image deactivate
— Deactivate imageosc image image delete
— Delete imageosc image image download
— Download binary image dataosc image image list
— List imagesosc image image reactivate
— Reactivate imageosc image image set
— Update imageosc image image show
— Show imageosc image image upload
— Upload binary image data
osc image image create
Creates a catalog record for an operating system disk image. (Since Image API v2.0)
The Location
response header contains the URI for the image.
A multiple store backend support is introduced in the Rocky release as a part of the EXPERIMENTAL Image API v2.8. Since Image API v2.8 a new header OpenStack-image-store-ids
which contains the list of available stores will be included in response. This header is only included if multiple backend stores are supported.
The response body contains the new image entity.
Synchronous Postconditions
Normal response codes: 201
Error response codes: 400, 401, 403, 409, 413, 415
Usage: osc image image create [OPTIONS]
Options:
-
--container-format <CONTAINER_FORMAT>
— Format of the image container.Values may vary based on the configuration available in a particular OpenStack cloud. See the Image Schema response from the cloud itself for the valid values available. See Container Format in the Glance documentation for more information.
Example formats are:
ami
,ari
,aki
,bare
,ovf
,ova
,docker
, orcompressed
.The value might be
null
(JSON null data type).Train changes: The
compressed
container format is a supported value.Possible values:
aki
,ami
,ari
,bare
,compressed
,docker
,ova
,ovf
-
--disk-format <DISK_FORMAT>
— The format of the disk.Values may vary based on the configuration available in a particular OpenStack cloud. See the Image Schema response from the cloud itself for the valid values available. See Disk Format in the Glance documentation for more information.
Example formats are:
ami
,ari
,aki
,vhd
,vhdx
,vmdk
,raw
,qcow2
,vdi
,ploop
oriso
.The value might be
null
(JSON null data type).Newton changes: The
vhdx
disk format is a supported value.\ Ocata changes: Theploop
disk format is a supported value.Possible values:
aki
,ami
,ari
,iso
,ploop
,qcow2
,raw
,vdi
,vhd
,vhdx
,vmdk
-
--id <ID>
— A unique, user-defined image UUID, in the format:Where n is a hexadecimal digit from 0 to f, or F.
For example:
If you omit this value, the API generates a UUID for the image. If you specify a value that has already been assigned, the request fails with a
409
response code. -
--locations <JSON>
— A set of URLs to access the image file kept in external storeParameter is an array, may be provided multiple times.
-
--min-disk <MIN_DISK>
— Amount of disk space in GB that is required to boot the image -
--min-ram <MIN_RAM>
— Amount of RAM in MB that is required to boot the image -
--name <NAME>
— The name of the image -
--os-hidden <OS_HIDDEN>
— If true, image will not appear in default image list responsePossible values:
true
,false
-
--owner <OWNER>
— Owner of the image -
--protected <PROTECTED>
— Image protection for deletion. Valid value istrue
orfalse
. Default isfalse
Possible values:
true
,false
-
--tags <TAGS>
— List of tags for this image. Each tag is a string of at most 255 chars. The maximum number of tags allowed on an image is set by the operator.Parameter is an array, may be provided multiple times.
-
--visibility <VISIBILITY>
— Visibility for this image. Valid value is one of:public
,private
,shared
, orcommunity
. At most sites, only an administrator can make an imagepublic
. Some sites may restrict what users can make an imagecommunity
. Some sites may restrict what users can perform member operations on ashared
image. Since the Image API v2.5, the default value isshared
.Possible values:
community
,private
,public
,shared
-
--property <key=value>
— Additional properties to be sent with the request
osc image image deactivate
Deactivates an image. (Since Image API v2.3)
By default, this operation is restricted to administrators only.
Usage: osc image image deactivate [OPTIONS] <ID>
Arguments:
<ID>
— image_id parameter for /v2/images/{image_id}/actions/deactivate API
Options:
--property <key=value>
osc image image delete
(Since Image API v2.0) Deletes an image.
You cannot delete images with the protected
attribute set to true
(boolean).
Preconditions
Synchronous Postconditions
Normal response codes: 204
Error response codes: 400, 401, 403, 404, 409
Usage: osc image image delete <ID>
Arguments:
<ID>
— image_id parameter for /v2/images/{image_id} API
osc image image download
Downloads binary image data. (Since Image API v2.0)
Example call: curl -i -X GET -H "X-Auth-Token: $token" $image_url/v2/images/{image_id}/file
The response body contains the raw binary data that represents the actual virtual disk. The Content-Type
header contains the application/octet-stream
value. The Content-MD5
header contains an MD5 checksum of the image data. Use this checksum to verify the integrity of the image data.
Preconditions
Synchronous Postconditions
Normal response codes: 200, 204, 206
Error response codes: 400, 403, 404, 416
Usage: osc image image download [OPTIONS] <IMAGE_ID>
Arguments:
<IMAGE_ID>
— image_id parameter for /v2/images/{image_id}/file API
Options:
--file <FILE>
— Destination filename (using "-" will print object to stdout)
osc image image list
Lists public virtual machine (VM) images. (Since Image API v2.0)
Pagination
Returns a subset of the larger collection of images and a link that you can use to get the next set of images. You should always check for the presence of a next
link and use it as the URI in a subsequent HTTP GET request. You should follow this pattern until a next
link is no longer provided.
The next
link preserves any query parameters that you send in your initial request. You can use the first
link to jump back to the first page of the collection. If you prefer to paginate through images manually, use the limit
and marker
parameters.
Query Filters
The list operation accepts query parameters to filter the response.
A client can provide direct comparison filters by using most image attributes, such as name=Ubuntu
, visibility=public
, and so on.
To filter using image tags, use the filter tag
(note the singular). To filter on multiple tags, include each tag separately in the query. For example, to find images with the tag ready, include tag=ready
in your query string. To find images tagged with ready and approved, include tag=ready&tag=approved
in your query string. (Note that only images containing both tags will be included in the response.)
A client cannot use any link
in the json-schema, such as self, file, or schema, to filter the response.
You can list VM images that have a status of active
, queued
, or saving
.
The in
Operator
As a convenience, you may specify several values for any of the following fields by using the in
operator:
For most of these, usage is straight forward. For example, to list images in queued or saving status, use:
GET /v2/images?status=in:saving,queued
To find images in a particular list of image IDs, use:
GET /v2/images?id=in:3afb79c1-131a-4c38-a87c-bc4b801d14e6,2e011209-660f-44b5-baf2-2eb4babae53d
Using the in
operator with the name
property of images can be a bit trickier, depending upon how creatively you have named your images. The general rule is that if an image name contains a comma (,
), you must enclose the entire name in quotation marks ("
). As usual, you must URL encode any characters that require it.
For example, to find images named glass, darkly
or share me
, you would use the following filter specification:
GET v2/images?name=in:"glass,%20darkly",share%20me
As with regular filtering by name, you must specify the complete name you are looking for. Thus, for example, the query string name=in:glass,share
will only match images with the exact name glass
or the exact name share
. It will not find an image named glass, darkly
or an image named share me
.
Size Comparison Filters
You can use the size_min
and size_max
query parameters to filter images that are greater than or less than the image size. The size, in bytes, is the size of an image on disk.
For example, to filter the container to include only images that are from 1 to 4 MB, set the size_min
query parameter to 1048576
and the size_max
query parameter to 4194304
.
Time Comparison Filters
You can use a comparison operator along with the created_at
or updated_at
fields to filter your results. Specify the operator first, a colon (:
) as a separator, and then the time in ISO 8601 Format. Available comparison operators are:
For example:
Sorting
You can use query parameters to sort the results of this operation.
To sort the response, use the sort_key
and sort_dir
query parameters:
Alternatively, specify the sort
query parameter:
Normal response codes: 200
Error response codes: 400, 401, 403
Usage: osc image image list [OPTIONS]
Options:
-
--created-at <CREATED_AT>
— Specify a comparison filter based on the date and time when the resource was created -
--id <ID>
— id filter parameter -
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--member-status <MEMBER_STATUS>
— Filters the response by a member status. A valid value is accepted, pending, rejected, or all. Default is acceptedPossible values:
accepted
,all
,pending
,rejected
-
--name <NAME>
— Filters the response by a name, as a string. A valid value is the name of an image -
--os-hidden <OS_HIDDEN>
— When true, filters the response to display only "hidden" images. By default, "hidden" images are not included in the image-list response. (Since Image API v2.7)Possible values:
true
,false
-
--owner <OWNER>
— Filters the response by a project (also called a “tenant”) ID. Shows only images that are shared with you by the specified owner -
--protected <PROTECTED>
— Filters the response by the ‘protected’ image property. A valid value is one of ‘true’, ‘false’ (must be all lowercase). Any other value will result in a 400 responsePossible values:
true
,false
-
--size-max <SIZE_MAX>
— Filters the response by a maximum image size, in bytes -
--size-min <SIZE_MIN>
— Filters the response by a minimum image size, in bytes -
--sort <SORT>
— Sorts the response by one or more attribute and sort direction combinations. You can also set multiple sort keys and directions. Default direction is desc. Use the comma (,) character to separate multiple values. For example:sort=name:asc,status:desc
-
--sort-dir <SORT_DIR>
— Sorts the response by a set of one or more sort direction and attribute (sort_key) combinations. A valid value for the sort direction is asc (ascending) or desc (descending). If you omit the sort direction in a set, the default is descPossible values:
asc
,desc
-
--sort-key <SORT_KEY>
— Sorts the response by an attribute, such as name, id, or updated_at. Default is created_at. The API uses the natural sorting direction of the sort_key image attribute -
--status <STATUS>
— Filters the response by an image status -
--tag <TAG>
— Filters the response by the specified tag value. May be repeated, but keep in mind that you're making a conjunctive query, so only images containing all the tags specified will appear in the response -
--updated-at <UPDATED_AT>
— Specify a comparison filter based on the date and time when the resource was most recently modified -
--visibility <VISIBILITY>
— Filters the response by an image visibility value. A valid value is public, private, community, shared, or all. (Note that if you filter on shared, the images included in the response will only be those where your member status is accepted unless you explicitly include a member_status filter in the request.) If you omit this parameter, the response shows public, private, and those shared images with a member status of acceptedPossible values:
all
,community
,private
,public
,shared
-
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc image image reactivate
Reactivates an image. (Since Image API v2.3)
By default, this operation is restricted to administrators only
Usage: osc image image reactivate [OPTIONS] <ID>
Arguments:
<ID>
— image_id parameter for /v2/images/{image_id}/actions/reactivate API
Options:
--property <key=value>
osc image image set
Updates an image. (Since Image API v2.0)
Conceptually, you update an image record by patching the JSON representation of the image, passing a request body conforming to one of the following media types:
Attempting to make a PATCH call using some other media type will provoke a response code of 415 (Unsupported media type).
The application/openstack-images-v2.1-json-patch
media type provides a useful and compatible subset of the functionality defined in JavaScript Object Notation (JSON) Patch RFC6902, which defines the application/json-patch+json
media type.
For information about the PATCH method and the available media types, see Image API v2 HTTP PATCH media types.
Attempting to modify some image properties will cause the entire request to fail with a 403 (Forbidden) response code:
Attempting to add a location path to an image that is not in queued
or active
state will result in a 409 (Conflict) response code (since Image API v2.4).
Normal response codes: 200
Error response codes: 400, 401, 403, 404, 409, 413, 415
Usage: osc image image set [OPTIONS] <ID>
Arguments:
<ID>
— image_id parameter for /v2/images/{image_id} API
Options:
-
--container-format <CONTAINER_FORMAT>
— Format of the image container.Values may vary based on the configuration available in a particular OpenStack cloud. See the Image Schema response from the cloud itself for the valid values available. See Container Format in the Glance documentation for more information.
Example formats are:
ami
,ari
,aki
,bare
,ovf
,ova
,docker
, orcompressed
.The value might be
null
(JSON null data type).Train changes: The
compressed
container format is a supported value. -
--disk-format <DISK_FORMAT>
— The format of the disk.Values may vary based on the configuration available in a particular OpenStack cloud. See the Image Schema response from the cloud itself for the valid values available. See Disk Format in the Glance documentation for more information.
Example formats are:
ami
,ari
,aki
,vhd
,vhdx
,vmdk
,raw
,qcow2
,vdi
,ploop
oriso
.The value might be
null
(JSON null data type).Newton changes: The
vhdx
disk format is a supported value.\ Ocata changes: Theploop
disk format is a supported value. -
--locations <JSON>
— A list of objects, each of which describes an image location. Each object contains aurl
key, whose value is a URL specifying a location, and ametadata
key, whose value is a dict of key:value pairs containing information appropriate to the use of whatever external store is indicated by the URL. This list appears only if theshow_multiple_locations
option is set totrue
in the Image service’s configuration file. Because it presents a security risk, this option is disabled by default.Parameter is an array, may be provided multiple times.
-
--min-disk <MIN_DISK>
— Amount of disk space in GB that is required to boot the image. The value might benull
(JSON null data type) -
--min-ram <MIN_RAM>
— Amount of RAM in MB that is required to boot the image. The value might benull
(JSON null data type) -
--name <NAME>
— The name of the image. Value might benull
(JSON null data type) -
--os-hidden <OS_HIDDEN>
— This field controls whether an image is displayed in the default image-list response. A “hidden” image is out of date somehow (for example, it may not have the latest updates applied) and hence should not be a user’s first choice, but it’s not deleted because it may be needed for server rebuilds. By hiding it from the default image list, it’s easier for end users to find and use a more up-to-date version of this image. (Since Image API v2.7)Possible values:
true
,false
-
--owner <OWNER>
— An identifier for the owner of the image, usually the project (also called the “tenant”) ID. The value might benull
(JSON null data type) -
--protected <PROTECTED>
— A boolean value that must befalse
or the image cannot be deletedPossible values:
true
,false
-
--tags <TAGS>
— List of tags for this image, possibly an empty list.Parameter is an array, may be provided multiple times.
-
--visibility <VISIBILITY>
— Image visibility, that is, the access permission for the imagePossible values:
community
,private
,public
,shared
-
--property <key=value>
— Additional properties to be sent with the request
osc image image show
Shows details for an image. (Since Image API v2.0)
The response body contains a single image entity.
Preconditions
Normal response codes: 200
Error response codes: 400, 401, 403, 404
Usage: osc image image show <ID>
Arguments:
<ID>
— image_id parameter for /v2/images/{image_id} API
osc image image upload
Uploads binary image data. (Since Image API v2.0)
Set the Content-Type
request header to application/octet-stream
.
A multiple store backend support is introduced in the Rocky release as a part of the EXPERIMENTAL Image API v2.8.
Beginning with API version 2.8, an optional X-Image-Meta-Store
header may be added to the request. When present, the image data will be placed into the backing store whose identifier is the value of this header. If the store identifier specified is not recognized, a 400 (Bad Request) response is returned. When the header is not present, the image data is placed into the default backing store.
Example call:
Preconditions
Before you can store binary image data, you must meet the following preconditions:
Synchronous Postconditions
Troubleshooting
Normal response codes: 204
Error response codes: 400, 401, 403, 404, 409, 410, 413, 415, 503
Usage: osc image image upload [OPTIONS] <IMAGE_ID>
Arguments:
<IMAGE_ID>
— image_id parameter for /v2/images/{image_id}/file API
Options:
--file <FILE>
— Source filename (using "-" will read object from stdout)
osc image metadef
Metadef commands
Usage: osc image metadef <COMMAND>
Available subcommands:
osc image metadef namespace
— Metadata definition namespacesosc image metadef resource-type
— Metadata definition namespaces
osc image metadef namespace
Metadata definition namespaces
Creates, lists, shows details for, updates, and deletes metadata definition namespaces. Defines namespaces that can contain property definitions, object definitions, and resource type associations.
Usage: osc image metadef namespace <COMMAND>
Available subcommands:
osc image metadef namespace create
— Command without description in OpenAPIosc image metadef namespace delete
— Command without description in OpenAPIosc image metadef namespace list
— Command without description in OpenAPIosc image metadef namespace object
— Metadata definition objectsosc image metadef namespace property
— Metadata definition propertiesosc image metadef namespace resource-type-association
— Metadata definition resource typesosc image metadef namespace set
— Command without description in OpenAPIosc image metadef namespace show
— Command without description in OpenAPIosc image metadef namespace tag
— Metadata definition tags
osc image metadef namespace create
Command without description in OpenAPI
Usage: osc image metadef namespace create [OPTIONS] --namespace <NAMESPACE>
Options:
-
--description <DESCRIPTION>
— Provides a user friendly description of the namespace -
--display-name <DISPLAY_NAME>
— The user friendly name for the namespace. Used by UI if available -
--namespace <NAMESPACE>
— The unique namespace text -
--objects <JSON>
— Parameter is an array, may be provided multiple times -
--owner <OWNER>
— Owner of the namespace -
--properties <key=value>
-
--protected <PROTECTED>
— If true, namespace will not be deletablePossible values:
true
,false
-
--resource-type-associations <JSON>
— Parameter is an array, may be provided multiple times -
--tags <TAGS>
— Parameter is an array, may be provided multiple times -
--visibility <VISIBILITY>
— Scope of namespace accessibilityPossible values:
private
,public
osc image metadef namespace delete
Command without description in OpenAPI
Usage: osc image metadef namespace delete <NAMESPACE_NAME>
Arguments:
<NAMESPACE_NAME>
— namespace_name parameter for /v2/metadefs/namespaces/{namespace_name} API
osc image metadef namespace list
Command without description in OpenAPI
Usage: osc image metadef namespace list
osc image metadef namespace object
Metadata definition objects
Creates, lists, shows details for, updates, and deletes metadata definition objects.
Usage: osc image metadef namespace object <COMMAND>
Available subcommands:
osc image metadef namespace object create
— Command without description in OpenAPIosc image metadef namespace object delete
— Command without description in OpenAPIosc image metadef namespace object list
— Command without description in OpenAPIosc image metadef namespace object purge
— Command without description in OpenAPIosc image metadef namespace object set
— Command without description in OpenAPIosc image metadef namespace object show
— Command without description in OpenAPI
osc image metadef namespace object create
Command without description in OpenAPI
Usage: osc image metadef namespace object create [OPTIONS] --name <NAME> <NAMESPACE_NAME>
Arguments:
<NAMESPACE_NAME>
— namespace_name parameter for /v2/metadefs/namespaces/{namespace_name}/objects/{object_name} API
Options:
--description <DESCRIPTION>
--name <NAME>
--properties <key=value>
--required <REQUIRED>
— Parameter is an array, may be provided multiple times
osc image metadef namespace object delete
Command without description in OpenAPI
Usage: osc image metadef namespace object delete <NAMESPACE_NAME> <OBJECT_NAME>
Arguments:
<NAMESPACE_NAME>
— namespace_name parameter for /v2/metadefs/namespaces/{namespace_name}/objects/{object_name} API<OBJECT_NAME>
— object_name parameter for /v2/metadefs/namespaces/{namespace_name}/objects/{object_name} API
osc image metadef namespace object list
Command without description in OpenAPI
Usage: osc image metadef namespace object list <NAMESPACE_NAME>
Arguments:
<NAMESPACE_NAME>
— namespace_name parameter for /v2/metadefs/namespaces/{namespace_name}/objects/{object_name} API
osc image metadef namespace object purge
Command without description in OpenAPI
Usage: osc image metadef namespace object purge <NAMESPACE_NAME>
Arguments:
<NAMESPACE_NAME>
— namespace_name parameter for /v2/metadefs/namespaces/{namespace_name}/objects/{object_name} API
osc image metadef namespace object set
Command without description in OpenAPI
Usage: osc image metadef namespace object set [OPTIONS] --name <NAME> <NAMESPACE_NAME> <OBJECT_NAME>
Arguments:
<NAMESPACE_NAME>
— namespace_name parameter for /v2/metadefs/namespaces/{namespace_name}/objects/{object_name} API<OBJECT_NAME>
— object_name parameter for /v2/metadefs/namespaces/{namespace_name}/objects/{object_name} API
Options:
--description <DESCRIPTION>
--name <NAME>
--properties <key=value>
--required <REQUIRED>
— Parameter is an array, may be provided multiple times
osc image metadef namespace object show
Command without description in OpenAPI
Usage: osc image metadef namespace object show <NAMESPACE_NAME> <OBJECT_NAME>
Arguments:
<NAMESPACE_NAME>
— namespace_name parameter for /v2/metadefs/namespaces/{namespace_name}/objects/{object_name} API<OBJECT_NAME>
— object_name parameter for /v2/metadefs/namespaces/{namespace_name}/objects/{object_name} API
osc image metadef namespace property
Metadata definition properties
Creates, lists, shows details for, updates, and deletes metadata definition properties.
Usage: osc image metadef namespace property <COMMAND>
Available subcommands:
osc image metadef namespace property create
— Command without description in OpenAPIosc image metadef namespace property delete
— Command without description in OpenAPIosc image metadef namespace property list
— Command without description in OpenAPIosc image metadef namespace property purge
— Command without description in OpenAPIosc image metadef namespace property set
— Command without description in OpenAPIosc image metadef namespace property show
— Command without description in OpenAPI
osc image metadef namespace property create
Command without description in OpenAPI
Usage: osc image metadef namespace property create [OPTIONS] --name <NAME> --title <TITLE> --type <TYPE> <NAMESPACE_NAME>
Arguments:
<NAMESPACE_NAME>
— namespace_name parameter for /v2/metadefs/namespaces/{namespace_name}/properties/{property_name} API
Options:
-
--additional-items <ADDITIONAL_ITEMS>
Possible values:
true
,false
-
--default <JSON>
-
--description <DESCRIPTION>
-
--enum <ENUM>
— Parameter is an array, may be provided multiple times -
--items <JSON>
-
--maximum <MAXIMUM>
-
--max-items <MAX_ITEMS>
-
--max-length <MAX_LENGTH>
-
--minimum <MINIMUM>
-
--min-items <MIN_ITEMS>
-
--min-length <MIN_LENGTH>
-
--name <NAME>
-
--operators <OPERATORS>
— Parameter is an array, may be provided multiple times -
--pattern <PATTERN>
-
--readonly <READONLY>
Possible values:
true
,false
-
--required <REQUIRED>
— Parameter is an array, may be provided multiple times -
--title <TITLE>
-
--type <TYPE>
Possible values:
array
,boolean
,integer
,number
,object
,string
-
--unique-items <UNIQUE_ITEMS>
Possible values:
true
,false
osc image metadef namespace property delete
Command without description in OpenAPI
Usage: osc image metadef namespace property delete <NAMESPACE_NAME> <PROPERTY_NAME>
Arguments:
<NAMESPACE_NAME>
— namespace_name parameter for /v2/metadefs/namespaces/{namespace_name}/properties/{property_name} API<PROPERTY_NAME>
— property_name parameter for /v2/metadefs/namespaces/{namespace_name}/properties/{property_name} API
osc image metadef namespace property list
Command without description in OpenAPI
Usage: osc image metadef namespace property list <NAMESPACE_NAME>
Arguments:
<NAMESPACE_NAME>
— namespace_name parameter for /v2/metadefs/namespaces/{namespace_name}/properties/{property_name} API
osc image metadef namespace property purge
Command without description in OpenAPI
Usage: osc image metadef namespace property purge <NAMESPACE_NAME>
Arguments:
<NAMESPACE_NAME>
— namespace_name parameter for /v2/metadefs/namespaces/{namespace_name}/properties/{property_name} API
osc image metadef namespace property set
Command without description in OpenAPI
Usage: osc image metadef namespace property set [OPTIONS] --name <NAME> --title <TITLE> --type <TYPE> <NAMESPACE_NAME> <PROPERTY_NAME>
Arguments:
<NAMESPACE_NAME>
— namespace_name parameter for /v2/metadefs/namespaces/{namespace_name}/properties/{property_name} API<PROPERTY_NAME>
— property_name parameter for /v2/metadefs/namespaces/{namespace_name}/properties/{property_name} API
Options:
-
--additional-items <ADDITIONAL_ITEMS>
Possible values:
true
,false
-
--default <JSON>
-
--description <DESCRIPTION>
-
--enum <ENUM>
— Parameter is an array, may be provided multiple times -
--items <JSON>
-
--maximum <MAXIMUM>
-
--max-items <MAX_ITEMS>
-
--max-length <MAX_LENGTH>
-
--minimum <MINIMUM>
-
--min-items <MIN_ITEMS>
-
--min-length <MIN_LENGTH>
-
--name <NAME>
-
--operators <OPERATORS>
— Parameter is an array, may be provided multiple times -
--pattern <PATTERN>
-
--readonly <READONLY>
Possible values:
true
,false
-
--required <REQUIRED>
— Parameter is an array, may be provided multiple times -
--title <TITLE>
-
--type <TYPE>
Possible values:
array
,boolean
,integer
,number
,object
,string
-
--unique-items <UNIQUE_ITEMS>
Possible values:
true
,false
osc image metadef namespace property show
Command without description in OpenAPI
Usage: osc image metadef namespace property show <NAMESPACE_NAME> <PROPERTY_NAME>
Arguments:
<NAMESPACE_NAME>
— namespace_name parameter for /v2/metadefs/namespaces/{namespace_name}/properties/{property_name} API<PROPERTY_NAME>
— property_name parameter for /v2/metadefs/namespaces/{namespace_name}/properties/{property_name} API
osc image metadef namespace resource-type-association
Metadata definition resource types
Lists resource types. Also, creates, lists, and removes resource type associations in a namespace.
Usage: osc image metadef namespace resource-type-association <COMMAND>
Available subcommands:
osc image metadef namespace resource-type-association create
— Command without description in OpenAPIosc image metadef namespace resource-type-association delete
— Command without description in OpenAPIosc image metadef namespace resource-type-association list
— Command without description in OpenAPI
osc image metadef namespace resource-type-association create
Command without description in OpenAPI
Usage: osc image metadef namespace resource-type-association create [OPTIONS] --name <NAME> <NAMESPACE_NAME>
Arguments:
<NAMESPACE_NAME>
— namespace_name parameter for /v2/metadefs/namespaces/{namespace_name}/resource_types/{resource_type} API
Options:
--name <NAME>
— Resource type names should be aligned with Heat resource types whenever possible: https://docs.openstack.org/heat/latest/template_guide/openstack.html--prefix <PREFIX>
— Specifies the prefix to use for the given resource type. Any properties in the namespace should be prefixed with this prefix when being applied to the specified resource type. Must include prefix separator (e.g. a colon :)--properties-target <PROPERTIES_TARGET>
— Some resource types allow more than one key / value pair per instance. For example, Cinder allows user and image metadata on volumes. Only the image properties metadata is evaluated by Nova (scheduling or drivers). This property allows a namespace target to remove the ambiguity
osc image metadef namespace resource-type-association delete
Command without description in OpenAPI
Usage: osc image metadef namespace resource-type-association delete <NAMESPACE_NAME> <RESOURCE_TYPE>
Arguments:
<NAMESPACE_NAME>
— namespace_name parameter for /v2/metadefs/namespaces/{namespace_name}/resource_types/{resource_type} API<RESOURCE_TYPE>
— resource_type parameter for /v2/metadefs/namespaces/{namespace_name}/resource_types/{resource_type} API
osc image metadef namespace resource-type-association list
Command without description in OpenAPI
Usage: osc image metadef namespace resource-type-association list <NAMESPACE_NAME>
Arguments:
<NAMESPACE_NAME>
— namespace_name parameter for /v2/metadefs/namespaces/{namespace_name}/resource_types/{resource_type} API
osc image metadef namespace set
Command without description in OpenAPI
Usage: osc image metadef namespace set [OPTIONS] --namespace <NAMESPACE> <NAMESPACE_NAME>
Arguments:
<NAMESPACE_NAME>
— namespace_name parameter for /v2/metadefs/namespaces/{namespace_name} API
Options:
-
--description <DESCRIPTION>
— Provides a user friendly description of the namespace -
--display-name <DISPLAY_NAME>
— The user friendly name for the namespace. Used by UI if available -
--namespace <NAMESPACE>
— The unique namespace text -
--objects <JSON>
— Parameter is an array, may be provided multiple times -
--owner <OWNER>
— Owner of the namespace -
--properties <key=value>
-
--protected <PROTECTED>
— If true, namespace will not be deletablePossible values:
true
,false
-
--resource-type-associations <JSON>
— Parameter is an array, may be provided multiple times -
--tags <TAGS>
— Parameter is an array, may be provided multiple times -
--visibility <VISIBILITY>
— Scope of namespace accessibilityPossible values:
private
,public
osc image metadef namespace show
Command without description in OpenAPI
Usage: osc image metadef namespace show <NAMESPACE_NAME>
Arguments:
<NAMESPACE_NAME>
— namespace_name parameter for /v2/metadefs/namespaces/{namespace_name} API
osc image metadef namespace tag
Metadata definition tags
Creates, lists, shows details for, updates, and deletes metadata definition tags.
Usage: osc image metadef namespace tag <COMMAND>
Available subcommands:
osc image metadef namespace tag create
— Command without description in OpenAPIosc image metadef namespace tag delete
— Command without description in OpenAPIosc image metadef namespace tag list
— Command without description in OpenAPIosc image metadef namespace tag purge
— Command without description in OpenAPIosc image metadef namespace tag set
— Command without description in OpenAPIosc image metadef namespace tag show
— Command without description in OpenAPI
osc image metadef namespace tag create
Command without description in OpenAPI
Usage: osc image metadef namespace tag create --name <NAME> <NAMESPACE_NAME> <TAG_NAME>
Arguments:
<NAMESPACE_NAME>
— namespace_name parameter for /v2/metadefs/namespaces/{namespace_name}/tags/{tag_name} API<TAG_NAME>
— tag_name parameter for /v2/metadefs/namespaces/{namespace_name}/tags/{tag_name} API
Options:
--name <NAME>
osc image metadef namespace tag delete
Command without description in OpenAPI
Usage: osc image metadef namespace tag delete <NAMESPACE_NAME> <TAG_NAME>
Arguments:
<NAMESPACE_NAME>
— namespace_name parameter for /v2/metadefs/namespaces/{namespace_name}/tags/{tag_name} API<TAG_NAME>
— tag_name parameter for /v2/metadefs/namespaces/{namespace_name}/tags/{tag_name} API
osc image metadef namespace tag list
Command without description in OpenAPI
Usage: osc image metadef namespace tag list <NAMESPACE_NAME>
Arguments:
<NAMESPACE_NAME>
— namespace_name parameter for /v2/metadefs/namespaces/{namespace_name}/tags/{tag_name} API
osc image metadef namespace tag purge
Command without description in OpenAPI
Usage: osc image metadef namespace tag purge <NAMESPACE_NAME>
Arguments:
<NAMESPACE_NAME>
— namespace_name parameter for /v2/metadefs/namespaces/{namespace_name}/tags/{tag_name} API
osc image metadef namespace tag set
Command without description in OpenAPI
Usage: osc image metadef namespace tag set --name <NAME> <NAMESPACE_NAME> <TAG_NAME>
Arguments:
<NAMESPACE_NAME>
— namespace_name parameter for /v2/metadefs/namespaces/{namespace_name}/tags/{tag_name} API<TAG_NAME>
— tag_name parameter for /v2/metadefs/namespaces/{namespace_name}/tags/{tag_name} API
Options:
--name <NAME>
osc image metadef namespace tag show
Command without description in OpenAPI
Usage: osc image metadef namespace tag show <NAMESPACE_NAME> <TAG_NAME>
Arguments:
<NAMESPACE_NAME>
— namespace_name parameter for /v2/metadefs/namespaces/{namespace_name}/tags/{tag_name} API<TAG_NAME>
— tag_name parameter for /v2/metadefs/namespaces/{namespace_name}/tags/{tag_name} API
osc image metadef resource-type
Metadata definition namespaces
Creates, lists, shows details for, updates, and deletes metadata definition namespaces. Defines namespaces that can contain property definitions, object definitions, and resource type associations.
Usage: osc image metadef resource-type <COMMAND>
Available subcommands:
osc image metadef resource-type list
— Command without description in OpenAPI
osc image metadef resource-type list
Command without description in OpenAPI
Usage: osc image metadef resource-type list
osc image schema
Schema commands
Usage: osc image schema <COMMAND>
Available subcommands:
osc image schema image
— Show Image Schemaosc image schema images
— Show Images Schemaosc image schema member
— Show Member Schemaosc image schema members
— Show Members Schemaosc image schema metadef
— Metadata definition schemas
osc image schema image
Show Image Schema
Usage: osc image schema image <COMMAND>
Available subcommands:
osc image schema image show
— Show Image Schema
osc image schema image show
Show Image Schema
Usage: osc image schema image show
osc image schema images
Show Images Schema
Usage: osc image schema images <COMMAND>
Available subcommands:
osc image schema images show
— Show Images Schema
osc image schema images show
Show Images Schema
Usage: osc image schema images show
osc image schema member
Show Member Schema
Usage: osc image schema member <COMMAND>
Available subcommands:
osc image schema member show
— Show Member Schema
osc image schema member show
Show Member Schema
Usage: osc image schema member show
osc image schema members
Show Members Schema
Usage: osc image schema members <COMMAND>
Available subcommands:
osc image schema members show
— Show Members Schema
osc image schema members show
Show Members Schema
Usage: osc image schema members show
osc image schema metadef
Metadata definition schemas
Gets a JSON-schema document that represents a metadata definition entity.
Usage: osc image schema metadef <COMMAND>
Available subcommands:
osc image schema metadef namespace
— Metadef Namespace Schema operationsosc image schema metadef namespaces
— Metadef Namespaces Schema operationsosc image schema metadef object
— Metadef Object Schema operationsosc image schema metadef objects
— Metadef Objects Schema operationsosc image schema metadef properties
— Metadef Properties Schema operationsosc image schema metadef property
— Metadef Property Schema operationsosc image schema metadef resource-type
— Metadef ResourceType Schema operationsosc image schema metadef resource-types
— Metadef ResourceTypes Schema operationsosc image schema metadef tag
— Metadef Tag Schema operationsosc image schema metadef tags
— Metadef Tags Schema operations
osc image schema metadef namespace
Metadef Namespace Schema operations
Usage: osc image schema metadef namespace <COMMAND>
Available subcommands:
osc image schema metadef namespace show
— Command without description in OpenAPI
osc image schema metadef namespace show
Command without description in OpenAPI
Usage: osc image schema metadef namespace show
osc image schema metadef namespaces
Metadef Namespaces Schema operations
Usage: osc image schema metadef namespaces <COMMAND>
Available subcommands:
osc image schema metadef namespaces show
— Command without description in OpenAPI
osc image schema metadef namespaces show
Command without description in OpenAPI
Usage: osc image schema metadef namespaces show
osc image schema metadef object
Metadef Object Schema operations
Usage: osc image schema metadef object <COMMAND>
Available subcommands:
osc image schema metadef object show
— Command without description in OpenAPI
osc image schema metadef object show
Command without description in OpenAPI
Usage: osc image schema metadef object show
osc image schema metadef objects
Metadef Objects Schema operations
Usage: osc image schema metadef objects <COMMAND>
Available subcommands:
osc image schema metadef objects show
— Command without description in OpenAPI
osc image schema metadef objects show
Command without description in OpenAPI
Usage: osc image schema metadef objects show
osc image schema metadef properties
Metadef Properties Schema operations
Usage: osc image schema metadef properties <COMMAND>
Available subcommands:
osc image schema metadef properties show
— Command without description in OpenAPI
osc image schema metadef properties show
Command without description in OpenAPI
Usage: osc image schema metadef properties show
osc image schema metadef property
Metadef Property Schema operations
Usage: osc image schema metadef property <COMMAND>
Available subcommands:
osc image schema metadef property show
— Command without description in OpenAPI
osc image schema metadef property show
Command without description in OpenAPI
Usage: osc image schema metadef property show
osc image schema metadef resource-type
Metadef ResourceType Schema operations
Usage: osc image schema metadef resource-type <COMMAND>
Available subcommands:
osc image schema metadef resource-type show
— Command without description in OpenAPI
osc image schema metadef resource-type show
Command without description in OpenAPI
Usage: osc image schema metadef resource-type show
osc image schema metadef resource-types
Metadef ResourceTypes Schema operations
Usage: osc image schema metadef resource-types <COMMAND>
Available subcommands:
osc image schema metadef resource-types show
— Command without description in OpenAPI
osc image schema metadef resource-types show
Command without description in OpenAPI
Usage: osc image schema metadef resource-types show
osc image schema metadef tag
Metadef Tag Schema operations
Usage: osc image schema metadef tag <COMMAND>
Available subcommands:
osc image schema metadef tag show
— Command without description in OpenAPI
osc image schema metadef tag show
Command without description in OpenAPI
Usage: osc image schema metadef tag show
osc image schema metadef tags
Metadef Tags Schema operations
Usage: osc image schema metadef tags <COMMAND>
Available subcommands:
osc image schema metadef tags show
— Command without description in OpenAPI
osc image schema metadef tags show
Command without description in OpenAPI
Usage: osc image schema metadef tags show
osc load-balancer
Load Balancer service operations
Usage: osc load-balancer <COMMAND>
Available subcommands:
osc load-balancer amphorae
— Amphorae (Octavia) commandsosc load-balancer availability-zone
— AvailabilityZone (Octavia) commandsosc load-balancer availability-zone-profile
— AvailabilityZoneProfile (Octavia) commandsosc load-balancer flavor
— Flavor (Octavia) commandsosc load-balancer flavor-profile
— FlavorProfile (Octavia) commandsosc load-balancer healthmonitor
— Healthmonitor (Octavia) commandsosc load-balancer l7policy
— L7Policy (Octavia) commandsosc load-balancer listener
— Listener (Octavia) commandsosc load-balancer loadbalancer
— Loadbalancer (Octavia) commandsosc load-balancer pool
— Pool (Octavia) commandsosc load-balancer provider
— Provider (Octavia) commandsosc load-balancer quota
— Quota commandsosc load-balancer version
— Version (Octavia) commands
osc load-balancer amphorae
Amphorae (Octavia) commands
Usage: osc load-balancer amphorae <COMMAND>
Available subcommands:
osc load-balancer amphorae config
— Configure Amphoraosc load-balancer amphorae delete
— Remove an Amphoraosc load-balancer amphorae failover
— Failover Amphoraosc load-balancer amphorae list
— List Amphoraosc load-balancer amphorae show
— Show Amphora detailsosc load-balancer amphorae stats
— Show Amphora Statistics
osc load-balancer amphorae config
Configure Amphora
Usage: osc load-balancer amphorae config [OPTIONS] <AMPHORA_ID>
Arguments:
<AMPHORA_ID>
— amphora_id parameter for /v2/octavia/amphorae/{amphora_id}/config API
Options:
--property <key=value>
osc load-balancer amphorae delete
Removes an amphora and its associated configuration.
The API immediately purges any and all configuration data, depending on the configuration settings. You cannot recover it.
New in version 2.20
Usage: osc load-balancer amphorae delete <AMPHORA_ID>
Arguments:
<AMPHORA_ID>
— amphora_id parameter for /v2/octavia/amphorae/{amphora_id} API
osc load-balancer amphorae failover
Force an amphora to failover.
If you are not an administrative user, the service returns the HTTP Forbidden (403)
response code.
This operation does not require a request body.
Usage: osc load-balancer amphorae failover <AMPHORA_ID>
Arguments:
<AMPHORA_ID>
— amphora_id parameter for /v2/octavia/amphorae/{amphora_id}/failover API
osc load-balancer amphorae list
Lists all amphora for the project.
If you are not an administrative user, the service returns the HTTP Forbidden (403)
response code.
Use the fields
query parameter to control which fields are returned in the response body. Additionally, you can filter results by using query string parameters. For information, see Filtering and column selection.
The list might be empty.
Usage: osc load-balancer amphorae list [OPTIONS]
Options:
-
--cached-zone <CACHED_ZONE>
-
--cert-busy <CERT_BUSY>
-
--cert-expiration <CERT_EXPIRATION>
-
--compute-flavor <COMPUTE_FLAVOR>
-
--compute-id <COMPUTE_ID>
-
--created-at <CREATED_AT>
-
--ha-ip <HA_IP>
-
--ha-port-id <HA_PORT_ID>
-
--id <ID>
-
--image-id <IMAGE_ID>
-
--lb-network-ip <LB_NETWORK_IP>
-
--limit <LIMIT>
— Page size -
--loadbalancer-id <LOADBALANCER_ID>
-
--marker <MARKER>
— ID of the last item in the previous list -
--page-reverse <PAGE_REVERSE>
— The page directionPossible values:
true
,false
-
--role <ROLE>
-
--status <STATUS>
-
--updated-at <UPDATED_AT>
-
--vrrp-id <VRRP_ID>
-
--vrrp-interface <VRRP_INTERFACE>
-
--vrrp-ip <VRRP_IP>
-
--vrrp-port-id <VRRP_PORT_ID>
-
--vrrp-priority <VRRP_PRIORITY>
-
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc load-balancer amphorae show
Shows the details of an amphora.
If you are not an administrative user, the service returns the HTTP Forbidden (403)
response code.
This operation does not require a request body.
Usage: osc load-balancer amphorae show <AMPHORA_ID>
Arguments:
<AMPHORA_ID>
— amphora_id parameter for /v2/octavia/amphorae/{amphora_id} API
osc load-balancer amphorae stats
Show the statistics for an amphora.
If you are not an administrative user, the service returns the HTTP Forbidden (403)
response code.
Use the fields
query parameter to control which fields are returned in the response body.
New in version 2.3
Usage: osc load-balancer amphorae stats <AMPHORA_ID>
Arguments:
<AMPHORA_ID>
— amphora_id parameter for /v2/octavia/amphorae/{amphora_id}/stats API
osc load-balancer availability-zone
AvailabilityZone (Octavia) commands
Usage: osc load-balancer availability-zone <COMMAND>
Available subcommands:
osc load-balancer availability-zone create
— Creates an Availability Zoneosc load-balancer availability-zone delete
— Deletes an Availability Zoneosc load-balancer availability-zone list
— Lists all Availability Zonesosc load-balancer availability-zone set
— Command without description in OpenAPIosc load-balancer availability-zone show
— Gets an Availability Zone's detail
osc load-balancer availability-zone create
Creates an Availability Zone
Usage: osc load-balancer availability-zone create [OPTIONS] --availability-zone-profile-id <AVAILABILITY_ZONE_PROFILE_ID> --name <NAME>
Options:
-
--availability-zone-profile-id <AVAILABILITY_ZONE_PROFILE_ID>
-
--description <DESCRIPTION>
-
--enabled <ENABLED>
Possible values:
true
,false
-
--name <NAME>
osc load-balancer availability-zone delete
Deletes an Availability Zone
Usage: osc load-balancer availability-zone delete <ID>
Arguments:
<ID>
— availabilityzone_id parameter for /v2/lbaas/availabilityzones/{availabilityzone_id} API
osc load-balancer availability-zone list
Lists all Availability Zones
Usage: osc load-balancer availability-zone list [OPTIONS]
Options:
-
--availability-zone-profile-id <AVAILABILITY_ZONE_PROFILE_ID>
-
--description <DESCRIPTION>
-
--limit <LIMIT>
— Page size -
--marker <MARKER>
— ID of the last item in the previous list -
--name <NAME>
-
--page-reverse <PAGE_REVERSE>
— The page directionPossible values:
true
,false
-
--status <STATUS>
-
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc load-balancer availability-zone set
Command without description in OpenAPI
Usage: osc load-balancer availability-zone set [OPTIONS] <ID>
Arguments:
<ID>
— availabilityzone_id parameter for /v2/lbaas/availabilityzones/{availabilityzone_id} API
Options:
-
--description <DESCRIPTION>
-
--enabled <ENABLED>
Possible values:
true
,false
osc load-balancer availability-zone show
Gets an Availability Zone's detail
Usage: osc load-balancer availability-zone show <ID>
Arguments:
<ID>
— availabilityzone_id parameter for /v2/lbaas/availabilityzones/{availabilityzone_id} API
osc load-balancer availability-zone-profile
AvailabilityZoneProfile (Octavia) commands
Usage: osc load-balancer availability-zone-profile <COMMAND>
Available subcommands:
osc load-balancer availability-zone-profile create
— Creates an Availability Zone Profileosc load-balancer availability-zone-profile delete
— Deletes an Availability Zone Profileosc load-balancer availability-zone-profile list
— Lists all Availability Zone Profilesosc load-balancer availability-zone-profile set
— Updates an Availability Zone Profileosc load-balancer availability-zone-profile show
— Gets an Availability Zone Profile's detail
osc load-balancer availability-zone-profile create
Creates an Availability Zone Profile
Usage: osc load-balancer availability-zone-profile create --availability-zone-data <AVAILABILITY_ZONE_DATA> --name <NAME> --provider-name <PROVIDER_NAME>
Options:
--availability-zone-data <AVAILABILITY_ZONE_DATA>
--name <NAME>
--provider-name <PROVIDER_NAME>
osc load-balancer availability-zone-profile delete
Deletes an Availability Zone Profile
Usage: osc load-balancer availability-zone-profile delete <ID>
Arguments:
<ID>
— availabilityzoneprofile_id parameter for /v2/lbaas/availabilityzoneprofiles/{availabilityzoneprofile_id} API
osc load-balancer availability-zone-profile list
Lists all Availability Zone Profiles
Usage: osc load-balancer availability-zone-profile list [OPTIONS]
Options:
-
--availability-zone-data <AVAILABILITY_ZONE_DATA>
-
--id <ID>
-
--limit <LIMIT>
— Page size -
--marker <MARKER>
— ID of the last item in the previous list -
--name <NAME>
-
--page-reverse <PAGE_REVERSE>
— The page directionPossible values:
true
,false
-
--provider-name <PROVIDER_NAME>
-
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc load-balancer availability-zone-profile set
Updates an Availability Zone Profile
Usage: osc load-balancer availability-zone-profile set [OPTIONS] <ID>
Arguments:
<ID>
— availabilityzoneprofile_id parameter for /v2/lbaas/availabilityzoneprofiles/{availabilityzoneprofile_id} API
Options:
--availability-zone-data <AVAILABILITY_ZONE_DATA>
--name <NAME>
--provider-name <PROVIDER_NAME>
osc load-balancer availability-zone-profile show
Gets an Availability Zone Profile's detail
Usage: osc load-balancer availability-zone-profile show <ID>
Arguments:
<ID>
— availabilityzoneprofile_id parameter for /v2/lbaas/availabilityzoneprofiles/{availabilityzoneprofile_id} API
osc load-balancer flavor
Flavor (Octavia) commands
Usage: osc load-balancer flavor <COMMAND>
Available subcommands:
osc load-balancer flavor create
— Creates a flavorosc load-balancer flavor delete
— Deletes a Flavorosc load-balancer flavor list
— Lists all flavorsosc load-balancer flavor set
— Command without description in OpenAPIosc load-balancer flavor show
— Gets a flavor's detail
osc load-balancer flavor create
Creates a flavor
Usage: osc load-balancer flavor create [OPTIONS] --flavor-profile-id <FLAVOR_PROFILE_ID> --name <NAME>
Options:
-
--description <DESCRIPTION>
-
--enabled <ENABLED>
Possible values:
true
,false
-
--flavor-profile-id <FLAVOR_PROFILE_ID>
-
--name <NAME>
osc load-balancer flavor delete
Deletes a Flavor
Usage: osc load-balancer flavor delete <ID>
Arguments:
<ID>
— flavor_id parameter for /v2/lbaas/flavors/{flavor_id} API
osc load-balancer flavor list
Lists all flavors
Usage: osc load-balancer flavor list [OPTIONS]
Options:
-
--description <DESCRIPTION>
-
--enabled <ENABLED>
Possible values:
true
,false
-
--flavor-profile-id <FLAVOR_PROFILE_ID>
-
--id <ID>
-
--limit <LIMIT>
— Page size -
--marker <MARKER>
— ID of the last item in the previous list -
--name <NAME>
-
--page-reverse <PAGE_REVERSE>
— The page directionPossible values:
true
,false
-
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc load-balancer flavor set
Command without description in OpenAPI
Usage: osc load-balancer flavor set [OPTIONS] <ID>
Arguments:
<ID>
— flavor_id parameter for /v2/lbaas/flavors/{flavor_id} API
Options:
-
--description <DESCRIPTION>
-
--enabled <ENABLED>
Possible values:
true
,false
-
--name <NAME>
osc load-balancer flavor show
Gets a flavor's detail
Usage: osc load-balancer flavor show <ID>
Arguments:
<ID>
— flavor_id parameter for /v2/lbaas/flavors/{flavor_id} API
osc load-balancer flavor-profile
FlavorProfile (Octavia) commands
Usage: osc load-balancer flavor-profile <COMMAND>
Available subcommands:
osc load-balancer flavor-profile create
— Creates a flavor Profileosc load-balancer flavor-profile delete
— Deletes a Flavor Profileosc load-balancer flavor-profile list
— Lists all flavor profilesosc load-balancer flavor-profile set
— Updates a flavor Profileosc load-balancer flavor-profile show
— Gets a flavor profile's detail
osc load-balancer flavor-profile create
Creates a flavor Profile
Usage: osc load-balancer flavor-profile create --flavor-data <FLAVOR_DATA> --name <NAME> --provider-name <PROVIDER_NAME>
Options:
--flavor-data <FLAVOR_DATA>
--name <NAME>
--provider-name <PROVIDER_NAME>
osc load-balancer flavor-profile delete
Deletes a Flavor Profile
Usage: osc load-balancer flavor-profile delete <ID>
Arguments:
<ID>
— flavorprofile_id parameter for /v2/lbaas/flavorprofiles/{flavorprofile_id} API
osc load-balancer flavor-profile list
Lists all flavor profiles
Usage: osc load-balancer flavor-profile list [OPTIONS]
Options:
-
--flavor-data <FLAVOR_DATA>
-
--id <ID>
-
--limit <LIMIT>
— Page size -
--marker <MARKER>
— ID of the last item in the previous list -
--name <NAME>
-
--page-reverse <PAGE_REVERSE>
— The page directionPossible values:
true
,false
-
--provider-name <PROVIDER_NAME>
-
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc load-balancer flavor-profile set
Updates a flavor Profile
Usage: osc load-balancer flavor-profile set [OPTIONS] <ID>
Arguments:
<ID>
— flavorprofile_id parameter for /v2/lbaas/flavorprofiles/{flavorprofile_id} API
Options:
--flavor-data <FLAVOR_DATA>
--name <NAME>
--provider-name <PROVIDER_NAME>
osc load-balancer flavor-profile show
Gets a flavor profile's detail
Usage: osc load-balancer flavor-profile show <ID>
Arguments:
<ID>
— flavorprofile_id parameter for /v2/lbaas/flavorprofiles/{flavorprofile_id} API
osc load-balancer healthmonitor
Healthmonitor (Octavia) commands
Usage: osc load-balancer healthmonitor <COMMAND>
Available subcommands:
osc load-balancer healthmonitor create
— Create Health Monitorosc load-balancer healthmonitor delete
— Remove a Health Monitorosc load-balancer healthmonitor list
— List Health Monitorsosc load-balancer healthmonitor set
— Update a Health Monitorosc load-balancer healthmonitor show
— Show Health Monitor details
osc load-balancer healthmonitor create
Creates a health monitor on a pool.
Health monitors define how the load balancer monitors backend servers to determine if they are available to service requests.
This operation provisions a new health monitor by using the configuration that you define in the request object. After the API validates the request and starts the provisioning process, the API returns a response object that contains a unique ID and the status of provisioning the health monitor.
In the response, the health monitor provisioning status is ACTIVE
, PENDING_CREATE
, or ERROR
.
If the status is PENDING_CREATE
, issue GET /v2/lbaas/healthmonitors/{healthmonitor_id}
to view the progress of the provisioning operation. When the health monitor status changes to ACTIVE
, the health monitor is successfully provisioned and is ready for further configuration.
If the API cannot fulfill the request due to insufficient data or data that is not valid, the service returns the HTTP Bad Request (400)
response code with information about the failure in the response body. Validation errors require that you correct the error and submit the request again.
Specifying a project_id is deprecated. The health monitor will inherit the project_id of the parent load balancer.
At a minimum, you must specify these health monitor attributes:
Some attributes receive default values if you omit them from the request:
To create a health monitor, the parent load balancer must have an ACTIVE
provisioning status.
Usage: osc load-balancer healthmonitor create [OPTIONS] --delay <DELAY> --max-retries <MAX_RETRIES> --pool-id <POOL_ID> --timeout <TIMEOUT> --type <TYPE>
Options:
-
--admin-state-up <ADMIN_STATE_UP>
— The administrative state of the resource, which is up (true
) or down (false
). Default istrue
Possible values:
true
,false
-
--delay <DELAY>
— The time, in seconds, between sending probes to members -
--domain-name <DOMAIN_NAME>
— The domain name, which be injected into the HTTP Host Header to the backend server for HTTP health check.New in version 2.10
-
--expected-codes <EXPECTED_CODES>
— The list of HTTP status codes expected in response from the member to declare it healthy. Specify one of the following values:- A single value, such as
200
- A list, such as200, 202
- A range, such as200-204
The default is 200.
- A single value, such as
-
--http-method <HTTP_METHOD>
— The HTTP method that the health monitor uses for requests. One ofCONNECT
,DELETE
,GET
,HEAD
,OPTIONS
,PATCH
,POST
,PUT
, orTRACE
. The default isGET
Possible values:
connect
,delete
,get
,head
,options
,patch
,post
,put
,trace
-
--http-version <HTTP_VERSION>
— The HTTP version. One of1.0
or1.1
. The default is1.0
.New in version 2.10
-
--max-retries <MAX_RETRIES>
— The number of successful checks before changing theoperating status
of the member toONLINE
. A valid value is from1
to10
-
--max-retries-down <MAX_RETRIES_DOWN>
— The number of allowed check failures before changing theoperating status
of the member toERROR
. A valid value is from1
to10
. The default is3
-
--name <NAME>
— Human-readable name of the resource -
--pool-id <POOL_ID>
— The ID of the pool -
--project-id <PROJECT_ID>
— The ID of the project owning this resource. (deprecated) -
--tags <TAGS>
— A list of simple strings assigned to the resource.New in version 2.5
Parameter is an array, may be provided multiple times.
-
--tenant-id <TENANT_ID>
-
--timeout <TIMEOUT>
— The maximum time, in seconds, that a monitor waits to connect before it times out. This value must be less than the delay value -
--type <TYPE>
— The type of health monitor. One ofHTTP
,HTTPS
,PING
,SCTP
,TCP
,TLS-HELLO
, orUDP-CONNECT
Possible values:
http
,https
,ping
,sctp
,tcp
,tls-hello
,udp-connect
-
--url-path <URL_PATH>
— The HTTP URL path of the request sent by the monitor to test the health of a backend member. Must be a string that begins with a forward slash (/
). The default URL path is/
osc load-balancer healthmonitor delete
Removes a health monitor and its associated configuration from the project.
The API immediately purges any and all configuration data, depending on the configuration settings. You cannot recover it.
Usage: osc load-balancer healthmonitor delete <ID>
Arguments:
<ID>
— healthmonitor_id parameter for /v2/lbaas/healthmonitors/{healthmonitor_id} API
osc load-balancer healthmonitor list
Lists all health monitors for the project.
Use the fields
query parameter to control which fields are returned in the response body. Additionally, you can filter results by using query string parameters. For information, see Filtering and column selection.
Administrative users can specify a project ID that is different than their own to list health monitors for other projects.
The list might be empty.
Usage: osc load-balancer healthmonitor list [OPTIONS]
Options:
-
--type <TYPE>
— The type of health monitorPossible values:
HTTP
,HTTPS
,PING
,SCTP
,TCP
,TLS-HELLO
,UDP-CONNECT
-
--admin-state-up <ADMIN_STATE_UP>
— The administrative state of the resourcePossible values:
true
,false
-
--created-at <CREATED_AT>
— The UTC date and timestamp when the resource was created -
--delay <DELAY>
— The time, in seconds, between sending probes to members -
--description <DESCRIPTION>
— A human-readable description for the resource -
--expected-codes <EXPECTED_CODES>
— The list of HTTP status codes expected in response from the member to declare it healthy -
--http-method <HTTP_METHOD>
— The HTTP method that the health monitor uses for requestsPossible values:
CONNECT
,DELETE
,GET
,HEAD
,OPTIONS
,PATCH
,POST
,PUT
,TRACE
-
--id <ID>
— The ID of the resource -
--limit <LIMIT>
— Page size -
--marker <MARKER>
— ID of the last item in the previous list -
--max-retries <MAX_RETRIES>
— The number of successful checks before changing the operating status of the member to ONLINE. A valid value is from 1 to 10 -
--max-retries-down <MAX_RETRIES_DOWN>
— The number of allowed check failures before changing the operating status of the member to ERROR. A valid value is from 1 to 10 -
--name <NAME>
— Human-readable name of the resource -
--not-tags <NOT_TAGS>
— Return the list of entities that do not have one or more of the given tags -
--not-tags-any <NOT_TAGS_ANY>
— Return the list of entities that do not have at least one of the given tags -
--operating-status <OPERATING_STATUS>
— The operating status of the resourcePossible values:
DEGRADED
,DRAINING
,ERROR
,NO_MONITOR
,OFFLINE
,ONLINE
-
--page-reverse <PAGE_REVERSE>
— The page directionPossible values:
true
,false
-
--pool-id <POOL_ID>
— The ID of the pool -
--project-name <PROJECT_NAME>
— Project Name -
--project-id <PROJECT_ID>
— Project ID -
--current-project
— Current project -
--provisioning-status <PROVISIONING_STATUS>
— The provisioning status of the resourcePossible values:
ACTIVE
,DELETED
,ERROR
,PENDING_CREATE
,PENDING_DELETE
,PENDING_UPDATE
-
--tags <TAGS>
— Return the list of entities that have this tag or tags -
--tags-any <TAGS_ANY>
— Return the list of entities that have one or more of the given tags -
--timeout <TIMEOUT>
— The maximum time, in seconds, that a monitor waits to connect before it times out -
--updated-at <UPDATED_AT>
— The UTC date and timestamp when the resource was last updated -
--url-path <URL_PATH>
— The HTTP URL path of the request sent by the monitor to test the health of a backend member. Must be a string that begins with a forward slash (/) -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc load-balancer healthmonitor set
Update an existing health monitor.
If the request is valid, the service returns the Accepted (202)
response code. To confirm the update, check that the health monitor provisioning status is ACTIVE
. If the status is PENDING_UPDATE
, use a GET operation to poll the health monitor object for changes.
This operation returns the updated health monitor object with the ACTIVE
, PENDING_UPDATE
, or ERROR
provisioning status.
Usage: osc load-balancer healthmonitor set [OPTIONS] <ID>
Arguments:
<ID>
— healthmonitor_id parameter for /v2/lbaas/healthmonitors/{healthmonitor_id} API
Options:
-
--admin-state-up <ADMIN_STATE_UP>
— The administrative state of the resource, which is up (true
) or down (false
). Default istrue
Possible values:
true
,false
-
--delay <DELAY>
— The time, in seconds, between sending probes to members -
--domain-name <DOMAIN_NAME>
— The domain name, which be injected into the HTTP Host Header to the backend server for HTTP health check.New in version 2.10
-
--expected-codes <EXPECTED_CODES>
— The list of HTTP status codes expected in response from the member to declare it healthy. Specify one of the following values:- A single value, such as
200
- A list, such as200, 202
- A range, such as200-204
The default is 200.
- A single value, such as
-
--http-method <HTTP_METHOD>
— The HTTP method that the health monitor uses for requests. One ofCONNECT
,DELETE
,GET
,HEAD
,OPTIONS
,PATCH
,POST
,PUT
, orTRACE
. The default isGET
Possible values:
connect
,delete
,get
,head
,options
,patch
,post
,put
,trace
-
--http-version <HTTP_VERSION>
— The HTTP version. One of1.0
or1.1
. The default is1.0
.New in version 2.10
-
--max-retries <MAX_RETRIES>
— The number of successful checks before changing theoperating status
of the member toONLINE
. A valid value is from1
to10
-
--max-retries-down <MAX_RETRIES_DOWN>
— The number of allowed check failures before changing theoperating status
of the member toERROR
. A valid value is from1
to10
. The default is3
-
--name <NAME>
— Human-readable name of the resource -
--tags <TAGS>
— A list of simple strings assigned to the resource.New in version 2.5
Parameter is an array, may be provided multiple times.
-
--timeout <TIMEOUT>
— The maximum time, in seconds, that a monitor waits to connect before it times out. This value must be less than the delay value -
--url-path <URL_PATH>
— The HTTP URL path of the request sent by the monitor to test the health of a backend member. Must be a string that begins with a forward slash (/
). The default URL path is/
osc load-balancer healthmonitor show
Shows the details of a health monitor.
If you are not an administrative user and the parent load balancer does not belong to your project, the service returns the HTTP Forbidden (403)
response code.
This operation does not require a request body.
Usage: osc load-balancer healthmonitor show <ID>
Arguments:
<ID>
— healthmonitor_id parameter for /v2/lbaas/healthmonitors/{healthmonitor_id} API
osc load-balancer l7policy
L7Policy (Octavia) commands
Usage: osc load-balancer l7policy <COMMAND>
Available subcommands:
osc load-balancer l7policy create
— Create an L7 Policyosc load-balancer l7policy delete
— Remove a L7 Policyosc load-balancer l7policy list
— List L7 Policiesosc load-balancer l7policy rule
—L7Policy Rule
commandsosc load-balancer l7policy set
— Update a L7 Policyosc load-balancer l7policy show
— Show L7 Policy details
osc load-balancer l7policy create
Creates a L7 policy.
This operation provisions a new L7 policy by using the configuration that you define in the request object. After the API validates the request and starts the provisioning process, the API returns a response object that contains a unique ID and the status of provisioning the L7 policy.
In the response, the L7 policy provisioning status is ACTIVE
, PENDING_CREATE
, or ERROR
.
If the status is PENDING_CREATE
, issue GET /v2/lbaas/l7policies/{l7policy_id}
to view the progress of the provisioning operation. When the L7 policy status changes to ACTIVE
, the L7 policy is successfully provisioned and is ready for further configuration.
If the API cannot fulfill the request due to insufficient data or data that is not valid, the service returns the HTTP Bad Request (400)
response code with information about the failure in the response body. Validation errors require that you correct the error and submit the request again.
All the rules associated with a given policy are logically ANDead together. A request must match all the policy’s rules to match the policy.
If you need to express a logical OR operation between rules, then do this by creating multiple policies with the same action.
If a new policy is created with a position that matches that of an existing policy, then the new policy is inserted at the given position.
L7 policies with action
of REDIRECT_TO_URL
will return the default HTTP Found (302)
response code with the redirect_url
. Also, specify redirect_http_code
to configure the needed HTTP response code, such as, 301, 302, 303, 307 and 308.
L7 policies with action
of REJECT
will return a Forbidden (403)
response code to the requester.
Usage: osc load-balancer l7policy create [OPTIONS] --action <ACTION> --listener-id <LISTENER_ID>
Options:
-
--action <ACTION>
— The L7 policy action. One ofREDIRECT_PREFIX
,REDIRECT_TO_POOL
,REDIRECT_TO_URL
, orREJECT
Possible values:
redirect-prefix
,redirect-to-pool
,redirect-to-url
,reject
-
--admin-state-up <ADMIN_STATE_UP>
— The administrative state of the resource, which is up (true
) or down (false
). Default istrue
Possible values:
true
,false
-
--description <DESCRIPTION>
— A human-readable description for the resource -
--listener-id <LISTENER_ID>
— The ID of the listener -
--name <NAME>
— Human-readable name of the resource -
--position <POSITION>
— The position of this policy on the listener. Positions start at 1 -
--project-id <PROJECT_ID>
— The ID of the project owning this resource -
--redirect-http-code <REDIRECT_HTTP_CODE>
— Requests matching this policy will be redirected to the specified URL or Prefix URL with the HTTP response code. Valid ifaction
isREDIRECT_TO_URL
orREDIRECT_PREFIX
. Valid options are: 301, 302, 303, 307, or 308. Default is 302.New in version 2.9
-
--redirect-pool-id <REDIRECT_POOL_ID>
— Requests matching this policy will be redirected to the pool with this ID. Only valid ifaction
isREDIRECT_TO_POOL
. The pool has some restrictions, See Protocol Combinations (Listener/Pool) -
--redirect-prefix <REDIRECT_PREFIX>
— Requests matching this policy will be redirected to this Prefix URL. Only valid ifaction
isREDIRECT_PREFIX
-
--redirect-url <REDIRECT_URL>
— Requests matching this policy will be redirected to this URL. Only valid ifaction
isREDIRECT_TO_URL
-
--rules <JSON>
— Parameter is an array, may be provided multiple times -
--tags <TAGS>
— Parameter is an array, may be provided multiple times -
--tenant-id <TENANT_ID>
osc load-balancer l7policy delete
Removes a L7 policy and its associated configuration from the project.
The API immediately purges any and all configuration data, depending on the configuration settings. You cannot recover it.
Usage: osc load-balancer l7policy delete <ID>
Arguments:
<ID>
— l7policy_id parameter for /v2/lbaas/l7policies/{l7policy_id} API
osc load-balancer l7policy list
Lists all L7 policies for the project.
Use the fields
query parameter to control which fields are returned in the response body. Additionally, you can filter results by using query string parameters. For information, see Filtering and column selection.
Administrative users can specify a project ID that is different than their own to list L7 policies for other projects.
The list might be empty.
Usage: osc load-balancer l7policy list [OPTIONS]
Options:
-
--action <ACTION>
-
--admin-state-up <ADMIN_STATE_UP>
Possible values:
true
,false
-
--description <DESCRIPTION>
-
--limit <LIMIT>
— Page size -
--listener-id <LISTENER_ID>
-
--marker <MARKER>
— ID of the last item in the previous list -
--name <NAME>
-
--operating-status <OPERATING_STATUS>
-
--page-reverse <PAGE_REVERSE>
— The page directionPossible values:
true
,false
-
--position <POSITION>
-
--project-name <PROJECT_NAME>
— Project Name -
--project-id <PROJECT_ID>
— Project ID -
--current-project
— Current project -
--provisioning-status <PROVISIONING_STATUS>
-
--redirect-pool-id <REDIRECT_POOL_ID>
-
--redirect-prefix <REDIRECT_PREFIX>
-
--redirect-url <REDIRECT_URL>
-
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc load-balancer l7policy rule
L7Policy Rule
commands
Usage: osc load-balancer l7policy rule <COMMAND>
Available subcommands:
osc load-balancer l7policy rule create
— Create an L7 Ruleosc load-balancer l7policy rule delete
— Remove a L7 Ruleosc load-balancer l7policy rule list
— List L7 Rulesosc load-balancer l7policy rule set
— Update a L7 Ruleosc load-balancer l7policy rule show
— Show L7 Rule details
osc load-balancer l7policy rule create
Creates a L7 rule.
This operation provisions a new L7 rule by using the configuration that you define in the request object. After the API validates the request and starts the provisioning process, the API returns a response object that contains a unique ID and the status of provisioning the L7 rule.
In the response, the L7 rule provisioning status is ACTIVE
, PENDING_CREATE
, or ERROR
.
If the status is PENDING_CREATE
, issue GET /v2/lbaas/l7policies/{l7policy_id}/rules/{l7rule_id}
to view the progress of the provisioning operation. When the L7 rule status changes to ACTIVE
, the L7 rule is successfully provisioned and is ready for further configuration.
If the API cannot fulfill the request due to insufficient data or data that is not valid, the service returns the HTTP Bad Request (400)
response code with information about the failure in the response body. Validation errors require that you correct the error and submit the request again.
All the rules associated with a given policy are logically ANDead together. A request must match all the policy’s rules to match the policy.
If you need to express a logical OR operation between rules, then do this by creating multiple policies with the same action.
Usage: osc load-balancer l7policy rule create [OPTIONS] --compare-type <COMPARE_TYPE> --type <TYPE> --value <VALUE> <L7POLICY_ID>
Arguments:
<L7POLICY_ID>
— l7policy_id parameter for /v2/lbaas/l7policies/{l7policy_id}/rules/{rule_id} API
Options:
-
--admin-state-up <ADMIN_STATE_UP>
— The administrative state of the resource, which is up (true
) or down (false
). Default istrue
Possible values:
true
,false
-
--compare-type <COMPARE_TYPE>
— The comparison type for the L7 rule. One ofCONTAINS
,ENDS_WITH
,EQUAL_TO
,REGEX
, orSTARTS_WITH
Possible values:
contains
,ends-with
,equal-to
,regex
,starts-with
-
--invert <INVERT>
— Whentrue
the logic of the rule is inverted. For example, with inverttrue
, equal to would become not equal to. Default isfalse
Possible values:
true
,false
-
--key <KEY>
— The key to use for the comparison. For example, the name of the cookie to evaluate -
--project-id <PROJECT_ID>
— The ID of the project owning this resource -
--tags <TAGS>
— A list of simple strings assigned to the resource.New in version 2.5
Parameter is an array, may be provided multiple times.
-
--tenant-id <TENANT_ID>
-
--type <TYPE>
— The L7 rule type. One ofCOOKIE
,FILE_TYPE
,HEADER
,HOST_NAME
,PATH
,SSL_CONN_HAS_CERT
,SSL_VERIFY_RESULT
, orSSL_DN_FIELD
Possible values:
cookie
,file-type
,header
,host-name
,path
,ssl-conn-has-cert
,ssl-dn-field
,ssl-verify-result
-
--value <VALUE>
— The value to use for the comparison. For example, the file type to compare
osc load-balancer l7policy rule delete
Removes a L7 rule and its associated configuration from the project.
The API immediately purges any and all configuration data, depending on the configuration settings. You cannot recover it.
Usage: osc load-balancer l7policy rule delete <L7POLICY_ID> <ID>
Arguments:
<L7POLICY_ID>
— l7policy_id parameter for /v2/lbaas/l7policies/{l7policy_id}/rules/{rule_id} API<ID>
— rule_id parameter for /v2/lbaas/l7policies/{l7policy_id}/rules/{rule_id} API
osc load-balancer l7policy rule list
Lists all L7 rules for the project.
Use the fields
query parameter to control which fields are returned in the response body. Additionally, you can filter results by using query string parameters. For information, see Filtering and column selection.
Administrative users can specify a project ID that is different than their own to list L7 policies for other projects.
The list might be empty.
Usage: osc load-balancer l7policy rule list [OPTIONS] <L7POLICY_ID>
Arguments:
<L7POLICY_ID>
— l7policy_id parameter for /v2/lbaas/l7policies/{l7policy_id}/rules/{rule_id} API
Options:
-
--type <TYPE>
-
--admin-state-up <ADMIN_STATE_UP>
Possible values:
true
,false
-
--compare-type <COMPARE_TYPE>
-
--created-at <CREATED_AT>
-
--invert <INVERT>
-
--key <KEY>
-
--limit <LIMIT>
— Page size -
--marker <MARKER>
— ID of the last item in the previous list -
--operating-status <OPERATING_STATUS>
-
--page-reverse <PAGE_REVERSE>
— The page directionPossible values:
true
,false
-
--project-name <PROJECT_NAME>
— Project Name -
--project-id <PROJECT_ID>
— Project ID -
--current-project
— Current project -
--provisioning-status <PROVISIONING_STATUS>
-
--rule-value <RULE_VALUE>
-
--updated-at <UPDATED_AT>
-
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc load-balancer l7policy rule set
Updates a L7 rule.
If the request is valid, the service returns the Accepted (202)
response code. To confirm the update, check that the L7 rule provisioning status is ACTIVE
. If the status is PENDING_UPDATE
, use a GET operation to poll the L7 rule object for changes.
This operation returns the updated L7 rule object with the ACTIVE
, PENDING_UPDATE
, or ERROR
provisioning status.
Usage: osc load-balancer l7policy rule set [OPTIONS] <L7POLICY_ID> <ID>
Arguments:
<L7POLICY_ID>
— l7policy_id parameter for /v2/lbaas/l7policies/{l7policy_id}/rules/{rule_id} API<ID>
— rule_id parameter for /v2/lbaas/l7policies/{l7policy_id}/rules/{rule_id} API
Options:
-
--admin-state-up <ADMIN_STATE_UP>
— The administrative state of the resource, which is up (true
) or down (false
). Default istrue
Possible values:
true
,false
-
--compare-type <COMPARE_TYPE>
— The comparison type for the L7 rule. One ofCONTAINS
,ENDS_WITH
,EQUAL_TO
,REGEX
, orSTARTS_WITH
Possible values:
contains
,ends-with
,equal-to
,regex
,starts-with
-
--invert <INVERT>
— Whentrue
the logic of the rule is inverted. For example, with inverttrue
, equal to would become not equal to. Default isfalse
Possible values:
true
,false
-
--key <KEY>
— The key to use for the comparison. For example, the name of the cookie to evaluate -
--tags <TAGS>
— A list of simple strings assigned to the resource.New in version 2.5
Parameter is an array, may be provided multiple times.
-
--type <TYPE>
— The L7 rule type. One ofCOOKIE
,FILE_TYPE
,HEADER
,HOST_NAME
,PATH
,SSL_CONN_HAS_CERT
,SSL_VERIFY_RESULT
, orSSL_DN_FIELD
Possible values:
cookie
,file-type
,header
,host-name
,path
,ssl-conn-has-cert
,ssl-dn-field
,ssl-verify-result
-
--value <VALUE>
— The value to use for the comparison. For example, the file type to compare
osc load-balancer l7policy rule show
Shows the details of a L7 rule.
If you are not an administrative user and the L7 rule object does not belong to your project, the service returns the HTTP Forbidden (403)
response code.
This operation does not require a request body.
Usage: osc load-balancer l7policy rule show <L7POLICY_ID> <ID>
Arguments:
<L7POLICY_ID>
— l7policy_id parameter for /v2/lbaas/l7policies/{l7policy_id}/rules/{rule_id} API<ID>
— rule_id parameter for /v2/lbaas/l7policies/{l7policy_id}/rules/{rule_id} API
osc load-balancer l7policy set
Updates a L7 policy.
If the request is valid, the service returns the Accepted (202)
response code. To confirm the update, check that the L7 policy provisioning status is ACTIVE
. If the status is PENDING_UPDATE
, use a GET operation to poll the L7 policy object for changes.
This operation returns the updated L7 policy object with the ACTIVE
, PENDING_UPDATE
, or ERROR
provisioning status.
If a policy is updated with a position that matches that of an existing policy, then the updated policy is inserted at the given position.
Usage: osc load-balancer l7policy set [OPTIONS] <ID>
Arguments:
<ID>
— l7policy_id parameter for /v2/lbaas/l7policies/{l7policy_id} API
Options:
-
--action <ACTION>
— The L7 policy action. One ofREDIRECT_PREFIX
,REDIRECT_TO_POOL
,REDIRECT_TO_URL
, orREJECT
Possible values:
redirect-prefix
,redirect-to-pool
,redirect-to-url
,reject
-
--admin-state-up <ADMIN_STATE_UP>
— The administrative state of the resource, which is up (true
) or down (false
). Default istrue
Possible values:
true
,false
-
--description <DESCRIPTION>
— A human-readable description for the resource -
--name <NAME>
— Human-readable name of the resource -
--position <POSITION>
— The position of this policy on the listener. Positions start at 1 -
--redirect-http-code <REDIRECT_HTTP_CODE>
— Requests matching this policy will be redirected to the specified URL or Prefix URL with the HTTP response code. Valid ifaction
isREDIRECT_TO_URL
orREDIRECT_PREFIX
. Valid options are: 301, 302, 303, 307, or 308. Default is 302.New in version 2.9
-
--redirect-pool-id <REDIRECT_POOL_ID>
— Requests matching this policy will be redirected to the pool with this ID. Only valid ifaction
isREDIRECT_TO_POOL
. The pool has some restrictions, See Protocol Combinations (Listener/Pool) -
--redirect-prefix <REDIRECT_PREFIX>
— Requests matching this policy will be redirected to this Prefix URL. Only valid ifaction
isREDIRECT_PREFIX
-
--redirect-url <REDIRECT_URL>
— Requests matching this policy will be redirected to this URL. Only valid ifaction
isREDIRECT_TO_URL
-
--tags <TAGS>
— A list of simple strings assigned to the resource.New in version 2.5
Parameter is an array, may be provided multiple times.
osc load-balancer l7policy show
Shows the details of a L7 policy.
If you are not an administrative user and the L7 policy object does not belong to your project, the service returns the HTTP Forbidden (403)
response code.
This operation does not require a request body.
Usage: osc load-balancer l7policy show <ID>
Arguments:
<ID>
— l7policy_id parameter for /v2/lbaas/l7policies/{l7policy_id} API
osc load-balancer listener
Listener (Octavia) commands
Usage: osc load-balancer listener <COMMAND>
Available subcommands:
osc load-balancer listener create
— Create Listenerosc load-balancer listener delete
— Remove a Listenerosc load-balancer listener list
— List Listenersosc load-balancer listener set
— Update a Listenerosc load-balancer listener show
— Show Listener detailsosc load-balancer listener stats
— Get Listener statistics
osc load-balancer listener create
Creates a listener for a load balancer.
The listener configures a port and protocol for the load balancer to listen on for incoming requests. A load balancer may have zero or more listeners configured.
This operation provisions a new listener by using the configuration that you define in the request object. After the API validates the request and starts the provisioning process, the API returns a response object that contains a unique ID and the status of provisioning the listener.
In the response, the listener provisioning status is ACTIVE
, PENDING_CREATE
, or ERROR
.
If the status is PENDING_CREATE
, issue GET /v2/lbaas/listeners/{listener_id}
to view the progress of the provisioning operation. When the listener status changes to ACTIVE
, the listener is successfully provisioned and is ready for further configuration.
If the API cannot fulfill the request due to insufficient data or data that is not valid, the service returns the HTTP Bad Request (400)
response code with information about the failure in the response body. Validation errors require that you correct the error and submit the request again.
Specifying a project_id is deprecated. The listener will inherit the project_id of the parent load balancer.
You can configure all documented features of the listener at creation time by specifying the additional elements or attributes in the request.
To create a listener, the parent load balancer must have an ACTIVE
provisioning status.
Usage: osc load-balancer listener create [OPTIONS] --loadbalancer-id <LOADBALANCER_ID> --protocol <PROTOCOL> --protocol-port <PROTOCOL_PORT>
Options:
-
--admin-state-up <ADMIN_STATE_UP>
— The administrative state of the resource, which is up (true
) or down (false
). Default istrue
Possible values:
true
,false
-
--allowed-cidrs <ALLOWED_CIDRS>
— A list of IPv4, IPv6 or mix of both CIDRs. The default is all allowed. When a list of CIDRs is provided, the default switches to deny all.New in version 2.12
Parameter is an array, may be provided multiple times.
-
--alpn-protocols <ALPN_PROTOCOLS>
— Parameter is an array, may be provided multiple times -
--client-authentication <CLIENT_AUTHENTICATION>
— The TLS client authentication mode. One of the optionsNONE
,OPTIONAL
orMANDATORY
.New in version 2.8
Possible values:
mandatory
,none
,optional
-
--client-ca-tls-container-ref <CLIENT_CA_TLS_CONTAINER_REF>
— The ref of the key manager service secret containing a PEM format client CA certificate bundle forTERMINATED_HTTPS
listeners.New in version 2.8
-
--client-crl-container-ref <CLIENT_CRL_CONTAINER_REF>
— The URI of the key manager service secret containing a PEM format CA revocation list file forTERMINATED_HTTPS
listeners.New in version 2.8
-
--connection-limit <CONNECTION_LIMIT>
— The maximum number of connections permitted for this listener. Default value is -1 which represents infinite connections or a default value defined by the provider driver -
--default-pool <JSON>
— A pool object -
--default-pool-id <DEFAULT_POOL_ID>
— The ID of the pool used by the listener if no L7 policies match. The pool has some restrictions. See Protocol Combinations (Listener/Pool) -
--default-tls-container-ref <DEFAULT_TLS_CONTAINER_REF>
— The URI of the key manager service secret containing a PKCS12 format certificate/key bundle forTERMINATED_HTTPS
listeners. DEPRECATED: A secret container of type “certificate” containing the certificate and key forTERMINATED_HTTPS
listeners -
--description <DESCRIPTION>
— A human-readable description for the resource -
--hsts-include-subdomains <HSTS_INCLUDE_SUBDOMAINS>
— Defines whether theincludeSubDomains
directive should be added to the Strict-Transport-Security HTTP response header. This requires setting thehsts_max_age
option as well in order to become effective.New in version 2.27
Possible values:
true
,false
-
--hsts-max-age <HSTS_MAX_AGE>
— The value of themax_age
directive for the Strict-Transport-Security HTTP response header. Setting this enables HTTP Strict Transport Security (HSTS) for the TLS-terminated listener.New in version 2.27
-
--hsts-preload <HSTS_PRELOAD>
— Defines whether thepreload
directive should be added to the Strict-Transport-Security HTTP response header. This requires setting thehsts_max_age
option as well in order to become effective.New in version 2.27
Possible values:
true
,false
-
--insert-headers <key=value>
— A dictionary of optional headers to insert into the request before it is sent to the backendmember
. See Supported HTTP Header Insertions. Both keys and values are always specified as strings -
--l7policies <JSON>
— A list of L7 policy objects.Parameter is an array, may be provided multiple times.
-
--loadbalancer-id <LOADBALANCER_ID>
— The ID of the load balancer -
--name <NAME>
— Human-readable name of the resource -
--project-id <PROJECT_ID>
— The ID of the project owning this resource. (deprecated) -
--protocol <PROTOCOL>
— The protocol for the resource. One ofHTTP
,HTTPS
,SCTP
,PROMETHEUS
,TCP
,TERMINATED_HTTPS
, orUDP
Possible values:
http
,https
,prometheus
,sctp
,tcp
,terminated-https
,udp
-
--protocol-port <PROTOCOL_PORT>
— The protocol port number for the resource -
--sni-container-refs <SNI_CONTAINER_REFS>
— A list of URIs to the key manager service secrets containing PKCS12 format certificate/key bundles forTERMINATED_HTTPS
listeners. (DEPRECATED) Secret containers of type “certificate” containing the certificates and keys forTERMINATED_HTTPS
listeners.Parameter is an array, may be provided multiple times.
-
--tags <TAGS>
— Parameter is an array, may be provided multiple times -
--tenant-id <TENANT_ID>
-
--timeout-client-data <TIMEOUT_CLIENT_DATA>
— Frontend client inactivity timeout in milliseconds. Default: 50000.New in version 2.1
-
--timeout-member-connect <TIMEOUT_MEMBER_CONNECT>
— Backend member connection timeout in milliseconds. Default: 5000.New in version 2.1
-
--timeout-member-data <TIMEOUT_MEMBER_DATA>
— Backend member inactivity timeout in milliseconds. Default: 50000.New in version 2.1
-
--timeout-tcp-inspect <TIMEOUT_TCP_INSPECT>
— Time, in milliseconds, to wait for additional TCP packets for content inspection. Default: 0.New in version 2.1
-
--tls-ciphers <TLS_CIPHERS>
-
--tls-versions <TLS_VERSIONS>
— Parameter is an array, may be provided multiple times
osc load-balancer listener delete
Removes a listener and its associated configuration from the project.
The API immediately purges any and all configuration data, depending on the configuration settings. You cannot recover it.
Usage: osc load-balancer listener delete <ID>
Arguments:
<ID>
— listener_id parameter for /v2/lbaas/listeners/{listener_id} API
osc load-balancer listener list
Lists all listeners for the project.
Use the fields
query parameter to control which fields are returned in the response body. Additionally, you can filter results by using query string parameters. For information, see Filtering and column selection.
Administrative users can specify a project ID that is different than their own to list listeners for other projects.
The list might be empty.
Usage: osc load-balancer listener list [OPTIONS]
Options:
-
--admin-state-up <ADMIN_STATE_UP>
— The administrative state of the resourcePossible values:
true
,false
-
--alpn-protocols <ALPN_PROTOCOLS>
— A list of ALPN protocols. Available protocols: http/1.0, http/1.1, h2 -
--connection-limit <CONNECTION_LIMIT>
— The maximum number of connections permitted for this listener. Default value is -1 which represents infinite connections or a default value defined by the provider driver -
--created-at <CREATED_AT>
— The UTC date and timestamp when the resource was created -
--default-pool-id <DEFAULT_POOL_ID>
— The ID of the pool used by the listener if no L7 policies match -
--description <DESCRIPTION>
— A human-readable description for the resource -
--hsts-include-subdomains <HSTS_INCLUDE_SUBDOMAINS>
— Defines whether the includeSubDomains directive should be added to the Strict-Transport-Security HTTP response headerPossible values:
true
,false
-
--hsts-max-age <HSTS_MAX_AGE>
— The value of the max_age directive for the Strict-Transport-Security HTTP response header -
--hsts-preload <HSTS_PRELOAD>
— Defines whether the preload directive should be added to the Strict-Transport-Security HTTP response headerPossible values:
true
,false
-
--id <ID>
— The ID of the resource -
--limit <LIMIT>
— Page size -
--load-balancer-id <LOAD_BALANCER_ID>
— Load balancer ID -
--marker <MARKER>
— ID of the last item in the previous list -
--name <NAME>
— Human-readable name of the resource -
--not-tags <NOT_TAGS>
— Return the list of entities that do not have one or more of the given tags -
--not-tags-any <NOT_TAGS_ANY>
— Return the list of entities that do not have at least one of the given tags -
--operating-status <OPERATING_STATUS>
— The operating status of the resourcePossible values:
DEGRADED
,DRAINING
,ERROR
,NO_MONITOR
,OFFLINE
,ONLINE
-
--page-reverse <PAGE_REVERSE>
— The page directionPossible values:
true
,false
-
--project-name <PROJECT_NAME>
— Project Name -
--project-id <PROJECT_ID>
— Project ID -
--current-project
— Current project -
--protocol <PROTOCOL>
— The protocol for the resourcePossible values:
HTTP
,HTTPS
,PROMETHEUS
,SCTP
,TCP
,TERMINATED_HTTPS
,UDP
-
--protocol-port <PROTOCOL_PORT>
— The protocol port number for the resource -
--provisioning-status <PROVISIONING_STATUS>
— The provisioning status of the resourcePossible values:
ACTIVE
,DELETED
,ERROR
,PENDING_CREATE
,PENDING_DELETE
,PENDING_UPDATE
-
--tags <TAGS>
— Return the list of entities that have this tag or tags -
--tags-any <TAGS_ANY>
— Return the list of entities that have one or more of the given tags -
--timeout-client-data <TIMEOUT_CLIENT_DATA>
— Frontend client inactivity timeout in milliseconds -
--timeout-member-connect <TIMEOUT_MEMBER_CONNECT>
— Backend member connection timeout in milliseconds -
--timeout-member-data <TIMEOUT_MEMBER_DATA>
— Backend member inactivity timeout in milliseconds -
--timeout-tcp-inspect <TIMEOUT_TCP_INSPECT>
— Time, in milliseconds, to wait for additional TCP packets for content inspection -
--tls-ciphers <TLS_CIPHERS>
— List of ciphers in OpenSSL format -
--tls-versions <TLS_VERSIONS>
— A list of TLS protocol versions -
--updated-at <UPDATED_AT>
— The UTC date and timestamp when the resource was last updated -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc load-balancer listener set
Update an existing listener.
If the request is valid, the service returns the Accepted (202)
response code. To confirm the update, check that the listener provisioning status is ACTIVE
. If the status is PENDING_UPDATE
, use a GET operation to poll the listener object for changes.
This operation returns the updated listener object with the ACTIVE
, PENDING_UPDATE
, or ERROR
provisioning status.
Usage: osc load-balancer listener set [OPTIONS] <ID>
Arguments:
<ID>
— listener_id parameter for /v2/lbaas/listeners/{listener_id} API
Options:
-
--admin-state-up <ADMIN_STATE_UP>
— The administrative state of the resource, which is up (true
) or down (false
). Default istrue
Possible values:
true
,false
-
--allowed-cidrs <ALLOWED_CIDRS>
— A list of IPv4, IPv6 or mix of both CIDRs. The default is all allowed. When a list of CIDRs is provided, the default switches to deny all.New in version 2.12
Parameter is an array, may be provided multiple times.
-
--alpn-protocols <ALPN_PROTOCOLS>
— A list of ALPN protocols. Available protocols: http/1.0, http/1.1, h2New in version 2.20
Parameter is an array, may be provided multiple times.
-
--client-authentication <CLIENT_AUTHENTICATION>
— The TLS client authentication mode. One of the optionsNONE
,OPTIONAL
orMANDATORY
.New in version 2.8
Possible values:
mandatory
,none
,optional
-
--client-ca-tls-container-ref <CLIENT_CA_TLS_CONTAINER_REF>
— The ref of the key manager service secret containing a PEM format client CA certificate bundle forTERMINATED_HTTPS
listeners.New in version 2.8
-
--client-crl-container-ref <CLIENT_CRL_CONTAINER_REF>
— The URI of the key manager service secret containing a PEM format CA revocation list file forTERMINATED_HTTPS
listeners.New in version 2.8
-
--connection-limit <CONNECTION_LIMIT>
— The maximum number of connections permitted for this listener. Default value is -1 which represents infinite connections or a default value defined by the provider driver -
--default-pool-id <DEFAULT_POOL_ID>
— The ID of the pool used by the listener if no L7 policies match. The pool has some restrictions. See Protocol Combinations (Listener/Pool) -
--default-tls-container-ref <DEFAULT_TLS_CONTAINER_REF>
— The URI of the key manager service secret containing a PKCS12 format certificate/key bundle forTERMINATED_HTTPS
listeners. DEPRECATED: A secret container of type “certificate” containing the certificate and key forTERMINATED_HTTPS
listeners -
--description <DESCRIPTION>
— A human-readable description for the resource -
--hsts-include-subdomains <HSTS_INCLUDE_SUBDOMAINS>
— Defines whether theincludeSubDomains
directive should be added to the Strict-Transport-Security HTTP response header. This requires setting thehsts_max_age
option as well in order to become effective.New in version 2.27
Possible values:
true
,false
-
--hsts-max-age <HSTS_MAX_AGE>
— The value of themax_age
directive for the Strict-Transport-Security HTTP response header. Setting this enables HTTP Strict Transport Security (HSTS) for the TLS-terminated listener.New in version 2.27
-
--hsts-preload <HSTS_PRELOAD>
— Defines whether thepreload
directive should be added to the Strict-Transport-Security HTTP response header. This requires setting thehsts_max_age
option as well in order to become effective.New in version 2.27
Possible values:
true
,false
-
--insert-headers <key=value>
— A dictionary of optional headers to insert into the request before it is sent to the backendmember
. See Supported HTTP Header Insertions. Both keys and values are always specified as strings -
--name <NAME>
— Human-readable name of the resource -
--sni-container-refs <SNI_CONTAINER_REFS>
— A list of URIs to the key manager service secrets containing PKCS12 format certificate/key bundles forTERMINATED_HTTPS
listeners. (DEPRECATED) Secret containers of type “certificate” containing the certificates and keys forTERMINATED_HTTPS
listeners.Parameter is an array, may be provided multiple times.
-
--tags <TAGS>
— A list of simple strings assigned to the resource.New in version 2.5
Parameter is an array, may be provided multiple times.
-
--timeout-client-data <TIMEOUT_CLIENT_DATA>
— Frontend client inactivity timeout in milliseconds. Default: 50000.New in version 2.1
-
--timeout-member-connect <TIMEOUT_MEMBER_CONNECT>
— Backend member connection timeout in milliseconds. Default: 5000.New in version 2.1
-
--timeout-member-data <TIMEOUT_MEMBER_DATA>
— Backend member inactivity timeout in milliseconds. Default: 50000.New in version 2.1
-
--timeout-tcp-inspect <TIMEOUT_TCP_INSPECT>
— Time, in milliseconds, to wait for additional TCP packets for content inspection. Default: 0.New in version 2.1
-
--tls-ciphers <TLS_CIPHERS>
— List of ciphers in OpenSSL format (colon-separated). See https://www.openssl.org/docs/man1.1.1/man1/ciphers.htmlNew in version 2.15
-
--tls-versions <TLS_VERSIONS>
— A list of TLS protocol versions. Available versions: SSLv3, TLSv1, TLSv1.1, TLSv1.2, TLSv1.3New in version 2.17
Parameter is an array, may be provided multiple times.
osc load-balancer listener show
Shows the details of a listener.
If you are not an administrative user and the parent load balancer does not belong to your project, the service returns the HTTP Forbidden (403)
response code.
This operation does not require a request body.
Usage: osc load-balancer listener show <ID>
Arguments:
<ID>
— listener_id parameter for /v2/lbaas/listeners/{listener_id} API
osc load-balancer listener stats
Shows the current statistics for a listener.
This operation returns the statistics of a listener object identified by listener_id.
If you are not an administrative user and the parent load balancer does not belong to your project, the service returns the HTTP Forbidden (403)
response code.
This operation does not require a request body.
Usage: osc load-balancer listener stats <ID>
Arguments:
<ID>
— listener_id parameter for /v2/lbaas/listeners/{listener_id}/stats API
osc load-balancer loadbalancer
Loadbalancer (Octavia) commands
Usage: osc load-balancer loadbalancer <COMMAND>
Available subcommands:
osc load-balancer loadbalancer create
— Create a Load Balancerosc load-balancer loadbalancer delete
— Remove a Load Balancerosc load-balancer loadbalancer failover
— Failover a load balancerosc load-balancer loadbalancer list
— List Load Balancersosc load-balancer loadbalancer set
— Update a Load Balancerosc load-balancer loadbalancer show
— Show Load Balancer detailsosc load-balancer loadbalancer stats
— Get Load Balancer statisticsosc load-balancer loadbalancer status
— Get the Load Balancer status tree
osc load-balancer loadbalancer create
Creates a load balancer.
This operation provisions a new load balancer by using the configuration that you define in the request object. After the API validates the request and starts the provisioning process, the API returns a response object that contains a unique ID and the status of provisioning the load balancer.
In the response, the load balancer provisioning status is ACTIVE
, PENDING_CREATE
, or ERROR
.
If the status is PENDING_CREATE
, issue GET /v2/lbaas/loadbalancers/{loadbalancer_id}
to view the progress of the provisioning operation. When the load balancer status changes to ACTIVE
, the load balancer is successfully provisioned and is ready for further configuration.
If the API cannot fulfill the request due to insufficient data or data that is not valid, the service returns the HTTP Bad Request (400)
response code with information about the failure in the response body. Validation errors require that you correct the error and submit the request again.
Administrative users can specify a project ID that is different than their own to create load balancers for other projects.
An optional flavor_id
attribute can be used to create the load balancer using a pre-configured octavia flavor. Flavors are created by the operator to allow custom load balancer configurations, such as allocating more memory for the load balancer.
An optional vip_qos_policy_id
attribute from Neutron can be used to apply QoS policies on a loadbalancer VIP, also could pass a ‘null’ value to remove QoS policies.
You can also specify the provider
attribute when you create a load balancer. The provider
attribute specifies which backend should be used to create the load balancer. This could be the default provider (octavia
) or a vendor supplied provider
if one has been installed. Setting both a flavor_id and a provider will result in a conflict error if the provider does not match the provider of the configured flavor profiles.
Specifying a Virtual IP (VIP) is mandatory. There are three ways to specify a VIP network for the load balancer:
Additional VIPs may also be specified in the additional_vips
field, by providing a list of JSON objects containing a subnet_id
and optionally an ip_address
. All additional subnets must be part of the same network as the primary VIP.
An optional vip_sg_ids
attribute can be used to set custom Neutron Security Groups that are applied on the VIP port of the Load Balancer. When this option is used, Octavia does not manage the security of the Listeners, the user must set Security Group Rules to allow the network traffic on the VIP port. vip_sg_ids
are incompatible with SR-IOV load balancer and cannot be set if the load balancer has a listener that uses allowed_cidrs
.
Usage: osc load-balancer loadbalancer create [OPTIONS]
Options:
-
--additional-vips <JSON>
— A list of JSON objects defining “additional VIPs”. The format for these is{"subnet_id": <subnet_id>, "ip_address": <ip_address>}
, where thesubnet_id
field is mandatory and theip_address
field is optional. Additional VIP subnets must all belong to the same network as the primary VIP.New in version 2.26
Parameter is an array, may be provided multiple times.
-
--admin-state-up <ADMIN_STATE_UP>
— The administrative state of the resource, which is up (true
) or down (false
). Default istrue
Possible values:
true
,false
-
--availability-zone <AVAILABILITY_ZONE>
— An availability zone name -
--description <DESCRIPTION>
— A human-readable description for the resource -
--flavor-id <FLAVOR_ID>
— The ID of the flavor -
--listeners <JSON>
— The associated listener IDs, if any.Parameter is an array, may be provided multiple times.
-
--name <NAME>
— Human-readable name of the resource -
--pools <JSON>
— Parameter is an array, may be provided multiple times -
--project-id <PROJECT_ID>
— The ID of the project owning this resource -
--provider <PROVIDER>
— Provider name for the load balancer. Default isoctavia
-
--tags <TAGS>
— Parameter is an array, may be provided multiple times -
--tenant-id <TENANT_ID>
-
--vip-address <VIP_ADDRESS>
— The IP address of the Virtual IP (VIP) -
--vip-network-id <VIP_NETWORK_ID>
— The ID of the network for the Virtual IP (VIP). One ofvip_network_id
,vip_port_id
, orvip_subnet_id
must be specified -
--vip-port-id <VIP_PORT_ID>
— The ID of the Virtual IP (VIP) port. One ofvip_network_id
,vip_port_id
, orvip_subnet_id
must be specified -
--vip-qos-policy-id <VIP_QOS_POLICY_ID>
— The ID of the QoS Policy which will apply to the Virtual IP (VIP) -
--vip-sg-ids <VIP_SG_IDS>
— The list of Security Group IDs of the Virtual IP (VIP) port of the Load Balancer.New in version 2.29
Parameter is an array, may be provided multiple times.
-
--vip-subnet-id <VIP_SUBNET_ID>
— The ID of the subnet for the Virtual IP (VIP). One ofvip_network_id
,vip_port_id
, orvip_subnet_id
must be specified
osc load-balancer loadbalancer delete
Removes a load balancer and its associated configuration from the project.
The optional parameter cascade
when defined as true
will delete all child objects of the load balancer.
The API immediately purges any and all configuration data, depending on the configuration settings. You cannot recover it.
Usage: osc load-balancer loadbalancer delete [OPTIONS] <ID>
Arguments:
<ID>
— loadbalancer_id parameter for /v2/lbaas/loadbalancers/{loadbalancer_id} API
Options:
-
--cascade <CASCADE>
— If true will delete all child objects of the load balancerPossible values:
true
,false
osc load-balancer loadbalancer failover
Performs a failover of a load balancer.
This operation is only available to users with load balancer administrative rights.
Usage: osc load-balancer loadbalancer failover <ID>
Arguments:
<ID>
— loadbalancer_id parameter for /v2/lbaas/loadbalancers/{loadbalancer_id}/failover API
osc load-balancer loadbalancer list
Lists all load balancers for the project.
Use the fields
query parameter to control which fields are returned in the response body. Additionally, you can filter results by using query string parameters. For information, see Filtering and column selection.
Administrative users can specify a project ID that is different than their own to list load balancers for other projects.
The list might be empty.
Usage: osc load-balancer loadbalancer list [OPTIONS]
Options:
-
--availability-zone <AVAILABILITY_ZONE>
— An availability zone name -
--created-at <CREATED_AT>
— The UTC date and timestamp when the resource was created -
--description <DESCRIPTION>
— A human-readable description for the resource -
--flavor-id <FLAVOR_ID>
— The ID of the flavor -
--id <ID>
— The ID of the resource -
--limit <LIMIT>
— Page size -
--marker <MARKER>
— ID of the last item in the previous list -
--name <NAME>
— Human-readable name of the resource -
--not-tags <NOT_TAGS>
— Return the list of entities that do not have one or more of the given tags -
--not-tags-any <NOT_TAGS_ANY>
— Return the list of entities that do not have at least one of the given tags -
--operating-status <OPERATING_STATUS>
— The operating status of the resourcePossible values:
DEGRADED
,DRAINING
,ERROR
,NO_MONITOR
,OFFLINE
,ONLINE
-
--page-reverse <PAGE_REVERSE>
— The page directionPossible values:
true
,false
-
--project-name <PROJECT_NAME>
— Project Name -
--project-id <PROJECT_ID>
— Project ID -
--current-project
— Current project -
--provider <PROVIDER>
— Provider name for the load balancer -
--provisioning-status <PROVISIONING_STATUS>
— The provisioning status of the resourcePossible values:
ACTIVE
,DELETED
,ERROR
,PENDING_CREATE
,PENDING_DELETE
,PENDING_UPDATE
-
--tags <TAGS>
— Return the list of entities that have this tag or tags -
--tags-any <TAGS_ANY>
— Return the list of entities that have one or more of the given tags -
--updated-at <UPDATED_AT>
— The UTC date and timestamp when the resource was last updated -
--vip-address <VIP_ADDRESS>
— The IP address of the Virtual IP (VIP) -
--vip-network-id <VIP_NETWORK_ID>
— The ID of the network for the Virtual IP (VIP) -
--vip-port-id <VIP_PORT_ID>
— The ID of the Virtual IP (VIP) port -
--vip-qos-policy-id <VIP_QOS_POLICY_ID>
— The ID of the QoS Policy which will apply to the Virtual IP (VIP) -
--vip-subnet-id <VIP_SUBNET_ID>
— The ID of the subnet for the Virtual IP (VIP) -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc load-balancer loadbalancer set
Updates a load balancer.
If the request is valid, the service returns the Accepted (202)
response code. To confirm the update, check that the load balancer provisioning status is ACTIVE
. If the status is PENDING_UPDATE
, use a GET operation to poll the load balancer object for changes.
This operation returns the updated load balancer object with the ACTIVE
, PENDING_UPDATE
, or ERROR
provisioning status.
Usage: osc load-balancer loadbalancer set [OPTIONS] <ID>
Arguments:
<ID>
— loadbalancer_id parameter for /v2/lbaas/loadbalancers/{loadbalancer_id} API
Options:
-
--admin-state-up <ADMIN_STATE_UP>
— The administrative state of the resource, which is up (true
) or down (false
)Possible values:
true
,false
-
--description <DESCRIPTION>
— A human-readable description for the resource -
--name <NAME>
— Human-readable name of the resource -
--tags <TAGS>
— A list of simple strings assigned to the resource.New in version 2.5
Parameter is an array, may be provided multiple times.
-
--vip-qos-policy-id <VIP_QOS_POLICY_ID>
— The ID of the QoS Policy which will apply to the Virtual IP (VIP) -
--vip-sg-ids <VIP_SG_IDS>
— Parameter is an array, may be provided multiple times
osc load-balancer loadbalancer show
Shows the details of a load balancer.
If you are not an administrative user and the load balancer object does not belong to your project, the service returns the HTTP Forbidden (403)
response code.
This operation does not require a request body.
Usage: osc load-balancer loadbalancer show <ID>
Arguments:
<ID>
— loadbalancer_id parameter for /v2/lbaas/loadbalancers/{loadbalancer_id} API
osc load-balancer loadbalancer stats
Shows the current statistics for a load balancer.
This operation returns the statistics of a load balancer object identified by loadbalancer_id.
If you are not an administrative user and the load balancer object does not belong to your project, the service returns the HTTP Forbidden (403)
response code.
This operation does not require a request body.
Usage: osc load-balancer loadbalancer stats <ID>
Arguments:
<ID>
— loadbalancer_id parameter for /v2/lbaas/loadbalancers/{loadbalancer_id}/stats API
osc load-balancer loadbalancer status
Shows the status tree for a load balancer.
This operation returns a status tree for a load balancer object, by load balancer ID.
provisioning_status
is the status associated with lifecycle of the resource. See Provisioning Status Codes for descriptions of the status codes.
operating_status
is the observed status of the resource. See Operating Status Codes for descriptions of the status codes.
If you are not an administrative user and the load balancer object does not belong to your project, the service returns the HTTP Forbidden (403)
response code.
If the operation succeeds, the returned element is a status tree that contains the load balancer and all provisioning and operating statuses for its children.
Usage: osc load-balancer loadbalancer status <ID>
Arguments:
<ID>
— loadbalancer_id parameter for /v2/lbaas/loadbalancers/{loadbalancer_id}/status API
osc load-balancer pool
Pool (Octavia) commands
Usage: osc load-balancer pool <COMMAND>
Available subcommands:
osc load-balancer pool create
— Create Poolosc load-balancer pool delete
— Remove a Poolosc load-balancer pool list
— List Poolsosc load-balancer pool member
— Pool Member commandsosc load-balancer pool set
— Update a Poolosc load-balancer pool show
— Show Pool details
osc load-balancer pool create
Creates a pool for a load balancer.
The pool defines how requests should be balanced across the backend member servers.
This operation provisions a pool by using the configuration that you define in the request object. After the API validates the request and starts the provisioning process, the API returns a response object, which contains a unique ID.
In the response, the pool provisioning status is ACTIVE
, PENDING_CREATE
, or ERROR
.
If the status is PENDING_CREATE
, issue GET /v2/lbaas/pools/{pool_id}
to view the progress of the provisioning operation. When the pool status changes to ACTIVE
, the pool is successfully provisioned and is ready for further configuration.
At a minimum, you must specify these pool attributes:
Some attributes receive default values if you omit them from the request:
If the API cannot fulfill the request due to insufficient data or data that is not valid, the service returns the HTTP Bad Request (400)
response code with information about the failure in the response body. Validation errors require that you correct the error and submit the request again.
Specifying a project_id is deprecated. The pool will inherit the project_id of the parent load balancer.
You can configure all documented features of the pool at creation time by specifying the additional elements or attributes in the request.
To create a pool, the parent load balancer must have an ACTIVE
provisioning status.
SOURCE_IP_PORT
algorithm is available from version 2.13.
Usage: osc load-balancer pool create [OPTIONS] --lb-algorithm <LB_ALGORITHM> --protocol <PROTOCOL>
Options:
-
--admin-state-up <ADMIN_STATE_UP>
— The administrative state of the resource, which is up (true
) or down (false
). Default istrue
Possible values:
true
,false
-
--alpn-protocols <ALPN_PROTOCOLS>
— A list of ALPN protocols. Available protocols: http/1.0, http/1.1, h2New in version 2.24
Parameter is an array, may be provided multiple times.
-
--ca-tls-container-ref <CA_TLS_CONTAINER_REF>
— The reference of the key manager service secret containing a PEM format CA certificate bundle fortls_enabled
pools.New in version 2.8
-
--crl-container-ref <CRL_CONTAINER_REF>
— The reference of the key manager service secret containing a PEM format CA revocation list file fortls_enabled
pools -
--description <DESCRIPTION>
— A human-readable description for the resource -
--healthmonitor <JSON>
— Defines mandatory and optional attributes of a POST request -
--lb-algorithm <LB_ALGORITHM>
— The load balancing algorithm for the pool. One ofLEAST_CONNECTIONS
,ROUND_ROBIN
,SOURCE_IP
, orSOURCE_IP_PORT
Possible values:
least-connections
,round-robin
,source-ip
,source-ip-port
-
--listener-id <LISTENER_ID>
— The ID of the listener for the pool. Eitherlistener_id
orloadbalancer_id
must be specified. The listener has some restrictions, See Protocol Combinations (Listener/Pool) -
--loadbalancer-id <LOADBALANCER_ID>
— The ID of the load balancer for the pool. Eitherlistener_id
orloadbalancer_id
must be specified -
--members <JSON>
— Parameter is an array, may be provided multiple times -
--name <NAME>
— Human-readable name of the resource -
--project-id <PROJECT_ID>
— The ID of the project owning this resource. (deprecated) -
--protocol <PROTOCOL>
— The protocol for the resource. One ofHTTP
,HTTPS
,PROXY
,PROXYV2
,SCTP
,TCP
, orUDP
Possible values:
http
,https
,proxy
,proxyv2
,sctp
,tcp
,udp
-
--cookie-name <COOKIE_NAME>
-
--persistence-granularity <PERSISTENCE_GRANULARITY>
-
--persistence-timeout <PERSISTENCE_TIMEOUT>
-
--type <TYPE>
Possible values:
app-cookie
,http-cookie
,source-ip
-
--tags <TAGS>
— Parameter is an array, may be provided multiple times -
--tenant-id <TENANT_ID>
-
--tls-ciphers <TLS_CIPHERS>
— List of ciphers in OpenSSL format (colon-separated). See https://www.openssl.org/docs/man1.1.1/man1/ciphers.htmlNew in version 2.15
-
--tls-container-ref <TLS_CONTAINER_REF>
— The reference to the key manager service secret containing a PKCS12 format certificate/key bundle fortls_enabled
pools for TLS client authentication to the member servers.New in version 2.8
-
--tls-enabled <TLS_ENABLED>
— Whentrue
connections to backend member servers will use TLS encryption. Default isfalse
.New in version 2.8
Possible values:
true
,false
-
--tls-versions <TLS_VERSIONS>
— A list of TLS protocol versions. Available versions: SSLv3, TLSv1, TLSv1.1, TLSv1.2, TLSv1.3New in version 2.17
Parameter is an array, may be provided multiple times.
osc load-balancer pool delete
Removes a pool and its associated configuration from the load balancer.
The API immediately purges any and all configuration data, depending on the configuration settings. You cannot recover it.
Usage: osc load-balancer pool delete <ID>
Arguments:
<ID>
— pool_id parameter for /v2/lbaas/pools/{pool_id} API
osc load-balancer pool list
Lists all pools for the project.
Use the fields
query parameter to control which fields are returned in the response body. Additionally, you can filter results by using query string parameters. For information, see Filtering and column selection.
Administrative users can specify a project ID that is different than their own to list pools for other projects.
The list might be empty.
Usage: osc load-balancer pool list [OPTIONS]
Options:
-
--admin-state-up <ADMIN_STATE_UP>
— The administrative state of the resourcePossible values:
true
,false
-
--alpn-protocols <ALPN_PROTOCOLS>
— A list of ALPN protocols. Available protocols: http/1.0, http/1.1, h2 -
--created-at <CREATED_AT>
— The UTC date and timestamp when the resource was created -
--description <DESCRIPTION>
— A human-readable description for the resource -
--id <ID>
— The ID of the resource -
--limit <LIMIT>
— Page size -
--loadbalancer-id <LOADBALANCER_ID>
— The ID of the load balancer for the pool -
--marker <MARKER>
— ID of the last item in the previous list -
--name <NAME>
— Human-readable name of the resource -
--not-tags <NOT_TAGS>
— Return the list of entities that do not have one or more of the given tags -
--not-tags-any <NOT_TAGS_ANY>
— Return the list of entities that do not have at least one of the given tags -
--operating-status <OPERATING_STATUS>
— The operating status of the resourcePossible values:
DEGRADED
,DRAINING
,ERROR
,NO_MONITOR
,OFFLINE
,ONLINE
-
--page-reverse <PAGE_REVERSE>
— The page directionPossible values:
true
,false
-
--project-name <PROJECT_NAME>
— Project Name -
--project-id <PROJECT_ID>
— Project ID -
--current-project
— Current project -
--provisioning-status <PROVISIONING_STATUS>
— The provisioning status of the resourcePossible values:
ACTIVE
,DELETED
,ERROR
,PENDING_CREATE
,PENDING_DELETE
,PENDING_UPDATE
-
--tags <TAGS>
— Return the list of entities that have this tag or tags -
--tags-any <TAGS_ANY>
— Return the list of entities that have one or more of the given tags -
--tls-ciphers <TLS_CIPHERS>
— List of ciphers in OpenSSL format -
--tls-enabled <TLS_ENABLED>
Possible values:
true
,false
-
--tls-versions <TLS_VERSIONS>
— A list of TLS protocol versions -
--updated-at <UPDATED_AT>
— The UTC date and timestamp when the resource was last updated -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc load-balancer pool member
Pool Member commands
Usage: osc load-balancer pool member <COMMAND>
Available subcommands:
osc load-balancer pool member create
— Create Memberosc load-balancer pool member delete
— Remove a Memberosc load-balancer pool member list
— List Membersosc load-balancer pool member set
— Update a Memberosc load-balancer pool member show
— Show Member details
osc load-balancer pool member create
This operation provisions a member and adds it to a pool by using the configuration that you define in the request object. After the API validates the request and starts the provisioning process, it returns a response object, which contains a unique ID.
In the response, the member provisioning status is ACTIVE
, PENDING_CREATE
, or ERROR
.
If the status is PENDING_CREATE
, issue GET /v2/lbaas/pools/{pool_id}/members/{member_id}
to view the progress of the provisioning operation. When the member status changes to ACTIVE
, the member is successfully provisioned and is ready for further configuration.
If the API cannot fulfill the request due to insufficient data or data that is not valid, the service returns the HTTP Bad Request (400)
response code with information about the failure in the response body. Validation errors require that you correct the error and submit the request again.
At a minimum, you must specify these member attributes:
Some attributes receive default values if you omit them from the request:
If you omit the subnet_id
parameter, the vip_subnet_id
for the parent load balancer will be used for the member subnet UUID.
The member address
does not necessarily need to be a member of the subnet_id
subnet. Members can be routable from the subnet specified either via the default route or by using host_routes
defined on the subnet.
Administrative users can specify a project ID that is different than their own to create members for other projects.
monitor_address
and/or monitor_port
can be used to have the health monitor, if one is configured for the pool, connect to an alternate IP address and port when executing a health check on the member.
To create a member, the load balancer must have an ACTIVE
provisioning status.
Usage: osc load-balancer pool member create [OPTIONS] --address <ADDRESS> --protocol-port <PROTOCOL_PORT> <POOL_ID>
Arguments:
<POOL_ID>
— pool_id parameter for /v2/lbaas/pools/{pool_id}/members/{member_id} API
Options:
-
--address <ADDRESS>
— The IP address of the resource -
--admin-state-up <ADMIN_STATE_UP>
— The administrative state of the resource, which is up (true
) or down (false
). Default istrue
Possible values:
true
,false
-
--backup <BACKUP>
— Is the member a backup? Backup members only receive traffic when all non-backup members are down.New in version 2.1
Possible values:
true
,false
-
--monitor-address <MONITOR_ADDRESS>
— An alternate IP address used for health monitoring a backend member. Default isnull
which monitors the memberaddress
-
--monitor-port <MONITOR_PORT>
— An alternate protocol port used for health monitoring a backend member. Default isnull
which monitors the memberprotocol_port
-
--name <NAME>
— Human-readable name of the resource -
--project-id <PROJECT_ID>
— The ID of the project owning this resource. (deprecated) -
--protocol-port <PROTOCOL_PORT>
— The protocol port number for the resource -
--request-sriov <REQUEST_SRIOV>
— Request that an SR-IOV VF be used for the member network port. Defaults tofalse
.New in version 2.29
Possible values:
true
,false
-
--subnet-id <SUBNET_ID>
— The subnet ID the member service is accessible from -
--tags <TAGS>
— A list of simple strings assigned to the resource.New in version 2.5
Parameter is an array, may be provided multiple times.
-
--tenant-id <TENANT_ID>
-
--weight <WEIGHT>
— The weight of a member determines the portion of requests or connections it services compared to the other members of the pool. For example, a member with a weight of 10 receives five times as many requests as a member with a weight of 2. A value of 0 means the member does not receive new connections but continues to service existing connections. A valid value is from0
to256
. Default is1
osc load-balancer pool member delete
Removes a member and its associated configuration from the pool.
The API immediately purges any and all configuration data, depending on the configuration settings. You cannot recover it.
Usage: osc load-balancer pool member delete <POOL_ID> <ID>
Arguments:
<POOL_ID>
— pool_id parameter for /v2/lbaas/pools/{pool_id}/members/{member_id} API<ID>
— member_id parameter for /v2/lbaas/pools/{pool_id}/members/{member_id} API
osc load-balancer pool member list
Lists all members for the project.
Use the fields
query parameter to control which fields are returned in the response body. Additionally, you can filter results by using query string parameters. For information, see Filtering and column selection.
Administrative users can specify a project ID that is different than their own to list members for other projects.
The list might be empty.
Usage: osc load-balancer pool member list [OPTIONS] <POOL_ID>
Arguments:
<POOL_ID>
— pool_id parameter for /v2/lbaas/pools/{pool_id}/members/{member_id} API
Options:
-
--address <ADDRESS>
— The IP address of the backend member server -
--admin-state-up <ADMIN_STATE_UP>
— The administrative state of the resourcePossible values:
true
,false
-
--backup <BACKUP>
— Is the member a backup?Possible values:
true
,false
-
--created-at <CREATED_AT>
— The UTC date and timestamp when the resource was created -
--description <DESCRIPTION>
— A human-readable description for the resource -
--id <ID>
— The ID of the resource -
--limit <LIMIT>
— Page size -
--marker <MARKER>
— ID of the last item in the previous list -
--monitor-address <MONITOR_ADDRESS>
— An alternate IP address used for health monitoring a backend member -
--monitor-port <MONITOR_PORT>
— An alternate protocol port used for health monitoring a backend member -
--name <NAME>
— Human-readable name of the resource -
--not-tags <NOT_TAGS>
— Return the list of entities that do not have one or more of the given tags -
--not-tags-any <NOT_TAGS_ANY>
— Return the list of entities that do not have at least one of the given tags -
--operating-status <OPERATING_STATUS>
— The operating status of the resourcePossible values:
DEGRADED
,DRAINING
,ERROR
,NO_MONITOR
,OFFLINE
,ONLINE
-
--page-reverse <PAGE_REVERSE>
— The page directionPossible values:
true
,false
-
--project-name <PROJECT_NAME>
— Project Name -
--project-id <PROJECT_ID>
— Project ID -
--current-project
— Current project -
--protocol-port <PROTOCOL_PORT>
— The protocol port number the backend member server is listening on -
--provisioning-status <PROVISIONING_STATUS>
— The provisioning status of the resourcePossible values:
ACTIVE
,DELETED
,ERROR
,PENDING_CREATE
,PENDING_DELETE
,PENDING_UPDATE
-
--subnet-id <SUBNET_ID>
— The subnet ID the member service is accessible from -
--tags <TAGS>
— Return the list of entities that have this tag or tags -
--tags-any <TAGS_ANY>
— Return the list of entities that have one or more of the given tags -
--updated-at <UPDATED_AT>
— The UTC date and timestamp when the resource was last updated -
--weight <WEIGHT>
— The weight of a member determines the portion of requests or connections it services compared to the other members of the pool -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc load-balancer pool member set
Update an existing member.
If the request is valid, the service returns the Accepted (202)
response code. To confirm the update, check that the member provisioning status is ACTIVE
. If the status is PENDING_UPDATE
, use a GET operation to poll the member object for changes.
Setting the member weight to 0
means that the member will not receive new requests but will finish any existing connections. This “drains” the backend member of active connections.
This operation returns the updated member object with the ACTIVE
, PENDING_UPDATE
, or ERROR
provisioning status.
Usage: osc load-balancer pool member set [OPTIONS] <POOL_ID> <ID>
Arguments:
<POOL_ID>
— pool_id parameter for /v2/lbaas/pools/{pool_id}/members/{member_id} API<ID>
— member_id parameter for /v2/lbaas/pools/{pool_id}/members/{member_id} API
Options:
-
--admin-state-up <ADMIN_STATE_UP>
— The administrative state of the resource, which is up (true
) or down (false
). Default istrue
Possible values:
true
,false
-
--backup <BACKUP>
— Is the member a backup? Backup members only receive traffic when all non-backup members are down.New in version 2.1
Possible values:
true
,false
-
--monitor-address <MONITOR_ADDRESS>
— An alternate IP address used for health monitoring a backend member. Default isnull
which monitors the memberaddress
-
--monitor-port <MONITOR_PORT>
— An alternate protocol port used for health monitoring a backend member. Default isnull
which monitors the memberprotocol_port
-
--name <NAME>
— Human-readable name of the resource -
--tags <TAGS>
— A list of simple strings assigned to the resource.New in version 2.5
Parameter is an array, may be provided multiple times.
-
--weight <WEIGHT>
— The weight of a member determines the portion of requests or connections it services compared to the other members of the pool. For example, a member with a weight of 10 receives five times as many requests as a member with a weight of 2. A value of 0 means the member does not receive new connections but continues to service existing connections. A valid value is from0
to256
. Default is1
osc load-balancer pool member show
Shows the details of a pool member.
If you are not an administrative user and the parent load balancer does not belong to your project, the service returns the HTTP Forbidden (403)
response code.
This operation does not require a request body.
Usage: osc load-balancer pool member show <POOL_ID> <ID>
Arguments:
<POOL_ID>
— pool_id parameter for /v2/lbaas/pools/{pool_id}/members/{member_id} API<ID>
— member_id parameter for /v2/lbaas/pools/{pool_id}/members/{member_id} API
osc load-balancer pool set
Update an existing pool.
If the request is valid, the service returns the Accepted (202)
response code. To confirm the update, check that the pool provisioning status is ACTIVE
. If the status is PENDING_UPDATE
, use a GET operation to poll the pool object for changes.
This operation returns the updated pool object with the ACTIVE
, PENDING_UPDATE
, or ERROR
provisioning status.
Usage: osc load-balancer pool set [OPTIONS] <ID>
Arguments:
<ID>
— pool_id parameter for /v2/lbaas/pools/{pool_id} API
Options:
-
--admin-state-up <ADMIN_STATE_UP>
— The administrative state of the resource, which is up (true
) or down (false
). Default istrue
Possible values:
true
,false
-
--alpn-protocols <ALPN_PROTOCOLS>
— A list of ALPN protocols. Available protocols: http/1.0, http/1.1, h2New in version 2.24
Parameter is an array, may be provided multiple times.
-
--ca-tls-container-ref <CA_TLS_CONTAINER_REF>
— The reference of the key manager service secret containing a PEM format CA certificate bundle fortls_enabled
pools.New in version 2.8
-
--crl-container-ref <CRL_CONTAINER_REF>
— The reference of the key manager service secret containing a PEM format CA revocation list file fortls_enabled
pools -
--description <DESCRIPTION>
— A human-readable description for the resource -
--lb-algorithm <LB_ALGORITHM>
— The load balancing algorithm for the pool. One ofLEAST_CONNECTIONS
,ROUND_ROBIN
, orSOURCE_IP
Possible values:
least-connections
,round-robin
,source-ip
,source-ip-port
-
--name <NAME>
— Human-readable name of the resource -
--cookie-name <COOKIE_NAME>
-
--persistence-granularity <PERSISTENCE_GRANULARITY>
-
--persistence-timeout <PERSISTENCE_TIMEOUT>
-
--type <TYPE>
Possible values:
app-cookie
,http-cookie
,source-ip
-
--tags <TAGS>
— A list of simple strings assigned to the resource.New in version 2.5
Parameter is an array, may be provided multiple times.
-
--tls-ciphers <TLS_CIPHERS>
— List of ciphers in OpenSSL format (colon-separated). See https://www.openssl.org/docs/man1.1.1/man1/ciphers.htmlNew in version 2.15
-
--tls-container-ref <TLS_CONTAINER_REF>
— The reference to the key manager service secret containing a PKCS12 format certificate/key bundle fortls_enabled
pools for TLS client authentication to the member servers.New in version 2.8
-
--tls-enabled <TLS_ENABLED>
— Whentrue
connections to backend member servers will use TLS encryption. Default isfalse
.New in version 2.8
Possible values:
true
,false
-
--tls-versions <TLS_VERSIONS>
— A list of TLS protocol versions. Available versions: SSLv3, TLSv1, TLSv1.1, TLSv1.2, TLSv1.3New in version 2.17
Parameter is an array, may be provided multiple times.
osc load-balancer pool show
Shows the details of a pool.
If you are not an administrative user and the parent load balancer does not belong to your project, the service returns the HTTP Forbidden (403)
response code.
This operation does not require a request body.
Usage: osc load-balancer pool show <ID>
Arguments:
<ID>
— pool_id parameter for /v2/lbaas/pools/{pool_id} API
osc load-balancer provider
Provider (Octavia) commands
Usage: osc load-balancer provider <COMMAND>
Available subcommands:
osc load-balancer provider availability-zone-capability
— AvailabilityZoneCapability (Octavia) commandsosc load-balancer provider flavor-capability
— FlavorCapability (Octavia) commandsosc load-balancer provider list
— List Providers
osc load-balancer provider availability-zone-capability
AvailabilityZoneCapability (Octavia) commands
Usage: osc load-balancer provider availability-zone-capability <COMMAND>
Available subcommands:
osc load-balancer provider availability-zone-capability list
— Show Provider Availability Zone Capabilities
osc load-balancer provider availability-zone-capability list
Shows the provider driver availability zone capabilities. These are the features of the provider driver that can be configured in an Octavia availability zone. This API returns a list of dictionaries with the name and description of each availability zone capability of the provider.
The list might be empty and a provider driver may not implement this feature.
New in version 2.14
Usage: osc load-balancer provider availability-zone-capability list <PROVIDER>
Arguments:
<PROVIDER>
— provider parameter for /v2/lbaas/providers/{provider}/availability_zone_capabilities API
osc load-balancer provider flavor-capability
FlavorCapability (Octavia) commands
Usage: osc load-balancer provider flavor-capability <COMMAND>
Available subcommands:
osc load-balancer provider flavor-capability list
— Show Provider Flavor Capabilities
osc load-balancer provider flavor-capability list
Shows the provider driver flavor capabilities. These are the features of the provider driver that can be configured in an Octavia flavor. This API returns a list of dictionaries with the name and description of each flavor capability of the provider.
The list might be empty and a provider driver may not implement this feature.
New in version 2.6
Usage: osc load-balancer provider flavor-capability list <PROVIDER>
Arguments:
<PROVIDER>
— provider parameter for /v2/lbaas/providers/{provider}/flavor_capabilities API
osc load-balancer provider list
Lists all enabled provider drivers.
Use the fields
query parameter to control which fields are returned in the response body.
The list might be empty.
Usage: osc load-balancer provider list [OPTIONS]
Options:
-
--description <DESCRIPTION>
-
--limit <LIMIT>
— Page size -
--marker <MARKER>
— ID of the last item in the previous list -
--name <NAME>
-
--page-reverse <PAGE_REVERSE>
— The page directionPossible values:
true
,false
-
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc load-balancer quota
Quota commands
Usage: osc load-balancer quota <COMMAND>
Available subcommands:
osc load-balancer quota delete
— Reset a Quotaosc load-balancer quota list
— List Quotaosc load-balancer quota set
— Update a Quotaosc load-balancer quota show
— Show Project Quota
osc load-balancer quota delete
Reset a Quota
Usage: osc load-balancer quota delete <--project-name <PROJECT_NAME>|--project-id <PROJECT_ID>|--current-project>
Options:
--project-name <PROJECT_NAME>
— Project Name--project-id <PROJECT_ID>
— Project ID--current-project
— Current project
osc load-balancer quota list
Lists all quotas for the project.
Use the fields
query parameter to control which fields are returned in the response body. Additionally, you can filter results by using query string parameters. For information, see Filtering and column selection.
Administrative users can specify a project ID that is different than their own to list quotas for other projects.
If the quota is listed as null
the quota is using the deployment default quota settings.
A quota of -1
means the quota is unlimited.
The list might be empty.
Usage: osc load-balancer quota list
osc load-balancer quota set
Updates a quota for a project.
If the request is valid, the service returns the Accepted (202)
response code.
This operation returns the updated quota object.
If the quota is specified as null
the quota will use the deployment default quota settings.
Specifying a quota of -1
means the quota is unlimited.
Specifying a quota of 0
means the project cannot create any of the resource.
Usage: osc load-balancer quota set [OPTIONS] <--project-name <PROJECT_NAME>|--project-id <PROJECT_ID>|--current-project>
Options:
--project-name <PROJECT_NAME>
— Project Name--project-id <PROJECT_ID>
— Project ID--current-project
— Current project--health-monitor <HEALTH_MONITOR>
--healthmonitor <HEALTHMONITOR>
— The configured health monitor quota limit. A setting ofnull
means it is using the deployment default quota. A setting of-1
means unlimited--l7policy <L7POLICY>
— The configured l7policy quota limit. A setting ofnull
means it is using the deployment default quota. A setting of-1
means unlimited--l7rule <L7RULE>
— The configured l7rule quota limit. A setting ofnull
means it is using the deployment default quota. A setting of-1
means unlimited--listener <LISTENER>
— The configured listener quota limit. A setting ofnull
means it is using the deployment default quota. A setting of-1
means unlimited--load-balancer <LOAD_BALANCER>
--loadbalancer <LOADBALANCER>
— The configured load balancer quota limit. A setting ofnull
means it is using the deployment default quota. A setting of-1
means unlimited--member <MEMBER>
— The configured member quota limit. A setting ofnull
means it is using the deployment default quota. A setting of-1
means unlimited--pool <POOL>
— The configured pool quota limit. A setting ofnull
means it is using the deployment default quota. A setting of-1
means unlimited
osc load-balancer quota show
Show the quota for the project.
Use the fields
query parameter to control which fields are returned in the response body. Additionally, you can filter results by using query string parameters. For information, see Filtering and column selection.
Administrative users can specify a project ID that is different than their own to show quota for other projects.
A quota of -1
means the quota is unlimited.
Usage: osc load-balancer quota show <--project-name <PROJECT_NAME>|--project-id <PROJECT_ID>|--current-project>
Options:
--project-name <PROJECT_NAME>
— Project Name--project-id <PROJECT_ID>
— Project ID--current-project
— Current project
osc load-balancer version
Version (Octavia) commands
Usage: osc load-balancer version <COMMAND>
Available subcommands:
osc load-balancer version get
— Command without description in OpenAPI
osc load-balancer version get
Command without description in OpenAPI
Usage: osc load-balancer version get
osc network
Network (Neutron) commands
Usage: osc network <COMMAND>
Available subcommands:
osc network address-group
— Address groupsosc network address-scope
— Address scopesosc network agent
— Address scopesosc network auto-allocated-topology
— Auto Allocated Topologiesosc network availability-zone
— Availability Zones commandsosc network default-security-group-rule
— Security group default rules (security-group-default-rules)osc network extension
— Extensions commandsosc network flavor
— Networking Flavors Frameworkosc network floating-ip
— Floating IP commandsosc network floating-ip-pool
— Floating IP poolsosc network local-ip
— Local IPs (local_ips)osc network log
— Loggingosc network metering
— Metering labels and rules (metering-labels, metering-label-rules)osc network ndp-proxy
— Router NDP proxy (ndp_proxies)osc network network
— Network commandsosc network network-ip-availability
— Network IP availability and usage statsosc network network-segment-range
— Network Segment Rangesosc network port
— Port commandsosc network qos
— Quality of Serviceosc network quota
— Quotas extension (quotas)osc network rbac-policy
— RbacPolicy commandsosc network router
— Router commandsosc network security-group
— SecurityGroup commandsosc network security-group-rule
— SecurityGroupRule commandsosc network segment
— Segmentsosc network subnet
— Subnet commandsosc network subnetpool
— SubnetPool commandsosc network vpn
— VPNaaS 2.0 (vpn, vpnservices, ikepolicies, ipsecpolicies, endpoint-groups, ipsec-site-connections)
osc network address-group
Address groups
Lists, creates, shows details for, updates, and deletes address groups.
Usage: osc network address-group <COMMAND>
Available subcommands:
osc network address-group add-address
— Command without description in OpenAPIosc network address-group create
— Create address grouposc network address-group delete
— Delete an address grouposc network address-group list
— List address groupsosc network address-group remove-address
— Command without description in OpenAPIosc network address-group set
— Update an address grouposc network address-group show
— Show address group
osc network address-group add-address
Command without description in OpenAPI
Usage: osc network address-group add-address [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.0/address-groups/{id} API
Options:
-
--addresses <ADDRESSES>
— A list of IP addresses.Parameter is an array, may be provided multiple times.
osc network address-group create
Creates an address group.
Normal response codes: 201
Error response codes: 400, 401, 403, 404
Usage: osc network address-group create [OPTIONS]
Options:
-
--addresses <ADDRESSES>
— A list of IP addresses.Parameter is an array, may be provided multiple times.
-
--description <DESCRIPTION>
— A human-readable description for the resource -
--name <NAME>
— Human-readable name of the resource. Default is an empty string -
--project-id <PROJECT_ID>
osc network address-group delete
Deletes an address group.
Normal response codes: 204
Error response codes: 401, 404, 412
Usage: osc network address-group delete <ID>
Arguments:
<ID>
— id parameter for /v2.0/address-groups/{id} API
osc network address-group list
Lists address groups that the project has access to.
Default policy settings return only the address groups owned by the project of the user submitting the request, unless the user has administrative role.
Standard query parameters are supported on the URI. For more information, see Filtering and Column Selection.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Pagination query parameters are supported if Neutron configuration supports it by overriding allow_pagination=false
. For more information, see Pagination.
Sorting query parameters are supported if Neutron configuration supports it with allow_sorting=true
. For more information, see Sorting.
Normal response codes: 200
Error response codes: 401
Usage: osc network address-group list [OPTIONS]
Options:
-
--description <DESCRIPTION>
— description query parameter for /v2.0/address-groups API -
--id <ID>
— id query parameter for /v2.0/address-groups API -
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--name <NAME>
— name query parameter for /v2.0/address-groups API -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--project-id <PROJECT_ID>
— project_id query parameter for /v2.0/address-groups API -
--revision-number <REVISION_NUMBER>
— revision_number query parameter for /v2.0/address-groups API -
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network address-group remove-address
Command without description in OpenAPI
Usage: osc network address-group remove-address [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.0/address-groups/{id} API
Options:
-
--addresses <ADDRESSES>
— A list of IP addresses.Parameter is an array, may be provided multiple times.
osc network address-group set
Updates an address group.
Normal response codes: 200
Error response codes: 400, 401, 403, 404, 412
Usage: osc network address-group set [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.0/address-groups/{id} API
Options:
--description <DESCRIPTION>
— A human-readable description for the resource--name <NAME>
— Human-readable name of the resource. Default is an empty string
osc network address-group show
Shows information for an address group.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Normal response codes: 200
Error response codes: 401, 404
Usage: osc network address-group show <ID>
Arguments:
<ID>
— id parameter for /v2.0/address-groups/{id} API
osc network address-scope
Address scopes
Lists, creates, shows details for, updates, and deletes address scopes.
Usage: osc network address-scope <COMMAND>
Available subcommands:
osc network address-scope create
— Create address scopeosc network address-scope delete
— Delete an address scopeosc network address-scope list
— List address scopesosc network address-scope set
— Update an address scopeosc network address-scope show
— Show address scope
osc network address-scope create
Creates an address scope.
Normal response codes: 201
Error response codes: 400, 401, 403, 404
Usage: osc network address-scope create [OPTIONS]
Options:
-
--ip-version <IP_VERSION>
— The IP protocol version. Valid value is4
or6
-
--name <NAME>
— Human-readable name of the resource. Default is an empty string -
--shared <SHARED>
— Indicates whether this resource is shared across all projects. By default, only administrative users can change this valuePossible values:
true
,false
-
--tenant-id <TENANT_ID>
— The ID of the project that owns the resource. Only administrative and users with advsvc role can specify a project ID other than their own. You cannot change this value through authorization policies
osc network address-scope delete
Deletes an address scope.
Normal response codes: 204
Error response codes: 401, 404, 412
Usage: osc network address-scope delete <ID>
Arguments:
<ID>
— id parameter for /v2.0/address-scopes/{id} API
osc network address-scope list
Lists address scopes that the project has access to.
Default policy settings return only the address scopes owned by the project of the user submitting the request, unless the user has administrative role.
Standard query parameters are supported on the URI. For more information, see Filtering and Column Selection.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Pagination query parameters are supported if Neutron configuration supports it by overriding allow_pagination=false
. For more information, see Pagination.
Sorting query parameters are supported if Neutron configuration supports it with allow_sorting=true
. For more information, see Sorting.
Normal response codes: 200
Error response codes: 401
Usage: osc network address-scope list [OPTIONS]
Options:
-
--id <ID>
— id query parameter for /v2.0/address-scopes API -
--ip-version <IP_VERSION>
— ip_version query parameter for /v2.0/address-scopes API -
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--name <NAME>
— name query parameter for /v2.0/address-scopes API -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--shared <SHARED>
— shared query parameter for /v2.0/address-scopes APIPossible values:
true
,false
-
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--tenant-id <TENANT_ID>
— tenant_id query parameter for /v2.0/address-scopes API -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network address-scope set
Updates an address scope.
Normal response codes: 200
Error response codes: 400, 401, 403, 404, 412
Usage: osc network address-scope set [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.0/address-scopes/{id} API
Options:
-
--name <NAME>
— Human-readable name of the resource. Default is an empty string -
--shared <SHARED>
— Indicates whether this resource is shared across all projects. By default, only administrative users can change this valuePossible values:
true
,false
osc network address-scope show
Shows information for an address scope.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Normal response codes: 200
Error response codes: 401, 404
Usage: osc network address-scope show <ID>
Arguments:
<ID>
— id parameter for /v2.0/address-scopes/{id} API
osc network agent
Address scopes
Lists, creates, shows details for, updates, and deletes address scopes.
Usage: osc network agent <COMMAND>
Available subcommands:
osc network agent create
— Command without description in OpenAPIosc network agent delete
— Delete agentosc network agent dhcp-network
— DHCP agent schedulerosc network agent l3-router
— L3 agent schedulerosc network agent list
— List all agentsosc network agent set
— Update agentosc network agent show
— Show agent details
osc network agent create
Command without description in OpenAPI
Usage: osc network agent create [OPTIONS]
Options:
--agent <key=value>
osc network agent delete
Agents that won’t be used anymore can be removed. Before deleting agents via API, the agent should be stopped/disabled.
Normal response codes: 204
Error response codes: 401, 404, 409
Usage: osc network agent delete <ID>
Arguments:
<ID>
— id parameter for /v2.0/agents/{id} API
osc network agent dhcp-network
DHCP agent scheduler
The DHCP agent scheduler extension (dhcp_agent_scheduler) enables administrators to assign DHCP servers for Neutron networks to given Neutron DHCP agents, and retrieve mappings between Neutron networks and DHCP agents.
Usage: osc network agent dhcp-network <COMMAND>
Available subcommands:
osc network agent dhcp-network create
— Schedule a network to a DHCP agentosc network agent dhcp-network delete
— Remove network from a DHCP agentosc network agent dhcp-network list
— List networks hosted by a DHCP agentosc network agent dhcp-network set
— Command without description in OpenAPIosc network agent dhcp-network show
— Command without description in OpenAPI
osc network agent dhcp-network create
Add a network to a DHCP agent
Normal response codes: 201
Error response codes: 400, 403, 409, 404
Usage: osc network agent dhcp-network create [OPTIONS] <AGENT_ID>
Arguments:
<AGENT_ID>
— agent_id parameter for /v2.0/agents/{agent_id}/dhcp-networks/{id} API
Options:
--property <key=value>
osc network agent dhcp-network delete
Removes a network from a dhcp agent.
Normal response codes: 204
Error response codes: 401, 403, 404, 409
Usage: osc network agent dhcp-network delete <AGENT_ID> <ID>
Arguments:
<AGENT_ID>
— agent_id parameter for /v2.0/agents/{agent_id}/dhcp-networks/{id} API<ID>
— id parameter for /v2.0/agents/{agent_id}/dhcp-networks/{id} API
osc network agent dhcp-network list
Lists networks that a DHCP agent hosts.
Standard query parameters are supported on the URI. For more information, see Filtering and Column Selection.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Pagination query parameters are supported if Neutron configuration supports it by overriding allow_pagination=false
. For more information, see Pagination.
Sorting query parameters are supported if Neutron configuration supports it with allow_sorting=true
. For more information, see Sorting.
Normal response codes: 200
Error response codes: 401, 403
Usage: osc network agent dhcp-network list [OPTIONS] <AGENT_ID>
Arguments:
<AGENT_ID>
— agent_id parameter for /v2.0/agents/{agent_id}/dhcp-networks/{id} API
Options:
-
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network agent dhcp-network set
Command without description in OpenAPI
Usage: osc network agent dhcp-network set [OPTIONS] <AGENT_ID> <ID>
Arguments:
<AGENT_ID>
— agent_id parameter for /v2.0/agents/{agent_id}/dhcp-networks/{id} API<ID>
— id parameter for /v2.0/agents/{agent_id}/dhcp-networks/{id} API
Options:
--property <key=value>
osc network agent dhcp-network show
Command without description in OpenAPI
Usage: osc network agent dhcp-network show <AGENT_ID> <ID>
Arguments:
<AGENT_ID>
— agent_id parameter for /v2.0/agents/{agent_id}/dhcp-networks/{id} API<ID>
— id parameter for /v2.0/agents/{agent_id}/dhcp-networks/{id} API
osc network agent l3-router
L3 agent scheduler
The L3 agent scheduler extension (l3_agent_scheduler) allows administrators to assign Neutron routers to Neutron L3 agents, and retrieve mappings between Neutron routers and L3 agents.
Usage: osc network agent l3-router <COMMAND>
Available subcommands:
osc network agent l3-router create
— Schedule router to an l3 agentosc network agent l3-router delete
— Remove l3 router from an l3 agentosc network agent l3-router list
— List routers hosted by an L3 agentosc network agent l3-router set
— Command without description in OpenAPIosc network agent l3-router show
— Command without description in OpenAPI
osc network agent l3-router create
Add a router to an l3 agent.
Normal response codes: 201
Error response codes: 400, 401, 404
Usage: osc network agent l3-router create --router-id <ROUTER_ID> <AGENT_ID>
Arguments:
<AGENT_ID>
— agent_id parameter for /v2.0/agents/{agent_id}/l3-routers/{id} API
Options:
--router-id <ROUTER_ID>
— The ID of the router
osc network agent l3-router delete
Removes a router from an l3 agent.
Normal response codes: 204
Error response codes: 401, 404
Usage: osc network agent l3-router delete <AGENT_ID> <ID>
Arguments:
<AGENT_ID>
— agent_id parameter for /v2.0/agents/{agent_id}/l3-routers/{id} API<ID>
— id parameter for /v2.0/agents/{agent_id}/l3-routers/{id} API
osc network agent l3-router list
Lists routers that an l3 agent hosts.
Standard query parameters are supported on the URI. For more information, see Filtering and Column Selection.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Pagination query parameters are supported if Neutron configuration supports it by overriding allow_pagination=false
. For more information, see Pagination.
Sorting query parameters are supported if Neutron configuration supports it with allow_sorting=true
. For more information, see Sorting.
Normal response codes: 200
Error response codes: 401, 404
Usage: osc network agent l3-router list [OPTIONS] <AGENT_ID>
Arguments:
<AGENT_ID>
— agent_id parameter for /v2.0/agents/{agent_id}/l3-routers/{id} API
Options:
-
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network agent l3-router set
Command without description in OpenAPI
Usage: osc network agent l3-router set <AGENT_ID> <ID>
Arguments:
<AGENT_ID>
— agent_id parameter for /v2.0/agents/{agent_id}/l3-routers/{id} API<ID>
— id parameter for /v2.0/agents/{agent_id}/l3-routers/{id} API
osc network agent l3-router show
Command without description in OpenAPI
Usage: osc network agent l3-router show <AGENT_ID> <ID>
Arguments:
<AGENT_ID>
— agent_id parameter for /v2.0/agents/{agent_id}/l3-routers/{id} API<ID>
— id parameter for /v2.0/agents/{agent_id}/l3-routers/{id} API
osc network agent list
Lists all agents.
Standard query parameters are supported on the URI. For more information, see Filtering and Column Selection.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Pagination query parameters are supported if Neutron configuration supports it by overriding allow_pagination=false
. For more information, see Pagination.
Sorting query parameters are supported if Neutron configuration supports it with allow_sorting=true
. For more information, see Sorting.
Normal response codes: 200
Error response codes: 401
Usage: osc network agent list [OPTIONS]
Options:
-
--admin-state-up <ADMIN_STATE_UP>
— admin_state_up query parameter for /v2.0/agents APIPossible values:
true
,false
-
--agent-type <AGENT_TYPE>
— agent_type query parameter for /v2.0/agents API -
--alive <ALIVE>
— alive query parameter for /v2.0/agents API -
--availability-zone <AVAILABILITY_ZONE>
— availability_zone query parameter for /v2.0/agents API -
--binary <BINARY>
— binary query parameter for /v2.0/agents API -
--description <DESCRIPTION>
— description query parameter for /v2.0/agents API -
--host <HOST>
— host query parameter for /v2.0/agents API -
--id <ID>
— id query parameter for /v2.0/agents API -
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--topic <TOPIC>
— topic query parameter for /v2.0/agents API -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network agent set
Updates an agent.
Normal response codes: 200
Error response codes: 400, 401, 403, 404
Usage: osc network agent set [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.0/agents/{id} API
Options:
-
--admin-state-up <ADMIN_STATE_UP>
— The administrative state of the resource, which is up (true
) or down (false
). Default istrue
Possible values:
true
,false
-
--description <DESCRIPTION>
— A human-readable description for the resource. Default is an empty string
osc network agent show
Shows details for an agent.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Normal response codes: 200
Error response codes: 401, 404
Usage: osc network agent show <ID>
Arguments:
<ID>
— id parameter for /v2.0/agents/{id} API
osc network auto-allocated-topology
Auto Allocated Topologies
Show details and delete the auto allocated topology for a given project. This API is only available when the auto-allocated-topology extension is enabled.
Usage: osc network auto-allocated-topology <COMMAND>
Available subcommands:
osc network auto-allocated-topology create
— Command without description in OpenAPIosc network auto-allocated-topology delete
— Delete the auto allocated topologyosc network auto-allocated-topology list
— Command without description in OpenAPIosc network auto-allocated-topology set
— Command without description in OpenAPIosc network auto-allocated-topology show
— Show auto allocated topology details
osc network auto-allocated-topology create
Command without description in OpenAPI
Usage: osc network auto-allocated-topology create [OPTIONS]
Options:
--auto-allocated-topology <key=value>
osc network auto-allocated-topology delete
Deletes the auto allocated topology.
Normal response codes: 204
Error response codes: 401, 403, 404
Usage: osc network auto-allocated-topology delete <ID>
Arguments:
<ID>
— id parameter for /v2.0/auto-allocated-topology/{id} API
osc network auto-allocated-topology list
Command without description in OpenAPI
Usage: osc network auto-allocated-topology list [OPTIONS]
Options:
-
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network auto-allocated-topology set
Command without description in OpenAPI
Usage: osc network auto-allocated-topology set [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.0/auto-allocated-topology/{id} API
Options:
--auto-allocated-topology <key=value>
osc network auto-allocated-topology show
Shows details for an auto allocated topology.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Normal response codes: 200
Error response codes: 401, 404
Usage: osc network auto-allocated-topology show <ID>
Arguments:
<ID>
— id parameter for /v2.0/auto-allocated-topology/{id} API
osc network availability-zone
Availability Zones commands
Usage: osc network availability-zone <COMMAND>
Available subcommands:
osc network availability-zone list
— List all availability zones
osc network availability-zone list
Lists all availability zones.
Standard query parameters are supported on the URI. For more information, see Filtering and Column Selection.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Pagination query parameters are supported if Neutron configuration supports it by overriding allow_pagination=false
. For more information, see Pagination.
Sorting query parameters are supported if Neutron configuration supports it with allow_sorting=true
. For more information, see Sorting.
Normal response codes: 200
Error response codes: 401
Usage: osc network availability-zone list [OPTIONS]
Options:
-
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--name <NAME>
— name query parameter for /v2.0/availability_zones API -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--resource <RESOURCE>
— resource query parameter for /v2.0/availability_zones API -
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--state <STATE>
— state query parameter for /v2.0/availability_zones API -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network default-security-group-rule
Security group default rules (security-group-default-rules)
Lists, creates, shows information for, and deletes security group default rules.
Usage: osc network default-security-group-rule <COMMAND>
Available subcommands:
osc network default-security-group-rule create
— Create security group default ruleosc network default-security-group-rule delete
— Delete security group default ruleosc network default-security-group-rule list
— List security group default rulesosc network default-security-group-rule set
— Command without description in OpenAPIosc network default-security-group-rule show
— Show security group default rule
osc network default-security-group-rule create
Creates an Openstack Networking security group rule template.
Normal response codes: 201
Error response codes: 400, 401, 404, 409
Usage: osc network default-security-group-rule create [OPTIONS]
Options:
-
--description <DESCRIPTION>
— A human-readable description for the resource. Default is an empty string -
--direction <DIRECTION>
— Ingress or egress, which is the direction in which the security group rule is appliedPossible values:
egress
,ingress
-
--ethertype <ETHERTYPE>
— Must be IPv4 or IPv6, and addresses represented in CIDR must match the ingress or egress rulesPossible values:
ipv4
,ipv6
-
--port-range-max <PORT_RANGE_MAX>
— The maximum port number in the range that is matched by the security group rule. If the protocol is TCP, UDP, DCCP, SCTP or UDP-Lite this value must be greater than or equal to theport_range_min
attribute value. If the protocol is ICMP, this value must be an ICMP code -
--port-range-min <PORT_RANGE_MIN>
— The minimum port number in the range that is matched by the security group rule. If the protocol is TCP, UDP, DCCP, SCTP or UDP-Lite this value must be less than or equal to theport_range_max
attribute value. If the protocol is ICMP, this value must be an ICMP type -
--protocol <PROTOCOL>
— The IP protocol can be represented by a string, an integer, ornull
. Valid string or integer values areany
or0
,ah
or51
,dccp
or33
,egp
or8
,esp
or50
,gre
or47
,icmp
or1
,icmpv6
or58
,igmp
or2
,ipip
or4
,ipv6-encap
or41
,ipv6-frag
or44
,ipv6-icmp
or58
,ipv6-nonxt
or59
,ipv6-opts
or60
,ipv6-route
or43
,ospf
or89
,pgm
or113
,rsvp
or46
,sctp
or132
,tcp
or6
,udp
or17
,udplite
or136
,vrrp
or112
. Additionally, any integer value between [0-255] is also valid. The stringany
(or integer0
) meansall
IP protocols. See the constants inneutron_lib.constants
for the most up-to-date list of supported strings -
--remote-address-group-id <REMOTE_ADDRESS_GROUP_ID>
-
--remote-group-id <REMOTE_GROUP_ID>
— The remote group UUID to associate with this security group rule. You can specify either theremote_group_id
orremote_ip_prefix
attribute in the request body -
--remote-ip-prefix <REMOTE_IP_PREFIX>
— The remote IP prefix that is matched by this security group rule -
--tenant-id <TENANT_ID>
-
--used-in-default-sg <USED_IN_DEFAULT_SG>
— Whether this security group rule template should be used in default security group created automatically for each new project. Default value isFalse
Possible values:
true
,false
-
--used-in-non-default-sg <USED_IN_NON_DEFAULT_SG>
— Whether this security group rule template should be used in custom security groups created by project user. Default value isTrue
Possible values:
true
,false
osc network default-security-group-rule delete
Deletes an OpenStack Networking security group rule template.
Normal response codes: 204
Error response codes: 401, 404, 412
Usage: osc network default-security-group-rule delete <ID>
Arguments:
<ID>
— id parameter for /v2.0/default-security-group-rules/{id} API
osc network default-security-group-rule list
Lists a summary of all OpenStack Networking security group rules that are used for every newly created Security Group.
The list provides the ID for each security group default rule.
Standard query parameters are supported on the URI. For more information, see Filtering and Column Selection.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Pagination query parameters are supported if Neutron configuration supports it by overriding allow_pagination=false
. For more information, see Pagination.
Sorting query parameters are supported if Neutron configuration supports it with allow_sorting=true
. For more information, see Sorting.
Normal response codes: 200
Error response codes: 401
Usage: osc network default-security-group-rule list [OPTIONS]
Options:
-
--description <DESCRIPTION>
— description query parameter for /v2.0/default-security-group-rules API -
--direction <DIRECTION>
— direction query parameter for /v2.0/default-security-group-rules APIPossible values:
egress
,ingress
-
--ethertype <ETHERTYPE>
— ethertype query parameter for /v2.0/default-security-group-rules APIPossible values:
IPv4
,IPv6
-
--id <ID>
— id query parameter for /v2.0/default-security-group-rules API -
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--port-range-max <PORT_RANGE_MAX>
— port_range_max query parameter for /v2.0/default-security-group-rules API -
--port-range-min <PORT_RANGE_MIN>
— port_range_min query parameter for /v2.0/default-security-group-rules API -
--protocol <PROTOCOL>
— protocol query parameter for /v2.0/default-security-group-rules API -
--remote-address-group-id <REMOTE_ADDRESS_GROUP_ID>
— remote_address_group_id query parameter for /v2.0/default-security-group-rules API -
--remote-group-id <REMOTE_GROUP_ID>
— Filter the security group rule list result by the ID of the remote group that associates with this security group rule. This field can contains uuid of the security group or special wordPARENT
which means that in the real rule created from this template, uuid of the owner Security Group will be put asremote_group_id
-
--remote-ip-prefix <REMOTE_IP_PREFIX>
— remote_ip_prefix query parameter for /v2.0/default-security-group-rules API -
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--used-in-default-sg <USED_IN_DEFAULT_SG>
— used_in_default_sg query parameter for /v2.0/default-security-group-rules APIPossible values:
true
,false
-
--used-in-non-default-sg <USED_IN_NON_DEFAULT_SG>
— used_in_non_default_sg query parameter for /v2.0/default-security-group-rules APIPossible values:
true
,false
-
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network default-security-group-rule set
Command without description in OpenAPI
Usage: osc network default-security-group-rule set [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.0/default-security-group-rules/{id} API
Options:
--default-security-group-rule <key=value>
osc network default-security-group-rule show
Shows detailed information for a security group default rule.
The response body contains the following information about the security group rule:
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Normal response codes: 200
Error response codes: 401, 404
Usage: osc network default-security-group-rule show <ID>
Arguments:
<ID>
— id parameter for /v2.0/default-security-group-rules/{id} API
osc network extension
Extensions commands
Usage: osc network extension <COMMAND>
Available subcommands:
osc network extension list
— List extensionsosc network extension show
— Show extension details
osc network extension list
Lists available extensions.
Lists available Networking API v2.0 extensions.
Standard query parameters are supported on the URI. For more information, see Filtering and Column Selection.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Pagination query parameters are supported if Neutron configuration supports it by overriding allow_pagination=false
. For more information, see Pagination.
Sorting query parameters are supported if Neutron configuration supports it with allow_sorting=true
. For more information, see Sorting.
Normal response codes: 200
Error response codes: 401
Usage: osc network extension list
osc network extension show
Shows details for an extension, by alias. The response shows the extension name and its alias. To show details for an extension, you specify the alias.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Normal response codes: 200
Error response codes: 401, 404
Usage: osc network extension show <ID>
Arguments:
<ID>
— id parameter for /v2.0/extensions/{id} API
osc network flavor
Networking Flavors Framework
Extension that allows user selection of operator-curated flavors during resource creation.
Users can check if flavor available by performing a GET on the /v2.0/extensions/flavors. If it is unavailable,there is an 404 error response (itemNotFound). Refer Show extension details for more details.
Usage: osc network flavor <COMMAND>
Available subcommands:
osc network flavor create
— Create flavorosc network flavor delete
— Delete flavorosc network flavor list
— List flavorsosc network flavor set
— Update flavorosc network flavor show
— Show flavor details
osc network flavor create
Creates a flavor.
This operation establishes a new flavor.
The service_type to which the flavor applies is a required parameter. The corresponding service plugin must have been activated as part of the configuration. Check Service providers for how to see currently loaded service types. Additionally the service plugin needs to support the use of flavors.
Creation currently limited to administrators. Other users will receive a Forbidden 403
response code with a response body NeutronError message expressing that creation is disallowed by policy.
Until one or more service profiles are associated with the flavor by the operator, attempts to use the flavor during resource creations will currently return a Not Found 404
with a response body that indicates no service profile could be found.
If the API cannot fulfill the request due to insufficient data or data that is not valid, the service returns the HTTP Bad Request (400)
response code with information about the failure in the response body. Validation errors require that you correct the error and submit the request again.
Normal response codes: 201
Error response codes: 400, 401, 403, 404
Usage: osc network flavor create [OPTIONS]
Options:
-
--description <DESCRIPTION>
— The human-readable description for the flavor -
--enabled <ENABLED>
— Indicates whether the flavor is enabled or not. Default is truePossible values:
true
,false
-
--name <NAME>
— Name of the flavor -
--service-profiles <SERVICE_PROFILES>
— Parameter is an array, may be provided multiple times -
--service-type <SERVICE_TYPE>
— Service type for the flavor. Example: FIREWALL
osc network flavor delete
Deletes a flavor.
Normal response codes: 204
Error response codes: 401, 403, 404
Usage: osc network flavor delete <ID>
Arguments:
<ID>
— id parameter for /v2.0/flavors/{id} API
osc network flavor list
Lists all flavors visible to the project.
The list can be empty.
Standard query parameters are supported on the URI. For more information, see Filtering and Column Selection.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Pagination query parameters are supported if Neutron configuration supports it by overriding allow_pagination=false
. For more information, see Pagination.
Sorting query parameters are supported if Neutron configuration supports it with allow_sorting=true
. For more information, see Sorting.
Normal response codes: 200
Error response codes: 401
Usage: osc network flavor list [OPTIONS]
Options:
-
--description <DESCRIPTION>
— description query parameter for /v2.0/flavors API -
--enabled <ENABLED>
— enabled query parameter for /v2.0/flavors APIPossible values:
true
,false
-
--id <ID>
— id query parameter for /v2.0/flavors API -
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--name <NAME>
— name query parameter for /v2.0/flavors API -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--service-type <SERVICE_TYPE>
— service_type query parameter for /v2.0/flavors API -
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network flavor set
Updates a flavor.
The service_type cannot be updated as there may be associated service profiles and consumers depending on the value.
Normal response codes: 200
Error response codes: 400, 401, 403, 404
Usage: osc network flavor set [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.0/flavors/{id} API
Options:
-
--description <DESCRIPTION>
— The human-readable description for the flavor -
--enabled <ENABLED>
— Indicates whether the flavor is enabled or not. Default is truePossible values:
true
,false
-
--name <NAME>
— Name of the flavor -
--service-profiles <SERVICE_PROFILES>
— Parameter is an array, may be provided multiple times
osc network flavor show
Shows details for a flavor.
This operation returns a flavor object by ID. If you are not an administrative user and the flavor object is not visible to your project account, the service returns the HTTP Forbidden (403)
response code.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Normal response codes: 200
Error response codes: 401, 403, 404
Usage: osc network flavor show <ID>
Arguments:
<ID>
— id parameter for /v2.0/flavors/{id} API
osc network floating-ip
Floating IP commands
Usage: osc network floating-ip <COMMAND>
Available subcommands:
osc network floating-ip create
— Create floating IPosc network floating-ip delete
— Delete floating IPosc network floating-ip list
— List floating IPsosc network floating-ip port-forwarding
— Floating IPs port forwardingosc network floating-ip set
— Update floating IPosc network floating-ip show
— Show floating IP detailsosc network floating-ip tag
— Lists tags, creates, replaces or deletes one or more tags for a resource, checks the existence of a tag for a resource
osc network floating-ip create
Creates a floating IP, and, if you specify port information, associates the floating IP with an internal port.
To associate the floating IP with an internal port, specify the port ID attribute in the request body. If you do not specify a port ID in the request, you can issue a PUT request instead of a POST request.
Default policy settings enable only administrative users to set floating IP addresses and some non-administrative users might require a floating IP address. If you do not specify a floating IP address in the request, the operation automatically allocates one.
By default, this operation associates the floating IP address with a single fixed IP address that is configured on an OpenStack Networking port. If a port has multiple IP addresses, you must specify the fixed_ip_address
attribute in the request body to associate a fixed IP address with the floating IP address.
You can create floating IPs on only external networks. When you create a floating IP, you must specify the ID of the network on which you want to create the floating IP. Alternatively, you can create a floating IP on a subnet in the external network, based on the costs and quality of that subnet.
You must configure an IP address with the internal OpenStack Networking port that is associated with the floating IP address.
The operation returns the Bad Request (400)
response code for one of reasons:
If the port ID is not valid, this operation returns 404
response code.
The operation returns the Conflict (409)
response code for one of reasons:
Normal response codes: 201
Error response codes: 400, 401, 404, 409
Usage: osc network floating-ip create [OPTIONS] --floating-network-id <FLOATING_NETWORK_ID>
Options:
--description <DESCRIPTION>
— A human-readable description for the resource. Default is an empty string--dns-domain <DNS_DOMAIN>
— A valid DNS domain--dns-name <DNS_NAME>
— A valid DNS name--fixed-ip-address <FIXED_IP_ADDRESS>
— The fixed IP address that is associated with the floating IP. If an internal port has multiple associated IP addresses, the service chooses the first IP address unless you explicitly define a fixed IP address in thefixed_ip_address
parameter--floating-ip-address <FLOATING_IP_ADDRESS>
— The floating IP address--floating-network-id <FLOATING_NETWORK_ID>
— The ID of the network associated with the floating IP--port-id <PORT_ID>
— The ID of a port associated with the floating IP. To associate the floating IP with a fixed IP at creation time, you must specify the identifier of the internal port--qos-policy-id <QOS_POLICY_ID>
— The ID of the QoS policy associated with the floating IP--subnet-id <SUBNET_ID>
— The subnet ID on which you want to create the floating IP--tenant-id <TENANT_ID>
— The ID of the project
osc network floating-ip delete
Deletes a floating IP and, if present, its associated port.
This example deletes a floating IP:
Normal response codes: 204
Error response codes: 401, 404, 412
Usage: osc network floating-ip delete <ID>
Arguments:
<ID>
— id parameter for /v2.0/floatingips/{id} API
osc network floating-ip list
Lists floating IPs visible to the user.
Default policy settings return only the floating IPs owned by the user’s project, unless the user has admin role.
Standard query parameters are supported on the URI. For more information, see Filtering and Column Selection.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Pagination query parameters are supported if Neutron configuration supports it by overriding allow_pagination=false
. For more information, see Pagination.
Sorting query parameters are supported if Neutron configuration supports it with allow_sorting=true
. For more information, see Sorting.
This example request lists floating IPs in JSON format:
Normal response codes: 200
Error response codes: 401
Usage: osc network floating-ip list [OPTIONS]
Options:
-
--description <DESCRIPTION>
— description query parameter for /v2.0/floatingips API -
--fixed-ip-address <FIXED_IP_ADDRESS>
— fixed_ip_address query parameter for /v2.0/floatingips API -
--floating-ip-address <FLOATING_IP_ADDRESS>
— floating_ip_address query parameter for /v2.0/floatingips API -
--floating-network-id <FLOATING_NETWORK_ID>
— floating_network_id query parameter for /v2.0/floatingips API -
--id <ID>
— id query parameter for /v2.0/floatingips API -
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--not-tags <NOT_TAGS>
— not-tags query parameter for /v2.0/floatingips API -
--not-tags-any <NOT_TAGS_ANY>
— not-tags-any query parameter for /v2.0/floatingips API -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--port-id <PORT_ID>
— port_id query parameter for /v2.0/floatingips API -
--revision-number <REVISION_NUMBER>
— revision_number query parameter for /v2.0/floatingips API -
--router-id <ROUTER_ID>
— router_id query parameter for /v2.0/floatingips API -
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--status <STATUS>
— status query parameter for /v2.0/floatingips API -
--tags <TAGS>
— tags query parameter for /v2.0/floatingips API -
--tags-any <TAGS_ANY>
— tags-any query parameter for /v2.0/floatingips API -
--tenant-id <TENANT_ID>
— tenant_id query parameter for /v2.0/floatingips API -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network floating-ip port-forwarding
Floating IPs port forwarding
Lists, creates, shows details for, updates, and deletes floating IPs port forwardings.
Port forwarding with port ranges
The floating-ip-port-forwarding-port-ranges extension adds the new attributes internal_port_range and external_port_range to the floating IP port forwardings. The value of these new attributes should be a string that represents a colon separated port range. You can not use the attributes internal_port_range and external_port_range with the attributes internal_port and external_port in the same request.
Port forwarding rule description
The floating-ip-port-forwarding-description extension adds the description attribute to the floating IP port forwardings. The value of the description attribute contains a text describing the rule, which helps users to manage/find easily theirs rules.
Usage: osc network floating-ip port-forwarding <COMMAND>
Available subcommands:
osc network floating-ip port-forwarding create
— Create port forwardingosc network floating-ip port-forwarding delete
— Delete a floating IP port forwardingosc network floating-ip port-forwarding list
— List floating IP port forwardingsosc network floating-ip port-forwarding set
— Update a port forwardingosc network floating-ip port-forwarding show
— Show port forwarding
osc network floating-ip port-forwarding create
Creates a floating IP port forwarding.
Normal response codes: 201
Error response codes: 400, 404
Usage: osc network floating-ip port-forwarding create [OPTIONS] <FLOATINGIP_ID>
Arguments:
<FLOATINGIP_ID>
— floatingip_id parameter for /v2.0/floatingips/{floatingip_id}/port_forwardings/{id} API
Options:
-
--description <DESCRIPTION>
— A text describing the rule, which helps users to manage/find easily theirs rules -
--external-port <EXTERNAL_PORT>
— The TCP/UDP/other protocol port number of the port forwarding’s floating IP address -
--external-port-range <EXTERNAL_PORT_RANGE>
— The TCP/UDP/other protocol port range of the port forwarding’s floating IP address -
--internal-ip-address <INTERNAL_IP_ADDRESS>
— The fixed IPv4 address of the Neutron port associated to the floating IP port forwarding -
--internal-port <INTERNAL_PORT>
— The TCP/UDP/other protocol port number of the Neutron port fixed IP address associated to the floating ip port forwarding -
--internal-port-id <INTERNAL_PORT_ID>
— The ID of the Neutron port associated to the floating IP port forwarding -
--internal-port-range <INTERNAL_PORT_RANGE>
— The TCP/UDP/other protocol port range of the Neutron port fixed IP address associated to the floating ip port forwarding -
--project-id <PROJECT_ID>
-
--protocol <PROTOCOL>
— The IP protocol used in the floating IP port forwardingPossible values:
dccp
,icmp
,ipv6-icmp
,sctp
,tcp
,udp
osc network floating-ip port-forwarding delete
Deletes a floating IP port forwarding.
Normal response codes: 204
Error response codes: 404
Usage: osc network floating-ip port-forwarding delete <FLOATINGIP_ID> <ID>
Arguments:
<FLOATINGIP_ID>
— floatingip_id parameter for /v2.0/floatingips/{floatingip_id}/port_forwardings/{id} API<ID>
— id parameter for /v2.0/floatingips/{floatingip_id}/port_forwardings/{id} API
osc network floating-ip port-forwarding list
Lists floating IP port forwardings that the project has access to.
Default policy settings return only the port forwardings associated to floating IPs owned by the project of the user submitting the request, unless the user has administrative role.
Standard query parameters are supported on the URI. For more information, see Filtering and Column Selection.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Pagination query parameters are supported if Neutron configuration supports it by overriding allow_pagination=false
. For more information, see Pagination.
Sorting query parameters are supported if Neutron configuration supports it with allow_sorting=true
. For more information, see Sorting.
Normal response codes: 200
Error response codes: 400, 404
Usage: osc network floating-ip port-forwarding list [OPTIONS] <FLOATINGIP_ID>
Arguments:
<FLOATINGIP_ID>
— floatingip_id parameter for /v2.0/floatingips/{floatingip_id}/port_forwardings/{id} API
Options:
-
--description <DESCRIPTION>
— description query parameter for /v2.0/floatingips/{floatingip_id}/port_forwardings API -
--external-port <EXTERNAL_PORT>
— external_port query parameter for /v2.0/floatingips/{floatingip_id}/port_forwardings API -
--external-port-range <EXTERNAL_PORT_RANGE>
— external_port_range query parameter for /v2.0/floatingips/{floatingip_id}/port_forwardings API -
--id <ID>
— id query parameter for /v2.0/floatingips/{floatingip_id}/port_forwardings API -
--internal-port-id <INTERNAL_PORT_ID>
— internal_port_id query parameter for /v2.0/floatingips/{floatingip_id}/port_forwardings API -
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--protocol <PROTOCOL>
— protocol query parameter for /v2.0/floatingips/{floatingip_id}/port_forwardings APIPossible values:
dccp
,icmp
,ipv6-icmp
,sctp
,tcp
,udp
-
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network floating-ip port-forwarding set
Updates a floating IP port forwarding.
Normal response codes: 200
Error response codes: 400, 404
Usage: osc network floating-ip port-forwarding set [OPTIONS] <FLOATINGIP_ID> <ID>
Arguments:
<FLOATINGIP_ID>
— floatingip_id parameter for /v2.0/floatingips/{floatingip_id}/port_forwardings/{id} API<ID>
— id parameter for /v2.0/floatingips/{floatingip_id}/port_forwardings/{id} API
Options:
-
--description <DESCRIPTION>
-
--external-port <EXTERNAL_PORT>
— The TCP/UDP/other protocol port number of the port forwarding’s floating IP address -
--external-port-range <EXTERNAL_PORT_RANGE>
— The TCP/UDP/other protocol port range of the port forwarding’s floating IP address -
--internal-ip-address <INTERNAL_IP_ADDRESS>
— The fixed IPv4 address of the Neutron port associated to the floating IP port forwarding -
--internal-port <INTERNAL_PORT>
— The TCP/UDP/other protocol port number of the Neutron port fixed IP address associated to the floating ip port forwarding -
--internal-port-id <INTERNAL_PORT_ID>
— The ID of the Neutron port associated to the floating IP port forwarding -
--internal-port-range <INTERNAL_PORT_RANGE>
— The TCP/UDP/other protocol port range of the Neutron port fixed IP address associated to the floating ip port forwarding -
--protocol <PROTOCOL>
— The IP protocol used in the floating IP port forwardingPossible values:
dccp
,icmp
,ipv6-icmp
,sctp
,tcp
,udp
osc network floating-ip port-forwarding show
Shows information for a floating IP port forwarding.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Normal response codes: 200
Error response codes: 400, 404
Usage: osc network floating-ip port-forwarding show <FLOATINGIP_ID> <ID>
Arguments:
<FLOATINGIP_ID>
— floatingip_id parameter for /v2.0/floatingips/{floatingip_id}/port_forwardings/{id} API<ID>
— id parameter for /v2.0/floatingips/{floatingip_id}/port_forwardings/{id} API
osc network floating-ip set
Updates a floating IP and its association with an internal port.
The association process is the same as the process for the create floating IP operation.
To disassociate a floating IP from a port, set the port_id
attribute to null or omit it from the request body.
This example updates a floating IP:
Depending on the request body that you submit, this request associates a port with or disassociates a port from a floating IP.
Normal response codes: 200
Error response codes: 400, 401, 404, 409, 412
Usage: osc network floating-ip set [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.0/floatingips/{id} API
Options:
--description <DESCRIPTION>
— A human-readable description for the resource. Default is an empty string--fixed-ip-address <FIXED_IP_ADDRESS>
— The fixed IP address that is associated with the floating IP. If an internal port has multiple associated IP addresses, the service chooses the first IP address unless you explicitly define a fixed IP address in thefixed_ip_address
parameter--port-id <PORT_ID>
— The ID of a port associated with the floating IP. To associate the floating IP with a fixed IP, you must specify the ID of the internal port. To disassociate the floating IP,null
should be specified--qos-policy-id <QOS_POLICY_ID>
osc network floating-ip show
Shows details for a floating IP.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
This example request shows details for a floating IP in JSON format. This example also filters the result by the fixed_ip_address
and floating_ip_address
fields.
Normal response codes: 200
Error response codes: 401, 403, 404
Usage: osc network floating-ip show <ID>
Arguments:
<ID>
— id parameter for /v2.0/floatingips/{id} API
osc network floating-ip tag
Lists tags, creates, replaces or deletes one or more tags for a resource, checks the existence of a tag for a resource
Usage: osc network floating-ip tag <COMMAND>
Available subcommands:
osc network floating-ip tag add
— Command without description in OpenAPIosc network floating-ip tag check
— Command without description in OpenAPIosc network floating-ip tag delete
— Command without description in OpenAPIosc network floating-ip tag list
— Command without description in OpenAPIosc network floating-ip tag purge
— Command without description in OpenAPI
osc network floating-ip tag add
Command without description in OpenAPI
Usage: osc network floating-ip tag add <FLOATINGIP_ID> <ID>
Arguments:
<FLOATINGIP_ID>
— floatingip_id parameter for /v2.0/floatingips/{floatingip_id}/tags/{id} API<ID>
— id parameter for /v2.0/floatingips/{floatingip_id}/tags/{id} API
osc network floating-ip tag check
Command without description in OpenAPI
Usage: osc network floating-ip tag check <FLOATINGIP_ID> <ID>
Arguments:
<FLOATINGIP_ID>
— floatingip_id parameter for /v2.0/floatingips/{floatingip_id}/tags/{id} API<ID>
— id parameter for /v2.0/floatingips/{floatingip_id}/tags/{id} API
osc network floating-ip tag delete
Command without description in OpenAPI
Usage: osc network floating-ip tag delete <FLOATINGIP_ID> <ID>
Arguments:
<FLOATINGIP_ID>
— floatingip_id parameter for /v2.0/floatingips/{floatingip_id}/tags/{id} API<ID>
— id parameter for /v2.0/floatingips/{floatingip_id}/tags/{id} API
osc network floating-ip tag list
Command without description in OpenAPI
Usage: osc network floating-ip tag list [OPTIONS] <FLOATINGIP_ID>
Arguments:
<FLOATINGIP_ID>
— floatingip_id parameter for /v2.0/floatingips/{floatingip_id}/tags/{id} API
Options:
-
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network floating-ip tag purge
Command without description in OpenAPI
Usage: osc network floating-ip tag purge <FLOATINGIP_ID>
Arguments:
<FLOATINGIP_ID>
— floatingip_id parameter for /v2.0/floatingips/{floatingip_id}/tags/{id} API
osc network floating-ip-pool
Floating IP pools
Usage: osc network floating-ip-pool <COMMAND>
Available subcommands:
osc network floating-ip-pool list
— Command without description in OpenAPI
osc network floating-ip-pool list
Command without description in OpenAPI
Usage: osc network floating-ip-pool list [OPTIONS]
Options:
-
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network local-ip
Local IPs (local_ips)
Extension that allows users to create a virtual IP that can later be assigned to multiple ports/VMs (similar to anycast IP) and is guaranteed to only be reachable within the same physical server/node boundaries.
Usage: osc network local-ip <COMMAND>
Available subcommands:
osc network local-ip create
— Command without description in OpenAPIosc network local-ip delete
— Command without description in OpenAPIosc network local-ip list
— Command without description in OpenAPIosc network local-ip port-association
— Local IP Associations (port_associations)osc network local-ip set
— Command without description in OpenAPIosc network local-ip show
— Command without description in OpenAPI
osc network local-ip create
Command without description in OpenAPI
Usage: osc network local-ip create [OPTIONS]
Options:
-
--description <DESCRIPTION>
-
--ip-mode <IP_MODE>
Possible values:
passthrough
,translate
-
--local-ip-address <LOCAL_IP_ADDRESS>
-
--local-port-id <LOCAL_PORT_ID>
-
--name <NAME>
-
--network-id <NETWORK_ID>
-
--project-id <PROJECT_ID>
osc network local-ip delete
Command without description in OpenAPI
Usage: osc network local-ip delete <ID>
Arguments:
<ID>
— id parameter for /v2.0/local-ips/{id} API
osc network local-ip list
Command without description in OpenAPI
Usage: osc network local-ip list [OPTIONS]
Options:
-
--description <DESCRIPTION>
— description query parameter for /v2.0/local-ips API -
--id <ID>
— id query parameter for /v2.0/local-ips API -
--ip-mode <IP_MODE>
— ip_mode query parameter for /v2.0/local-ips APIPossible values:
passthrough
,translate
-
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--local-ip-address <LOCAL_IP_ADDRESS>
— local_ip_address query parameter for /v2.0/local-ips API -
--local-port-id <LOCAL_PORT_ID>
— local_port_id query parameter for /v2.0/local-ips API -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--name <NAME>
— name query parameter for /v2.0/local-ips API -
--network-id <NETWORK_ID>
— network_id query parameter for /v2.0/local-ips API -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--project-id <PROJECT_ID>
— project_id query parameter for /v2.0/local-ips API -
--revision-number <REVISION_NUMBER>
— revision_number query parameter for /v2.0/local-ips API -
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network local-ip port-association
Local IP Associations (port_associations)
The resource lets users assign Local IPs to user Ports. This is a sub-resource of the Local IP resource.
Usage: osc network local-ip port-association <COMMAND>
Available subcommands:
osc network local-ip port-association create
— Create Local IP Associationosc network local-ip port-association delete
— Delete Local IP Associationosc network local-ip port-association list
— List Local IP Associations
osc network local-ip port-association create
Creates a Local IP association with a given Port. If a Port has multiple fixed IPs user must specify which IP to use for association.
The operation returns the Conflict (409)
response code for one of reasons:
Normal response codes: 201
Error response codes: 400, 401, 404, 409
Usage: osc network local-ip port-association create [OPTIONS] <LOCAL_IP_ID>
Arguments:
<LOCAL_IP_ID>
— local_ip_id parameter for /v2.0/local_ips/{local_ip_id}/port_associations/{id} API
Options:
--fixed-ip <FIXED_IP>
— The requested IP of the port associated with the Local IP--fixed-port-id <FIXED_PORT_ID>
— The requested ID of the port associated with the Local IP--project-id <PROJECT_ID>
osc network local-ip port-association delete
Deletes a Local IP association.
Normal response codes: 204
Error response codes: 400, 401, 403, 404
Usage: osc network local-ip port-association delete <LOCAL_IP_ID> <ID>
Arguments:
<LOCAL_IP_ID>
— local_ip_id parameter for /v2.0/local_ips/{local_ip_id}/port_associations/{id} API<ID>
— id parameter for /v2.0/local_ips/{local_ip_id}/port_associations/{id} API
osc network local-ip port-association list
Lists Associations for the given Local IP.
Standard query parameters are supported on the URI. For more information, see Filtering and Column Selection.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Pagination query parameters are supported if Neutron configuration supports it by overriding allow_pagination=false
. For more information, see Pagination.
Sorting query parameters are supported if Neutron configuration supports it with allow_sorting=true
. For more information, see Sorting.
Normal response codes: 200
Error response codes: 401
Usage: osc network local-ip port-association list [OPTIONS] <LOCAL_IP_ID>
Arguments:
<LOCAL_IP_ID>
— local_ip_id parameter for /v2.0/local_ips/{local_ip_id}/port_associations/{id} API
Options:
-
--fixed-ip <FIXED_IP>
— fixed_ip query parameter for /v2.0/local_ips/{local_ip_id}/port_associations API -
--fixed-port-id <FIXED_PORT_ID>
— fixed_port_id query parameter for /v2.0/local_ips/{local_ip_id}/port_associations API -
--host <HOST>
— host query parameter for /v2.0/local_ips/{local_ip_id}/port_associations API -
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--local-ip-address <LOCAL_IP_ADDRESS>
— local_ip_address query parameter for /v2.0/local_ips/{local_ip_id}/port_associations API -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network local-ip set
Command without description in OpenAPI
Usage: osc network local-ip set [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.0/local-ips/{id} API
Options:
--description <DESCRIPTION>
--name <NAME>
osc network local-ip show
Command without description in OpenAPI
Usage: osc network local-ip show <ID>
Arguments:
<ID>
— id parameter for /v2.0/local-ips/{id} API
osc network log
Logging
Log resource
The logging extension lists, creates, shows information for, and updates log resource.
Usage: osc network log <COMMAND>
Available subcommands:
osc network log log
— Log resourceosc network log loggable-resource
— Loggable resources
osc network log log
Log resource
The logging extension lists, creates, shows information for, and updates log resource.
Usage: osc network log log <COMMAND>
Available subcommands:
osc network log log create
— Create logosc network log log delete
— Delete logosc network log log list
— List logsosc network log log set
— Update logosc network log log show
— Show log
osc network log log create
Creates a log resource.
Creates a log resource by using the configuration that you define in the request object. A response object is returned. The object contains a unique ID.
If the caller is not an administrative user, this call returns the HTTP Forbidden (403)
response code.
Users with an administrative role can create policies on behalf of other projects by specifying a project ID that is different than their own.
Normal response codes: 201
Error response codes: 400, 401, 403, 409
Usage: osc network log log create [OPTIONS]
Options:
-
--description <DESCRIPTION>
— A human-readable description for the resource. Default is an empty string -
--enabled <ENABLED>
— Indicates whether this log object is enabled or disabled. Default is truePossible values:
true
,false
-
--event <EVENT>
— Type of security events to log.ACCEPT
,DROP
, orALL
. Default isALL
Possible values:
accept
,all
,drop
-
--name <NAME>
— Human-readable name of the resource. Default is an empty string -
--project-id <PROJECT_ID>
— The ID of the project that owns the resource. Only administrative and users with advsvc role can specify a project ID other than their own. You cannot change this value through authorization policies -
--resource-id <RESOURCE_ID>
— The ID of resource log (e.g security group ID) -
--resource-type <RESOURCE_TYPE>
— The resource log type such as ‘security_group’ -
--target-id <TARGET_ID>
— The ID of resource target log such as port ID
osc network log log delete
Deletes a log resource.
Normal response codes: 204
Error response codes: 400, 401, 404, 412
Usage: osc network log log delete <ID>
Arguments:
<ID>
— id parameter for /v2.0/log/logs/{id} API
osc network log log list
Lists all log resources associated with your project.
Standard query parameters are supported on the URI. For more information, see Filtering and Column Selection.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Pagination query parameters are supported if Neutron configuration supports it by overriding allow_pagination=false
. For more information, see Pagination.
Sorting query parameters are supported if Neutron configuration supports it with allow_sorting=true
. For more information, see Sorting.
The list might be empty.
Normal response codes: 200
Error response codes: 401
Usage: osc network log log list [OPTIONS]
Options:
-
--description <DESCRIPTION>
— description query parameter for /v2.0/log/logs API -
--enabled <ENABLED>
— enabled query parameter for /v2.0/log/logs APIPossible values:
true
,false
-
--event <EVENT>
— event query parameter for /v2.0/log/logs APIPossible values:
ACCEPT
,ALL
,DROP
-
--id <ID>
— id query parameter for /v2.0/log/logs API -
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--name <NAME>
— name query parameter for /v2.0/log/logs API -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--project-id <PROJECT_ID>
— project_id query parameter for /v2.0/log/logs API -
--resource-id <RESOURCE_ID>
— resource_id query parameter for /v2.0/log/logs API -
--resource-type <RESOURCE_TYPE>
— resource_type query parameter for /v2.0/log/logs API -
--revision-number <REVISION_NUMBER>
— revision_number query parameter for /v2.0/log/logs API -
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--target-id <TARGET_ID>
— target_id query parameter for /v2.0/log/logs API -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network log log set
Updates a log resource.
Normal response codes: 200
Error response codes: 400, 401, 404, 412
Usage: osc network log log set [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.0/log/logs/{id} API
Options:
-
--description <DESCRIPTION>
— A human-readable description for the resource. Default is an empty string -
--enabled <ENABLED>
— Indicates whether this log object is enabled or disabledPossible values:
true
,false
-
--name <NAME>
— Human-readable name of the resource. Default is an empty string
osc network log log show
Shows details log resource.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Normal response codes: 200
Error response codes: 401, 404
Usage: osc network log log show <ID>
Arguments:
<ID>
— id parameter for /v2.0/log/logs/{id} API
osc network log loggable-resource
Loggable resources
Usage: osc network log loggable-resource <COMMAND>
Available subcommands:
osc network log loggable-resource list
— List loggable resources
osc network log loggable-resource list
Lists all resource log types are supporting.
Standard query parameters are supported on the URI. For more information, see Filtering and Column Selection.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Pagination query parameters are supported if Neutron configuration supports it by overriding allow_pagination=false
. For more information, see Pagination.
Sorting query parameters are supported if Neutron configuration supports it with allow_sorting=true
. For more information, see Sorting.
Normal response codes: 200
Error response codes: 401
Usage: osc network log loggable-resource list [OPTIONS]
Options:
-
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network metering
Metering labels and rules (metering-labels, metering-label-rules)
Creates, modifies, and deletes OpenStack Layer3 metering labels and rules.
Usage: osc network metering <COMMAND>
Available subcommands:
osc network metering metering-label
— Metering Label commandsosc network metering metering-label-rule
— Metering Label Rule commands
osc network metering metering-label
Metering Label commands
Usage: osc network metering metering-label <COMMAND>
Available subcommands:
osc network metering metering-label create
— Create metering labelosc network metering metering-label delete
— Delete metering labelosc network metering metering-label list
— List metering labelsosc network metering metering-label show
— Show metering label details
osc network metering metering-label create
Creates an L3 metering label.
Normal response codes: 201
Error response codes: 400, 401, 403
Usage: osc network metering metering-label create [OPTIONS]
Options:
-
--description <DESCRIPTION>
— A human-readable description for the resource. Default is an empty string -
--name <NAME>
— Human-readable name of the resource. Default is an empty string -
--shared <SHARED>
— Indicates whether this metering label is shared across all projectsPossible values:
true
,false
-
--tenant-id <TENANT_ID>
— The ID of the project that owns the resource. Only administrative and users with advsvc role can specify a project ID other than their own. You cannot change this value through authorization policies
osc network metering metering-label delete
Deletes an L3 metering label.
Normal response codes: 204
Error response codes: 401, 404
Usage: osc network metering metering-label delete <ID>
Arguments:
<ID>
— id parameter for /v2.0/metering/metering-labels/{id} API
osc network metering metering-label list
Lists all L3 metering labels that belong to the project.
The list shows the ID for each metering label.
Standard query parameters are supported on the URI. For more information, see Filtering and Column Selection.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Pagination query parameters are supported if Neutron configuration supports it by overriding allow_pagination=false
. For more information, see Pagination.
Sorting query parameters are supported if Neutron configuration supports it with allow_sorting=true
. For more information, see Sorting.
Normal response codes: 200
Error response codes: 401
Usage: osc network metering metering-label list [OPTIONS]
Options:
-
--description <DESCRIPTION>
— description query parameter for /v2.0/metering/metering-labels API -
--id <ID>
— id query parameter for /v2.0/metering/metering-labels API -
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--name <NAME>
— name query parameter for /v2.0/metering/metering-labels API -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--shared <SHARED>
— shared query parameter for /v2.0/metering/metering-labels APIPossible values:
true
,false
-
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--tenant-id <TENANT_ID>
— tenant_id query parameter for /v2.0/metering/metering-labels API -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network metering metering-label show
Shows details for a metering label.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Normal response codes: 200
Error response codes: 401, 404
Usage: osc network metering metering-label show <ID>
Arguments:
<ID>
— id parameter for /v2.0/metering/metering-labels/{id} API
osc network metering metering-label-rule
Metering Label Rule commands
Usage: osc network metering metering-label-rule <COMMAND>
Available subcommands:
osc network metering metering-label-rule create
— Create metering label ruleosc network metering metering-label-rule delete
— Delete metering label ruleosc network metering metering-label-rule list
— List metering label rulesosc network metering metering-label-rule show
— Show metering label rule details
osc network metering metering-label-rule create
Creates an L3 metering label rule.
Normal response codes: 201
Error response codes: 400, 401, 403, 404, 409
Usage: osc network metering metering-label-rule create [OPTIONS]
Options:
-
--destination-ip-prefix <DESTINATION_IP_PREFIX>
-
--direction <DIRECTION>
— Ingress or egress, which is the direction in which the metering rule is appliedPossible values:
egress
,ingress
-
--excluded <EXCLUDED>
— Indicates whether to count the traffic of a specific IP address with theremote_ip_prefix
,source_ip_prefix
, ordestination_ip_prefix
values. Default isfalse
Possible values:
true
,false
-
--metering-label-id <METERING_LABEL_ID>
— The metering label ID associated with this metering rule -
--remote-ip-prefix <REMOTE_IP_PREFIX>
— (deprecated) The source IP prefix that is matched by this metering rule. By source IP prefix, one should read the internal/private IPs used in OpenStack -
--source-ip-prefix <SOURCE_IP_PREFIX>
-
--tenant-id <TENANT_ID>
osc network metering metering-label-rule delete
Deletes an L3 metering label rule.
Normal response codes: 204
Error response codes: 401, 404
Usage: osc network metering metering-label-rule delete <ID>
Arguments:
<ID>
— id parameter for /v2.0/metering/metering-label-rules/{id} API
osc network metering metering-label-rule list
Lists a summary of all L3 metering label rules that belong to the project.
The list shows the ID for each metering label rule.
Standard query parameters are supported on the URI. For more information, see Filtering and Column Selection.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Pagination query parameters are supported if Neutron configuration supports it by overriding allow_pagination=false
. For more information, see Pagination.
Sorting query parameters are supported if Neutron configuration supports it with allow_sorting=true
. For more information, see Sorting.
Normal response codes: 200
Error response codes: 401
Usage: osc network metering metering-label-rule list [OPTIONS]
Options:
-
--destination-ip-prefix <DESTINATION_IP_PREFIX>
— The destination IP prefix that the metering rule is associated with; in this context, destination IP prefix represents the destination IP of the network packet. Therefore, for an ingress rule, the destination IP is the internal IP associated with some OpenStack VM. On the other hand, for an egress rule, the destination IP prefix is the IP of some external system that an application running inside some OpenStack virtual machine is trying to access. Moreover, instead of an IP, one can also use a CIDR as the destination IP prefix -
--direction <DIRECTION>
— direction query parameter for /v2.0/metering/metering-label-rules APIPossible values:
egress
,ingress
-
--excluded <EXCLUDED>
— excluded query parameter for /v2.0/metering/metering-label-rules APIPossible values:
true
,false
-
--id <ID>
— id query parameter for /v2.0/metering/metering-label-rules API -
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--metering-label-id <METERING_LABEL_ID>
— metering_label_id query parameter for /v2.0/metering/metering-label-rules API -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--remote-ip-prefix <REMOTE_IP_PREFIX>
— remote_ip_prefix query parameter for /v2.0/metering/metering-label-rules API -
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--source-ip-prefix <SOURCE_IP_PREFIX>
— The source IP prefix that the metering rule is associated with; in this context, source IP prefix represents the source IP of the network packet. Therefore, for an ingress rule, the source IP is the IP of the system accessing something inside OpenStack. On the other hand, for an egress rule, the source IP is the internal IP associated with some OpenStack VM. Moreover, instead of an IP, one can also use a CIDR as the source IP prefix -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network metering metering-label-rule show
Shows details for a metering label rule.
The response body shows this information for each metering label rule:
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Normal response codes: 200
Error response codes: 401, 404
Usage: osc network metering metering-label-rule show <ID>
Arguments:
<ID>
— id parameter for /v2.0/metering/metering-label-rules/{id} API
osc network ndp-proxy
Router NDP proxy (ndp_proxies)
A ndp_proxy is a logical entity for annunciate a unique IPv6 address to external network. It depends on a router entity on which external gateway is enabled.
Usage: osc network ndp-proxy <COMMAND>
Available subcommands:
osc network ndp-proxy create
— Command without description in OpenAPIosc network ndp-proxy delete
— Command without description in OpenAPIosc network ndp-proxy list
— Command without description in OpenAPIosc network ndp-proxy set
— Command without description in OpenAPIosc network ndp-proxy show
— Command without description in OpenAPI
osc network ndp-proxy create
Command without description in OpenAPI
Usage: osc network ndp-proxy create [OPTIONS]
Options:
--description <DESCRIPTION>
--ip-address <IP_ADDRESS>
--name <NAME>
--port-id <PORT_ID>
--project-id <PROJECT_ID>
--router-id <ROUTER_ID>
osc network ndp-proxy delete
Command without description in OpenAPI
Usage: osc network ndp-proxy delete <ID>
Arguments:
<ID>
— id parameter for /v2.0/ndp-proxies/{id} API
osc network ndp-proxy list
Command without description in OpenAPI
Usage: osc network ndp-proxy list [OPTIONS]
Options:
-
--description <DESCRIPTION>
— description query parameter for /v2.0/ndp-proxies API -
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--name <NAME>
— name query parameter for /v2.0/ndp-proxies API -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--revision-number <REVISION_NUMBER>
— revision_number query parameter for /v2.0/ndp-proxies API -
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network ndp-proxy set
Command without description in OpenAPI
Usage: osc network ndp-proxy set [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.0/ndp-proxies/{id} API
Options:
--description <DESCRIPTION>
--name <NAME>
osc network ndp-proxy show
Command without description in OpenAPI
Usage: osc network ndp-proxy show <ID>
Arguments:
<ID>
— id parameter for /v2.0/ndp-proxies/{id} API
osc network network
Network commands
Usage: osc network network <COMMAND>
Available subcommands:
osc network network create
— Create networkosc network network delete
— Delete networkosc network network dhcp-agent
— DHCP agent schedulerosc network network list
— List networksosc network network show
— Show network detailsosc network network tag
— Lists tags, creates, replaces or deletes one or more tags for a resource, checks the existence of a tag for a resource
osc network network create
Creates a network.
A request body is optional. An administrative user can specify another project ID, which is the project that owns the network, in the request body.
Normal response codes: 201
Error response codes: 400, 401
Usage: osc network network create [OPTIONS]
Options:
-
--admin-state-up <ADMIN_STATE_UP>
— The administrative state of the network, which is up (true
) or down (false
)Possible values:
true
,false
-
--availability-zone-hints <AVAILABILITY_ZONE_HINTS>
— The availability zone candidate for the network.Parameter is an array, may be provided multiple times.
-
--description <DESCRIPTION>
— A human-readable description for the resource. Default is an empty string -
--dns-domain <DNS_DOMAIN>
— A valid DNS domain -
--ha <HA>
Possible values:
true
,false
-
--is-default <IS_DEFAULT>
— The network is default or notPossible values:
true
,false
-
--mtu <MTU>
— The maximum transmission unit (MTU) value to address fragmentation. Minimum value is 68 for IPv4, and 1280 for IPv6 -
--name <NAME>
— Human-readable name of the network -
--port-security-enabled <PORT_SECURITY_ENABLED>
— The port security status of the network. Valid values are enabled (true
) and disabled (false
). This value is used as the default value ofport_security_enabled
field of a newly created portPossible values:
true
,false
-
--provider-network-type <PROVIDER_NETWORK_TYPE>
-
--provider-physical-network <PROVIDER_PHYSICAL_NETWORK>
-
--provider-segmentation-id <PROVIDER_SEGMENTATION_ID>
-
--qos-policy-id <QOS_POLICY_ID>
— The ID of the QoS policy associated with the network -
--router-external <ROUTER_EXTERNAL>
— Indicates whether the network has an external routing facility that’s not managed by the networking servicePossible values:
true
,false
-
--segments <JSON>
— A list of providersegment
objects.Parameter is an array, may be provided multiple times.
-
--shared <SHARED>
— Indicates whether this resource is shared across all projects. By default, only administrative users can change this valuePossible values:
true
,false
-
--tenant-id <TENANT_ID>
— The ID of the project that owns the resource. Only administrative and users with advsvc role can specify a project ID other than their own. You cannot change this value through authorization policies
osc network network delete
Deletes a network and its associated resources.
Normal response codes: 204
Error response codes: 401, 404, 409, 412
Usage: osc network network delete <ID>
Arguments:
<ID>
— network_id parameter for /v2.0/networks/{network_id} API
osc network network dhcp-agent
DHCP agent scheduler
The DHCP agent scheduler extension (dhcp_agent_scheduler) enables administrators to assign DHCP servers for Neutron networks to given Neutron DHCP agents, and retrieve mappings between Neutron networks and DHCP agents.
Usage: osc network network dhcp-agent <COMMAND>
Available subcommands:
osc network network dhcp-agent list
— List DHCP agents hosting a network
osc network network dhcp-agent list
Lists DHCP agents hosting a network.
Standard query parameters are supported on the URI. For more information, see Filtering and Column Selection.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Pagination query parameters are supported if Neutron configuration supports it by overriding allow_pagination=false
. For more information, see Pagination.
Sorting query parameters are supported if Neutron configuration supports it with allow_sorting=true
. For more information, see Sorting.
Normal response codes: 200
Error response codes: 401, 403
Usage: osc network network dhcp-agent list [OPTIONS] <NETWORK_ID>
Arguments:
<NETWORK_ID>
— network_id parameter for /v2.0/networks/{network_id}/dhcp-agents/{id} API
Options:
-
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network network list
Lists networks to which the project has access.
Default policy settings return only networks that the project who submits the request owns, unless an administrative user submits the request. In addition, networks shared with the project who submits the request are also returned.
Standard query parameters are supported on the URI. For more information, see Filtering and Column Selection.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Pagination query parameters are supported if Neutron configuration supports it by overriding allow_pagination=false
. For more information, see Pagination.
Sorting query parameters are supported if Neutron configuration supports it with allow_sorting=true
. For more information, see Sorting.
You can also use the tags
, tags-any
, not-tags
, not-tags-any
query parameter to filter the response with tags. For information, see REST API Impact.
Normal response codes: 200
Error response codes: 401
Usage: osc network network list [OPTIONS]
Options:
-
--admin-state-up <ADMIN_STATE_UP>
— admin_state_up query parameter for /v2.0/networks APIPossible values:
true
,false
-
--description <DESCRIPTION>
— description query parameter for /v2.0/networks API -
--id <ID>
— id query parameter for /v2.0/networks API -
--is-default <IS_DEFAULT>
— is_default query parameter for /v2.0/networks APIPossible values:
true
,false
-
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--mtu <MTU>
— mtu query parameter for /v2.0/networks API -
--name <NAME>
— name query parameter for /v2.0/networks API -
--not-tags <NOT_TAGS>
— not-tags query parameter for /v2.0/networks API -
--not-tags-any <NOT_TAGS_ANY>
— not-tags-any query parameter for /v2.0/networks API -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--provider-network-type <PROVIDER_NETWORK_TYPE>
— provider:network_type query parameter for /v2.0/networks API -
--provider-physical-network <PROVIDER_PHYSICAL_NETWORK>
— provider:physical_network query parameter for /v2.0/networks API -
--provider-segmentation-id <PROVIDER_SEGMENTATION_ID>
— provider:segmentation_id query parameter for /v2.0/networks API -
--revision-number <REVISION_NUMBER>
— revision_number query parameter for /v2.0/networks API -
--router-external <ROUTER_EXTERNAL>
— router:external query parameter for /v2.0/networks APIPossible values:
true
,false
-
--shared <SHARED>
— shared query parameter for /v2.0/networks APIPossible values:
true
,false
-
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--status <STATUS>
— status query parameter for /v2.0/networks API -
--tags <TAGS>
— tags query parameter for /v2.0/networks API -
--tags-any <TAGS_ANY>
— tags-any query parameter for /v2.0/networks API -
--tenant-id <TENANT_ID>
— tenant_id query parameter for /v2.0/networks API -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network network show
Shows details for a network.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Normal response codes: 200
Error response codes: 401, 404
Usage: osc network network show <ID>
Arguments:
<ID>
— network_id parameter for /v2.0/networks/{network_id} API
osc network network tag
Lists tags, creates, replaces or deletes one or more tags for a resource, checks the existence of a tag for a resource
Usage: osc network network tag <COMMAND>
Available subcommands:
osc network network tag add
— Command without description in OpenAPIosc network network tag check
— Command without description in OpenAPIosc network network tag delete
— Command without description in OpenAPIosc network network tag list
— Command without description in OpenAPIosc network network tag purge
— Command without description in OpenAPI
osc network network tag add
Command without description in OpenAPI
Usage: osc network network tag add <NETWORK_ID> <ID>
Arguments:
<NETWORK_ID>
— network_id parameter for /v2.0/networks/{network_id}/tags/{id} API<ID>
— id parameter for /v2.0/networks/{network_id}/tags/{id} API
osc network network tag check
Command without description in OpenAPI
Usage: osc network network tag check <NETWORK_ID> <ID>
Arguments:
<NETWORK_ID>
— network_id parameter for /v2.0/networks/{network_id}/tags/{id} API<ID>
— id parameter for /v2.0/networks/{network_id}/tags/{id} API
osc network network tag delete
Command without description in OpenAPI
Usage: osc network network tag delete <NETWORK_ID> <ID>
Arguments:
<NETWORK_ID>
— network_id parameter for /v2.0/networks/{network_id}/tags/{id} API<ID>
— id parameter for /v2.0/networks/{network_id}/tags/{id} API
osc network network tag list
Command without description in OpenAPI
Usage: osc network network tag list [OPTIONS] <NETWORK_ID>
Arguments:
<NETWORK_ID>
— network_id parameter for /v2.0/networks/{network_id}/tags/{id} API
Options:
-
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network network tag purge
Command without description in OpenAPI
Usage: osc network network tag purge <NETWORK_ID>
Arguments:
<NETWORK_ID>
— network_id parameter for /v2.0/networks/{network_id}/tags/{id} API
osc network network-ip-availability
Network IP availability and usage stats
The extension network-ip-availability allows users to list and show the network IP usage stats of all networks or of a specified network. By default policy configuration, only administrative users can use this API.
Usage: osc network network-ip-availability <COMMAND>
Available subcommands:
osc network network-ip-availability list
— List Network IP Availabilityosc network network-ip-availability show
— Show Network IP Availability
osc network network-ip-availability list
Lists network IP availability of all networks.
By default policy configuration, only administrative users can retrieve IP availabilities. Otherwise, an empty list will be returned.
Standard query parameters are supported on the URI. For more information, see Filtering and Column Selection.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Pagination query parameters are supported if Neutron configuration supports it by overriding allow_pagination=false
. For more information, see Pagination.
Sorting query parameters are supported if Neutron configuration supports it with allow_sorting=true
. For more information, see Sorting.
Normal response codes: 200
Error response codes: 401
Usage: osc network network-ip-availability list [OPTIONS]
Options:
-
--ip-version <IP_VERSION>
— ip_version query parameter for /v2.0/network-ip-availabilities API -
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--network-id <NETWORK_ID>
— network_id query parameter for /v2.0/network-ip-availabilities API -
--network-name <NETWORK_NAME>
— network_name query parameter for /v2.0/network-ip-availabilities API -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--tenant-id <TENANT_ID>
— tenant_id query parameter for /v2.0/network-ip-availabilities API -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network network-ip-availability show
Shows network IP availability details for a network.
By default policy configuration, only administrative users can retrieve IP availability. Otherwise, Not Found (404)
will be returned.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Normal response codes: 200
Error response codes: 401, 404
Usage: osc network network-ip-availability show <ID>
Arguments:
<ID>
— id parameter for /v2.0/network-ip-availabilities/{id} API
osc network network-segment-range
Network Segment Ranges
The network segment range extension exposes the segment range management to be administered via the Neutron API. It introduces the network-segment-range resource for tenant network segment allocation. In addition, it introduces the ability for the administrator to control the segment ranges globally or on a per-tenant basis.
Lists, shows details for, creates, updates, and deletes network segment ranges. The network segment ranges API is admin-only.
Usage: osc network network-segment-range <COMMAND>
Available subcommands:
osc network network-segment-range create
— Command without description in OpenAPIosc network network-segment-range delete
— Command without description in OpenAPIosc network network-segment-range list
— Command without description in OpenAPIosc network network-segment-range set
— Command without description in OpenAPIosc network network-segment-range show
— Command without description in OpenAPIosc network network-segment-range tag
— Lists tags, creates, replaces or deletes one or more tags for a resource, checks the existence of a tag for a resource
osc network network-segment-range create
Command without description in OpenAPI
Usage: osc network network-segment-range create [OPTIONS]
Options:
-
--description <DESCRIPTION>
-
--maximum <MAXIMUM>
-
--minimum <MINIMUM>
-
--name <NAME>
-
--network-type <NETWORK_TYPE>
Possible values:
geneve
,gre
,vlan
,vxlan
-
--physical-network <PHYSICAL_NETWORK>
-
--project-id <PROJECT_ID>
-
--shared <SHARED>
Possible values:
true
,false
osc network network-segment-range delete
Command without description in OpenAPI
Usage: osc network network-segment-range delete <ID>
Arguments:
<ID>
— id parameter for /v2.0/network-segment-ranges/{id} API
osc network network-segment-range list
Command without description in OpenAPI
Usage: osc network network-segment-range list [OPTIONS]
Options:
-
--description <DESCRIPTION>
— description query parameter for /v2.0/network-segment-ranges API -
--id <ID>
— id query parameter for /v2.0/network-segment-ranges API -
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--name <NAME>
— name query parameter for /v2.0/network-segment-ranges API -
--network-type <NETWORK_TYPE>
— network_type query parameter for /v2.0/network-segment-ranges APIPossible values:
geneve
,gre
,vlan
,vxlan
-
--not-tags <NOT_TAGS>
— not-tags query parameter for /v2.0/network-segment-ranges API -
--not-tags-any <NOT_TAGS_ANY>
— not-tags-any query parameter for /v2.0/network-segment-ranges API -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--physical-network <PHYSICAL_NETWORK>
— physical_network query parameter for /v2.0/network-segment-ranges API -
--project-id <PROJECT_ID>
— project_id query parameter for /v2.0/network-segment-ranges API -
--revision-number <REVISION_NUMBER>
— revision_number query parameter for /v2.0/network-segment-ranges API -
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--tags <TAGS>
— tags query parameter for /v2.0/network-segment-ranges API -
--tags-any <TAGS_ANY>
— tags-any query parameter for /v2.0/network-segment-ranges API -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network network-segment-range set
Command without description in OpenAPI
Usage: osc network network-segment-range set [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.0/network-segment-ranges/{id} API
Options:
--description <DESCRIPTION>
--maximum <MAXIMUM>
--minimum <MINIMUM>
--name <NAME>
osc network network-segment-range show
Command without description in OpenAPI
Usage: osc network network-segment-range show <ID>
Arguments:
<ID>
— id parameter for /v2.0/network-segment-ranges/{id} API
osc network network-segment-range tag
Lists tags, creates, replaces or deletes one or more tags for a resource, checks the existence of a tag for a resource
Usage: osc network network-segment-range tag <COMMAND>
Available subcommands:
osc network network-segment-range tag add
— Command without description in OpenAPIosc network network-segment-range tag check
— Command without description in OpenAPIosc network network-segment-range tag delete
— Command without description in OpenAPIosc network network-segment-range tag list
— Command without description in OpenAPIosc network network-segment-range tag purge
— Command without description in OpenAPI
osc network network-segment-range tag add
Command without description in OpenAPI
Usage: osc network network-segment-range tag add <NETWORK_SEGMENT_RANGE_ID> <ID>
Arguments:
<NETWORK_SEGMENT_RANGE_ID>
— network_segment_range_id parameter for /v2.0/network_segment_ranges/{network_segment_range_id}/tags/{id} API<ID>
— id parameter for /v2.0/network_segment_ranges/{network_segment_range_id}/tags/{id} API
osc network network-segment-range tag check
Command without description in OpenAPI
Usage: osc network network-segment-range tag check <NETWORK_SEGMENT_RANGE_ID> <ID>
Arguments:
<NETWORK_SEGMENT_RANGE_ID>
— network_segment_range_id parameter for /v2.0/network_segment_ranges/{network_segment_range_id}/tags/{id} API<ID>
— id parameter for /v2.0/network_segment_ranges/{network_segment_range_id}/tags/{id} API
osc network network-segment-range tag delete
Command without description in OpenAPI
Usage: osc network network-segment-range tag delete <NETWORK_SEGMENT_RANGE_ID> <ID>
Arguments:
<NETWORK_SEGMENT_RANGE_ID>
— network_segment_range_id parameter for /v2.0/network_segment_ranges/{network_segment_range_id}/tags/{id} API<ID>
— id parameter for /v2.0/network_segment_ranges/{network_segment_range_id}/tags/{id} API
osc network network-segment-range tag list
Command without description in OpenAPI
Usage: osc network network-segment-range tag list [OPTIONS] <NETWORK_SEGMENT_RANGE_ID>
Arguments:
<NETWORK_SEGMENT_RANGE_ID>
— network_segment_range_id parameter for /v2.0/network_segment_ranges/{network_segment_range_id}/tags/{id} API
Options:
-
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network network-segment-range tag purge
Command without description in OpenAPI
Usage: osc network network-segment-range tag purge <NETWORK_SEGMENT_RANGE_ID>
Arguments:
<NETWORK_SEGMENT_RANGE_ID>
— network_segment_range_id parameter for /v2.0/network_segment_ranges/{network_segment_range_id}/tags/{id} API
osc network port
Port commands
Usage: osc network port <COMMAND>
Available subcommands:
osc network port create
— Create portosc network port delete
— Delete portosc network port list
— List portsosc network port show
— Show port detailsosc network port tag
— Lists tags, creates, replaces or deletes one or more tags for a resource, checks the existence of a tag for a resource
osc network port create
Creates a port on a network.
To define the network in which to create the port, specify the network_id
attribute in the request body.
Normal response codes: 201
Error response codes: 400, 401, 403, 404
Usage: osc network port create [OPTIONS]
Options:
-
--admin-state-up <ADMIN_STATE_UP>
— The administrative state of the resource, which is up (true
) or down (false
). Default istrue
Possible values:
true
,false
-
--allowed-address-pairs <JSON>
— A set of zero or more allowed address pair objects each where address pair object contains anip_address
andmac_address
. While theip_address
is required, themac_address
will be taken from the port if not specified. The value ofip_address
can be an IP Address or a CIDR (if supported by the underlying extension plugin). A server connected to the port can send a packet with source address which matches one of the specified allowed address pairs.Parameter is an array, may be provided multiple times.
-
--binding-host-id <BINDING_HOST_ID>
— The ID of the host where the port resides. The default is an empty string -
--binding-profile <key=value>
— A dictionary that enables the application running on the specific host to pass and receive vif port information specific to the networking back-end. This field is only meant for machine-machine communication for compute services like Nova, Ironic or Zun to pass information to a Neutron back-end. It should not be used by multiple services concurrently or by cloud end users. The existing counterexamples (capabilities: [switchdev]
for Open vSwitch hardware offload andtrusted=true
for Trusted Virtual Functions) are due to be cleaned up. The networking API does not define a specific format of this field. The default is an empty dictionary. If you update it with null then it is treated like {} in the response. Since the port-mac-address-override extension thedevice_mac_address
field of the binding:profile can be used to provide the MAC address of the physical device a direct-physical port is being bound to. If provided, then themac_address
field of the port resource will be updated to the MAC from the active binding -
--binding-vnic-type <BINDING_VNIC_TYPE>
— The type of vNIC which this port should be attached to. This is used to determine which mechanism driver(s) to be used to bind the port. The valid values arenormal
,macvtap
,direct
,baremetal
,direct-physical
,virtio-forwarder
,smart-nic
andremote-managed
. What type of vNIC is actually available depends on deployments. The default isnormal
Possible values:
accelerator-direct
,accelerator-direct-physical
,baremetal
,direct
,direct-physical
,macvtap
,normal
,remote-managed
,smart-nic
,vdpa
,virtio-forwarder
-
--description <DESCRIPTION>
— A human-readable description for the resource. Default is an empty string -
--device-id <DEVICE_ID>
— The ID of the device that uses this port. For example, a server instance or a logical router -
--device-owner <DEVICE_OWNER>
— The entity type that uses this port. For example,compute:nova
(server instance),network:dhcp
(DHCP agent) ornetwork:router_interface
(router interface) -
--device-profile <DEVICE_PROFILE>
-
--dns-domain <DNS_DOMAIN>
— A valid DNS domain -
--dns-name <DNS_NAME>
— A valid DNS name -
--extra-dhcp-opts <JSON>
— A set of zero or more extra DHCP option pairs. An option pair consists of an option value and name.Parameter is an array, may be provided multiple times.
-
--fixed-ips <JSON>
— The IP addresses for the port. If you would like to assign multiple IP addresses for the port, specify multiple entries in this field. Each entry consists of IP address (ip_address
) and the subnet ID from which the IP address is assigned (subnet_id
).- If you specify both a subnet ID and an IP address, OpenStack Networking tries to allocate the IP address on that subnet to the port. - If you specify only a subnet ID, OpenStack Networking allocates an available IP from that subnet to the port. - If you specify only an IP address, OpenStack Networking tries to allocate the IP address if the address is a valid IP for any of the subnets on the specified network.
Parameter is an array, may be provided multiple times.
-
--hints <key=value>
— Admin-only. A dict, at the top level keyed by mechanism driver aliases (as defined in setup.cfg). To following values can be used to control Open vSwitch’s Userspace Tx packet steering feature:{"openvswitch": {"other_config": {"tx-steering": "hash"}}}
-{"openvswitch": {"other_config": {"tx-steering": "thread"}}}
If omitted the default is defined by Open vSwitch. The field cannot be longer than 4095 characters.
-
--mac-address <MAC_ADDRESS>
— The MAC address of the port. If unspecified, a MAC address is automatically generated -
--name <NAME>
— Human-readable name of the resource. Default is an empty string -
--network-id <NETWORK_ID>
— The ID of the attached network -
--numa-affinity-policy <NUMA_AFFINITY_POLICY>
— The port NUMA affinity policy requested during the virtual machine scheduling. Values:None
,required
,preferred
orlegacy
Possible values:
legacy
,preferred
,required
,socket
-
--port-security-enabled <PORT_SECURITY_ENABLED>
— The port security status. A valid value is enabled (true
) or disabled (false
). If port security is enabled for the port, security group rules and anti-spoofing rules are applied to the traffic on the port. If disabled, no such rules are appliedPossible values:
true
,false
-
--propagate-uplink-status <PROPAGATE_UPLINK_STATUS>
— The uplink status propagation of the port. Valid values are enabled (true
) and disabled (false
)Possible values:
true
,false
-
--qos-policy-id <QOS_POLICY_ID>
— QoS policy associated with the port -
--security-groups <SECURITY_GROUPS>
— The IDs of security groups applied to the port.Parameter is an array, may be provided multiple times.
-
--tags <TAGS>
— Parameter is an array, may be provided multiple times -
--tenant-id <TENANT_ID>
— The ID of the project that owns the resource. Only administrative and users with advsvc role can specify a project ID other than their own. You cannot change this value through authorization policies
osc network port delete
Deletes a port.
Any IP addresses that are associated with the port are returned to the respective subnets allocation pools.
Normal response codes: 204
Error response codes: 401, 403, 404, 412
Usage: osc network port delete <ID>
Arguments:
<ID>
— port_id parameter for /v2.0/ports/{port_id}/add_allowed_address_pairs API
osc network port list
Lists ports to which the user has access.
Default policy settings return only those ports that are owned by the project of the user who submits the request, unless the request is submitted by a user with administrative rights.
Standard query parameters are supported on the URI. For more information, see Filtering and Column Selection.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Pagination query parameters are supported if Neutron configuration supports it by overriding allow_pagination=false
. For more information, see Pagination.
Sorting query parameters are supported if Neutron configuration supports it with allow_sorting=true
. For more information, see Sorting.
If the ip-substring-filtering
extension is enabled, the Neutron API supports IP address substring filtering on the fixed_ips
attribute. If you specify an IP address substring (ip_address_substr
) in an entry of the fixed_ips
attribute, the Neutron API will list all ports that have an IP address matching the substring.
Normal response codes: 200
Error response codes: 401
Usage: osc network port list [OPTIONS]
Options:
-
--admin-state-up <ADMIN_STATE_UP>
— admin_state_up query parameter for /v2.0/ports APIPossible values:
true
,false
-
--binding-host-id <BINDING_HOST_ID>
— binding:host_id query parameter for /v2.0/ports API -
--description <DESCRIPTION>
— description query parameter for /v2.0/ports API -
--device-id <DEVICE_ID>
— device_id query parameter for /v2.0/ports API -
--device-owner <DEVICE_OWNER>
— device_owner query parameter for /v2.0/ports API -
--fixed-ips <FIXED_IPS>
— fixed_ips query parameter for /v2.0/ports API -
--id <ID>
— id query parameter for /v2.0/ports API -
--ip-allocation <IP_ALLOCATION>
— ip_allocation query parameter for /v2.0/ports API -
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--mac-address <MAC_ADDRESS>
— mac_address query parameter for /v2.0/ports API -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--name <NAME>
— name query parameter for /v2.0/ports API -
--network-id <NETWORK_ID>
— network_id query parameter for /v2.0/ports API -
--not-tags <NOT_TAGS>
— not-tags query parameter for /v2.0/ports API -
--not-tags-any <NOT_TAGS_ANY>
— not-tags-any query parameter for /v2.0/ports API -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--revision-number <REVISION_NUMBER>
— revision_number query parameter for /v2.0/ports API -
--security-groups <SECURITY_GROUPS>
— security_groups query parameter for /v2.0/ports API -
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--status <STATUS>
— status query parameter for /v2.0/ports API -
--tags <TAGS>
— tags query parameter for /v2.0/ports API -
--tags-any <TAGS_ANY>
— tags-any query parameter for /v2.0/ports API -
--tenant-id <TENANT_ID>
— tenant_id query parameter for /v2.0/ports API -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network port show
Shows details for a port.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Normal response codes: 200
Error response codes: 401, 404
Usage: osc network port show <ID>
Arguments:
<ID>
— port_id parameter for /v2.0/ports/{port_id}/add_allowed_address_pairs API
osc network port tag
Lists tags, creates, replaces or deletes one or more tags for a resource, checks the existence of a tag for a resource
Usage: osc network port tag <COMMAND>
Available subcommands:
osc network port tag add
— Command without description in OpenAPIosc network port tag check
— Command without description in OpenAPIosc network port tag delete
— Command without description in OpenAPIosc network port tag list
— Command without description in OpenAPIosc network port tag purge
— Command without description in OpenAPI
osc network port tag add
Command without description in OpenAPI
Usage: osc network port tag add <PORT_ID> <ID>
Arguments:
<PORT_ID>
— port_id parameter for /v2.0/ports/{port_id}/tags/{id} API<ID>
— id parameter for /v2.0/ports/{port_id}/tags/{id} API
osc network port tag check
Command without description in OpenAPI
Usage: osc network port tag check <PORT_ID> <ID>
Arguments:
<PORT_ID>
— port_id parameter for /v2.0/ports/{port_id}/tags/{id} API<ID>
— id parameter for /v2.0/ports/{port_id}/tags/{id} API
osc network port tag delete
Command without description in OpenAPI
Usage: osc network port tag delete <PORT_ID> <ID>
Arguments:
<PORT_ID>
— port_id parameter for /v2.0/ports/{port_id}/tags/{id} API<ID>
— id parameter for /v2.0/ports/{port_id}/tags/{id} API
osc network port tag list
Command without description in OpenAPI
Usage: osc network port tag list [OPTIONS] <PORT_ID>
Arguments:
<PORT_ID>
— port_id parameter for /v2.0/ports/{port_id}/tags/{id} API
Options:
-
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network port tag purge
Command without description in OpenAPI
Usage: osc network port tag purge <PORT_ID>
Arguments:
<PORT_ID>
— port_id parameter for /v2.0/ports/{port_id}/tags/{id} API
osc network qos
Quality of Service
Usage: osc network qos <COMMAND>
Available subcommands:
osc network qos alias-bandwidth-limit-rule
— Quality of Service rules alias APIosc network qos alias-dscp-marking-rule
— QoS DSCP marking rulesosc network qos alias-minimum-bandwidth-rule
— QoS minimum bandwidth rulesosc network qos alias-minimum-packet-rate-rule
— QoS minimum packet rate rulesosc network qos policy
— QoS minimum packet rate rulesosc network qos rule-type
— QoS rule types
osc network qos alias-bandwidth-limit-rule
Quality of Service rules alias API
The purpose of this API extension is to enable callers to execute the requests to delete, show and update QoS rules without specifying the corresponding policy ID. Otherwise, these requests have the exact same behavior as their counterparts described in other parts of this documentation. The requests available in this API extension are:
Usage: osc network qos alias-bandwidth-limit-rule <COMMAND>
Available subcommands:
osc network qos alias-bandwidth-limit-rule create
— Command without description in OpenAPIosc network qos alias-bandwidth-limit-rule delete
— Command without description in OpenAPIosc network qos alias-bandwidth-limit-rule list
— Command without description in OpenAPIosc network qos alias-bandwidth-limit-rule set
— Command without description in OpenAPIosc network qos alias-bandwidth-limit-rule show
— Command without description in OpenAPI
osc network qos alias-bandwidth-limit-rule create
Command without description in OpenAPI
Usage: osc network qos alias-bandwidth-limit-rule create [OPTIONS]
Options:
--alias-bandwidth-limit-rule <key=value>
osc network qos alias-bandwidth-limit-rule delete
Command without description in OpenAPI
Usage: osc network qos alias-bandwidth-limit-rule delete <ID>
Arguments:
<ID>
— id parameter for /v2.0/qos/alias-bandwidth-limit-rules/{id} API
osc network qos alias-bandwidth-limit-rule list
Command without description in OpenAPI
Usage: osc network qos alias-bandwidth-limit-rule list [OPTIONS]
Options:
-
--direction <DIRECTION>
— direction query parameter for /v2.0/qos/alias-bandwidth-limit-rules APIPossible values:
egress
,ingress
-
--id <ID>
— id query parameter for /v2.0/qos/alias-bandwidth-limit-rules API -
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--max-burst-kbps <MAX_BURST_KBPS>
— max_burst_kbps query parameter for /v2.0/qos/alias-bandwidth-limit-rules API -
--max-kbps <MAX_KBPS>
— max_kbps query parameter for /v2.0/qos/alias-bandwidth-limit-rules API -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network qos alias-bandwidth-limit-rule set
Command without description in OpenAPI
Usage: osc network qos alias-bandwidth-limit-rule set [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.0/qos/alias-bandwidth-limit-rules/{id} API
Options:
-
--direction <DIRECTION>
Possible values:
egress
,ingress
-
--max-burst-kbps <MAX_BURST_KBPS>
-
--max-kbps <MAX_KBPS>
osc network qos alias-bandwidth-limit-rule show
Command without description in OpenAPI
Usage: osc network qos alias-bandwidth-limit-rule show <ID>
Arguments:
<ID>
— id parameter for /v2.0/qos/alias-bandwidth-limit-rules/{id} API
osc network qos alias-dscp-marking-rule
QoS DSCP marking rules
Lists, creates, deletes, shows information for, and updates QoS DSCP marking rules.
Usage: osc network qos alias-dscp-marking-rule <COMMAND>
Available subcommands:
osc network qos alias-dscp-marking-rule create
— Command without description in OpenAPIosc network qos alias-dscp-marking-rule delete
— Command without description in OpenAPIosc network qos alias-dscp-marking-rule list
— Command without description in OpenAPIosc network qos alias-dscp-marking-rule set
— Command without description in OpenAPIosc network qos alias-dscp-marking-rule show
— Command without description in OpenAPI
osc network qos alias-dscp-marking-rule create
Command without description in OpenAPI
Usage: osc network qos alias-dscp-marking-rule create [OPTIONS]
Options:
--alias-dscp-marking-rule <key=value>
osc network qos alias-dscp-marking-rule delete
Command without description in OpenAPI
Usage: osc network qos alias-dscp-marking-rule delete <ID>
Arguments:
<ID>
— id parameter for /v2.0/qos/alias-dscp-marking-rules/{id} API
osc network qos alias-dscp-marking-rule list
Command without description in OpenAPI
Usage: osc network qos alias-dscp-marking-rule list [OPTIONS]
Options:
-
--dscp-mark <DSCP_MARK>
— dscp_mark query parameter for /v2.0/qos/alias-dscp-marking-rules API -
--id <ID>
— id query parameter for /v2.0/qos/alias-dscp-marking-rules API -
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network qos alias-dscp-marking-rule set
Command without description in OpenAPI
Usage: osc network qos alias-dscp-marking-rule set [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.0/qos/alias-dscp-marking-rules/{id} API
Options:
--dscp-mark <DSCP_MARK>
osc network qos alias-dscp-marking-rule show
Command without description in OpenAPI
Usage: osc network qos alias-dscp-marking-rule show <ID>
Arguments:
<ID>
— id parameter for /v2.0/qos/alias-dscp-marking-rules/{id} API
osc network qos alias-minimum-bandwidth-rule
QoS minimum bandwidth rules
Lists, creates, deletes, shows information for, and updates QoS minimum bandwidth rules.
Usage: osc network qos alias-minimum-bandwidth-rule <COMMAND>
Available subcommands:
osc network qos alias-minimum-bandwidth-rule create
— Command without description in OpenAPIosc network qos alias-minimum-bandwidth-rule delete
— Command without description in OpenAPIosc network qos alias-minimum-bandwidth-rule list
— Command without description in OpenAPIosc network qos alias-minimum-bandwidth-rule set
— Command without description in OpenAPIosc network qos alias-minimum-bandwidth-rule show
— Command without description in OpenAPI
osc network qos alias-minimum-bandwidth-rule create
Command without description in OpenAPI
Usage: osc network qos alias-minimum-bandwidth-rule create [OPTIONS]
Options:
--alias-minimum-bandwidth-rule <key=value>
osc network qos alias-minimum-bandwidth-rule delete
Command without description in OpenAPI
Usage: osc network qos alias-minimum-bandwidth-rule delete <ID>
Arguments:
<ID>
— id parameter for /v2.0/qos/alias-minimum-bandwidth-rules/{id} API
osc network qos alias-minimum-bandwidth-rule list
Command without description in OpenAPI
Usage: osc network qos alias-minimum-bandwidth-rule list [OPTIONS]
Options:
-
--direction <DIRECTION>
— direction query parameter for /v2.0/qos/alias-minimum-bandwidth-rules APIPossible values:
egress
,ingress
-
--id <ID>
— id query parameter for /v2.0/qos/alias-minimum-bandwidth-rules API -
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--min-kbps <MIN_KBPS>
— min_kbps query parameter for /v2.0/qos/alias-minimum-bandwidth-rules API -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network qos alias-minimum-bandwidth-rule set
Command without description in OpenAPI
Usage: osc network qos alias-minimum-bandwidth-rule set [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.0/qos/alias-minimum-bandwidth-rules/{id} API
Options:
-
--direction <DIRECTION>
Possible values:
egress
,ingress
-
--min-kbps <MIN_KBPS>
osc network qos alias-minimum-bandwidth-rule show
Command without description in OpenAPI
Usage: osc network qos alias-minimum-bandwidth-rule show <ID>
Arguments:
<ID>
— id parameter for /v2.0/qos/alias-minimum-bandwidth-rules/{id} API
osc network qos alias-minimum-packet-rate-rule
QoS minimum packet rate rules
Lists, creates, deletes, shows information for, and updates QoS minimum packet rate rules.
Usage: osc network qos alias-minimum-packet-rate-rule <COMMAND>
Available subcommands:
osc network qos alias-minimum-packet-rate-rule create
— Command without description in OpenAPIosc network qos alias-minimum-packet-rate-rule delete
— Command without description in OpenAPIosc network qos alias-minimum-packet-rate-rule list
— Command without description in OpenAPIosc network qos alias-minimum-packet-rate-rule set
— Command without description in OpenAPIosc network qos alias-minimum-packet-rate-rule show
— Command without description in OpenAPI
osc network qos alias-minimum-packet-rate-rule create
Command without description in OpenAPI
Usage: osc network qos alias-minimum-packet-rate-rule create [OPTIONS]
Options:
--alias-minimum-packet-rate-rule <key=value>
osc network qos alias-minimum-packet-rate-rule delete
Command without description in OpenAPI
Usage: osc network qos alias-minimum-packet-rate-rule delete <ID>
Arguments:
<ID>
— id parameter for /v2.0/qos/alias-minimum-packet-rate-rules/{id} API
osc network qos alias-minimum-packet-rate-rule list
Command without description in OpenAPI
Usage: osc network qos alias-minimum-packet-rate-rule list [OPTIONS]
Options:
-
--direction <DIRECTION>
— direction query parameter for /v2.0/qos/alias-minimum-packet-rate-rules APIPossible values:
any
,egress
,ingress
-
--id <ID>
— id query parameter for /v2.0/qos/alias-minimum-packet-rate-rules API -
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--min-kpps <MIN_KPPS>
— min_kpps query parameter for /v2.0/qos/alias-minimum-packet-rate-rules API -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network qos alias-minimum-packet-rate-rule set
Command without description in OpenAPI
Usage: osc network qos alias-minimum-packet-rate-rule set [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.0/qos/alias-minimum-packet-rate-rules/{id} API
Options:
-
--direction <DIRECTION>
Possible values:
any
,egress
,ingress
-
--min-kpps <MIN_KPPS>
osc network qos alias-minimum-packet-rate-rule show
Command without description in OpenAPI
Usage: osc network qos alias-minimum-packet-rate-rule show <ID>
Arguments:
<ID>
— id parameter for /v2.0/qos/alias-minimum-packet-rate-rules/{id} API
osc network qos policy
QoS minimum packet rate rules
Lists, creates, deletes, shows information for, and updates QoS minimum packet rate rules.
Usage: osc network qos policy <COMMAND>
Available subcommands:
osc network qos policy bandwidth-limit-rule
— QoS bandwidth limit rulesosc network qos policy create
— Create QoS policyosc network qos policy delete
— Delete QoS policyosc network qos policy dscp-marking-rule
— QoS DSCP marking rulesosc network qos policy list
— List QoS policiesosc network qos policy minimum-bandwidth-rule
— QoS minimum bandwidth rulesosc network qos policy minimum-packet-rate-rule
— QoS minimum packet rate rulesosc network qos policy set
— Update QoS policyosc network qos policy show
— Show QoS policy details
osc network qos policy bandwidth-limit-rule
QoS bandwidth limit rules
Lists, creates, deletes, shows information for, and updates QoS bandwidth limit rules.
Bandwidth limit direction
The qos-bw-limit-direction extension adds the direction attribute to QoS rule types. The direction attribute allows to configure QoS bandwidth limit rule with specific direction: ingress or egress. Default is egress.
Usage: osc network qos policy bandwidth-limit-rule <COMMAND>
Available subcommands:
osc network qos policy bandwidth-limit-rule create
— Create bandwidth limit ruleosc network qos policy bandwidth-limit-rule delete
— Delete bandwidth limit ruleosc network qos policy bandwidth-limit-rule list
— List bandwidth limit rules for QoS policyosc network qos policy bandwidth-limit-rule set
— Update bandwidth limit ruleosc network qos policy bandwidth-limit-rule show
— Show bandwidth limit rule details
osc network qos policy bandwidth-limit-rule create
Creates a bandwidth limit rule for a QoS policy.
Normal response codes: 201
Error response codes: 400, 401, 404, 409
Usage: osc network qos policy bandwidth-limit-rule create [OPTIONS] <POLICY_ID>
Arguments:
<POLICY_ID>
— policy_id parameter for /v2.0/qos/policies/{policy_id}/bandwidth_limit_rules/{id} API
Options:
-
--direction <DIRECTION>
— The direction of the traffic to which the QoS rule is applied, as seen from the point of view of theport
. Valid values areegress
andingress
. Default value isegress
Possible values:
egress
,ingress
-
--max-burst-kbps <MAX_BURST_KBPS>
— The maximum burst size (in kilobits). Default is0
-
--max-kbps <MAX_KBPS>
— The maximum KBPS (kilobits per second) value. If you specify this value, must be greater than 0 otherwise max_kbps will have no value
osc network qos policy bandwidth-limit-rule delete
Deletes a bandwidth limit rule for a QoS policy.
Normal response codes: 204
Error response codes: 400, 401, 404
Usage: osc network qos policy bandwidth-limit-rule delete <POLICY_ID> <ID>
Arguments:
<POLICY_ID>
— policy_id parameter for /v2.0/qos/policies/{policy_id}/bandwidth_limit_rules/{id} API<ID>
— id parameter for /v2.0/qos/policies/{policy_id}/bandwidth_limit_rules/{id} API
osc network qos policy bandwidth-limit-rule list
Lists all bandwidth limit rules for a QoS policy.
The list might be empty.
Standard query parameters are supported on the URI. For more information, see Filtering and Column Selection.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Pagination query parameters are supported if Neutron configuration supports it by overriding allow_pagination=false
. For more information, see Pagination.
Sorting query parameters are supported if Neutron configuration supports it with allow_sorting=true
. For more information, see Sorting.
Normal response codes: 200
Error response codes: 401, 404
Usage: osc network qos policy bandwidth-limit-rule list [OPTIONS] <POLICY_ID>
Arguments:
<POLICY_ID>
— policy_id parameter for /v2.0/qos/policies/{policy_id}/bandwidth_limit_rules/{id} API
Options:
-
--id <ID>
— id query parameter for /v2.0/qos/policies/{policy_id}/bandwidth_limit_rules API -
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--max-burst-kbps <MAX_BURST_KBPS>
— max_burst_kbps query parameter for /v2.0/qos/policies/{policy_id}/bandwidth_limit_rules API -
--max-kbps <MAX_KBPS>
— max_kbps query parameter for /v2.0/qos/policies/{policy_id}/bandwidth_limit_rules API -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network qos policy bandwidth-limit-rule set
Updates a bandwidth limit rule for a QoS policy.
Normal response codes: 200
Error response codes: 400, 401, 404
Usage: osc network qos policy bandwidth-limit-rule set [OPTIONS] <POLICY_ID> <ID>
Arguments:
<POLICY_ID>
— policy_id parameter for /v2.0/qos/policies/{policy_id}/bandwidth_limit_rules/{id} API<ID>
— id parameter for /v2.0/qos/policies/{policy_id}/bandwidth_limit_rules/{id} API
Options:
-
--direction <DIRECTION>
— The direction of the traffic to which the QoS rule is applied, as seen from the point of view of theport
. Valid values areegress
andingress
Possible values:
egress
,ingress
-
--max-burst-kbps <MAX_BURST_KBPS>
— The maximum burst size (in kilobits). Default is0
-
--max-kbps <MAX_KBPS>
— The maximum KBPS (kilobits per second) value. If you specify this value, must be greater than 0 otherwise max_kbps will have no value
osc network qos policy bandwidth-limit-rule show
Shows details for a bandwidth limit rule for a QoS policy.
Normal response codes: 200
Error response codes: 401, 404
Usage: osc network qos policy bandwidth-limit-rule show <POLICY_ID> <ID>
Arguments:
<POLICY_ID>
— policy_id parameter for /v2.0/qos/policies/{policy_id}/bandwidth_limit_rules/{id} API<ID>
— id parameter for /v2.0/qos/policies/{policy_id}/bandwidth_limit_rules/{id} API
osc network qos policy create
Creates a QoS policy.
Creates a QoS policy by using the configuration that you define in the request object. A response object is returned. The object contains a unique ID.
By the default policy configuration, if the caller is not an administrative user, this call returns the HTTP Forbidden (403)
response code.
Users with an administrative role can create policies on behalf of other projects by specifying a project ID that is different than their own.
Normal response codes: 201
Error response codes: 401, 403, 404, 409
Usage: osc network qos policy create [OPTIONS]
Options:
-
--description <DESCRIPTION>
— A human-readable description for the resource. Default is an empty string -
--is-default <IS_DEFAULT>
— Iftrue
, the QoSpolicy
is the default policyPossible values:
true
,false
-
--name <NAME>
— Human-readable name of the resource -
--shared <SHARED>
— Set totrue
to share this policy with other projects. Default isfalse
Possible values:
true
,false
-
--tenant-id <TENANT_ID>
— The ID of the project that owns the resource. Only administrative and users with advsvc role can specify a project ID other than their own. You cannot change this value through authorization policies
osc network qos policy delete
Deletes a QoS policy.
Normal response codes: 204
Error response codes: 400, 401, 404, 412
Usage: osc network qos policy delete <ID>
Arguments:
<ID>
— id parameter for /v2.0/qos/policies/{id} API
osc network qos policy dscp-marking-rule
QoS DSCP marking rules
Lists, creates, deletes, shows information for, and updates QoS DSCP marking rules.
Usage: osc network qos policy dscp-marking-rule <COMMAND>
Available subcommands:
osc network qos policy dscp-marking-rule create
— Create DSCP marking ruleosc network qos policy dscp-marking-rule delete
— Delete DSCP marking ruleosc network qos policy dscp-marking-rule list
— List DSCP marking rules for QoS policyosc network qos policy dscp-marking-rule set
— Update DSCP marking ruleosc network qos policy dscp-marking-rule show
— Show DSCP marking rule details
osc network qos policy dscp-marking-rule create
Creates a DSCP marking rule for a QoS policy.
Normal response codes: 201
Error response codes: 400, 401, 404, 409
Usage: osc network qos policy dscp-marking-rule create [OPTIONS] <POLICY_ID>
Arguments:
<POLICY_ID>
— policy_id parameter for /v2.0/qos/policies/{policy_id}/dscp_marking_rules/{id} API
Options:
--dscp-mark <DSCP_MARK>
— The DSCP mark value
osc network qos policy dscp-marking-rule delete
Deletes a DSCP marking rule for a QoS policy.
Normal response codes: 204
Error response codes: 400, 401, 404
Usage: osc network qos policy dscp-marking-rule delete <POLICY_ID> <ID>
Arguments:
<POLICY_ID>
— policy_id parameter for /v2.0/qos/policies/{policy_id}/dscp_marking_rules/{id} API<ID>
— id parameter for /v2.0/qos/policies/{policy_id}/dscp_marking_rules/{id} API
osc network qos policy dscp-marking-rule list
Lists all DSCP marking rules for a QoS policy.
The list may be empty.
Standard query parameters are supported on the URI. For more information, see Filtering and Column Selection.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Pagination query parameters are supported if Neutron configuration supports it by overriding allow_pagination=false
. For more information, see Pagination.
Sorting query parameters are supported if Neutron configuration supports it with allow_sorting=true
. For more information, see Sorting.
Normal response codes: 200
Error response codes: 401, 404
Usage: osc network qos policy dscp-marking-rule list [OPTIONS] <POLICY_ID>
Arguments:
<POLICY_ID>
— policy_id parameter for /v2.0/qos/policies/{policy_id}/dscp_marking_rules/{id} API
Options:
-
--dscp-mark <DSCP_MARK>
— dscp_mark query parameter for /v2.0/qos/policies/{policy_id}/dscp_marking_rules API -
--id <ID>
— id query parameter for /v2.0/qos/policies/{policy_id}/dscp_marking_rules API -
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network qos policy dscp-marking-rule set
Updates a DSCP marking rule for a QoS policy.
Normal response codes: 200
Error response codes: 400, 401, 404
Usage: osc network qos policy dscp-marking-rule set [OPTIONS] <POLICY_ID> <ID>
Arguments:
<POLICY_ID>
— policy_id parameter for /v2.0/qos/policies/{policy_id}/dscp_marking_rules/{id} API<ID>
— id parameter for /v2.0/qos/policies/{policy_id}/dscp_marking_rules/{id} API
Options:
--dscp-mark <DSCP_MARK>
— The DSCP mark value
osc network qos policy dscp-marking-rule show
Shows details for a DSCP marking rule for a QoS policy.
Normal response codes: 200
Error response codes: 401, 404
Usage: osc network qos policy dscp-marking-rule show <POLICY_ID> <ID>
Arguments:
<POLICY_ID>
— policy_id parameter for /v2.0/qos/policies/{policy_id}/dscp_marking_rules/{id} API<ID>
— id parameter for /v2.0/qos/policies/{policy_id}/dscp_marking_rules/{id} API
osc network qos policy list
Lists all QoS policies associated with your project. One policy can contain more than one rule type.
The list might be empty.
Standard query parameters are supported on the URI. For more information, see Filtering and Column Selection.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Pagination query parameters are supported if Neutron configuration supports it by overriding allow_pagination=false
. For more information, see Pagination.
Sorting query parameters are supported if Neutron configuration supports it with allow_sorting=true
. For more information, see Sorting.
Normal response codes: 200
Error response codes: 401
Usage: osc network qos policy list [OPTIONS]
Options:
-
--description <DESCRIPTION>
— description query parameter for /v2.0/qos/policies API -
--id <ID>
— id query parameter for /v2.0/qos/policies API -
--is-default <IS_DEFAULT>
— is_default query parameter for /v2.0/qos/policies APIPossible values:
true
,false
-
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--name <NAME>
— name query parameter for /v2.0/qos/policies API -
--not-tags <NOT_TAGS>
— not-tags query parameter for /v2.0/qos/policies API -
--not-tags-any <NOT_TAGS_ANY>
— not-tags-any query parameter for /v2.0/qos/policies API -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--revision-number <REVISION_NUMBER>
— revision_number query parameter for /v2.0/qos/policies API -
--shared <SHARED>
— shared query parameter for /v2.0/qos/policies APIPossible values:
true
,false
-
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--tags <TAGS>
— tags query parameter for /v2.0/qos/policies API -
--tags-any <TAGS_ANY>
— tags-any query parameter for /v2.0/qos/policies API -
--tenant-id <TENANT_ID>
— tenant_id query parameter for /v2.0/qos/policies API -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network qos policy minimum-bandwidth-rule
QoS minimum bandwidth rules
Lists, creates, deletes, shows information for, and updates QoS minimum bandwidth rules.
Usage: osc network qos policy minimum-bandwidth-rule <COMMAND>
Available subcommands:
osc network qos policy minimum-bandwidth-rule create
— Create minimum bandwidth ruleosc network qos policy minimum-bandwidth-rule delete
— Delete minimum bandwidth ruleosc network qos policy minimum-bandwidth-rule list
— List minimum bandwidth rules for QoS policyosc network qos policy minimum-bandwidth-rule set
— Update minimum bandwidth ruleosc network qos policy minimum-bandwidth-rule show
— Show minimum bandwidth rule details
osc network qos policy minimum-bandwidth-rule create
Creates a minimum bandwidth rule for a QoS policy.
Normal response codes: 201
Error response codes: 400, 401, 404, 409
Usage: osc network qos policy minimum-bandwidth-rule create [OPTIONS] <POLICY_ID>
Arguments:
<POLICY_ID>
— policy_id parameter for /v2.0/qos/policies/{policy_id}/minimum_bandwidth_rules/{id} API
Options:
-
--direction <DIRECTION>
— The direction of the traffic to which the QoS rule is applied, as seen from the point of view of theport
. Valid values areegress
andingress
. Default value isegress
Possible values:
egress
,ingress
-
--min-kbps <MIN_KBPS>
— The minimum KBPS (kilobits per second) value which should be available for port
osc network qos policy minimum-bandwidth-rule delete
Deletes a minimum bandwidth rule for a QoS policy.
Normal response codes: 204
Error response codes: 400, 401, 404
Usage: osc network qos policy minimum-bandwidth-rule delete <POLICY_ID> <ID>
Arguments:
<POLICY_ID>
— policy_id parameter for /v2.0/qos/policies/{policy_id}/minimum_bandwidth_rules/{id} API<ID>
— id parameter for /v2.0/qos/policies/{policy_id}/minimum_bandwidth_rules/{id} API
osc network qos policy minimum-bandwidth-rule list
Lists all minimum bandwidth rules for a QoS policy.
The list might be empty.
Standard query parameters are supported on the URI. For more information, see Filtering and Column Selection.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Pagination query parameters are supported if Neutron configuration supports it by overriding allow_pagination=false
. For more information, see Pagination.
Sorting query parameters are supported if Neutron configuration supports it with allow_sorting=true
. For more information, see Sorting.
Normal response codes: 200
Error response codes: 401, 404
Usage: osc network qos policy minimum-bandwidth-rule list [OPTIONS] <POLICY_ID>
Arguments:
<POLICY_ID>
— policy_id parameter for /v2.0/qos/policies/{policy_id}/minimum_bandwidth_rules/{id} API
Options:
-
--direction <DIRECTION>
— direction query parameter for /v2.0/qos/policies/{policy_id}/minimum_bandwidth_rules APIPossible values:
egress
,ingress
-
--id <ID>
— id query parameter for /v2.0/qos/policies/{policy_id}/minimum_bandwidth_rules API -
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--min-kbps <MIN_KBPS>
— min_kbps query parameter for /v2.0/qos/policies/{policy_id}/minimum_bandwidth_rules API -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network qos policy minimum-bandwidth-rule set
Updates a minimum bandwidth rule for a QoS policy.
Note that the rule cannot be updated, and the update is rejected with error code 501, if there is any bound port referring to the rule via the qos policy.
Normal response codes: 200
Error response codes: 400, 401, 404, 501
Usage: osc network qos policy minimum-bandwidth-rule set [OPTIONS] <POLICY_ID> <ID>
Arguments:
<POLICY_ID>
— policy_id parameter for /v2.0/qos/policies/{policy_id}/minimum_bandwidth_rules/{id} API<ID>
— id parameter for /v2.0/qos/policies/{policy_id}/minimum_bandwidth_rules/{id} API
Options:
-
--direction <DIRECTION>
— The direction of the traffic to which the QoS rule is applied, as seen from the point of view of theport
. Valid values areegress
andingress
Possible values:
egress
,ingress
-
--min-kbps <MIN_KBPS>
— The minimum KBPS (kilobits per second) value which should be available for port
osc network qos policy minimum-bandwidth-rule show
Shows details for a minimum bandwidth rule for a QoS policy.
Normal response codes: 200
Error response codes: 401, 404
Usage: osc network qos policy minimum-bandwidth-rule show <POLICY_ID> <ID>
Arguments:
<POLICY_ID>
— policy_id parameter for /v2.0/qos/policies/{policy_id}/minimum_bandwidth_rules/{id} API<ID>
— id parameter for /v2.0/qos/policies/{policy_id}/minimum_bandwidth_rules/{id} API
osc network qos policy minimum-packet-rate-rule
QoS minimum packet rate rules
Lists, creates, deletes, shows information for, and updates QoS minimum packet rate rules.
Usage: osc network qos policy minimum-packet-rate-rule <COMMAND>
Available subcommands:
osc network qos policy minimum-packet-rate-rule create
— Command without description in OpenAPIosc network qos policy minimum-packet-rate-rule delete
— Command without description in OpenAPIosc network qos policy minimum-packet-rate-rule list
— Command without description in OpenAPIosc network qos policy minimum-packet-rate-rule set
— Command without description in OpenAPIosc network qos policy minimum-packet-rate-rule show
— Command without description in OpenAPI
osc network qos policy minimum-packet-rate-rule create
Command without description in OpenAPI
Usage: osc network qos policy minimum-packet-rate-rule create [OPTIONS] <POLICY_ID>
Arguments:
<POLICY_ID>
— policy_id parameter for /v2.0/qos/policies/{policy_id}/minimum-packet-rate-rules/{id} API
Options:
-
--direction <DIRECTION>
Possible values:
any
,egress
,ingress
-
--min-kpps <MIN_KPPS>
osc network qos policy minimum-packet-rate-rule delete
Command without description in OpenAPI
Usage: osc network qos policy minimum-packet-rate-rule delete <POLICY_ID> <ID>
Arguments:
<POLICY_ID>
— policy_id parameter for /v2.0/qos/policies/{policy_id}/minimum-packet-rate-rules/{id} API<ID>
— id parameter for /v2.0/qos/policies/{policy_id}/minimum-packet-rate-rules/{id} API
osc network qos policy minimum-packet-rate-rule list
Command without description in OpenAPI
Usage: osc network qos policy minimum-packet-rate-rule list [OPTIONS] <POLICY_ID>
Arguments:
<POLICY_ID>
— policy_id parameter for /v2.0/qos/policies/{policy_id}/minimum-packet-rate-rules/{id} API
Options:
-
--direction <DIRECTION>
— direction query parameter for /v2.0/qos/policies/{policy_id}/minimum-packet-rate-rules APIPossible values:
any
,egress
,ingress
-
--id <ID>
— id query parameter for /v2.0/qos/policies/{policy_id}/minimum-packet-rate-rules API -
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--min-kpps <MIN_KPPS>
— min_kpps query parameter for /v2.0/qos/policies/{policy_id}/minimum-packet-rate-rules API -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network qos policy minimum-packet-rate-rule set
Command without description in OpenAPI
Usage: osc network qos policy minimum-packet-rate-rule set [OPTIONS] <POLICY_ID> <ID>
Arguments:
<POLICY_ID>
— policy_id parameter for /v2.0/qos/policies/{policy_id}/minimum-packet-rate-rules/{id} API<ID>
— id parameter for /v2.0/qos/policies/{policy_id}/minimum-packet-rate-rules/{id} API
Options:
-
--direction <DIRECTION>
Possible values:
any
,egress
,ingress
-
--min-kpps <MIN_KPPS>
osc network qos policy minimum-packet-rate-rule show
Command without description in OpenAPI
Usage: osc network qos policy minimum-packet-rate-rule show <POLICY_ID> <ID>
Arguments:
<POLICY_ID>
— policy_id parameter for /v2.0/qos/policies/{policy_id}/minimum-packet-rate-rules/{id} API<ID>
— id parameter for /v2.0/qos/policies/{policy_id}/minimum-packet-rate-rules/{id} API
osc network qos policy set
Updates a QoS policy.
Normal response codes: 200
Error response codes: 400, 401, 404, 412
Usage: osc network qos policy set [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.0/qos/policies/{id} API
Options:
-
--description <DESCRIPTION>
— A human-readable description for the resource. Default is an empty string -
--is-default <IS_DEFAULT>
— Iftrue
, the QoSpolicy
is the default policyPossible values:
true
,false
-
--name <NAME>
— Human-readable name of the resource -
--shared <SHARED>
— Set totrue
to share this policy with other projects. Default isfalse
Possible values:
true
,false
osc network qos policy show
Shows details for a QoS policy. One policy can contain more than one rule type.
Normal response codes: 200
Error response codes: 401, 404
Usage: osc network qos policy show <ID>
Arguments:
<ID>
— id parameter for /v2.0/qos/policies/{id} API
osc network qos rule-type
QoS rule types
Lists and shows information for QoS rule types available in current deployment.
Rule type details
The qos-rule-type-details extension adds the drivers attribute to QoS rule types. The drivers attribute’s value is a list of driver objects. Each driver object represents a loaded backend QoS driver and includes the driver’s name as well as a list of its supported_parameters and acceptable values.
Usage: osc network qos rule-type <COMMAND>
Available subcommands:
osc network qos rule-type list
— List QoS rule typesosc network qos rule-type show
— Show QoS rule type details
osc network qos rule-type list
Lists available qos rule types.
Standard query parameters are supported on the URI. For more information, see Filtering and Column Selection.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Pagination query parameters are supported if Neutron configuration supports it by overriding allow_pagination=false
. For more information, see Pagination.
Sorting query parameters are supported if Neutron configuration supports it with allow_sorting=true
. For more information, see Sorting.
Normal response codes: 200
Error response codes: 401
Usage: osc network qos rule-type list [OPTIONS]
Options:
-
--all-rules <ALL_RULES>
— all_rules query parameter for /v2.0/qos/rule-types APIPossible values:
true
,false
-
--all-supported <ALL_SUPPORTED>
— all_supported query parameter for /v2.0/qos/rule-types APIPossible values:
true
,false
-
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network qos rule-type show
Shows details for an available QoS rule type.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Normal response codes: 200
Error response codes: 401, 404
Usage: osc network qos rule-type show <ID>
Arguments:
<ID>
— id parameter for /v2.0/qos/rule-types/{id} API
osc network quota
Quotas extension (quotas)
Lists default quotas, current quotas for projects with non-default quota values, and shows, updates, and resets quotas for a project.
A quota value of -1 means that quota has no limit.
Usage: osc network quota <COMMAND>
Available subcommands:
osc network quota defaults
— List default quotas for a projectosc network quota delete
— Reset quota for a projectosc network quota details
— Command without description in OpenAPIosc network quota list
— List quotas for projects with non-default quota valuesosc network quota set
— Update quota for a projectosc network quota show
— List quotas for a project
osc network quota defaults
Lists default quotas for a project.
Standard query parameters are supported on the URI. For more information, see Filtering and Column Selection.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Pagination query parameters are supported if Neutron configuration supports it by overriding allow_pagination=false
. For more information, see Pagination.
Sorting query parameters are supported if Neutron configuration supports it with allow_sorting=true
. For more information, see Sorting.
Normal response codes: 200
Error response codes: 401, 403
Usage: osc network quota defaults <ID>
Arguments:
<ID>
— id parameter for /v2.0/quotas/{id}/default API
osc network quota delete
Resets quotas to default values for a project.
Normal response codes: 204
Error response codes: 401, 403, 404
Usage: osc network quota delete <ID>
Arguments:
<ID>
— id parameter for /v2.0/quotas/{id} API
osc network quota details
Command without description in OpenAPI
Usage: osc network quota details <ID>
Arguments:
<ID>
— id parameter for /v2.0/quotas/{id}/details API
osc network quota list
Lists quotas for projects with non-default quota values.
Standard query parameters are supported on the URI. For more information, see Filtering and Column Selection.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Pagination query parameters are supported if Neutron configuration supports it by overriding allow_pagination=false
. For more information, see Pagination.
Sorting query parameters are supported if Neutron configuration supports it with allow_sorting=true
. For more information, see Sorting.
Normal response codes: 200
Error response codes: 401, 403
Usage: osc network quota list [OPTIONS]
Options:
-
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network quota set
Updates quotas for a project. Use when non-default quotas are desired.
Normal response codes: 200
Error response codes: 401, 403
Usage: osc network quota set [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.0/quotas/{id} API
Options:
--floatingip <FLOATINGIP>
— The number of floating IP addresses allowed for each project. A value of-1
means no limit--network <NETWORK>
— The number of networks allowed for each project. A value of-1
means no limit--port <PORT>
— The number of ports allowed for each project. A value of-1
means no limit--project-id <PROJECT_ID>
— The ID of the project--rbac-policy <RBAC_POLICY>
— The number of role-based access control (RBAC) policies for each project. A value of-1
means no limit--router <ROUTER>
— The number of routers allowed for each project. A value of-1
means no limit--security-group <SECURITY_GROUP>
— The number of security groups allowed for each project. A value of-1
means no limit--security-group-rule <SECURITY_GROUP_RULE>
— The number of security group rules allowed for each project. A value of-1
means no limit--subnet <SUBNET>
— The number of subnets allowed for each project. A value of-1
means no limit--subnetpool <SUBNETPOOL>
— The number of subnet pools allowed for each project. A value of-1
means no limit
osc network quota show
Lists quotas for a project.
Standard query parameters are supported on the URI. For more information, see Filtering and Column Selection.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Pagination query parameters are supported if Neutron configuration supports it by overriding allow_pagination=false
. For more information, see Pagination.
Sorting query parameters are supported if Neutron configuration supports it with allow_sorting=true
. For more information, see Sorting.
Normal response codes: 200
Error response codes: 401, 403
Usage: osc network quota show <ID>
Arguments:
<ID>
— id parameter for /v2.0/quotas/{id} API
osc network rbac-policy
RbacPolicy commands
Usage: osc network rbac-policy <COMMAND>
Available subcommands:
osc network rbac-policy create
— Create RBAC policyosc network rbac-policy delete
— Delete RBAC policyosc network rbac-policy list
— List RBAC policiesosc network rbac-policy set
— Update RBAC policyosc network rbac-policy show
— Show RBAC policy details
osc network rbac-policy create
Create RBAC policy for given tenant.
Normal response codes: 201
Error response codes: 400, 401
Usage: osc network rbac-policy create [OPTIONS]
Options:
--action <ACTION>
— Action for the RBAC policy which isaccess_as_external
oraccess_as_shared
--object-id <OBJECT_ID>
— The ID of theobject_type
resource. Anobject_type
ofnetwork
returns a network ID, anobject_type
ofqos-policy
returns a QoS policy ID, anobject_type
ofsecurity-group
returns a security group ID, anobject_type
ofaddress-scope
returns a address scope ID, anobject_type
ofsubnetpool
returns a subnetpool ID and anobject_type
ofaddress-group
returns an address group ID--object-type <OBJECT_TYPE>
— The type of the object that the RBAC policy affects. Types includeqos-policy
,network
,security-group
,address-scope
,subnetpool
oraddress-group
--target-tenant <TARGET_TENANT>
— The ID of the tenant to which the RBAC policy will be enforced. Please note that Neutron does not perform any type of validation that the value provided is actually the ID of the existing project. If, for example, the name of the project is provided here, it will be accepted by the Neutron API, but the RBAC rule created will not work as expected--tenant-id <TENANT_ID>
osc network rbac-policy delete
Delete an RBAC policy.
Normal response codes: 204
Error response codes: 401, 404, 409
Usage: osc network rbac-policy delete <ID>
Arguments:
<ID>
— id parameter for /v2.0/rbac-policies/{id} API
osc network rbac-policy list
List RBAC policies that belong to a given tenant.
Standard query parameters are supported on the URI. For more information, see Filtering and Column Selection.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Pagination query parameters are supported if Neutron configuration supports it by overriding allow_pagination=false
. For more information, see Pagination.
Sorting query parameters are supported if Neutron configuration supports it with allow_sorting=true
. For more information, see Sorting.
Normal response codes: 200
Error response codes: 401
Usage: osc network rbac-policy list [OPTIONS]
Options:
-
--action <ACTION>
— action query parameter for /v2.0/rbac-policies API -
--id <ID>
— id query parameter for /v2.0/rbac-policies API -
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--object-id <OBJECT_ID>
— object_id query parameter for /v2.0/rbac-policies API -
--object-type <OBJECT_TYPE>
— object_type query parameter for /v2.0/rbac-policies API -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--target-tenant <TARGET_TENANT>
— target_tenant query parameter for /v2.0/rbac-policies API -
--tenant-id <TENANT_ID>
— tenant_id query parameter for /v2.0/rbac-policies API -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network rbac-policy set
Update RBAC policy for given tenant.
Normal response codes: 200
Error response codes: 400, 401, 403, 404
Usage: osc network rbac-policy set [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.0/rbac-policies/{id} API
Options:
--target-tenant <TARGET_TENANT>
— The ID of the tenant to which the RBAC policy will be enforced. Please note that Neutron does not perform any type of validation that the value provided is actually the ID of the existing project. If, for example, the name of the project is provided here, it will be accepted by the Neutron API, but the RBAC rule created will not work as expected
osc network rbac-policy show
Show details for a given RBAC policy.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Normal response codes: 200
Error response codes: 401, 404
Usage: osc network rbac-policy show <ID>
Arguments:
<ID>
— id parameter for /v2.0/rbac-policies/{id} API
osc network router
Router commands
Usage: osc network router <COMMAND>
Available subcommands:
osc network router add-external-gateway
— Add external gateways to routerosc network router add-extraroute
— Add extra routes to routerosc network router add-router-interface
— Add interface to routerosc network router conntrack-helper
— Lists, creates, shows details for, updates, and deletes router conntrack helper (CT) target rulesosc network router create
— Create routerosc network router delete
— Delete routerosc network router l3-agent
— L3 agent schedulerosc network router list
— List routersosc network router remove-external-gateway
— Remove external gateways from routerosc network router remove-extraroute
— Remove extra routes from routerosc network router remove-router-interface
— Remove interface from routerosc network router set
— Update routerosc network router show
— Show router detailsosc network router tag
— Lists tags, creates, replaces or deletes one or more tags for a resource, checks the existence of a tag for a resource
osc network router add-external-gateway
Add external gateways to router
Usage: osc network router add-external-gateway [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.0/routers/{id} API
Options:
-
--external-gateways <JSON>
— The list of external gateways of the router.Parameter is an array, may be provided multiple times.
osc network router add-extraroute
Add extra routes to router
Usage: osc network router add-extraroute [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.0/routers/{id} API
Options:
-
--routes <JSON>
— The extra routes configuration for L3 router. A list of dictionaries withdestination
andnexthop
parameters. It is available whenextraroute
extension is enabled.Parameter is an array, may be provided multiple times.
osc network router add-router-interface
Add interface to router
Usage: osc network router add-router-interface [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.0/routers/{id} API
Options:
--port-id <PORT_ID>
— The ID of the port. One of subnet_id or port_id must be specified--subnet-id <SUBNET_ID>
— The ID of the subnet. One of subnet_id or port_id must be specified
osc network router conntrack-helper
Lists, creates, shows details for, updates, and deletes router conntrack helper (CT) target rules
Usage: osc network router conntrack-helper <COMMAND>
Available subcommands:
osc network router conntrack-helper create
— Create conntrack helperosc network router conntrack-helper delete
— Delete a conntrack helperosc network router conntrack-helper list
— List router conntrack helpersosc network router conntrack-helper set
— Update a conntrack helperosc network router conntrack-helper show
— Show conntrack helper
osc network router conntrack-helper create
Creates a router conntrack helper.
Normal response codes: 201
Error response codes: 400, 404
Usage: osc network router conntrack-helper create [OPTIONS] <ROUTER_ID>
Arguments:
<ROUTER_ID>
— router_id parameter for /v2.0/routers/{router_id}/conntrack_helpers/{id} API
Options:
-
--helper <HELPER>
— The netfilter conntrack helper module -
--port <PORT>
— The network port for the netfilter conntrack target rule -
--project-id <PROJECT_ID>
-
--protocol <PROTOCOL>
— The network protocol for the netfilter conntrack target rulePossible values:
dccp
,icmp
,ipv6-icmp
,sctp
,tcp
,udp
osc network router conntrack-helper delete
Deletes a router conntrack helper.
Normal response codes: 204
Error response codes: 404
Usage: osc network router conntrack-helper delete <ROUTER_ID> <ID>
Arguments:
<ROUTER_ID>
— router_id parameter for /v2.0/routers/{router_id}/conntrack_helpers/{id} API<ID>
— id parameter for /v2.0/routers/{router_id}/conntrack_helpers/{id} API
osc network router conntrack-helper list
Lists router conntrack helpers associated with a router.
Standard query parameters are supported on the URI. For more information, see Filtering and Column Selection.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Pagination query parameters are supported if Neutron configuration supports it by overriding allow_pagination=false
. For more information, see Pagination.
Sorting query parameters are supported if Neutron configuration supports it with allow_sorting=true
. For more information, see Sorting.
Normal response codes: 200
Error response codes: 400, 404
Usage: osc network router conntrack-helper list [OPTIONS] <ROUTER_ID>
Arguments:
<ROUTER_ID>
— router_id parameter for /v2.0/routers/{router_id}/conntrack_helpers/{id} API
Options:
-
--helper <HELPER>
— helper query parameter for /v2.0/routers/{router_id}/conntrack_helpers API -
--id <ID>
— id query parameter for /v2.0/routers/{router_id}/conntrack_helpers API -
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--port <PORT>
— port query parameter for /v2.0/routers/{router_id}/conntrack_helpers API -
--protocol <PROTOCOL>
— protocol query parameter for /v2.0/routers/{router_id}/conntrack_helpers APIPossible values:
dccp
,icmp
,ipv6-icmp
,sctp
,tcp
,udp
-
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network router conntrack-helper set
Updates a router conntrack helper.
Normal response codes: 200
Error response codes: 400, 404
Usage: osc network router conntrack-helper set [OPTIONS] <ROUTER_ID> <ID>
Arguments:
<ROUTER_ID>
— router_id parameter for /v2.0/routers/{router_id}/conntrack_helpers/{id} API<ID>
— id parameter for /v2.0/routers/{router_id}/conntrack_helpers/{id} API
Options:
-
--helper <HELPER>
— The netfilter conntrack helper module -
--port <PORT>
— The network port for the netfilter conntrack target rule -
--protocol <PROTOCOL>
— The network protocol for the netfilter conntrack target rulePossible values:
dccp
,icmp
,ipv6-icmp
,sctp
,tcp
,udp
osc network router conntrack-helper show
Shows information for a router conntrack helper.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Normal response codes: 200
Error response codes: 400, 404
Usage: osc network router conntrack-helper show <ROUTER_ID> <ID>
Arguments:
<ROUTER_ID>
— router_id parameter for /v2.0/routers/{router_id}/conntrack_helpers/{id} API<ID>
— id parameter for /v2.0/routers/{router_id}/conntrack_helpers/{id} API
osc network router create
Creates a logical router.
This operation creates a logical router. The logical router does not have any internal interface and it is not associated with any subnet. You can optionally specify an external gateway for a router at create time. The external gateway for the router must be plugged into an external network. An external network has its router:external
extended field set to true
. To specify an external gateway, the ID of the external network must be passed in the network_id
parameter of the external_gateway_info
attribute in the request body.
Normal response codes: 201
Error response codes: 400, 401
Usage: osc network router create [OPTIONS]
Options:
-
--admin-state-up <ADMIN_STATE_UP>
— The administrative state of the resource, which is up (true
) or down (false
). Default istrue
Possible values:
true
,false
-
--availability-zone-hints <AVAILABILITY_ZONE_HINTS>
— The availability zone candidates for the router. It is available whenrouter_availability_zone
extension is enabled.Parameter is an array, may be provided multiple times.
-
--description <DESCRIPTION>
— A human-readable description for the resource. Default is an empty string -
--distributed <DISTRIBUTED>
—true
indicates a distributed router. It is available whendvr
extension is enabledPossible values:
true
,false
-
--enable-ndp-proxy <ENABLE_NDP_PROXY>
— Enable NDP proxy attribute. Default isfalse
, To persist this attribute value, set theenable_ndp_proxy_by_default
option in theneutron.conf
file. It is available whenrouter-extend-ndp-proxy
extension is enabledPossible values:
true
,false
-
--enable-snat <ENABLE_SNAT>
Possible values:
true
,false
-
--external-fixed-ips <JSON>
— Parameter is an array, may be provided multiple times -
--network-id <NETWORK_ID>
-
--qos-policy-id <QOS_POLICY_ID>
-
--flavor-id <FLAVOR_ID>
— The ID of the flavor associated with the router -
--ha <HA>
—true
indicates a highly-available router. It is available whenl3-ha
extension is enabledPossible values:
true
,false
-
--name <NAME>
— Human-readable name of the resource. Default is an empty string -
--tenant-id <TENANT_ID>
— The ID of the project that owns the resource. Only administrative and users with advsvc role can specify a project ID other than their own. You cannot change this value through authorization policies
osc network router delete
Deletes a logical router and, if present, its external gateway interface.
This operation fails if the router has attached interfaces. Use the remove router interface operation to remove all router interfaces before you delete the router.
Normal response codes: 204
Error response codes: 401, 404, 409, 412
Usage: osc network router delete <ID>
Arguments:
<ID>
— id parameter for /v2.0/routers/{id} API
osc network router l3-agent
L3 agent scheduler
The L3 agent scheduler extension (l3_agent_scheduler) allows administrators to assign Neutron routers to Neutron L3 agents, and retrieve mappings between Neutron routers and L3 agents.
Usage: osc network router l3-agent <COMMAND>
Available subcommands:
osc network router l3-agent list
— List L3 agents hosting a router
osc network router l3-agent list
Lists l3 agents hosting a specific router.
Standard query parameters are supported on the URI. For more information, see Filtering and Column Selection.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Pagination query parameters are supported if Neutron configuration supports it by overriding allow_pagination=false
. For more information, see Pagination.
Sorting query parameters are supported if Neutron configuration supports it with allow_sorting=true
. For more information, see Sorting.
Normal response codes: 200
Error response codes: 401, 404
Usage: osc network router l3-agent list [OPTIONS] <ROUTER_ID>
Arguments:
<ROUTER_ID>
— router_id parameter for /v2.0/routers/{router_id}/l3-agents/{id} API
Options:
-
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network router list
Lists logical routers that the project who submits the request can access.
Default policy settings return only those routers that the project who submits the request owns, unless an administrative user submits the request.
Standard query parameters are supported on the URI. For more information, see Filtering and Column Selection.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Pagination query parameters are supported if Neutron configuration supports it by overriding allow_pagination=false
. For more information, see Pagination.
Sorting query parameters are supported if Neutron configuration supports it with allow_sorting=true
. For more information, see Sorting.
Normal response codes: 200
Error response codes: 401
Usage: osc network router list [OPTIONS]
Options:
-
--admin-state-up <ADMIN_STATE_UP>
— admin_state_up query parameter for /v2.0/routers APIPossible values:
true
,false
-
--description <DESCRIPTION>
— description query parameter for /v2.0/routers API -
--enable-ndp-proxy <ENABLE_NDP_PROXY>
— enable_ndp_proxy query parameter for /v2.0/routers APIPossible values:
true
,false
-
--id <ID>
— id query parameter for /v2.0/routers API -
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--name <NAME>
— name query parameter for /v2.0/routers API -
--not-tags <NOT_TAGS>
— not-tags query parameter for /v2.0/routers API -
--not-tags-any <NOT_TAGS_ANY>
— not-tags-any query parameter for /v2.0/routers API -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--revision-number <REVISION_NUMBER>
— revision_number query parameter for /v2.0/routers API -
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--tags <TAGS>
— tags query parameter for /v2.0/routers API -
--tags-any <TAGS_ANY>
— tags-any query parameter for /v2.0/routers API -
--tenant-id <TENANT_ID>
— tenant_id query parameter for /v2.0/routers API -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network router remove-external-gateway
Remove external gateways from router
Usage: osc network router remove-external-gateway [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.0/routers/{id} API
Options:
-
--external-gateways <JSON>
— The list of external gateways of the router.Parameter is an array, may be provided multiple times.
osc network router remove-extraroute
Remove extra routes from router
Usage: osc network router remove-extraroute [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.0/routers/{id} API
Options:
-
--routes <JSON>
— The extra routes configuration for L3 router. A list of dictionaries withdestination
andnexthop
parameters. It is available whenextraroute
extension is enabled.Parameter is an array, may be provided multiple times.
osc network router remove-router-interface
Remove interface from router
Usage: osc network router remove-router-interface [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.0/routers/{id} API
Options:
--port-id <PORT_ID>
— The ID of the port. One of subnet_id or port_id must be specified--subnet-id <SUBNET_ID>
— The ID of the subnet. One of subnet_id or port_id must be specified
osc network router set
Updates a logical router.
This operation does not enable the update of router interfaces. To update a router interface, use the add router interface and remove router interface operations.
Normal response codes: 200
Error response codes: 400, 401, 404, 412
Usage: osc network router set [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.0/routers/{id} API
Options:
-
--admin-state-up <ADMIN_STATE_UP>
— The administrative state of the resource, which is up (true
) or down (false
)Possible values:
true
,false
-
--description <DESCRIPTION>
— A human-readable description for the resource. Default is an empty string -
--distributed <DISTRIBUTED>
—true
indicates a distributed router. It is available whendvr
extension is enabledPossible values:
true
,false
-
--enable-ndp-proxy <ENABLE_NDP_PROXY>
— Enable NDP proxy attribute. Default isfalse
, To persist this attribute value, set theenable_ndp_proxy_by_default
option in theneutron.conf
file. It is available whenrouter-extend-ndp-proxy
extension is enabledPossible values:
true
,false
-
--enable-snat <ENABLE_SNAT>
Possible values:
true
,false
-
--external-fixed-ips <JSON>
— Parameter is an array, may be provided multiple times -
--network-id <NETWORK_ID>
-
--qos-policy-id <QOS_POLICY_ID>
-
--ha <HA>
—true
indicates a highly-available router. It is available whenl3-ha
extension is enabledPossible values:
true
,false
-
--name <NAME>
— Human-readable name of the resource -
--routes <JSON>
— The extra routes configuration for L3 router. A list of dictionaries withdestination
andnexthop
parameters. It is available whenextraroute
extension is enabled. Default is an empty list ([]
).Parameter is an array, may be provided multiple times.
osc network router show
Shows details for a router.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Normal response codes: 200
Error response codes: 401, 403, 404
Usage: osc network router show <ID>
Arguments:
<ID>
— id parameter for /v2.0/routers/{id} API
osc network router tag
Lists tags, creates, replaces or deletes one or more tags for a resource, checks the existence of a tag for a resource
Usage: osc network router tag <COMMAND>
Available subcommands:
osc network router tag add
— Command without description in OpenAPIosc network router tag check
— Command without description in OpenAPIosc network router tag delete
— Command without description in OpenAPIosc network router tag list
— Command without description in OpenAPIosc network router tag purge
— Command without description in OpenAPI
osc network router tag add
Command without description in OpenAPI
Usage: osc network router tag add <ROUTER_ID> <ID>
Arguments:
<ROUTER_ID>
— router_id parameter for /v2.0/routers/{router_id}/tags/{id} API<ID>
— id parameter for /v2.0/routers/{router_id}/tags/{id} API
osc network router tag check
Command without description in OpenAPI
Usage: osc network router tag check <ROUTER_ID> <ID>
Arguments:
<ROUTER_ID>
— router_id parameter for /v2.0/routers/{router_id}/tags/{id} API<ID>
— id parameter for /v2.0/routers/{router_id}/tags/{id} API
osc network router tag delete
Command without description in OpenAPI
Usage: osc network router tag delete <ROUTER_ID> <ID>
Arguments:
<ROUTER_ID>
— router_id parameter for /v2.0/routers/{router_id}/tags/{id} API<ID>
— id parameter for /v2.0/routers/{router_id}/tags/{id} API
osc network router tag list
Command without description in OpenAPI
Usage: osc network router tag list [OPTIONS] <ROUTER_ID>
Arguments:
<ROUTER_ID>
— router_id parameter for /v2.0/routers/{router_id}/tags/{id} API
Options:
-
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network router tag purge
Command without description in OpenAPI
Usage: osc network router tag purge <ROUTER_ID>
Arguments:
<ROUTER_ID>
— router_id parameter for /v2.0/routers/{router_id}/tags/{id} API
osc network security-group
SecurityGroup commands
Usage: osc network security-group <COMMAND>
Available subcommands:
osc network security-group create
— Create security grouposc network security-group delete
— Delete security grouposc network security-group list
— List security groupsosc network security-group set
— Update security grouposc network security-group show
— Show security grouposc network security-group tag
— Lists tags, creates, replaces or deletes one or more tags for a resource, checks the existence of a tag for a resource
osc network security-group create
Creates an OpenStack Networking security group.
This operation creates a security group with default security group rules for the IPv4 and IPv6 ether types.
Normal response codes: 201
Error response codes: 400, 401, 409
Usage: osc network security-group create [OPTIONS]
Options:
-
--description <DESCRIPTION>
— A human-readable description for the resource. Default is an empty string -
--name <NAME>
— Human-readable name of the resource -
--stateful <STATEFUL>
— Indicates if the security group is stateful or statelessPossible values:
true
,false
-
--tenant-id <TENANT_ID>
— The ID of the project
osc network security-group delete
Deletes an OpenStack Networking security group.
This operation deletes an OpenStack Networking security group and its associated security group rules, provided that a port is not associated with the security group. If a port is associated with the security group 409 (Conflict) is returned.
This operation does not require a request body. This operation does not return a response body.
Normal response codes: 204
Error response codes: 401, 404, 409, 412
Usage: osc network security-group delete <ID>
Arguments:
<ID>
— id parameter for /v2.0/security-groups/{id} API
osc network security-group list
Lists OpenStack Networking security groups to which the project has access.
The response is an array of security_group
objects which contains a list of security_group_rules
objects.
Standard query parameters are supported on the URI. For more information, see Filtering and Column Selection.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Pagination query parameters are supported if Neutron configuration supports it by overriding allow_pagination=false
. For more information, see Pagination.
Sorting query parameters are supported if Neutron configuration supports it with allow_sorting=true
. For more information, see Sorting.
Normal response codes: 200
Error response codes: 401
Usage: osc network security-group list [OPTIONS]
Options:
-
--description <DESCRIPTION>
— description query parameter for /v2.0/security-groups API -
--id <ID>
— id query parameter for /v2.0/security-groups API -
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--name <NAME>
— name query parameter for /v2.0/security-groups API -
--not-tags <NOT_TAGS>
— not-tags query parameter for /v2.0/security-groups API -
--not-tags-any <NOT_TAGS_ANY>
— not-tags-any query parameter for /v2.0/security-groups API -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--revision-number <REVISION_NUMBER>
— revision_number query parameter for /v2.0/security-groups API -
--shared <SHARED>
— shared query parameter for /v2.0/security-groups APIPossible values:
true
,false
-
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--tags <TAGS>
— tags query parameter for /v2.0/security-groups API -
--tags-any <TAGS_ANY>
— tags-any query parameter for /v2.0/security-groups API -
--tenant-id <TENANT_ID>
— tenant_id query parameter for /v2.0/security-groups API -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network security-group set
Updates a security group.
Normal response codes: 200
Error response codes: 400, 401, 403, 404, 412
Usage: osc network security-group set [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.0/security-groups/{id} API
Options:
-
--description <DESCRIPTION>
— A human-readable description for the resource. Default is an empty string -
--name <NAME>
— Human-readable name of the resource -
--stateful <STATEFUL>
Possible values:
true
,false
osc network security-group show
Shows details for a security group.
The associated security group rules are contained in the response.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Normal response codes: 200
Error response codes: 401, 404
Usage: osc network security-group show <ID>
Arguments:
<ID>
— id parameter for /v2.0/security-groups/{id} API
osc network security-group tag
Lists tags, creates, replaces or deletes one or more tags for a resource, checks the existence of a tag for a resource
Usage: osc network security-group tag <COMMAND>
Available subcommands:
osc network security-group tag add
— Command without description in OpenAPIosc network security-group tag check
— Command without description in OpenAPIosc network security-group tag delete
— Command without description in OpenAPIosc network security-group tag list
— Command without description in OpenAPIosc network security-group tag purge
— Command without description in OpenAPI
osc network security-group tag add
Command without description in OpenAPI
Usage: osc network security-group tag add <SECURITY_GROUP_ID> <ID>
Arguments:
<SECURITY_GROUP_ID>
— security_group_id parameter for /v2.0/security-groups/{security_group_id}/tags/{id} API<ID>
— id parameter for /v2.0/security-groups/{security_group_id}/tags/{id} API
osc network security-group tag check
Command without description in OpenAPI
Usage: osc network security-group tag check <SECURITY_GROUP_ID> <ID>
Arguments:
<SECURITY_GROUP_ID>
— security_group_id parameter for /v2.0/security-groups/{security_group_id}/tags/{id} API<ID>
— id parameter for /v2.0/security-groups/{security_group_id}/tags/{id} API
osc network security-group tag delete
Command without description in OpenAPI
Usage: osc network security-group tag delete <SECURITY_GROUP_ID> <ID>
Arguments:
<SECURITY_GROUP_ID>
— security_group_id parameter for /v2.0/security-groups/{security_group_id}/tags/{id} API<ID>
— id parameter for /v2.0/security-groups/{security_group_id}/tags/{id} API
osc network security-group tag list
Command without description in OpenAPI
Usage: osc network security-group tag list [OPTIONS] <SECURITY_GROUP_ID>
Arguments:
<SECURITY_GROUP_ID>
— security_group_id parameter for /v2.0/security-groups/{security_group_id}/tags/{id} API
Options:
-
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network security-group tag purge
Command without description in OpenAPI
Usage: osc network security-group tag purge <SECURITY_GROUP_ID>
Arguments:
<SECURITY_GROUP_ID>
— security_group_id parameter for /v2.0/security-groups/{security_group_id}/tags/{id} API
osc network security-group-rule
SecurityGroupRule commands
Usage: osc network security-group-rule <COMMAND>
Available subcommands:
osc network security-group-rule create
— Create security group ruleosc network security-group-rule delete
— Delete security group ruleosc network security-group-rule list
— List security group rulesosc network security-group-rule set
— Command without description in OpenAPIosc network security-group-rule show
— Show security group rule
osc network security-group-rule create
Creates an OpenStack Networking security group rule.
Normal response codes: 201
Error response codes: 400, 401, 404, 409
Usage: osc network security-group-rule create [OPTIONS]
Options:
-
--description <DESCRIPTION>
— A human-readable description for the resource. Default is an empty string -
--direction <DIRECTION>
— Ingress or egress, which is the direction in which the security group rule is appliedPossible values:
egress
,ingress
-
--ethertype <ETHERTYPE>
— Must be IPv4 or IPv6, and addresses represented in CIDR must match the ingress or egress rulesPossible values:
ipv4
,ipv6
-
--port-range-max <PORT_RANGE_MAX>
— The maximum port number in the range that is matched by the security group rule. If the protocol is TCP, UDP, DCCP, SCTP or UDP-Lite this value must be greater than or equal to theport_range_min
attribute value. If the protocol is ICMP, this value must be an ICMP code -
--port-range-min <PORT_RANGE_MIN>
— The minimum port number in the range that is matched by the security group rule. If the protocol is TCP, UDP, DCCP, SCTP or UDP-Lite this value must be less than or equal to theport_range_max
attribute value. If the protocol is ICMP, this value must be an ICMP type -
--protocol <PROTOCOL>
— The IP protocol can be represented by a string, an integer, ornull
. Valid string or integer values areany
or0
,ah
or51
,dccp
or33
,egp
or8
,esp
or50
,gre
or47
,icmp
or1
,icmpv6
or58
,igmp
or2
,ipip
or4
,ipv6-encap
or41
,ipv6-frag
or44
,ipv6-icmp
or58
,ipv6-nonxt
or59
,ipv6-opts
or60
,ipv6-route
or43
,ospf
or89
,pgm
or113
,rsvp
or46
,sctp
or132
,tcp
or6
,udp
or17
,udplite
or136
,vrrp
or112
. Additionally, any integer value between [0-255] is also valid. The stringany
(or integer0
) meansall
IP protocols. See the constants inneutron_lib.constants
for the most up-to-date list of supported strings -
--remote-address-group-id <REMOTE_ADDRESS_GROUP_ID>
-
--remote-group-id <REMOTE_GROUP_ID>
— The remote group UUID to associate with this security group rule. You can specify either theremote_group_id
orremote_ip_prefix
attribute in the request body -
--remote-ip-prefix <REMOTE_IP_PREFIX>
— The remote IP prefix that is matched by this security group rule -
--security-group-id <SECURITY_GROUP_ID>
— The security group ID to associate with this security group rule -
--tenant-id <TENANT_ID>
osc network security-group-rule delete
Deletes a rule from an OpenStack Networking security group.
Normal response codes: 204
Error response codes: 401, 404, 412
Usage: osc network security-group-rule delete <ID>
Arguments:
<ID>
— id parameter for /v2.0/security-group-rules/{id} API
osc network security-group-rule list
Lists a summary of all OpenStack Networking security group rules that the project can access.
The list provides the ID for each security group rule.
Standard query parameters are supported on the URI. For more information, see Filtering and Column Selection.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Pagination query parameters are supported if Neutron configuration supports it by overriding allow_pagination=false
. For more information, see Pagination.
Sorting query parameters are supported if Neutron configuration supports it with allow_sorting=true
. For more information, see Sorting.
Normal response codes: 200
Error response codes: 401
Usage: osc network security-group-rule list [OPTIONS]
Options:
-
--belongs-to-default-sg <BELONGS_TO_DEFAULT_SG>
— belongs_to_default_sg query parameter for /v2.0/security-group-rules APIPossible values:
true
,false
-
--description <DESCRIPTION>
— description query parameter for /v2.0/security-group-rules API -
--direction <DIRECTION>
— direction query parameter for /v2.0/security-group-rules APIPossible values:
egress
,ingress
-
--ethertype <ETHERTYPE>
— ethertype query parameter for /v2.0/security-group-rules APIPossible values:
IPv4
,IPv6
-
--id <ID>
— id query parameter for /v2.0/security-group-rules API -
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--normalized-cidr <NORMALIZED_CIDR>
— normalized_cidr query parameter for /v2.0/security-group-rules API -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--port-range-max <PORT_RANGE_MAX>
— port_range_max query parameter for /v2.0/security-group-rules API -
--port-range-min <PORT_RANGE_MIN>
— port_range_min query parameter for /v2.0/security-group-rules API -
--protocol <PROTOCOL>
— protocol query parameter for /v2.0/security-group-rules API -
--remote-address-group-id <REMOTE_ADDRESS_GROUP_ID>
— remote_address_group_id query parameter for /v2.0/security-group-rules API -
--remote-group-id <REMOTE_GROUP_ID>
— remote_group_id query parameter for /v2.0/security-group-rules API -
--remote-ip-prefix <REMOTE_IP_PREFIX>
— remote_ip_prefix query parameter for /v2.0/security-group-rules API -
--revision-number <REVISION_NUMBER>
— revision_number query parameter for /v2.0/security-group-rules API -
--security-group-id <SECURITY_GROUP_ID>
— security_group_id query parameter for /v2.0/security-group-rules API -
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--tenant-id <TENANT_ID>
— tenant_id query parameter for /v2.0/security-group-rules API -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network security-group-rule set
Command without description in OpenAPI
Usage: osc network security-group-rule set [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.0/security-group-rules/{id} API
Options:
--description <DESCRIPTION>
osc network security-group-rule show
Shows detailed information for a security group rule.
The response body contains the following information about the security group rule:
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Normal response codes: 200
Error response codes: 401, 404
Usage: osc network security-group-rule show <ID>
Arguments:
<ID>
— id parameter for /v2.0/security-group-rules/{id} API
osc network segment
Segments
Lists, shows details for, creates, updates, and deletes segments. The segments API is admin-only.
Usage: osc network segment <COMMAND>
Available subcommands:
osc network segment create
— Create segmentosc network segment delete
— Delete segmentosc network segment list
— List segmentsosc network segment set
— Update segmentosc network segment show
— Show segment details
osc network segment create
Creates a segment.
Normal response codes: 201
Error response codes: 400, 401
Usage: osc network segment create [OPTIONS]
Options:
--description <DESCRIPTION>
— A human-readable description for the resource. Default is an empty string--name <NAME>
— Human-readable name of the segment--network-id <NETWORK_ID>
— The ID of the attached network--network-type <NETWORK_TYPE>
— The type of physical network that maps to this network resource. For example,flat
,vlan
,vxlan
, orgre
--physical-network <PHYSICAL_NETWORK>
— The physical network where this network/segment is implemented--segmentation-id <SEGMENTATION_ID>
— The ID of the isolated segment on the physical network. Thenetwork_type
attribute defines the segmentation model. For example, if thenetwork_type
value is vlan, this ID is a vlan identifier. If thenetwork_type
value is gre, this ID is a gre key.Note
that only the segmentation-id of VLAN type networks can be changed!--tenant-id <TENANT_ID>
osc network segment delete
Deletes a segment and its associated resources.
Normal response codes: 204
Error response codes: 401, 404, 409, 412
Usage: osc network segment delete <ID>
Arguments:
<ID>
— id parameter for /v2.0/segments/{id} API
osc network segment list
Lists segments to which the project has access.
Standard query parameters are supported on the URI. For more information, see Filtering and Column Selection.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Pagination query parameters are supported if Neutron configuration supports it by overriding allow_pagination=false
. For more information, see Pagination.
Sorting query parameters are supported if Neutron configuration supports it with allow_sorting=true
. For more information, see Sorting.
Normal response codes: 200
Error response codes: 401
Usage: osc network segment list [OPTIONS]
Options:
-
--description <DESCRIPTION>
— description query parameter for /v2.0/segments API -
--id <ID>
— id query parameter for /v2.0/segments API -
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--name <NAME>
— name query parameter for /v2.0/segments API -
--network-id <NETWORK_ID>
— network_id query parameter for /v2.0/segments API -
--network-type <NETWORK_TYPE>
— network_type query parameter for /v2.0/segments API -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--physical-network <PHYSICAL_NETWORK>
— physical_network query parameter for /v2.0/segments API -
--revision-number <REVISION_NUMBER>
— revision_number query parameter for /v2.0/segments API -
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network segment set
Updates a segment.
Normal response codes: 200
Error response codes: 400, 401, 403, 404, 412
Usage: osc network segment set [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.0/segments/{id} API
Options:
--description <DESCRIPTION>
— A human-readable description for the resource. Default is an empty string--name <NAME>
— Human-readable name of the segment
osc network segment show
Shows details for a segment.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Normal response codes: 200
Error response codes: 401, 404
Usage: osc network segment show <ID>
Arguments:
<ID>
— id parameter for /v2.0/segments/{id} API
osc network subnet
Subnet commands
Usage: osc network subnet <COMMAND>
Available subcommands:
osc network subnet create
— Create subnetosc network subnet delete
— Delete subnetosc network subnet list
— List subnetsosc network subnet set
— Update subnetosc network subnet show
— Show subnet detailsosc network subnet tag
— Lists tags, creates, replaces or deletes one or more tags for a resource, checks the existence of a tag for a resource
osc network subnet create
Creates a subnet on a network.
OpenStack Networking does not try to derive the correct IP version from the CIDR. If you do not specify the gateway_ip
attribute, OpenStack Networking allocates an address from the CIDR for the gateway for the subnet.
To specify a subnet without a gateway, set the gateway_ip
attribute to null
in the request body. If you do not specify the allocation_pools
attribute, OpenStack Networking automatically allocates pools for covering all IP addresses in the CIDR, excluding the address reserved for the subnet gateway. Otherwise, you can explicitly specify allocation pools as shown in the following example.
When you specify both the allocation_pools
and gateway_ip
attributes, you must ensure that the gateway IP does not overlap with the allocation pools; otherwise, the call returns the Conflict (409)
response code.
A subnet can have one or more name servers and host routes. Hosts in this subnet use the name servers. Devices with IP addresses from this subnet, not including the local subnet route, use the host routes.
Specify the ipv6_ra_mode
and ipv6_address_mode
attributes to create subnets that support IPv6 configurations, such as stateless address autoconfiguration (SLAAC), DHCPv6 stateful, and DHCPv6 stateless configurations.
A subnet can optionally be associated with a network segment when it is created by specifying the segment_id
of a valid segment on the specified network. A network with subnets associated in this way is called a routed network. On any given network, all of the subnets must be associated with segments or none of them can be. Neutron enforces this invariant. Currently, routed networks are only supported for provider networks.
Normal response codes: 201
Error response codes: 400, 401, 403, 404, 409
Usage: osc network subnet create [OPTIONS] --ip-version <IP_VERSION> --network-id <NETWORK_ID>
Options:
-
--allocation-pools <JSON>
— Allocation pools withstart
andend
IP addresses for this subnet. If allocation_pools are not specified, OpenStack Networking automatically allocates pools for covering all IP addresses in the CIDR, excluding the address reserved for the subnet gateway by default.Parameter is an array, may be provided multiple times.
-
--cidr <CIDR>
— The CIDR of the subnet -
--description <DESCRIPTION>
— A human-readable description for the resource. Default is an empty string -
--dns-nameservers <DNS_NAMESERVERS>
— List of dns name servers associated with the subnet. Default is an empty list.Parameter is an array, may be provided multiple times.
-
--dns-publish-fixed-ip <DNS_PUBLISH_FIXED_IP>
— Whether to publish DNS records for IPs from this subnet. Default isfalse
Possible values:
true
,false
-
--enable-dhcp <ENABLE_DHCP>
— Indicates whether dhcp is enabled or disabled for the subnet. Default istrue
Possible values:
true
,false
-
--gateway-ip <GATEWAY_IP>
— Gateway IP of this subnet. If the value isnull
that implies no gateway is associated with the subnet. If the gateway_ip is not specified, OpenStack Networking allocates an address from the CIDR for the gateway for the subnet by default -
--host-routes <JSON>
— Additional routes for the subnet. A list of dictionaries withdestination
andnexthop
parameters. Default value is an empty list.Parameter is an array, may be provided multiple times.
-
--ip-version <IP_VERSION>
— The IP protocol version. Value is4
or6
-
--ipv6-address-mode <IPV6_ADDRESS_MODE>
— The IPv6 address modes specifies mechanisms for assigning IP addresses. Value isslaac
,dhcpv6-stateful
,dhcpv6-stateless
Possible values:
dhcpv6-stateful
,dhcpv6-stateless
,slaac
-
--ipv6-ra-mode <IPV6_RA_MODE>
— The IPv6 router advertisement specifies whether the networking service should transmit ICMPv6 packets, for a subnet. Value isslaac
,dhcpv6-stateful
,dhcpv6-stateless
Possible values:
dhcpv6-stateful
,dhcpv6-stateless
,slaac
-
--name <NAME>
— Human-readable name of the resource. Default is an empty string -
--network-id <NETWORK_ID>
— The ID of the network to which the subnet belongs -
--prefixlen <PREFIXLEN>
— The prefix length to use for subnet allocation from a subnet pool. If not specified, thedefault_prefixlen
value of the subnet pool will be used -
--segment-id <SEGMENT_ID>
— The ID of a network segment the subnet is associated with. It is available whensegment
extension is enabled -
--service-types <SERVICE_TYPES>
— The service types associated with the subnet.Parameter is an array, may be provided multiple times.
-
--subnetpool-id <SUBNETPOOL_ID>
— The ID of the subnet pool associated with the subnet -
--tenant-id <TENANT_ID>
— The ID of the project that owns the resource. Only administrative and users with advsvc role can specify a project ID other than their own. You cannot change this value through authorization policies -
--use-default-subnetpool <USE_DEFAULT_SUBNETPOOL>
— Whether to allocate this subnet from the default subnet poolPossible values:
true
,false
osc network subnet delete
Deletes a subnet.
The operation fails if subnet IP addresses are still allocated.
Normal response codes: 204
Error response codes: 401, 404, 412
Usage: osc network subnet delete <ID>
Arguments:
<ID>
— subnet_id parameter for /v2.0/subnets/{subnet_id} API
osc network subnet list
Lists subnets that the project has access to.
Default policy settings return only subnets owned by the project of the user submitting the request, unless the user has administrative role. You can control which attributes are returned by using the fields query parameter. You can filter results by using query string parameters.
Standard query parameters are supported on the URI. For more information, see Filtering and Column Selection.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Pagination query parameters are supported if Neutron configuration supports it by overriding allow_pagination=false
. For more information, see Pagination.
Sorting query parameters are supported if Neutron configuration supports it with allow_sorting=true
. For more information, see Sorting.
Normal response codes: 200
Error response codes: 401
Usage: osc network subnet list [OPTIONS]
Options:
-
--cidr <CIDR>
— cidr query parameter for /v2.0/subnets API -
--description <DESCRIPTION>
— description query parameter for /v2.0/subnets API -
--enable-dhcp <ENABLE_DHCP>
— enable_dhcp query parameter for /v2.0/subnets APIPossible values:
true
,false
-
--gateway-ip <GATEWAY_IP>
— gateway_ip query parameter for /v2.0/subnets API -
--id <ID>
— id query parameter for /v2.0/subnets API -
--ip-version <IP_VERSION>
— ip_version query parameter for /v2.0/subnets API -
--ipv6-address-mode <IPV6_ADDRESS_MODE>
— ipv6_address_mode query parameter for /v2.0/subnets APIPossible values:
dhcpv6-stateful
,dhcpv6-stateless
,slaac
-
--ipv6-ra-mode <IPV6_RA_MODE>
— ipv6_ra_mode query parameter for /v2.0/subnets APIPossible values:
dhcpv6-stateful
,dhcpv6-stateless
,slaac
-
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--name <NAME>
— name query parameter for /v2.0/subnets API -
--network-id <NETWORK_ID>
— network_id query parameter for /v2.0/subnets API -
--not-tags <NOT_TAGS>
— not-tags query parameter for /v2.0/subnets API -
--not-tags-any <NOT_TAGS_ANY>
— not-tags-any query parameter for /v2.0/subnets API -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--revision-number <REVISION_NUMBER>
— revision_number query parameter for /v2.0/subnets API -
--router-external <ROUTER_EXTERNAL>
— The membership of a subnet to an external networkPossible values:
true
,false
-
--segment-id <SEGMENT_ID>
— segment_id query parameter for /v2.0/subnets API -
--shared <SHARED>
— shared query parameter for /v2.0/subnets APIPossible values:
true
,false
-
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--subnetpool-id <SUBNETPOOL_ID>
— subnetpool_id query parameter for /v2.0/subnets API -
--tags <TAGS>
— tags query parameter for /v2.0/subnets API -
--tags-any <TAGS_ANY>
— tags-any query parameter for /v2.0/subnets API -
--tenant-id <TENANT_ID>
— tenant_id query parameter for /v2.0/subnets API -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network subnet set
Updates a subnet.
Some attributes, such as IP version (ip_version), CIDR (cidr), and segment (segment_id) cannot be updated. Attempting to update these attributes results in a 400 Bad Request
error.
Normal response codes: 200
Error response codes: 400, 401, 403, 404, 412
Usage: osc network subnet set [OPTIONS] <ID>
Arguments:
<ID>
— subnet_id parameter for /v2.0/subnets/{subnet_id} API
Options:
-
--allocation-pools <JSON>
— Allocation pools withstart
andend
IP addresses for this subnet. If allocation_pools are not specified, OpenStack Networking automatically allocates pools for covering all IP addresses in the CIDR, excluding the address reserved for the subnet gateway by default.Parameter is an array, may be provided multiple times.
-
--description <DESCRIPTION>
— A human-readable description for the resource. Default is an empty string -
--dns-nameservers <DNS_NAMESERVERS>
— List of dns name servers associated with the subnet. Default is an empty list.Parameter is an array, may be provided multiple times.
-
--dns-publish-fixed-ip <DNS_PUBLISH_FIXED_IP>
— Whether to publish DNS records for IPs from this subnet. Default isfalse
Possible values:
true
,false
-
--enable-dhcp <ENABLE_DHCP>
— Indicates whether dhcp is enabled or disabled for the subnet. Default istrue
Possible values:
true
,false
-
--gateway-ip <GATEWAY_IP>
— Gateway IP of this subnet. If the value isnull
that implies no gateway is associated with the subnet. If the gateway_ip is not specified, OpenStack Networking allocates an address from the CIDR for the gateway for the subnet by default -
--host-routes <JSON>
— Additional routes for the subnet. A list of dictionaries withdestination
andnexthop
parameters. Default value is an empty list.Parameter is an array, may be provided multiple times.
-
--name <NAME>
— Human-readable name of the resource -
--segment-id <SEGMENT_ID>
— The ID of a network segment the subnet is associated with. It is available whensegment
extension is enabled -
--service-types <SERVICE_TYPES>
— The service types associated with the subnet.Parameter is an array, may be provided multiple times.
osc network subnet show
Shows details for a subnet.
Use the fields query parameter to filter the results.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Normal response codes: 200
Error response codes: 401, 404
Usage: osc network subnet show <ID>
Arguments:
<ID>
— subnet_id parameter for /v2.0/subnets/{subnet_id} API
osc network subnet tag
Lists tags, creates, replaces or deletes one or more tags for a resource, checks the existence of a tag for a resource
Usage: osc network subnet tag <COMMAND>
Available subcommands:
osc network subnet tag add
— Command without description in OpenAPIosc network subnet tag check
— Command without description in OpenAPIosc network subnet tag delete
— Command without description in OpenAPIosc network subnet tag list
— Command without description in OpenAPIosc network subnet tag purge
— Command without description in OpenAPI
osc network subnet tag add
Command without description in OpenAPI
Usage: osc network subnet tag add <SUBNET_ID> <ID>
Arguments:
<SUBNET_ID>
— subnet_id parameter for /v2.0/subnets/{subnet_id}/tags/{id} API<ID>
— id parameter for /v2.0/subnets/{subnet_id}/tags/{id} API
osc network subnet tag check
Command without description in OpenAPI
Usage: osc network subnet tag check <SUBNET_ID> <ID>
Arguments:
<SUBNET_ID>
— subnet_id parameter for /v2.0/subnets/{subnet_id}/tags/{id} API<ID>
— id parameter for /v2.0/subnets/{subnet_id}/tags/{id} API
osc network subnet tag delete
Command without description in OpenAPI
Usage: osc network subnet tag delete <SUBNET_ID> <ID>
Arguments:
<SUBNET_ID>
— subnet_id parameter for /v2.0/subnets/{subnet_id}/tags/{id} API<ID>
— id parameter for /v2.0/subnets/{subnet_id}/tags/{id} API
osc network subnet tag list
Command without description in OpenAPI
Usage: osc network subnet tag list [OPTIONS] <SUBNET_ID>
Arguments:
<SUBNET_ID>
— subnet_id parameter for /v2.0/subnets/{subnet_id}/tags/{id} API
Options:
-
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network subnet tag purge
Command without description in OpenAPI
Usage: osc network subnet tag purge <SUBNET_ID>
Arguments:
<SUBNET_ID>
— subnet_id parameter for /v2.0/subnets/{subnet_id}/tags/{id} API
osc network subnetpool
SubnetPool commands
Usage: osc network subnetpool <COMMAND>
Available subcommands:
osc network subnetpool add-prefixes
— Add prefixesosc network subnetpool create
— Create subnet poolosc network subnetpool delete
— Delete subnet poolosc network subnetpool list
— List subnet poolsosc network subnetpool remove-prefixes
— Remove prefixesosc network subnetpool set
— Update subnet poolosc network subnetpool show
— Show subnet poolosc network subnetpool tag
— Lists tags, creates, replaces or deletes one or more tags for a resource, checks the existence of a tag for a resource
osc network subnetpool add-prefixes
Add prefixes
Usage: osc network subnetpool add-prefixes [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.0/subnetpools/{id}/remove_prefixes API
Options:
--property <key=value>
osc network subnetpool create
Creates a subnet pool.
Normal response codes: 201
Error response codes: 400, 401, 403, 404
Usage: osc network subnetpool create [OPTIONS]
Options:
-
--address-scope-id <ADDRESS_SCOPE_ID>
— An address scope to assign to the subnet pool -
--default-prefixlen <DEFAULT_PREFIXLEN>
— The size of the prefix to allocate when thecidr
orprefixlen
attributes are omitted when you create the subnet. Default ismin_prefixlen
-
--default-quota <DEFAULT_QUOTA>
— A per-project quota on the prefix space that can be allocated from the subnet pool for project subnets. Default is no quota is enforced on allocations from the subnet pool. For IPv4 subnet pools,default_quota
is measured in units of /32. For IPv6 subnet pools,default_quota
is measured units of /64. All projects that use the subnet pool have the same prefix quota applied -
--description <DESCRIPTION>
— A human-readable description for the resource. Default is an empty string -
--is-default <IS_DEFAULT>
— The subnetpool is default pool or notPossible values:
true
,false
-
--max-prefixlen <MAX_PREFIXLEN>
— The maximum prefix size that can be allocated from the subnet pool. For IPv4 subnet pools, default is32
. For IPv6 subnet pools, default is128
-
--min-prefixlen <MIN_PREFIXLEN>
— The smallest prefix that can be allocated from a subnet pool. For IPv4 subnet pools, default is8
. For IPv6 subnet pools, default is64
-
--name <NAME>
— Human-readable name of the resource -
--prefixes <PREFIXES>
— A list of subnet prefixes to assign to the subnet pool. The API merges adjacent prefixes and treats them as a single prefix. Each subnet prefix must be unique among all subnet prefixes in all subnet pools that are associated with the address scope.Parameter is an array, may be provided multiple times.
-
--shared <SHARED>
— Indicates whether this resource is shared across all projects. By default, only administrative users can change this valuePossible values:
true
,false
-
--tenant-id <TENANT_ID>
— The ID of the project
osc network subnetpool delete
Deletes a subnet pool.
The operation fails if any subnets allocated from the subnet pool are still in use.
Normal response codes: 204
Error response codes: 401, 404, 412
Usage: osc network subnetpool delete <ID>
Arguments:
<ID>
— id parameter for /v2.0/subnetpools/{id}/remove_prefixes API
osc network subnetpool list
Lists subnet pools that the project has access to.
Default policy settings return only the subnet pools owned by the project of the user submitting the request, unless the user has administrative role.
Standard query parameters are supported on the URI. For more information, see Filtering and Column Selection.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Pagination query parameters are supported if Neutron configuration supports it by overriding allow_pagination=false
. For more information, see Pagination.
Sorting query parameters are supported if Neutron configuration supports it with allow_sorting=true
. For more information, see Sorting.
Normal response codes: 200
Error response codes: 401
Usage: osc network subnetpool list [OPTIONS]
Options:
-
--address-scope-id <ADDRESS_SCOPE_ID>
— address_scope_id query parameter for /v2.0/subnetpools API -
--default-prefixlen <DEFAULT_PREFIXLEN>
— default_prefixlen query parameter for /v2.0/subnetpools API -
--default-quota <DEFAULT_QUOTA>
— default_quota query parameter for /v2.0/subnetpools API -
--description <DESCRIPTION>
— description query parameter for /v2.0/subnetpools API -
--id <ID>
— id query parameter for /v2.0/subnetpools API -
--ip-version <IP_VERSION>
— ip_version query parameter for /v2.0/subnetpools API -
--is-default <IS_DEFAULT>
— is_default query parameter for /v2.0/subnetpools APIPossible values:
true
,false
-
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--max-prefixlen <MAX_PREFIXLEN>
— max_prefixlen query parameter for /v2.0/subnetpools API -
--min-prefixlen <MIN_PREFIXLEN>
— min_prefixlen query parameter for /v2.0/subnetpools API -
--name <NAME>
— name query parameter for /v2.0/subnetpools API -
--not-tags <NOT_TAGS>
— not-tags query parameter for /v2.0/subnetpools API -
--not-tags-any <NOT_TAGS_ANY>
— not-tags-any query parameter for /v2.0/subnetpools API -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--revision-number <REVISION_NUMBER>
— revision_number query parameter for /v2.0/subnetpools API -
--shared <SHARED>
— shared query parameter for /v2.0/subnetpools APIPossible values:
true
,false
-
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--tags <TAGS>
— tags query parameter for /v2.0/subnetpools API -
--tags-any <TAGS_ANY>
— tags-any query parameter for /v2.0/subnetpools API -
--tenant-id <TENANT_ID>
— tenant_id query parameter for /v2.0/subnetpools API -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network subnetpool remove-prefixes
Remove prefixes
Usage: osc network subnetpool remove-prefixes [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.0/subnetpools/{id}/remove_prefixes API
Options:
--property <key=value>
osc network subnetpool set
Updates a subnet pool.
Normal response codes: 200
Error response codes: 400, 401, 403, 404, 412
Usage: osc network subnetpool set [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.0/subnetpools/{id}/remove_prefixes API
Options:
-
--address-scope-id <ADDRESS_SCOPE_ID>
— An address scope to assign to the subnet pool -
--default-prefixlen <DEFAULT_PREFIXLEN>
— The size of the prefix to allocate when thecidr
orprefixlen
attributes are omitted when you create the subnet. Default ismin_prefixlen
-
--default-quota <DEFAULT_QUOTA>
— A per-project quota on the prefix space that can be allocated from the subnet pool for project subnets. Default is no quota is enforced on allocations from the subnet pool. For IPv4 subnet pools,default_quota
is measured in units of /32. For IPv6 subnet pools,default_quota
is measured units of /64. All projects that use the subnet pool have the same prefix quota applied -
--description <DESCRIPTION>
— A human-readable description for the resource. Default is an empty string -
--is-default <IS_DEFAULT>
— The subnetpool is default pool or notPossible values:
true
,false
-
--max-prefixlen <MAX_PREFIXLEN>
— The maximum prefix size that can be allocated from the subnet pool. For IPv4 subnet pools, default is32
. For IPv6 subnet pools, default is128
-
--min-prefixlen <MIN_PREFIXLEN>
— The smallest prefix that can be allocated from a subnet pool. For IPv4 subnet pools, default is8
. For IPv6 subnet pools, default is64
-
--name <NAME>
— Human-readable name of the resource -
--prefixes <PREFIXES>
— A list of subnet prefixes to assign to the subnet pool. The API merges adjacent prefixes and treats them as a single prefix. Each subnet prefix must be unique among all subnet prefixes in all subnet pools that are associated with the address scope.Parameter is an array, may be provided multiple times.
osc network subnetpool show
Shows information for a subnet pool.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Normal response codes: 200
Error response codes: 401, 404
Usage: osc network subnetpool show <ID>
Arguments:
<ID>
— id parameter for /v2.0/subnetpools/{id}/remove_prefixes API
osc network subnetpool tag
Lists tags, creates, replaces or deletes one or more tags for a resource, checks the existence of a tag for a resource
Usage: osc network subnetpool tag <COMMAND>
Available subcommands:
osc network subnetpool tag add
— Command without description in OpenAPIosc network subnetpool tag check
— Command without description in OpenAPIosc network subnetpool tag delete
— Command without description in OpenAPIosc network subnetpool tag list
— Command without description in OpenAPIosc network subnetpool tag purge
— Command without description in OpenAPI
osc network subnetpool tag add
Command without description in OpenAPI
Usage: osc network subnetpool tag add <SUBNETPOOL_ID> <ID>
Arguments:
<SUBNETPOOL_ID>
— subnetpool_id parameter for /v2.0/subnetpools/{subnetpool_id}/tags/{id} API<ID>
— id parameter for /v2.0/subnetpools/{subnetpool_id}/tags/{id} API
osc network subnetpool tag check
Command without description in OpenAPI
Usage: osc network subnetpool tag check <SUBNETPOOL_ID> <ID>
Arguments:
<SUBNETPOOL_ID>
— subnetpool_id parameter for /v2.0/subnetpools/{subnetpool_id}/tags/{id} API<ID>
— id parameter for /v2.0/subnetpools/{subnetpool_id}/tags/{id} API
osc network subnetpool tag delete
Command without description in OpenAPI
Usage: osc network subnetpool tag delete <SUBNETPOOL_ID> <ID>
Arguments:
<SUBNETPOOL_ID>
— subnetpool_id parameter for /v2.0/subnetpools/{subnetpool_id}/tags/{id} API<ID>
— id parameter for /v2.0/subnetpools/{subnetpool_id}/tags/{id} API
osc network subnetpool tag list
Command without description in OpenAPI
Usage: osc network subnetpool tag list [OPTIONS] <SUBNETPOOL_ID>
Arguments:
<SUBNETPOOL_ID>
— subnetpool_id parameter for /v2.0/subnetpools/{subnetpool_id}/tags/{id} API
Options:
-
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network subnetpool tag purge
Command without description in OpenAPI
Usage: osc network subnetpool tag purge <SUBNETPOOL_ID>
Arguments:
<SUBNETPOOL_ID>
— subnetpool_id parameter for /v2.0/subnetpools/{subnetpool_id}/tags/{id} API
osc network vpn
VPNaaS 2.0 (vpn, vpnservices, ikepolicies, ipsecpolicies, endpoint-groups, ipsec-site-connections)
The Virtual-Private-Network-as-a-Service (VPNaaS) extension enables OpenStack projects to extend private networks across the public telecommunication infrastructure.
This initial implementation of the VPNaaS extension provides:
-
Site-to-site VPN that connects two private networks.
-
Multiple VPN connections per project.
-
IKEv1 policy support with 3des, aes-128, aes-256, or aes-192 encryption.
-
IPsec policy support with 3des, aes-128, aes-192, or aes-256 encryption, sha1 authentication, ESP, AH, or AH-ESP transform protocol, and tunnel or transport mode encapsulation.
-
Dead Peer Detection (DPD) with hold, clear, restart, disabled, or restart-by-peer actions.
This extension introduces these resources:
-
service. A parent object that associates VPN with a specific subnet and router.
-
ikepolicy. The Internet Key Exchange (IKE) policy that identifies the authentication and encryption algorithm to use during phase one and two negotiation of a VPN connection.
-
ipsecpolicy. The IP security policy that specifies the authentication and encryption algorithm and encapsulation mode to use for the established VPN connection.
-
ipsec-site-connection. Details for the site-to-site IPsec connection, including the peer CIDRs, MTU, authentication mode, peer address, DPD settings, and status.
VPN Endpoint Groups
The endpoint-groups extension adds support for defining one or more endpoints of a specific type, and can be used to specify both local and peer endpoints for IPsec connections.
VPN Flavors
The vpn-flavors extension adds the flavor_id attribute to vpnservices resources. During vpnservice creation, if a flavor_id is passed, it is used to find the provider for the driver which would handle the newly created vpnservice.
Usage: osc network vpn <COMMAND>
Available subcommands:
osc network vpn endpoint-group
— VPN Endpoint Groupsosc network vpn ikepolicy
— The Internet Key Exchange (IKE) policy that identifies the authentication and encryption algorithm to use during phase one and two negotiation of a VPN connectionosc network vpn ipsec-site-connection
— Details for the site-to-site IPsec connection, including the peer CIDRs, MTU, authentication mode, peer address, DPD settings, and statusosc network vpn ipsecpolicy
— The IP security policy that specifies the authentication and encryption algorithm and encapsulation mode to use for the established VPN connectionosc network vpn vpnservice
— VPN Service - A parent object that associates VPN with a specific subnet and router
osc network vpn endpoint-group
VPN Endpoint Groups
The endpoint-groups extension adds support for defining one or more endpoints of a specific type, and can be used to specify both local and peer endpoints for IPsec connections.
Usage: osc network vpn endpoint-group <COMMAND>
Available subcommands:
osc network vpn endpoint-group create
— Create VPN endpoint grouposc network vpn endpoint-group delete
— Remove VPN endpoint grouposc network vpn endpoint-group list
— List VPN endpoint groupsosc network vpn endpoint-group set
— Update VPN endpoint grouposc network vpn endpoint-group show
— Show VPN endpoint group
osc network vpn endpoint-group create
Creates a VPN endpoint group.
The endpoint group contains one or more endpoints of a specific type that you can use to create a VPN connections.
Normal response codes: 201
Error response codes: 400, 401
Usage: osc network vpn endpoint-group create [OPTIONS]
Options:
-
--description <DESCRIPTION>
— A human-readable description for the resource. Default is an empty string -
--endpoints <ENDPOINTS>
— List of endpoints of the same type, for the endpoint group. The values will depend on type.Parameter is an array, may be provided multiple times.
-
--name <NAME>
— Human-readable name of the resource. Default is an empty string -
--tenant-id <TENANT_ID>
— The ID of the project -
--type <TYPE>
— The type of the endpoints in the group. A valid value issubnet
,cidr
,network
,router
, orvlan
. Onlysubnet
andcidr
are supported at this momentPossible values:
cidr
,network
,router
,subnet
,vlan
osc network vpn endpoint-group delete
Removes a VPN endpoint group.
Normal response codes: 204
Error response codes: 401, 404, 409
Usage: osc network vpn endpoint-group delete <ID>
Arguments:
<ID>
— id parameter for /v2.0/vpn/endpoint-groups/{id} API
osc network vpn endpoint-group list
Lists VPN endpoint groups.
Standard query parameters are supported on the URI. For more information, see Filtering and Column Selection.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Pagination query parameters are supported if Neutron configuration supports it by overriding allow_pagination=false
. For more information, see Pagination.
Sorting query parameters are supported if Neutron configuration supports it with allow_sorting=true
. For more information, see Sorting.
Normal response codes: 200
Error response codes: 401, 403
Usage: osc network vpn endpoint-group list [OPTIONS]
Options:
-
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network vpn endpoint-group set
Updates settings for a VPN endpoint group.
Normal response codes: 200
Error response codes: 400, 401, 404
Usage: osc network vpn endpoint-group set [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.0/vpn/endpoint-groups/{id} API
Options:
--description <DESCRIPTION>
— A human-readable description for the resource. Default is an empty string--name <NAME>
— Human-readable name of the resource. Default is an empty string
osc network vpn endpoint-group show
Shows details for a VPN endpoint group.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Normal response codes: 200
Error response codes: 401, 403, 404
Usage: osc network vpn endpoint-group show <ID>
Arguments:
<ID>
— id parameter for /v2.0/vpn/endpoint-groups/{id} API
osc network vpn ikepolicy
The Internet Key Exchange (IKE) policy that identifies the authentication and encryption algorithm to use during phase one and two negotiation of a VPN connection
Usage: osc network vpn ikepolicy <COMMAND>
Available subcommands:
osc network vpn ikepolicy create
— Create IKE policyosc network vpn ikepolicy delete
— Remove IKE policyosc network vpn ikepolicy list
— List IKE policiesosc network vpn ikepolicy set
— Update IKE policyosc network vpn ikepolicy show
— Show IKE policy details
osc network vpn ikepolicy create
Creates an IKE policy.
The IKE policy is used for phases one and two negotiation of the VPN connection. You can specify both the authentication and encryption algorithms for connections.
Normal response codes: 201
Error response codes: 400, 401
Usage: osc network vpn ikepolicy create [OPTIONS]
Options:
-
--auth-algorithm <AUTH_ALGORITHM>
— The authentication hash algorithm. Valid values aresha1
,sha256
,sha384
,sha512
,aes-xcbc
,aes-cmac
. The default issha1
Possible values:
aes-cmac
,aes-xcbc
,sha1
,sha256
,sha384
,sha512
-
--description <DESCRIPTION>
— A human-readable description for the resource. Default is an empty string -
--encryption-algorithm <ENCRYPTION_ALGORITHM>
— The encryption algorithm. A valid value is3des
,aes-128
,aes-192
,aes-256
,aes-128-ctr
,aes-192-ctr
,aes-256-ctr
. Additional values for AES CCM and GCM modes are defined (e.g.aes-256-ccm-16
,aes-256-gcm-16
) for all combinations of key length 128, 192, 256 bits and ICV length 8, 12, 16 octets. Default isaes-128
Possible values:
3des
,aes128
,aes128-ccm12
,aes128-ccm16
,aes128-ccm8
,aes128-ctr
,aes128-gcm12
,aes128-gcm16
,aes128-gcm8
,aes192
,aes192-ccm12
,aes192-ccm16
,aes192-ccm8
,aes192-ctr
,aes192-gcm12
,aes192-gcm16
,aes192-gcm8
,aes256
,aes256-ccm12
,aes256-ccm16
,aes256-ccm8
,aes256-ctr
,aes256-gcm12
,aes256-gcm16
,aes256-gcm8
-
--ike-version <IKE_VERSION>
— The IKE version. A valid value isv1
orv2
. Default isv1
Possible values:
v1
,v2
-
--lifetime <LIFETIME>
— The lifetime of the security association. The lifetime consists of a unit and integer value. You can omit either the unit or value portion of the lifetime. Default unit is seconds and default value is 3600 -
--name <NAME>
— Human-readable name of the resource. Default is an empty string -
--pfs <PFS>
— Perfect forward secrecy (PFS). A valid value isGroup2
,Group5
,Group14
toGroup31
. Default isGroup5
Possible values:
group14
,group15
,group16
,group17
,group18
,group19
,group2
,group20
,group21
,group22
,group23
,group24
,group25
,group26
,group27
,group28
,group29
,group30
,group31
,group5
-
--phase1-negotiation-mode <PHASE1_NEGOTIATION_MODE>
— The IKE mode. A valid value ismain
, which is the defaultPossible values:
aggressive
,main
-
--tenant-id <TENANT_ID>
— The ID of the project
osc network vpn ikepolicy delete
Removes an IKE policy.
Normal response codes: 204
Error response codes: 401, 404, 409
Usage: osc network vpn ikepolicy delete <ID>
Arguments:
<ID>
— id parameter for /v2.0/vpn/ikepolicies/{id} API
osc network vpn ikepolicy list
Lists IKE policies.
Standard query parameters are supported on the URI. For more information, see Filtering and Column Selection.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Pagination query parameters are supported if Neutron configuration supports it by overriding allow_pagination=false
. For more information, see Pagination.
Sorting query parameters are supported if Neutron configuration supports it with allow_sorting=true
. For more information, see Sorting.
Normal response codes: 200
Error response codes: 401, 403
Usage: osc network vpn ikepolicy list [OPTIONS]
Options:
-
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network vpn ikepolicy set
Updates policy settings in an IKE policy.
Normal response codes: 200
Error response codes: 400, 401, 404
Usage: osc network vpn ikepolicy set [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.0/vpn/ikepolicies/{id} API
Options:
-
--auth-algorithm <AUTH_ALGORITHM>
— The authentication hash algorithm. Valid values aresha1
,sha256
,sha384
,sha512
,aes-xcbc
,aes-cmac
. The default issha1
Possible values:
aes-cmac
,aes-xcbc
,sha1
,sha256
,sha384
,sha512
-
--description <DESCRIPTION>
— A human-readable description for the resource. Default is an empty string -
--encryption-algorithm <ENCRYPTION_ALGORITHM>
— The encryption algorithm. A valid value is3des
,aes-128
,aes-192
,aes-256
,aes-128-ctr
,aes-192-ctr
,aes-256-ctr
. Additional values for AES CCM and GCM modes are defined (e.g.aes-256-ccm-16
,aes-256-gcm-16
) for all combinations of key length 128, 192, 256 bits and ICV length 8, 12, 16 octets. Default isaes-128
Possible values:
3des
,aes128
,aes128-ccm12
,aes128-ccm16
,aes128-ccm8
,aes128-ctr
,aes128-gcm12
,aes128-gcm16
,aes128-gcm8
,aes192
,aes192-ccm12
,aes192-ccm16
,aes192-ccm8
,aes192-ctr
,aes192-gcm12
,aes192-gcm16
,aes192-gcm8
,aes256
,aes256-ccm12
,aes256-ccm16
,aes256-ccm8
,aes256-ctr
,aes256-gcm12
,aes256-gcm16
,aes256-gcm8
-
--ike-version <IKE_VERSION>
— The IKE version. A valid value isv1
orv2
. Default isv1
Possible values:
v1
,v2
-
--lifetime <LIFETIME>
— The lifetime of the security association. The lifetime consists of a unit and integer value. You can omit either the unit or value portion of the lifetime. Default unit is seconds and default value is 3600 -
--name <NAME>
— Human-readable name of the resource. Default is an empty string -
--pfs <PFS>
— Perfect forward secrecy (PFS). A valid value isGroup2
,Group5
,Group14
toGroup31
. Default isGroup5
Possible values:
group14
,group15
,group16
,group17
,group18
,group19
,group2
,group20
,group21
,group22
,group23
,group24
,group25
,group26
,group27
,group28
,group29
,group30
,group31
,group5
-
--phase1-negotiation-mode <PHASE1_NEGOTIATION_MODE>
— The IKE mode. A valid value ismain
, which is the defaultPossible values:
aggressive
,main
osc network vpn ikepolicy show
Shows details for an IKE policy.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Normal response codes: 200
Error response codes: 401, 403, 404
Usage: osc network vpn ikepolicy show <ID>
Arguments:
<ID>
— id parameter for /v2.0/vpn/ikepolicies/{id} API
osc network vpn ipsec-site-connection
Details for the site-to-site IPsec connection, including the peer CIDRs, MTU, authentication mode, peer address, DPD settings, and status
Usage: osc network vpn ipsec-site-connection <COMMAND>
Available subcommands:
osc network vpn ipsec-site-connection create
— Create IPsec connectionosc network vpn ipsec-site-connection delete
— Remove IPsec connectionosc network vpn ipsec-site-connection list
— List IPsec connectionsosc network vpn ipsec-site-connection set
— Update IPsec connectionosc network vpn ipsec-site-connection show
— Show IPsec connection
osc network vpn ipsec-site-connection create
Creates a site-to-site IPsec connection for a service.
Normal response codes: 201
Error response codes: 400, 401
Usage: osc network vpn ipsec-site-connection create [OPTIONS]
Options:
-
--admin-state-up <ADMIN_STATE_UP>
— The administrative state of the resource, which is up (true
) or down (false
)Possible values:
true
,false
-
--description <DESCRIPTION>
— A human-readable description for the resource. Default is an empty string -
--dpd <DPD>
— A dictionary with dead peer detection (DPD) protocol controls -
--ikepolicy-id <IKEPOLICY_ID>
— The ID of the IKE policy -
--initiator <INITIATOR>
— Indicates whether this VPN can only respond to connections or both respond to and initiate connections. A valid value isresponse- only
orbi-directional
. Default isbi-directional
Possible values:
bi-directional
,response-only
-
--ipsecpolicy-id <IPSECPOLICY_ID>
— The ID of the IPsec policy -
--local-ep-group-id <LOCAL_EP_GROUP_ID>
— The ID for the endpoint group that contains private subnets for the local side of the connection. Yo must specify this parameter with thepeer_ep_group_id
parameter unless in backward- compatible mode wherepeer_cidrs
is provided with asubnet_id
for the VPN service -
--local-id <LOCAL_ID>
— An ID to be used instead of the external IP address for a virtual router used in traffic between instances on different networks in east-west traffic. Most often, local ID would be domain name, email address, etc. If this is not configured then the external IP address will be used as the ID -
--mtu <MTU>
— The maximum transmission unit (MTU) value to address fragmentation. Minimum value is 68 for IPv4, and 1280 for IPv6 -
--name <NAME>
— Human-readable name of the resource. Default is an empty string -
--peer-address <PEER_ADDRESS>
— The peer gateway public IPv4 or IPv6 address or FQDN -
--peer-cidrs <PEER_CIDRS>
— (Deprecated) Unique list of valid peer private CIDRs in the form < net_address > / < prefix > .Parameter is an array, may be provided multiple times.
-
--peer-ep-group-id <PEER_EP_GROUP_ID>
— The ID for the endpoint group that contains private CIDRs in the form < net_address > / < prefix > for the peer side of the connection. You must specify this parameter with thelocal_ep_group_id
parameter unless in backward-compatible mode wherepeer_cidrs
is provided with asubnet_id
for the VPN service -
--peer-id <PEER_ID>
— The peer router identity for authentication. A valid value is an IPv4 address, IPv6 address, e-mail address, key ID, or FQDN. Typically, this value matches thepeer_address
value -
--psk <PSK>
— The pre-shared key. A valid value is any string -
--tenant-id <TENANT_ID>
— The ID of the project -
--vpnservice-id <VPNSERVICE_ID>
— The ID of the VPN service
osc network vpn ipsec-site-connection delete
Removes an IPsec connection.
Normal response codes: 204
Error response codes: 401, 404, 409
Usage: osc network vpn ipsec-site-connection delete <ID>
Arguments:
<ID>
— id parameter for /v2.0/vpn/ipsec-site-connections/{id} API
osc network vpn ipsec-site-connection list
Lists all IPsec connections.
Standard query parameters are supported on the URI. For more information, see Filtering and Column Selection.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Pagination query parameters are supported if Neutron configuration supports it by overriding allow_pagination=false
. For more information, see Pagination.
Sorting query parameters are supported if Neutron configuration supports it with allow_sorting=true
. For more information, see Sorting.
Normal response codes: 200
Error response codes: 401, 403
Usage: osc network vpn ipsec-site-connection list [OPTIONS]
Options:
-
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network vpn ipsec-site-connection set
Updates connection settings for an IPsec connection.
Normal response codes: 200
Error response codes: 400, 401, 404
Usage: osc network vpn ipsec-site-connection set [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.0/vpn/ipsec-site-connections/{id} API
Options:
-
--admin-state-up <ADMIN_STATE_UP>
— The administrative state of the resource, which is up (true
) or down (false
)Possible values:
true
,false
-
--description <DESCRIPTION>
— A human-readable description for the resource. Default is an empty string -
--dpd <DPD>
— A dictionary with dead peer detection (DPD) protocol controls -
--initiator <INITIATOR>
— Indicates whether this VPN can only respond to connections or both respond to and initiate connections. A valid value isresponse- only
orbi-directional
. Default isbi-directional
Possible values:
bi-directional
,response-only
-
--local-ep-group-id <LOCAL_EP_GROUP_ID>
— The ID for the endpoint group that contains private subnets for the local side of the connection. Yo must specify this parameter with thepeer_ep_group_id
parameter unless in backward- compatible mode wherepeer_cidrs
is provided with asubnet_id
for the VPN service -
--local-id <LOCAL_ID>
— An ID to be used instead of the external IP address for a virtual router used in traffic between instances on different networks in east-west traffic. Most often, local ID would be domain name, email address, etc. If this is not configured then the external IP address will be used as the ID -
--mtu <MTU>
— The maximum transmission unit (MTU) value to address fragmentation. Minimum value is 68 for IPv4, and 1280 for IPv6 -
--name <NAME>
— Human-readable name of the resource. Default is an empty string -
--peer-address <PEER_ADDRESS>
— The peer gateway public IPv4 or IPv6 address or FQDN -
--peer-cidrs <PEER_CIDRS>
— (Deprecated) Unique list of valid peer private CIDRs in the form < net_address > / < prefix > .Parameter is an array, may be provided multiple times.
-
--peer-ep-group-id <PEER_EP_GROUP_ID>
— The ID for the endpoint group that contains private CIDRs in the form < net_address > / < prefix > for the peer side of the connection. You must specify this parameter with thelocal_ep_group_id
parameter unless in backward-compatible mode wherepeer_cidrs
is provided with asubnet_id
for the VPN service -
--peer-id <PEER_ID>
— The peer router identity for authentication. A valid value is an IPv4 address, IPv6 address, e-mail address, key ID, or FQDN. Typically, this value matches thepeer_address
value -
--psk <PSK>
— The pre-shared key. A valid value is any string
osc network vpn ipsec-site-connection show
Shows details for an IPsec connection.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Normal response codes: 200
Error response codes: 401, 403, 404
Usage: osc network vpn ipsec-site-connection show <ID>
Arguments:
<ID>
— id parameter for /v2.0/vpn/ipsec-site-connections/{id} API
osc network vpn ipsecpolicy
The IP security policy that specifies the authentication and encryption algorithm and encapsulation mode to use for the established VPN connection
Usage: osc network vpn ipsecpolicy <COMMAND>
Available subcommands:
osc network vpn ipsecpolicy create
— Create IPsec policyosc network vpn ipsecpolicy delete
— Remove IPsec policyosc network vpn ipsecpolicy list
— List IPsec policiesosc network vpn ipsecpolicy set
— Update IPsec policyosc network vpn ipsecpolicy show
— Show IPsec policy
osc network vpn ipsecpolicy create
Creates an IP security (IPsec) policy.
The IPsec policy specifies the authentication and encryption algorithms and encapsulation mode to use for the established VPN connection.
Normal response codes: 201
Error response codes: 400, 401
Usage: osc network vpn ipsecpolicy create [OPTIONS]
Options:
-
--auth-algorithm <AUTH_ALGORITHM>
— The authentication hash algorithm. Valid values aresha1
,sha256
,sha384
,sha512
,aes-xcbc
,aes-cmac
. The default issha1
Possible values:
aes-cmac
,aes-xcbc
,sha1
,sha256
,sha384
,sha512
-
--description <DESCRIPTION>
— A human-readable description for the resource. Default is an empty string -
--encapsulation-mode <ENCAPSULATION_MODE>
— The encapsulation mode. A valid value istunnel
ortransport
. Default istunnel
Possible values:
transport
,tunnel
-
--encryption-algorithm <ENCRYPTION_ALGORITHM>
— The encryption algorithm. A valid value is3des
,aes-128
,aes-192
,aes-256
,aes-128-ctr
,aes-192-ctr
,aes-256-ctr
. Additional values for AES CCM and GCM modes are defined (e.g.aes-256-ccm-16
,aes-256-gcm-16
) for all combinations of key length 128, 192, 256 bits and ICV length 8, 12, 16 octets. Default isaes-128
Possible values:
3des
,aes128
,aes128-ccm12
,aes128-ccm16
,aes128-ccm8
,aes128-ctr
,aes128-gcm12
,aes128-gcm16
,aes128-gcm8
,aes192
,aes192-ccm12
,aes192-ccm16
,aes192-ccm8
,aes192-ctr
,aes192-gcm12
,aes192-gcm16
,aes192-gcm8
,aes256
,aes256-ccm12
,aes256-ccm16
,aes256-ccm8
,aes256-ctr
,aes256-gcm12
,aes256-gcm16
,aes256-gcm8
-
--lifetime <LIFETIME>
— The lifetime of the security association. The lifetime consists of a unit and integer value. You can omit either the unit or value portion of the lifetime. Default unit is seconds and default value is 3600 -
--name <NAME>
— Human-readable name of the resource. Default is an empty string -
--pfs <PFS>
— Perfect forward secrecy (PFS). A valid value isGroup2
,Group5
,Group14
toGroup31
. Default isGroup5
Possible values:
group14
,group15
,group16
,group17
,group18
,group19
,group2
,group20
,group21
,group22
,group23
,group24
,group25
,group26
,group27
,group28
,group29
,group30
,group31
,group5
-
--tenant-id <TENANT_ID>
— The ID of the project -
--transform-protocol <TRANSFORM_PROTOCOL>
— The transform protocol. A valid value isESP
,AH
, orAH- ESP
. Default isESP
Possible values:
ah
,ah-esp
,esp
osc network vpn ipsecpolicy delete
Removes an IPsec policy.
Normal response codes: 204
Error response codes: 401, 404, 409
Usage: osc network vpn ipsecpolicy delete <ID>
Arguments:
<ID>
— id parameter for /v2.0/vpn/ipsecpolicies/{id} API
osc network vpn ipsecpolicy list
Lists all IPsec policies.
Standard query parameters are supported on the URI. For more information, see Filtering and Column Selection.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Pagination query parameters are supported if Neutron configuration supports it by overriding allow_pagination=false
. For more information, see Pagination.
Sorting query parameters are supported if Neutron configuration supports it with allow_sorting=true
. For more information, see Sorting.
Normal response codes: 200
Error response codes: 401, 403
Usage: osc network vpn ipsecpolicy list [OPTIONS]
Options:
-
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network vpn ipsecpolicy set
Updates policy settings in an IPsec policy.
Normal response codes: 200
Error response codes: 400, 401, 404
Usage: osc network vpn ipsecpolicy set [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.0/vpn/ipsecpolicies/{id} API
Options:
-
--auth-algorithm <AUTH_ALGORITHM>
— The authentication hash algorithm. Valid values aresha1
,sha256
,sha384
,sha512
,aes-xcbc
,aes-cmac
. The default issha1
Possible values:
aes-cmac
,aes-xcbc
,sha1
,sha256
,sha384
,sha512
-
--description <DESCRIPTION>
— A human-readable description for the resource. Default is an empty string -
--encapsulation-mode <ENCAPSULATION_MODE>
— The encapsulation mode. A valid value istunnel
ortransport
. Default istunnel
Possible values:
transport
,tunnel
-
--encryption-algorithm <ENCRYPTION_ALGORITHM>
— The encryption algorithm. A valid value is3des
,aes-128
,aes-192
,aes-256
,aes-128-ctr
,aes-192-ctr
,aes-256-ctr
. Additional values for AES CCM and GCM modes are defined (e.g.aes-256-ccm-16
,aes-256-gcm-16
) for all combinations of key length 128, 192, 256 bits and ICV length 8, 12, 16 octets. Default isaes-128
Possible values:
3des
,aes128
,aes128-ccm12
,aes128-ccm16
,aes128-ccm8
,aes128-ctr
,aes128-gcm12
,aes128-gcm16
,aes128-gcm8
,aes192
,aes192-ccm12
,aes192-ccm16
,aes192-ccm8
,aes192-ctr
,aes192-gcm12
,aes192-gcm16
,aes192-gcm8
,aes256
,aes256-ccm12
,aes256-ccm16
,aes256-ccm8
,aes256-ctr
,aes256-gcm12
,aes256-gcm16
,aes256-gcm8
-
--lifetime <LIFETIME>
— The lifetime of the security association. The lifetime consists of a unit and integer value. You can omit either the unit or value portion of the lifetime. Default unit is seconds and default value is 3600 -
--name <NAME>
— Human-readable name of the resource. Default is an empty string -
--pfs <PFS>
— Perfect forward secrecy (PFS). A valid value isGroup2
,Group5
,Group14
toGroup31
. Default isGroup5
Possible values:
group14
,group15
,group16
,group17
,group18
,group19
,group2
,group20
,group21
,group22
,group23
,group24
,group25
,group26
,group27
,group28
,group29
,group30
,group31
,group5
-
--transform-protocol <TRANSFORM_PROTOCOL>
— The transform protocol. A valid value isESP
,AH
, orAH- ESP
. Default isESP
Possible values:
ah
,ah-esp
,esp
osc network vpn ipsecpolicy show
Shows details for an IPsec policy.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Normal response codes: 200
Error response codes: 401, 403, 404
Usage: osc network vpn ipsecpolicy show <ID>
Arguments:
<ID>
— id parameter for /v2.0/vpn/ipsecpolicies/{id} API
osc network vpn vpnservice
VPN Service - A parent object that associates VPN with a specific subnet and router
Usage: osc network vpn vpnservice <COMMAND>
Available subcommands:
osc network vpn vpnservice create
— Create VPN serviceosc network vpn vpnservice delete
— Remove VPN serviceosc network vpn vpnservice list
— List VPN servicesosc network vpn vpnservice set
— Update VPN serviceosc network vpn vpnservice show
— Show VPN service details
osc network vpn vpnservice create
Creates a VPN service.
The service is associated with a router. After you create the service, it can contain multiple VPN connections.
An optional flavor_id
attribute can be passed to enable dynamic selection of an appropriate provider if configured by the operator. It is only available when vpn-flavors
extension is enabled. The basic selection algorithm chooses the provider in the first service profile currently associated with flavor. This option can only be set in POST
operation.
Normal response codes: 201
Error response codes: 400, 401
Usage: osc network vpn vpnservice create [OPTIONS]
Options:
-
--admin-state-up <ADMIN_STATE_UP>
— The administrative state of the resource, which is up (true
) or down (false
)Possible values:
true
,false
-
--description <DESCRIPTION>
— A human-readable description for the resource. Default is an empty string -
--flavor-id <FLAVOR_ID>
— The ID of the flavor -
--name <NAME>
— Human-readable name of the resource. Default is an empty string -
--router-id <ROUTER_ID>
-
--subnet-id <SUBNET_ID>
— If you specify only a subnet UUID, OpenStack Networking allocates an available IP from that subnet to the port. If you specify both a subnet UUID and an IP address, OpenStack Networking tries to allocate the address to the port -
--tenant-id <TENANT_ID>
— The ID of the project
osc network vpn vpnservice delete
Removes a VPN service.
If the service has connections, the request is rejected.
Normal response codes: 204
Error response codes: 401, 404, 409
Usage: osc network vpn vpnservice delete <ID>
Arguments:
<ID>
— id parameter for /v2.0/vpn/vpnservices/{id} API
osc network vpn vpnservice list
Lists all VPN services.
The list might be empty.
Standard query parameters are supported on the URI. For more information, see Filtering and Column Selection.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Pagination query parameters are supported if Neutron configuration supports it by overriding allow_pagination=false
. For more information, see Pagination.
Sorting query parameters are supported if Neutron configuration supports it with allow_sorting=true
. For more information, see Sorting.
Normal response codes: 200
Error response codes: 401, 403
Usage: osc network vpn vpnservice list [OPTIONS]
Options:
-
--limit <LIMIT>
— Requests a page size of items. Returns a number of items up to a limit value. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--marker <MARKER>
— The ID of the last-seen item. Use the limit parameter to make an initial limited request and use the ID of the last-seen item from the response as the marker parameter value in a subsequent limited request -
--page-reverse <PAGE_REVERSE>
— Reverse the page directionPossible values:
true
,false
-
--sort-dir <SORT_DIR>
— Sort direction. This is an optional feature and may be silently ignored by the server -
--sort-key <SORT_KEY>
— Sort results by the attribute. This is an optional feature and may be silently ignored by the server -
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc network vpn vpnservice set
Updates a VPN service.
Updates the attributes of a VPN service. You cannot update a service with a PENDING_*
status.
Normal response codes: 200
Error response codes: 400, 401, 404
Usage: osc network vpn vpnservice set [OPTIONS] <ID>
Arguments:
<ID>
— id parameter for /v2.0/vpn/vpnservices/{id} API
Options:
-
--admin-state-up <ADMIN_STATE_UP>
— The administrative state of the resource, which is up (true
) or down (false
)Possible values:
true
,false
-
--description <DESCRIPTION>
— A human-readable description for the resource. Default is an empty string -
--name <NAME>
— Human-readable name of the resource. Default is an empty string
osc network vpn vpnservice show
Shows details for a VPN service.
If the user is not an administrative user and the VPN service object does not belong to the tenant account for the user, the operation returns the Forbidden (403)
response code.
Use the fields
query parameter to control which fields are returned in the response body. For more information, see Fields.
Normal response codes: 200
Error response codes: 401, 403, 404
Usage: osc network vpn vpnservice show <ID>
Arguments:
<ID>
— id parameter for /v2.0/vpn/vpnservices/{id} API
osc object-store
Object Store service (Swift) commands
Usage: osc object-store <COMMAND>
Available subcommands:
osc object-store account
— Account commandsosc object-store container
— Container commandsosc object-store object
— Object commands
osc object-store account
Account commands
Usage: osc object-store account <COMMAND>
Available subcommands:
osc object-store account show
— Shows metadata for an account. Because the storage system can store large amounts of data, take care when you represent the total bytes response as an integer; when possible, convert it to a 64-bit unsigned integer if your platform supports that primitive type. Do not include metadata headers in this requestosc object-store account set
— Creates, updates, or deletes account metadata. To create, update, or delete custom metadata, use the X-Account-Meta-{name} request header, where {name} is the name of the metadata item. Account metadata operations work differently than how object metadata operations work. Depending on the contents of your POST account metadata request, the Object Storage API updates the metadata as shown in the following table: TODO: fill the rest To delete a metadata header, send an empty value for that header, such as for the X-Account-Meta-Book header. If the tool you use to communicate with Object Storage, such as an older version of cURL, does not support empty headers, send the X-Remove-Account- Meta-{name} header with an arbitrary value. For example, X-Remove-Account-Meta-Book: x. The operation ignores the arbitrary value
osc object-store account show
Shows metadata for an account. Because the storage system can store large amounts of data, take care when you represent the total bytes response as an integer; when possible, convert it to a 64-bit unsigned integer if your platform supports that primitive type. Do not include metadata headers in this request
Usage: osc object-store account show
osc object-store account set
Creates, updates, or deletes account metadata. To create, update, or delete custom metadata, use the X-Account-Meta-{name} request header, where {name} is the name of the metadata item. Account metadata operations work differently than how object metadata operations work. Depending on the contents of your POST account metadata request, the Object Storage API updates the metadata as shown in the following table: TODO: fill the rest To delete a metadata header, send an empty value for that header, such as for the X-Account-Meta-Book header. If the tool you use to communicate with Object Storage, such as an older version of cURL, does not support empty headers, send the X-Remove-Account- Meta-{name} header with an arbitrary value. For example, X-Remove-Account-Meta-Book: x. The operation ignores the arbitrary value
Usage: osc object-store account set [OPTIONS]
Options:
--property <key=value>
— Property to be set
osc object-store container
Container commands
Usage: osc object-store container <COMMAND>
Available subcommands:
osc object-store container create
— Creates a container. You do not need to check whether a container already exists before issuing a PUT operation because the operation is idempotent: It creates a container or updates an existing container, as appropriateosc object-store container delete
— Deletes an empty container. This operation fails unless the container is empty. An empty container has no objectsosc object-store container list
— Shows details for an account and lists containers, sorted by name, in the accountosc object-store container prune
— Prune objects in a containerosc object-store container set
— Creates, updates, or deletes custom metadata for a containerosc object-store container show
— Shows container metadata, including the number of objects and the total bytes of all objects stored in the container
osc object-store container create
Creates a container. You do not need to check whether a container already exists before issuing a PUT operation because the operation is idempotent: It creates a container or updates an existing container, as appropriate
Usage: osc object-store container create <CONTAINER>
Arguments:
<CONTAINER>
— The unique (within an account) name for the container. The container name must be from 1 to 256 characters long and can start with any character and contain any pattern. Character set must be UTF-8. The container name cannot contain a slash (/) character because this character delimits the container and object name. For example, the path /v1/account/www/pages specifies the www container, not the www/pages container
osc object-store container delete
Deletes an empty container. This operation fails unless the container is empty. An empty container has no objects
Usage: osc object-store container delete <CONTAINER>
Arguments:
<CONTAINER>
— The unique (within an account) name for the container. The container name must be from 1 to 256 characters long and can start with any character and contain any pattern. Character set must be UTF-8. The container name cannot contain a slash (/) character because this character delimits the container and object name. For example, the path /v1/account/www/pages specifies the www container, not the www/pages container
osc object-store container list
Shows details for an account and lists containers, sorted by name, in the account
Usage: osc object-store container list [OPTIONS]
Options:
-
--limit <LIMIT>
— For an integer value n, limits the number of results to n -
--marker <MARKER>
— For a string value, x, constrains the list to items whose names are greater than x -
--end-marker <END_MARKER>
— For a string value, x, constrains the list to items whose names are less than x -
--format <FORMAT>
— The response format. Valid values are json, xml, or plain. The default is plain. If you append the format=xml or format=json query parameter to the storage account URL, the response shows extended container information serialized in that format. If you append the format=plain query parameter, the response lists the container names separated by newlines -
--prefix <PREFIX>
— Only objects with this prefix will be returned. When combined with a delimiter query, this enables API users to simulate and traverse the objects in a container as if they were in a directory tree -
--delimiter <DELIMITER>
— The delimiter is a single character used to split object names to present a pseudo-directory hierarchy of objects. When combined with a prefix query, this enables API users to simulate and traverse the objects in a container as if they were in a directory tree -
--reverse <REVERSE>
— By default, listings are returned sorted by name, ascending. If you include the reverse=true query parameter, the listing will be returned sorted by name, descendingPossible values:
true
,false
-
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc object-store container prune
Prune objects in a container
Usage: osc object-store container prune [OPTIONS] <CONTAINER>
Arguments:
<CONTAINER>
— The unique (within an account) name for the container. The container name must be from 1 to 256 characters long and can start with any character and contain any pattern. Character set must be UTF-8. The container name cannot contain a slash (/) character because this character delimits the container and object name
Options:
--prefix <PREFIX>
— Only objects with this prefix will be deleted
osc object-store container set
Creates, updates, or deletes custom metadata for a container
Usage: osc object-store container set [OPTIONS] <CONTAINER>
Arguments:
<CONTAINER>
— The unique (within an account) name for the container. The container name must be from 1 to 256 characters long and can start with any character and contain any pattern. Character set must be UTF-8. The container name cannot contain a slash (/) character because this character delimits the container and object name. For example, the path /v1/account/www/pages specifies the www container, not the www/pages container
Options:
--property <key=value>
— Property to be set
osc object-store container show
Shows container metadata, including the number of objects and the total bytes of all objects stored in the container
Usage: osc object-store container show <CONTAINER>
Arguments:
<CONTAINER>
— The unique (within an account) name for the container. The container name must be from 1 to 256 characters long and can start with any character and contain any pattern. Character set must be UTF-8. The container name cannot contain a slash (/) character because this character delimits the container and object name. For example, the path /v1/account/www/pages specifies the www container, not the www/pages container
osc object-store object
Object commands
Usage: osc object-store object <COMMAND>
Available subcommands:
osc object-store object delete
— Permanently deletes an object from the object store. Object deletion occurs immediately at request time. Any subsequent GET, HEAD, POST, or DELETE operations will return a 404 Not Found error code. For static large object manifests, you can add the ?multipart- manifest=delete query parameter. This operation deletes the segment objects and, if all deletions succeed, this operation deletes the manifest object. A DELETE request made to a symlink path will delete the symlink rather than the target object. An alternative to using the DELETE operation is to use the POST operation with the bulk-delete query parameterosc object-store object download
— Downloads the object content and gets the object metadata. This operation returns the object metadata in the response headers and the object content in the response bodyosc object-store object list
— Shows details for a container and lists objects, sorted by name, in the container. Specify query parameters in the request to filter the list and return a subset of objects. Omit query parameters to return a list of objects that are stored in the container, up to 10,000 names. The 10,000 maximum value is configurable. To view the value for the cluster, issue a GET /info requestosc object-store object show
— Shows object metadataosc object-store object upload
— Creates an object with data content and metadata, or replaces an existing object with data content and metadata. The PUT operation always creates an object. If you use this operation on an existing object, you replace the existing object and metadata rather than modifying the object. Consequently, this operation returns the Created (201) response code. If you use this operation to copy a manifest object, the new object is a normal object and not a copy of the manifest. Instead it is a concatenation of all the segment objects. This means that you cannot copy objects larger than 5 GB. Note that the provider may have limited the characters which are allowed in an object name. Any name limits are exposed under the name_check key in the /info discoverability response. Regardless of name_check limitations, names must be URL quoted UTF-8. To create custom metadata, use the X-Object-Meta-name header, where name is the name of the metadata item
osc object-store object delete
Permanently deletes an object from the object store. Object deletion occurs immediately at request time. Any subsequent GET, HEAD, POST, or DELETE operations will return a 404 Not Found error code. For static large object manifests, you can add the ?multipart- manifest=delete query parameter. This operation deletes the segment objects and, if all deletions succeed, this operation deletes the manifest object. A DELETE request made to a symlink path will delete the symlink rather than the target object. An alternative to using the DELETE operation is to use the POST operation with the bulk-delete query parameter
Usage: osc object-store object delete [OPTIONS] <CONTAINER> <OBJECT>
Arguments:
<CONTAINER>
— The unique name for the account. An account is also known as the project or tenant<OBJECT>
— The unique name for the object
Options:
--multipart-manifest <MULTIPART_MANIFEST>
— If you include the multipart-manifest=get query parameter and the object is a large object, the object contents are not returned. Instead, the manifest is returned in the X-Object-Manifest response header for dynamic large objects or in the response body for static large objects
osc object-store object download
Downloads the object content and gets the object metadata. This operation returns the object metadata in the response headers and the object content in the response body
Usage: osc object-store object download [OPTIONS] <CONTAINER> <OBJECT>
Arguments:
<CONTAINER>
— The unique name for the account. An account is also known as the project or tenant<OBJECT>
— The unique name for the object
Options:
--multipart-manifest <MULTIPART_MANIFEST>
— If you include the multipart-manifest=get query parameter and the object is a large object, the object contents are not returned. Instead, the manifest is returned in the X-Object-Manifest response header for dynamic large objects or in the response body for static large objects--temp-url-sig <TEMP_URL_SIG>
— Used with temporary URLs to sign the request with an HMAC-SHA1 cryptographic signature that defines the allowed HTTP method, expiration date, full path to the object, and the secret key for the temporary URL. For more information about temporary URLs, see Temporary URL middleware--temp-url-expires <TEMP_URL_EXPIRES>
— The date and time in UNIX Epoch time stamp format or ISO 8601 UTC timestamp when the signature for temporary URLs expires. For example, 1440619048 or 2015-08-26T19:57:28Z is equivalent to Mon, Wed, 26 Aug 2015 19:57:28 GMT. For more information about temporary URLs, see Temporary URL middleware--filename <FILENAME>
— Overrides the default file name. Object Storage generates a default file name for GET temporary URLs that is based on the object name. Object Storage returns this value in the Content-Disposition response header. Browsers can interpret this file name value as a file attachment to save. For more information about temporary URLs, see Temporary URL middleware--symlink <SYMLINK>
— If you include the symlink=get query parameter and the object is a symlink, then the response will include data and metadata from the symlink itself rather than from the target--file <FILE>
— Destination filename (using "-" will print object to stdout)
osc object-store object list
Shows details for a container and lists objects, sorted by name, in the container. Specify query parameters in the request to filter the list and return a subset of objects. Omit query parameters to return a list of objects that are stored in the container, up to 10,000 names. The 10,000 maximum value is configurable. To view the value for the cluster, issue a GET /info request
Usage: osc object-store object list [OPTIONS] <CONTAINER>
Arguments:
<CONTAINER>
— The unique (within an account) name for the container. The container name must be from 1 to 256 characters long and can start with any character and contain any pattern. Character set must be UTF-8. The container name cannot contain a slash (/) character because this character delimits the container and object name. For example, the path /v1/account/www/pages specifies the www container, not the www/pages container
Options:
-
--limit <LIMIT>
— For an integer value n, limits the number of results to n -
--marker <MARKER>
— For a string value, x, constrains the list to items whose names are greater than x -
--end-marker <END_MARKER>
— For a string value, x, constrains the list to items whose names are less than x -
--format <FORMAT>
— The response format. Valid values are json, xml, or plain. The default is plain. If you append the format=xml or format=json query parameter to the storage account URL, the response shows extended container information serialized in that format. If you append the format=plain query parameter, the response lists the container names separated by newlines -
--prefix <PREFIX>
— Only objects with this prefix will be returned. When combined with a delimiter query, this enables API users to simulate and traverse the objects in a container as if they were in a directory tree -
--delimiter <DELIMITER>
— The delimiter is a single character used to split object names to present a pseudo-directory hierarchy of objects. When combined with a prefix query, this enables API users to simulate and traverse the objects in a container as if they were in a directory tree -
--reverse <REVERSE>
— By default, listings are returned sorted by name, ascending. If you include the reverse=true query parameter, the listing will be returned sorted by name, descendingPossible values:
true
,false
-
--max-items <MAX_ITEMS>
— Total limit of entities count to return. Use this when there are too many entriesDefault value:
10000
osc object-store object show
Shows object metadata
Usage: osc object-store object show [OPTIONS] <CONTAINER> <OBJECT>
Arguments:
<CONTAINER>
— The unique name for the account. An account is also known as the project or tenant<OBJECT>
— The unique name for the object
Options:
--multipart-manifest <MULTIPART_MANIFEST>
— If you include the multipart-manifest=get query parameter and the object is a large object, the object contents are not returned. Instead, the manifest is returned in the X-Object-Manifest response header for dynamic large objects or in the response body for static large objects--temp-url-sig <TEMP_URL_SIG>
— Used with temporary URLs to sign the request with an HMAC-SHA1 cryptographic signature that defines the allowed HTTP method, expiration date, full path to the object, and the secret key for the temporary URL. For more information about temporary URLs, see Temporary URL middleware--temp-url-expires <TEMP_URL_EXPIRES>
— The date and time in UNIX Epoch time stamp format or ISO 8601 UTC timestamp when the signature for temporary URLs expires. For example, 1440619048 or 2015-08-26T19:57:28Z is equivalent to Mon, Wed, 26 Aug 2015 19:57:28 GMT. For more information about temporary URLs, see Temporary URL middleware--filename <FILENAME>
— Overrides the default file name. Object Storage generates a default file name for GET temporary URLs that is based on the object name. Object Storage returns this value in the Content-Disposition response header. Browsers can interpret this file name value as a file attachment to save. For more information about temporary URLs, see Temporary URL middleware--symlink <SYMLINK>
— If you include the symlink=get query parameter and the object is a symlink, then the response will include data and metadata from the symlink itself rather than from the target
osc object-store object upload
Creates an object with data content and metadata, or replaces an existing object with data content and metadata. The PUT operation always creates an object. If you use this operation on an existing object, you replace the existing object and metadata rather than modifying the object. Consequently, this operation returns the Created (201) response code. If you use this operation to copy a manifest object, the new object is a normal object and not a copy of the manifest. Instead it is a concatenation of all the segment objects. This means that you cannot copy objects larger than 5 GB. Note that the provider may have limited the characters which are allowed in an object name. Any name limits are exposed under the name_check key in the /info discoverability response. Regardless of name_check limitations, names must be URL quoted UTF-8. To create custom metadata, use the X-Object-Meta-name header, where name is the name of the metadata item
Usage: osc object-store object upload [OPTIONS] <CONTAINER> <OBJECT>
Arguments:
<CONTAINER>
— The unique name for the account. An account is also known as the project or tenant<OBJECT>
— The unique name for the object
Options:
--multipart-manifest <MULTIPART_MANIFEST>
— If you include the multipart-manifest=get query parameter and the object is a large object, the object contents are not returned. Instead, the manifest is returned in the X-Object-Manifest response header for dynamic large objects or in the response body for static large objects--temp-url-sig <TEMP_URL_SIG>
— Used with temporary URLs to sign the request with an HMAC-SHA1 cryptographic signature that defines the allowed HTTP method, expiration date, full path to the object, and the secret key for the temporary URL. For more information about temporary URLs, see Temporary URL middleware--temp-url-expires <TEMP_URL_EXPIRES>
— The date and time in UNIX Epoch time stamp format or ISO 8601 UTC timestamp when the signature for temporary URLs expires. For example, 1440619048 or 2015-08-26T19:57:28Z is equivalent to Mon, Wed, 26 Aug 2015 19:57:28 GMT. For more information about temporary URLs, see Temporary URL middleware--filename <FILENAME>
— Overrides the default file name. Object Storage generates a default file name for GET temporary URLs that is based on the object name. Object Storage returns this value in the Content-Disposition response header. Browsers can interpret this file name value as a file attachment to save. For more information about temporary URLs, see Temporary URL middleware--symlink <SYMLINK>
— If you include the symlink=get query parameter and the object is a symlink, then the response will include data and metadata from the symlink itself rather than from the target--file <FILE>
— Source filename (using "-" will read object from stdout)
osc placement
The placement API service was introduced in the 14.0.0 Newton release within the nova repository and extracted to the placement repository in the 19.0.0 Stein release. This is a REST API stack and data model used to track resource provider inventories and usages, along with different classes of resources. For example, a resource provider can be a compute node, a shared storage pool, or an IP allocation pool. The placement service tracks the inventory and usage of each provider. For example, an instance created on a compute node may be a consumer of resources such as RAM and CPU from a compute node resource provider, disk from an external shared storage pool resource provider and IP addresses from an external IP pool resource provider.
The types of resources consumed are tracked as classes. The service provides a set of standard resource classes (for example DISK_GB, MEMORY_MB, and VCPU) and provides the ability to define custom resource classes as needed.
Each resource provider may also have a set of traits which describe qualitative aspects of the resource provider. Traits describe an aspect of a resource provider that cannot itself be consumed but a workload may wish to specify. For example, available disk may be solid state drives (SSD).
Usage: osc placement <COMMAND>
Available subcommands:
osc placement allocation
— Allocationsosc placement allocation-candidate
— Allocation candidatesosc placement reshaper
— Reshaperosc placement resource-class
— Resource Classosc placement resource-provider
— Resource Providersosc placement trait
— Traitsosc placement usage
— Usages
osc placement allocation
Allocations
Allocations are records representing resources that have been assigned and used by some consumer of that resource. They indicate the amount of a particular resource that has been allocated to a given consumer of that resource from a particular resource provider.
Usage: osc placement allocation <COMMAND>
Available subcommands:
osc placement allocation create138
— Manage allocations (microversion = 1.38)osc placement allocation create134
— Manage allocations (microversion = 1.34)osc placement allocation create128
— Manage allocations (microversion = 1.28)osc placement allocation create113
— Manage allocations (microversion = 1.13)osc placement allocation delete
— Delete allocationsosc placement allocation set138
— Update allocations (microversion = 1.38)osc placement allocation set128
— Update allocations (microversion = 1.28)osc placement allocation set112
— Update allocations (microversion = 1.12)osc placement allocation set18
— Update allocations (microversion = 1.8)osc placement allocation set10
— Update allocations (microversion = 1.0)osc placement allocation show
— List allocations
osc placement allocation create138
Create, update or delete allocations for multiple consumers in a single request. This allows a client to atomically set or swap allocations for multiple consumers as may be required during a migration or move type operation.
The allocations for an individual consumer uuid mentioned in the request can be removed by setting the allocations to an empty object (see the example below).
Available as of microversion 1.13.
Normal response codes: 204
Error response codes: badRequest(400), conflict(409)
Usage: osc placement allocation create138 [OPTIONS]
Options:
--property <key=value>
osc placement allocation create134
Create, update or delete allocations for multiple consumers in a single request. This allows a client to atomically set or swap allocations for multiple consumers as may be required during a migration or move type operation.
The allocations for an individual consumer uuid mentioned in the request can be removed by setting the allocations to an empty object (see the example below).
Available as of microversion 1.13.
Normal response codes: 204
Error response codes: badRequest(400), conflict(409)
Usage: osc placement allocation create134 [OPTIONS]
Options:
--property <key=value>
osc placement allocation create128
Create, update or delete allocations for multiple consumers in a single request. This allows a client to atomically set or swap allocations for multiple consumers as may be required during a migration or move type operation.
The allocations for an individual consumer uuid mentioned in the request can be removed by setting the allocations to an empty object (see the example below).
Available as of microversion 1.13.
Normal response codes: 204
Error response codes: badRequest(400), conflict(409)
Usage: osc placement allocation create128 [OPTIONS]
Options:
--property <key=value>
osc placement allocation create113
Create, update or delete allocations for multiple consumers in a single request. This allows a client to atomically set or swap allocations for multiple consumers as may be required during a migration or move type operation.
The allocations for an individual consumer uuid mentioned in the request can be removed by setting the allocations to an empty object (see the example below).
Available as of microversion 1.13.
Normal response codes: 204
Error response codes: badRequest(400), conflict(409)
Usage: osc placement allocation create113 [OPTIONS]
Options:
--property <key=value>
osc placement allocation delete
Delete all allocation records for the consumer identified by {consumer_uuid} on all resource providers it is consuming.
Normal Response Codes: 204
Error response codes: itemNotFound(404)
Usage: osc placement allocation delete <CONSUMER_UUID>
Arguments:
<CONSUMER_UUID>
— consumer_uuid parameter for /allocations/{consumer_uuid} API
osc placement allocation set138
Create or update one or more allocation records representing the consumption of one or more classes of resources from one or more resource providers by the consumer identified by {consumer_uuid}. If allocations already exist for this consumer, they are replaced.
Normal Response Codes: 204
Error response codes: badRequest(400), itemNotFound(404), conflict(409)
Usage: osc placement allocation set138 [OPTIONS] --consumer-type <CONSUMER_TYPE> --project-id <PROJECT_ID> --user-id <USER_ID> <CONSUMER_UUID>
Arguments:
<CONSUMER_UUID>
— consumer_uuid parameter for /allocations/{consumer_uuid} API
Options:
--allocations <key=value>
--consumer-generation <CONSUMER_GENERATION>
--consumer-type <CONSUMER_TYPE>
--mappings <key=value>
--project-id <PROJECT_ID>
--user-id <USER_ID>
osc placement allocation set128
Create or update one or more allocation records representing the consumption of one or more classes of resources from one or more resource providers by the consumer identified by {consumer_uuid}. If allocations already exist for this consumer, they are replaced.
Normal Response Codes: 204
Error response codes: badRequest(400), itemNotFound(404), conflict(409)
Usage: osc placement allocation set128 [OPTIONS] --project-id <PROJECT_ID> --user-id <USER_ID> <CONSUMER_UUID>
Arguments:
<CONSUMER_UUID>
— consumer_uuid parameter for /allocations/{consumer_uuid} API
Options:
--allocations <key=value>
--consumer-generation <CONSUMER_GENERATION>
--project-id <PROJECT_ID>
--user-id <USER_ID>
osc placement allocation set112
Create or update one or more allocation records representing the consumption of one or more classes of resources from one or more resource providers by the consumer identified by {consumer_uuid}. If allocations already exist for this consumer, they are replaced.
Normal Response Codes: 204
Error response codes: badRequest(400), itemNotFound(404), conflict(409)
Usage: osc placement allocation set112 [OPTIONS] --project-id <PROJECT_ID> --user-id <USER_ID> <CONSUMER_UUID>
Arguments:
<CONSUMER_UUID>
— consumer_uuid parameter for /allocations/{consumer_uuid} API
Options:
--allocations <key=value>
--project-id <PROJECT_ID>
--user-id <USER_ID>
osc placement allocation set18
Create or update one or more allocation records representing the consumption of one or more classes of resources from one or more resource providers by the consumer identified by {consumer_uuid}. If allocations already exist for this consumer, they are replaced.
Normal Response Codes: 204
Error response codes: badRequest(400), itemNotFound(404), conflict(409)
Usage: osc placement allocation set18 [OPTIONS] --project-id <PROJECT_ID> --user-id <USER_ID> <CONSUMER_UUID>
Arguments:
<CONSUMER_UUID>
— consumer_uuid parameter for /allocations/{consumer_uuid} API
Options:
--allocations <JSON>
— Parameter is an array, may be provided multiple times--project-id <PROJECT_ID>
--user-id <USER_ID>
osc placement allocation set10
Create or update one or more allocation records representing the consumption of one or more classes of resources from one or more resource providers by the consumer identified by {consumer_uuid}. If allocations already exist for this consumer, they are replaced.
Normal Response Codes: 204
Error response codes: badRequest(400), itemNotFound(404), conflict(409)
Usage: osc placement allocation set10 [OPTIONS] <CONSUMER_UUID>
Arguments:
<CONSUMER_UUID>
— consumer_uuid parameter for /allocations/{consumer_uuid} API
Options:
--allocations <JSON>
— Parameter is an array, may be provided multiple times
osc placement allocation show
List all allocation records for the consumer identified by {consumer_uuid} on all the resource providers it is consuming.
Normal Response Codes: 200
Usage: osc placement allocation show <CONSUMER_UUID>
Arguments:
<CONSUMER_UUID>
— consumer_uuid parameter for /allocations/{consumer_uuid} API
osc placement allocation-candidate
Allocation candidates
Usage: osc placement allocation-candidate <COMMAND>
Available subcommands:
osc placement allocation-candidate list
— List allocation candidates
osc placement allocation-candidate list
Returns a dictionary representing a collection of allocation requests and resource provider summaries. Each allocation request has information to form a PUT /allocations/{consumer_uuid}
request to claim resources against a related set of resource providers. Additional parameters might be required, see Update allocations. As several allocation requests are available it’s necessary to select one. To make a decision, resource provider summaries are provided with the inventory/capacity information. For example, this information is used by nova-scheduler’s FilterScheduler to make decisions about on which compute host to build a server.
You can also find additional case studies of the request parameters in the Modeling with Provider Trees document.
Normal Response Codes: 200
Error response codes: badRequest(400)
Usage: osc placement allocation-candidate list [OPTIONS]
Options:
--group-policy <GROUP_POLICY>
— When more than one resourcesN query parameter is supplied, group_policy is required to indicate how the groups should interact. With group_policy=none, separate groupings - with or without a suffix - may or may not be satisfied by the same provider. With group_policy=isolate, suffixed groups are guaranteed to be satisfied by different providers - though there may still be overlap with the suffixless group--in-tree <IN_TREE>
— A string representing a resource provider uuid. When supplied, it will filter the returned allocation candidates to only those resource providers that are in the same tree with the given resource provider--limit <LIMIT>
— A positive integer used to limit the maximum number of allocation candidates returned in the response--member-of <MEMBER_OF>
— A string representing an aggregate uuid; or the prefix in: followed by a comma-separated list of strings representing aggregate uuids. The resource providers in the allocation request in the response must directly or via the root provider be associated with the aggregate or aggregates identified by uuid:member_of=5e08ea53-c4c6-448e-9334-ac4953de3cfa
,member_of=in:42896e0d-205d-4fe3-bd1e-100924931787,5e08ea53-c4c6-448e-9334-ac4953de3cfa
Starting from microversion 1.24 specifying multiple member_of query string parameters is possible. Multiple member_of parameters will result in filtering providers that are directly or via root provider associated with aggregates listed in all of the member_of query string values. For example, to get the providers that are associated with aggregate A as well as associated with any of aggregates B or C, the user could issue the following query:member_of=AGGA_UUID&member_of=in:AGGB_UUID,AGGC_UUID
Starting from microversion 1.32 specifying forbidden aggregates is supported in the member_of query string parameter. Forbidden aggregates are prefixed with a !. This negative expression can also be used in multiple member_of parameters:member_of=AGGA_UUID&member_of=!AGGB_UUID
would translate logically to “Candidate resource providers must be in AGGA and not in AGGB.” We do NOT support ! on the values within in:, but we support !in:. Both of the following two example queries return candidate resource providers that are NOT in AGGA, AGGB, or AGGC:member_of=!in:AGGA_UUID,AGGB_UUID,AGGC_UUID
,member_of=!AGGA_UUID&member_of=!AGGB_UUID&member_of=!AGGC_UUID
We do not check if the same aggregate uuid is in both positive and negative expression to return 400 BadRequest. We still return 200 for such cases. For example:member_of=AGGA_UUID&member_of=!AGGA_UUID
would return empty allocation_requests and provider_summaries, while:member_of=in:AGGA_UUID,AGGB_UUID&member_of=!AGGA_UUID
would return resource providers that are NOT in AGGA but in AGGB--required <REQUIRED>
— A comma-separated list of traits that a provider must have:required=HW_CPU_X86_AVX,HW_CPU_X86_SSE
Allocation requests in the response will be for resource providers that have capacity for all requested resources and the set of those resource providers will collectively contain all of the required traits. These traits may be satisfied by any provider in the same non-sharing tree or associated via aggregate as far as that provider also contributes resource to the request. Starting from microversion 1.22 traits which are forbidden from any resource provider contributing resources to the request may be expressed by prefixing a trait with a!
. Starting from microversion 1.39 the required query parameter can be repeated. The trait lists from the repeated parameters are AND-ed together. So:required=T1,!T2&required=T3
means T1 and not T2 and T3. Also starting from microversion 1.39 the required parameter supports the syntax:required=in:T1,T2,T3
which means T1 or T2 or T3. Mixing forbidden traits into an in: prefixed value is not supported and rejected. But mixing a normal trait list and an in: prefixed trait list in two query params within the same request is supported. So:required=in:T3,T4&required=T1,!T2
is supported and it means T1 and not T2 and (T3 or T4)--resources <RESOURCES>
— A comma-separated list of strings indicating an amount of resource of a specified class that providers in each allocation request must collectively have the capacity and availability to serve:resources=VCPU:4,DISK_GB:64,MEMORY_MB:2048
These resources may be satisfied by any provider in the same non-sharing tree or associated via aggregate--root-required <ROOT_REQUIRED>
— A comma-separated list of trait requirements that the root provider of the (non-sharing) tree must satisfy:root_required=COMPUTE_SUPPORTS_MULTI_ATTACH,!CUSTOM_WINDOWS_LICENSED
Allocation requests in the response will be limited to those whose (non-sharing) tree’s root provider satisfies the specified trait requirements. Traits which are forbidden (must not be present on the root provider) are expressed by prefixing the trait with a !--same-subtree <SAME_SUBTREE>
— A comma-separated list of request group suffix strings ($S). Each must exactly match a suffix on a granular group somewhere else in the request. Importantly, the identified request groups need not have a resources[$S]. If this is provided, at least one of the resource providers satisfying a specified request group must be an ancestor of the rest. The same_subtree query parameter can be repeated and each repeat group is treated independently
osc placement reshaper
Reshaper
Usage: osc placement reshaper <COMMAND>
Available subcommands:
osc placement reshaper create138
— Reshaper (microversion = 1.38)osc placement reshaper create134
— Reshaper (microversion = 1.34)
osc placement reshaper create138
Atomically migrate resource provider inventories and associated allocations. This is used when some of the inventory needs to move from one resource provider to another, such as when a class of inventory moves from a parent provider to a new child provider.
Normal Response Codes: 204
Error Response Codes: badRequest(400), conflict(409)
Usage: osc placement reshaper create138 [OPTIONS]
Options:
--allocations <key=value>
— A dictionary of multiple allocations, keyed by consumer uuid. Each collection of allocations describes the full set of allocations for each consumer. Each consumer allocations dict is itself a dictionary of resource allocations keyed by resource provider uuid. An empty dictionary indicates no change in existing allocations, whereas an emptyallocations
dictionary within a consumer dictionary indicates that all allocations for that consumer should be deleted--inventories <key=value>
— A dictionary of multiple inventories, keyed by resource provider uuid. Each inventory describes the desired full inventory for each resource provider. An empty dictionary causes the inventory for that provider to be deleted
osc placement reshaper create134
Atomically migrate resource provider inventories and associated allocations. This is used when some of the inventory needs to move from one resource provider to another, such as when a class of inventory moves from a parent provider to a new child provider.
Normal Response Codes: 204
Error Response Codes: badRequest(400), conflict(409)
Usage: osc placement reshaper create134 [OPTIONS]
Options:
--allocations <key=value>
— A dictionary of multiple allocations, keyed by consumer uuid. Each collection of allocations describes the full set of allocations for each consumer. Each consumer allocations dict is itself a dictionary of resource allocations keyed by resource provider uuid. An empty dictionary indicates no change in existing allocations, whereas an emptyallocations
dictionary within a consumer dictionary indicates that all allocations for that consumer should be deleted--inventories <key=value>
— A dictionary of multiple inventories, keyed by resource provider uuid. Each inventory describes the desired full inventory for each resource provider. An empty dictionary causes the inventory for that provider to be deleted
osc placement resource-class
Resource Class
Resource classes are entities that indicate standard or deployer-specific resources that can be provided by a resource provider. This group of API calls works with a single resource class identified by name. One resource class can be listed, updated and deleted.
Usage: osc placement resource-class <COMMAND>
Available subcommands:
osc placement resource-class create
— Create resource classosc placement resource-class delete
— Delete resource classosc placement resource-class list
— List resource classesosc placement resource-class set17
— Update resource class (microversion = 1.7)osc placement resource-class show
— Show resource class
osc placement resource-class create
Create a new resource class. The new class must be a custom resource class, prefixed with CUSTOM_ and distinct from the standard resource classes.
Normal Response Codes: 201
Error response codes: badRequest(400), conflict(409)
A 400 BadRequest response code will be returned if the resource class does not have prefix CUSTOM_.
A 409 Conflict response code will be returned if another resource class exists with the provided name.
Usage: osc placement resource-class create --name <NAME>
Options:
--name <NAME>
— The name of one resource class
osc placement resource-class delete
Delete the resource class identified by {name}.
Normal Response Codes: 204
Error response codes: badRequest(400), itemNotFound(404), conflict(409)
A 400 BadRequest response code will be returned if trying to delete a standard resource class.
A 409 Conflict response code will be returned if there exist inventories for the resource class.
Usage: osc placement resource-class delete <NAME>
Arguments:
<NAME>
— name parameter for /resource_classes/{name} API
osc placement resource-class list
Return a list of all resource classes.
Normal Response Codes: 200
Usage: osc placement resource-class list
osc placement resource-class set17
Create or validate the existence of single resource class identified by {name}.
Normal Response Codes: 201, 204
A 201 Created response code will be returned if the new resource class is successfully created. A 204 No Content response code will be returned if the resource class already exists.
Error response codes: badRequest(400)
Usage: osc placement resource-class set17 <NAME>
Arguments:
<NAME>
— name parameter for /resource_classes/{name} API
osc placement resource-class show
Return a representation of the resource class identified by {name}.
Normal Response Codes: 200
Error response codes: itemNotFound(404)
Usage: osc placement resource-class show <NAME>
Arguments:
<NAME>
— name parameter for /resource_classes/{name} API
osc placement resource-provider
Resource Providers
Resource providers are entities which provide consumable inventory of one or more classes of resource (such as disk or memory). They can be listed (with filters), created, updated and deleted.
Usage: osc placement resource-provider <COMMAND>
Available subcommands:
osc placement resource-provider aggregate
— Resource provider aggregatesosc placement resource-provider allocation
— Resource provider allocationsosc placement resource-provider create114
— Create resource provider (microversion = 1.14)osc placement resource-provider create10
— Create resource provider (microversion = 1.0)osc placement resource-provider delete
— Delete resource providerosc placement resource-provider inventory
— Resource provider inventoriesosc placement resource-provider list
— List resource providersosc placement resource-provider set114
— Update resource provider (microversion = 1.14)osc placement resource-provider set10
— Update resource provider (microversion = 1.0)osc placement resource-provider show
— Show resource providerosc placement resource-provider trait
— Resource provider traitsosc placement resource-provider usage
— Resource provider usages
osc placement resource-provider aggregate
Resource provider aggregates
Each resource provider can be associated with one or more other resource providers in groups called aggregates. API calls in this section are used to list and update the aggregates that are associated with one resource provider.
Provider aggregates are used for modeling relationships among providers. Examples may include:
-
A shared storage pool providing DISK_GB resources to compute node providers that provide VCPU and MEMORY_MB resources.
-
Affinity/anti-affinity relationships such as physical location, power failure domains, or other reliability/availability constructs.
-
Groupings of compute host providers corresponding to Nova host aggregates or availability zones.
Note: Placement aggregates are not the same as Nova host aggregates and should not be considered equivalent.
The primary differences between Nova’s host aggregates and placement aggregates are the following:
-
In Nova, a host aggregate associates a nova-compute service with other nova-compute services. Placement aggregates are not specific to a nova-compute service and are, in fact, not compute-specific at all. A resource provider in the Placement API is generic, and placement aggregates are simply groups of generic resource providers. This is an important difference especially for Ironic, which when used with Nova, has many Ironic baremetal nodes attached to a single nova-compute service. In the Placement API, each Ironic baremetal node is its own resource provider and can therefore be associated to other Ironic baremetal nodes via a placement aggregate association.
-
In Nova, a host aggregate may have metadata key/value pairs attached to it. All nova-compute services associated with a Nova host aggregate share the same metadata. Placement aggregates have no such metadata because placement aggregates only represent the grouping of resource providers. In the Placement API, resource providers are individually decorated with traits that provide qualitative information about the resource provider.
-
In Nova, a host aggregate dictates the availability zone within which one or more nova-compute services reside. While placement aggregates may be used to model availability zones, they have no inherent concept thereof.
Usage: osc placement resource-provider aggregate <COMMAND>
Available subcommands:
osc placement resource-provider aggregate list
— List resource provider aggregatesosc placement resource-provider aggregate set119
— Update resource provider aggregates (microversion = 1.19)osc placement resource-provider aggregate set11
— Update resource provider aggregates (microversion = 1.1)
osc placement resource-provider aggregate list
Return a list of aggregates associated with the resource provider identified by {uuid}.
Normal Response Codes: 200
Error response codes: itemNotFound(404) if the provider does not exist. (If the provider has no aggregates, the result is 200 with an empty aggregate list.)
Usage: osc placement resource-provider aggregate list <UUID>
Arguments:
<UUID>
— uuid parameter for /resource_providers/{uuid}/aggregates API
osc placement resource-provider aggregate set119
Associate a list of aggregates with the resource provider identified by {uuid}.
Normal Response Codes: 200
Error response codes: badRequest(400), itemNotFound(404), conflict(409)
Usage: osc placement resource-provider aggregate set119 [OPTIONS] --resource-provider-generation <RESOURCE_PROVIDER_GENERATION> <UUID>
Arguments:
<UUID>
— uuid parameter for /resource_providers/{uuid}/aggregates API
Options:
--aggregates <AGGREGATES>
— Parameter is an array, may be provided multiple times--resource-provider-generation <RESOURCE_PROVIDER_GENERATION>
osc placement resource-provider aggregate set11
Associate a list of aggregates with the resource provider identified by {uuid}.
Normal Response Codes: 200
Error response codes: badRequest(400), itemNotFound(404), conflict(409)
Usage: osc placement resource-provider aggregate set11 <UUID>
Arguments:
<UUID>
— uuid parameter for /resource_providers/{uuid}/aggregates API
osc placement resource-provider allocation
Resource provider allocations
Usage: osc placement resource-provider allocation <COMMAND>
Available subcommands:
osc placement resource-provider allocation list
— List resource provider allocations
osc placement resource-provider allocation list
Return a representation of all allocations made against this resource provider, keyed by consumer uuid. Each allocation includes one or more classes of resource and the amount consumed.
Normal Response Codes: 200
Error response codes: itemNotFound(404)
Usage: osc placement resource-provider allocation list <UUID>
Arguments:
<UUID>
— uuid parameter for /resource_providers/{uuid}/allocations API
osc placement resource-provider create114
Create a new resource provider.
Normal Response Codes: 201 (microversions 1.0 - 1.19), 200 (microversions 1.20 - )
Error response codes: conflict(409)
A 409 Conflict response code will be returned if another resource provider exists with the provided name or uuid.
Usage: osc placement resource-provider create114 [OPTIONS] --name <NAME>
Options:
-
--name <NAME>
— The name of one resource provider -
--parent-provider-uuid <PARENT_PROVIDER_UUID>
— The UUID of the immediate parent of the resource provider.- Before version
1.37
, once set, the parent of a resource provider cannot be changed. - Since version1.37
, it can be set to any existing provider UUID excepts to providers that would cause a loop. Also it can be set to null to transform the provider to a new root provider. This operation needs to be used carefully. Moving providers can mean that the original rules used to create the existing resource allocations may be invalidated by that move.
New in version 1.14
- Before version
-
--uuid <UUID>
— The uuid of a resource provider
osc placement resource-provider create10
Create a new resource provider.
Normal Response Codes: 201 (microversions 1.0 - 1.19), 200 (microversions 1.20 - )
Error response codes: conflict(409)
A 409 Conflict response code will be returned if another resource provider exists with the provided name or uuid.
Usage: osc placement resource-provider create10 [OPTIONS] --name <NAME>
Options:
--name <NAME>
— The name of one resource provider--uuid <UUID>
— The uuid of a resource provider
osc placement resource-provider delete
Delete the resource provider identified by {uuid}. This will also disassociate aggregates and delete inventories.
Normal Response Codes: 204
Error response codes: itemNotFound(404), conflict(409)
A 409 Conflict response code will be returned if there exist allocations records for any of the inventories that would be deleted as a result of removing the resource provider.
This error code will be also returned if there are existing child resource providers under the parent resource provider being deleted.
Usage: osc placement resource-provider delete <UUID>
Arguments:
<UUID>
— uuid parameter for /resource_providers/{uuid} API
osc placement resource-provider inventory
Resource provider inventories
Each resource provider has inventory records for one or more classes of resources. An inventory record contains information about the total and reserved amounts of the resource and any consumption constraints for that resource against the provider.
Usage: osc placement resource-provider inventory <COMMAND>
Available subcommands:
osc placement resource-provider inventory create
— POST to create one inventoryosc placement resource-provider inventory delete
— Delete resource provider inventoryosc placement resource-provider inventory purge
— Delete resource provider inventoriesosc placement resource-provider inventory list
— List resource provider inventoriesosc placement resource-provider inventory replace
— Update resource provider inventoriesosc placement resource-provider inventory set
— Update resource provider inventoryosc placement resource-provider inventory show
— Show resource provider inventory
osc placement resource-provider inventory create
POST to create one inventory.
On success return a 201 response, a location header pointing to the newly created inventory and an application/json representation of the inventory.
Usage: osc placement resource-provider inventory create [OPTIONS] --resource-provider-generation <RESOURCE_PROVIDER_GENERATION> <UUID>
Arguments:
<UUID>
— uuid parameter for /resource_providers/{uuid}/inventories/{resource_class} API
Options:
--inventories <key=value>
— A dictionary of inventories keyed by resource classes--resource-provider-generation <RESOURCE_PROVIDER_GENERATION>
— A consistent view marker that assists with the management of concurrent resource provider updates
osc placement resource-provider inventory delete
Delete the inventory record of the {resource_class} for the resource provider identified by {uuid}.
See Troubleshooting section in Delete resource provider inventories
for a description. In addition, the request returns HTTP 409 when there are allocations for the specified resource provider and resource class.
Normal Response Codes: 204
Error response codes: itemNotFound(404), conflict(409)
Usage: osc placement resource-provider inventory delete <UUID> <RESOURCE_CLASS>
Arguments:
<UUID>
— uuid parameter for /resource_providers/{uuid}/inventories/{resource_class} API<RESOURCE_CLASS>
— resource_class parameter for /resource_providers/{uuid}/inventories/{resource_class} API
osc placement resource-provider inventory purge
Deletes all inventory records for the resource provider identified by {uuid}.
Troubleshooting
The request returns an HTTP 409 when there are allocations against the provider or if the provider’s inventory is updated by another thread while attempting the operation.
Normal Response Codes: 204
Error response codes: itemNotFound(404), conflict(409)
Usage: osc placement resource-provider inventory purge <UUID>
Arguments:
<UUID>
— uuid parameter for /resource_providers/{uuid}/inventories/{resource_class} API
osc placement resource-provider inventory list
Normal Response Codes: 200
Error response codes: itemNotFound(404)
Usage: osc placement resource-provider inventory list <UUID>
Arguments:
<UUID>
— uuid parameter for /resource_providers/{uuid}/inventories/{resource_class} API
osc placement resource-provider inventory replace
Replaces the set of inventory records for the resource provider identified by {uuid}.
Normal Response Codes: 200
Error response codes: badRequest(400), itemNotFound(404), conflict(409)
Usage: osc placement resource-provider inventory replace [OPTIONS] --resource-provider-generation <RESOURCE_PROVIDER_GENERATION> <UUID>
Arguments:
<UUID>
— uuid parameter for /resource_providers/{uuid}/inventories/{resource_class} API
Options:
--inventories <key=value>
— A dictionary of inventories keyed by resource classes--resource-provider-generation <RESOURCE_PROVIDER_GENERATION>
— A consistent view marker that assists with the management of concurrent resource provider updates
osc placement resource-provider inventory set
Replace the inventory record of the {resource_class} for the resource provider identified by {uuid}.
Normal Response Codes: 200
Error response codes: badRequest(400), itemNotFound(404), conflict(409)
Usage: osc placement resource-provider inventory set [OPTIONS] --resource-provider-generation <RESOURCE_PROVIDER_GENERATION> --total <TOTAL> <UUID> <RESOURCE_CLASS>
Arguments:
<UUID>
— uuid parameter for /resource_providers/{uuid}/inventories/{resource_class} API<RESOURCE_CLASS>
— resource_class parameter for /resource_providers/{uuid}/inventories/{resource_class} API
Options:
-
--allocation-ratio <ALLOCATION_RATIO>
— It is used in determining whether consumption of the resource of the provider can exceed physical constraints.For example, for a vCPU resource with:
Overall capacity is equal to 128 vCPUs.
-
--max-unit <MAX_UNIT>
— A maximum amount any single allocation against an inventory can have -
--min-unit <MIN_UNIT>
— A minimum amount any single allocation against an inventory can have -
--reserved <RESERVED>
— The amount of the resource a provider has reserved for its own use -
--resource-provider-generation <RESOURCE_PROVIDER_GENERATION>
— A consistent view marker that assists with the management of concurrent resource provider updates -
--step-size <STEP_SIZE>
— A representation of the divisible amount of the resource that may be requested. For example, step_size = 5 means that only values divisible by 5 (5, 10, 15, etc.) can be requested -
--total <TOTAL>
— The actual amount of the resource that the provider can accommodate
osc placement resource-provider inventory show
Normal Response Codes: 200
Error response codes: itemNotFound(404)
Usage: osc placement resource-provider inventory show <UUID> <RESOURCE_CLASS>
Arguments:
<UUID>
— uuid parameter for /resource_providers/{uuid}/inventories/{resource_class} API<RESOURCE_CLASS>
— resource_class parameter for /resource_providers/{uuid}/inventories/{resource_class} API
osc placement resource-provider list
List an optionally filtered collection of resource providers.
Normal Response Codes: 200
Error response codes: badRequest(400)
A 400 BadRequest response code will be returned if a resource class specified in resources
request parameter does not exist.
Usage: osc placement resource-provider list [OPTIONS]
Options:
--in-tree <IN_TREE>
— A string representing a resource provider uuid. When supplied, it will filter the returned allocation candidates to only those resource providers that are in the same tree with the given resource provider--member-of <MEMBER_OF>
— A string representing an aggregate uuid; or the prefix in: followed by a comma-separated list of strings representing aggregate uuids. The resource providers in the allocation request in the response must directly or via the root provider be associated with the aggregate or aggregates identified by uuid:member_of=5e08ea53-c4c6-448e-9334-ac4953de3cfa
,member_of=in:42896e0d-205d-4fe3-bd1e-100924931787,5e08ea53-c4c6-448e-9334-ac4953de3cfa
Starting from microversion 1.24 specifying multiple member_of query string parameters is possible. Multiple member_of parameters will result in filtering providers that are directly or via root provider associated with aggregates listed in all of the member_of query string values. For example, to get the providers that are associated with aggregate A as well as associated with any of aggregates B or C, the user could issue the following query:member_of=AGGA_UUID&member_of=in:AGGB_UUID,AGGC_UUID
Starting from microversion 1.32 specifying forbidden aggregates is supported in the member_of query string parameter. Forbidden aggregates are prefixed with a !. This negative expression can also be used in multiple member_of parameters:member_of=AGGA_UUID&member_of=!AGGB_UUID
would translate logically to “Candidate resource providers must be in AGGA and not in AGGB.” We do NOT support ! on the values within in:, but we support !in:. Both of the following two example queries return candidate resource providers that are NOT in AGGA, AGGB, or AGGC:member_of=!in:AGGA_UUID,AGGB_UUID,AGGC_UUID
,member_of=!AGGA_UUID&member_of=!AGGB_UUID&member_of=!AGGC_UUID
We do not check if the same aggregate uuid is in both positive and negative expression to return 400 BadRequest. We still return 200 for such cases. For example:member_of=AGGA_UUID&member_of=!AGGA_UUID
would return empty allocation_requests and provider_summaries, while:member_of=in:AGGA_UUID,AGGB_UUID&member_of=!AGGA_UUID
would return resource providers that are NOT in AGGA but in AGGB--name <NAME>
— The name of a resource provider to filter the list--required <REQUIRED>
— A comma-separated list of traits that a provider must have:required=HW_CPU_X86_AVX,HW_CPU_X86_SSE
Allocation requests in the response will be for resource providers that have capacity for all requested resources and the set of those resource providers will collectively contain all of the required traits. These traits may be satisfied by any provider in the same non-sharing tree or associated via aggregate as far as that provider also contributes resource to the request. Starting from microversion 1.22 traits which are forbidden from any resource provider contributing resources to the request may be expressed by prefixing a trait with a!
. Starting from microversion 1.39 the required query parameter can be repeated. The trait lists from the repeated parameters are AND-ed together. So:required=T1,!T2&required=T3
means T1 and not T2 and T3. Also starting from microversion 1.39 the required parameter supports the syntax:required=in:T1,T2,T3
which means T1 or T2 or T3. Mixing forbidden traits into an in: prefixed value is not supported and rejected. But mixing a normal trait list and an in: prefixed trait list in two query params within the same request is supported. So:required=in:T3,T4&required=T1,!T2
is supported and it means T1 and not T2 and (T3 or T4)--resources <RESOURCES>
— A comma-separated list of strings indicating an amount of resource of a specified class that providers in each allocation request must collectively have the capacity and availability to serve:resources=VCPU:4,DISK_GB:64,MEMORY_MB:2048
These resources may be satisfied by any provider in the same non-sharing tree or associated via aggregate--uuid <UUID>
— The uuid of a resource provider
osc placement resource-provider set114
Update the name of the resource provider identified by {uuid}.
Normal Response Codes: 200
Error response codes: badRequest(400), itemNotFound(404), conflict(409)
A 409 Conflict response code will be returned if another resource provider exists with the provided name.
Usage: osc placement resource-provider set114 [OPTIONS] --name <NAME> <UUID>
Arguments:
<UUID>
— uuid parameter for /resource_providers/{uuid} API
Options:
-
--name <NAME>
— The name of one resource provider -
--parent-provider-uuid <PARENT_PROVIDER_UUID>
— The UUID of the immediate parent of the resource provider.- Before version
1.37
, once set, the parent of a resource provider cannot be changed. - Since version1.37
, it can be set to any existing provider UUID excepts to providers that would cause a loop. Also it can be set to null to transform the provider to a new root provider. This operation needs to be used carefully. Moving providers can mean that the original rules used to create the existing resource allocations may be invalidated by that move.
New in version 1.14
- Before version
osc placement resource-provider set10
Update the name of the resource provider identified by {uuid}.
Normal Response Codes: 200
Error response codes: badRequest(400), itemNotFound(404), conflict(409)
A 409 Conflict response code will be returned if another resource provider exists with the provided name.
Usage: osc placement resource-provider set10 --name <NAME> <UUID>
Arguments:
<UUID>
— uuid parameter for /resource_providers/{uuid} API
Options:
--name <NAME>
— The name of one resource provider
osc placement resource-provider show
Return a representation of the resource provider identified by {uuid}.
Normal Response Codes: 200
Error response codes: itemNotFound(404)
Usage: osc placement resource-provider show <UUID>
Arguments:
<UUID>
— uuid parameter for /resource_providers/{uuid} API
osc placement resource-provider trait
Resource provider traits
This group of API requests queries/edits the association between traits and resource providers.
Usage: osc placement resource-provider trait <COMMAND>
Available subcommands:
osc placement resource-provider trait delete
— Delete resource provider traitsosc placement resource-provider trait list
— List resource provider traitsosc placement resource-provider trait set
— Update resource provider traits
osc placement resource-provider trait delete
Dissociate all the traits from the resource provider identified by {uuid}.
Normal Response Codes: 204
Error response codes: itemNotFound(404), conflict(409)
Usage: osc placement resource-provider trait delete <UUID>
Arguments:
<UUID>
— uuid parameter for /resource_providers/{uuid}/traits API
osc placement resource-provider trait list
Return a list of traits for the resource provider identified by {uuid}.
Normal Response Codes: 200
Error response codes: itemNotFound(404)
Usage: osc placement resource-provider trait list <UUID>
Arguments:
<UUID>
— uuid parameter for /resource_providers/{uuid}/traits API
osc placement resource-provider trait set
Associate traits with the resource provider identified by {uuid}. All the associated traits will be replaced by the traits specified in the request body.
Normal Response Codes: 200
Error response codes: badRequest(400), itemNotFound(404), conflict(409)
Usage: osc placement resource-provider trait set [OPTIONS] <UUID>
Arguments:
<UUID>
— uuid parameter for /resource_providers/{uuid}/traits API
Options:
-
--traits <TRAITS>
— A list of traits.Parameter is an array, may be provided multiple times.
osc placement resource-provider usage
Resource provider usages
Show the consumption of resources for a resource provider in an aggregated form, i.e. without information for a particular consumer.
Usage: osc placement resource-provider usage <COMMAND>
Available subcommands:
osc placement resource-provider usage get
— List resource provider usages
osc placement resource-provider usage get
Return a report of usage information for resources associated with the resource provider identified by {uuid}. The value is a dictionary of resource classes paired with the sum of the allocations of that resource class for this resource provider.
Normal Response Codes: 200
Error response codes: itemNotFound(404)
Usage: osc placement resource-provider usage get <UUID>
Arguments:
<UUID>
— uuid parameter for /resource_providers/{uuid}/usages API
osc placement trait
Traits
Traits are qualitative characteristics of resource providers. The classic example for traits can be requesting disk from different providers: a user may request 80GiB of disk space for an instance (quantitative), but may also expect that the disk be SSD instead of spinning disk (qualitative). Traits provide a way to mark that a storage provider is SSD or spinning.
Usage: osc placement trait <COMMAND>
Available subcommands:
osc placement trait create
— Update traitsosc placement trait delete
— Delete traitsosc placement trait list
— List traitsosc placement trait show
— Show traits
osc placement trait create
Insert a new custom trait. If traits already exists 204 will be returned.
There are two kinds of traits: the standard traits and the custom traits. The standard traits are interoperable across different OpenStack cloud deployments. The definition of standard traits comes from the os-traits library. The standard traits are read-only in the placement API which means that the user can’t modify any standard traits through API. The custom traits are used by admin users to manage the non-standard qualitative information of resource providers.
Normal Response Codes: 201, 204
Error response codes: badRequest(400)
Usage: osc placement trait create <NAME>
Arguments:
<NAME>
— name parameter for /traits/{name} API
osc placement trait delete
Delete the trait specified be {name}. Note that only custom traits can be deleted.
Normal Response Codes: 204
Error response codes: badRequest(400), itemNotFound(404), conflict(409)
Usage: osc placement trait delete <NAME>
Arguments:
<NAME>
— name parameter for /traits/{name} API
osc placement trait list
Return a list of valid trait strings according to parameters specified.
Normal Response Codes: 200
Usage: osc placement trait list [OPTIONS]
Options:
--associated
— If this parameter has a true value, the returned traits will be those that are associated with at least one resource provider. Available values for the parameter are true and false--name <NAME>
— The name of a resource provider to filter the list
osc placement trait show
Check if a trait name exists in this cloud.
Normal Response Codes: 204
Error response codes: itemNotFound(404)
Usage: osc placement trait show <NAME>
Arguments:
<NAME>
— name parameter for /traits/{name} API
osc placement usage
Usages
Represent the consumption of resources for a project and user.
Usage: osc placement usage <COMMAND>
Available subcommands:
osc placement usage list
— List usages
osc placement usage list
Return a report of usage information for resources associated with the project identified by project_id and user identified by user_id. The value is a dictionary of resource classes paired with the sum of the allocations of that resource class for provided parameters.
Normal Response Codes: 200
Error response codes: badRequest(400)
Usage: osc placement usage list [OPTIONS] <--project-name <PROJECT_NAME>|--project-id <PROJECT_ID>|--current-project>
Options:
--consumer-type <CONSUMER_TYPE>
— A string that consists of numbers, A-Z, and _ describing the consumer type by which to filter usage results. For example, to retrieve only usage information for ‘INSTANCE’ type consumers a parameter of consumer_type=INSTANCE should be provided. The all query parameter may be specified to group all results under one key, all. The unknown query parameter may be specified to group all results under one key, unknown--project-name <PROJECT_NAME>
— Project Name--project-id <PROJECT_ID>
— Project ID--current-project
— Current project--user-name <USER_NAME>
— User Name--user-id <USER_ID>
— User ID--current-user
— Current authenticated user
osc completion
Output shell completion code for the specified shell (bash, zsh, fish, or powershell). The shell code must be evaluated to provide interactive completion of osc
commands. This can be done by sourcing it from the .bash_profile.
Examples:
Enable completion at a shell start:
echo 'source <(osc completion bash)' >>~/.bashrc
Usage: osc completion [SHELL]
Arguments:
-
<SHELL>
— If provided, outputs the completion file for given shellDefault value:
bash
Possible values:
bash
,elvish
,fish
,powershell
,zsh
CLI configuration
It is possible to configure different aspects of the OpenStackClient (not the
clouds connection credentials) using the configuration file
($XDG_CONFIG_DIR/osc/config.yaml
). This enables user to configurate which
columns should be returned when no corresponding run time arguments on a
resource base.
views:
compute.server:
# Listing compute servers will only return ID, NAME and IMAGE columns unless `-o wide` or
`-f XXX` parameters are being passed
fields: [id, name, image]
dns.zone/recordset:
# DNS zone recordsets are listed in the wide mode by default.
wide: true
The key of the views
map is a resource key shared among all
openstack_rust
tools and is built in the following form:
<SERVICE-TYPE>.<RESOURCE_NAME>[/<SUBRESOURCE_NAME>]
where
<RESOURCE_NAME>[/<SUBRESOURCE_NAME>
is a url based naming (for designate
/zone/<ID>/recordset/<RS_ID>
would be names as zone.recordset and
/volumes/{volume_id}/metadata
would become volume.metadata. Please consult
Codegenerator
metadata
for known resource keys.
Text (Terminal) User Interface
Live navigating through OpenStack resources using CLI can be very cumbersome.
This is where ostui
(a terminal user interface for OpenStack) rushes to help.
At the moment it is in a very early prototyping state but already saved my day
multiple times.
It is not trivial to implement UX with modification capabilities for OpenStack,
especially in the terminal this is even harder. Therefore primary goal of
ostui
is to provide a fast navigation through existing resources eventually
with some operations on those.
Support for new resources is being permanently worked on (feel free to open issue asking for the desired resource/action or contribute with the implementation). For the moment documentation and implementation are not directly in sync therefore much more resources may be implemented than included in documentation.
Features
- switching clouds within tui session
- switching project scope without exploding
clouds.yaml
- resource filtering
- navigation through dependent resources
- k9s similar interface with (hopefully) intuitive navigation
Installation
Compiled binaries are always uploaded as release artifacts for every
openstack_tui_vX.Y.Z
tag. Binary for the desired platform can be downloaded
from GitHub directly or alternatively compiled locally when rust toolchain is
present.
Cloud connection
ostui
reads your clouds.yaml
file. When started with --os-cloud devstack
argument connection to the specified cloud is being immediately established.
Otherwise a popup is opened offering connection to clouds configured in the
regular clouds.yaml
file.
While staying in the TUI it is always possible to always switch the cloud by
pressing <F2>
Resource selection
Picking up a desired resource is done triggering the ResourceSelect
popup
which is by default invoked by pressing <:>
(like in vim or k9s). The popup
displays a list with fuzzy search where user is able to select the necessary
resource using one of the pre-configured aliases.
Header
In every mode TUI header show the context specific information. It is represented in few columns using different colors.
First column shows information about current connection
Second column shows selected global keybindings that are not context specific (a sort of magenta color).
Following columns are context specific and may be present or not.
Keybindings in blue color are used to filter results of the current view.
Red color is used by the keybindings as an action on the selected entry.
Note: colors are subjective and may be changed by the configuration file as well as altered by the terminal or display device.
Home
A Home
view serves an entry point for the cloud. It shows some service quotas
to have a better overview of currently available and provisioned resources.
Volumes
A Volumes
view lists all block storage volume accessible in the current
project. Few additional filters are available to further fine-tune results
list.
Volume Snapshots
A VolumeSnapshots
view lists all image snapshots in the current project. Few
additional filters are available to further fine-tune results list.
Volume Backups
A VolumeBackups
view lists all block storage volume backups in the current
project. Few additional filters are available to further fine-tune results
list.
Compute Servers
A Servers
view lists all servers in the current project. Few additional
filters are available (i.e. all_domains
).
Currently it is possible also to list all instance actions performed on the
selected entry (by pressing <a>
on the selected entry) as well as for the
selected instance actions list related events (by pressing <e>
).
Flavors
A Flavors
view lists all flavors accessible in the current project. Few
additional filters are available to further fine-tune results list.
It may be useful to list all VMs started with the selected flavor what is shown
after the user presses <s>
.
Compute Aggregates
A ComputeAggregates
view lists all aggregates configured in the cloud. Typically
this is only accessible for administrators.
Compute Hypervisors
A Hypervisors
view lists all hypervisors configured in the cloud. Typically this is only accessible for administrators.
DNS Zones
This view lists all DNS zones.
Pressing <r>
on the zone will switch to listing recorsets of the selected
zone.
DNS Recordsets
This view lists all DNS recordsets.
Identity Application Credentials
As a regular user ApplicationCredentials
view shows application credentials
of the current user. As an admin or a user with privilege it is also possible
to show application credentials of a specific user from the Users
view.
Identity Groups
By default all groups in the current domain are shown. When using admin connection this may differ, but in general results are equal to invocation of the CLI for the current connection.
Pressing <u>
on the group will switch to listing users member of the selected
group.
Identity Users
By default all users in the current domain are shown. When using admin connection this may differ, but in general results are equal to invocation of the CLI for the current connection.
The view gives possibility to enable/disable current selected user as well as list user application credentials. More possibilities are going to be added in the future (i.e. changing user password, deletion, e-mail update, etc)
Images
A Images
view lists all images accessible in the current project. Few
additional filters are available to further fine-tune results list.
Load Balancer
Load Balancer views allow traversing through the load-balancer
(Octavia)
resources.
Networks
A Network
view lists all networks in the current project. Pressing Enter
of
a selected entry by default switches to the Subnet
view with filter being set
to the currently selected network.
Subnetworks
A Subnet
view lists all subnets in the current project.
Network Security Groups
A SecurityGroups
view lists all security groups in the current project.
Pressing Enter
of a selected entry by default switches to the
SecurityGroupRules
view with filter being set to the currently selected
security group.
Network Security Group Rules
A SecurityGroupRules
view lists all security group rules in the current project.
Configuration
Certain aspects of the TUI can be configured using configuration file.
Config file can be in the yaml or json format and placed under
XDG_CONFIG_HOME/openstack_tui
named as config.yaml
or views.yaml
.
It is possible to split configuration parts into dediated files (i.e.
views.yaml
for configuring views). Files are merged in no particular order
(in difference to the clouds.yaml/secure.yaml
) so it is not possible to
predict the behavior when configuration option is being set in different files
with different value.
Default config
---
# Mode keybindings in the following form
# <Mode>:
# <shortcut>:
# action: <ACTION TO PERFORM>
# description: <DESCRIPTION USED IN TUI>
mode_keybindings:
Home: {}
# Block Storage views
BlockStorageBackups:
"y":
action: DescribeApiResponse
description: YAML
BlockStorageSnapshots:
"y":
action: DescribeApiResponse
description: YAML
BlockStorageVolumes:
"y":
action: DescribeApiResponse
description: YAML
"ctrl-d":
action: DeleteBlockStorageVolume
description: Delete
# Compute views
ComputeAggregates:
"y":
action: DescribeApiResponse
description: YAML
ComputeServers:
"y":
action: DescribeApiResponse
description: YAML
"0":
action:
SetComputeServerListFilters: {}
description: Default filters
type: Filter
"1":
action:
SetComputeServerListFilters: {"all_tenants": "true"}
description: All tenants (admin)
type: Filter
"ctrl-d":
action: DeleteComputeServer
description: Delete
"c":
action: ShowServerConsoleOutput
description: Console output
"a":
action: ShowComputeServerInstanceActions
description: Instance actions
ComputeServerInstanceActions:
"y":
action: DescribeApiResponse
description: YAML
"e":
action: ShowComputeServerInstanceActionEvents
description: Events
ComputeFlavors:
"y":
action: DescribeApiResponse
description: YAML
"s":
action: ShowComputeServersWithFlavor
description: Servers
ComputeHypervisors:
"y":
action: DescribeApiResponse
description: YAML
# DNS views
DnsRecordsets:
"y":
action: DescribeApiResponse
description: YAML
"0":
action:
SetDnsRecordsetListFilters: {}
description: Default filters
type: Filter
DnsZones:
"y":
action: DescribeApiResponse
description: YAML
"r":
action: ShowDnsZoneRecordsets
description: Recordsets
"ctrl-d":
action: DeleteDnsZone
description: Delete
# Identity views
IdentityApplicationCredentials:
"y":
action: DescribeApiResponse
description: YAML
IdentityGroups:
"y":
action: DescribeApiResponse
description: YAML
"u":
action: ShowIdentityGroupUsers
description: Group users
"d":
action: IdentityGroupDelete
description: Delete (todo!)
"a":
action: IdentityGroupCreate
description: Create new group (todo!)
IdentityGroupUsers:
"y":
action: DescribeApiResponse
description: YAML
"a":
action: IdentityGroupUserAdd
description: Add new user into group (todo!)
"r":
action: IdentityGroupUserRemove
description: Remove user from group (todo!)
IdentityProjects:
"y":
action: DescribeApiResponse
description: YAML
"s":
action: SwitchToProject
description: Switch to project
IdentityUsers:
"y":
action: DescribeApiResponse
description: YAML
"ctrl-d":
action: IdentityUserDelete
description: Delete
"e":
action: IdentityUserFlipEnable
description: Enable/Disable user
"a":
action: IdentityUserCreate
description: Create new user (todo!)
"p":
action: IdentityUserSetPassword
description: Set user password (todo!)
"c":
action: ShowIdentityUserApplicationCredentials
description: Application credentials
# Image views
ImageImages:
"y":
action: DescribeApiResponse
description: YAML
"0":
action:
SetImageListFilters: {}
description: Default filters
type: Filter
"1":
action:
SetImageListFilters: {"visibility": "public"}
description: public
type: Filter
"2":
action:
SetImageListFilters: {"visibility": "shared"}
description: shared
type: Filter
"3":
action:
SetImageListFilters: {"visibility": "private"}
description: private
type: Filter
"ctrl-d":
action: DeleteImage
description: Delete
# LoadBalancer views
LoadBalancers:
"y":
action: DescribeApiResponse
description: YAML
"l":
action: ShowLoadBalancerListeners
description: Listeners
"p":
action: ShowLoadBalancerPools
description: Pools
LoadBalancerListeners:
"y":
action: DescribeApiResponse
description: YAML
LoadBalancerPools:
"y":
action: DescribeApiResponse
description: YAML
"<enter>":
action: ShowLoadBalancerPoolMembers
description: Members
"h":
action: ShowLoadBalancerPoolHealthMonitors
description: HealthMonitors
LoadBalancerPoolMembers:
"y":
action: DescribeApiResponse
description: YAML
LoadBalancerHealthMonitors:
"y":
action: DescribeApiResponse
description: YAML
# Network views
NetworkNetworks:
"y":
action: DescribeApiResponse
description: YAML
"<enter>":
action: ShowNetworkSubnets
description: Subnets
NetworkRouters:
"y":
action: DescribeApiResponse
description: YAML
NetworkSubnets:
"y":
action: DescribeApiResponse
description: YAML
"0":
action:
SetNetworkSubnetListFilters: {}
description: All
type: Filter
NetworkSecurityGroups:
"y":
action: DescribeApiResponse
description: YAML
"l":
action: ShowNetworkSecurityGroupRules
description: Rules
NetworkSecurityGroupRules:
"y":
action: DescribeApiResponse
description: YAML
"0":
action:
SetNetworkSecurityGroupRuleListFilters: {}
description: All
type: Filter
# Global keybindings
# <KEYBINDING>:
# action: <ACTION>
# description: <TEXT>
global_keybindings:
"<q>":
action: Quit
description: Quit
"<Ctrl-c>":
action: Quit
description: Quit
"<Ctrl-z>":
action: Suspend
description: Suspend
"F1":
action:
Mode:
mode: Home
stack: false
description: Home
"F2":
action: CloudSelect
description: Select cloud
":":
action: ApiRequestSelect
description: Select resource
"<F4>":
action: SelectProject
description: Select project
"<ctrl-r>":
action: Refresh
description: Reload data
# Mode aliases
# <ALIAS>: <MODE>
mode_aliases:
"aggregates (compute)": "ComputeAggregates"
"application credentials (identity)": "IdentityApplicationCredentials"
"backups": "BlockStorageBackups"
"flavors": "ComputeFlavors"
"groups (identity)": "IdentityGroups"
"host-aggregates (compute)": "ComputeAggregates"
"hypervisors (compute)": "ComputeHypervisors"
"images": "ImageImages"
"loadbalancers": "LoadBalancers"
"lb (loadbalancers)": "LoadBalancers"
"listeners (loadbalancer)": "LoadBalancerListeners"
"lbl (loadbalancer listeners)": "LoadBalancerListeners"
"pool (loadbalancer)": "LoadBalancerPools"
"lbp (loadbalancer pools)": "LoadBalancerPools"
"healthmonitors (loadbalancer)": "LoadBalancerHealthMonitors"
"lbhm (loadbalancer health monitors)": "LoadBalancerHealthMonitors"
"nets": "NetworkNetworks"
"networks": "NetworkNetworks"
"projects": "IdentityProjects"
"recordsets (dns)": "DnsRecordsets"
"routers": "NetworkRouters"
"security groups (network)": "NetworkSecurityGroups"
"security group rules (network)": "NetworkSecurityGroupRules"
"servers": "ComputeServers"
"sg": "NetworkSecurityGroups"
"sgr": "NetworkSecurityGroupRules"
"snapshots": "BlockStorageSnapshots"
"subnets (network)": "NetworkSubnets"
"volumes": "BlockStorageVolumes"
"users": "IdentityUsers"
"zones (dns)": "DnsZones"
# View output
# <RESOURCE_KEY>:
# fields: <ARRAY OF COLUMNS TO SHOW>
# wide: true
views:
# Block Storage
block_storage.backup:
fields: [ID, NAME, AZ, SIZE, STATUS, CREATED_AT]
block_storage.snapshots:
fields: [ID, NAME, STATUS, CREATED_AT]
block_storage.volume:
fields: [ID, NAME, AZ, SIZE, STATUS, UPDATED_AT]
# Compute
compute.aggregate:
fields: [NAME, UUID, AZ, UPDATED_AT]
compute.flavor:
fields: [id, name, vcpus, ram, disk]
compute.hypervisor:
fields: [IP, HOSTNAME, STATUS, STATE]
compute.server/instance_action/event:
fields: [EVENT, RESULT, START_TIME, FINISH_TIME, HOST]
compute.server/instance_action:
fields: [ID, ACTION, MESSAGE, START_TIME, USER_ID]
compute.server:
fields: [ID, NAME, STATUS, CREATED, UPDATED]
# DNS
dns.recordset:
fields: [ID, NAME, STATUS, CREATED, UPDATED]
dns.zone:
fields: [ID, NAME, STATUS, CREATED, UPDATED]
# Identity
identity.group:
fields: [ID, NAME, DOMAIN, DESCRIPTION]
identity.project:
fields: [ID, NAME, "PARENT ID", ENABLED, "DOMAIN ID"]
identity.user/application_credential:
fields: [ID, NAME, "EXPIRES AT", "UNRESTRICTED"]
identity.user:
fields: [NAME, DOMAIN, ENABLED, EMAIL, "PWD EXPIRY"]
# Image
image.image:
fields: [ID, NAME, DISTRO, VERSION, ARCH, VISIBILITY]
# Load Balancer
load-balancer.healthmonitor:
fields: [ID, NAME, STATUS, TYPE]
load-balancer.listener:
fields: [ID, NAME, STATUS, PROTOCOL, PORT]
load-balancer.loadbalancer:
fields: [ID, NAME, STATUS, ADDRESS]
load-balancer.pool/member:
fields: [ID, NAME, STATUS, PORT]
load-balancer.pool:
fields: [ID, NAME, STATUS, PROTOCOL]
# Network
network.network:
fields: [ID, NAME, STATUS, CREATED_AT, UPDATED_AT]
network.router:
fields: [ID, NAME, STATUS, CREATED_AT, UPDATED_AT]
network.subnet:
fields: [ID, NAME, CIDR, DESCRIPTION, CREATED_AT]
network.security_group_rule:
fields: [ID, ETHERTYPE, DIRECTION, PROTOCOL, "RANGE MIN", "RANGE MAX"]
network.security_group:
fields: [ID, NAME, CREATED_AT, UPDATED_AT]
Views configuration
Every resource view can be configured in a separate section of the config.
Resource key in a form fields
is an array of field names to be
populated. All column names are forcibly converted to the UPPER CASE.